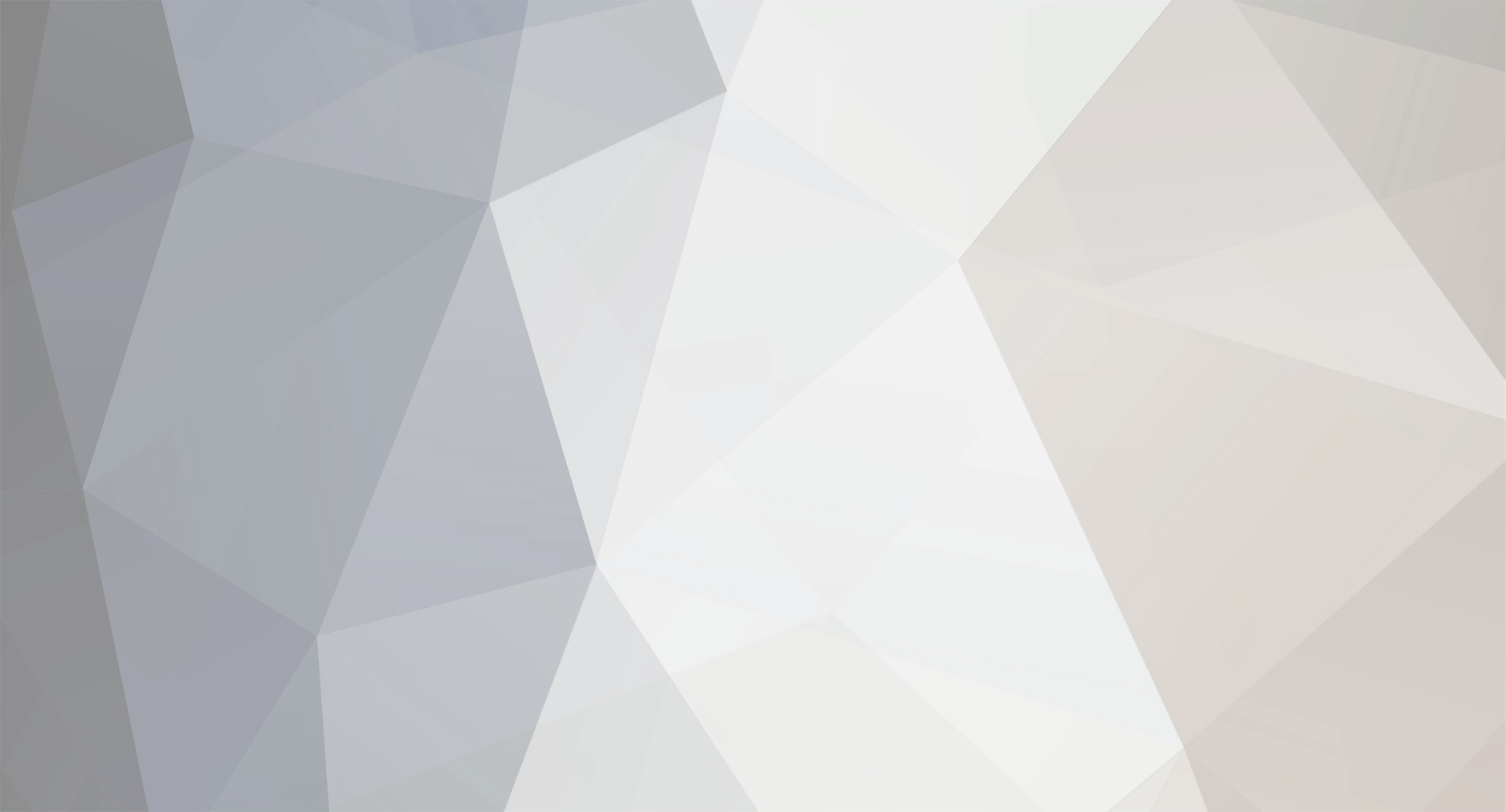
Ronaldi2001
Members-
Posts
91 -
Joined
-
Last visited
Everything posted by Ronaldi2001
-
Im trying to create a block where you put in an item and the block reads the nbt on that item and puts different items in other slots, and when those other items are places in the slots it updates the nbt of the original item? I have a tile entity designed but when I put the item in the game crashes due to ticking block. I want to start fresh but dont know how to succeed without being in the same boat.
-
Yes I do program my own mod. I was wondering if you could help with the problem I asked
-
So with that how would I access everything in the TileEntity class? Also would that fix the crash?
-
The code above im planning on moving it into another class so each upgrade can be modular. This is the code of the tile entity: public class DataEncoderTileEntity extends LockableLootTileEntity implements ITickableTileEntity, INamedContainerProvider{ public static final int SLOTS = 13; private static final DataEncoderTileEntity INSTANCE = new DataEncoderTileEntity(); public NonNullList<ItemStack> inventory; public boolean EditMode = false; CompoundNBT nbtTagCompound; public DataEncoderTileEntity() { super(MITileEntities.DATAENCODER_TILEENTITY); this.inventory = NonNullList.withSize(SLOTS, ItemStack.EMPTY); } public static DataEncoderTileEntity getInstance() { return INSTANCE; } @Override public void tick() { if(getStackInSlot(0).isEmpty()) EditMode = true; else EditMode = false; if(!EditMode) nbtTagCompound = getStackInSlot(0).getTag(); if(!EditMode && getItem(getStackInSlot(0)) && nbtTagCompound.size() == isEnchanted()) addNBT(getStackInSlot(0)); if(!EditMode) if(getItem(getStackInSlot(0))) init(); if(EditMode) clear(); } public static void init() { Fireproof.init(); } @Mod.EventBusSubscriber(bus=Mod.EventBusSubscriber.Bus.MOD) public static class Fireproof { static int index; static Boolean EditMode; public static Boolean editThis; static ItemStack item; static CompoundNBT nbtTagCompound; static NonNullList<ItemStack> inventory; public static Item upgrade = MIItems.upgrade_card_fireproof; public static String name = "Fireproof"; public static void init() { inventory = DataEncoderTileEntity.getInstance().getItems(); index = SlotFireproof.index; EditMode = DataEncoderTileEntity.getInstance().getEdit(); item = inventory.get(0); if(!EditMode) nbtTagCompound = item.getTag(); if(EditMode) editThis = false; if(!EditMode && nbtTagCompound.contains(name)) { editThis = true; run(); } else { editThis = false; } } static void run() { DataEncoderTileEntity.getInstance().setInventorySlotContents(index, item); if(!DataEncoderTileEntity.getInstance().getStackInSlot(index).isEmpty()) { nbtTagCompound.putBoolean(name, true); } else { nbtTagCompound.putBoolean(name, false); } } } void addNBT(ItemStack stack) { if(stack.getItem() == MIItems.ultimate_boots) { nbtTagCompound.putBoolean(Fireproof.name, false); } } public Boolean getItem(ItemStack stack) { if(stack.getItem() == MIItems.ultimate_boots) return true; else return false; } public int isEnchanted() { Boolean ench = getStackInSlot(0).isEnchanted(); if(ench) return 2; else return 1; } @Override public void read(CompoundNBT compound) { super.read(compound); this.loadFromNbt(compound); } @Override public CompoundNBT write(CompoundNBT compound) { super.write(compound); return this.saveToNbt(compound); } public void loadFromNbt(CompoundNBT compound) { this.inventory = NonNullList.withSize(this.getSizeInventory(), ItemStack.EMPTY); if (!this.checkLootAndRead(compound) && compound.contains("Items", 9)) { ItemStackHelper.loadAllItems(compound, this.inventory); } } public CompoundNBT saveToNbt(CompoundNBT compound) { if (!this.checkLootAndWrite(compound)) { ItemStackHelper.saveAllItems(compound, this.inventory, false); } return compound; } public int getSizeInventory() { return inventory.size(); } public boolean isEmpty() { return inventory.isEmpty(); } public ItemStack getStackInSlot(int index) { return inventory.get(index); } public ItemStack decrStackSize(int index, int count) { ItemStack itemStack = getStackInSlot(index); if (!itemStack.isEmpty()) { if (itemStack.getCount() <= count) { setInventorySlotContents(index, ItemStack.EMPTY); } else { itemStack = itemStack.split(count); if (itemStack.getCount() == 0) { setInventorySlotContents(index, ItemStack.EMPTY); } } } return itemStack; } public ItemStack removeStackFromSlot(int index) { return getStackInSlot(index); } public void setInventorySlotContents(int index, ItemStack stack) { inventory.set(index, stack); } public void clear() { for(int i = 1; i < SLOTS; i++) { setInventorySlotContents(i, ItemStack.EMPTY); } } @Override protected NonNullList<ItemStack> getItems() { return this.inventory; } @Override protected void setItems(NonNullList<ItemStack> itemsIn) { this.inventory = itemsIn; } @Override protected ITextComponent getDefaultName() { return new StringTextComponent("Data Encoder"); } @Override protected Container createMenu(int id, PlayerInventory player) { return new DataEncoderContainer(id, player, this); } public Boolean getEdit() { return EditMode; } } And the code for the block: public class DataEncoderBlock extends Block { public static final DirectionProperty FACING = HorizontalBlock.HORIZONTAL_FACING; public DataEncoderBlock(String name) { super(Properties.create(Material.IRON) .sound(SoundType.METAL) .hardnessAndResistance(2.0F) ); this.setDefaultState(this.stateContainer.getBaseState().with(FACING, Direction.NORTH)); setRegistryName(name); } public BlockState getStateForPlacement(BlockItemUseContext context) { return this.getDefaultState().with(FACING, context.getPlacementHorizontalFacing().getOpposite()); } @Override public boolean hasTileEntity(BlockState state) { return true; } @Nullable @Override public TileEntity createTileEntity(BlockState state, IBlockReader world) { return new DataEncoderTileEntity(); } public ActionResultType onBlockActivated(BlockState state, World world, BlockPos pos, PlayerEntity player, Hand handIn, BlockRayTraceResult hit) { if (!world.isRemote) { TileEntity tileEntity = world.getTileEntity(pos); if (tileEntity instanceof INamedContainerProvider) { NetworkHooks.openGui((ServerPlayerEntity) player, (INamedContainerProvider) tileEntity, tileEntity.getPos()); } } return ActionResultType.SUCCESS; } public static Direction getFacingFromEntity(BlockPos clickedBlock, LivingEntity entity) { return Direction.getFacingFromVector((float) (entity.prevPosX - clickedBlock.getX()), (float) (entity.prevPosY - clickedBlock.getY()), (float) (entity.prevPosZ - clickedBlock.getZ())); } @Override protected void fillStateContainer(StateContainer.Builder<Block, BlockState> builder) { builder.add(FACING); } }
-
I have a block that checks the nbt of an item in it to display upgrades, when the item is in the slot the game crashes. How would I go about to not tick the block entity so the game doesn't crash? public static class Fireproof { static int index; static Boolean EditMode; public static Boolean editThis; static ItemStack item; static CompoundNBT nbtTagCompound; static NonNullList<ItemStack> inventory; public static Item upgrade = MIItems.upgrade_card_fireproof; public static String name = "Fireproof"; public static void init() { inventory = DataEncoderTileEntity.getInstance().getItems(); index = SlotFireproof.index; EditMode = DataEncoderTileEntity.getInstance().getEdit(); item = inventory.get(0); if(!EditMode) nbtTagCompound = item.getTag(); if(EditMode) editThis = false; if(!EditMode && nbtTagCompound.contains(name)) { editThis = true; run(); } else { editThis = false; } } static void run() { DataEncoderTileEntity.getInstance().setInventorySlotContents(index, item); if(!DataEncoderTileEntity.getInstance().getStackInSlot(index).isEmpty()) { nbtTagCompound.putBoolean(name, true); } else { nbtTagCompound.putBoolean(name, false); } } }
-
Thank you!! This worked, one more question. Is there anyway to carry over NBT data with a crafting recipe? I have different tiers of Air Tanks and when crafting a lower one to get a higher one I want the air to carry over. I don't know if that is even possible??
-
Problem #9 says to make a tile entity. My understanding of a tile entity is a block not an item. So how would I make that just an item? I don't know if i'm understanding this correctly.
-
What do you mean I can't do this? Like I can't store tankAir to not?
-
I am trying to add NBT to my item, I want it to store the int tankAir but I can't figure it out.. How would I go about adding this to the item. package ronaldi2001.moreitems.Items; import java.util.List; import javax.annotation.Nullable; import net.minecraft.client.util.ITooltipFlag; import net.minecraft.entity.Entity; import net.minecraft.item.Item; import net.minecraft.item.ItemStack; import net.minecraft.util.text.ITextComponent; import net.minecraft.util.text.TextFormatting; import net.minecraft.util.text.TranslationTextComponent; import net.minecraft.world.World; import ronaldi2001.moreitems.MoreItems; public class AirTank extends Item{ public int playerAir; boolean Swimming; public int tankAir = 0; public int addAir; public int MaxtankAir; boolean active; public AirTank(String name, int Max, int Add) { super(new Item.Properties() .group(MoreItems.moreitemsitems) .maxStackSize(1) ); setRegistryName(name); MaxtankAir = Max; addAir = Add; } @Override public void inventoryTick(ItemStack stack, World worldIn, Entity player, int itemSlot, boolean isSelected) { playerAir = player.getAir(); Swimming = player.isInWater(); if(Swimming == false && playerAir == 300) { active = false; if(tankAir != MaxtankAir) { tankAir = tankAir + addAir; } } else { if(playerAir < 240 && tankAir > 0) { tankAir--; player.setAir(player.getAir() + 1); active = true; } if(tankAir == 0) { active = false; } } } @Override public void addInformation(ItemStack itemStack, @Nullable World world, List<ITextComponent> tooltip, ITooltipFlag flag) { tooltip.add(new TranslationTextComponent(TextFormatting.YELLOW + "Air: " + String.valueOf(Math.round((tankAir * 100) / MaxtankAir)) + "%")); } public boolean hasEffect(ItemStack par1ItemStack){ return active; } }
-
I am trying to port my 1.14 mod to 1.15 but when I start minecraft I get an error message, is it something im doing wrong with JEI or is it JEI not working properly? [24Mar2020 15:12:20.087] [modloading-worker-5/ERROR] [net.minecraftforge.fml.javafmlmod.FMLModContainer/]: Exception caught during firing event: net/minecraftforge/fml/client/event/ConfigChangedEvent$OnConfigChangedEvent Index: 1 Listeners: 0: NORMAL 1: net.minecraftforge.eventbus.EventBus$$Lambda$2075/36315889@399dc37 java.lang.NoClassDefFoundError: net/minecraftforge/fml/client/event/ConfigChangedEvent$OnConfigChangedEvent at mezz.jei.startup.ClientLifecycleHandler.<init>(ClientLifecycleHandler.java:79) at mezz.jei.JustEnoughItems.lambda$null$2(JustEnoughItems.java:43) at net.minecraftforge.eventbus.EventBus.doCastFilter(EventBus.java:212) at net.minecraftforge.eventbus.EventBus.lambda$addListener$11(EventBus.java:204) at net.minecraftforge.eventbus.EventBus.post(EventBus.java:258) at net.minecraftforge.fml.javafmlmod.FMLModContainer.fireEvent(FMLModContainer.java:106) at java.util.function.Consumer.lambda$andThen$0(Consumer.java:65) at java.util.function.Consumer.lambda$andThen$0(Consumer.java:65) at net.minecraftforge.fml.ModContainer.transitionState(ModContainer.java:112) at net.minecraftforge.fml.ModList.lambda$null$10(ModList.java:134) at java.util.stream.ForEachOps$ForEachOp$OfRef.accept(ForEachOps.java:184) at java.util.ArrayList$ArrayListSpliterator.forEachRemaining(ArrayList.java:1382) at java.util.stream.AbstractPipeline.copyInto(AbstractPipeline.java:481) at java.util.stream.ForEachOps$ForEachTask.compute(ForEachOps.java:291) at java.util.concurrent.CountedCompleter.exec(CountedCompleter.java:731) at java.util.concurrent.ForkJoinTask.doExec(ForkJoinTask.java:289) at java.util.concurrent.ForkJoinPool$WorkQueue.runTask(ForkJoinPool.java:1056) at java.util.concurrent.ForkJoinPool.runWorker(ForkJoinPool.java:1692) at java.util.concurrent.ForkJoinWorkerThread.run(ForkJoinWorkerThread.java:157) Caused by: java.lang.ClassNotFoundException: net.minecraftforge.fml.client.event.ConfigChangedEvent$OnConfigChangedEvent at java.lang.ClassLoader.findClass(ClassLoader.java:530) at java.lang.ClassLoader.loadClass(ClassLoader.java:424) at cpw.mods.modlauncher.TransformingClassLoader.loadClass(TransformingClassLoader.java:101) at java.lang.ClassLoader.loadClass(ClassLoader.java:357) ... 19 more [24Mar2020 15:12:20.089] [modloading-worker-5/ERROR] [net.minecraftforge.fml.javafmlmod.FMLModContainer/LOADING]: Caught exception during event FMLLoadCompleteEvent dispatch for modid jei java.lang.NoClassDefFoundError: net/minecraftforge/fml/client/event/ConfigChangedEvent$OnConfigChangedEvent at mezz.jei.startup.ClientLifecycleHandler.<init>(ClientLifecycleHandler.java:79) ~[jei-1.15.1-6.0.0.1_mapped_snapshot_20190719-1.14.3.jar:6.0.0.1] at mezz.jei.JustEnoughItems.lambda$null$2(JustEnoughItems.java:43) ~[jei-1.15.1-6.0.0.1_mapped_snapshot_20190719-1.14.3.jar:6.0.0.1] at net.minecraftforge.eventbus.EventBus.doCastFilter(EventBus.java:212) ~[eventbus-2.0.0-milestone.1-service.jar:?] at net.minecraftforge.eventbus.EventBus.lambda$addListener$11(EventBus.java:204) ~[eventbus-2.0.0-milestone.1-service.jar:?] at net.minecraftforge.eventbus.EventBus.post(EventBus.java:258) ~[eventbus-2.0.0-milestone.1-service.jar:?] at net.minecraftforge.fml.javafmlmod.FMLModContainer.fireEvent(FMLModContainer.java:106) ~[?:30.0] at java.util.function.Consumer.lambda$andThen$0(Consumer.java:65) ~[?:1.8.0_161] at java.util.function.Consumer.lambda$andThen$0(Consumer.java:65) ~[?:1.8.0_161] at net.minecraftforge.fml.ModContainer.transitionState(ModContainer.java:112) ~[?:?] at net.minecraftforge.fml.ModList.lambda$null$10(ModList.java:134) ~[?:?] at java.util.stream.ForEachOps$ForEachOp$OfRef.accept(ForEachOps.java:184) [?:1.8.0_161] at java.util.ArrayList$ArrayListSpliterator.forEachRemaining(ArrayList.java:1382) [?:1.8.0_161] at java.util.stream.AbstractPipeline.copyInto(AbstractPipeline.java:481) [?:1.8.0_161] at java.util.stream.ForEachOps$ForEachTask.compute(ForEachOps.java:291) [?:1.8.0_161] at java.util.concurrent.CountedCompleter.exec(CountedCompleter.java:731) [?:1.8.0_161] at java.util.concurrent.ForkJoinTask.doExec(ForkJoinTask.java:289) [?:1.8.0_161] at java.util.concurrent.ForkJoinPool$WorkQueue.runTask(ForkJoinPool.java:1056) [?:1.8.0_161] at java.util.concurrent.ForkJoinPool.runWorker(ForkJoinPool.java:1692) [?:1.8.0_161] at java.util.concurrent.ForkJoinWorkerThread.run(ForkJoinWorkerThread.java:157) [?:1.8.0_161] Caused by: java.lang.ClassNotFoundException: net.minecraftforge.fml.client.event.ConfigChangedEvent$OnConfigChangedEvent at java.lang.ClassLoader.findClass(ClassLoader.java:530) ~[?:1.8.0_161] at java.lang.ClassLoader.loadClass(ClassLoader.java:424) ~[?:1.8.0_161] at cpw.mods.modlauncher.TransformingClassLoader.loadClass(TransformingClassLoader.java:101) ~[modlauncher-5.0.0-milestone.4.jar:?] at java.lang.ClassLoader.loadClass(ClassLoader.java:357) ~[?:1.8.0_161]
-
So is there anyway to replace it in code instead of using json files?
-
moreitems is my mod id, I placed my new recipes under that and the recipes im trying to remove/replace is under minecraft.
-
I have done this with golden and iron armor but it still shows the recipes that forge has added to work with the ingots tag in data/minecraft/recipes. How would you replace this because following what you said and it adds my new recipe that I placed in data/moreitems/recipes and keeps the old recipe that im trying to remove.
-
I am also having this issue.. What have you done to fix this?
-
I am trying to replace the armor recipes with my own, I places my recipes in data/minecraft/recipes and the leather armor recipe got replaced but not iron, golden, and diamond. Is there another way to replace a recipe because this currently isn't working. I also tried placing a recipe that creates air in the data/minecraft/recipes with the same file name as the item im trying to replace then putting my custom recipe in data/moreitems/recipes, but that didn't work either.
-
Im trying to figure out how to add burn time to a block, I can add It to an item no problem but I can't figure it out for a block. I tried looking through the Minecraft code for what they do with a coal block but I can't find where they do it.
-
Im trying to port my mod from 1.12 to 1.14 and can't figure out how to make an item stay in the crafting grid after its used to craft and then take damage. Ive tried many different things and can't quite figure out how to make it work..
-
Tile Entitry Extracting on One Side Only
Ronaldi2001 replied to Ronaldi2001's topic in Modder Support
I can't get my head around capabilities could you please give an example? -
Tile Entitry Extracting on One Side Only
Ronaldi2001 replied to Ronaldi2001's topic in Modder Support
When I return a slot I want, it says says "change method to return int"? Also I deleted the line about energy I don't use that. -
I have a machine that works perfectly fine until you put a hopper under it, the hopper extracts from all three slots instead of just the output. how do I only set the output slot to be extract only and the input slots be input only? Thanks in advanced!
-
That was it I couldn't see what you were talking about but when I deleted that bracket it worked! Thank you!
-
https://github.com/Ronaldi2001/MoreItems/tree/1.12.2
-
What files are you looking for?
-
I am trying to run gradlew setupDecompWorkspace but it fails at root project and I get this error message: * Where: Build file '/Users/ronnie_williams/Desktop/MC Mods/More Items/More Items 1.12/build.gradle' line: 45 * What went wrong: A problem occurred evaluating root project 'More Items 1.12'. > Cannot get property '' on null object This is my build.gradle buildscript { repositories { jcenter() maven { url = "http://files.minecraftforge.net/maven" } } dependencies { classpath 'net.minecraftforge.gradle:ForgeGradle:2.3-SNAPSHOT' } } apply plugin: 'net.minecraftforge.gradle.forge' version = "1.2" group = "ronaldi2001.moreitems" archivesBaseName = "moreitems" sourceCompatibility = 1.8 targetCompatibility = 1.8 minecraft { version = "1.12-14.21.1.2443" runDir = "run" // the mappings can be changed at any time, and must be in the following format. // snapshot_YYYYMMDD snapshot are built nightly. // stable_# stables are built at the discretion of the MCP team. // Use non-default mappings at your own risk. they may not always work. // simply re-run your setup task after changing the mappings to update your workspace. mappings = "snapshot_20170624" // makeObfSourceJar = false // an Srg named sources jar is made by default. uncomment this to disable. } repositories { maven { // location of the maven that hosts JEI files name = "Progwml6 maven" url = "http://dvs1.progwml6.com/files/maven" } maven { // location of a maven mirror for JEI files, as a fallback name = "ModMaven" url = "modmaven.k-4u.nl" } } dependencies { // compile against the JEI API but do not include it at runtime deobfProvided "mezz.jei:jei_${mc_version}:${jei_version}:api" // at runtime, use the full JEI jar runtime "mezz.jei:jei_${mc_version}:${jei_version}" } processResources { // this will ensure that this task is redone when the versions change. inputs.property "version", project.version inputs.property "mcversion", project.minecraft.version // replace stuff in mcmod.info, nothing else from(sourceSets.main.resources.srcDirs) { include 'mcmod.info' // replace version and mcversion expand 'version':project.version, 'mcversion':project.minecraft.version } // copy everything else except the mcmod.info from(sourceSets.main.resources.srcDirs) { exclude 'mcmod.info' } } This is my grade.properties # Sets default memory used for gradle commands. Can be overridden by user or command line properties. # This is required to provide enough memory for the Minecraft decompilation process. org.gradle.jvmargs=-Xmx3G mc_version=1.12.2 jei_version=4.12.0.215 This worked for someone else and I don't know why its not working for me? Thank you for your help!
-
I am getting this error message when trying to write NBT to save the inventory, if anyone could tell me what I am doing wrong I would appreciate it! [02:15:01] [Server thread/ERROR] [FML]: A TileEntity type ronaldi2001.MoreItems.blocks.tileentity.TileEntityUpgrader has throw an exception trying to write state. It will not persist. Report this to the mod author java.lang.RuntimeException: class ronaldi2001.MoreItems.blocks.tileentity.TileEntityUpgrader is missing a mapping! This is a bug! at net.minecraft.tileentity.TileEntity.writeInternal(TileEntity.java:89) ~[TileEntity.class:?] at net.minecraft.tileentity.TileEntity.writeToNBT(TileEntity.java:80) ~[TileEntity.class:?] at ronaldi2001.MoreItems.blocks.tileentity.TileEntityUpgrader.writeToNBT(TileEntityUpgrader.java:124) ~[TileEntityUpgrader.class:?] at net.minecraft.world.chunk.storage.AnvilChunkLoader.writeChunkToNBT(AnvilChunkLoader.java:416) [AnvilChunkLoader.class:?] at net.minecraft.world.chunk.storage.AnvilChunkLoader.saveChunk(AnvilChunkLoader.java:191) [AnvilChunkLoader.class:?] at net.minecraft.world.gen.ChunkProviderServer.saveChunkData(ChunkProviderServer.java:214) [ChunkProviderServer.class:?] at net.minecraft.world.gen.ChunkProviderServer.saveChunks(ChunkProviderServer.java:242) [ChunkProviderServer.class:?] at net.minecraft.world.WorldServer.saveAllChunks(WorldServer.java:1062) [WorldServer.class:?] at net.minecraft.server.MinecraftServer.saveAllWorlds(MinecraftServer.java:467) [MinecraftServer.class:?] at net.minecraft.server.integrated.IntegratedServer.saveAllWorlds(IntegratedServer.java:274) [IntegratedServer.class:?] at net.minecraft.server.integrated.IntegratedServer.tick(IntegratedServer.java:177) [IntegratedServer.class:?] at net.minecraft.server.MinecraftServer.run(MinecraftServer.java:589) [MinecraftServer.class:?] at java.lang.Thread.run(Thread.java:748) [?:1.8.0_161] This is what is in my tile entity class @Override public void readFromNBT(NBTTagCompound compound) { super.readFromNBT(compound); this.inventory = NonNullList.<ItemStack>withSize(this.getSizeInventory(), ItemStack.EMPTY); ItemStackHelper.loadAllItems(compound, inventory); this.burnTime = compound.getInteger("BurnTime"); this.cookTime = compound.getInteger("CookTime"); this.totalCookTime = compound.getInteger("CookTimeTotal"); this.currentBurnTime = getItemBurnTime((ItemStack)this.inventory.get(2)); if(compound.hasKey("CustomName", 8)) this.customName = compound.getString("CustomName"); } @Override public NBTTagCompound writeToNBT(NBTTagCompound compound) { super.writeToNBT(compound); compound.setInteger("BurnTime", (short)this.burnTime); compound.setInteger("CookTime", (short)this.cookTime); compound.setInteger("CookTimeTotal", (short)this.totalCookTime); ItemStackHelper.saveAllItems(compound, inventory); if(compound.hasKey("CustomName", 8)) compound.setString("CustomName", this.customName); return compound; }