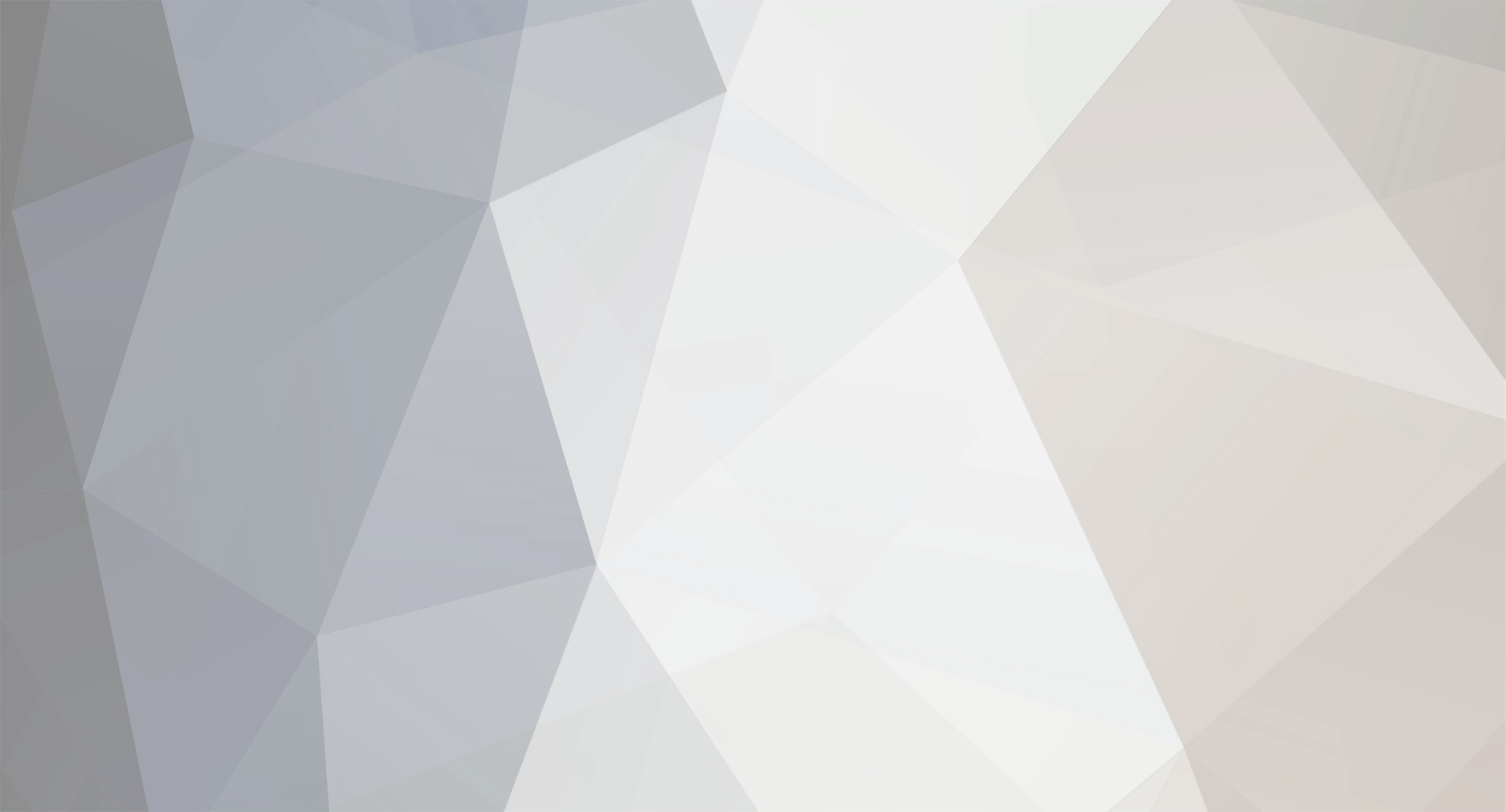
Kriptikz
-
Posts
81 -
Joined
-
Last visited
Posts posted by Kriptikz
-
-
1 hour ago, Leomelonseeds said:
This is actually a good way to register blocks and items! I will probably switch to this in the future.
Also, is this 1.11 exclusive?
I do believe this is in 1.10.2 as well.
-
21 minutes ago, diesieben07 said:
Not sure if you have a question or anything. All I can say is: kudos to you for actually doing research and not blindly copying outdated terrible tutorials.
Thanks. I went through multiple iterations of this setup before I landed on this one. I edited my post to actually have a solid question. Basically I want a solid foundation before I start building and want to know if anything at all can be done better.
-
I'm brand new to modding and a noob programmer. I learned the old way to register blocks and items using GameRegistry.register() then I found out there is actually a new, better way to register blocks, items, and even models. The new way is to use RegistryEvents and I'm a sucker for new and better things so I tried to figure out how it works. My goal with this post is to see if anything I did can be done better and whether or not this is correct. Just because it works doesn't mean it's correct. I want to have a solid base before I build on it too much. Here is what I came up with:
RegistryEventHandler.java
@Mod.EventBusSubscriber public class RegistryEventHandler { @SubscribeEvent public static void registerBlocks(RegistryEvent.Register<Block> event) { event.getRegistry().registerAll(ModBlocks.BLOCKS); Utils.getLogger().info("Registered blocks"); } @SubscribeEvent public static void registerItems(RegistryEvent.Register<Item> event) { event.getRegistry().registerAll(ModItems.ITEMS); for (Block block : ModBlocks.BLOCKS) { event.getRegistry().register(new ItemBlock(block).setRegistryName(block.getRegistryName())); } Utils.getLogger().info("Registered items"); } @SubscribeEvent public static void registerModels(ModelRegistryEvent event) { for (Block block: ModBlocks.BLOCKS) { ModelLoader.setCustomModelResourceLocation(Item.getItemFromBlock(block), 0, new ModelResourceLocation(block.getRegistryName(), "inventory")); } for (Item item: ModItems.ITEMS) { ModelLoader.setCustomModelResourceLocation(item, 0, new ModelResourceLocation(item.getRegistryName(), "inventory")); } Utils.getLogger().info("Registered models"); } }
ModBlocks.java
public class ModBlocks { public static final Block[] BLOCKS = { new BlockTinOre("tin_ore", Material.ROCK), new BlockTinBlock("tin_block", Material.ROCK) }; }
ModItems.java
public class ModItems { public static final Item[] ITEMS = { new ItemTinIngot("tin_ingot") }; }
BlockTinOre.java
public class BlockTinOre extends BlockBase { public BlockTinOre(String name, Material material) { super(name, material); } }
BlockTinBlock.java
public class BlockTinBlock extends BlockBase { public BlockTinBlock(String name, Material material) { super(name, material); } }
BlockBase.java
public class BlockBase extends Block { BlockBase(String name, Material material) { super(material); this.setRegistryName(Reference.MODID, name); this.setUnlocalizedName(this.getRegistryName().toString()); } }
ItemTinIngot.java
public class ItemTinIngot extends ItemBase { public ItemTinIngot(String name) { super(name); } }
ItemBase.java
public class ItemBase extends Item { ItemBase(String name) { this.setRegistryName(new ResourceLocation(Reference.MODID, name)); this.setUnlocalizedName(this.getRegistryName().toString()); } }
-
4
-
(SOLVED) How to make custom Mob targetable by other hostile mobs?
in Modder Support
Posted · Edited by Kriptikz
I'm working on creating an item that spawns an entity that looks like the player that used the item. This entity just runs forward for about 5-10 seconds then disappears. I want this entity to be targetable by hostile mobs.
I'm thinking of creating a custom entity for this, how would I go about giving it the player model and texture? If this is too complex for me atm then I will just give it a default model and texture for now.
My main concern is how I get hostile mobs to target this entity. It seems like hostile mobs AI have an attack nearest attackable target that selects an EntityPlayer. Extending from EntityPlayer requires a gameprofile, so I don't think I can make that work.
I don't really want to replace all hostile mob AI just to add a single line of code to them all.
It seems like I can add the task on LivingUpdateEvent. Will this mess up any of the hostile mobs AI?
UPDATE: So I tested this with EntityAnimal instead of custom mob, which I haven't made yet, and it was pretty hilarious, so it seems the AI does work fine like this. Now to figure out how to set the entities texture to the players texture on spawn, if this is even possible.
To give the custom mob the players texture I had my mob extend EntityTameable and using preRenderCallback I get the owner from the entity, and set the texture using AbstractClientPlayer#getLocationSkin()
This works in single player for sure, for servers further testing is required.