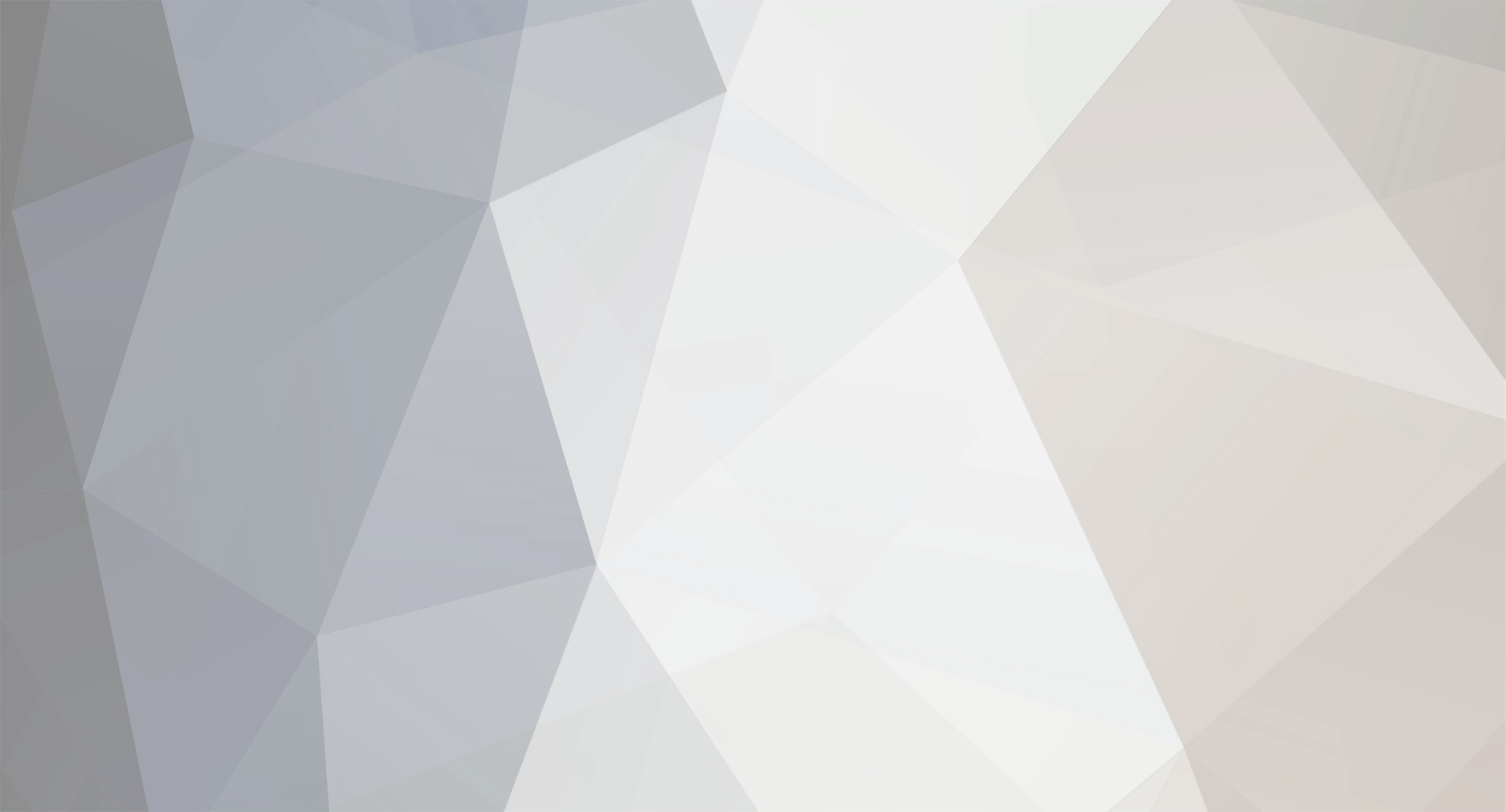
SanaRinomi
-
Posts
72 -
Joined
-
Last visited
Posts posted by SanaRinomi
-
-
Try doing event.getDrops().add instead of resetting the list.
I tried doing that and it didn't work so what I did was add " || true" to the if statements and it still didn't drop the item... And before you ask, I did move it to the load method.
-
My problem is that my custom item never droppes... ;(
-
I admit, I've never worked with events so it's probably something I'm overlooking but anyhow...
I'm trying to get a vanilla block to drop not only it's own item drop but also to have a chance of dropping a custom one.
Here's my Event code:
package com.holydevils.event; import java.util.List; import com.holydevils.items.ModItems; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.init.Blocks; import net.minecraft.init.Items; import net.minecraft.item.ItemStack; import net.minecraftforge.event.world.BlockEvent; import net.minecraftforge.fml.common.eventhandler.SubscribeEvent; public class ModHarvestDropsEvent { @SubscribeEvent public void onHarvestBlock(BlockEvent.HarvestDropsEvent event) { List<ItemStack> currentDrops = event.getDrops(); final EntityPlayer PLAYER = event.getHarvester(); if(null == PLAYER || null == PLAYER.getHeldItemMainhand()) return; if(event.getState().getBlock() == Blocks.DIAMOND_ORE && event.getWorld().rand.nextInt(100) < 75) { currentDrops.add(new ItemStack(ModItems.diamondDust, 1)); } if(event.getState().getBlock() == Blocks.OBSIDIAN && event.getWorld().rand.nextInt(100) < 75) { currentDrops.add(new ItemStack(ModItems.obsidianDust, 1)); } event.getDrops().clear(); event.getDrops().addAll(currentDrops); } }
And here's my main registry:
package com.holydevils.main; import com.holydevils.blocks.ModBlocks; import com.holydevils.client.render.items.ItemRenderRegistry; import com.holydevils.event.ModHarvestDropsEvent; import com.holydevils.items.ModItems; import com.holydevils.lib.StringReferences; import com.holydevils.world.ModWorld; import net.minecraftforge.common.MinecraftForge; import net.minecraftforge.fml.common.FMLCommonHandler; import net.minecraftforge.fml.common.Mod; import net.minecraftforge.fml.common.Mod.EventHandler; import net.minecraftforge.fml.common.SidedProxy; import net.minecraftforge.fml.common.event.FMLInitializationEvent; import net.minecraftforge.fml.common.event.FMLPostInitializationEvent; import net.minecraftforge.fml.common.event.FMLPreInitializationEvent; import net.minecraftforge.fml.common.registry.GameRegistry; @Mod(modid = StringReferences.MODID, name =StringReferences.NAME, version =StringReferences.VERSION) public class MainRegistry { @SidedProxy(clientSide = StringReferences.CLIENTPROXY, serverSide = StringReferences.SERVERPROXY) public static ServerProxy proxyServer; public static ModWorld mWorld = new ModWorld(); @EventHandler public static void preLoad(FMLPreInitializationEvent PreEvent){ ModItems.createItems(); ModBlocks.manageBlocks(); CraftingManager.craftRegister(); GameRegistry.registerWorldGenerator(mWorld, 1); proxyServer.registerRenderInfo(); } public static void load(FMLInitializationEvent Event){ } public static void postLoad(FMLPostInitializationEvent PostEvent){ MinecraftForge.EVENT_BUS.register(new ModHarvestDropsEvent()); } }
Note: I've tried registering it in both load and postLoad. If I put it into preLoad the default item it's self only droppes randomly.
-
- Why are you still on 1.8? Update.
I'm more familiar with 1.8 and my goal is to create a special mod which is based around Hypixel, which supports 1.8. 1.9 and 1.10 is new territory for me.
10/10 IGN will never update again!
EDIT: I felt like adding some humor to this thead... So I did.
-
I've been reading though it, also looked at said Event class, but I still can't seem to get how I'm suposed to make a block drop a custom item.
-
EventHandler here is a great tutorial on it by cool alias.
Thx! Been looking for a good tutorial on that.
-
How can I control said event?
-
I want to add custom drops to Diamond Ore and Obsidian.
-
The
ItemAxe(ToolMaterial)
constructor only works for vanilla
ToolMaterial
s. Use the Forge-provided
ItemAxe(ToolMaterial, float, float)
constructor for modded
ToolMaterial
s.
And may I ask what the two floats represent?
Look at their names in your IDE.
The current version of Neon Eclipse is being stupid and only tells me the type. Nothing else...
EDIT: I retract my statement.
-
The
ItemAxe(ToolMaterial)
constructor only works for vanilla
ToolMaterial
s. Use the Forge-provided
ItemAxe(ToolMaterial, float, float)
constructor for modded
ToolMaterial
s.
And may I ask what the two floats represent?
-
It's probably a stupid mistake that I can't seem to realize what it is, but when creating the axe for my mod the game crashes.
Crash Report:
---- Minecraft Crash Report ---- // Shall we play a game? Time: 12/08/16 11:50 Description: Initializing game java.lang.ArrayIndexOutOfBoundsException: 5 at net.minecraft.item.ItemAxe.<init>(ItemAxe.java:19) at com.holydevils.items.tools.AxeItems.<init>(AxeItems.java:11) at com.holydevils.items.ModItems.createItems(ModItems.java:38) at com.holydevils.main.MainRegistry.preLoad(MainRegistry.java:25) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:498) at net.minecraftforge.fml.common.FMLModContainer.handleModStateEvent(FMLModContainer.java:579) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:498) at com.google.common.eventbus.EventSubscriber.handleEvent(EventSubscriber.java:74) at com.google.common.eventbus.SynchronizedEventSubscriber.handleEvent(SynchronizedEventSubscriber.java:47) at com.google.common.eventbus.EventBus.dispatch(EventBus.java:322) at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:304) at com.google.common.eventbus.EventBus.post(EventBus.java:275) at net.minecraftforge.fml.common.LoadController.sendEventToModContainer(LoadController.java:235) at net.minecraftforge.fml.common.LoadController.propogateStateMessage(LoadController.java:213) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:498) at com.google.common.eventbus.EventSubscriber.handleEvent(EventSubscriber.java:74) at com.google.common.eventbus.SynchronizedEventSubscriber.handleEvent(SynchronizedEventSubscriber.java:47) at com.google.common.eventbus.EventBus.dispatch(EventBus.java:322) at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:304) at com.google.common.eventbus.EventBus.post(EventBus.java:275) at net.minecraftforge.fml.common.LoadController.distributeStateMessage(LoadController.java:142) at net.minecraftforge.fml.common.Loader.preinitializeMods(Loader.java:593) at net.minecraftforge.fml.client.FMLClientHandler.beginMinecraftLoading(FMLClientHandler.java:255) at net.minecraft.client.Minecraft.startGame(Minecraft.java:477) at net.minecraft.client.Minecraft.run(Minecraft.java:386) at net.minecraft.client.main.Main.main(Main.java:118) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:498) at net.minecraft.launchwrapper.Launch.launch(Launch.java:135) at net.minecraft.launchwrapper.Launch.main(Launch.java:28) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:498) at net.minecraftforge.gradle.GradleStartCommon.launch(GradleStartCommon.java:97) at GradleStart.main(GradleStart.java:26) A detailed walkthrough of the error, its code path and all known details is as follows: --------------------------------------------------------------------------------------- -- Head -- Thread: Client thread Stacktrace: at net.minecraft.item.ItemAxe.<init>(ItemAxe.java:19) at com.holydevils.items.tools.AxeItems.<init>(AxeItems.java:11) at com.holydevils.items.ModItems.createItems(ModItems.java:38) at com.holydevils.main.MainRegistry.preLoad(MainRegistry.java:25) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:498) at net.minecraftforge.fml.common.FMLModContainer.handleModStateEvent(FMLModContainer.java:579) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:498) at com.google.common.eventbus.EventSubscriber.handleEvent(EventSubscriber.java:74) at com.google.common.eventbus.SynchronizedEventSubscriber.handleEvent(SynchronizedEventSubscriber.java:47) at com.google.common.eventbus.EventBus.dispatch(EventBus.java:322) at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:304) at com.google.common.eventbus.EventBus.post(EventBus.java:275) at net.minecraftforge.fml.common.LoadController.sendEventToModContainer(LoadController.java:235) at net.minecraftforge.fml.common.LoadController.propogateStateMessage(LoadController.java:213) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:498) at com.google.common.eventbus.EventSubscriber.handleEvent(EventSubscriber.java:74) at com.google.common.eventbus.SynchronizedEventSubscriber.handleEvent(SynchronizedEventSubscriber.java:47) at com.google.common.eventbus.EventBus.dispatch(EventBus.java:322) at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:304) at com.google.common.eventbus.EventBus.post(EventBus.java:275) at net.minecraftforge.fml.common.LoadController.distributeStateMessage(LoadController.java:142) at net.minecraftforge.fml.common.Loader.preinitializeMods(Loader.java:593) at net.minecraftforge.fml.client.FMLClientHandler.beginMinecraftLoading(FMLClientHandler.java:255) at net.minecraft.client.Minecraft.startGame(Minecraft.java:477) -- Initialization -- Details: Stacktrace: at net.minecraft.client.Minecraft.run(Minecraft.java:386) at net.minecraft.client.main.Main.main(Main.java:118) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:498) at net.minecraft.launchwrapper.Launch.launch(Launch.java:135) at net.minecraft.launchwrapper.Launch.main(Launch.java:28) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:498) at net.minecraftforge.gradle.GradleStartCommon.launch(GradleStartCommon.java:97) at GradleStart.main(GradleStart.java:26) -- System Details -- Details: Minecraft Version: 1.10.2 Operating System: Windows 7 (amd64) version 6.1 Java Version: 1.8.0_91, Oracle Corporation Java VM Version: Java HotSpot(TM) 64-Bit Server VM (mixed mode), Oracle Corporation Memory: 709310744 bytes (676 MB) / 1037959168 bytes (989 MB) up to 1037959168 bytes (989 MB) JVM Flags: 3 total; -Xincgc -Xmx1024M -Xms1024M IntCache: cache: 0, tcache: 0, allocated: 0, tallocated: 0 FML: MCP 9.32 Powered by Forge 12.18.1.2011 4 mods loaded, 4 mods active States: 'U' = Unloaded 'L' = Loaded 'C' = Constructed 'H' = Pre-initialized 'I' = Initialized 'J' = Post-initialized 'A' = Available 'D' = Disabled 'E' = Errored UCH mcp{9.19} [Minecraft Coder Pack] (minecraft.jar) UCH FML{8.0.99.99} [Forge Mod Loader] (forgeSrc-1.10.2-12.18.1.2011.jar) UCH Forge{12.18.1.2011} [Minecraft Forge] (forgeSrc-1.10.2-12.18.1.2011.jar) UCE morethingsmod{v0.05} [More Things Mod] (bin) Loaded coremods (and transformers): GL info: ' Vendor: 'ATI Technologies Inc.' Version: '4.5.13447 Compatibility Profile Context 16.300.2311.0' Renderer: 'AMD Radeon R7 200 Series' Launched Version: 1.10.2 LWJGL: 2.9.4 OpenGL: AMD Radeon R7 200 Series GL version 4.5.13447 Compatibility Profile Context 16.300.2311.0, ATI Technologies Inc. GL Caps: Using GL 1.3 multitexturing. Using GL 1.3 texture combiners. Using framebuffer objects because OpenGL 3.0 is supported and separate blending is supported. Shaders are available because OpenGL 2.1 is supported. VBOs are available because OpenGL 1.5 is supported. Using VBOs: Yes Is Modded: Definitely; Client brand changed to 'fml,forge' Type: Client (map_client.txt) Resource Packs: Current Language: English (United Kingdom) Profiler Position: N/A (disabled) CPU: 8x AMD FX(tm)-8350 Eight-Core Processor
ModItems class:
package com.holydevils.items; import com.holydevils.items.tools.AxeItems; import com.holydevils.items.tools.HoeItems; import com.holydevils.items.tools.PickaxeItems; import com.holydevils.items.tools.ShovelItems; import com.holydevils.items.tools.SwordItems; import net.minecraft.item.Item; import net.minecraft.item.Item.ToolMaterial; import net.minecraftforge.common.util.EnumHelper; import net.minecraftforge.fml.common.registry.GameRegistry; public class ModItems { public static ToolMaterial reinforcedObsidian = EnumHelper.addToolMaterial("reinforcedObsidian", 3, 1600, 7.5F, 4.5F, 10); public static Item obsidianStick; public static Item purpleGlowstoneDust; public static Item glueBottle; public static Item glueMixture; public static Item obsidianDust; public static Item diamondDust; public static Item reinforcedObsidianDust; public static Item reinforcedObsidianAxe; public static Item reinforcedObsidianPickaxe; public static Item reinforcedObsidianShovel; public static Item reinforcedObsidianHoe; public static Item reinforcedObsidianSword; public static void createItems() { regItems(obsidianStick = new BasicItems("obsidianStick")); regItems(purpleGlowstoneDust = new BasicItems("purpleGlowstoneDust")); regItems(glueBottle = new BasicItems("glueBottle")); regItems(glueMixture = new BasicItems("glueMixture")); regItems(obsidianDust = new BasicItems("obsidianDust")); regItems(diamondDust = new BasicItems("diamondDust")); regItems(diamondDust = new BasicItems("reinforcedObsidianDust")); regItems(diamondDust = new AxeItems(reinforcedObsidian, "reinforcedObsidianAxe")); regItems(diamondDust = new PickaxeItems(reinforcedObsidian, "reinforcedObsidianPickaxe")); regItems(diamondDust = new ShovelItems(reinforcedObsidian, "reinforcedObsidianShovel")); regItems(diamondDust = new HoeItems(reinforcedObsidian, "reinforcedObsidianHoe")); regItems(diamondDust = new SwordItems(reinforcedObsidian, "reinforcedObsidianSword")); } public static void regItems(Item item){ item.setUnlocalizedName(item.getRegistryName().toString()); GameRegistry.register(item); } }
AxeItem class:
package com.holydevils.items.tools; import com.holydevils.main.MTCreativeTabs; import net.minecraft.item.Item; import net.minecraft.item.ItemAxe; public class AxeItems extends ItemAxe { public AxeItems(ToolMaterial material, String name) { super(material); this.setRegistryName(name); this.setCreativeTab(MTCreativeTabs.tabMoreThingsItems); } }
-
Well, thanks for the help. <3
-
Updated code:
package com.holydevils.blocks; import com.holydevils.items.ModItems; import com.holydevils.lib.StringReferences; import com.holydevils.main.MTCreativeTabs; import net.minecraft.block.Block; import net.minecraft.block.material.Material; import net.minecraft.item.Item; import net.minecraft.item.ItemBlock; import net.minecraftforge.fml.common.registry.GameRegistry; public class ModBlocks { public static Block industrializedWood; public static Block purpleGlowstone; public static Block hardenedIndustrializedWood; public static ItemBlock industrializedWoodItem; public static ItemBlock purpleGlowstoneItem; public static ItemBlock hardenedIndustrializedWoodItem; public static void createBlocks(){ regBlocks(industrializedWoodItem = new ItemBlock(industrializedWood),industrializedWood = new BasicBlocks("industrializedWood", Material.WOOD, 1.0F, 5.0F, "axe", 0)); regBlocks(hardenedIndustrializedWoodItem = new ItemBlock(hardenedIndustrializedWood),hardenedIndustrializedWood = new BasicBlocks("hardenedIndustrializedWood", Material.WOOD, 20.0F, 150.0F, "axe", 2)); regBlocks(purpleGlowstoneItem = new ItemBlock(purpleGlowstone),purpleGlowstone = new SpecialBlocks("purpleGlowstone", Material.GLASS, MTCreativeTabs.tabMoreThingsBlocks, 0.5F, 2.5F, 16, 0.9F, "pickaxe", 0, ModItems.purpleGlowstoneDust, 0, 2, 4)); } public static void regBlocks(ItemBlock IBlock, Block block){ IBlock.setRegistryName(block.getUnlocalizedName().substring(5)); GameRegistry.register(IBlock); GameRegistry.register(block); } }
Note: I put IBlock.setRegistryName(block.getUnlocalizedName().substring(5)); because the unlocalizedName (except the tile. part) is the same as the registry name.
-
You are trying to use the fields that store your block instance (e.g.
industrializedWood
) before you initialize them.
Also I think you need to register the Block before the ItemBlock.
Crash Report...
---- Minecraft Crash Report ---- // Surprise! Haha. Well, this is awkward. Time: 11/08/16 22:46 Description: Initializing game java.lang.NullPointerException: Initializing game at net.minecraftforge.fml.common.registry.GameData$ItemCallbacks.onAdd(GameData.java:324) at net.minecraftforge.fml.common.registry.GameData$ItemCallbacks.onAdd(GameData.java:313) at net.minecraftforge.fml.common.registry.FMLControlledNamespacedRegistry.addObjectRaw(FMLControlledNamespacedRegistry.java:586) at net.minecraftforge.fml.common.registry.FMLControlledNamespacedRegistry.add(FMLControlledNamespacedRegistry.java:484) at net.minecraftforge.fml.common.registry.FMLControlledNamespacedRegistry.register(FMLControlledNamespacedRegistry.java:822) at net.minecraftforge.fml.common.registry.GameData.register_impl(GameData.java:250) at net.minecraftforge.fml.common.registry.GameRegistry.register(GameRegistry.java:153) at com.holydevils.blocks.ModBlocks.regBlocks(ModBlocks.java:30) at com.holydevils.blocks.ModBlocks.createBlocks(ModBlocks.java:23) at com.holydevils.main.MainRegistry.preLoad(MainRegistry.java:26) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:498) at net.minecraftforge.fml.common.FMLModContainer.handleModStateEvent(FMLModContainer.java:579) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:498) at com.google.common.eventbus.EventSubscriber.handleEvent(EventSubscriber.java:74) at com.google.common.eventbus.SynchronizedEventSubscriber.handleEvent(SynchronizedEventSubscriber.java:47) at com.google.common.eventbus.EventBus.dispatch(EventBus.java:322) at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:304) at com.google.common.eventbus.EventBus.post(EventBus.java:275) at net.minecraftforge.fml.common.LoadController.sendEventToModContainer(LoadController.java:235) at net.minecraftforge.fml.common.LoadController.propogateStateMessage(LoadController.java:213) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:498) at com.google.common.eventbus.EventSubscriber.handleEvent(EventSubscriber.java:74) at com.google.common.eventbus.SynchronizedEventSubscriber.handleEvent(SynchronizedEventSubscriber.java:47) at com.google.common.eventbus.EventBus.dispatch(EventBus.java:322) at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:304) at com.google.common.eventbus.EventBus.post(EventBus.java:275) at net.minecraftforge.fml.common.LoadController.distributeStateMessage(LoadController.java:142) at net.minecraftforge.fml.common.Loader.preinitializeMods(Loader.java:593) at net.minecraftforge.fml.client.FMLClientHandler.beginMinecraftLoading(FMLClientHandler.java:255) at net.minecraft.client.Minecraft.startGame(Minecraft.java:477) at net.minecraft.client.Minecraft.run(Minecraft.java:386) at net.minecraft.client.main.Main.main(Main.java:118) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:498) at net.minecraft.launchwrapper.Launch.launch(Launch.java:135) at net.minecraft.launchwrapper.Launch.main(Launch.java:28) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:498) at net.minecraftforge.gradle.GradleStartCommon.launch(GradleStartCommon.java:97) at GradleStart.main(GradleStart.java:26) A detailed walkthrough of the error, its code path and all known details is as follows: --------------------------------------------------------------------------------------- -- Head -- Thread: Client thread Stacktrace: at net.minecraftforge.fml.common.registry.GameData$ItemCallbacks.onAdd(GameData.java:324) at net.minecraftforge.fml.common.registry.GameData$ItemCallbacks.onAdd(GameData.java:313) at net.minecraftforge.fml.common.registry.FMLControlledNamespacedRegistry.addObjectRaw(FMLControlledNamespacedRegistry.java:586) at net.minecraftforge.fml.common.registry.FMLControlledNamespacedRegistry.add(FMLControlledNamespacedRegistry.java:484) at net.minecraftforge.fml.common.registry.FMLControlledNamespacedRegistry.register(FMLControlledNamespacedRegistry.java:822) at net.minecraftforge.fml.common.registry.GameData.register_impl(GameData.java:250) at net.minecraftforge.fml.common.registry.GameRegistry.register(GameRegistry.java:153) at com.holydevils.blocks.ModBlocks.regBlocks(ModBlocks.java:30) at com.holydevils.blocks.ModBlocks.createBlocks(ModBlocks.java:23) at com.holydevils.main.MainRegistry.preLoad(MainRegistry.java:26) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:498) at net.minecraftforge.fml.common.FMLModContainer.handleModStateEvent(FMLModContainer.java:579) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:498) at com.google.common.eventbus.EventSubscriber.handleEvent(EventSubscriber.java:74) at com.google.common.eventbus.SynchronizedEventSubscriber.handleEvent(SynchronizedEventSubscriber.java:47) at com.google.common.eventbus.EventBus.dispatch(EventBus.java:322) at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:304) at com.google.common.eventbus.EventBus.post(EventBus.java:275) at net.minecraftforge.fml.common.LoadController.sendEventToModContainer(LoadController.java:235) at net.minecraftforge.fml.common.LoadController.propogateStateMessage(LoadController.java:213) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:498) at com.google.common.eventbus.EventSubscriber.handleEvent(EventSubscriber.java:74) at com.google.common.eventbus.SynchronizedEventSubscriber.handleEvent(SynchronizedEventSubscriber.java:47) at com.google.common.eventbus.EventBus.dispatch(EventBus.java:322) at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:304) at com.google.common.eventbus.EventBus.post(EventBus.java:275) at net.minecraftforge.fml.common.LoadController.distributeStateMessage(LoadController.java:142) at net.minecraftforge.fml.common.Loader.preinitializeMods(Loader.java:593) at net.minecraftforge.fml.client.FMLClientHandler.beginMinecraftLoading(FMLClientHandler.java:255) at net.minecraft.client.Minecraft.startGame(Minecraft.java:477) -- Initialization -- Details: Stacktrace: at net.minecraft.client.Minecraft.run(Minecraft.java:386) at net.minecraft.client.main.Main.main(Main.java:118) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:498) at net.minecraft.launchwrapper.Launch.launch(Launch.java:135) at net.minecraft.launchwrapper.Launch.main(Launch.java:28) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:498) at net.minecraftforge.gradle.GradleStartCommon.launch(GradleStartCommon.java:97) at GradleStart.main(GradleStart.java:26) -- System Details -- Details: Minecraft Version: 1.10.2 Operating System: Windows 7 (amd64) version 6.1 Java Version: 1.8.0_91, Oracle Corporation Java VM Version: Java HotSpot(TM) 64-Bit Server VM (mixed mode), Oracle Corporation Memory: 742279208 bytes (707 MB) / 1037959168 bytes (989 MB) up to 1037959168 bytes (989 MB) JVM Flags: 3 total; -Xincgc -Xmx1024M -Xms1024M IntCache: cache: 0, tcache: 0, allocated: 0, tallocated: 0 FML: MCP 9.32 Powered by Forge 12.18.1.2011 4 mods loaded, 4 mods active States: 'U' = Unloaded 'L' = Loaded 'C' = Constructed 'H' = Pre-initialized 'I' = Initialized 'J' = Post-initialized 'A' = Available 'D' = Disabled 'E' = Errored UCH mcp{9.19} [Minecraft Coder Pack] (minecraft.jar) UCH FML{8.0.99.99} [Forge Mod Loader] (forgeSrc-1.10.2-12.18.1.2011.jar) UCH Forge{12.18.1.2011} [Minecraft Forge] (forgeSrc-1.10.2-12.18.1.2011.jar) UCE morethingsmod{v0.05} [More Things Mod] (bin) Loaded coremods (and transformers): GL info: ' Vendor: 'ATI Technologies Inc.' Version: '4.5.13447 Compatibility Profile Context 16.300.2311.0' Renderer: 'AMD Radeon R7 200 Series' Launched Version: 1.10.2 LWJGL: 2.9.4 OpenGL: AMD Radeon R7 200 Series GL version 4.5.13447 Compatibility Profile Context 16.300.2311.0, ATI Technologies Inc. GL Caps: Using GL 1.3 multitexturing. Using GL 1.3 texture combiners. Using framebuffer objects because OpenGL 3.0 is supported and separate blending is supported. Shaders are available because OpenGL 2.1 is supported. VBOs are available because OpenGL 1.5 is supported. Using VBOs: Yes Is Modded: Definitely; Client brand changed to 'fml,forge' Type: Client (map_client.txt) Resource Packs: Current Language: English (United Kingdom) Profiler Position: N/A (disabled) CPU: 8x AMD FX(tm)-8350 Eight-Core Processor
-
Both.
It's still crashes... just for different reasons... Of which I don't know of.
Here's the crash report:
---- Minecraft Crash Report ---- // Oh - I know what I did wrong! Time: 11/08/16 22:32 Description: Initializing game java.lang.NullPointerException: Initializing game at net.minecraftforge.fml.common.registry.GameData$ItemCallbacks.onAdd(GameData.java:324) at net.minecraftforge.fml.common.registry.GameData$ItemCallbacks.onAdd(GameData.java:313) at net.minecraftforge.fml.common.registry.FMLControlledNamespacedRegistry.addObjectRaw(FMLControlledNamespacedRegistry.java:586) at net.minecraftforge.fml.common.registry.FMLControlledNamespacedRegistry.add(FMLControlledNamespacedRegistry.java:484) at net.minecraftforge.fml.common.registry.FMLControlledNamespacedRegistry.register(FMLControlledNamespacedRegistry.java:822) at net.minecraftforge.fml.common.registry.GameData.register_impl(GameData.java:250) at net.minecraftforge.fml.common.registry.GameRegistry.register(GameRegistry.java:153) at com.holydevils.blocks.ModBlocks.regBlocks(ModBlocks.java:29) at com.holydevils.blocks.ModBlocks.createBlocks(ModBlocks.java:22) at com.holydevils.main.MainRegistry.preLoad(MainRegistry.java:26) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:498) at net.minecraftforge.fml.common.FMLModContainer.handleModStateEvent(FMLModContainer.java:579) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:498) at com.google.common.eventbus.EventSubscriber.handleEvent(EventSubscriber.java:74) at com.google.common.eventbus.SynchronizedEventSubscriber.handleEvent(SynchronizedEventSubscriber.java:47) at com.google.common.eventbus.EventBus.dispatch(EventBus.java:322) at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:304) at com.google.common.eventbus.EventBus.post(EventBus.java:275) at net.minecraftforge.fml.common.LoadController.sendEventToModContainer(LoadController.java:235) at net.minecraftforge.fml.common.LoadController.propogateStateMessage(LoadController.java:213) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:498) at com.google.common.eventbus.EventSubscriber.handleEvent(EventSubscriber.java:74) at com.google.common.eventbus.SynchronizedEventSubscriber.handleEvent(SynchronizedEventSubscriber.java:47) at com.google.common.eventbus.EventBus.dispatch(EventBus.java:322) at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:304) at com.google.common.eventbus.EventBus.post(EventBus.java:275) at net.minecraftforge.fml.common.LoadController.distributeStateMessage(LoadController.java:142) at net.minecraftforge.fml.common.Loader.preinitializeMods(Loader.java:593) at net.minecraftforge.fml.client.FMLClientHandler.beginMinecraftLoading(FMLClientHandler.java:255) at net.minecraft.client.Minecraft.startGame(Minecraft.java:477) at net.minecraft.client.Minecraft.run(Minecraft.java:386) at net.minecraft.client.main.Main.main(Main.java:118) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:498) at net.minecraft.launchwrapper.Launch.launch(Launch.java:135) at net.minecraft.launchwrapper.Launch.main(Launch.java:28) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:498) at net.minecraftforge.gradle.GradleStartCommon.launch(GradleStartCommon.java:97) at GradleStart.main(GradleStart.java:26) A detailed walkthrough of the error, its code path and all known details is as follows: --------------------------------------------------------------------------------------- -- Head -- Thread: Client thread Stacktrace: at net.minecraftforge.fml.common.registry.GameData$ItemCallbacks.onAdd(GameData.java:324) at net.minecraftforge.fml.common.registry.GameData$ItemCallbacks.onAdd(GameData.java:313) at net.minecraftforge.fml.common.registry.FMLControlledNamespacedRegistry.addObjectRaw(FMLControlledNamespacedRegistry.java:586) at net.minecraftforge.fml.common.registry.FMLControlledNamespacedRegistry.add(FMLControlledNamespacedRegistry.java:484) at net.minecraftforge.fml.common.registry.FMLControlledNamespacedRegistry.register(FMLControlledNamespacedRegistry.java:822) at net.minecraftforge.fml.common.registry.GameData.register_impl(GameData.java:250) at net.minecraftforge.fml.common.registry.GameRegistry.register(GameRegistry.java:153) at com.holydevils.blocks.ModBlocks.regBlocks(ModBlocks.java:29) at com.holydevils.blocks.ModBlocks.createBlocks(ModBlocks.java:22) at com.holydevils.main.MainRegistry.preLoad(MainRegistry.java:26) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:498) at net.minecraftforge.fml.common.FMLModContainer.handleModStateEvent(FMLModContainer.java:579) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:498) at com.google.common.eventbus.EventSubscriber.handleEvent(EventSubscriber.java:74) at com.google.common.eventbus.SynchronizedEventSubscriber.handleEvent(SynchronizedEventSubscriber.java:47) at com.google.common.eventbus.EventBus.dispatch(EventBus.java:322) at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:304) at com.google.common.eventbus.EventBus.post(EventBus.java:275) at net.minecraftforge.fml.common.LoadController.sendEventToModContainer(LoadController.java:235) at net.minecraftforge.fml.common.LoadController.propogateStateMessage(LoadController.java:213) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:498) at com.google.common.eventbus.EventSubscriber.handleEvent(EventSubscriber.java:74) at com.google.common.eventbus.SynchronizedEventSubscriber.handleEvent(SynchronizedEventSubscriber.java:47) at com.google.common.eventbus.EventBus.dispatch(EventBus.java:322) at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:304) at com.google.common.eventbus.EventBus.post(EventBus.java:275) at net.minecraftforge.fml.common.LoadController.distributeStateMessage(LoadController.java:142) at net.minecraftforge.fml.common.Loader.preinitializeMods(Loader.java:593) at net.minecraftforge.fml.client.FMLClientHandler.beginMinecraftLoading(FMLClientHandler.java:255) at net.minecraft.client.Minecraft.startGame(Minecraft.java:477) -- Initialization -- Details: Stacktrace: at net.minecraft.client.Minecraft.run(Minecraft.java:386) at net.minecraft.client.main.Main.main(Main.java:118) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:498) at net.minecraft.launchwrapper.Launch.launch(Launch.java:135) at net.minecraft.launchwrapper.Launch.main(Launch.java:28) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:498) at net.minecraftforge.gradle.GradleStartCommon.launch(GradleStartCommon.java:97) at GradleStart.main(GradleStart.java:26) -- System Details -- Details: Minecraft Version: 1.10.2 Operating System: Windows 7 (amd64) version 6.1 Java Version: 1.8.0_91, Oracle Corporation Java VM Version: Java HotSpot(TM) 64-Bit Server VM (mixed mode), Oracle Corporation Memory: 741413096 bytes (707 MB) / 1037959168 bytes (989 MB) up to 1037959168 bytes (989 MB) JVM Flags: 3 total; -Xincgc -Xmx1024M -Xms1024M IntCache: cache: 0, tcache: 0, allocated: 0, tallocated: 0 FML: MCP 9.32 Powered by Forge 12.18.1.2011 4 mods loaded, 4 mods active States: 'U' = Unloaded 'L' = Loaded 'C' = Constructed 'H' = Pre-initialized 'I' = Initialized 'J' = Post-initialized 'A' = Available 'D' = Disabled 'E' = Errored UCH mcp{9.19} [Minecraft Coder Pack] (minecraft.jar) UCH FML{8.0.99.99} [Forge Mod Loader] (forgeSrc-1.10.2-12.18.1.2011.jar) UCH Forge{12.18.1.2011} [Minecraft Forge] (forgeSrc-1.10.2-12.18.1.2011.jar) UCE morethingsmod{v0.05} [More Things Mod] (bin) Loaded coremods (and transformers): GL info: ' Vendor: 'ATI Technologies Inc.' Version: '4.5.13447 Compatibility Profile Context 16.300.2311.0' Renderer: 'AMD Radeon R7 200 Series' Launched Version: 1.10.2 LWJGL: 2.9.4 OpenGL: AMD Radeon R7 200 Series GL version 4.5.13447 Compatibility Profile Context 16.300.2311.0, ATI Technologies Inc. GL Caps: Using GL 1.3 multitexturing. Using GL 1.3 texture combiners. Using framebuffer objects because OpenGL 3.0 is supported and separate blending is supported. Shaders are available because OpenGL 2.1 is supported. VBOs are available because OpenGL 1.5 is supported. Using VBOs: Yes Is Modded: Definitely; Client brand changed to 'fml,forge' Type: Client (map_client.txt) Resource Packs: Current Language: English (United Kingdom) Profiler Position: N/A (disabled) CPU: 8x AMD FX(tm)-8350 Eight-Core Processor
And here's the ModBlocks class:
package com.holydevils.blocks; import com.holydevils.items.ModItems; import com.holydevils.main.MTCreativeTabs; import net.minecraft.block.Block; import net.minecraft.block.material.Material; import net.minecraft.item.Item; import net.minecraft.item.ItemBlock; import net.minecraftforge.fml.common.registry.GameRegistry; public class ModBlocks { public static Block industrializedWood; public static Block purpleGlowstone; public static Block hardenedIndustrializedWood; public static ItemBlock industrializedWoodItem; public static ItemBlock purpleGlowstoneItem; public static ItemBlock hardenedIndustrializedWoodItem; public static void createBlocks(){ regBlocks(industrializedWoodItem = new ItemBlock(industrializedWood),industrializedWood = new BasicBlocks("industrializedWood", Material.WOOD, 1.0F, 5.0F, "axe", 0)); regBlocks(hardenedIndustrializedWoodItem = new ItemBlock(hardenedIndustrializedWood),hardenedIndustrializedWood = new BasicBlocks("hardenedIndustrializedWood", Material.WOOD, 20.0F, 150.0F, "axe", 2)); regBlocks(purpleGlowstoneItem = new ItemBlock(purpleGlowstone),purpleGlowstone = new SpecialBlocks("purpleGlowstone", Material.GLASS, MTCreativeTabs.tabMoreThingsBlocks, 0.5F, 2.5F, 16, 0.9F, "pickaxe", 0, ModItems.purpleGlowstoneDust, 0, 2, 4)); } public static void regBlocks(ItemBlock IBlock, Block block){ GameRegistry.register(block.setRegistryName(block.getUnlocalizedName().substring(5))); GameRegistry.register(IBlock.setRegistryName(block.getRegistryName())); } }
EDIT: It will still crash the same way even if I change GameRegistry.register(IBlock.setRegistryName(block.getRegistryName())); to GameRegistry.register(IBlock.setRegistryName(block.getUnlocalizedName().substring(5)));
-
Oh... yeah. You are not creating an ItemBlock for any of your Blocks, hence Item.getItemFromBlock returns null.
Ok, so will I have to register the the Block and the ItemBlock or just the ItemBlock?
EDIT: Let me refrase that, how on earth do I fix this?
-
You are most likely trying to register your block models before even creating your Blocks.
My proxy is the last piece of code read. Here:
Main class:
package com.holydevils.main; import com.holydevils.blocks.ModBlocks; import com.holydevils.client.render.items.ItemRenderRegistry; import com.holydevils.items.ModItems; import com.holydevils.lib.StringReferences; import com.holydevils.world.ModWorld; import net.minecraftforge.fml.common.Mod; import net.minecraftforge.fml.common.Mod.EventHandler; import net.minecraftforge.fml.common.SidedProxy; import net.minecraftforge.fml.common.event.FMLInitializationEvent; import net.minecraftforge.fml.common.event.FMLPostInitializationEvent; import net.minecraftforge.fml.common.event.FMLPreInitializationEvent; import net.minecraftforge.fml.common.registry.GameRegistry; @Mod(modid = StringReferences.MODID, name =StringReferences.NAME, version =StringReferences.VERSION) public class MainRegistry { @SidedProxy(clientSide = StringReferences.CLIENTPROXY, serverSide = StringReferences.SERVERPROXY) public static ServerProxy proxyServer; public static ModWorld mWorld = new ModWorld(); @EventHandler public static void preLoad(FMLPreInitializationEvent PreEvent){ ModItems.createItems(); ModBlocks.createBlocks(); CraftingManager.craftRegister(); GameRegistry.registerWorldGenerator(mWorld, 1); proxyServer.registerRenderInfo(); } public static void load(FMLInitializationEvent Event){ } public static void postLoad(FMLPostInitializationEvent PostEvent){ } }
ServerProxy class:
package com.holydevils.main; import com.holydevils.world.ModWorld; import net.minecraftforge.fml.common.event.FMLInitializationEvent; import net.minecraftforge.fml.common.registry.GameRegistry; public class ServerProxy { public void registerRenderInfo(){ } }
Client proxy:
package com.holydevils.main; import com.holydevils.client.render.blocks.BlockRenderRegistry; import com.holydevils.client.render.items.ItemRenderRegistry; import com.holydevils.world.ModWorld; import net.minecraftforge.fml.common.registry.GameRegistry; public class ClientProxy extends ServerProxy{ @Override public void registerRenderInfo(){ ItemRenderRegistry.registerItemRenderer(); BlockRenderRegistry.registerBlockRenderer(); } }
-
Where are you calling your BlockRenderRegistry? It must be called client-side only, and after you initialize ModBlocks
I'm calling it from my client proxy.
-
After some constructive criticism from Draco18s (Sorry if I spelled it wrong) I changed my register for my blocks and items to the GameRegister.register(...) fuction.
Now, upon registering the textures for the blocks my game crashes for some reason. Here's extra info if you need it.
Crash Report:
---- Minecraft Crash Report ---- // Why is it breaking Time: 11/08/16 18:16 Description: Initializing game java.lang.NullPointerException: Initializing game at net.minecraftforge.client.model.ModelLoader.setCustomModelResourceLocation(ModelLoader.java:1094) at com.holydevils.client.render.blocks.BlockRenderRegistry.regBlockTexture(BlockRenderRegistry.java:22) at com.holydevils.client.render.blocks.BlockRenderRegistry.registerBlockRenderer(BlockRenderRegistry.java:14) at com.holydevils.main.ClientProxy.registerRenderInfo(ClientProxy.java:13) at com.holydevils.main.MainRegistry.preLoad(MainRegistry.java:32) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:498) at net.minecraftforge.fml.common.FMLModContainer.handleModStateEvent(FMLModContainer.java:579) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:498) at com.google.common.eventbus.EventSubscriber.handleEvent(EventSubscriber.java:74) at com.google.common.eventbus.SynchronizedEventSubscriber.handleEvent(SynchronizedEventSubscriber.java:47) at com.google.common.eventbus.EventBus.dispatch(EventBus.java:322) at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:304) at com.google.common.eventbus.EventBus.post(EventBus.java:275) at net.minecraftforge.fml.common.LoadController.sendEventToModContainer(LoadController.java:235) at net.minecraftforge.fml.common.LoadController.propogateStateMessage(LoadController.java:213) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:498) at com.google.common.eventbus.EventSubscriber.handleEvent(EventSubscriber.java:74) at com.google.common.eventbus.SynchronizedEventSubscriber.handleEvent(SynchronizedEventSubscriber.java:47) at com.google.common.eventbus.EventBus.dispatch(EventBus.java:322) at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:304) at com.google.common.eventbus.EventBus.post(EventBus.java:275) at net.minecraftforge.fml.common.LoadController.distributeStateMessage(LoadController.java:142) at net.minecraftforge.fml.common.Loader.preinitializeMods(Loader.java:593) at net.minecraftforge.fml.client.FMLClientHandler.beginMinecraftLoading(FMLClientHandler.java:255) at net.minecraft.client.Minecraft.startGame(Minecraft.java:477) at net.minecraft.client.Minecraft.run(Minecraft.java:386) at net.minecraft.client.main.Main.main(Main.java:118) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:498) at net.minecraft.launchwrapper.Launch.launch(Launch.java:135) at net.minecraft.launchwrapper.Launch.main(Launch.java:28) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:498) at net.minecraftforge.gradle.GradleStartCommon.launch(GradleStartCommon.java:97) at GradleStart.main(GradleStart.java:26) A detailed walkthrough of the error, its code path and all known details is as follows: --------------------------------------------------------------------------------------- -- Head -- Thread: Client thread Stacktrace: at net.minecraftforge.client.model.ModelLoader.setCustomModelResourceLocation(ModelLoader.java:1094) at com.holydevils.client.render.blocks.BlockRenderRegistry.regBlockTexture(BlockRenderRegistry.java:22) at com.holydevils.client.render.blocks.BlockRenderRegistry.registerBlockRenderer(BlockRenderRegistry.java:14) at com.holydevils.main.ClientProxy.registerRenderInfo(ClientProxy.java:13) at com.holydevils.main.MainRegistry.preLoad(MainRegistry.java:32) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:498) at net.minecraftforge.fml.common.FMLModContainer.handleModStateEvent(FMLModContainer.java:579) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:498) at com.google.common.eventbus.EventSubscriber.handleEvent(EventSubscriber.java:74) at com.google.common.eventbus.SynchronizedEventSubscriber.handleEvent(SynchronizedEventSubscriber.java:47) at com.google.common.eventbus.EventBus.dispatch(EventBus.java:322) at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:304) at com.google.common.eventbus.EventBus.post(EventBus.java:275) at net.minecraftforge.fml.common.LoadController.sendEventToModContainer(LoadController.java:235) at net.minecraftforge.fml.common.LoadController.propogateStateMessage(LoadController.java:213) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:498) at com.google.common.eventbus.EventSubscriber.handleEvent(EventSubscriber.java:74) at com.google.common.eventbus.SynchronizedEventSubscriber.handleEvent(SynchronizedEventSubscriber.java:47) at com.google.common.eventbus.EventBus.dispatch(EventBus.java:322) at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:304) at com.google.common.eventbus.EventBus.post(EventBus.java:275) at net.minecraftforge.fml.common.LoadController.distributeStateMessage(LoadController.java:142) at net.minecraftforge.fml.common.Loader.preinitializeMods(Loader.java:593) at net.minecraftforge.fml.client.FMLClientHandler.beginMinecraftLoading(FMLClientHandler.java:255) at net.minecraft.client.Minecraft.startGame(Minecraft.java:477) -- Initialization -- Details: Stacktrace: at net.minecraft.client.Minecraft.run(Minecraft.java:386) at net.minecraft.client.main.Main.main(Main.java:118) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:498) at net.minecraft.launchwrapper.Launch.launch(Launch.java:135) at net.minecraft.launchwrapper.Launch.main(Launch.java:28) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:498) at net.minecraftforge.gradle.GradleStartCommon.launch(GradleStartCommon.java:97) at GradleStart.main(GradleStart.java:26) -- System Details -- Details: Minecraft Version: 1.10.2 Operating System: Windows 7 (amd64) version 6.1 Java Version: 1.8.0_91, Oracle Corporation Java VM Version: Java HotSpot(TM) 64-Bit Server VM (mixed mode), Oracle Corporation Memory: 712786512 bytes (679 MB) / 1037959168 bytes (989 MB) up to 1037959168 bytes (989 MB) JVM Flags: 3 total; -Xincgc -Xmx1024M -Xms1024M IntCache: cache: 0, tcache: 0, allocated: 0, tallocated: 0 FML: MCP 9.32 Powered by Forge 12.18.1.2011 4 mods loaded, 4 mods active States: 'U' = Unloaded 'L' = Loaded 'C' = Constructed 'H' = Pre-initialized 'I' = Initialized 'J' = Post-initialized 'A' = Available 'D' = Disabled 'E' = Errored UCH mcp{9.19} [Minecraft Coder Pack] (minecraft.jar) UCH FML{8.0.99.99} [Forge Mod Loader] (forgeSrc-1.10.2-12.18.1.2011.jar) UCH Forge{12.18.1.2011} [Minecraft Forge] (forgeSrc-1.10.2-12.18.1.2011.jar) UCE morethingsmod{v0.05} [More Things Mod] (bin) Loaded coremods (and transformers): GL info: ' Vendor: 'ATI Technologies Inc.' Version: '4.5.13447 Compatibility Profile Context 16.300.2311.0' Renderer: 'AMD Radeon R7 200 Series' Launched Version: 1.10.2 LWJGL: 2.9.4 OpenGL: AMD Radeon R7 200 Series GL version 4.5.13447 Compatibility Profile Context 16.300.2311.0, ATI Technologies Inc. GL Caps: Using GL 1.3 multitexturing. Using GL 1.3 texture combiners. Using framebuffer objects because OpenGL 3.0 is supported and separate blending is supported. Shaders are available because OpenGL 2.1 is supported. VBOs are available because OpenGL 1.5 is supported. Using VBOs: Yes Is Modded: Definitely; Client brand changed to 'fml,forge' Type: Client (map_client.txt) Resource Packs: Current Language: English (United Kingdom) Profiler Position: N/A (disabled) CPU: 8x AMD FX(tm)-8350 Eight-Core Processor
Block Texture Render class:
package com.holydevils.client.render.blocks; import com.holydevils.blocks.ModBlocks; import com.holydevils.items.ModItems; import com.holydevils.lib.StringReferences; import net.minecraft.block.Block; import net.minecraft.client.renderer.block.model.ModelResourceLocation; import net.minecraft.item.Item; import net.minecraftforge.client.model.ModelLoader; public class BlockRenderRegistry { public static void registerBlockRenderer(){ regBlockTexture(ModBlocks.industrializedWood); regBlockTexture(ModBlocks.purpleGlowstone); regBlockTexture(ModBlocks.hardenedIndustrializedWood); } public static String modid = StringReferences.MODID; public static void regBlockTexture(Block block) { ModelLoader.setCustomModelResourceLocation(Item.getItemFromBlock(block), 0, new ModelResourceLocation(block.getRegistryName(), "inventory")); } }
ModBlocks class:
package com.holydevils.blocks; import com.holydevils.items.ModItems; import com.holydevils.main.MTCreativeTabs; import net.minecraft.block.Block; import net.minecraft.block.material.Material; import net.minecraft.item.Item; import net.minecraftforge.fml.common.registry.GameRegistry; public class ModBlocks { public static Block industrializedWood; public static Block purpleGlowstone; public static Block hardenedIndustrializedWood; public static void createBlocks(){ regBlocks(industrializedWood = new BasicBlocks("industrializedWood", Material.WOOD, 1.0F, 5.0F, "axe", 0), "industrializedWood"); regBlocks(hardenedIndustrializedWood = new BasicBlocks("hardenedIndustrializedWood", Material.WOOD, 20.0F, 150.0F, "axe", 2), "hardenedIndustrializedWood"); regBlocks(purpleGlowstone = new SpecialBlocks("purpleGlowstone", Material.GLASS, MTCreativeTabs.tabMoreThingsBlocks, 0.5F, 2.5F, 16, 0.9F, "pickaxe", 0, ModItems.purpleGlowstoneDust, 0, 2, 4), "purpleGlowstone"); } public static void regBlocks(Block block, String name){ GameRegistry.register(block.setRegistryName(name)); } }
BasicBlock class:
package com.holydevils.blocks; import com.holydevils.lib.StringReferences; import com.holydevils.main.MTCreativeTabs; import net.minecraft.block.Block; import net.minecraft.block.material.Material; public class BasicBlocks extends Block{ public BasicBlocks(String name, Material material, float hardness, float resistance, int opacity, int lightLevel, String toolClass, int toolLevel, boolean shouldTick) { super(material); this.setUnlocalizedName(name); this.setCreativeTab(MTCreativeTabs.tabMoreThingsBlocks); this.setHardness(hardness); this.setResistance(resistance); this.setLightOpacity(opacity); this.setLightLevel(lightLevel); this.setHarvestLevel(toolClass, toolLevel); this.setTickRandomly(shouldTick); } public BasicBlocks(String name, Material material, float hardness, float resistance, int opacity, float lightLevel, String toolClass, int toolLevel) { super(material); this.setUnlocalizedName(name); this.setCreativeTab(MTCreativeTabs.tabMoreThingsBlocks); this.setHardness(hardness); this.setResistance(resistance); this.setLightOpacity(opacity); this.setLightLevel(lightLevel); this.setHarvestLevel(toolClass, toolLevel); } public BasicBlocks(String name, Material material, float hardness, float resistance, String toolClass, int toolLevel) { super(material); this.setUnlocalizedName(name); this.setCreativeTab(MTCreativeTabs.tabMoreThingsBlocks); this.setHardness(hardness); this.setResistance(resistance); this.setHarvestLevel(toolClass, toolLevel); } public BasicBlocks(String name, Material material, String toolClass, int toolLevel) { super(material); this.setUnlocalizedName(name); this.setCreativeTab(MTCreativeTabs.tabMoreThingsBlocks); this.setHarvestLevel(toolClass, toolLevel); } }
-
C# had ways to mark methods as deprecated too.
Yes, but I wrote all my code aswell. Which doesn't help when making mods.
-
Yes, "Really!"... That's what @Deprecated means... it's not there for show...
Sorry! I'm used to using C# not Java!
-
For registering blocks and items I have in both item and block libraries a register fuction for when I'm registering things.
Here's the item version:
public static void regItems(Item item){ GameRegistry.registerItem(item, item.getUnlocalizedName().substring(5)); } }
Here's the block version:
public static void regBlocks(Block block){ GameRegistry.registerBlock(block, block.getUnlocalizedName().substring(5)); } }
All in All, they're very similar.
EDIT: And I've just posted for no reason it seemes. I just looked at the date of the first post and BAM! from the 9.
:P
Well if someone else is having trouble with the same thing, they could get some info out of this.
Dont use the deprecated version use the GameRegistry.register (item.setRegistryName (name) not unlocalized name
I don't see the problem with the code I gave. I use it in my 1.10.2 mod and it works perfectly.
The problem is that it will be removed in the next version of minecraft and you will need to change it anyway.
Really!? I really need to get up-to-date with the next updates then!
-
Yeah! Thanks for the help!
:D
-
For registering blocks and items I have in both item and block libraries a register fuction for when I'm registering things.
Here's the item version:
public static void regItems(Item item){ GameRegistry.registerItem(item, item.getUnlocalizedName().substring(5)); } }
Here's the block version:
public static void regBlocks(Block block){ GameRegistry.registerBlock(block, block.getUnlocalizedName().substring(5)); } }
All in All, they're very similar.
EDIT: And I've just posted for no reason it seemes. I just looked at the date of the first post and BAM! from the 9.
:P
Well if someone else is having trouble with the same thing, they could get some info out of this.
Dont use the deprecated version use the GameRegistry.register (item.setRegistryName (name) not unlocalized name
I don't see the problem with the code I gave. I use it in my 1.10.2 mod and it works perfectly.
[Solved][1.10.2] Need help with HarvestDropsEvent...
in Modder Support
Posted
It only drops the default item and yes I did remove the things that you mentioned
Updated code: