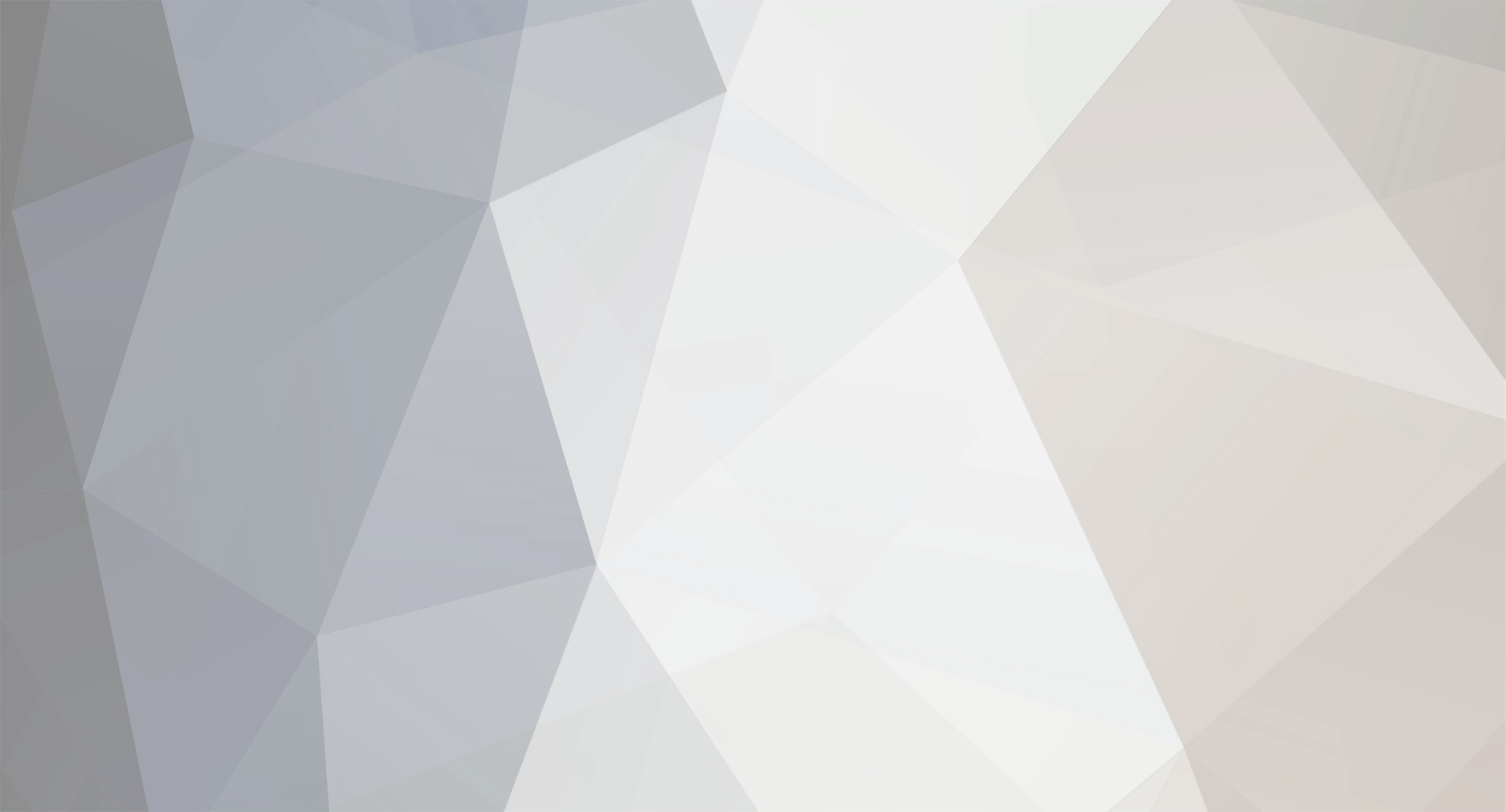
HashtagShell
Members-
Posts
94 -
Joined
-
Last visited
Everything posted by HashtagShell
-
Right, because type erasure is a thing... Of course communicating is the preferred way for humans to handle things, I was just making a point. Thanks for clarifying!
-
rebuild the workspace with ./gradlew eclipse And gradle will link it in the project
-
I've thought of this scenario, and I'm not sure how the capability system would behave in it: Say mod A defines a handler interface adev.amod.AHandler, and uses it as a capability: @CapabilityInject(AHandler.class) public static Capability<IItemHandler> A_HANDLER_CAPABILITY = null; However, it doesn't publish the capability as part of its API and it doesn't public its source, so I cannot compile against it. If I, in my apis source set, in the same package define structurally the same interface, and use @CapabilityInject on it, will I get the same instance of the Capability as the one in mod A? How does change when I do/don't ship the AHandler interface with my mod? What if the mod is not present at runtime, will @CapabilityInject still try to reference the missing interface and crash? (I cannot really do any programmatic checks for the presence of the mod since annotations are compile-time constants) This is a theoretical scenario, any mod that is worth building against will have their capabilities published.
-
Is instrumenting own code a good idea?
HashtagShell replied to HashtagShell's topic in Modder Support
So I looked at a few popular mods' build script's and found this, which looks like it might do what I wanted: https://github.com/SleepyTrousers/EnderIO/blob/1.10/build.gradle#L132-L141 -
Overriding Server Mod with Client Mod
HashtagShell replied to SecondAmendment's topic in Modder Support
RayTraceResult can hold entities, but I don't see any being assigned to it except in objectMouseOver(), which is client-only. Someone a little more familiar can probably point you to a method that ray traces entities on the server. However, if you look at the objectMouseOver() method, you can see that it uses the hitVector, which you get from yaw and pitch, the player position, which you have, and a world object, which you also have. So you could adapt the objectMouseOver() method's logic to use server side data. -
Overriding Server Mod with Client Mod
HashtagShell replied to SecondAmendment's topic in Modder Support
There is even this method, ForgeHooks#rayTraceEyes(EntityLivingBase,double)RayTraceResult, which makes use of World#rayTraceBlocks and is even more convenient for what you want to do. -
Overriding Server Mod with Client Mod
HashtagShell replied to SecondAmendment's topic in Modder Support
Also take note of the World#rayTraceBlocks method and its overloads -
Overriding Server Mod with Client Mod
HashtagShell replied to SecondAmendment's topic in Modder Support
Every entity has posX, posY, posZ, rotationYaw, rotationPitch. The server has the world object available. This is enough data to do a ray trace. -
Is instrumenting own code a good idea?
HashtagShell replied to HashtagShell's topic in Modder Support
Alright. Is there somewhere in gradle I can put a source processor that would strip debug lines, etc? Kinda like the conditional compiling in C# -
Installing forge in none default location?
HashtagShell replied to antonyvw's topic in Support & Bug Reports
As far as I know (smarter people correcteth me), the Minecraft launcher only looks in ~/.minecraft or %appdata%\.minecraft for versions/profiles, and there is no way to change that. If you need it to be somewhere else due to for instance space concerns, consider symlinking the folder somewhere else (does windows allow cross-device symlinks?). Also note that while you can install the forge binary in the default location, your mods/saves/resourcepacks/servers (the big files) can be saved elsewhere by editing the "Game directory" property of any profile. This is probably what you are after. -
Coremodding, and bytecode instrumentation in general, are said to leads to compatibility issues with other instrumenters. This I agree with and try to avoid it as much as possible. However, one could argue that, if they follow the principle above, no modder should ever tinker with my own code. That would mean that I am free to tinker with it without any compatibility issues. Uses that come into mind are dynamically generated register/init methods, custom run-time methods for debugging purposes, late removal of debug statements, and a few more. Some of these can be accomplished with other safer, albeit slower, techniques (eg. reflection), so my question is: Is the efficiency worth it?
-
Overriding Server Mod with Client Mod
HashtagShell replied to SecondAmendment's topic in Modder Support
Yes, it can do that without help from the client. Keep in mind, the server manages the player's position, the client only keeps a copy that is synchronised every so often, and it sends changes to the server. All server commands are processed strictly on the server unless the programmer adds custom packets to execute functionality on the client. This is almost never the case, since the server knows pretty much everything about the player, except stuff about rendering, which should never be used to make any decisions in functionality (The rendering state is mostly derived from stuff the server knows, so even then it wouldn't need the client, it would calculate it for itself (again, making decisions based on rendering stuff is a bad idea)) -
Overriding Server Mod with Client Mod
HashtagShell replied to SecondAmendment's topic in Modder Support
If you are only trying to do commands, you only need a server-side mod, since the server sends its command list to the client. Server commands are processed on the server and the client doesn't even need to know what effect the command will have - if there is any, the server will tell the client with standard packets. That means that server commands don't have to be registered on the client (and shouldn't, unless it's a local world). This is how bukkit/sponge plugins work, they don't need to be present on the clientside. -
There are a lot of deprecated methods in the Block class (and probably elsewhere, too) that I would consider standard functionality (Compare IInventory which should be deprecated but isn't). The methods are just annotated with @Deprecated, no explanation in their JavaDoc or in a comment is given as to why it would be deprecated (JavaDocs even have a special field for that). I would very much value an explanation as to why they are deprecated, and potentially a non-deprecated replacement. Some of the methods include: Note that while getStateFromMeta is deprecated, getMetaFromState isn't.
-
Removing final on classes (Access Transformers)
HashtagShell replied to HashtagShell's topic in Modder Support
Not sure, but as long as FG's/SS's code supports it, I'm cool. Probably some stupid mistake or typo, which I feel it isn't necessary to hunt down anymore. Thanks a lot! -
Removing final on classes (Access Transformers)
HashtagShell replied to HashtagShell's topic in Modder Support
It didn't work, ItemStack still was final, even though I added the AT. If it had worked the first time, I wouldn't have reason to post anything in the first place. (Just to be clear I won't be subclassing it but using the Cap system instead, tis just theoretical now) -
Removing final on classes (Access Transformers)
HashtagShell replied to HashtagShell's topic in Modder Support
Thanks, this pretty much solved problem. Coming back to the original question, are we not supposed to de-finalize classes, am I doing something wrong, or is this a bug and should be reported? -
Removing final on classes (Access Transformers)
HashtagShell replied to HashtagShell's topic in Modder Support
Hmm... that method is just doing an unchecked cast and suppressing the warning - which I can also do. A suppressed unchecked cast seems more readable to me. Besides, Forge (in the Furnace for example) just casts it directly. Not that there is a big difference, except maybe a method call overhead, which is like nonexistent these days. -
Removing final on classes (Access Transformers)
HashtagShell replied to HashtagShell's topic in Modder Support
So I don't implement anything, but create IItemHandler instances for every side that I want to handle, store them in instance fields, and if <T> in getCapability is of type IItemHandler (for which I check by reference comparing the queried capability with net.minecraftforge.items.CapabilityItemHandler.ITEM_HANDLER_CAPABILITY) I return the IItemHandler instance for the appropriate side, but I need to do an unchecked cast to <T>, because the compiler doesn't know I verified <T> though the capability? This capability system looks cool, also appreciate the unified energy storage capability -
Removing final on classes (Access Transformers)
HashtagShell replied to HashtagShell's topic in Modder Support
Vanilla tiles still do implement IInventory, don't they? Wouldn't that cause compatibility issues? Say that I wanted to check if a block is an inventory, how would I go about that when there are still IInventories around? -
Removing final on classes (Access Transformers)
HashtagShell replied to HashtagShell's topic in Modder Support
This looks nice, however: Won't many modded tiles try to cast my tile to IInventory? What if I want to use ISided? Why can the IItemHandler#getStackInSlot function return null, all (at least Mojang's) code uses NonNullLists and ItemStack.EMPTY everywhere? -
Removing final on classes (Access Transformers)
HashtagShell replied to HashtagShell's topic in Modder Support
So, specifics: I am making an IInventory that doesn't store its contents as a whole but shares contents with another tile in the world. You are of course familiar with the getStackInSlot function, which (by reference) returns the ItemStack in a slot. I need to return the unity of two stacks to this function, so I create a new one with its count being sum of the counts of the two input stacks. Say, that any tile (picture hopper, etc.) can query this stack, and because it expects the by reference thing, it will directly modify it. But, because I created a new stack in the getStackInSlot function, the changes will not be automatically reflected in my inventory. Plus, I need to split the change evenly between the two original ItemStacks, which is what I hoped to do in the overridden ItemStack class. Essentially, I am trying to have an inventory act as a unity of two, but machines expecting ItemStacks to be passed by reference are making this painful. -
Removing final on classes (Access Transformers)
HashtagShell replied to HashtagShell's topic in Modder Support
No. If I meant after the chunk was reloaded, I would say I needed it be persistent or something like that. "A few years later" means that even if this instance (instance requires no serialisation, cloning, etc.) of the ItemStack was kept around for years, I still would want the callback. This was a very unreal and impractical example of a chunk that would be kept loaded for years. -
Removing final on classes (Access Transformers)
HashtagShell replied to HashtagShell's topic in Modder Support
"I am afraid not" and the rest of the comment was in response to loordgek's question, if it was an inventory that I had created. This "automation function" is any function that can make changes to the ItemStack in general - usually this is a method in some TileEntity. Picture me having a TileEntity, which a hopper is pumping into. The hopper will query for a stack in my slot, and directly make changes to it, without returning it - it expects that I passed the stack by reference. I need to complement this by reference passing with some extra functionality. -
Removing final on classes (Access Transformers)
HashtagShell replied to HashtagShell's topic in Modder Support
I actually want my subclass to vanish as soon as the item is serialised, cloned, etc. Picture the following: Function gets an ItemStack instance from me It expects a reference to the same instance to exist in my code (ie. the stack is passed by reference) So it can edit the stack without the need to give it back to me Usually, this is fine, but I need to do extra things when it edits this particular instance, to simulate the "passed by reference" contract Once it clones, serialises, etc. the stack, the function can no longer expect the "passed by reference" contract to be kept, so I don't want to take any actions. If it needs this contract, it will re-query the instance from me, at which point #2 applies.