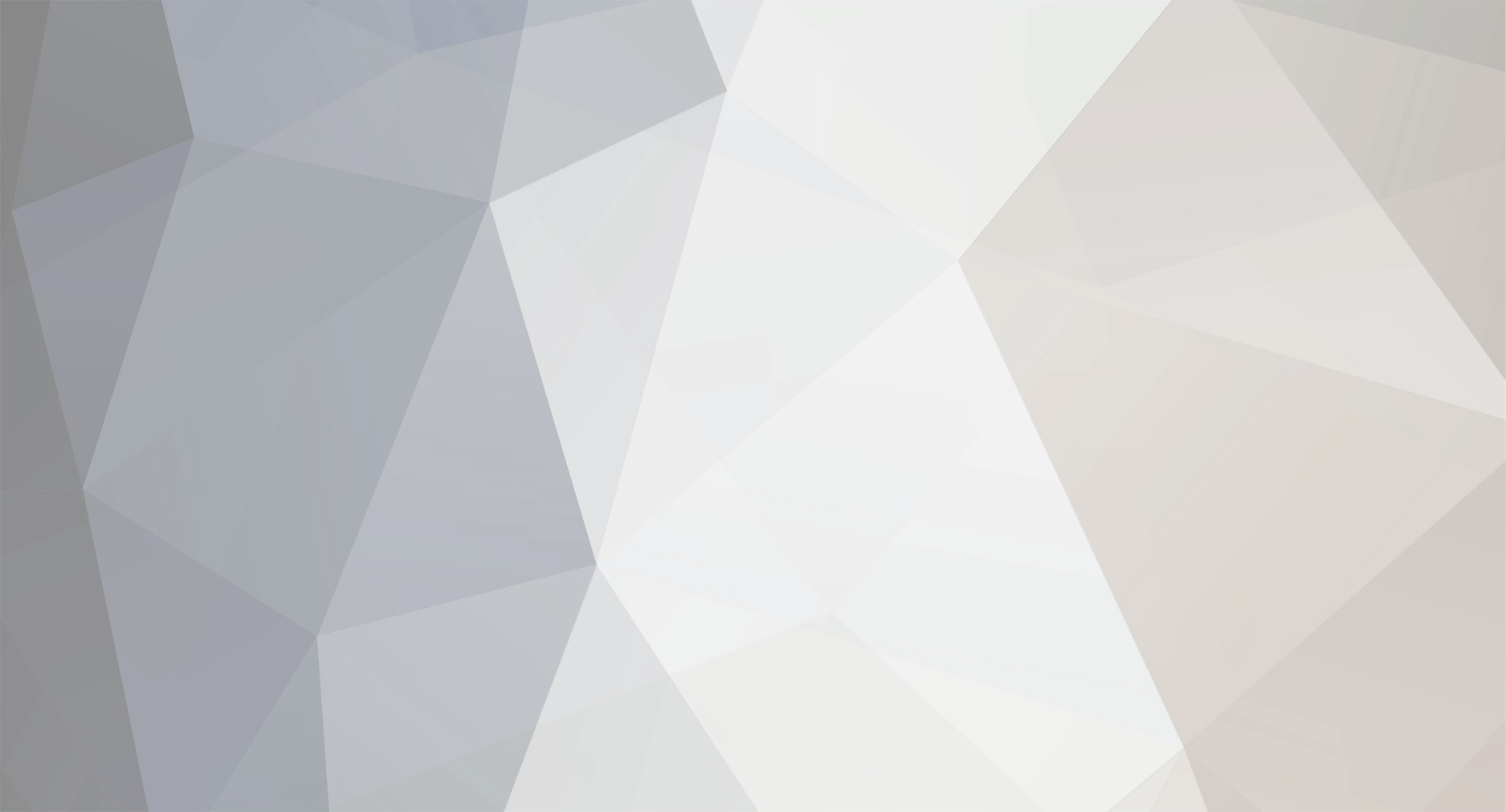
Kaneka
-
Posts
110 -
Joined
-
Last visited
Posts posted by Kaneka
-
-
Just what I read in the tutorials about the "texture block" tutorial, but not that much
-
ok, thanks.
Now I just need a tutorial or examplecode on how to make stuff render, cause all I found is just "how to texture blocks in 1.7", nothing about 1.10. I just need to render red crosses.
-
Is there a tutorial or a codeexample where I can see how to add rendering processes when pushing a button in a gui?
-
ok, thanks a lot!
-
The method addVertex isnt defined in the VertexBuffer class
-
I cant find the WorldRenderer, was it changed?
-
Ok, thanks for all the answers, I will have a look which idea fits my needs best!
-
Hi everyone,
I am thinking of adding something more complicated and dont know how to do it.
I want to add a non existing block which marks blocks that needs to be removed by the player, like clicking on a button and see which blocks need to be removed, that the tileentity have enought space to go on. Like marking the blocks, that needs to be removed with a red X or something like this. It should not change the blocks at all.
Hope it is understandable.
Thanks for your answers,
Kaneka
-
it works, thanks
-
public class BlockMold extends Block
{
public static final PropertyInteger MOLD_STATE = PropertyInteger.create("mold_state", 0, 4);
protected static final AxisAlignedBB AABB_BOTTOM_HALF = new AxisAlignedBB(0.0D, 0.0D, 0.0D, 1.0D, 0.5D, 1.0D);
public BlockMold(String uname)
{
super(Material.IRON);
this.setCreativeTab(Main.planttech);
this.setUnlocalizedName(uname);
this.setHardness(0.8F);
this.setTickRandomly(true);
this.setDefaultState(this.blockState.getBaseState().withProperty(MOLD_STATE, 0));
}
@Override
protected BlockStateContainer createBlockState()
{
return new BlockStateContainer(this, new IProperty[] { MOLD_STATE });
}
@Override
public IBlockState getActualState(IBlockState state, IBlockAccess worldIn, BlockPos pos)
{
return state;
}
@Override
public int getMetaFromState(final IBlockState state) {
return 0;
}
@Override
public AxisAlignedBB getBoundingBox(IBlockState state, IBlockAccess source, BlockPos pos)
{
return AABB_BOTTOM_HALF;
}
@Override
public boolean isOpaqueCube(IBlockState state) {
return false;
}
@Override
public BlockRenderLayer getBlockLayer() {
return BlockRenderLayer.CUTOUT;
}
@Override
public boolean isFullCube(IBlockState state) {
return false;
}
@Override
public boolean isFullyOpaque(IBlockState state) {
return false;
}
@Override
public boolean onBlockActivated(World worldIn, BlockPos pos, IBlockState state, EntityPlayer playerIn, EnumHand hand, ItemStack heldItem, EnumFacing side, float hitX, float hitY, float hitZ)
{
System.out.println(this.getMetaFromState(state));
if(state.getValue(MOLD_STATE) != 4)
{
if(heldItem != null)
{
if(heldItem.isItemEqual(new ItemStack(Moditems.plantophen)) && !plantophen)
{
if(state.getValue(MOLD_STATE) == 0)
{
worldIn.setBlockState(pos, this.blockState.getBaseState().withProperty(MOLD_STATE, 1));
if(!playerIn.isCreative())
{
if(heldItem.stackSize > 1)
{
heldItem.stackSize--;
}
else if(heldItem.stackSize == 1)
{
playerIn.setItemStackToSlot(EntityEquipmentSlot.MAINHAND, null);;
}
}
}
if(state.getValue(MOLD_STATE) == 2)
{
worldIn.setBlockState(pos, this.blockState.getBaseState().withProperty(MOLD_STATE, 3));
if(!playerIn.isCreative())
{
if(heldItem.stackSize > 1)
{
heldItem.stackSize--;
}
else if(heldItem.stackSize == 1)
{
playerIn.setItemStackToSlot(EntityEquipmentSlot.MAINHAND, null);;
}
}
}
}
if(heldItem.isItemEqual(new ItemStack(Moditems.plantodur)) && !plantodur)
{
if(state.getValue(MOLD_STATE) == 0)
{
worldIn.setBlockState(pos, this.blockState.getBaseState().withProperty(MOLD_STATE, 2));
if(!playerIn.isCreative())
{
if(heldItem.stackSize > 1)
{
heldItem.stackSize--;
}
else if(heldItem.stackSize == 1)
{
playerIn.setItemStackToSlot(EntityEquipmentSlot.MAINHAND, null);;
}
}
}
if(state.getValue(MOLD_STATE) == 1)
{
worldIn.setBlockState(pos, this.blockState.getBaseState().withProperty(MOLD_STATE, 3));
if(!playerIn.isCreative())
{
if(heldItem.stackSize > 1)
{
heldItem.stackSize--;
}
else if(heldItem.stackSize == 1)
{
playerIn.setItemStackToSlot(EntityEquipmentSlot.MAINHAND, null);;
}
}
}
}
}
}
else
{
worldIn.setBlockState(pos, this.blockState.getBaseState().withProperty(MOLD_STATE, 0));
if(!worldIn.isRemote)
{
worldIn.spawnEntityInWorld(new EntityItem(worldIn, pos.getX()+ 0.5D , pos.getY() + 1.0D, pos.getZ() + 0.5D, new ItemStack(Items.ELYTRA)));
}
}
return super.onBlockActivated(worldIn, pos, state, playerIn, hand, heldItem, side, hitX, hitY, hitZ);
}
@Override
public void updateTick(World worldIn, BlockPos pos, IBlockState state, Random rand) {
if(state.getValue(MOLD_STATE) == 3)
{
System.out.println("test2 ");
worldIn.setBlockState(pos, this.blockState.getBaseState().withProperty(MOLD_STATE, 4));
}
}
}
-
hello everyone,
I created a block with PropertyInteger of 0 - 4, and almost everything works fine.
But when leave the world and join again, the block is set on 4, dosn´t matter which value was there before.
example: Before relog: anyblock:0 .After relog: anyblock:4
Which methods defines the value?
thanks for the answer
-
Just override the isFullyOpaque method:
@Override public boolean isFullyOpaque(IBlockState state) { return false; }
For my mod it works
-
When the item is one of your modded items, override the onItemRightClick method, if it is a vanilla item, use the LivingEntityUseItemEvent
here an example:
public ActionResult<ItemStack> onItemRightClick(ItemStack itemStackIn, World worldIn, EntityPlayer playerIn, EnumHand hand) { if(worldIn.isRemote) { playerIn.openGui(Main.instance, Main.GUI_ENUM.YOURITEM.ordinal(), worldIn, (int)playerIn.posX, (int)playerIn.posY (int)playerIn.posZ); } } }
-
ok thanks
-
isnt there a event in the ItemArmor or Item class like there is the hitEntity for sword
-
When the player is attacked while wearing my armor, so it dosnt matter if there is unbreaking or not
-
Hi everyone,
I look for a method in ItemArmor that is only called when the armor gets damage.
Like there is the hitEntity for sword or onBlockdestroyed for tools.
I looked for this methis a long time, but cant find it, can someone help me?
Thanks for the answers
Kaneka
-
Thanks, it works now
-
Thanks for the answer, is there a example?
-
Hi everyone,
I try to use loot_tables in my mod but get these error(I cant see how to fix this)
[20:49:51] [server thread/ERROR]: Couldn't load loot table planttech:loot_table_leaved_floating_island from file:/C:/Users/chris/Documents/Minecraft%20Mod/Planttech/forge-1.9.4-12.17.0.1937-mdk/bin/assets/planttech/loot_tables/loot_table_leaved_floating_island.json
com.google.gson.JsonParseException: Loot Table "planttech:loot_table_leaved_floating_island" Missing `name` entry for pool #0
at net.minecraftforge.common.ForgeHooks.readPoolName(ForgeHooks.java:1089) ~[ForgeHooks.class:?]
at net.minecraft.world.storage.loot.LootPool$Serializer.deserialize(LootPool.java:152) ~[LootPool$Serializer.class:?]
at net.minecraft.world.storage.loot.LootPool$Serializer.deserialize(LootPool.java:147) ~[LootPool$Serializer.class:?]
at com.google.gson.TreeTypeAdapter.read(TreeTypeAdapter.java:58) ~[TreeTypeAdapter.class:?]
at com.google.gson.internal.bind.TypeAdapterRuntimeTypeWrapper.read(TypeAdapterRuntimeTypeWrapper.java:40) ~[TypeAdapterRuntimeTypeWrapper.class:?]
at com.google.gson.internal.bind.ArrayTypeAdapter.read(ArrayTypeAdapter.java:72) ~[ArrayTypeAdapter.class:?]
at com.google.gson.Gson.fromJson(Gson.java:803) ~[Gson.class:?]
at com.google.gson.Gson.fromJson(Gson.java:868) ~[Gson.class:?]
at com.google.gson.Gson$1.deserialize(Gson.java:126) ~[Gson$1.class:?]
at net.minecraft.util.JsonUtils.deserializeClass(JsonUtils.java:378) ~[JsonUtils.class:?]
at net.minecraft.util.JsonUtils.deserializeClass(JsonUtils.java:400) ~[JsonUtils.class:?]
at net.minecraft.world.storage.loot.LootTable$Serializer.deserialize(LootTable.java:209) ~[LootTable$Serializer.class:?]
at net.minecraft.world.storage.loot.LootTable$Serializer.deserialize(LootTable.java:204) ~[LootTable$Serializer.class:?]
at com.google.gson.TreeTypeAdapter.read(TreeTypeAdapter.java:58) ~[TreeTypeAdapter.class:?]
at com.google.gson.Gson.fromJson(Gson.java:803) ~[Gson.class:?]
at com.google.gson.Gson.fromJson(Gson.java:768) ~[Gson.class:?]
at com.google.gson.Gson.fromJson(Gson.java:717) ~[Gson.class:?]
at com.google.gson.Gson.fromJson(Gson.java:689) ~[Gson.class:?]
at net.minecraftforge.common.ForgeHooks.loadLootTable(ForgeHooks.java:1013) ~[ForgeHooks.class:?]
at net.minecraft.world.storage.loot.LootTableManager$Loader.loadBuiltinLootTable(LootTableManager.java:148) [LootTableManager$Loader.class:?]
at net.minecraft.world.storage.loot.LootTableManager$Loader.load(LootTableManager.java:71) [LootTableManager$Loader.class:?]
at net.minecraft.world.storage.loot.LootTableManager$Loader.load(LootTableManager.java:52) [LootTableManager$Loader.class:?]
at com.google.common.cache.LocalCache$LoadingValueReference.loadFuture(LocalCache.java:3524) [guava-17.0.jar:?]
at com.google.common.cache.LocalCache$Segment.loadSync(LocalCache.java:2317) [guava-17.0.jar:?]
at com.google.common.cache.LocalCache$Segment.lockedGetOrLoad(LocalCache.java:2280) [guava-17.0.jar:?]
at com.google.common.cache.LocalCache$Segment.get(LocalCache.java:2195) [guava-17.0.jar:?]
at com.google.common.cache.LocalCache.get(LocalCache.java:3934) [guava-17.0.jar:?]
at com.google.common.cache.LocalCache.getOrLoad(LocalCache.java:3938) [guava-17.0.jar:?]
at com.google.common.cache.LocalCache$LocalLoadingCache.get(LocalCache.java:4821) [guava-17.0.jar:?]
at com.google.common.cache.LocalCache$LocalLoadingCache.getUnchecked(LocalCache.java:4827) [guava-17.0.jar:?]
at net.minecraft.world.storage.loot.LootTableManager.getLootTableFromLocation(LootTableManager.java:39) [LootTableManager.class:?]
at net.minecraft.tileentity.TileEntityLockableLoot.fillWithLoot(TileEntityLockableLoot.java:55) [TileEntityLockableLoot.class:?]
at net.minecraft.tileentity.TileEntityChest.createContainer(TileEntityChest.java:487) [TileEntityChest.class:?]
at net.minecraft.entity.player.EntityPlayerMP.displayGUIChest(EntityPlayerMP.java:860) [EntityPlayerMP.class:?]
at net.minecraft.block.BlockChest.onBlockActivated(BlockChest.java:420) [blockChest.class:?]
at net.minecraft.server.management.PlayerInteractionManager.processRightClickBlock(PlayerInteractionManager.java:475) [PlayerInteractionManager.class:?]
at net.minecraft.network.NetHandlerPlayServer.processRightClickBlock(NetHandlerPlayServer.java:707) [NetHandlerPlayServer.class:?]
at net.minecraft.network.play.client.CPacketPlayerTryUseItemOnBlock.processPacket(CPacketPlayerTryUseItemOnBlock.java:68) [CPacketPlayerTryUseItemOnBlock.class:?]
at net.minecraft.network.play.client.CPacketPlayerTryUseItemOnBlock.processPacket(CPacketPlayerTryUseItemOnBlock.java:13) [CPacketPlayerTryUseItemOnBlock.class:?]
at net.minecraft.network.PacketThreadUtil$1.run(PacketThreadUtil.java:15) [PacketThreadUtil$1.class:?]
at java.util.concurrent.Executors$RunnableAdapter.call(Unknown Source) [?:1.8.0_73]
at java.util.concurrent.FutureTask.run(Unknown Source) [?:1.8.0_73]
at net.minecraft.util.Util.runTask(Util.java:25) [util.class:?]
at net.minecraft.server.MinecraftServer.updateTimeLightAndEntities(MinecraftServer.java:740) [MinecraftServer.class:?]
at net.minecraft.server.MinecraftServer.tick(MinecraftServer.java:685) [MinecraftServer.class:?]
at net.minecraft.server.integrated.IntegratedServer.tick(IntegratedServer.java:155) [integratedServer.class:?]
at net.minecraft.server.MinecraftServer.run(MinecraftServer.java:534) [MinecraftServer.class:?]
at java.lang.Thread.run(Unknown Source) [?:1.8.0_73]
here is the loot_table:
{ "pools": [ { "rolls": { "min": 2, "max": 4 }, "entries": [ { "type": "item", "name": "planttech:cookie_blue", "functions": [ { "function": "set_count", "count": { "min": 2, "max": 5 } } ], "weight": 25 }, { "type": "item", "name": "planttech:cookie_yellow", "functions": [ { "function": "set_count", "count": { "min": 2, "max": 5 } } ], "weight": 25 }, { "type": "item", "name": "planttech:cookie_pink", "functions": [ { "function": "set_count", "count": { "min": 2, "max": 5 } } ], "weight": 25 }, { "type": "item", "name": "planttech:cookie_red", "functions": [ { "function": "set_count", "count": { "min": 2, "max": 5 } } ], "weight": 25 }, { "type": "item", "name": "planttech:cookie_white", "functions": [ { "function": "set_count", "count": { "min": 2, "max": 5 } } ], "weight": 25 }, { "type": "item", "name": "planttech:metalblock", "functions": [ { "function": "set_count", "count": { "min": 2, "max": 5 } } ], "weight": 25 }, { "type": "item", "name": "planttech:plantwire", "functions": [ { "function": "set_count", "count": { "min": 10, "max": 25 } } ], "weight": 25 }, { "type": "item", "name": "planttech:crystal_diamond", "functions": [ { "function": "set_count", "count": { "min": 1, "max": 2 } } ], "weight": 15 } ] } ] }
-
Feeling so dump....it causes an exeption when trying to do writetoNBT cause controllercoordinates was null....
Thanks for the help jerryfisher
-
GameRegistry.registerTileEntity(TileEntitySolarPlant.class, "tileentitysolarplant");
it is like all my other are and these works fine. Thats what disturb me, everyone of my other TE works. And the compiler don´t tell me what I did wrong...then I could correct it....
-
Hi everyone,
I am trying to add a solar plants that produces energy. But after a restart the already created energy is removed and until I click on the block, it wont produce energy(when I click on it after waiting a bit, it says "Energy 0/16000")
Here is the code:
Here the SolarPlant.class
package net.kaneka.planttech.blocks.machines.energy.producer; import net.kaneka.planttech.Main; import net.kaneka.planttech.blocks.tileentities.TileEntityCompostBin; import net.kaneka.planttech.blocks.tileentities.energy.producer.TileEntityCreativeEnergyProducer; import net.kaneka.planttech.blocks.tileentities.energy.producer.TileEntitySolarPlant; import net.minecraft.block.Block; import net.minecraft.block.BlockContainer; import net.minecraft.block.material.Material; import net.minecraft.block.state.IBlockState; import net.minecraft.entity.EntityLivingBase; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.item.ItemStack; import net.minecraft.tileentity.TileEntity; import net.minecraft.util.BlockRenderLayer; import net.minecraft.util.EnumBlockRenderType; import net.minecraft.util.EnumFacing; import net.minecraft.util.EnumHand; import net.minecraft.util.math.BlockPos; import net.minecraft.util.text.TextComponentString; import net.minecraft.util.text.TextFormatting; import net.minecraft.world.World; public class SolarPlant extends BlockContainer { public SolarPlant() { super(Material.wood); this.setUnlocalizedName("solarplant"); this.setCreativeTab(Main.planttech3); this.setHardness(0.5F); } @Override public TileEntity createNewTileEntity(World worldIn, int meta) { return new TileEntitySolarPlant(); } @Override public EnumBlockRenderType getRenderType(IBlockState state) { return EnumBlockRenderType.MODEL; } @Override public boolean onBlockActivated(World worldIn, BlockPos pos, IBlockState state, EntityPlayer playerIn, EnumHand hand, ItemStack heldItem, EnumFacing side, float hitX, float hitY, float hitZ) { if(!worldIn.isRemote) { TileEntity tileentity = worldIn.getTileEntity(pos); if (tileentity instanceof TileEntitySolarPlant) { playerIn.addChatMessage(new TextComponentString("Energy:"+((TileEntitySolarPlant) tileentity).getCurrentEnergy() + "/" + ((TileEntitySolarPlant) tileentity).getEnergyStorageLimit())); } } return true; } @Override public IBlockState onBlockPlaced(World worldIn, BlockPos pos, EnumFacing facing, float hitX, float hitY, float hitZ, int meta, EntityLivingBase placer) { worldIn.addTileEntity(new TileEntitySolarPlant()); return super.onBlockPlaced(worldIn, pos, facing, hitX, hitY, hitZ, meta, placer); } @Override public boolean isFullBlock(IBlockState state) { return false; } @Override public BlockRenderLayer getBlockLayer() { return BlockRenderLayer.CUTOUT; } @Override public boolean isOpaqueCube(IBlockState state) { return false; } }
and here the TileEntitySolarPlant.class
package net.kaneka.planttech.blocks.tileentities.energy.producer; import net.kaneka.planttech.energysystem.interfaces.IEnergyConsumer; import net.kaneka.planttech.energysystem.interfaces.IEnergyController; import net.kaneka.planttech.energysystem.interfaces.IEnergyProducer; import net.minecraft.block.Block; import net.minecraft.nbt.NBTTagCompound; import net.minecraft.tileentity.TileEntity; import net.minecraft.util.ITickable; import net.minecraft.util.math.BlockPos; import net.minecraft.world.World; public class TileEntitySolarPlant extends TileEntity implements ITickable, IEnergyProducer{ private int maxEnergy = 16000; private int energyatm = 0; private int energyPerTick = 1; private int tickstillremove = 25; private BlockPos controllercoordinates; @Override public void setNetwork(World world, BlockPos controller, int cableAmount, int cableTier) { boolean alreadyregisted = false; tickstillremove = 25; if(controllercoordinates != controller) { alreadyregisted = true; } if(alreadyregisted) { this.controllercoordinates = controller; Block controllerblock = world.getBlockState(controller).getBlock(); if(controllerblock.hasTileEntity(controllerblock.getDefaultState())) { TileEntity te = world.getTileEntity(controller); if(te instanceof IEnergyController) { ((IEnergyController) te).registerEnergyConsumer(this.pos, cableAmount, cableTier); } } } } @Override public int getEnergyStorageLimit() { return maxEnergy; } @Override public int getCurrentEnergy() { return energyatm; } @Override public void reduceCE(int amount) { if(energyatm >= amount) { energyatm = energyatm - amount; } } @Override public void update() { if(this.getWorld().getLightFromNeighbors(pos.up()) > 14) { if(maxEnergy >= (energyatm + energyPerTick)) { energyatm = energyatm + energyPerTick; } if(maxEnergy < (energyatm + energyPerTick)) { energyatm = maxEnergy; } } } @Override public void readFromNBT(NBTTagCompound compound) { super.readFromNBT(compound); energyatm = compound.getInteger("energyatm"); tickstillremove = compound.getShort("TicksTillRemove"); int x = compound.getShort("ControllerX"); int y = compound.getShort("ControllerY"); int z = compound.getShort("ControllerZ"); controllercoordinates = new BlockPos(x, y, z); } @Override public void writeToNBT(NBTTagCompound compound) { super.writeToNBT(compound); compound.setInteger("energyatm", energyatm); compound.setShort("TicksTillRemove", (short)tickstillremove); compound.setShort("ControllerX", (short)controllercoordinates.getX()); compound.setShort("ControllerY", (short)controllercoordinates.getY()); compound.setShort("ControllerZ", (short)controllercoordinates.getZ()); } }
I hope someone can help me.
Thanks for every answer!
Kaneka
-
Thanks, and how do I set my own ChestGenHook?
Not just adding Blocks and Items, I want a complete own.
[1.10.2][CLOSED]Creating Non Existing Blocks
in Modder Support
Posted
That was how to render a block, I just want to draw lines to make crosses to mark some existing blocks. best would be examplecode, but thanks for xou effort till here