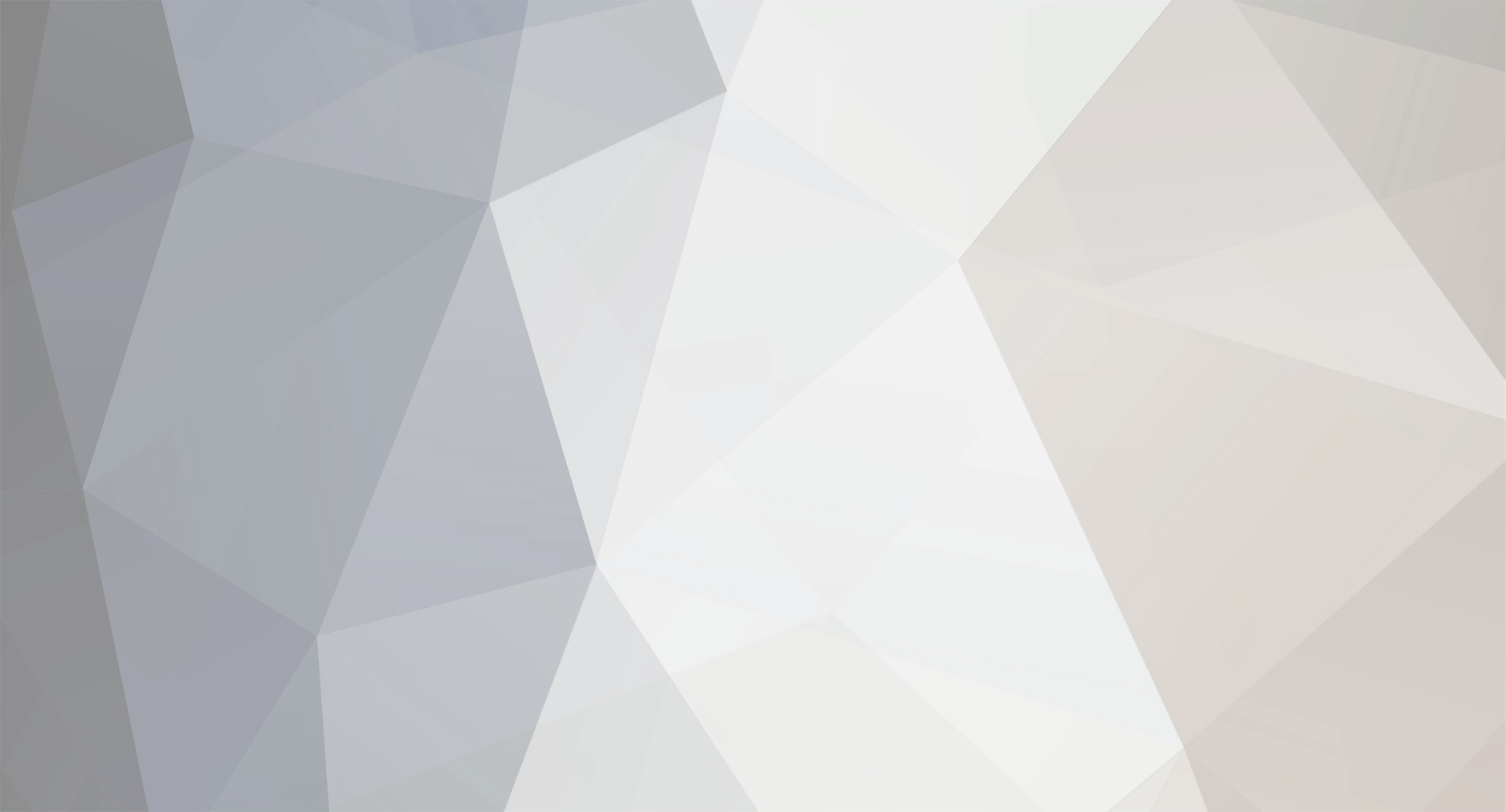
Malkierian
-
Posts
42 -
Joined
-
Last visited
Posts posted by Malkierian
-
-
So I have a custom TNT-type block, Acid TNT, that I have implemented, and the block and entity are working just fine (I can activate it, the explosion is run, etc), however the rendering for EntityAcidTNTPrimed is non-existent. I have another entity being rendered properly in the mod, so I know my registration process isn't at fault. I tried decompiling another mod, TNT Mod, to have a look at their code, but I couldn't see anything different between their process and mine. I really can't figure it out. Here is the relevant code.
Registration (called from preInit())
SpoilerRenderingRegistry.registerEntityRenderingHandler(EntityAcidTNTPrimed.class, RenderAcidTNTPrimed::new);
BlockAcidTNT.java
Spoilerpackage malkierian.plasmacraft.blocks; import javax.annotation.Nullable; import malkierian.plasmacraft.PlasmaCraft; import malkierian.plasmacraft.entity.EntityAcidTNTPrimed; import net.minecraft.block.Block; import net.minecraft.block.material.Material; import net.minecraft.block.properties.IProperty; import net.minecraft.block.properties.PropertyBool; import net.minecraft.block.state.BlockStateContainer; import net.minecraft.block.state.IBlockState; import net.minecraft.entity.Entity; import net.minecraft.entity.EntityLivingBase; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.entity.projectile.EntityArrow; import net.minecraft.init.Blocks; import net.minecraft.init.Items; import net.minecraft.init.SoundEvents; import net.minecraft.item.ItemStack; import net.minecraft.util.EnumFacing; import net.minecraft.util.EnumHand; import net.minecraft.util.SoundCategory; import net.minecraft.util.math.BlockPos; import net.minecraft.world.Explosion; import net.minecraft.world.World; public class BlockAcidTNT extends Block { public static final PropertyBool EXPLODE = PropertyBool.create("explode"); public BlockAcidTNT() { super(Material.TNT); setDefaultState(blockState.getBaseState().withProperty(EXPLODE, Boolean.valueOf(false))); this.setCreativeTab(PlasmaCraft.plasmaTab); } /** * Called after the block is set in the Chunk data, but before the Tile Entity is set */ public void onBlockAdded(World worldIn, BlockPos pos, IBlockState state) { super.onBlockAdded(worldIn, pos, state); if (worldIn.isBlockPowered(pos)) { this.onBlockDestroyedByPlayer(worldIn, pos, state.withProperty(EXPLODE, Boolean.valueOf(true))); worldIn.setBlockToAir(pos); } } /** * Called when a neighboring block was changed and marks that this state should perform any checks during a neighbor * change. Cases may include when redstone power is updated, cactus blocks popping off due to a neighboring solid * block, etc. */ public void neighborChanged(IBlockState state, World worldIn, BlockPos pos, Block blockIn) { if (worldIn.isBlockPowered(pos)) { this.onBlockDestroyedByPlayer(worldIn, pos, state.withProperty(EXPLODE, Boolean.valueOf(true))); worldIn.setBlockToAir(pos); } } /** * Called when this Block is destroyed by an Explosion */ public void onBlockDestroyedByExplosion(World worldIn, BlockPos pos, Explosion explosionIn) { if (!worldIn.isRemote) { EntityAcidTNTPrimed entitytntprimed = new EntityAcidTNTPrimed(worldIn, (double)((float)pos.getX() + 0.5F), (double)pos.getY(), (double)((float)pos.getZ() + 0.5F), explosionIn.getExplosivePlacedBy()); entitytntprimed.setFuse((short)(worldIn.rand.nextInt(entitytntprimed.getFuse() / 4) + entitytntprimed.getFuse() / 8)); worldIn.spawnEntityInWorld(entitytntprimed); } } /** * Called when a player destroys this Block */ public void onBlockDestroyedByPlayer(World worldIn, BlockPos pos, IBlockState state) { this.explode(worldIn, pos, state, (EntityLivingBase)null); } public void explode(World worldIn, BlockPos pos, IBlockState state, EntityLivingBase igniter) { if (!worldIn.isRemote) { if (((Boolean)state.getValue(EXPLODE)).booleanValue()) { EntityAcidTNTPrimed entitytntprimed = new EntityAcidTNTPrimed(worldIn, (double)((float)pos.getX() + 0.5F), (double)pos.getY(), (double)((float)pos.getZ() + 0.5F), igniter); worldIn.spawnEntityInWorld(entitytntprimed); worldIn.playSound((EntityPlayer)null, entitytntprimed.posX, entitytntprimed.posY, entitytntprimed.posZ, SoundEvents.ENTITY_TNT_PRIMED, SoundCategory.BLOCKS, 1.0F, 1.0F); } } } public boolean onBlockActivated(World worldIn, BlockPos pos, IBlockState state, EntityPlayer playerIn, EnumHand hand, @Nullable ItemStack heldItem, EnumFacing side, float hitX, float hitY, float hitZ) { if (heldItem != null && (heldItem.getItem() == Items.FLINT_AND_STEEL || heldItem.getItem() == Items.FIRE_CHARGE)) { this.explode(worldIn, pos, state.withProperty(EXPLODE, Boolean.valueOf(true)), playerIn); worldIn.setBlockState(pos, Blocks.AIR.getDefaultState(), 11); if (heldItem.getItem() == Items.FLINT_AND_STEEL) { heldItem.damageItem(1, playerIn); } else if (!playerIn.capabilities.isCreativeMode) { --heldItem.stackSize; } return true; } else { return super.onBlockActivated(worldIn, pos, state, playerIn, hand, heldItem, side, hitX, hitY, hitZ); } } /** * Called When an Entity Collided with the Block */ public void onEntityCollidedWithBlock(World worldIn, BlockPos pos, IBlockState state, Entity entityIn) { if (!worldIn.isRemote && entityIn instanceof EntityArrow) { EntityArrow entityarrow = (EntityArrow)entityIn; if (entityarrow.isBurning()) { this.explode(worldIn, pos, worldIn.getBlockState(pos).withProperty(EXPLODE, Boolean.valueOf(true)), entityarrow.shootingEntity instanceof EntityLivingBase ? (EntityLivingBase)entityarrow.shootingEntity : null); worldIn.setBlockToAir(pos); } } } /** * Return whether this block can drop from an explosion. */ public boolean canDropFromExplosion(Explosion explosionIn) { return false; } /** * Convert the given metadata into a BlockState for this Block */ public IBlockState getStateFromMeta(int meta) { return this.getDefaultState().withProperty(EXPLODE, Boolean.valueOf((meta & 1) > 0)); } /** * Convert the BlockState into the correct metadata value */ public int getMetaFromState(IBlockState state) { return ((Boolean)state.getValue(EXPLODE)).booleanValue() ? 1 : 0; } protected BlockStateContainer createBlockState() { return new BlockStateContainer(this, new IProperty[] {EXPLODE}); } }
EntityAcidTNT.java
Spoilerpackage malkierian.plasmacraft.entity; import malkierian.plasmacraft.world.AcidExplosion; import net.minecraft.entity.Entity; import net.minecraft.entity.EntityLivingBase; import net.minecraft.entity.item.EntityTNTPrimed; import net.minecraft.nbt.NBTTagCompound; import net.minecraft.network.datasync.DataParameter; import net.minecraft.network.datasync.DataSerializers; import net.minecraft.network.datasync.EntityDataManager; import net.minecraft.util.EnumParticleTypes; import net.minecraft.world.Explosion; import net.minecraft.world.World; public class EntityAcidTNTPrimed extends Entity { private static final DataParameter<Integer> FUSE = EntityDataManager.<Integer>createKey(EntityAcidTNTPrimed.class, DataSerializers.VARINT); private EntityLivingBase tntPlacedBy; /** How long the fuse is */ private int fuse; public EntityAcidTNTPrimed(World worldIn) { super(worldIn); this.fuse = 80; this.preventEntitySpawning = true; this.setSize(0.98F, 0.98F); } public EntityAcidTNTPrimed(World worldIn, double x, double y, double z, EntityLivingBase igniter) { this(worldIn); this.setPosition(x, y, z); float f = (float)(Math.random() * (Math.PI * 2D)); this.motionX = (double)(-((float)Math.sin((double)f)) * 0.02F); this.motionY = 0.20000000298023224D; this.motionZ = (double)(-((float)Math.cos((double)f)) * 0.02F); this.setFuse(80); this.prevPosX = x; this.prevPosY = y; this.prevPosZ = z; this.tntPlacedBy = igniter; } protected void entityInit() { this.dataManager.register(FUSE, Integer.valueOf(80)); } /** * returns if this entity triggers Block.onEntityWalking on the blocks they walk on. used for spiders and wolves to * prevent them from trampling crops */ protected boolean canTriggerWalking() { return false; } /** * Returns true if other Entities should be prevented from moving through this Entity. */ public boolean canBeCollidedWith() { return !this.isDead; } /** * Called to update the entity's position/logic. */ public void onUpdate() { this.prevPosX = this.posX; this.prevPosY = this.posY; this.prevPosZ = this.posZ; if (!this.hasNoGravity()) { this.motionY -= 0.03999999910593033D; } this.moveEntity(this.motionX, this.motionY, this.motionZ); this.motionX *= 0.9800000190734863D; this.motionY *= 0.9800000190734863D; this.motionZ *= 0.9800000190734863D; if (this.onGround) { this.motionX *= 0.699999988079071D; this.motionZ *= 0.699999988079071D; this.motionY *= -0.5D; } --this.fuse; if (this.fuse <= 0) { this.setDead(); if (!this.worldObj.isRemote) { this.explode(); } } else { this.handleWaterMovement(); this.worldObj.spawnParticle(EnumParticleTypes.SMOKE_NORMAL, this.posX, this.posY + 0.5D, this.posZ, 0.0D, 0.0D, 0.0D, new int[0]); } } private void explode() { float f = 10F; AcidExplosion explosion = new AcidExplosion(worldObj, null, posX, posY, posZ, f, false, true); if (net.minecraftforge.event.ForgeEventFactory.onExplosionStart(worldObj, explosion)) return; explosion.doExplosionA(); explosion.doExplosionB(true); } /** * (abstract) Protected helper method to write subclass entity data to NBT. */ protected void writeEntityToNBT(NBTTagCompound compound) { compound.setShort("Fuse", (short)this.getFuse()); } /** * (abstract) Protected helper method to read subclass entity data from NBT. */ protected void readEntityFromNBT(NBTTagCompound compound) { this.setFuse(compound.getShort("Fuse")); } /** * returns null or the entityliving it was placed or ignited by */ public EntityLivingBase getTntPlacedBy() { return this.tntPlacedBy; } public float getEyeHeight() { return 0.0F; } public void setFuse(int fuseIn) { this.dataManager.set(FUSE, Integer.valueOf(fuseIn)); this.fuse = fuseIn; } public void notifyDataManagerChange(DataParameter<?> key) { if (FUSE.equals(key)) { this.fuse = this.getFuseDataManager(); } } /** * Gets the fuse from the data manager */ public int getFuseDataManager() { return ((Integer)this.dataManager.get(FUSE)).intValue(); } public int getFuse() { return this.fuse; } }
RenderAcidTNTPrimed
Spoilerpackage malkierian.plasmacraft.client.renderers; import malkierian.plasmacraft.PlasmaCraft; import malkierian.plasmacraft.entity.EntityAcidTNTPrimed; import malkierian.plasmacraft.init.PCBlocks; import net.minecraft.client.Minecraft; import net.minecraft.client.renderer.BlockRendererDispatcher; import net.minecraft.client.renderer.GlStateManager; import net.minecraft.client.renderer.entity.Render; import net.minecraft.client.renderer.entity.RenderManager; import net.minecraft.client.renderer.texture.TextureMap; import net.minecraft.init.Blocks; import net.minecraft.util.ResourceLocation; import net.minecraft.util.math.MathHelper; import net.minecraftforge.fml.relauncher.Side; import net.minecraftforge.fml.relauncher.SideOnly; @SideOnly(Side.CLIENT) public class RenderAcidTNTPrimed extends Render<EntityAcidTNTPrimed> { public RenderAcidTNTPrimed(RenderManager renderManagerIn) { super(renderManagerIn); this.shadowSize = 0.5F; } /** * Renders the desired {@code T} type Entity. */ public void doRender(EntityAcidTNTPrimed entity, double x, double y, double z, float entityYaw, float partialTicks) { BlockRendererDispatcher blockrendererdispatcher = Minecraft.getMinecraft().getBlockRendererDispatcher(); GlStateManager.pushMatrix(); GlStateManager.translate((float)x, (float)y + 0.5F, (float)z); if ((float)entity.getFuse() - partialTicks + 1.0F < 10.0F) { float f = 1.0F - ((float)entity.getFuse() - partialTicks + 1.0F) / 10.0F; f = MathHelper.clamp_float(f, 0.0F, 1.0F); f = f * f; f = f * f; float f1 = 1.0F + f * 0.3F; GlStateManager.scale(f1, f1, f1); } float f2 = (1.0F - ((float)entity.getFuse() - partialTicks + 1.0F) / 100.0F) * 0.8F; this.bindEntityTexture(entity); GlStateManager.rotate(-90.0F, 0.0F, 1.0F, 0.0F); GlStateManager.translate(-0.5F, -0.5F, 0.5F); blockrendererdispatcher.renderBlockBrightness(PCBlocks.acidTnt.getDefaultState(), entity.getBrightness(partialTicks)); GlStateManager.translate(0.0F, 0.0F, 1.0F); if (this.renderOutlines) { GlStateManager.enableColorMaterial(); GlStateManager.enableOutlineMode(this.getTeamColor(entity)); blockrendererdispatcher.renderBlockBrightness(PCBlocks.acidTnt.getDefaultState(), 1.0F); GlStateManager.disableOutlineMode(); GlStateManager.disableColorMaterial(); } else if (entity.getFuse() / 5 % 2 == 0) { GlStateManager.disableTexture2D(); GlStateManager.disableLighting(); GlStateManager.enableBlend(); GlStateManager.blendFunc(GlStateManager.SourceFactor.SRC_ALPHA, GlStateManager.DestFactor.DST_ALPHA); GlStateManager.color(1.0F, 1.0F, 1.0F, f2); GlStateManager.doPolygonOffset(-3.0F, -3.0F); GlStateManager.enablePolygonOffset(); blockrendererdispatcher.renderBlockBrightness(PCBlocks.acidTnt.getDefaultState(), 1.0F); GlStateManager.doPolygonOffset(0.0F, 0.0F); GlStateManager.disablePolygonOffset(); GlStateManager.color(1.0F, 1.0F, 1.0F, 1.0F); GlStateManager.disableBlend(); GlStateManager.enableLighting(); GlStateManager.enableTexture2D(); } GlStateManager.popMatrix(); super.doRender(entity, x, y, z, entityYaw, partialTicks); } /** * Returns the location of an entity's texture. Doesn't seem to be called unless you call Render.bindEntityTexture. */ @Override protected ResourceLocation getEntityTexture(EntityAcidTNTPrimed entity) { return TextureMap.LOCATION_BLOCKS_TEXTURE; } }
The weird thing is that all three are almost identical to their vanilla TNT equivalents, so... Also, I tried using RenderTNTPrimed as the renderer registered for EntityAcidTNTPrimed, and that still made the block disappear (which is the root problem for the primed rendering).
-
So I have my code set up such that (following diesieben07's tutorial here) I have the ordering list setup, and it works just fine, but I have to use individual Item references in order to get all of my blocks in the ordering list. My question is, since a good number of them are instances of just a few classes, is it possible to send a reference to the class into the sorter instead of having to list every one by itself?
For example:
SpoilerList<Item> order = Arrays.asList(Item.getItemFromBlock(PCBlocks.glowClothAcid), Item.getItemFromBlock(PCBlocks.glowClothCryonite), Item.getItemFromBlock(PCBlocks.glowClothNeptunium), Item.getItemFromBlock(PCBlocks.glowClothNetherflow), Item.getItemFromBlock(PCBlocks.glowClothObsidium), Item.getItemFromBlock(PCBlocks.glowClothPlutonium), Item.getItemFromBlock(PCBlocks.glowClothRadionite), Item.getItemFromBlock(PCBlocks.glowClothUranium), Item.getItemFromBlock(PCBlocks.oreLead), Item.getItemFromBlock(PCBlocks.oreNeptunium), Item.getItemFromBlock(PCBlocks.oreObsidium), Item.getItemFromBlock(PCBlocks.orePlutonium), Item.getItemFromBlock(PCBlocks.oreRadionite), Item.getItemFromBlock(PCBlocks.oreUranium), Item.getItemFromBlock(PCBlocks.frozenCryonite), Item.getItemFromBlock(PCBlocks.reinforcedGlass), /*Item.getItemFromBlock(PCBlocks.acidTnt),*/ Item.getItemFromBlock(PCBlocks.acidBarrier), PCItems.GOOP, PCItems.INGOTS, PCItems.VIALS /*PCItems.causticBoat, PCItems.battery, PCItems.beamSplitter, PCItems.energyCell*/, PCItems.THERMOPELLET, PCItems.ACID_GUN/*, PCItems.cryoblaster, PCItems.lasershotgun, PCItems.lasergun, PCItems.lasergunsplit, PCItems.plasmagun, PCItems.plasmagunsplit, PCItems.railgun, PCItems.acidGrenade, PCItems.hazmatBoots, PCItems.hazmatHood, PCItems.hazmatJacket, PCItems.hazmatPants, PCItems.plasmaLeather*/); tabSorter = Ordering.explicit(order).onResultOf(new Function<ItemStack, Item>() { @Override public Item apply(ItemStack input) { return input.getItem(); } });
All of those glowcloths listed first. It would cut down my code by 7 references if I could just use the BlockGlowCloth class as the sorting element instead of all these instances. I initially moved away from the metadata block process because it seemed unnecessary anymore. Is having them be subitems on an ItemBlock the only option I have?
-
1 hour ago, Jay Avery said:
All asset names must be completely lower case - names of blockstates files, models, and textures.
Was this a change in 1.11? Because that's not true in 1.10. Just want to know for when I update my mod to 1.11.
-
Little bit more information, if I set the viscosity of my caustic fluid to a certain level, then the interactions manage to keep up (with how I've set flowInto et al), but below that level, it's like the block change events can't keep up with the speed of the fluid, and it still flows into the water sources. Now THAT sounds like a bug in Forge, I may just have to work around it and not have super fast liquids.
-
Alright, so I changed canFlowInto and even displaceIfPossible to only return true when the block it's flowing into is air or replaceable (check by Material::isReplaceable()), and I still get the checkerboard problem, but at the very least I don't get that constant back and forth shown in the first gif/video. I didn't see any reason to override flowIntoBlock, since it only used displaceIfPossible anyway.
23 hours ago, V0idWa1k3r said:If that is the reason for custom materials then you are doing it wrong, as there is Block::getMapColor.
Also, Block::getMapColor() is deprecated in 1.10, meaning it's likely to be removed soon, so your point about using that instead of MaterialLiquid to customize map colors seems invalid.
-
9 hours ago, V0idWa1k3r said:
If that is the reason for custom materials then you are doing it wrong, as there is Block::getMapColor.
Well, they're not water, so I wouldn't want them to be handled in that way. What other reasons would you use MaterialLiquid than to make it something other than water? I'm not trying to be belligerent or anything, I honestly want to know. Why would you want to set a fluid's material to water when it isn't water? Or, what other way is there to handle that without making its material water?
But thanks for the information about canFlowInto and flowIntoBlock, I'll look into those.
-
So I guess people need more information, so here's a video (gif) showing what I'm talking about:
This happens even if I don't have my own custom class and just use BlockFluidClassic for my fluids, so there's no custom code to worry about interfering. They are all of MaterialLiquid with varying map colors. But, even if I completely disable my custom blocks' onNeghborChange methods by not calling the superclass's method, it still does it, so it must be in water's code. And it doesn't check my liquid's stuff first, so it ends up doing this, if I have my interactions enabled:
And this was the result on the other side:
SpoilerWhen it should have done this:
SpoilerPlease tell me this isn't intended behavior...
-
So, most parts of my liquids are working properly now, thanks to my other thread, but that thread is long gone now, so no necro here.
Anyway, my new problem is that when I place my custom liquid (which is made with MaterialLiquid), when it flows into a water source block, it deletes it. This is default behavior, I commented out all references to onBlockAdded or onNeighborChanged. It must be behavior in the water block itself, which means I can't change it by normal means. This throws all of my intended interactions for a loop (when I have them enabled), causing any of my fluids' interactions with water to checkerboard (for example, when I have acid flow over water, it's supposed to turn the source blocks to clay. This interaction works for the first time a flow is calculated, but on the next one, the water source block gets deleted, causing the acid flow to go under the surface of the water, which then causes the next one down to be hardened, and then the next ones out from there horizontally get deleted, etc). Is this a problem with my liquid implementation that I can fix, or is this merely a known bug that cannot be remedied? I get the feeling that this is because of the instantaneous change that happens from a static block to a flowing block before it sees that the source that was turned to clay is a solid block, and my code (which only compares to Blocks.WATER) doesn't account for that change, and thus the water source interaction is allowed to happen, but I can't find out how to check for a flowing source block in 1.10.
-
You mean getting the block that a thrown item rests on?
-
1 minute ago, bradylox said:
okay wait im gonna do a bit of reaserch bc thats supposed to work
The method you were using was outdated a long time ago when we stopped worrying about block IDs.
-
Which version of MC are you modding for? At the very least, 1.10 and above (and probably 1.9, as well), would require you do something like this:
Block blockClicked = world.getBlockState(new BlockPos(x, y, z)).getBlock();
EDIT: On top of that, I would think that any method in Item that would give you the block clicked would give you the Block, or at least a BlockPos, not xyz coordinates.
-
1
-
-
Yes! That's it! Most excellent. The only thing left for me to figure out is how to prevent the default water-colored splash and make splash particles of my fluid's colors...
-
Yes, that almost could do it, except that there's no way to change how the water texture is rendered. I managed to cancel the event for rendering the water overlay, but I can't seem to get it to use my own fluid blocks to overlay. Even posting a new event with the OverlayType.BLOCK, with one of my other blocks, it still only cancels. It doesn't do another overlay. And there's no way to change the block used in the same event that was fired. And since I can't follow the code in the normal way, I don't know how to find out how it processes the overlay events to make my fluids compatible.
-
As far as I can tell, RenderGameOverlayEvent doesn't have anything on the color an overlay has from being in a liquid. If I'm looking at it wrong, then I need better documentation.
Thankfully, though, Block::isEntityInsideMaterial did most of what I was wanting (as far as interacting with the liquid, being pushed by flow, swimming instead of jumping, etc). It's unfortunate that going under the surface of the liquid can only turn the screen bluish for now, but it's not like I haven't been dealing with that since I started handling the mod anyway. Hopefully SOMETHING will crop up somewhere.
-
Well, thanks you to talking about the customMeshDefinition, I remembered the place where that was called, and realized that it was registering a new ItemBlock in the code, and was then trying to set the customMeshDefinition to a block model that didn't exist. Guess that's what I get for blindly following tutorials... But, I removed the ItemBlock registration and removed the one line trying to set the customMeshDefinition and everything's back to normal. I never wanted to have a liquid block available in the creative tabs anyway, I have other items for placing these things. Hopefully now the inventory variants in the blockstates will work (if I decide I even want them).
However, I've still had no answer about the water-like entity interactions for my fluids.
-
Cool, thanks for that info.
As a matter of fact, the blocks/ prefix being there or not had no impact on where the game looked to find the blockstate files, which was merely assets/plasmacraft/blockstates. If I moved them from there, the textures for the blocks didn't even load.
However, I tried just replacing the model for the inventory variant, I tried removing different portions of the inventory variant, and I tried removing the inventory variant altogether, and it still keeps failing (with that exact same error) and trying to find the item model json file separately (which, of course, I don't have). I can't find any info on what a fluid blockstate file is SUPPOSED to look like.
EDIT: Just realized how the blockstate files are found, and no, they were in the right place and being loaded. I just can't figure out how to get it to load the inventory variant properly.
-
Quite frankly, I'm surprised this hasn't been added as default behavior into Forge (being able to handle fluid interactions independent of it being water). I have 8 custom fluids I've added right now, but because they don't have Material.WATER (thus failing the Entity.isInWater() method), entities don't interact with them as liquids. Is there a way to keep custom map colors and such and still make entities interact with my fluids properly, or am I going to have to suck it up and keep them water? I'd like to easily be able to set the "underwater" fog color, too, to mach the liquid color.
Also, I'm having visual glitches like this gap between side textures:
I'm using BlockFluidClassic. Is this a problem with my fluid textures, or perhaps a model or is there something wrong with the renderer? Also, I have a blockstate JSON file, but I keep getting errors like this, too:Spoiler[11:00:22] [Client thread/ERROR] [FML]: Exception loading model for variant plasmacraft:blocks/fluid/plutonium#inventory for item "plasmacraft:blocks/fluid/plutonium", normal location exception: net.minecraftforge.client.model.ModelLoaderRegistry$LoaderException: Exception loading model plasmacraft:item/blocks/fluid/plutonium with loader VanillaLoader.INSTANCE, skipping at net.minecraftforge.client.model.ModelLoaderRegistry.getModel(ModelLoaderRegistry.java:153) ~[ModelLoaderRegistry.class:?] at net.minecraftforge.client.model.ModelLoader.loadItemModels(ModelLoader.java:317) ~[ModelLoader.class:?] at net.minecraft.client.renderer.block.model.ModelBakery.loadVariantItemModels(ModelBakery.java:170) ~[ModelBakery.class:?] at net.minecraftforge.client.model.ModelLoader.setupModelRegistry(ModelLoader.java:147) ~[ModelLoader.class:?] at net.minecraft.client.renderer.block.model.ModelManager.onResourceManagerReload(ModelManager.java:28) [ModelManager.class:?] at net.minecraft.client.resources.SimpleReloadableResourceManager.notifyReloadListeners(SimpleReloadableResourceManager.java:132) [SimpleReloadableResourceManager.class:?] at net.minecraft.client.resources.SimpleReloadableResourceManager.reloadResources(SimpleReloadableResourceManager.java:113) [SimpleReloadableResourceManager.class:?] at net.minecraft.client.Minecraft.refreshResources(Minecraft.java:799) [Minecraft.class:?] at net.minecraftforge.fml.client.FMLClientHandler.finishMinecraftLoading(FMLClientHandler.java:340) [FMLClientHandler.class:?] at net.minecraft.client.Minecraft.startGame(Minecraft.java:561) [Minecraft.class:?] at net.minecraft.client.Minecraft.run(Minecraft.java:386) [Minecraft.class:?] at net.minecraft.client.main.Main.main(Main.java:118) [Main.class:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_74] at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_74] at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_74] at java.lang.reflect.Method.invoke(Unknown Source) ~[?:1.8.0_74] at net.minecraft.launchwrapper.Launch.launch(Launch.java:135) [launchwrapper-1.12.jar:?] at net.minecraft.launchwrapper.Launch.main(Launch.java:28) [launchwrapper-1.12.jar:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_74] at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_74] at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) ~[?:1.8.0_74] at java.lang.reflect.Method.invoke(Unknown Source) ~[?:1.8.0_74] at net.minecraftforge.gradle.GradleStartCommon.launch(GradleStartCommon.java:97) [start/:?] at GradleStart.main(GradleStart.java:26) [start/:?] Caused by: java.io.FileNotFoundException: plasmacraft:models/item/blocks/fluid/plutonium.json at net.minecraft.client.resources.FallbackResourceManager.getResource(FallbackResourceManager.java:68) ~[FallbackResourceManager.class:?] at net.minecraft.client.resources.SimpleReloadableResourceManager.getResource(SimpleReloadableResourceManager.java:65) ~[SimpleReloadableResourceManager.class:?] at net.minecraft.client.renderer.block.model.ModelBakery.loadModel(ModelBakery.java:311) ~[ModelBakery.class:?] at net.minecraftforge.client.model.ModelLoader.access$1100(ModelLoader.java:118) ~[ModelLoader.class:?] at net.minecraftforge.client.model.ModelLoader$VanillaLoader.loadModel(ModelLoader.java:868) ~[ModelLoader$VanillaLoader.class:?] at net.minecraftforge.client.model.ModelLoaderRegistry.getModel(ModelLoaderRegistry.java:149) ~[ModelLoaderRegistry.class:?] ... 23 more
Does this have anything to do with it? I was under the impression that I would only need a blockstate, not a model, and I can't find anything on a water block model anyway. Perhaps something is wrong with my blockstate?
Spoiler{ "forge_marker": 1, "variants": { "fluid": { "model": "forge:fluid", "custom": { "fluid": "plutonium" } }, "inventory": { "model": "forge:forge_bucket", "textures": { "base": "forge:items/bucket_base", "fluid": "sw:items/bucket_fluid", "cover": "forge:items/bucket_cover" }, "transform": "forge:default-item", "custom": { "fluid": "plutonium", "flipGas": true } } } }
And, I don't plan on having buckets able to interact with these. Is all of that necessary if that's the case?
-
Gah, I didn't even think about checking the imports.... Thanks.
-
So, before anyone says anything, yes, I followed this tutorial:
However, when I set up the tabSorter, looking like this:
tabSorter = Ordering.explicit(order).onResultOf(new Function<ItemStack, Item>() {
I get this error:
The method onResultOf(Function<F,? extends Item>) in the type Ordering<Item> is not applicable for the arguments (new Function<ItemStack,Item>(){})
And I can't figure out why. I followed the tutorial exactly. I'm in Eclipse, and I think I have Java 1.6 as my compatibility and runtime environment, but I tried 1.7 and 1.8 and there wasn't a difference. Any ideas?
-
This can be done in the json model. You can write it manually, but it's easier to use MCMC. What you're looking for is Rotation, which can be found under Faces.
Yeah, there was another model creator that I use that I remembered having that, so I looked there. Needed to make a custom model, but yes, that is exactly what I was looking for.
-
But I want to keep the UV lock, I just also want to keep the organization, such as the four cardinal faces having the up and down textures, and then the top and bottom having linked textures. Basically, I want to be able to rotate the texture before or during application to a block's model without having to make a custom texture just to rotate it 90 degrees.
-
Say, for example, I wanted to make a block that looked exactly like the oak planks in Minecraft, except that the slats should go vertically instead of horizontally. Is there a way to accomplish this in model or blockstate files? All I've seen is the ability to rotate the block itself.
-
Indeed, thank you. Those were the only resources left that hadn't already been converted to all lower case.
-
So I've been trying to update a mod (not mine, but for private use) from 1.10.2 to 1.11, and I've managed to resolve all of the error-generating problems, but now I can't figure out why the mod's localization .lang files aren't being parsed and injected. This is happening both in Eclipse's debug and in an obfuscated runtime environment. I've debugged the entire process down to the point of seeing that the mod is added to the resource pack, but the lang files are never parsed by the LanguageMap. I've double checked the file and folder structure a dozen times at least, and it is proper ("assets/{MOD_ID}/lang" from the mod root) in both environments. In the FMLClientHandler class, under addModAsResource, resourcePackType is not null, and obviously the mod is getting added to the active list and the modNames table (the items and blocks show up in the creative tabs). Thus, when I load the game, I get tile.{MOD_ID}:{UL_NAME}.name for all of the tooltips over the blocks. And when I look in the lang file, it's an exact match to whatever block or item may be in question.
I am thoroughly lost on this one. Where can I look next to try to resolve this?
[1.10.2][Unsolved] Custom TNT Entity Not Rendering
in Modder Support
Posted
Second page bump. No one has anything?