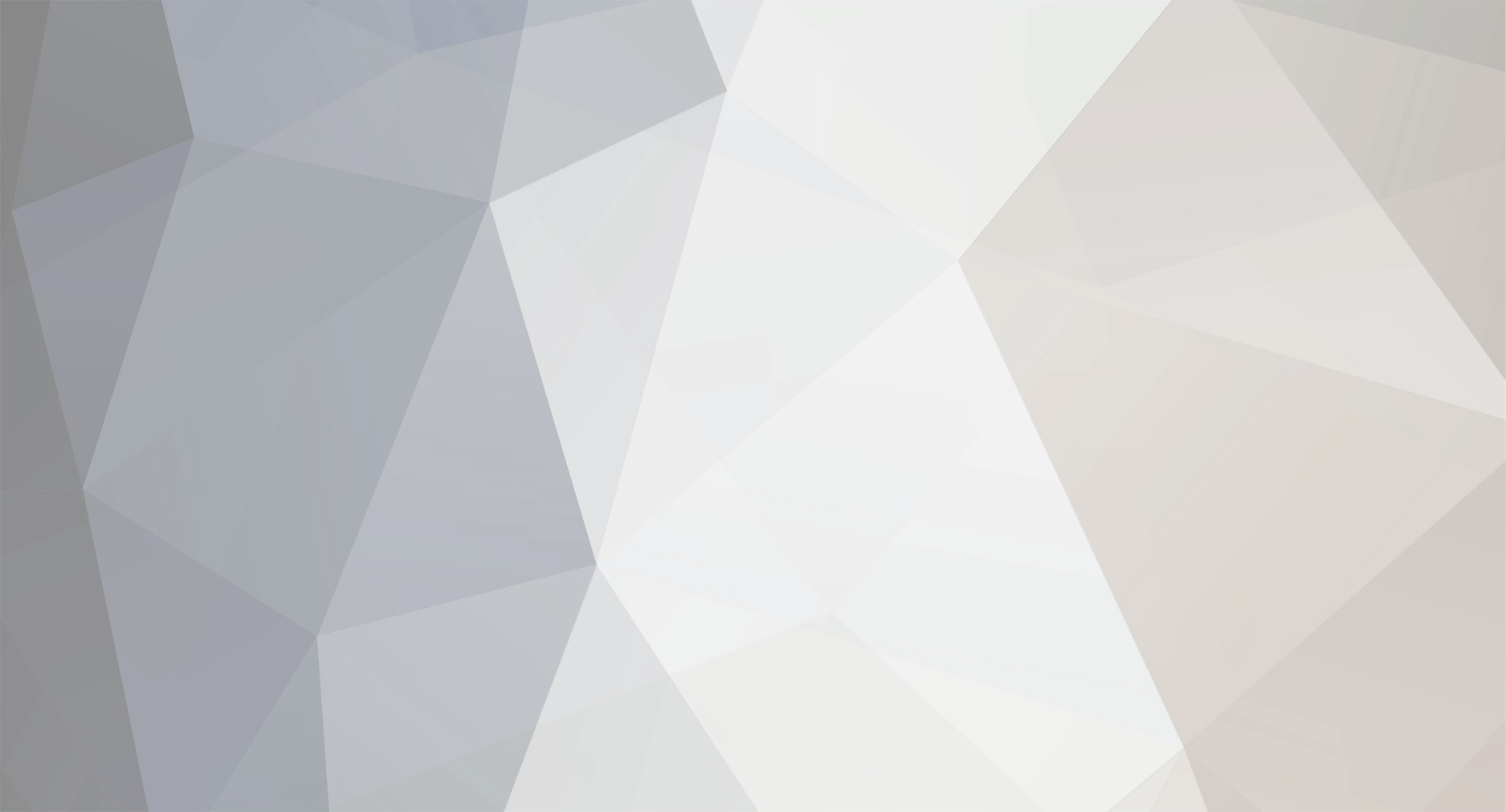
Malkierian
-
Posts
42 -
Joined
-
Last visited
Posts posted by Malkierian
-
-
So this is a problem I have been dealing with since at least 1.5.2, if not sooner, and I'm hoping the community has matured enough to be able to help out with this now (couldn't back then).
Anyway, I have a custom TNT block, acid TNT, that functions perfectly, and has for a long time. However, when it is activated, instead of getting the primed render texture on top of the acid TNT block texture, it becomes a white cube, and only looks like it's animated if I'm moving in the world, otherwise it's static. Here's the example:
This is before activating:
And after:
Here is all the relevant code:
BlockAcidTNT
package com.malkierian.plasmacraft.core.blocks;
import net.minecraft.block.Block;
import net.minecraft.block.BlockTNT;
import net.minecraft.client.renderer.texture.IIconRegister;
import net.minecraft.entity.EntityLivingBase;
import net.minecraft.entity.item.EntityTNTPrimed;
import net.minecraft.entity.player.EntityPlayer;
import net.minecraft.init.Items;
import net.minecraft.util.IIcon;
import net.minecraft.world.Explosion;
import net.minecraft.world.World;
import com.malkierian.plasmacraft.core.PlasmaCraft;
import com.malkierian.plasmacraft.core.entities.EntityAcidTNTPrimed;
import cpw.mods.fml.relauncher.Side;
import cpw.mods.fml.relauncher.SideOnly;
public class BlockAcidTNT extends BlockTNT
{
private IIcon topIcon;
private IIcon bottomIcon;
public BlockAcidTNT()
{
super();
setHardness(0.0F);
setStepSound(Block.soundTypeGrass);
setCreativeTab(PlasmaCraft.plasmaTab);
}
@Override
public IIcon getIcon(int i, int j)
{
if(i == 0)
{
return bottomIcon;
}
if(i == 1)
{
return topIcon;
} else
{
return blockIcon;
}
}
@Override
public void onBlockDestroyedByExplosion(World p_149723_1_, int p_149723_2_, int p_149723_3_, int p_149723_4_, Explosion p_149723_5_)
{
if (!p_149723_1_.isRemote)
{
EntityAcidTNTPrimed entitytntprimed = new EntityAcidTNTPrimed(p_149723_1_, (double)((float)p_149723_2_ + 0.5F), (double)((float)p_149723_3_ + 0.5F), (double)((float)p_149723_4_ + 0.5F), p_149723_5_.getExplosivePlacedBy());
entitytntprimed.fuse = p_149723_1_.rand.nextInt(entitytntprimed.fuse / 4) + entitytntprimed.fuse / 8;
p_149723_1_.spawnEntityInWorld(entitytntprimed);
}
}
/**
* Called right before the block is destroyed by a player. Args: world, x, y, z, metaData
*/
public void onBlockDestroyedByPlayer(World p_149664_1_, int p_149664_2_, int p_149664_3_, int p_149664_4_, int p_149664_5_)
{
this.func_150114_a(p_149664_1_, p_149664_2_, p_149664_3_, p_149664_4_, p_149664_5_, (EntityLivingBase)null);
}
public void func_150114_a(World p_150114_1_, int p_150114_2_, int p_150114_3_, int p_150114_4_, int p_150114_5_, EntityLivingBase p_150114_6_)
{
if (!p_150114_1_.isRemote)
{
if ((p_150114_5_ & 1) == 1)
{
EntityAcidTNTPrimed entitytntprimed = new EntityAcidTNTPrimed(p_150114_1_, (double)((float)p_150114_2_ + 0.5F), (double)((float)p_150114_3_ + 0.5F), (double)((float)p_150114_4_ + 0.5F), p_150114_6_);
p_150114_1_.spawnEntityInWorld(entitytntprimed);
p_150114_1_.playSoundAtEntity(entitytntprimed, "game.tnt.primed", 1.0F, 1.0F);
}
}
}
/**
* Called upon block activation (right click on the block.)
*/
public boolean onBlockActivated(World p_149727_1_, int p_149727_2_, int p_149727_3_, int p_149727_4_, EntityPlayer p_149727_5_, int p_149727_6_, float p_149727_7_, float p_149727_8_, float p_149727_9_)
{
if (p_149727_5_.getCurrentEquippedItem() != null && p_149727_5_.getCurrentEquippedItem().getItem() == Items.flint_and_steel)
{
this.func_150114_a(p_149727_1_, p_149727_2_, p_149727_3_, p_149727_4_, 1, p_149727_5_);
p_149727_1_.setBlockToAir(p_149727_2_, p_149727_3_, p_149727_4_);
p_149727_5_.getCurrentEquippedItem().damageItem(1, p_149727_5_);
return true;
}
else
{
return super.onBlockActivated(p_149727_1_, p_149727_2_, p_149727_3_, p_149727_4_, p_149727_5_, p_149727_6_, p_149727_7_, p_149727_8_, p_149727_9_);
}
}
@Override
@SideOnly(Side.CLIENT)
public void registerBlockIcons(IIconRegister par1IconRegister)
{
blockIcon = par1IconRegister.registerIcon("plasmacraft:acidTnt");
topIcon = par1IconRegister.registerIcon("plasmacraft:acidTnt_top");
bottomIcon = par1IconRegister.registerIcon("plasmacraft:acidTnt_bottom");
}
}
EntityAcidTNTPrimed
package com.malkierian.plasmacraft.core.entities;
import net.minecraft.entity.Entity;
import net.minecraft.entity.EntityLivingBase;
import net.minecraft.nbt.NBTTagCompound;
import net.minecraft.world.World;
import com.malkierian.plasmacraft.core.AcidExplosion;
public class EntityAcidTNTPrimed extends Entity
{
public int fuse;
private EntityLivingBase tntPlacedBy;
public EntityAcidTNTPrimed(World world)
{
super(world);
fuse = 80;
preventEntitySpawning = true;
setSize(0.98F, 0.98F);
yOffset = height / 2.0F;
}
public EntityAcidTNTPrimed(World p_i1730_1_, double p_i1730_2_, double p_i1730_4_, double p_i1730_6_, EntityLivingBase p_i1730_8_)
{
this(p_i1730_1_);
this.setPosition(p_i1730_2_, p_i1730_4_, p_i1730_6_);
float f = (float)(Math.random() * Math.PI * 2.0D);
this.motionX = (double)(-((float)Math.sin((double)f)) * 0.02F);
this.motionY = 0.20000000298023224D;
this.motionZ = (double)(-((float)Math.cos((double)f)) * 0.02F);
this.fuse = 80;
this.prevPosX = p_i1730_2_;
this.prevPosY = p_i1730_4_;
this.prevPosZ = p_i1730_6_;
this.tntPlacedBy = p_i1730_8_;
}
protected void entityInit()
{
}
@Override
public boolean canBeCollidedWith()
{
return !isDead;
}
@Override
public void onUpdate()
{
prevPosX = posX;
prevPosY = posY;
prevPosZ = posZ;
motionY -= 0.039999999105930328D;
moveEntity(motionX, motionY, motionZ);
motionX *= 0.98000001907348633D;
motionY *= 0.98000001907348633D;
motionZ *= 0.98000001907348633D;
if(onGround)
{
motionX *= 0.69999998807907104D;
motionZ *= 0.69999998807907104D;
motionY *= -0.5D;
}
if (!this.worldObj.isRemote)
{
if(fuse-- <= 0)
{
setDead();
explode();
}
}
else
{
worldObj.spawnParticle("smoke", posX, posY + 0.5D, posZ, 0.0D, 0.0D, 0.0D);
}
}
private void explode()
{
float f = 10F;
AcidExplosion smacidexplosion = new AcidExplosion(worldObj, null, posX, posY, posZ, f);
smacidexplosion.isFlaming = false;
smacidexplosion.doExplosionA();
smacidexplosion.doExplosionB();
}
protected void writeEntityToNBT(NBTTagCompound nbttagcompound)
{
nbttagcompound.setInteger("Fuse", fuse);
}
protected void readEntityFromNBT(NBTTagCompound nbttagcompound)
{
fuse = nbttagcompound.getInteger("Fuse");
}
public float getShadowSize()
{
return 0.0F;
}
public EntityLivingBase getTntPlacedBy()
{
return this.tntPlacedBy;
}
}
RenderAcidTNTPrimed
package com.malkierian.plasmacraft.client.renderers;
import net.minecraft.client.renderer.RenderBlocks;
import net.minecraft.client.renderer.entity.Render;
import net.minecraft.entity.Entity;
import net.minecraft.util.ResourceLocation;
import org.lwjgl.opengl.GL11;
import com.malkierian.plasmacraft.core.PlasmaCraft;
import com.malkierian.plasmacraft.core.entities.EntityAcidTNTPrimed;
import cpw.mods.fml.relauncher.Side;
import cpw.mods.fml.relauncher.SideOnly;
@SideOnly(Side.CLIENT)
public class RenderAcidTNTPrimed extends Render
{
private static final ResourceLocation TEXTURE = new ResourceLocation(PlasmaCraft.MOD_ID, "textures/blocks/acidTnt.png");
private RenderBlocks blockRenderer = new RenderBlocks();
public RenderAcidTNTPrimed()
{
this.shadowSize = 0.5F;
}
public void doRender(EntityAcidTNTPrimed p_76986_1_, double p_76986_2_, double p_76986_4_, double p_76986_6_, float p_76986_8_, float p_76986_9_, int bs)
{
GL11.glPushMatrix();
GL11.glTranslatef((float)p_76986_2_, (float)p_76986_4_, (float)p_76986_6_);
float f2;
if ((float)p_76986_1_.fuse - p_76986_9_ + 1.0F < 10.0F)
{
f2 = 1.0F - ((float)p_76986_1_.fuse - p_76986_9_ + 1.0F) / 10.0F;
if (f2 < 0.0F)
{
f2 = 0.0F;
}
if (f2 > 1.0F)
{
f2 = 1.0F;
}
f2 *= f2;
f2 *= f2;
float f3 = 1.0F + f2 * 0.3F;
GL11.glScalef(f3, f3, f3);
}
f2 = (1.0F - ((float)p_76986_1_.fuse - p_76986_9_ + 1.0F) / 100.0F) * 0.8F;
this.bindEntityTexture(p_76986_1_);
this.blockRenderer.renderBlockAsItem(PlasmaCraft.acidTnt, 0, p_76986_1_.getBrightness(p_76986_9_));
if (p_76986_1_.fuse / 5 % 2 == 0)
{
GL11.glDisable(GL11.GL_TEXTURE_2D);
GL11.glDisable(GL11.GL_LIGHTING);
GL11.glEnable(GL11.GL_BLEND);
GL11.glBlendFunc(GL11.GL_SRC_ALPHA, GL11.GL_DST_ALPHA);
GL11.glColor4f(1.0F, 1.0F, 1.0F, f2);
this.blockRenderer.renderBlockAsItem(PlasmaCraft.acidTnt, 0, 1.0F);
GL11.glColor4f(1.0F, 1.0F, 1.0F, 1.0F);
GL11.glDisable(GL11.GL_BLEND);
GL11.glEnable(GL11.GL_LIGHTING);
GL11.glEnable(GL11.GL_TEXTURE_2D);
}
GL11.glPopMatrix();
}
protected ResourceLocation getEntityTexture(EntityAcidTNTPrimed p_110775_1_)
{
return TEXTURE;
}
protected ResourceLocation getEntityTexture(Entity p_110775_1_)
{
return this.getEntityTexture((EntityAcidTNTPrimed)p_110775_1_);
}
public void doRender(Entity p_76986_1_, double p_76986_2_, double p_76986_4_, double p_76986_6_, float p_76986_8_, float p_76986_9_)
{
this.doRender((EntityAcidTNTPrimed)p_76986_1_, p_76986_2_, p_76986_4_, p_76986_6_, p_76986_8_, p_76986_9_, 0);
}
}
Main mod file
package com.malkierian.plasmacraft.core;
import java.io.File;
import java.util.Arrays;
import java.util.Comparator;
import java.util.List;
import net.minecraft.block.Block;
import net.minecraft.block.material.Material;
import net.minecraft.client.Minecraft;
import net.minecraft.init.Blocks;
import net.minecraft.init.Items;
import net.minecraft.item.Item;
import net.minecraft.item.ItemArmor.ArmorMaterial;
import net.minecraft.item.ItemStack;
import net.minecraftforge.common.config.Configuration;
import net.minecraftforge.fluids.Fluid;
import net.minecraftforge.fluids.FluidRegistry;
import net.minecraftforge.oredict.OreDictionary;
import com.google.common.base.Function;
import com.google.common.collect.Ordering;
import com.malkierian.plasmacraft.client.gui.GuiHandler;
import com.malkierian.plasmacraft.client.gui.PlasmaTab;
import com.malkierian.plasmacraft.core.blocks.BlockAcidBarrier;
import com.malkierian.plasmacraft.core.blocks.BlockAcidTNT;
import com.malkierian.plasmacraft.core.blocks.BlockCausticFluids;
import com.malkierian.plasmacraft.core.blocks.BlockGlowCloth;
import com.malkierian.plasmacraft.core.blocks.BlockPlasmaBench;
import com.malkierian.plasmacraft.core.blocks.BlockPlasmaOre;
import com.malkierian.plasmacraft.core.blocks.BlockReinforcedGlass;
import com.malkierian.plasmacraft.core.entities.EntityAcid;
import com.malkierian.plasmacraft.core.entities.EntityAcidGrenade;
import com.malkierian.plasmacraft.core.entities.EntityAcidTNTPrimed;
import com.malkierian.plasmacraft.core.entities.EntityCausticBoat;
import com.malkierian.plasmacraft.core.entities.EntityCryoBlast;
import com.malkierian.plasmacraft.core.entities.EntityLaser;
import com.malkierian.plasmacraft.core.entities.EntityLaserShotgun;
import com.malkierian.plasmacraft.core.entities.EntityPlasma;
import com.malkierian.plasmacraft.core.entities.EntityRailGun;
import com.malkierian.plasmacraft.core.items.ItemAcidGrenade;
import com.malkierian.plasmacraft.core.items.ItemCausticBoat;
import com.malkierian.plasmacraft.core.items.ItemEnergyWeapon;
import com.malkierian.plasmacraft.core.items.ItemPlasma;
import com.malkierian.plasmacraft.core.items.ItemPlasmaArmor;
import com.malkierian.plasmacraft.core.items.ItemVial;
import com.malkierian.plasmacraft.core.worldgen.WorldGenerator;
import cpw.mods.fml.common.FMLCommonHandler;
import cpw.mods.fml.common.Mod;
import cpw.mods.fml.common.Mod.EventHandler;
import cpw.mods.fml.common.Mod.Instance;
import cpw.mods.fml.common.SidedProxy;
import cpw.mods.fml.common.event.FMLInitializationEvent;
import cpw.mods.fml.common.event.FMLPostInitializationEvent;
import cpw.mods.fml.common.event.FMLPreInitializationEvent;
import cpw.mods.fml.common.network.NetworkRegistry;
import cpw.mods.fml.common.registry.EntityRegistry;
import cpw.mods.fml.common.registry.GameRegistry;
import cpw.mods.fml.relauncher.Side;
@Mod(modid = "plasmacraft")
public class PlasmaCraft
{
public static String MOD_ID = "plasmacraft";
public static PlasmaTab plasmaTab;
public static Block acidBarrier;
public static Block orePlasma;
public static Block oreLeadBlock;
public static Block glowCloth;
public static Block plasmaBench;
public static int causticID = 180;
public static BlockCausticFluids acidBlock;
public static BlockCausticFluids cryoniteBlock;
public static BlockCausticFluids neptuniumBlock;
public static BlockCausticFluids netherflowBlock;
public static BlockCausticFluids obsidiumBlock;
public static BlockCausticFluids plutoniumBlock;
public static BlockCausticFluids radioniteBlock;
public static BlockCausticFluids uraniumBlock;
public static Fluid acidFluid;
public static Fluid cryoniteFluid;
public static Fluid neptuniumFluid;
public static Fluid netherflowFluid;
public static Fluid obsidiumFluid;
public static Fluid plutoniumFluid;
public static Fluid radioniteFluid;
public static Fluid uraniumFluid;
public static Block acidTnt;
public static Block frozenCryonite;
public static Block reinforcedGlass;
public static Item goopAcid;
public static Item goopCryonite;
public static Item goopNeptunium;
public static Item goopNetherflow;
public static Item goopObsidium;
public static Item goopPlutonium;
public static Item goopRadionite;
public static Item goopUranium;
public static Item plasma;
public static Item acidGrenade;
public static Item causticBoat;
public static Item thermopellet;
public static Item ingotCryonite;
public static Item ingotLead;
public static Item ingotNeptunium;
public static Item ingotNetherflow;
public static Item ingotObsidium;
public static Item ingotPlutonium;
public static Item ingotRadionite;
public static Item ingotUranium;
public static Item hazmatBoots;
public static Item hazmatHood;
public static Item hazmatJacket;
public static Item hazmatPants;
public static Item plasmaLeather;
public static Item acidVial;
public static Item causticVial;
public static Item cryoniteVial;
public static Item neptuniumVial;
public static Item netherflowVial;
public static Item obsidiumVial;
public static Item plutoniumVial;
public static Item radioniteVial;
public static Item uraniumVial;
public static Item acidgun;
public static Item batteryCharged;
public static Item batteryCryo;
public static Item batteryEmpty;
public static Item batteryOvercharged;
public static Item batteryPlasma;
public static Item beamSplitter;
public static Item cryoblaster;
public static Item energyCell;
public static Item lasergun;
public static Item lasergunsplit;
public static Item lasershotgun;
public static Item plasmagun;
public static Item plasmagunsplit;
public static Item railgun;
public static final int plutoniumMeta = 0;
public static final int radioniteMeta = 1;
public static final int neptuniumMeta = 2;
public static final int obsidiumMeta = 3;
public static final int uraniumMeta = 4;
public static final int leadMeta = 5;
public static boolean liquidSourceExplodesAfterCausticExplosion;
public static int acidLakeYCutoff = 48;
public static int acidSpoutCount = 20;
public static int acidSpoutYRange = 30;
public static int acidSpoutYStart = 8;
public static int leadOreVeinCount = 6;
public static int leadOreVeinSize = 6;
public static int leadOreYRange = 80;
public static int leadOreYStart = 4;
public static int neptuniumOreVeinCount = 6;
public static int neptuniumOreVeinSize = 10;
public static int neptuniumOreYRange = 64;
public static int neptuniumOreYStart = 32;
public static int neptuniumSpoutCount = 20;
public static int neptuniumSpoutYRange = 64;
public static int neptuniumSpoutYStart = 8;
public static int netherflowLakeChance = 32;
public static int netherflowLakeYCutoff = 96;
public static int netherflowLakeYRange = 16;
public static int netherflowLakeYStart = 56;
public static int netherflowSpoutCount = 20;
public static int netherflowSpoutYRange = 96;
public static int netherflowSpoutYStart = 16;
public static int obsidiumOreVeinCount = 4;
public static int obsidiumOreVeinSize = 10;
public static int obsidiumOreYRange = 64;
public static int obsidiumOreYStart = 32;
public static int plutoniumOreVeinCount = 4;
public static int plutoniumOreVeinSize = 6;
public static int plutoniumOreYRange = 16;
public static int plutoniumOreYStart = 4;
public static int radioniteOreVeinCount = 4;
public static int radioniteOreVeinSize = 6;
public static int radioniteOreYRange = 24;
public static int radioniteOreYStart = 4;
public static int uraniumOreVeinCount = 4;
public static int uraniumOreVeinSize = 6;
public static int uraniumOreYRange = 16;
public static int uraniumOreYStart = 4;
public static final int glowClothAcidMeta = 0;
public static final int glowClothRadioniteMeta = 1;
public static final int glowClothNetherflowMeta = 2;
public static final int glowClothNeptuniumMeta = 3;
public static final int glowClothUraniumMeta = 4;
public static final int glowClothPlutoniumMeta = 5;
public static final int glowClothCryoniteMeta = 6;
public static final int glowClothObsidiumMeta = 7;
public static boolean generateLead;
public static boolean generateUranium;
public static boolean generatePlutonium;
public static int plasmaBenchFrontAnim;
public static Comparator<ItemStack> tabSorter;
// The instance of your mod that Forge uses.
@Instance("PlasmaCraft")
public static PlasmaCraft instance;
private GuiHandler guiHandler = new GuiHandler();
// Says where the client and server 'proxy' code is loaded.
@SidedProxy(clientSide="com.malkierian.plasmacraft.client.ClientProxy", serverSide="com.malkierian.plasmacraft.common.CommonProxy")
public static CommonProxy proxy;
@EventHandler
public void preInit(FMLPreInitializationEvent event)
{
instance = this;
}
@EventHandler
public void load(FMLInitializationEvent event)
{
loadConfig();
NetworkRegistry.INSTANCE.registerGuiHandler(this, guiHandler);
proxy.registerRenderers();
// MinecraftForge.EVENT_BUS.register(new PCBucketFillEvent());
plasmaTab = new PlasmaTab("PlasmaCraft");
registerBlocks();
registerFuel();
registerItems();
List<Item> order = Arrays.asList(Item.getItemFromBlock(orePlasma), Item.getItemFromBlock(glowCloth), Item.getItemFromBlock(frozenCryonite), Item.getItemFromBlock(reinforcedGlass),
Item.getItemFromBlock(acidTnt), Item.getItemFromBlock(acidBarrier), Item.getItemFromBlock(plasmaBench),
goopAcid, goopCryonite, goopNeptunium, goopNetherflow, goopObsidium, goopPlutonium, goopRadionite, goopUranium,
ingotCryonite, ingotLead, ingotNeptunium, ingotNetherflow, ingotObsidium, ingotPlutonium, ingotRadionite, ingotUranium, plasma,
acidVial, causticVial, cryoniteVial, neptuniumVial, netherflowVial, obsidiumVial, plutoniumVial, radioniteVial, uraniumVial,
causticBoat,
batteryEmpty, batteryCryo, batteryCharged, batteryOvercharged, batteryPlasma, beamSplitter, energyCell, thermopellet,
acidgun, cryoblaster, lasershotgun, lasergun, lasergunsplit, plasmagun, plasmagunsplit, railgun,
acidGrenade,
hazmatBoots, hazmatHood, hazmatJacket, hazmatPants,
plasmaLeather);
tabSorter = Ordering.explicit(order).onResultOf(new Function<ItemStack, Item>(){
@Override
public Item apply(ItemStack input)
{
return input.getItem();
}
});
registerRecipes();
registerOres();
registerTileEntities();
proxy.registerTextureFX();
registerEntities();
GameRegistry.registerWorldGenerator(new WorldGenerator(), 20);
}
@EventHandler
public void postInit(FMLPostInitializationEvent event)
{
// Stub Method
}
private void registerBlocks()
{
orePlasma = new BlockPlasmaOre().setLightLevel(0.5334f).setBlockName("orePlasma");
GameRegistry.registerBlock(orePlasma, com.malkierian.plasmacraft.core.items.ItemPlasmaOre.class, "orePlasma");
acidFluid = new Fluid("acid").setDensity(80).setViscosity(400);
cryoniteFluid = new Fluid("cryonite").setDensity(80).setViscosity(600);
neptuniumFluid = new Fluid("neptunium").setDensity(80).setViscosity(300);
netherflowFluid = new Fluid("netherflow").setDensity(80).setViscosity(450);
obsidiumFluid = new Fluid("obsidium").setDensity(80).setViscosity(1200);
plutoniumFluid = new Fluid("plutonium").setDensity(80).setViscosity(800);
radioniteFluid = new Fluid("radionite").setDensity(80).setViscosity(1000);
uraniumFluid = new Fluid("uranium").setDensity(150).setViscosity(800);
FluidRegistry.registerFluid(acidFluid);
FluidRegistry.registerFluid(cryoniteFluid);
FluidRegistry.registerFluid(neptuniumFluid);
FluidRegistry.registerFluid(netherflowFluid);
FluidRegistry.registerFluid(obsidiumFluid);
FluidRegistry.registerFluid(plutoniumFluid);
FluidRegistry.registerFluid(radioniteFluid);
FluidRegistry.registerFluid(uraniumFluid);
acidBlock = (BlockCausticFluids) new BlockCausticFluids(acidFluid, Material.water).setBlockName("acid");
cryoniteBlock = (BlockCausticFluids) new BlockCausticFluids(cryoniteFluid, Material.water).setBlockName("cryonite");
neptuniumBlock = (BlockCausticFluids) new BlockCausticFluids(neptuniumFluid, Material.water).setBlockName("neptunium");
netherflowBlock = (BlockCausticFluids) new BlockCausticFluids(netherflowFluid, Material.water).setBlockName("netherflow");
obsidiumBlock = (BlockCausticFluids) new BlockCausticFluids(obsidiumFluid, Material.water).setBlockName("obsidium");
plutoniumBlock = (BlockCausticFluids) new BlockCausticFluids(plutoniumFluid, Material.water).setBlockName("plutonium");
radioniteBlock = (BlockCausticFluids) new BlockCausticFluids(radioniteFluid, Material.water).setBlockName("radionite");
uraniumBlock = (BlockCausticFluids) new BlockCausticFluids(uraniumFluid, Material.water).setBlockName("uranium");
GameRegistry.registerBlock(acidBlock, "Acid");
GameRegistry.registerBlock(cryoniteBlock, "Cryonite");
GameRegistry.registerBlock(neptuniumBlock, "Neptunium");
GameRegistry.registerBlock(netherflowBlock, "Netherflow");
GameRegistry.registerBlock(obsidiumBlock, "Obsidium");
GameRegistry.registerBlock(plutoniumBlock, "Plutonium");
GameRegistry.registerBlock(radioniteBlock, "Radionite");
GameRegistry.registerBlock(uraniumBlock, "Uranium");
glowCloth = new BlockGlowCloth().setBlockName("glowCloth");
GameRegistry.registerBlock(glowCloth, com.malkierian.plasmacraft.core.items.ItemGlowCloth.class, "glowCloth");
frozenCryonite = (new BlockReinforcedGlass("frozenCryonite", Material.glass, false, 1.0F)).setBlockName("frozenCryonite");
reinforcedGlass = (new BlockReinforcedGlass("reinforcedGlass", Material.glass, false, 500.0F)).setBlockName("reinforcedGlass");
GameRegistry.registerBlock(frozenCryonite, "Frozen Cryonite");
GameRegistry.registerBlock(reinforcedGlass, "Reinforced Glass");
plasmaBench = (new BlockPlasmaBench()).setBlockName("plasmaBench");
GameRegistry.registerBlock(plasmaBench, "Plasmificator");
acidBarrier = (new BlockAcidBarrier()).setBlockName("acidBarrier");
GameRegistry.registerBlock(acidBarrier, "Acid Barrier");
acidTnt = (new BlockAcidTNT()).setBlockName("acidTnt");
GameRegistry.registerBlock(acidTnt, "Acid TNT");
}
private void registerEntities()
{
EntityRegistry.registerGlobalEntityID(EntityCausticBoat.class, "causticBoat", EntityRegistry.findGlobalUniqueEntityId());
EntityRegistry.registerModEntity(EntityCausticBoat.class, "causticBoat", 0, this, 128, 1, true);
EntityRegistry.registerGlobalEntityID(EntityAcidTNTPrimed.class, "acidTntPrimed", EntityRegistry.findGlobalUniqueEntityId());
EntityRegistry.registerModEntity(EntityAcidTNTPrimed.class, "acidTntPrimed", 1, this, 128, 1, false);
EntityRegistry.registerGlobalEntityID(EntityAcidGrenade.class, "acidGrenade", EntityRegistry.findGlobalUniqueEntityId());
EntityRegistry.registerModEntity(EntityAcidGrenade.class, "acidGrenade", 2, this, 128, 1, true);
EntityRegistry.registerGlobalEntityID(EntityLaser.class, "laser", EntityRegistry.findGlobalUniqueEntityId());
EntityRegistry.registerModEntity(EntityLaser.class, "laser", 3, this, 128, 1, true);
EntityRegistry.registerGlobalEntityID(EntityLaserShotgun.class, "laserShotgun", EntityRegistry.findGlobalUniqueEntityId());
EntityRegistry.registerModEntity(EntityLaserShotgun.class, "laserShotgun", 4, this, 128, 1, true);
EntityRegistry.registerGlobalEntityID(EntityPlasma.class, "plasma", EntityRegistry.findGlobalUniqueEntityId());
EntityRegistry.registerModEntity(EntityPlasma.class, "plasma", 5, this, 128, 1, true);
EntityRegistry.registerGlobalEntityID(EntityRailGun.class, "railGun", EntityRegistry.findGlobalUniqueEntityId());
EntityRegistry.registerModEntity(EntityRailGun.class, "railGun", 6, this, 128, 1, true);
EntityRegistry.registerGlobalEntityID(EntityAcid.class, "acid", EntityRegistry.findGlobalUniqueEntityId());
EntityRegistry.registerModEntity(EntityAcid.class, "acid", 7, this, 128, 1, true);
EntityRegistry.registerGlobalEntityID(EntityCryoBlast.class, "cryoBlast", EntityRegistry.findGlobalUniqueEntityId());
EntityRegistry.registerModEntity(EntityCryoBlast.class, "cryoBlast", 8, this, 128, 1, true);
// EntityRegistry.findGlobalUniqueEntityId() = EntityRegistry.findGlobalUniqueEntityId();
// MinecraftForge.registerEntity(EntityMutantCow.class, this, 170, 32, 100, true);
}
private void registerFuel()
{
GameRegistry.registerFuelHandler(new FuelHandler());
}
private void registerItems()
{
goopAcid = (new ItemPlasma()).setUnlocalizedName("goopAcid");
goopCryonite = (new ItemPlasma()).setUnlocalizedName("goopCryonite");
goopNeptunium = (new ItemPlasma()).setUnlocalizedName("goopNeptunium");
goopNetherflow = (new ItemPlasma()).setUnlocalizedName("goopNetherflow");
goopObsidium = (new ItemPlasma()).setUnlocalizedName("goopObsidium");
goopPlutonium = (new ItemPlasma()).setUnlocalizedName("goopPlutonium");
goopRadionite = (new ItemPlasma()).setUnlocalizedName("goopRadionite");
goopUranium = (new ItemPlasma()).setUnlocalizedName("goopUranium");
ingotCryonite = (new ItemPlasma()).setUnlocalizedName("ingotCryonite");
ingotLead = (new ItemPlasma()).setUnlocalizedName("ingotLead");
ingotNeptunium = (new ItemPlasma()).setUnlocalizedName("ingotNeptunium");
ingotNetherflow = (new ItemPlasma()).setUnlocalizedName("ingotNetherflow");
ingotObsidium = (new ItemPlasma()).setUnlocalizedName("ingotObsidium");
ingotPlutonium = (new ItemPlasma()).setUnlocalizedName("ingotPlutonium");
ingotRadionite = (new ItemPlasma()).setUnlocalizedName("ingotRadionite");
ingotUranium = (new ItemPlasma()).setUnlocalizedName("ingotUranium");
plasma = (new ItemPlasma()).setUnlocalizedName("plasma");
acidVial = (new ItemVial(acidBlock)).setUnlocalizedName("vial_acid");
causticVial = (new ItemVial(Blocks.air)).setUnlocalizedName("vial_empty");
cryoniteVial = (new ItemVial(cryoniteBlock)).setUnlocalizedName("vial_cryonite");
neptuniumVial = (new ItemVial(neptuniumBlock)).setUnlocalizedName("vial_neptunium");
netherflowVial = (new ItemVial(netherflowBlock)).setUnlocalizedName("vial_netherflow");
obsidiumVial = (new ItemVial(obsidiumBlock)).setUnlocalizedName("vial_obsidium");
plutoniumVial = (new ItemVial(plutoniumBlock)).setUnlocalizedName("vial_plutonium");
radioniteVial = (new ItemVial(radioniteBlock)).setUnlocalizedName("vial_radionite");
uraniumVial = (new ItemVial(uraniumBlock)).setUnlocalizedName("vial_uranium");
causticBoat = (new ItemCausticBoat()).setUnlocalizedName("causticBoat");
batteryEmpty = (new ItemPlasma()).setUnlocalizedName("batteryEmpty");
batteryCryo = (new ItemPlasma()).setUnlocalizedName("batteryCryonite");
batteryCharged = (new ItemPlasma()).setUnlocalizedName("batteryCharged");
batteryOvercharged = (new ItemPlasma()).setUnlocalizedName("batteryOvercharged");
batteryPlasma = (new ItemPlasma()).setUnlocalizedName("batteryPlasma");
beamSplitter = (new ItemPlasma()).setUnlocalizedName("beamSplitter");
energyCell = (new ItemPlasma()).setUnlocalizedName("energyCell");
thermopellet = (new ItemPlasma()).setUnlocalizedName("thermopellet");
acidgun = (new ItemEnergyWeapon(200)).setUnlocalizedName("acidGun");
cryoblaster = (new ItemEnergyWeapon(100)).setUnlocalizedName("cryoBlaster");
lasershotgun = (new ItemEnergyWeapon(200)).setUnlocalizedName("laserShotgun");
lasergun = (new ItemEnergyWeapon(200)).setUnlocalizedName("laserGun");
lasergunsplit = (new ItemEnergyWeapon(300)).setUnlocalizedName("laserGunSplit");
plasmagun = (new ItemEnergyWeapon(200)).setUnlocalizedName("plasmaGun");
plasmagunsplit = (new ItemEnergyWeapon(300)).setUnlocalizedName("plasmaGunSplit");
railgun = (new ItemEnergyWeapon(200)).setUnlocalizedName("railGun");
acidGrenade = new ItemAcidGrenade().setUnlocalizedName("acidGrenade");
hazmatBoots = (new ItemPlasmaArmor(ArmorMaterial.GOLD, proxy.addArmor("hazmat"), 3)).setUnlocalizedName("hazmatBoots");
hazmatHood = (new ItemPlasmaArmor(ArmorMaterial.GOLD, proxy.addArmor("hazmat"), 0)).setUnlocalizedName("hazmatHood");
hazmatJacket = (new ItemPlasmaArmor(ArmorMaterial.GOLD, proxy.addArmor("hazmat"), 1)).setUnlocalizedName("hazmatJacket");
hazmatPants = (new ItemPlasmaArmor(ArmorMaterial.GOLD, proxy.addArmor("hazmat"), 2)).setUnlocalizedName("hazmatPants");
plasmaLeather = (new ItemPlasma()).setUnlocalizedName("plasmaLeather");
GameRegistry.registerItem(goopAcid, "Acid Goop");
GameRegistry.registerItem(goopCryonite, "Cryonite Goop");
GameRegistry.registerItem(goopNeptunium, "Neptunium Goop");
GameRegistry.registerItem(goopNetherflow, "Netherflow Goop");
GameRegistry.registerItem(goopObsidium, "Obsidium Goop");
GameRegistry.registerItem(goopPlutonium, "Plutonium Goop");
GameRegistry.registerItem(goopRadionite, "Radionite Goop");
GameRegistry.registerItem(goopUranium, "Uranium Goop");
GameRegistry.registerItem(ingotCryonite, "Cryonite Ingot");
GameRegistry.registerItem(ingotLead, "Lead Ingot");
GameRegistry.registerItem(ingotNeptunium, "Neptunium Ingot");
GameRegistry.registerItem(ingotNetherflow, "Netherflow Ingot");
GameRegistry.registerItem(ingotObsidium, "Obsidium Ingot");
GameRegistry.registerItem(ingotPlutonium, "Plutonium Ingot");
GameRegistry.registerItem(ingotRadionite, "Radionite Ingot");
GameRegistry.registerItem(ingotUranium, "Uranium Ingot");
GameRegistry.registerItem(plasma, "Plasma");
GameRegistry.registerItem(acidVial, "Acid Vial");
GameRegistry.registerItem(causticVial, "Caustic Vial");
GameRegistry.registerItem(cryoniteVial, "Cryonite Vial");
GameRegistry.registerItem(neptuniumVial, "Neptunium Vial");
GameRegistry.registerItem(netherflowVial, "Netherflow Vial");
GameRegistry.registerItem(obsidiumVial, "Obsidium Vial");
GameRegistry.registerItem(plutoniumVial, "Plutonium Vial");
GameRegistry.registerItem(radioniteVial, "Radionite Vial");
GameRegistry.registerItem(uraniumVial, "Uranium Vial");
GameRegistry.registerItem(causticBoat, "Caustic Boat");
GameRegistry.registerItem(batteryEmpty, "Empty Battery");
GameRegistry.registerItem(batteryCryo, "Cryo Battery");
GameRegistry.registerItem(batteryCharged, "Charged Caustic Battery");
GameRegistry.registerItem(batteryOvercharged, "Overcharged Caustic Battery");
GameRegistry.registerItem(batteryPlasma, "Plasma Battery");
GameRegistry.registerItem(beamSplitter, "Beam Splitter");
GameRegistry.registerItem(energyCell, "Energy Cell");
GameRegistry.registerItem(thermopellet, "Thermopellet");
GameRegistry.registerItem(acidgun, "Acid Launcher");
GameRegistry.registerItem(cryoblaster, "Cryo Blaster");
GameRegistry.registerItem(lasershotgun, "Laser Shotgun");
GameRegistry.registerItem(lasergun, "Laser Rifle");
GameRegistry.registerItem(lasergunsplit, "Split Beam Laser Rifle");
GameRegistry.registerItem(plasmagun, "Plasma Rifle");
GameRegistry.registerItem(plasmagunsplit, "Split Beam Plasma Rifle");
GameRegistry.registerItem(railgun, "Railgun");
GameRegistry.registerItem(acidGrenade, "Acid Grenade");
GameRegistry.registerItem(plasmaLeather, "Plasma Leather");
GameRegistry.registerItem(hazmatBoots, "Hazmat Boots");
GameRegistry.registerItem(hazmatHood, "Hazmat Hood");
GameRegistry.registerItem(hazmatJacket, "Hazmat Jacket");
GameRegistry.registerItem(hazmatPants, "Hazmat Pants");
}
private void registerOres()
{
OreDictionary.registerOre("orePlutonium", new ItemStack(orePlasma, 1, plutoniumMeta));
OreDictionary.registerOre("oreUranium", new ItemStack(orePlasma, 1, uraniumMeta));
OreDictionary.registerOre("oreLead", new ItemStack(orePlasma, 1, leadMeta));
OreDictionary.registerOre("ingotPlutonium", new ItemStack(ingotPlutonium, 1));
OreDictionary.registerOre("ingotUranium", new ItemStack(ingotUranium, 1));
OreDictionary.registerOre("ingotLead", ingotLead);
}
private void registerRecipes()
{
GameRegistry.addShapelessRecipe(new ItemStack(glowCloth, 1, glowClothAcidMeta), goopAcid, new ItemStack(Blocks.wool, 1, 0));
GameRegistry.addShapelessRecipe(new ItemStack(glowCloth, 1, glowClothPlutoniumMeta), goopPlutonium, new ItemStack(Blocks.wool, 1, 0));
GameRegistry.addShapelessRecipe(new ItemStack(glowCloth, 1, glowClothRadioniteMeta), goopRadionite, new ItemStack(Blocks.wool, 1, 0));
GameRegistry.addShapelessRecipe(new ItemStack(glowCloth, 1, glowClothNeptuniumMeta), goopNeptunium, new ItemStack(Blocks.wool, 1, 0));
GameRegistry.addShapelessRecipe(new ItemStack(glowCloth, 1, glowClothNetherflowMeta), goopNetherflow, new ItemStack(Blocks.wool, 1, 0));
GameRegistry.addShapelessRecipe(new ItemStack(glowCloth, 1, glowClothObsidiumMeta), goopObsidium, new ItemStack(Blocks.wool, 1, 0));
GameRegistry.addShapelessRecipe(new ItemStack(glowCloth, 1, glowClothCryoniteMeta), goopCryonite, new ItemStack(Blocks.wool, 1, 0));
GameRegistry.addShapelessRecipe(new ItemStack(glowCloth, 1, glowClothUraniumMeta), goopUranium, new ItemStack(Blocks.wool, 1, 0));
GameRegistry.addRecipe(new ItemStack(reinforcedGlass, 4), new Object[] {
" X ", "X#X", " X ", Character.valueOf('#'), Blocks.glass, Character.valueOf('X'), Items.iron_ingot
});
GameRegistry.addRecipe(new ItemStack(causticVial, 1), new Object[] {
"X#X", "Y Y", "X#X", Character.valueOf('#'), Items.iron_ingot, Character.valueOf('Y'), reinforcedGlass, Character.valueOf('X'), Blocks.glass
});
GameRegistry.addRecipe(new ItemStack(plasmaBench, 1), new Object[] {
"X#X", "# #", "X#X", Character.valueOf('#'), Items.iron_ingot, Character.valueOf('X'), acidVial
});
GameRegistry.addRecipe(new ItemStack(acidBarrier, 1), new Object[] {
" X ", "XZX", " X ", Character.valueOf('Z'), reinforcedGlass, Character.valueOf('X'), goopAcid
});
GameRegistry.addRecipe(new ItemStack(causticBoat, 1), new Object[] {
"R R", "RRR", Character.valueOf('R'), ingotRadionite
});
GameRegistry.addRecipe(new ItemStack(acidTnt, 4), new Object[] {
"APA", "GAG", "APA", Character.valueOf('A'), acidVial, Character.valueOf('G'), Items.gunpowder, Character.valueOf('P'), plasma
});
GameRegistry.addRecipe(new ItemStack(acidGrenade, 4), new Object[] {
"X", "Y", "Z", Character.valueOf('X'), Items.iron_ingot, Character.valueOf('Y'), acidVial, Character.valueOf('Z'), plasma
});
GameRegistry.addRecipe(new ItemStack(hazmatHood, 1), new Object[] {
"LLL", "L L", Character.valueOf('L'), plasmaLeather
});
GameRegistry.addRecipe(new ItemStack(hazmatJacket, 1), new Object[] {
"L L", "LLL", "LLL", Character.valueOf('L'), plasmaLeather
});
GameRegistry.addRecipe(new ItemStack(hazmatPants, 1), new Object[] {
"LLL", "L L", "L L", Character.valueOf('L'), plasmaLeather
});
GameRegistry.addRecipe(new ItemStack(hazmatBoots, 1), new Object[] {
"L L", "L L", Character.valueOf('L'), plasmaLeather
});
GameRegistry.addRecipe(new ItemStack(plasmaLeather, 1), new Object[] {
"N", "J", Character.valueOf('N'), goopAcid, Character.valueOf('J'), Items.leather
});
GameRegistry.addRecipe(new ItemStack(plasmagunsplit, 1), new Object[] {
"YB", Character.valueOf('B'), plasmagun, Character.valueOf('Y'), beamSplitter
});
GameRegistry.addRecipe(new ItemStack(lasergunsplit, 1), new Object[] {
"YB", Character.valueOf('B'), lasergun, Character.valueOf('Y'), beamSplitter
});
GameRegistry.addRecipe(new ItemStack(cryoblaster, 1), new Object[] {
" A", "CBX", " DE", Character.valueOf('A'), ingotUranium, Character.valueOf('B'), goopCryonite, Character.valueOf('C'), ingotCryonite, Character.valueOf('D'),
ingotObsidium, Character.valueOf('X'), batteryCryo, Character.valueOf('E'), ingotPlutonium
});
GameRegistry.addRecipe(new ItemStack(lasershotgun, 1), new Object[] {
" A", "BCD", " EF", Character.valueOf('A'), Items.repeater, Character.valueOf('B'), beamSplitter, Character.valueOf('C'), ingotNetherflow, Character.valueOf('D'),
batteryCharged, Character.valueOf('E'), ingotRadionite, Character.valueOf('F'), ingotPlutonium
});
GameRegistry.addRecipe(new ItemStack(lasergun, 1), new Object[] {
"ABC", " BD", Character.valueOf('A'), ingotNetherflow, Character.valueOf('B'), ingotObsidium, Character.valueOf('C'), goopNetherflow, Character.valueOf('D'), ingotPlutonium,
});
GameRegistry.addRecipe(new ItemStack(plasmagun, 1), new Object[] {
"ABC", " DC", Character.valueOf('A'), Items.diamond, Character.valueOf('B'), plasma, Character.valueOf('C'), ingotPlutonium, Character.valueOf('D'), ingotObsidium,
});
GameRegistry.addRecipe(new ItemStack(energyCell, 5), new Object[] {
" R ", "RXR", " R ", Character.valueOf('R'), ingotNeptunium, Character.valueOf('X'), goopAcid
});
GameRegistry.addRecipe(new ItemStack(batteryEmpty,
, new Object[] {
"IRI", "I I", "IRI", Character.valueOf('R'), ingotRadionite, Character.valueOf('I'), Items.iron_ingot
});
GameRegistry.addRecipe(new ItemStack(batteryCryo, 1), new Object[] {
"R", "X", Character.valueOf('R'), goopCryonite, Character.valueOf('X'), batteryEmpty
});
GameRegistry.addRecipe(new ItemStack(batteryPlasma, 1), new Object[] {
"R", "X", Character.valueOf('R'), plasma, Character.valueOf('X'), batteryEmpty
});
GameRegistry.addRecipe(new ItemStack(batteryCharged, 1), new Object[] {
"R", "X", Character.valueOf('R'), goopPlutonium, Character.valueOf('X'), batteryEmpty
});
GameRegistry.addShapelessRecipe(new ItemStack(goopCryonite, 4), plasma, goopCryonite);
GameRegistry.addShapelessRecipe(new ItemStack(goopNeptunium, 4), plasma, goopNeptunium);
GameRegistry.addShapelessRecipe(new ItemStack(goopNetherflow, 4), plasma, goopNetherflow);
GameRegistry.addShapelessRecipe(new ItemStack(goopObsidium, 4), plasma, goopObsidium);
GameRegistry.addShapelessRecipe(new ItemStack(goopPlutonium, 4), plasma, goopPlutonium);
GameRegistry.addShapelessRecipe(new ItemStack(goopRadionite, 4), plasma, goopRadionite);
GameRegistry.addShapelessRecipe(new ItemStack(goopUranium, 4), plasma, goopUranium);
GameRegistry.addShapelessRecipe(new ItemStack(acidVial, 1), goopAcid, causticVial);
GameRegistry.addShapelessRecipe(new ItemStack(cryoniteVial, 1), goopCryonite, causticVial);
GameRegistry.addShapelessRecipe(new ItemStack(neptuniumVial, 1), goopNeptunium, causticVial);
GameRegistry.addShapelessRecipe(new ItemStack(netherflowVial, 1), goopNetherflow, causticVial);
GameRegistry.addShapelessRecipe(new ItemStack(obsidiumVial, 1), goopObsidium, causticVial);
GameRegistry.addShapelessRecipe(new ItemStack(plutoniumVial, 1), goopPlutonium, causticVial);
GameRegistry.addShapelessRecipe(new ItemStack(radioniteVial, 1), goopRadionite, causticVial);
GameRegistry.addShapelessRecipe(new ItemStack(uraniumVial, 1), goopUranium, causticVial);
GameRegistry.addSmelting(oreLeadBlock, new ItemStack(ingotLead, 1), 0.1f);
}
public static void loadConfig()
{
Configuration c;
if(FMLCommonHandler.instance().getSide() == Side.CLIENT)
{
c = new Configuration(new File(Minecraft.getMinecraft().mcDataDir, "/config/PlasmaCraft.cfg"));
}
else
{
c = new Configuration(new File("./config/PlasmaCraft.cfg"));
}
c.load();
liquidSourceExplodesAfterCausticExplosion = c.get(Configuration.CATEGORY_GENERAL, "LiquidSourceExplodesAfterCausticExplosion", true).getBoolean(true);
generateLead = c.get(Configuration.CATEGORY_GENERAL, "General.GenerateLead", true).getBoolean(true);
generateUranium = c.get(Configuration.CATEGORY_GENERAL, "General.GenerateUranium", true).getBoolean(true);
generatePlutonium = c.get(Configuration.CATEGORY_GENERAL, "General.GeneratePlutonium", true).getBoolean(true);
c.save();
}
private void registerTileEntities()
{
GameRegistry.registerTileEntity(TilePlasmaBench.class, "tilePlasmaBench");
}
}
ClientProxy
package com.malkierian.plasmacraft.client;
import net.minecraft.client.entity.EntityPlayerSP;
import net.minecraft.entity.Entity;
import com.malkierian.plasmacraft.client.renderers.ModelMutantCow;
import com.malkierian.plasmacraft.client.renderers.RenderAcid;
import com.malkierian.plasmacraft.client.renderers.RenderAcidTNTPrimed;
import com.malkierian.plasmacraft.client.renderers.RenderCausticBoat;
import com.malkierian.plasmacraft.client.renderers.RenderCryoBlast;
import com.malkierian.plasmacraft.client.renderers.RenderLaser;
import com.malkierian.plasmacraft.client.renderers.RenderLaserShotgun;
import com.malkierian.plasmacraft.client.renderers.RenderMutantCow;
import com.malkierian.plasmacraft.client.renderers.RenderPlasma;
import com.malkierian.plasmacraft.client.renderers.RenderRailGun;
import com.malkierian.plasmacraft.core.CommonProxy;
import com.malkierian.plasmacraft.core.PlasmaCraft;
import com.malkierian.plasmacraft.core.entities.EntityAcid;
import com.malkierian.plasmacraft.core.entities.EntityAcidTNTPrimed;
import com.malkierian.plasmacraft.core.entities.EntityCausticBoat;
import com.malkierian.plasmacraft.core.entities.EntityCryoBlast;
import com.malkierian.plasmacraft.core.entities.EntityLaser;
import com.malkierian.plasmacraft.core.entities.EntityLaserShotgun;
import com.malkierian.plasmacraft.core.entities.EntityMutantCow;
import com.malkierian.plasmacraft.core.entities.EntityPlasma;
import com.malkierian.plasmacraft.core.entities.EntityRailGun;
import cpw.mods.fml.client.registry.RenderingRegistry;
public class ClientProxy extends CommonProxy
{
@Override
public void registerRenderers()
{
PlasmaCraft.causticID = RenderingRegistry.getNextAvailableRenderId();
RenderingRegistry.registerEntityRenderingHandler(EntityCausticBoat.class, new RenderCausticBoat());
RenderingRegistry.registerEntityRenderingHandler(EntityAcidTNTPrimed.class, new RenderAcidTNTPrimed());
RenderingRegistry.registerEntityRenderingHandler(EntityAcid.class, new RenderAcid());
RenderingRegistry.registerEntityRenderingHandler(EntityCryoBlast.class, new RenderCryoBlast());
RenderingRegistry.registerEntityRenderingHandler(EntityLaser.class, new RenderLaser());
RenderingRegistry.registerEntityRenderingHandler(EntityLaserShotgun.class, new RenderLaserShotgun());
RenderingRegistry.registerEntityRenderingHandler(EntityMutantCow.class, new RenderMutantCow(new ModelMutantCow(), 1.0f));
RenderingRegistry.registerEntityRenderingHandler(EntityPlasma.class, new RenderPlasma());
RenderingRegistry.registerEntityRenderingHandler(EntityRailGun.class, new RenderRailGun());
}
@Override
public boolean getEntityInstanceOf(Entity entity)
{
return entity instanceof EntityPlayerSP;
}
@Override
public int addArmor(String name)
{
return RenderingRegistry.addNewArmourRendererPrefix(name);
}
}
Most of the code is still the same on my repo, too, if you need to see anything else:
https://github.com/Malkierian/PlasmaCraft
So is there any way I can fix this, or am I going to have to remove the rendering and entity and just leave it as a block?
-
Guess what? I found out what it was. GSON apparently is incapable of reading UTF-8. Here, I thought I was doing what I was supposed to by making sure the .info file was being saved as UTF-8, and apparently those first three identifying bytes of the file were what were causing all the ruckus... I found this out by pulling the mcmod.info file out of ForgeEssentials and opened it up with Notepad, and lo and behold the newlines weren't working there, like they usually don't, but still were in Notepad++. So I edited that file without changing encoding and it works fine now.
-
I just don't understand it either. I went ahead and removed all of the newlines and extraneous spacing (so it was all on one line and the only spaces were in the string values), and it STILL gave me the error. I even did the extended searching in Notepad++ to find and remove any individual return or newline characters, and there weren't any, and STILL that line 1 column 5. I'm so confused...
-
Alright, so I've been going at this for hours now. I can't seem to get FML to parse my mcmod.info file. Here it is:
I've validated it almost 10 times now, with varying arrangements of spacing, brackets, etc. Every single time, I get this error, or something like it:
[19:30:37] [Client thread/ERROR] [FML]: The mcmod.info file in PlasmaCraft-0.3.5.jar cannot be parsed as valid JSON. It will be ignored com.google.gson.JsonSyntaxException: com.google.gson.stream.MalformedJsonException: Use JsonReader.setLenient(true) to accept malformed JSON at line 1 column 5 at com.google.gson.JsonParser.parse(JsonParser.java:65) ~[JsonParser.class:?] at cpw.mods.fml.common.MetadataCollection.from(MetadataCollection.java:55) [MetadataCollection.class:?] at cpw.mods.fml.common.discovery.JarDiscoverer.discover(JarDiscoverer.java:53) [JarDiscoverer.class:?] at cpw.mods.fml.common.discovery.ContainerType.findMods(ContainerType.java:42) [ContainerType.class:?] at cpw.mods.fml.common.discovery.ModCandidate.explore(ModCandidate.java:71) [ModCandidate.class:?] at cpw.mods.fml.common.discovery.ModDiscoverer.identifyMods(ModDiscoverer.java:127) [ModDiscoverer.class:?] at cpw.mods.fml.common.Loader.identifyMods(Loader.java:347) [Loader.class:?] at cpw.mods.fml.common.Loader.loadMods(Loader.java:468) [Loader.class:?] at cpw.mods.fml.client.FMLClientHandler.beginMinecraftLoading(FMLClientHandler.java:204) [FMLClientHandler.class:?] at net.minecraft.client.Minecraft.func_71384_a(Minecraft.java:480) [bao.class:?] at net.minecraft.client.Minecraft.func_99999_d(Minecraft.java:867) [bao.class:?] at net.minecraft.client.main.Main.main(SourceFile:148) [Main.class:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.7.0_67] at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) ~[?:1.7.0_67] at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) ~[?:1.7.0_67] at java.lang.reflect.Method.invoke(Unknown Source) ~[?:1.7.0_67] at net.minecraft.launchwrapper.Launch.launch(Launch.java:135) [launchwrapper-1.11.jar:?] at net.minecraft.launchwrapper.Launch.main(Launch.java:28) [launchwrapper-1.11.jar:?] Caused by: com.google.gson.stream.MalformedJsonException: Use JsonReader.setLenient(true) to accept malformed JSON at line 1 column 5 at com.google.gson.stream.JsonReader.syntaxError(JsonReader.java:1505) ~[JsonReader.class:?] at com.google.gson.stream.JsonReader.checkLenient(JsonReader.java:1386) ~[JsonReader.class:?] at com.google.gson.stream.JsonReader.doPeek(JsonReader.java:531) ~[JsonReader.class:?] at com.google.gson.stream.JsonReader.peek(JsonReader.java:414) ~[JsonReader.class:?] at com.google.gson.JsonParser.parse(JsonParser.java:60) ~[JsonParser.class:?] ... 17 more
What the heck is going on? I've remade the file, making sure the right encoding is being used, and rewrote the file from scratch at the same time, and still no difference.
-
OK, so I see the getItemFromBlock method, but are you saying I'm supposed to keep a custom list that is fed to the tab instead of using the one from displayReleventItems?
And besides, that still doesn't explain why they're not being sorted in the order they were added.
EDIT: Ohhhhhhhh, I think I get it now. The list fed to the comparator is the order you want the displayReleventItems list to be sorted by, that's why it's an explicit order. I should know better than to look at tutorials when I'm tired...
Still would like to know why any of this is necessary, though, instead of it being handled automatically the way it should through the registration order...
-
Yes, I saw that, but it doesn't say anything about sorting blocks and items in the same tab, and I tried putting the explicit thing in there and nothing changed.
-
So I've finally been getting around to updating my mod from 1.5 to 1.7. A lot of things are going well, but for the life of me I can't get the items in my tab to sort. I've read many times that items are added to the creative tab as they're registered in the GameRegistry, but no amount of rearranging in the code changes any order in the tab. Here's my mod file:
And here's a couple screenshots of the tab:
http://gyazo.com/38a1d65dc9af1ecc897df1092bb84ccd
http://gyazo.com/02a4e4413e5df75bdfb80bda07497280
As you can see, the items are all jumbled up, despite being registered in a particular order. So I decided to try to sort the tab myself, but I don't know how to do a multisort with the Comparator (specifically sorting by block first, then by name or type or whatever. Right now they're not even sorted alphabetically). Is there something I'm missing that would make this easier, or do I need to custom sort it, and how would I go about doing it?
-
... I am flabbergasted... Thanks, diesieben07, I never would have thought of that...
-
This is the fml-client-latest.log, minus the mentions of the registry and patch application and the mod file check (the whole log was way too big to put in pastebin). I realize the first set of errors is known, I just included all the errors I could find.
My problem is, I just barely installed a fresh profile for Forge 1.7.10 using the Windows Forge installer. No extra mods, no settings changes, and when I load up the profile, everything looks fine. But then I try to start any world, or log into a Forge server, and within seconds it tells me it's shutting down the internal server, then it crashes back to the launcher. I'm using the last version of Java 7.
-
mozziedoo, it is the responsibility of the mod maker to provide compatibility. If Forge was required to provide that for every mod, it would never get released.
Now GTFO and find the mod creator to pester.
[1.7.10] Primed Custom TNT Block Renders As White Cube
in Modder Support
Posted
I would love to have a look at it to see if I can glean anything.