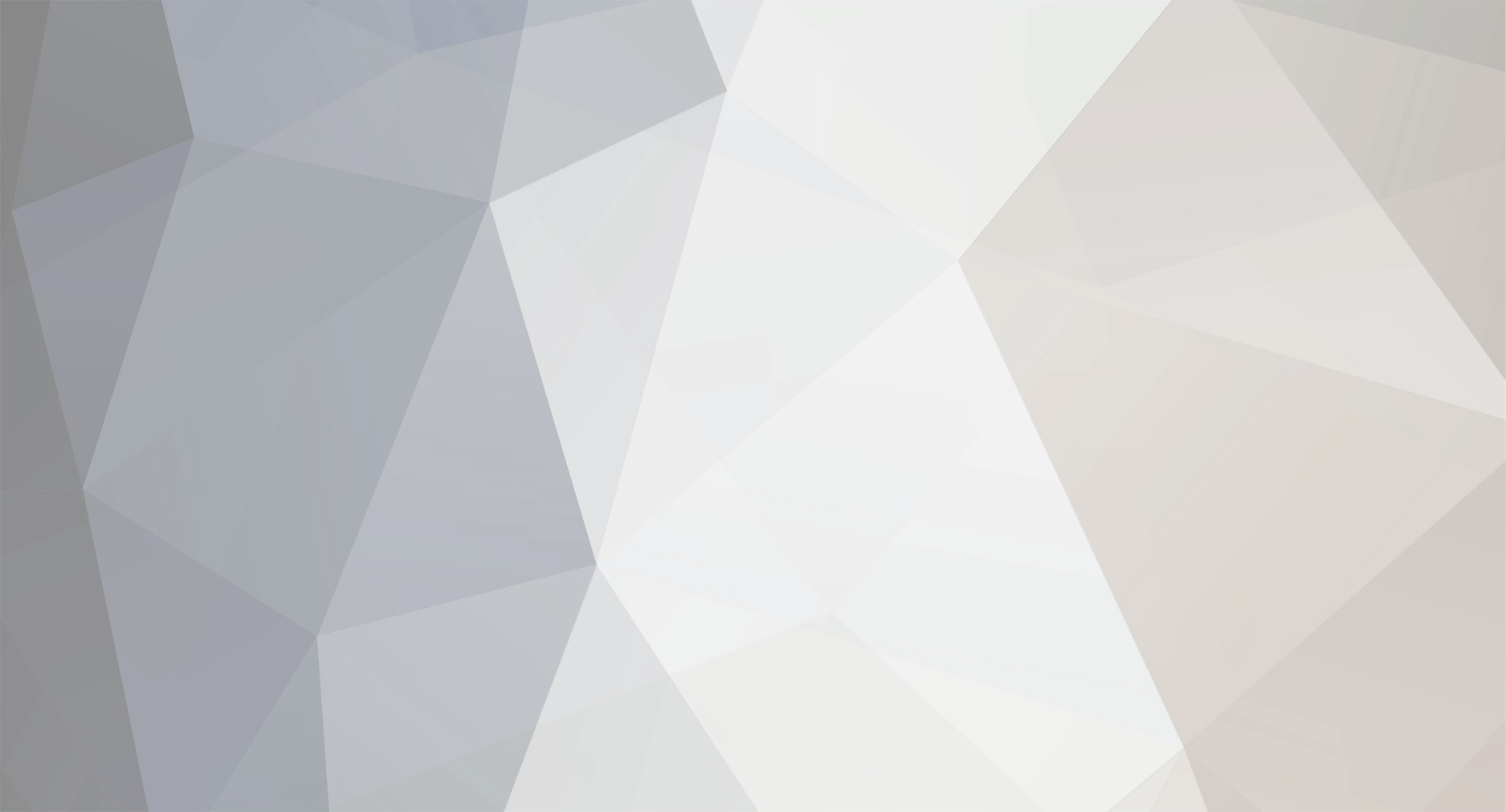
Groxkiller
Members-
Posts
51 -
Joined
-
Last visited
Everything posted by Groxkiller
-
Tile Entites or Metadata for Sided Textures
Groxkiller replied to Lycanite's topic in Modder Support
If you want to use one blockID for all furnaces cases then yes it's applicable to put the rotation and glowstone into the tile entity and have the metadata represent the furnace type. Remember, while a block only have one global instance, tile entities do not and thus can store any data you need on a per-block basis. As far as I know there is no drawbacks to this. -
[Solved] Particles are never semi-transparent
Groxkiller replied to Hunternif's topic in Modder Support
Ok, I haven't checked with this, but one thing you can try is see how Slimes render the outer block of thier model (ie how it's transparent) and try to transplant it over to your particles. It's probably a series of openGL calls to turn transparency on. -
Glad I could help!
-
I had this problem for a long time before I figured out the problem: public void openChest() { ++this.numUsingPlayers; this.worldObj.addBlockEvent(this.xCoord, this.yCoord, this.zCoord, Block.chest.blockID, 1, this.numUsingPlayers); } public void closeChest() { --this.numUsingPlayers; this.worldObj.addBlockEvent(this.xCoord, this.yCoord, this.zCoord, Block.chest.blockID, 1, this.numUsingPlayers); } This is from TileEntityChest. Notice something with the block events? it sends a default chest blockID - not your block's blockID. To fix it is simple: public void openChest() { ++this.numUsingPlayers; this.worldObj.addBlockEvent(this.xCoord, this.yCoord, this.zCoord, getBlockType().blockID, 1, this.numUsingPlayers); } public void closeChest() { --this.numUsingPlayers; this.worldObj.addBlockEvent(this.xCoord, this.yCoord, this.zCoord, getBlockType().blockID, 1, this.numUsingPlayers); } This should fix it's animations, if this for some odd reason crashes, then replace getBlockType().blockID with a call to your chest block's blockID. Tell me if it worked!
-
[Solved] Particles are never semi-transparent
Groxkiller replied to Hunternif's topic in Modder Support
use GL11.glColor4f(1.0F, 1.0F, 1.0F, alphaHere) just before rendering the particle, where 'alphaHere' is a float value between 0 and 1. (0 is fully transparent, 1 is opaque) -
ModelRenderer.addChild() is what your looking for: head.addChild(leftEar); head.addChild(rightEar); Please note, you will need to reoffset the ears because it offsets thier rotation points.
-
Block Rendering Hooks (NOT custom block hooks)
Groxkiller replied to Groxkiller's topic in Suggestions
Yes but it won't work for glowing entities who are currently occupying the space of a non-normal block. (such as a fence, tall grass, cobble wall, glass panes, doors, trapdoors, webs, etc. etc.) If your going to suggest making the block simply render the block it is occuping that won't work for every case (such as cobble walls, fences and doors) and if I make a new block for each non-normal one I'd be cloning a lot of blocks for one small job a hook could do. For an example, Minecarts can't just plop an invisible block down as the block they are occupying already has a block in it - the rail. Putting it above the cart won't work either - you'd suddenly have a hollowed-out hole above the cart if it went down a 1 X 1 rail tunnel. The only solution in this case that I know of that would be practial would be the hook. (trust me, I tried doing it with the current system - at best it removes lighting already there, at worst it creates horrible light artifacts). -
Block Rendering Hooks (NOT custom block hooks)
Groxkiller replied to Groxkiller's topic in Suggestions
Thank you for the clarification, but after testing I can definitely confirm the last hook - for block brightness - will work. I still think it would be useful to make say a spotlight entity that can shoot a light from afar or a block that has a faked brightness radius greater than 16. -
What about sun/moon texture? And could I have the whole dimension one biome? You'd need to register a sky renderer to change the sun and moon textures, (just copypaste how it's normally rendered into the renderer and replace the sun and moon texture calls with your texturepath) and yes you can have the entire world be one biome; see how the Nether stuff does it. (usually the postfix is Hell, ie WorldProviderHell)
-
add a options button for each mod on the mod list
Groxkiller replied to uyjulian's topic in Suggestions
Sliders can simply be a percentage inpurt (ie my_slider_command <0-100>) and you wouldn't need Enums with commands, you can just check for certain strings, since the data the command is given is strings. (my_string_command Creeper Explode) -
add a options button for each mod on the mod list
Groxkiller replied to uyjulian's topic in Suggestions
I get that, but my post explained why the guis wouldn't even be needed if the modders used console commands. It's up to preference of the modder though. Guis are more user-friendly but at the cost of the binding and on-screen buttons. -
add a options button for each mod on the mod list
Groxkiller replied to uyjulian's topic in Suggestions
...The point is that the gui doesn't need to exist, because the config would handle changes. If you want to change properties ingame, why not use a console command instead (ie mod_changeProp <propID> <propValue>) or a command for each property depending on which is more applicable. And FYI, you COULD make a gui from a config file. It'd be kinda dumb to do so (and the graphics would be fugly unless you use a preexisting one), but it's possible. -
add a options button for each mod on the mod list
Groxkiller replied to uyjulian's topic in Suggestions
Not sure this is needed since you can just make a config file, most anyone who is not new at modding knows what they are and where to put/edit them. -
You already CAN do this for every mob, using living spawn living events and EntityLiving.setMaxHealth(). As for the player it probably is as it is because if you made it any higher the GUI wouldn't know how to handle it. Have you considered using resistance potion effects with higher levels than normal (ie 5, 6 etc.) or armor (depending on which is more applicable)?
-
Before someone tells me they have block render hooks, that is for custom blocks. What I'm suggesting is hooks for individual block renders, ie: onBlockRenderPre(RenderBlocks renderer, Block block, int metadata, int x, int y, int z) - called before any world block render onBlockRenderPost(RenderBlocks renderer, Block block, int metadata, int x, int y, int z) - called after any world block render getBlockBrightness(EnumSkyBlock lightType, Block block, int metadata, int x, int y, int z, int currentBrightness) - Hook called to get the world brightness of the block. These kind of hooks would be primarily useful in cases of wanting to alter coloration, add some render effect to a vanilla block, change light values, or some effect to a block's render. This would also allow for entities to (somewhat awkwardly) produce light on thier own. The first two can easily be injected here (RenderBlocks.java): public boolean renderBlockByRenderType(Block par1Block, int par2, int par3, int par4) { int var5 = par1Block.getRenderType(); par1Block.setBlockBoundsBasedOnState(this.blockAccess, par2, par3, par4); this.setRenderBoundsFromBlock(par1Block); //PRE HOOK HERE switch (var5) { // ... block render stuff } //POST HOOK HERE } The Block Brightness hook would need to be put in the World class; it has far, far too many calls in RenderBlocks to be overridden there: public int getLightBrightnessForSkyBlocks(int par1, int par2, int par3, int par4) { //Sky brightness hook int var5 = eventMethodHere(EnumSkyBlock.Sky, this.getSkyBlockTypeBrightness(EnumSkyBlock.Sky, par1, par2, par3)); // Block brightness hook int var6 = eventMethodHere(EnumSkyBlock.Block, this.getSkyBlockTypeBrightness(EnumSkyBlock.Block, par1, par2, par3)); if (var6 < par4) { var6 = par4; } return var5 << 20 | var6 << 4; } The Block Brightness hook would have complications if two or more people used it at once, so my suggestion would be to check which subscriber has the highest value returned and use that, or have a priority system like in other parts of Forge. I hope this can be implemented as it would make custom lighting and block render control a lot easier.
-
What I think is happening is the value is correct Serverside but not Clientside. This is why when it drops it, the itemstack has the right value. That's handled serverside. The clientside half, however, never is updated and so the metadata is off. The easy solution is to add to EntityFoo's dataWatcher instance. Add a mapping for the metadata and update it accordingly. (To see how this is done, check out the various classes, such as Sheep, and Skeletons, which utilize it)
-
I don't think this is a wise move, as iron ore is something that's used a LOT, so removing it and putting your own might cause a lot of incompatibility issues. Is there any way of doing what you want to accomplish without replacing the ore?
-
[1.4.4] Help with ISimpleBlockRenderingHandler
Groxkiller replied to Narrakan's topic in Modder Support
What I suggest is, since your using a tile entity, to use a custom tile entity renderer instead of the block renderer. Like so: In your Client Proxy: ClientRegistry.bindTileEntitySpecialRenderer(MyTileEntity.class, new MyTileEntityRenderer()); From there, you'd just need to render the model in the renderer, something like this: MyModel model = new MyModel(); ... public void renderTileEntityAt(TileEntity tileentity, double d, double d1, double d2, float f) { GL11.glPushMatrix(); GL11.glTranslatef((float)d + 0.5F, (float)d1 - 0.5F, (float)d2 + 0.5F); GL11.glRotatef(180F, 0.0F, 0.0F, 1.0F); GL11.glTranslatef(0.0F, -2.0F, 0.0F); bindTextureByName(texturePathHere); model.renderModelAsTileEntity(0.0625F); (or something like that) GL11.glPopMatrix(); } -
[FML] ModLoader.addTrade() Causes a Crash
Groxkiller replied to Groxkiller's topic in Modder Support
Because there is no Forge version of the addTrade function that I know of, without making a handler. FML ModLoader simply set up all the stuff, so I decided to try using it first before I made my own trade handler just to see if it worked. Since it didn't I just tried with a custom handler and the results are the same. Thanks for responding! -
As the title suggests, whenever I use ModLoader.addTrade(), regardless of what I use in it, it always crashes when I click the villager and that was the trade chosen. I think the problem is it adds the trade clientside, but not on the integrated server, and when it goes to grab the item from the server it isn't there and it crashes. My Code: @ServerStarted public void onServerStarted(FMLServerStartedEvent evt) //used this because I assumed it was not setting it when the server started up, // and thus had it clientside but not serverside, but this appears not to be the issue. { for(int i = 0; i < 20; i++) //done 20 times for it to appear more often. It still happens even with only one call. { ModLoader.addTrade(0, new TradeEntry(Item.stick.shiftedIndex, 0.9F, true, 12, 14)); } } @Init public void init(FMLInitializationEvent evt) { for(int i = 0; i < 20; i++) //done 20 times for it to appear more often. It still happens even with only one call. { ModLoader.addTrade(0, new TradeEntry(Item.stick.shiftedIndex, 0.9F, true, 12, 14)); } } The actual crash:
-
I am not sure what is going wrong, but I'm converting my mods to SMP and all is working as planned except I keep getting this: Specifically: 2012-11-09 09:41:30 [FINER] [ForgeModLoader] Sending event FMLInitializationEvent to mod mcp 2012-11-09 09:41:30 [FINER] [ForgeModLoader] Sent event FMLInitializationEvent to mod mcp 2012-11-09 09:41:30 [FINER] [ForgeModLoader] Sending event FMLInitializationEvent to mod FML 2012-11-09 09:41:30 [FINER] [ForgeModLoader] Sent event FMLInitializationEvent to mod FML 2012-11-09 09:41:30 [FINER] [ForgeModLoader] Sending event FMLInitializationEvent to mod Forge 2012-11-09 09:41:30 [FINER] [ForgeModLoader] Sent event FMLInitializationEvent to mod Forge 2012-11-09 09:41:30 [FINER] [ForgeModLoader] Sending event FMLInitializationEvent to mod ModHelper 2012-11-09 09:41:30 [FINER] [ForgeModLoader] Sent event FMLInitializationEvent to mod ModHelper 2012-11-09 09:41:30 [FINER] [ForgeModLoader] Sending event FMLInitializationEvent to mod ModHelperExample 2012-11-09 09:41:30 [FINER] [ForgeModLoader] Sent event FMLInitializationEvent to mod ModHelperExample 2012-11-09 09:41:30 [FINER] [ForgeModLoader] Sending event FMLInitializationEvent to mod MobVsMachine 2012-11-09 09:41:30 [FINER] [ForgeModLoader] Sent event FMLInitializationEvent to mod MobVsMachine 2012-11-09 09:41:30 [FINER] [ForgeModLoader] Sending event FMLInitializationEvent to mod NetherBetter 2012-11-09 09:41:30 [FINER] [ForgeModLoader] Sent event FMLInitializationEvent to mod NetherBetter 2012-11-09 09:41:30 [sEVERE] [ForgeModLoader] Fatal errors were detected during the transition from INITIALIZATION to POSTINITIALIZATION. Loading cannot continue 2012-11-09 09:41:30 [iNFO] [sTDERR] java.lang.NullPointerException 2012-11-09 09:41:30 [iNFO] [sTDERR] at cpw.mods.fml.common.LoadController.printModStates(LoadController.java:194) 2012-11-09 09:41:30 [iNFO] [sTDERR] at cpw.mods.fml.common.LoadController.transition(LoadController.java:96) 2012-11-09 09:41:30 [iNFO] [sTDERR] at cpw.mods.fml.common.Loader.initializeMods(Loader.java:652) 2012-11-09 09:41:30 [iNFO] [sTDERR] at cpw.mods.fml.server.FMLServerHandler.finishServerLoading(FMLServerHandler.java:88) 2012-11-09 09:41:30 [iNFO] [sTDERR] at cpw.mods.fml.common.FMLCommonHandler.onServerStarted(FMLCommonHandler.java:344) 2012-11-09 09:41:30 [iNFO] [sTDERR] at net.minecraft.src.DedicatedServer.startServer(DedicatedServer.java:110) 2012-11-09 09:41:30 [iNFO] [sTDERR] at net.minecraft.server.MinecraftServer.run(MinecraftServer.java:454) 2012-11-09 09:41:30 [iNFO] [sTDERR] at net.minecraft.src.ThreadMinecraftServer.run(ThreadMinecraftServer.java:17) As soon as the server finished the InitializationEvents it crashes, and I haven't been able to pinpoint the error. None of the mods use PostInitializationEvent, only InitializationEvent, so that's not it either. Anyone have any insight on this?
-
I have looked into this extensively, and while it is very easy to make a custom potion item, making it brew is another matter altogether. The current setup is confusing at best and downright cryptic at worst. Sure, I can understand bits of it but nowhere near enough to add more potions to the current potion item, without causing potentially disasterous consequences. If you still think I am wrong, though, and by all means I might be, can you link to a topic explaining how to do so as I cannot find one on this forum nor the offical MC forum. @OP I am working on hooks to allow for more control over brewing, will be posting them here once it's in a respectable state, and maybe it'll get accepted.
-
[SOLVED] Server is attempting to call Client-marked code.
Groxkiller replied to Groxkiller's topic in Support & Bug Reports
Ok, I think I get what the problem is now, thanks for the help! -
Hi, new to Forge and i've been converting my mod to it as it seems to be the best option to use. However, I have hit a roadblock with the server. The second I try to start it, I get errors about classes not being found, and the pattern seems to be client-only classes: 2012-10-08 22:10:17 [iNFO] [sTDERR] java.lang.NoClassDefFoundError: net/minecraft/src/WorldClient Just for testing, I removed WorldClient's SideOnly line, and the next class that came up was ItemRenderer. Neither of these classes exist, let alone should be called, on the server, so I am a bit confused. Here is the ForgeModLoader-server-0 log: