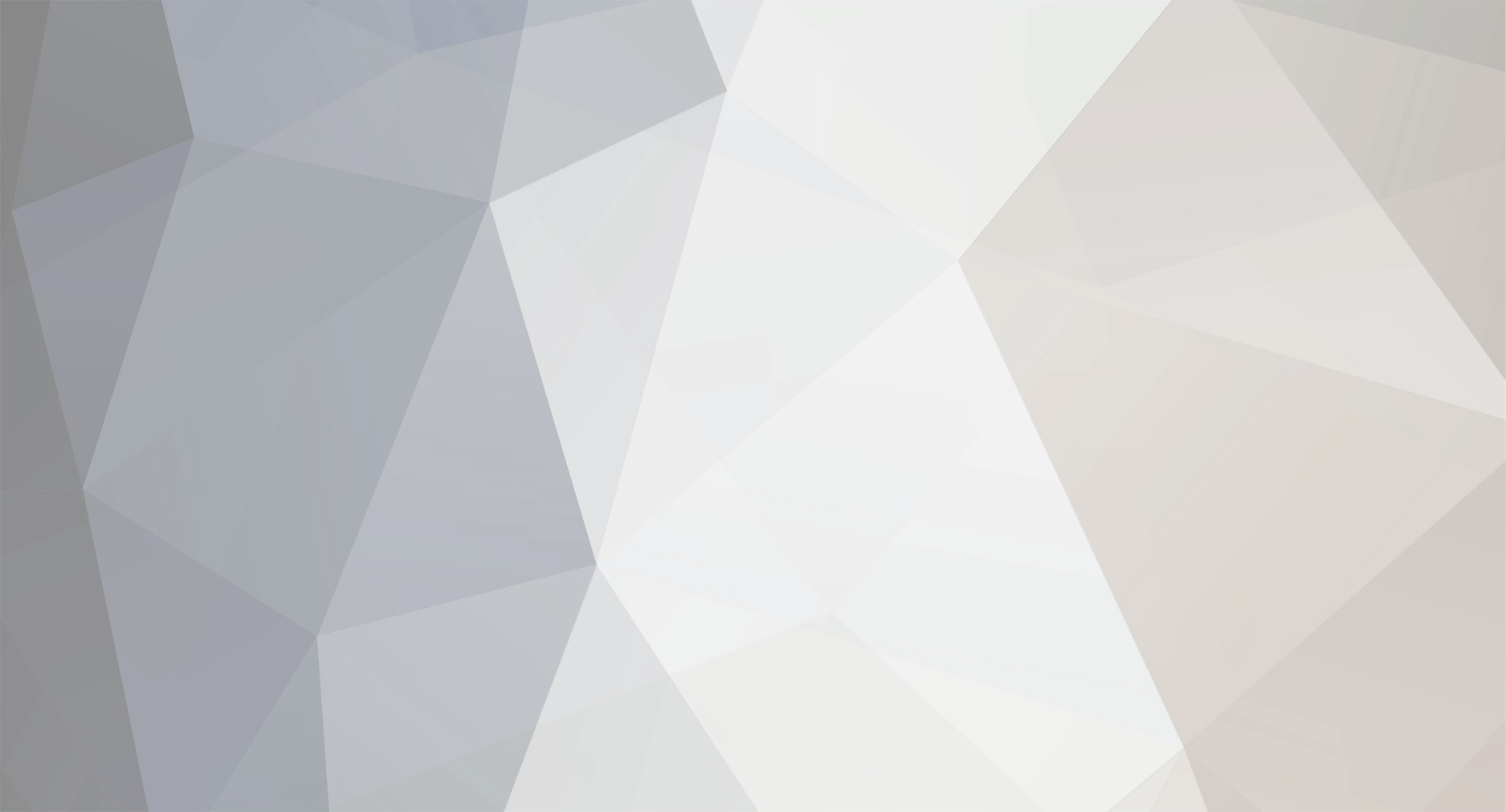
SanaRinomi
-
Posts
72 -
Joined
-
Last visited
Posts posted by SanaRinomi
-
-
For registering blocks and items I have in both item and block libraries a register fuction for when I'm registering things.
Here's the item version:
public static void regItems(Item item){ GameRegistry.registerItem(item, item.getUnlocalizedName().substring(5)); } }
Here's the block version:
public static void regBlocks(Block block){ GameRegistry.registerBlock(block, block.getUnlocalizedName().substring(5)); } }
All in All, they're very similar.
EDIT: And I've just posted for no reason it seemes. I just looked at the date of the first post and BAM! from the 9.
:P
Well if someone else is having trouble with the same thing, they could get some info out of this.
-
First you could register a world generator or you could use the generation events
I'm using for normal ores WorldGenerator, but I also have a Glowstone class because I've added purple glowstone.
Here's my ModWorld class:
package com.holydevils.world; import java.util.Random; import com.holydevils.world.special.PurpleGlowstoneNetherGenOne; import com.holydevils.world.special.PurpleGlowstoneNetherGenTwo; import net.minecraft.server.MinecraftServer; import net.minecraft.util.math.BlockPos; import net.minecraft.world.World; import net.minecraft.world.chunk.IChunkGenerator; import net.minecraft.world.chunk.IChunkProvider; import net.minecraft.world.gen.feature.WorldGenerator; import net.minecraftforge.fml.common.IWorldGenerator; import net.minecraftforge.fml.common.registry.GameRegistry; public class ModWorld implements IWorldGenerator{ public static void worldRegistry(){ } public static void initializeWorldGen(){ generateSpecial(); } public static void generateSpecial(World world){ switch(world.provider.getDimension()){ case -1: //Nether new PurpleGlowstoneNetherGenOne(); new PurpleGlowstoneNetherGenTwo(); break; case 0: //Overworld break; case 1: //End break; } } @Override public void generate(Random random, int chunkX, int chunkZ, World world, IChunkGenerator chunkGenerator, IChunkProvider chunkProvider) { switch(world.provider.getDimension()){ case -1: //Nether new PurpleGlowstoneNetherGenOne(); new PurpleGlowstoneNetherGenTwo(); break; case 0: //Overworld break; case 1: //End break; } } private void registerWorldGen(WorldGenerator worldGen, World world, Random random, int chunkX, int chunkZ, int chanceToSpawn, int minY, int maxY){ if(minY < 0 || maxY >world.getHeight() || minY > maxY) throw new IllegalArgumentException("Minimum or Maximum Height out of bounds"); int layerDiff = maxY - minY + 1; for(int i = 0; i < chanceToSpawn; i++){ int x = chunkX * 16 + random.nextInt(16); int y = minY + random.nextInt(layerDiff); int z = chunkZ * 16 + random.nextInt(16); worldGen.generate(world, random, new BlockPos(x, y, z)); } } }
generateSpecial will give off errors but that's because I hadn't put in World and that why I consulted you to make it both server and client side.
And these are both Glowstone Generation classes:
PurpleGlowstoneNetherGenOne class:
package com.holydevils.world.special; import java.util.Random; import com.holydevils.blocks.ModBlocks; import net.minecraft.init.Blocks; import net.minecraft.util.EnumFacing; import net.minecraft.util.math.BlockPos; import net.minecraft.world.World; import net.minecraft.world.gen.feature.WorldGenerator; public class PurpleGlowstoneNetherGenOne extends WorldGenerator { public boolean generate(World worldIn, Random rand, BlockPos position) { if (!worldIn.isAirBlock(position)) { return false; } else if (worldIn.getBlockState(position.up()).getBlock() != Blocks.NETHERRACK) { return false; } else { worldIn.setBlockState(position, ModBlocks.purpleGlowstone.getDefaultState(), 2); for (int i = 0; i < 1500; ++i) { BlockPos blockpos = position.add(rand.nextInt( - rand.nextInt(, -rand.nextInt(12), rand.nextInt( - rand.nextInt(); if (worldIn.isAirBlock(blockpos)) { int j = 0; for (EnumFacing enumfacing : EnumFacing.values()) { if (worldIn.getBlockState(blockpos.offset(enumfacing)).getBlock() == ModBlocks.purpleGlowstone) { ++j; } if (j > 1) { break; } } if (j == 1) { worldIn.setBlockState(blockpos, ModBlocks.purpleGlowstone.getDefaultState(), 2); } } } return true; } } }
PurpleGlowstoneNetherGenTwo class:
package com.holydevils.world.special; import java.util.Random; import com.holydevils.blocks.ModBlocks; import net.minecraft.block.Block; import net.minecraft.init.Blocks; import net.minecraft.util.EnumFacing; import net.minecraft.util.math.BlockPos; import net.minecraft.world.World; import net.minecraft.world.gen.feature.WorldGenerator; public class PurpleGlowstoneNetherGenTwo extends WorldGenerator { public boolean generate(World worldIn, Random rand, BlockPos position) { if (!worldIn.isAirBlock(position)) { return false; } else if (worldIn.getBlockState(position.up()).getBlock() != Blocks.NETHERRACK) { return false; } else { worldIn.setBlockState(position, ModBlocks.purpleGlowstone.getDefaultState(), 2); for (int i = 0; i < 1500; ++i) { BlockPos blockpos = position.add(rand.nextInt( - rand.nextInt(, -rand.nextInt(12), rand.nextInt( - rand.nextInt(); if (worldIn.isAirBlock(blockpos)) { int j = 0; for (EnumFacing enumfacing : EnumFacing.values()) { if (worldIn.getBlockState(blockpos.offset(enumfacing)).getBlock() == ModBlocks.purpleGlowstone) { ++j; } if (j > 1) { break; } } if (j == 1) { worldIn.setBlockState(blockpos, ModBlocks.purpleGlowstone.getDefaultState(), 2); } } } return true; } } }
If you wonder why I have two, it's because Minecrafts normal Glowstone has two, so to be on the safe side, I implemented both.
-
How can I get World so that I can generate glowstone in the Nether or Ores overworld, while keeping the compatibility both server and client side? Thanks in advanced!
-
Thank you for the nformation, it really helped!
-
For the normal variant you need [] as well as {}
Could you send me an example?
-
If doing this from the proxy: Make sure that the proxy actually gets called.
Blocks & Items should be registered in preInit, just like the renderer.
Any errors in console after launching client?
The proxy is fully fuctioning and in PreInit. The console gave me these errors:
[16:38:27] [Client thread/ERROR] [FML]: Exception loading model for variant morethingsmod:industrializedWood#normal for blockstate "morethingsmod:industrializedWood" net.minecraftforge.client.model.ModelLoaderRegistry$LoaderException: Exception loading model morethingsmod:industrializedWood#normal with loader VariantLoader.INSTANCE, skipping at net.minecraftforge.client.model.ModelLoaderRegistry.getModel(ModelLoaderRegistry.java:153) ~[ModelLoaderRegistry.class:?] at net.minecraftforge.client.model.ModelLoader.registerVariant(ModelLoader.java:241) ~[ModelLoader.class:?] at net.minecraft.client.renderer.block.model.ModelBakery.loadBlock(ModelBakery.java:145) ~[ModelBakery.class:?] at net.minecraftforge.client.model.ModelLoader.loadBlocks(ModelLoader.java:229) ~[ModelLoader.class:?] at net.minecraftforge.client.model.ModelLoader.setupModelRegistry(ModelLoader.java:146) ~[ModelLoader.class:?] at net.minecraft.client.renderer.block.model.ModelManager.onResourceManagerReload(ModelManager.java:28) [ModelManager.class:?] at net.minecraft.client.resources.SimpleReloadableResourceManager.registerReloadListener(SimpleReloadableResourceManager.java:122) [simpleReloadableResourceManager.class:?] at net.minecraft.client.Minecraft.startGame(Minecraft.java:540) [Minecraft.class:?] at net.minecraft.client.Minecraft.run(Minecraft.java:386) [Minecraft.class:?] at net.minecraft.client.main.Main.main(Main.java:118) [Main.class:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_91] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_91] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_91] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_91] at net.minecraft.launchwrapper.Launch.launch(Launch.java:135) [launchwrapper-1.12.jar:?] at net.minecraft.launchwrapper.Launch.main(Launch.java:28) [launchwrapper-1.12.jar:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_91] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_91] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_91] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_91] at net.minecraftforge.gradle.GradleStartCommon.launch(GradleStartCommon.java:97) [start/:?] at GradleStart.main(GradleStart.java:26) [start/:?] Caused by: net.minecraftforge.client.model.ModelLoaderRegistry$LoaderException: Exception loading model morethingsmod:block/blocks/industrializedWood with loader VanillaLoader.INSTANCE, skipping at net.minecraftforge.client.model.ModelLoaderRegistry.getModel(ModelLoaderRegistry.java:153) ~[ModelLoaderRegistry.class:?] at net.minecraftforge.client.model.ModelLoader$WeightedRandomModel.<init>(ModelLoader.java:752) ~[ModelLoader$WeightedRandomModel.class:?] at net.minecraftforge.client.model.ModelLoader$VariantLoader.loadModel(ModelLoader.java:1184) ~[ModelLoader$VariantLoader.class:?] at net.minecraftforge.client.model.ModelLoaderRegistry.getModel(ModelLoaderRegistry.java:149) ~[ModelLoaderRegistry.class:?] ... 21 more Caused by: java.io.FileNotFoundException: morethingsmod:models/block/blocks/industrializedWood.json at net.minecraft.client.resources.FallbackResourceManager.getResource(FallbackResourceManager.java:68) ~[FallbackResourceManager.class:?] at net.minecraft.client.resources.SimpleReloadableResourceManager.getResource(SimpleReloadableResourceManager.java:65) ~[simpleReloadableResourceManager.class:?] at net.minecraft.client.renderer.block.model.ModelBakery.loadModel(ModelBakery.java:311) ~[ModelBakery.class:?] at net.minecraftforge.client.model.ModelLoader.access$1100(ModelLoader.java:118) ~[ModelLoader.class:?] at net.minecraftforge.client.model.ModelLoader$VanillaLoader.loadModel(ModelLoader.java:868) ~[ModelLoader$VanillaLoader.class:?] at net.minecraftforge.client.model.ModelLoaderRegistry.getModel(ModelLoaderRegistry.java:149) ~[ModelLoaderRegistry.class:?] at net.minecraftforge.client.model.ModelLoader$WeightedRandomModel.<init>(ModelLoader.java:752) ~[ModelLoader$WeightedRandomModel.class:?] at net.minecraftforge.client.model.ModelLoader$VariantLoader.loadModel(ModelLoader.java:1184) ~[ModelLoader$VariantLoader.class:?] at net.minecraftforge.client.model.ModelLoaderRegistry.getModel(ModelLoaderRegistry.java:149) ~[ModelLoaderRegistry.class:?] ... 21 more
[16:38:35] [Client thread/ERROR] [FML]: Exception loading model for variant morethingsmod:industrializedWood#normal for blockstate "morethingsmod:industrializedWood" net.minecraftforge.client.model.ModelLoaderRegistry$LoaderException: Exception loading model morethingsmod:industrializedWood#normal with loader VariantLoader.INSTANCE, skipping at net.minecraftforge.client.model.ModelLoaderRegistry.getModel(ModelLoaderRegistry.java:153) ~[ModelLoaderRegistry.class:?] at net.minecraftforge.client.model.ModelLoader.registerVariant(ModelLoader.java:241) ~[ModelLoader.class:?] at net.minecraft.client.renderer.block.model.ModelBakery.loadBlock(ModelBakery.java:145) ~[ModelBakery.class:?] at net.minecraftforge.client.model.ModelLoader.loadBlocks(ModelLoader.java:229) ~[ModelLoader.class:?] at net.minecraftforge.client.model.ModelLoader.setupModelRegistry(ModelLoader.java:146) ~[ModelLoader.class:?] at net.minecraft.client.renderer.block.model.ModelManager.onResourceManagerReload(ModelManager.java:28) [ModelManager.class:?] at net.minecraft.client.resources.SimpleReloadableResourceManager.notifyReloadListeners(SimpleReloadableResourceManager.java:132) [simpleReloadableResourceManager.class:?] at net.minecraft.client.resources.SimpleReloadableResourceManager.reloadResources(SimpleReloadableResourceManager.java:113) [simpleReloadableResourceManager.class:?] at net.minecraft.client.Minecraft.refreshResources(Minecraft.java:799) [Minecraft.class:?] at net.minecraftforge.fml.client.FMLClientHandler.finishMinecraftLoading(FMLClientHandler.java:338) [FMLClientHandler.class:?] at net.minecraft.client.Minecraft.startGame(Minecraft.java:561) [Minecraft.class:?] at net.minecraft.client.Minecraft.run(Minecraft.java:386) [Minecraft.class:?] at net.minecraft.client.main.Main.main(Main.java:118) [Main.class:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_91] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_91] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_91] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_91] at net.minecraft.launchwrapper.Launch.launch(Launch.java:135) [launchwrapper-1.12.jar:?] at net.minecraft.launchwrapper.Launch.main(Launch.java:28) [launchwrapper-1.12.jar:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.8.0_91] at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) ~[?:1.8.0_91] at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) ~[?:1.8.0_91] at java.lang.reflect.Method.invoke(Method.java:498) ~[?:1.8.0_91] at net.minecraftforge.gradle.GradleStartCommon.launch(GradleStartCommon.java:97) [start/:?] at GradleStart.main(GradleStart.java:26) [start/:?] Caused by: net.minecraftforge.client.model.ModelLoaderRegistry$LoaderException: Exception loading model morethingsmod:block/blocks/industrializedWood with loader VanillaLoader.INSTANCE, skipping at net.minecraftforge.client.model.ModelLoaderRegistry.getModel(ModelLoaderRegistry.java:153) ~[ModelLoaderRegistry.class:?] at net.minecraftforge.client.model.ModelLoader$WeightedRandomModel.<init>(ModelLoader.java:752) ~[ModelLoader$WeightedRandomModel.class:?] at net.minecraftforge.client.model.ModelLoader$VariantLoader.loadModel(ModelLoader.java:1184) ~[ModelLoader$VariantLoader.class:?] at net.minecraftforge.client.model.ModelLoaderRegistry.getModel(ModelLoaderRegistry.java:149) ~[ModelLoaderRegistry.class:?] ... 24 more Caused by: java.io.FileNotFoundException: morethingsmod:models/block/blocks/industrializedWood.json at net.minecraft.client.resources.FallbackResourceManager.getResource(FallbackResourceManager.java:68) ~[FallbackResourceManager.class:?] at net.minecraft.client.resources.SimpleReloadableResourceManager.getResource(SimpleReloadableResourceManager.java:65) ~[simpleReloadableResourceManager.class:?] at net.minecraft.client.renderer.block.model.ModelBakery.loadModel(ModelBakery.java:311) ~[ModelBakery.class:?] at net.minecraftforge.client.model.ModelLoader.access$1100(ModelLoader.java:118) ~[ModelLoader.class:?] at net.minecraftforge.client.model.ModelLoader$VanillaLoader.loadModel(ModelLoader.java:868) ~[ModelLoader$VanillaLoader.class:?] at net.minecraftforge.client.model.ModelLoaderRegistry.getModel(ModelLoaderRegistry.java:149) ~[ModelLoaderRegistry.class:?] at net.minecraftforge.client.model.ModelLoader$WeightedRandomModel.<init>(ModelLoader.java:752) ~[ModelLoader$WeightedRandomModel.class:?] at net.minecraftforge.client.model.ModelLoader$VariantLoader.loadModel(ModelLoader.java:1184) ~[ModelLoader$VariantLoader.class:?] at net.minecraftforge.client.model.ModelLoaderRegistry.getModel(ModelLoaderRegistry.java:149) ~[ModelLoaderRegistry.class:?] ... 24 more
-
Even with blockstates JSON it had no textures. Here's the file:
{ "variants": { "normal": { "model": "morethingsmod:blocks/industrializedWood" } } }
-
I haven't managed to find a help file or whatever on the ModelLoader fuction so for me it's been alot of trial and error mixed in with help from other users when I got some errors.
My problem now is how to render the block texture in the World. I can see the texture on the item, but having a hard time finding something that loads it into the world. Here's the code:
Block Rendering Class:
package com.holydevils.client.render.blocks; import com.holydevils.blocks.ModBlocks; import com.holydevils.items.ModItems; import com.holydevils.lib.StringReferences; import net.minecraft.block.Block; import net.minecraft.client.renderer.block.model.ModelResourceLocation; import net.minecraft.item.Item; import net.minecraftforge.client.model.ModelLoader; public class BlockRenderRegistry { public static void registerBlockRenderer(){ regBlockTexture(ModBlocks.industrializedWood); } public static String modid = StringReferences.MODID; public static void regBlockTexture(Block block) { ModelLoader.setCustomModelResourceLocation(Item.getItemFromBlock(block), 0, new ModelResourceLocation(block.getRegistryName(), "inventory")); } }
Block .JSON
{ "parent": "block/cube_all", "textures": { "all": "morethingsmod:blocks/industrializedWood" } }
-
Thank you very much for helping me with this!
-
After you said that I went to my createItem code to find one of the stupidest and mostembarassing errors I had ever done
and for some reason Eclipse didn't pick up on it.
-
Yes, why?
-
Ok, well... I've updated my code and I've tried it two different ways. One way was calling he proxyServer on Initialization and the other one PreInitialization.
The fist way didn't even execute the command to load the texture, and the second instance crashed my game. I'll provide you with more info below.
MainRegistry.java
package com.holydevils.main; import com.holydevils.blocks.ModBlocks; import com.holydevils.client.render.items.ItemRenderRegistry; import com.holydevils.items.ModItems; import com.holydevils.lib.StringReferences; import net.minecraftforge.fml.common.Mod; import net.minecraftforge.fml.common.Mod.EventHandler; import net.minecraftforge.fml.common.SidedProxy; import net.minecraftforge.fml.common.event.FMLInitializationEvent; import net.minecraftforge.fml.common.event.FMLPostInitializationEvent; import net.minecraftforge.fml.common.event.FMLPreInitializationEvent; @Mod(modid = StringReferences.MODID, name =StringReferences.NAME, version =StringReferences.VERSION) public class MainRegistry { @SidedProxy(clientSide = StringReferences.CLIENTPROXY, serverSide = StringReferences.SERVERPROXY) public static ServerProxy proxyServer; @EventHandler public static void preLoad(FMLPreInitializationEvent PreEvent){ ModItems.createItems(); ModBlocks.createBlocks(); proxyServer.registerRenderInfo(); } public static void load(FMLInitializationEvent Event){ } public static void postLoad(FMLPostInitializationEvent PostEvent){ } }
ServerProxy.java
package com.holydevils.main; public class ServerProxy { public void registerRenderInfo(){ } }
ClientProxy.java
package com.holydevils.main; import com.holydevils.client.render.items.ItemRenderRegistry; public class ClientProxy extends ServerProxy{ @Override public void registerRenderInfo(){ ItemRenderRegistry.registerItemRenderer(); } }
Crash Report
---- Minecraft Crash Report ---- // Sorry Time: 9/08/16 12:57 Description: Initializing game java.lang.NullPointerException: Initializing game at com.holydevils.client.render.items.ItemRenderRegistry.regItemTexture(ItemRenderRegistry.java:19) at com.holydevils.client.render.items.ItemRenderRegistry.registerItemRenderer(ItemRenderRegistry.java:13) at com.holydevils.main.ClientProxy.registerRenderInfo(ClientProxy.java:7) at com.holydevils.main.MainRegistry.preLoad(MainRegistry.java:24) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:498) at net.minecraftforge.fml.common.FMLModContainer.handleModStateEvent(FMLModContainer.java:579) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:498) at com.google.common.eventbus.EventSubscriber.handleEvent(EventSubscriber.java:74) at com.google.common.eventbus.SynchronizedEventSubscriber.handleEvent(SynchronizedEventSubscriber.java:47) at com.google.common.eventbus.EventBus.dispatch(EventBus.java:322) at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:304) at com.google.common.eventbus.EventBus.post(EventBus.java:275) at net.minecraftforge.fml.common.LoadController.sendEventToModContainer(LoadController.java:235) at net.minecraftforge.fml.common.LoadController.propogateStateMessage(LoadController.java:213) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:498) at com.google.common.eventbus.EventSubscriber.handleEvent(EventSubscriber.java:74) at com.google.common.eventbus.SynchronizedEventSubscriber.handleEvent(SynchronizedEventSubscriber.java:47) at com.google.common.eventbus.EventBus.dispatch(EventBus.java:322) at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:304) at com.google.common.eventbus.EventBus.post(EventBus.java:275) at net.minecraftforge.fml.common.LoadController.distributeStateMessage(LoadController.java:142) at net.minecraftforge.fml.common.Loader.preinitializeMods(Loader.java:593) at net.minecraftforge.fml.client.FMLClientHandler.beginMinecraftLoading(FMLClientHandler.java:255) at net.minecraft.client.Minecraft.startGame(Minecraft.java:477) at net.minecraft.client.Minecraft.run(Minecraft.java:386) at net.minecraft.client.main.Main.main(Main.java:118) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:498) at net.minecraft.launchwrapper.Launch.launch(Launch.java:135) at net.minecraft.launchwrapper.Launch.main(Launch.java:28) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:498) at net.minecraftforge.gradle.GradleStartCommon.launch(GradleStartCommon.java:97) at GradleStart.main(GradleStart.java:26) A detailed walkthrough of the error, its code path and all known details is as follows: --------------------------------------------------------------------------------------- -- Head -- Thread: Client thread Stacktrace: at com.holydevils.client.render.items.ItemRenderRegistry.regItemTexture(ItemRenderRegistry.java:19) at com.holydevils.client.render.items.ItemRenderRegistry.registerItemRenderer(ItemRenderRegistry.java:13) at com.holydevils.main.ClientProxy.registerRenderInfo(ClientProxy.java:7) at com.holydevils.main.MainRegistry.preLoad(MainRegistry.java:24) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:498) at net.minecraftforge.fml.common.FMLModContainer.handleModStateEvent(FMLModContainer.java:579) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:498) at com.google.common.eventbus.EventSubscriber.handleEvent(EventSubscriber.java:74) at com.google.common.eventbus.SynchronizedEventSubscriber.handleEvent(SynchronizedEventSubscriber.java:47) at com.google.common.eventbus.EventBus.dispatch(EventBus.java:322) at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:304) at com.google.common.eventbus.EventBus.post(EventBus.java:275) at net.minecraftforge.fml.common.LoadController.sendEventToModContainer(LoadController.java:235) at net.minecraftforge.fml.common.LoadController.propogateStateMessage(LoadController.java:213) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:498) at com.google.common.eventbus.EventSubscriber.handleEvent(EventSubscriber.java:74) at com.google.common.eventbus.SynchronizedEventSubscriber.handleEvent(SynchronizedEventSubscriber.java:47) at com.google.common.eventbus.EventBus.dispatch(EventBus.java:322) at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:304) at com.google.common.eventbus.EventBus.post(EventBus.java:275) at net.minecraftforge.fml.common.LoadController.distributeStateMessage(LoadController.java:142) at net.minecraftforge.fml.common.Loader.preinitializeMods(Loader.java:593) at net.minecraftforge.fml.client.FMLClientHandler.beginMinecraftLoading(FMLClientHandler.java:255) at net.minecraft.client.Minecraft.startGame(Minecraft.java:477) -- Initialization -- Details: Stacktrace: at net.minecraft.client.Minecraft.run(Minecraft.java:386) at net.minecraft.client.main.Main.main(Main.java:118) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:498) at net.minecraft.launchwrapper.Launch.launch(Launch.java:135) at net.minecraft.launchwrapper.Launch.main(Launch.java:28) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:498) at net.minecraftforge.gradle.GradleStartCommon.launch(GradleStartCommon.java:97) at GradleStart.main(GradleStart.java:26) -- System Details -- Details: Minecraft Version: 1.10.2 Operating System: Windows 7 (amd64) version 6.1 Java Version: 1.8.0_91, Oracle Corporation Java VM Version: Java HotSpot(TM) 64-Bit Server VM (mixed mode), Oracle Corporation Memory: 714655696 bytes (681 MB) / 1037959168 bytes (989 MB) up to 1037959168 bytes (989 MB) JVM Flags: 3 total; -Xincgc -Xmx1024M -Xms1024M IntCache: cache: 0, tcache: 0, allocated: 0, tallocated: 0 FML: MCP 9.32 Powered by Forge 12.18.1.2011 4 mods loaded, 4 mods active States: 'U' = Unloaded 'L' = Loaded 'C' = Constructed 'H' = Pre-initialized 'I' = Initialized 'J' = Post-initialized 'A' = Available 'D' = Disabled 'E' = Errored UCH mcp{9.19} [Minecraft Coder Pack] (minecraft.jar) UCH FML{8.0.99.99} [Forge Mod Loader] (forgeSrc-1.10.2-12.18.1.2011.jar) UCH Forge{12.18.1.2011} [Minecraft Forge] (forgeSrc-1.10.2-12.18.1.2011.jar) UCE morethingsmod{v0.01} [More Things Mod] (bin) Loaded coremods (and transformers): GL info: ' Vendor: 'ATI Technologies Inc.' Version: '4.5.13447 Compatibility Profile Context 16.300.2311.0' Renderer: 'AMD Radeon R7 200 Series' Launched Version: 1.10.2 LWJGL: 2.9.4 OpenGL: AMD Radeon R7 200 Series GL version 4.5.13447 Compatibility Profile Context 16.300.2311.0, ATI Technologies Inc. GL Caps: Using GL 1.3 multitexturing. Using GL 1.3 texture combiners. Using framebuffer objects because OpenGL 3.0 is supported and separate blending is supported. Shaders are available because OpenGL 2.1 is supported. VBOs are available because OpenGL 1.5 is supported. Using VBOs: Yes Is Modded: Definitely; Client brand changed to 'fml,forge' Type: Client (map_client.txt) Resource Packs: Current Language: English (US) Profiler Position: N/A (disabled) CPU: 8x AMD FX(tm)-8350 Eight-Core Processor
-
Here is my MainRegistry class:
package com.holydevils.main; import com.holydevils.blocks.ModBlocks; import com.holydevils.client.render.items.ItemRenderRegistry; import com.holydevils.items.ModItems; import com.holydevils.lib.StringReferences; import net.minecraftforge.fml.common.Mod; import net.minecraftforge.fml.common.Mod.EventHandler; import net.minecraftforge.fml.common.SidedProxy; import net.minecraftforge.fml.common.event.FMLInitializationEvent; import net.minecraftforge.fml.common.event.FMLPostInitializationEvent; import net.minecraftforge.fml.common.event.FMLPreInitializationEvent; @Mod(modid = StringReferences.MODID, name =StringReferences.NAME, version =StringReferences.VERSION) public class MainRegistry { @SidedProxy(clientSide = StringReferences.CLIENTPROXY, serverSide = StringReferences.SERVERPROXY) public static ServerProxy proxyServer; public static ClientProxy proxyClient; @EventHandler public static void preLoad(FMLPreInitializationEvent PreEvent){ ModItems.createItems(); ModBlocks.createBlocks(); ItemRenderRegistry.registerItemRenderer(); } public static void load(FMLInitializationEvent Event){ proxyClient.registerRenderInfo(); } public static void postLoad(FMLPostInitializationEvent PostEvent){ } }
-
Here's the crash log as requested:
---- Minecraft Crash Report ---- // Don't do that. Time: 9/08/16 12:18 Description: Initializing game java.lang.NullPointerException: Initializing game at com.holydevils.main.MainRegistry.preLoad(MainRegistry.java:26) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:498) at net.minecraftforge.fml.common.FMLModContainer.handleModStateEvent(FMLModContainer.java:579) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:498) at com.google.common.eventbus.EventSubscriber.handleEvent(EventSubscriber.java:74) at com.google.common.eventbus.SynchronizedEventSubscriber.handleEvent(SynchronizedEventSubscriber.java:47) at com.google.common.eventbus.EventBus.dispatch(EventBus.java:322) at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:304) at com.google.common.eventbus.EventBus.post(EventBus.java:275) at net.minecraftforge.fml.common.LoadController.sendEventToModContainer(LoadController.java:235) at net.minecraftforge.fml.common.LoadController.propogateStateMessage(LoadController.java:213) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:498) at com.google.common.eventbus.EventSubscriber.handleEvent(EventSubscriber.java:74) at com.google.common.eventbus.SynchronizedEventSubscriber.handleEvent(SynchronizedEventSubscriber.java:47) at com.google.common.eventbus.EventBus.dispatch(EventBus.java:322) at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:304) at com.google.common.eventbus.EventBus.post(EventBus.java:275) at net.minecraftforge.fml.common.LoadController.distributeStateMessage(LoadController.java:142) at net.minecraftforge.fml.common.Loader.preinitializeMods(Loader.java:593) at net.minecraftforge.fml.client.FMLClientHandler.beginMinecraftLoading(FMLClientHandler.java:255) at net.minecraft.client.Minecraft.startGame(Minecraft.java:477) at net.minecraft.client.Minecraft.run(Minecraft.java:386) at net.minecraft.client.main.Main.main(Main.java:118) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:498) at net.minecraft.launchwrapper.Launch.launch(Launch.java:135) at net.minecraft.launchwrapper.Launch.main(Launch.java:28) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:498) at net.minecraftforge.gradle.GradleStartCommon.launch(GradleStartCommon.java:97) at GradleStart.main(GradleStart.java:26) A detailed walkthrough of the error, its code path and all known details is as follows: --------------------------------------------------------------------------------------- -- Head -- Thread: Client thread Stacktrace: at com.holydevils.main.MainRegistry.preLoad(MainRegistry.java:26) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:498) at net.minecraftforge.fml.common.FMLModContainer.handleModStateEvent(FMLModContainer.java:579) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:498) at com.google.common.eventbus.EventSubscriber.handleEvent(EventSubscriber.java:74) at com.google.common.eventbus.SynchronizedEventSubscriber.handleEvent(SynchronizedEventSubscriber.java:47) at com.google.common.eventbus.EventBus.dispatch(EventBus.java:322) at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:304) at com.google.common.eventbus.EventBus.post(EventBus.java:275) at net.minecraftforge.fml.common.LoadController.sendEventToModContainer(LoadController.java:235) at net.minecraftforge.fml.common.LoadController.propogateStateMessage(LoadController.java:213) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:498) at com.google.common.eventbus.EventSubscriber.handleEvent(EventSubscriber.java:74) at com.google.common.eventbus.SynchronizedEventSubscriber.handleEvent(SynchronizedEventSubscriber.java:47) at com.google.common.eventbus.EventBus.dispatch(EventBus.java:322) at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:304) at com.google.common.eventbus.EventBus.post(EventBus.java:275) at net.minecraftforge.fml.common.LoadController.distributeStateMessage(LoadController.java:142) at net.minecraftforge.fml.common.Loader.preinitializeMods(Loader.java:593) at net.minecraftforge.fml.client.FMLClientHandler.beginMinecraftLoading(FMLClientHandler.java:255) at net.minecraft.client.Minecraft.startGame(Minecraft.java:477) -- Initialization -- Details: Stacktrace: at net.minecraft.client.Minecraft.run(Minecraft.java:386) at net.minecraft.client.main.Main.main(Main.java:118) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:498) at net.minecraft.launchwrapper.Launch.launch(Launch.java:135) at net.minecraft.launchwrapper.Launch.main(Launch.java:28) at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:62) at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43) at java.lang.reflect.Method.invoke(Method.java:498) at net.minecraftforge.gradle.GradleStartCommon.launch(GradleStartCommon.java:97) at GradleStart.main(GradleStart.java:26) -- System Details -- Details: Minecraft Version: 1.10.2 Operating System: Windows 7 (amd64) version 6.1 Java Version: 1.8.0_91, Oracle Corporation Java VM Version: Java HotSpot(TM) 64-Bit Server VM (mixed mode), Oracle Corporation Memory: 741533296 bytes (707 MB) / 1037959168 bytes (989 MB) up to 1037959168 bytes (989 MB) JVM Flags: 3 total; -Xincgc -Xmx1024M -Xms1024M IntCache: cache: 0, tcache: 0, allocated: 0, tallocated: 0 FML: MCP 9.32 Powered by Forge 12.18.1.2011 4 mods loaded, 4 mods active States: 'U' = Unloaded 'L' = Loaded 'C' = Constructed 'H' = Pre-initialized 'I' = Initialized 'J' = Post-initialized 'A' = Available 'D' = Disabled 'E' = Errored UCH mcp{9.19} [Minecraft Coder Pack] (minecraft.jar) UCH FML{8.0.99.99} [Forge Mod Loader] (forgeSrc-1.10.2-12.18.1.2011.jar) UCH Forge{12.18.1.2011} [Minecraft Forge] (forgeSrc-1.10.2-12.18.1.2011.jar) UCE morethingsmod{v0.01} [More Things Mod] (bin) Loaded coremods (and transformers): GL info: ' Vendor: 'ATI Technologies Inc.' Version: '4.5.13447 Compatibility Profile Context 16.300.2311.0' Renderer: 'AMD Radeon R7 200 Series' Launched Version: 1.10.2 LWJGL: 2.9.4 OpenGL: AMD Radeon R7 200 Series GL version 4.5.13447 Compatibility Profile Context 16.300.2311.0, ATI Technologies Inc. GL Caps: Using GL 1.3 multitexturing. Using GL 1.3 texture combiners. Using framebuffer objects because OpenGL 3.0 is supported and separate blending is supported. Shaders are available because OpenGL 2.1 is supported. VBOs are available because OpenGL 1.5 is supported. Using VBOs: Yes Is Modded: Definitely; Client brand changed to 'fml,forge' Type: Client (map_client.txt) Resource Packs: Current Language: English (US) Profiler Position: N/A (disabled) CPU: 8x AMD FX(tm)-8350 Eight-Core Processor
-
I've tried with both the ModelLoader and the default minecraft way! Even trying it with and without client proxy.
Here's the code I use to load the textures to the item:
public class ItemRenderRegistry { public static void registerItemRenderer(){ regItemTexture(ModItems.obsidianStick); } public static String modid = StringReferences.MODID; public static void regItemTexture(Item item) { ModelLoader.setCustomModelResourceLocation(item, 0, new ModelResourceLocation(item.getRegistryName(), "inventory")); } }
Please help.
-
Given?
I'll just link you the "Getting Started" documentation: http://mcforge.readthedocs.io/en/latest/gettingstarted/
I'm so sorry for the trouble Ive caused! Thank you! I downloaded the one straight from GitHUB and didn't realize that it wasn't a good download! Sorry!
:-[
-
This is just what I was given. O_O Is there a wrong way of downloading the API.
-
Sorry! I meant to open the build.gradle but instead I opened the gradle file!
. Here:
buildscript { repositories { mavenCentral() maven { name = "forge" url = "http://files.minecraftforge.net/maven" } maven { name = "sonatype" url = "https://oss.sonatype.org/content/repositories/snapshots/" } } dependencies { classpath 'net.minecraftforge.gradle:ForgeGradle:2.2-SNAPSHOT' } } repositories { maven { name = "local" url = "file:///home/cpw/projects/repo" } } apply plugin: "maven" apply plugin: "net.minecraftforge.gradle.patcher" apply plugin: "net.minecraftforge.gradle.launch4j" minecraft.version = "1.10.2" minecraft { mappings = 'snapshot_nodoc_20160518' workspaceDir = "projects" versionJson = "jsons/${minecraft.version}-dev.json" buildUserdev = true buildInstaller = true installerVersion = "1.4" def common = { patchPrefixOriginal "../src-base/minecraft" patchPrefixChanged "../src-work/minecraft" mainClassClient "net.minecraft.launchwrapper.Launch" tweakClassClient "net.minecraftforge.fml.common.launcher.FMLTweaker" mainClassServer "net.minecraft.launchwrapper.Launch" tweakClassServer "net.minecraftforge.fml.common.launcher.FMLServerTweaker" } projects { forge { rootDir "." patchDir "patches/minecraft" patchAfter "clean" genPatchesFrom "clean" genMcpPatches = false applyMcpPatches = false s2sKeepImports = true with common } } } group = 'net.minecraftforge' version = getVersionFromJava(file("src/main/java/net/minecraftforge/common/ForgeVersion.java")) extractForgeSources { exclude "**/SideOnly.java", "**/Side.java" } extractForgeResources { exclude "**/log4j2.xml" } genGradleProjects { addTestCompileDep "junit:junit:4.12" } processJson { releaseJson = "jsons/${minecraft.version}-rel.json" addReplacements([ "@minecraft_version@": project.minecraft.version, "@version@": project.version, "@project@": "forge", "@artifact@": "net.minecraftforge:forge:${project.version}", "@universal_jar@": { outputJar.archiveName }, "@timestamp@": new Date().format("yyyy-MM-dd'T'HH:mm:ssZ"), ]) } task changelog(type: JenkinsChangelog) { // skip if there is no forge jenkins pass onlyIf { project.hasProperty('forgeJenkinsPass') // Not sure what abrar was trying to do here... //project.file("build/distributions/${project.name}-${project.version}-changelog.txt").text = "" } outputs.upToDateWhen { false } // never up to date serverRoot = "http://ci.jenkins.minecraftforge.net/" jobName = "minecraftforge" targetBuild = System.env['BUILD_NUMBER'] ?: project.ext.properties.buildNumber ?:0; authName = "console_script" authPassword = project.hasProperty('forgeJenkinsPass') ? project.getProperty('forgeJenkinsPass') : ""; output = "build/distributions/${project.name}-${project.version}-changelog.txt" } task crowdin(type: CrowdinDownload) { output = "build/crowdin.zip" projectId = 'minecraft-forge' extract = false // we wanna keep it as a zip. not extract it to a folder named "crowdin.zip" // task auomatically skips if this is null if (project.hasProperty('crowdinKey')) apiKey = project.crowdinKey } def extraTxts = [ changelog, // yeah we can do thi, because gradle and groovy are awesome "CREDITS-fml.txt", "LICENSE-new.txt", "MinecraftForge-Credits.txt", "Paulscode SoundSystem CodecIBXM License.txt", "Paulscode IBXM Library License.txt" ] outputJar { classifier = 'universal' from extraTxts // add crowdin locales from { crowdin.getDidWork() ? zipTree(crowdin.output) : null} dependsOn 'crowdin' manifest.attributes([ "Main-Class": "net.minecraftforge.fml.relauncher.ServerLaunchWrapper", "TweakClass": "net.minecraftforge.fml.common.launcher.FMLTweaker", "Class-Path": getServerClasspath(file("jsons/${minecraft.version}-rel.json")) ]) } installer { classifier = 'installer' from extraTxts from "src/main/resources/forge_logo.png" rename "forge_logo\\.png", "big_logo.png" } task signUniversal(type: SignJar, dependsOn: 'outputJar') { onlyIf { project.hasProperty('jarsigner') } def jarsigner = [:]; if (project.hasProperty('jarsigner')) jarsigner = project.jarsigner; alias = 'forge' exclude "paulscode/**" storePass = jarsigner.storepass keyPass = jarsigner.keypass keyStore = jarsigner.keystore inputFile = outputJar.archivePath outputFile = outputJar.archivePath } uploadArchives.dependsOn signUniversal build.dependsOn signUniversal installer.dependsOn signUniversal // MDK package import org.apache.tools.ant.filters.ReplaceTokens task makeMdk(type: Zip) { baseName = project.name classifier = "mdk" version = project.version destinationDir = file('build/distributions') from 'gradlew' from 'gradlew.bat' into ('gradle') { from 'gradle' } into ('eclipse') { from 'mdk/eclipse' } from changelog.output from ('mdk') { filter(ReplaceTokens, tokens: [ VERSION: project.version, MAPPINGS: minecraft.mappings.replace('nodoc_', '') ]) exclude 'eclipse' rename 'gitignore\\.txt', '.gitignore' from 'MinecraftForge-Credits.txt' from 'LICENSE-new.txt' from 'Paulscode IBXM Library License.txt' from 'Paulscode SoundSystem CodecIBXM License.txt' from 'CREDITS-fml.txt' } } tasks.build.dependsOn makeMdk // launch4j launch4j { jar = installer.archivePath.canonicalPath outfile = file("build/distributions/${project.name}-${project.version}-installer-win.exe").canonicalPath icon = file('icon.ico').canonicalPath manifest = file('l4jManifest.xml').canonicalPath jreMinVersion = '1.6.0' initialHeapPercent = 5; maxHeapPercent = 100; } tasks.generateXmlConfig.dependsOn installer tasks.build.dependsOn 'launch4j' // MAVEN artifacts { archives changelog.output archives file("build/distributions/${project.name}-${project.version}-installer-win.exe") archives makeMdk } task ciWriteBuildNumber << { def file = file("src/main/java/net/minecraftforge/common/ForgeVersion.java"); def bn = System.getenv("BUILD_NUMBER")?:project.ext.properties.buildNumber?:0; def outfile = ""; def ln = "\n"; //Linux line endings because we're on git! file.eachLine{ String s -> if (s.matches("^ public static final int buildVersion = [\\d]+;\$")) s = " public static final int buildVersion = ${bn};"; if (s.matches('^ public static final String mcVersion = "[^\\"]+";')) s = " public static final String mcVersion = \"${minecraft.version}\";"; outfile += (s+ln); } file.write(outfile); } uploadArchives { repositories.mavenDeployer { dependsOn 'build' if (project.hasProperty('forgeMavenPass')) { repository(url: "http://files.minecraftforge.net/maven/manage/upload") { authentication(userName: "forge", password: project.getProperty('forgeMavenPass')) // the elvis operator. look it up. } } else { // local repo folder. Might wanna juset use gradle install if you wanans end it to maven-local repository(url: 'file://localhost/' + project.file('repo').getAbsolutePath()) } pom { groupId = project.group version = project.version artifactId = project.archivesBaseName project { name project.archivesBaseName packaging 'jar' description 'Minecraft Forge API' url 'https://github.com/MinecraftForge/MinecraftForge' scm { url 'https://github.com/MinecraftForge/MinecraftForge' connection 'scm:git:git://github.com/MinecraftForge/MinecraftForge.git' developerConnection 'scm:git:[email protected]:MinecraftForge/MinecraftForge.git' } issueManagement { system 'github' url 'https://github.com/MinecraftForge/MinecraftForge/issues' } licenses { license { name 'Forge Public License' url 'https://raw.github.com/MinecraftForge/MinecraftForge/master/MinecraftForge-License.txt' distribution 'repo' } } developers { developer { id 'cpw' name 'cpw' roles { role 'developer' } } developer { id 'LexManos' name 'Lex Manos' roles { role 'developer' } } developer { id 'AbrarSyed' name 'Abrar Syed' roles { role 'contributor' } } } } } } } // HELPER METHODS import groovy.json.JsonSlurper; String getServerClasspath(File file) { def node = new JsonSlurper().parse(file); def out = new StringBuilder() node.versionInfo.libraries.each { lib -> if (lib.serverreq) { // group : artifact : version def split = lib.name.split(':') def group = split[0].replace('.', '/') def artifact = split[1] def version = split[2] out += "libraries/$group/$artifact/$version/$artifact-${version}.jar " } } out += "minecraft_server.${minecraft.version}.jar" return out.toString(); } String getVersionFromJava(File file) { String major = "0"; String minor = "0"; String revision = "0"; String build = "0"; String prefix = "public static final int"; file.eachLine{ String s -> s = s.trim(); if (s.startsWith(prefix)) { s = s.substring(prefix.length(), s.length() - 1); s = s.replace('=', ' ').replace("Version", "").replaceAll(" +", " ").trim(); String[] pts = s.split(" "); if (pts[0].equals("major")) major = pts[pts.length - 1]; else if (pts[0] == "minor") minor = pts[pts.length - 1]; else if (pts[0] == "revision") revision = pts[pts.length - 1]; } } build = System.getenv("BUILD_NUMBER") ?: project.ext.properties.buildNumber ?: 0 String branch = null; if (!System.getenv().containsKey("GIT_BRANCH")) { // TODO: use grgit - Tried to switch 07/07/16 - jgit broken on windows? branch = "git rev-parse --abbrev-ref HEAD".execute().text.trim() } else { branch = System.getenv("GIT_BRANCH"); branch = branch.substring(branch.lastIndexOf('/') + 1); } def out = "${minecraft.version.replace('-', '_')}-$major.$minor.$revision.$build" if (branch && branch != 'master' && branch != 'HEAD' && branch != minecraft.version && branch != minecraft.version + '.0') { if (!(branch.endsWith('.x') && minecraft.version.startsWith(branch.substring(0, branch.length() -1)))) out += "-$branch" } return out; } task resetBuildNumber << { project.ext.properties.buildNumber = 0; ciWriteBuildNumber.execute() } // re-add old tasks for jenkins compat // should be remvoed, and the jenkisn fixed when no longer building with FG 1.2 task setupForge { dependsOn 'setup', 'ciWriteBuildNumber' } task buildPackages { dependsOn 'build' } //Temporary hack to fix compile errors caused by mappings shading in Bootstrap /* task fixParams << { logger.lifecycle('Fixing param names!') def params = new File(extractMcpMappings.destinationDir, 'params.csv') def text = params.text text = text.replaceAll('p_180276_1_,biome,', 'p_180276_1_,biomeIn,') params.write(text) } fixParams.dependsOn('extractMcpMappings') extractMcpMappings.finalizedBy('fixParams') */
Sorry!
-
Here it is:
#!/usr/bin/env bash ############################################################################## ## ## Gradle start up script for UN*X ## ############################################################################## # Add default JVM options here. You can also use JAVA_OPTS and GRADLE_OPTS to pass JVM options to this script. DEFAULT_JVM_OPTS="" APP_NAME="Gradle" APP_BASE_NAME=`basename "$0"` # Use the maximum available, or set MAX_FD != -1 to use that value. MAX_FD="maximum" warn ( ) { echo "$*" } die ( ) { echo echo "$*" echo exit 1 } # OS specific support (must be 'true' or 'false'). cygwin=false msys=false darwin=false case "`uname`" in CYGWIN* ) cygwin=true ;; Darwin* ) darwin=true ;; MINGW* ) msys=true ;; esac # For Cygwin, ensure paths are in UNIX format before anything is touched. if $cygwin ; then [ -n "$JAVA_HOME" ] && JAVA_HOME=`cygpath --unix "$JAVA_HOME"` fi # Attempt to set APP_HOME # Resolve links: $0 may be a link PRG="$0" # Need this for relative symlinks. while [ -h "$PRG" ] ; do ls=`ls -ld "$PRG"` link=`expr "$ls" : '.*-> \(.*\)$'` if expr "$link" : '/.*' > /dev/null; then PRG="$link" else PRG=`dirname "$PRG"`"/$link" fi done SAVED="`pwd`" cd "`dirname \"$PRG\"`/" >&- APP_HOME="`pwd -P`" cd "$SAVED" >&- CLASSPATH=$APP_HOME/gradle/wrapper/gradle-wrapper.jar # Determine the Java command to use to start the JVM. if [ -n "$JAVA_HOME" ] ; then if [ -x "$JAVA_HOME/jre/sh/java" ] ; then # IBM's JDK on AIX uses strange locations for the executables JAVACMD="$JAVA_HOME/jre/sh/java" else JAVACMD="$JAVA_HOME/bin/java" fi if [ ! -x "$JAVACMD" ] ; then die "ERROR: JAVA_HOME is set to an invalid directory: $JAVA_HOME Please set the JAVA_HOME variable in your environment to match the location of your Java installation." fi else JAVACMD="java" which java >/dev/null 2>&1 || die "ERROR: JAVA_HOME is not set and no 'java' command could be found in your PATH. Please set the JAVA_HOME variable in your environment to match the location of your Java installation." fi # Increase the maximum file descriptors if we can. if [ "$cygwin" = "false" -a "$darwin" = "false" ] ; then MAX_FD_LIMIT=`ulimit -H -n` if [ $? -eq 0 ] ; then if [ "$MAX_FD" = "maximum" -o "$MAX_FD" = "max" ] ; then MAX_FD="$MAX_FD_LIMIT" fi ulimit -n $MAX_FD if [ $? -ne 0 ] ; then warn "Could not set maximum file descriptor limit: $MAX_FD" fi else warn "Could not query maximum file descriptor limit: $MAX_FD_LIMIT" fi fi # For Darwin, add options to specify how the application appears in the dock if $darwin; then GRADLE_OPTS="$GRADLE_OPTS \"-Xdock:name=$APP_NAME\" \"-Xdock:icon=$APP_HOME/media/gradle.icns\"" fi # For Cygwin, switch paths to Windows format before running java if $cygwin ; then APP_HOME=`cygpath --path --mixed "$APP_HOME"` CLASSPATH=`cygpath --path --mixed "$CLASSPATH"` # We build the pattern for arguments to be converted via cygpath ROOTDIRSRAW=`find -L / -maxdepth 1 -mindepth 1 -type d 2>/dev/null` SEP="" for dir in $ROOTDIRSRAW ; do ROOTDIRS="$ROOTDIRS$SEP$dir" SEP="|" done OURCYGPATTERN="(^($ROOTDIRS))" # Add a user-defined pattern to the cygpath arguments if [ "$GRADLE_CYGPATTERN" != "" ] ; then OURCYGPATTERN="$OURCYGPATTERN|($GRADLE_CYGPATTERN)" fi # Now convert the arguments - kludge to limit ourselves to /bin/sh i=0 for arg in "$@" ; do CHECK=`echo "$arg"|egrep -c "$OURCYGPATTERN" -` CHECK2=`echo "$arg"|egrep -c "^-"` ### Determine if an option if [ $CHECK -ne 0 ] && [ $CHECK2 -eq 0 ] ; then ### Added a condition eval `echo args$i`=`cygpath --path --ignore --mixed "$arg"` else eval `echo args$i`="\"$arg\"" fi i=$((i+1)) done case $i in (0) set -- ;; (1) set -- "$args0" ;; (2) set -- "$args0" "$args1" ;; (3) set -- "$args0" "$args1" "$args2" ;; (4) set -- "$args0" "$args1" "$args2" "$args3" ;; (5) set -- "$args0" "$args1" "$args2" "$args3" "$args4" ;; (6) set -- "$args0" "$args1" "$args2" "$args3" "$args4" "$args5" ;; (7) set -- "$args0" "$args1" "$args2" "$args3" "$args4" "$args5" "$args6" ;; ( set -- "$args0" "$args1" "$args2" "$args3" "$args4" "$args5" "$args6" "$args7" ;; (9) set -- "$args0" "$args1" "$args2" "$args3" "$args4" "$args5" "$args6" "$args7" "$args8" ;; esac fi # Split up the JVM_OPTS And GRADLE_OPTS values into an array, following the shell quoting and substitution rules function splitJvmOpts() { JVM_OPTS=("$@") } eval splitJvmOpts $DEFAULT_JVM_OPTS $JAVA_OPTS $GRADLE_OPTS JVM_OPTS[${#JVM_OPTS[*]}]="-Dorg.gradle.appname=$APP_BASE_NAME" exec "$JAVACMD" "${JVM_OPTS[@]}" -classpath "$CLASSPATH" org.gradle.wrapper.GradleWrapperMain "$@"
-
So here's my problem.
I try to build my workspace for eclipse using the gradlew setupDecompWorkspace command you have to run.
I do this and I've done this multiple times, except the first time where it was downloading everything (still same error though) the same thing happens. Here:
C:\Users\[user Name]\Desktop\MinecraftForge-1.10.x\MinecraftForge-1.10.x>gradle w setupDecompWorkspace eclipse --info Starting Build Settings evaluated using settings file 'C:\Users\[user Name]\Desktop\MinecraftF orge-1.10.x\MinecraftForge-1.10.x\settings.gradle'. Projects loaded. Root project using build file 'C:\Users\[user Name]\Desktop\Mi necraftForge-1.10.x\MinecraftForge-1.10.x\build.gradle'. Included projects: [root project 'forge'] Evaluating root project 'forge' using build file 'C:\Users\[user Name]\Desktop\ MinecraftForge-1.10.x\MinecraftForge-1.10.x\build.gradle'. This mapping 'snapshot_nodoc_20160518' was designed for MC 1.9.4! Use at your ow n peril. ################################################# ForgeGradle 2.2-SNAPSHOT-0447b4e https://github.com/MinecraftForge/ForgeGradle ################################################# Powered by MCP unknown http://modcoderpack.com by: Searge, ProfMobius, Fesh0r, R4wk, ZeuX, IngisKahn, bspkrs ################################################# All projects evaluated. FAILURE: Build failed with an exception. * What went wrong: Task 'setupDecompWorkspace' not found in root project 'forge'. * Try: Run gradlew tasks to get a list of available tasks. Run with --stacktrace option to get the stack trace. Run with - -debug option to get more log output. BUILD FAILED Total time: 5.24 secs
[1.10.2] How to get World and make it compatible both server and client side?
in Modder Support
Posted
I need it in the ModWorld class