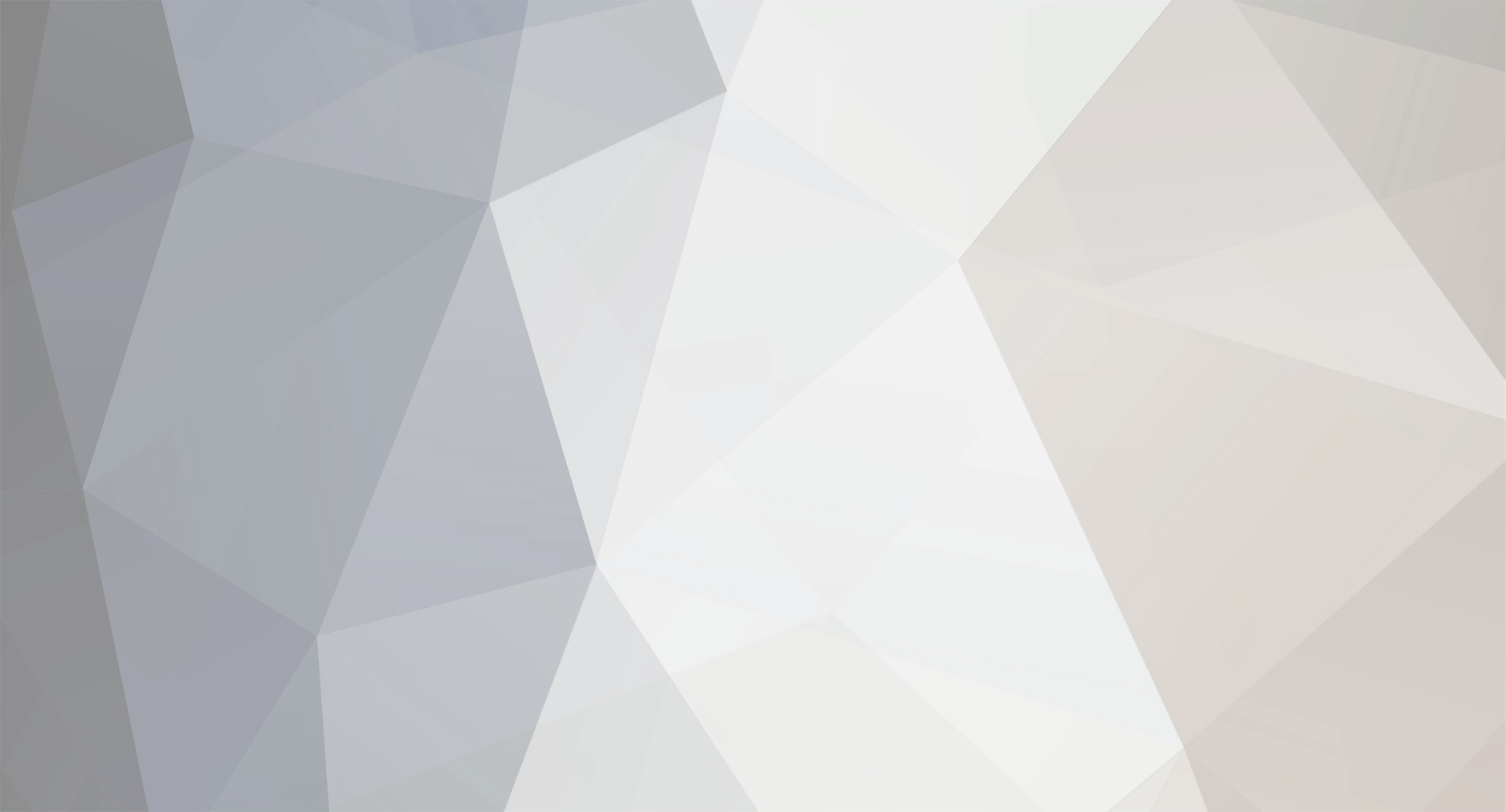
clowcadia
-
Posts
458 -
Joined
-
Last visited
Posts posted by clowcadia
-
-
Problem is my texture is meant to be match alex(colors tweeked to be on a red scale) but i get a semi transparent rendered mob.
I made a model through techne, just plain biped model nothing changed
package com.clowcadia.test.model; import net.minecraft.client.model.ModelBase; import net.minecraft.client.model.ModelRenderer; import net.minecraft.entity.Entity; public class ModelTest extends ModelBase { //fields ModelRenderer head; ModelRenderer body; ModelRenderer rightarm; ModelRenderer leftarm; ModelRenderer rightleg; ModelRenderer leftleg; public ModelTest() { textureWidth = 64; textureHeight = 32; head = new ModelRenderer(this, 0, 0); head.addBox(-4F, -8F, -4F, 8, 8, 8); head.setRotationPoint(0F, 0F, 0F); head.setTextureSize(64, 32); head.mirror = true; setRotation(head, 0F, 0F, 0F); body = new ModelRenderer(this, 16, 16); body.addBox(-4F, 0F, -2F, 8, 12, 4); body.setRotationPoint(0F, 0F, 0F); body.setTextureSize(64, 32); body.mirror = true; setRotation(body, 0F, 0F, 0F); rightarm = new ModelRenderer(this, 40, 16); rightarm.addBox(-3F, -2F, -2F, 4, 12, 4); rightarm.setRotationPoint(-5F, 2F, 0F); rightarm.setTextureSize(64, 32); rightarm.mirror = true; setRotation(rightarm, 0F, 0F, 0F); leftarm = new ModelRenderer(this, 40, 16); leftarm.addBox(-1F, -2F, -2F, 4, 12, 4); leftarm.setRotationPoint(5F, 2F, 0F); leftarm.setTextureSize(64, 32); leftarm.mirror = true; setRotation(leftarm, 0F, 0F, 0F); rightleg = new ModelRenderer(this, 0, 16); rightleg.addBox(-2F, 0F, -2F, 4, 12, 4); rightleg.setRotationPoint(-2F, 12F, 0F); rightleg.setTextureSize(64, 32); rightleg.mirror = true; setRotation(rightleg, 0F, 0F, 0F); leftleg = new ModelRenderer(this, 0, 16); leftleg.addBox(-2F, 0F, -2F, 4, 12, 4); leftleg.setRotationPoint(2F, 12F, 0F); leftleg.setTextureSize(64, 32); leftleg.mirror = true; setRotation(leftleg, 0F, 0F, 0F); } public void render(Entity entity, float f, float f1, float f2, float f3, float f4, float f5) { super.render(entity, f, f1, f2, f3, f4, f5); setRotationAngles(f, f1, f2, f3, f4, f5, entity); head.render(f5); body.render(f5); rightarm.render(f5); leftarm.render(f5); rightleg.render(f5); leftleg.render(f5); } private void setRotation(ModelRenderer model, float x, float y, float z) { model.rotateAngleX = x; model.rotateAngleY = y; model.rotateAngleZ = z; } public void setRotationAngles(float f, float f1, float f2, float f3, float f4, float f5, Entity entity) { super.setRotationAngles(f, f1, f2, f3, f4, f5, entity); } }
I am assuming this is all the code that needs to be posted
-
-
-
I keep running into this syntax error, can anyone help me sort this out
(registerMobModel is where error is)
package com.clowcadia.test; import com.clowcadia.test.entities.Test; import com.clowcadia.test.render.RenderEntityTest; import net.minecraft.entity.Entity; import net.minecraftforge.fml.client.registry.IRenderFactory; import net.minecraftforge.fml.client.registry.RenderingRegistry; public class TestModelManager { public static void registerAllModels(){ registerMobModels(); } private static void registerMobModels(){ registerMobModel(Test.class, RenderEntityTest::new); } private static <T extends Entity> void registerMobModel(Class<T> entity, IRenderFactory<? super T> renderFactory) { RenderingRegistry.registerEntityRenderingHandler(entity, renderFactory); } }
-
There is no white box, just a flat thing that is shaded almost trasparent
-
Any chance you could give me an example? goign through forums searching for updated help
-
Yes I am following a outdated 1.7 tutorial on basic entity rendering.
If anyone got any better tutorials to follow would be nice to see
-
34 minutes ago, clowcadia said:
i dont have any renderers , i just want a simple white box i want to model once the entity is functional
my livingEntity before did not require any renderers does the entityTamabel require something else?
-
i dont have any renderers , i just want a simple white box i want to model once the entity is functional
-
package com.clowcadia.test; import com.clowcadia.test.npc.Basic; import net.minecraft.util.ResourceLocation; import net.minecraftforge.fml.common.registry.EntityRegistry; public class NPCHandler { public static void registerNPCs(){ EntityRegistry.registerModEntity(new ResourceLocation(TestModHandler.modId,"basicNPC"), Basic.class, "BasicNPC", 0, TestModHandler.instance, 64, 1, true, 0x4286f4, 0x606e84); } }
-
i cant seem to summon, and the egg spawns an entity as like flat white /transparent thing
-
Ok i realized egg summons something like a flat pannel that semi invinceble and it dies after a little bit
-
i forgot the how summon command works any chance anyone can refresh my memory
-
nothing happens at all, no error
there is apperantly something that returns the stomach value to the console 0 concurrently and 800 on trying to spawn through egg, have nto tried the summon yet
-
package com.clowcadia.test; import com.clowcadia.test.npc.Basic; import net.minecraft.util.ResourceLocation; import net.minecraftforge.fml.common.registry.EntityRegistry; public class NPCHandler { public static void registerNPCs(){ EntityRegistry.registerModEntity(new ResourceLocation(TestModHandler.modId,"basicNPC"), Basic.class, "BasicNPC", 0, TestModHandler.instance, 64, 1, true, 0x4286f4, 0x606e84); } }
package com.clowcadia.test; import net.minecraftforge.fml.common.Mod; import net.minecraftforge.fml.common.SidedProxy; import net.minecraftforge.fml.common.Mod.EventHandler; import net.minecraftforge.fml.common.event.FMLInitializationEvent; import net.minecraftforge.fml.common.event.FMLPostInitializationEvent; import net.minecraftforge.fml.common.event.FMLPreInitializationEvent; @Mod(modid = TestModHandler.modId, name = TestModHandler.name, version = TestModHandler.version) public class TestModHandler { public static final String modId = "testmod"; public static final String name = "Test Mod"; public static final String version = "1.0.0"; @Mod.Instance(modId) public static TestModHandler instance; @SidedProxy(serverSide = "com.clowcadia.test.CommonProxy", clientSide = "com.clowcadia.test.ClientProxy") public static CommonProxy proxy; @EventHandler public void preInit(FMLPreInitializationEvent event){ NPCHandler.registerNPCs(); } @EventHandler public void init(FMLInitializationEvent event){ proxy.init(); } @EventHandler public void postInit(FMLPostInitializationEvent event){ } }
-
As the title explains i cant use an egg to summon my entity, what is wrong
package com.clowcadia.test.npc; import com.clowcadia.test.GuiHandler; import com.clowcadia.test.TestModHandler; import com.clowcadia.test.utils.Utils; import net.minecraft.entity.EntityAgeable; import net.minecraft.entity.EntityLiving; import net.minecraft.entity.passive.EntityTameable; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.init.Items; import net.minecraft.item.ItemStack; import net.minecraft.nbt.NBTTagCompound; import net.minecraft.network.NetworkManager; import net.minecraft.util.EnumFacing; import net.minecraft.util.EnumHand; import net.minecraft.world.World; import net.minecraftforge.common.capabilities.Capability; import net.minecraftforge.common.capabilities.ICapabilityProvider; import net.minecraftforge.items.CapabilityItemHandler; import net.minecraftforge.items.ItemStackHandler; public class Basic extends EntityTameable implements ICapabilityProvider{ private final ItemStackHandler handler; private String tagStomach = "Stomach"; private int stomach; private int stomachCap = 800; public Basic(World world) { super(world); this.handler = new ItemStackHandler(9); this.stomach = 0; } public int getStomach(){ return this.stomach; } public void setStomach(int value){ this.stomach = value; } @Override public boolean processInteract(EntityPlayer player, EnumHand hand) { if (!this.world.isRemote) { int basicID = this.getEntityId(); //for inputing into x/y/z in the opne gui to pass the entety id System.out.println("Player has interacted with the mob"); player.openGui(TestModHandler.instance, GuiHandler.BASIC, this.world, basicID,0, 0); } return true; } @Override public void onEntityUpdate() { ItemStack foodStack = handler.getStackInSlot(0); if (this.world != null){ if(!this.world.isRemote){ Utils.getLogger().info("Stomach: "+stomach); if(foodStack.getUnlocalizedName().equals(Items.APPLE.getUnlocalizedName()) && stomach != stomachCap){ foodStack.splitStack(1); stomach += 40; } } } super.onEntityUpdate(); } @Override public EntityAgeable createChild(EntityAgeable ageable) { // TODO Auto-generated method stub return null; } @Override public <T> T getCapability(Capability<T> capability, EnumFacing facing) { if(capability == CapabilityItemHandler.ITEM_HANDLER_CAPABILITY) return (T) this.handler; return super.getCapability(capability, facing); } @Override public boolean hasCapability(Capability<?> capability, EnumFacing facing) { if(capability == CapabilityItemHandler.ITEM_HANDLER_CAPABILITY) return true; return super.hasCapability(capability, facing); } @Override public void readEntityFromNBT(NBTTagCompound compound) { this.handler.deserializeNBT(compound.getCompoundTag("ItemStackHandler")); this.stomach = compound.getInteger("Stomach"); if(compound.hasKey(tagStomach)) Utils.getLogger().info("Reading Stomach"); super.readEntityFromNBT(compound); } @Override public void writeEntityToNBT(NBTTagCompound compound) { super.writeEntityToNBT(compound); compound.setTag("ItemStackHandler", this.handler.serializeNBT()); compound.setInteger("Stomach", this.stomach); if(compound.hasKey(tagStomach)) Utils.getLogger().info("Writing Stomach"); } }
-
Second thought i think i should make it ownable, just case if some how one day my mod can be server used....
Still i want to see if anyone thinks or knows a work around
-
What i have is a entityLiving like a villager, but hopefully smarter or more useful. What I am trying to do is set it a task where after a certain condition it would follow me. Condition is there, response to condition is understood. But making a costume AI Task that extends follow owner, only allows tameable entity, where is i am trying to keep my entity livingEntity, or should i change my entity to entityTamable. Prefer to make it more like a general living entity maybe because personal reasons like my workers are not my pets, maybe for minecraft i have to realize it aint real lol. Is there a way to have a livingEntity to follow?
-
-
I have a living entity, i understand how the ai task work they pre-set with their own events and handling. What i am trying to do is have my entity wonder until a certai condition is met in its class. more specificly my antity eats, and the player feeeds it, once the entity had enough food it stops eating, what i want it to do once it is full i want it to follow the person who fed it the owner but only once it is full
so the condition its stomach value to be at its max before following owner, how could i call the task from onupdate method or is there a different way like, in the add task zone set it to if(stomach==full)add task follow owner?
-
woohooo it works
-
How exactly do I access. When I was accessing with (basic) entity.get stomach() it would not react when I had it used as an integer added to a string
-
On 2017-03-08 at 2:31 PM, loordgek said:
in the container override detectAndSendChanges check if the value is not the same on the server and the client is not send a packet
look in ContainerFurnace how mojang does it
ok i tried this but nothign
package com.clowcadia.test.containers; import com.clowcadia.test.npc.Basic; import com.clowcadia.test.utils.Utils; import net.minecraft.entity.Entity; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.inventory.Container; import net.minecraft.inventory.IContainerListener; import net.minecraft.inventory.IInventory; import net.minecraft.inventory.Slot; import net.minecraft.world.World; import net.minecraftforge.common.capabilities.ICapabilityProvider; import net.minecraftforge.items.CapabilityItemHandler; import net.minecraftforge.items.IItemHandler; import net.minecraftforge.items.SlotItemHandler; public class ContainerBasic extends Container{ public Entity entity; private int stomach; public ContainerBasic(IInventory playerInv, Entity entity) { this.entity = entity; IItemHandler handler = entity.getCapability(CapabilityItemHandler.ITEM_HANDLER_CAPABILITY, null); //Gets the inventory from our tile entity //Our tile entity slots this.addSlotToContainer(new SlotItemHandler(handler, 0, 8,8)); //The player's inventory slots int xPos = 8; //The x position of the top left player inventory slot on our texture int yPos = 84; //The y position of the top left player inventory slot on our texture //Player slots for (int y = 0; y < 3; ++y) { for (int x = 0; x < 9; ++x) { this.addSlotToContainer(new Slot(playerInv, x + y * 9 + 9, xPos + x * 18, yPos + y * 18)); } } for (int x = 0; x < 9; ++x) { this.addSlotToContainer(new Slot(playerInv, x, xPos + x * 18, yPos + 58)); } Utils.getLogger().info("Finish ContainerBasic"); } @Override public boolean canInteractWith(EntityPlayer player) { return !player.isSpectator(); } @Override public void detectAndSendChanges() { IContainerListener icontainerlistener = this.listeners.get(0); if(this.stomach!=((Basic) this.entity).getStomach()){ icontainerlistener.sendProgressBarUpdate(this, 0, ((Basic) this.entity).getStomach()); } this.stomach = ((Basic) this.entity).getStomach(); super.detectAndSendChanges(); } }
package com.clowcadia.test; import java.security.PublicKey; import com.clowcadia.test.containers.ContainerBasic; import com.clowcadia.test.gui.GuiBasic; import com.clowcadia.test.npc.Basic; import net.minecraft.entity.Entity; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.util.datafix.fixes.EntityId; import net.minecraft.util.math.BlockPos; import net.minecraft.util.math.RayTraceResult; import net.minecraft.world.World; import net.minecraftforge.fml.common.network.IGuiHandler; public class GuiHandler implements IGuiHandler{ public static final int BASIC = 0; @Override public Object getServerGuiElement(int ID, EntityPlayer player, World world, int x, int y, int z) { if(ID==BASIC){ Entity basic = world.getEntityByID(x); return new ContainerBasic(player.inventory,basic); } return null; } @Override public Object getClientGuiElement(int ID, EntityPlayer player, World world, int x, int y, int z) { if(ID==BASIC){ Entity basic = world.getEntityByID(x); return new GuiBasic(player.inventory, basic,((Basic) basic).getStomach()); } return null; } }
package com.clowcadia.test.gui; import java.util.ArrayList; import java.util.List; import com.clowcadia.test.TestModHandler; import com.clowcadia.test.containers.ContainerBasic; import com.clowcadia.test.npc.Basic; import com.clowcadia.tutorial3.Reference; import net.minecraft.client.gui.inventory.GuiContainer; import net.minecraft.client.renderer.GlStateManager; import net.minecraft.client.resources.I18n; import net.minecraft.entity.Entity; import net.minecraft.inventory.Container; import net.minecraft.inventory.IInventory; import net.minecraft.item.ItemStack; import net.minecraft.util.ResourceLocation; import net.minecraft.util.math.RayTraceResult; import net.minecraft.util.text.TextFormatting; import net.minecraft.world.World; import net.minecraftforge.common.capabilities.ICapabilityProvider; import net.minecraftforge.items.CapabilityItemHandler; import scala.reflect.internal.Trees.This; public class GuiBasic extends GuiContainer{ private IInventory playerInv; private Entity entity; private int stomach; public GuiBasic(IInventory playerInv, Entity entity, int stomach) { super(new ContainerBasic(playerInv, entity)); this.stomach = stomach; this.xSize=176; this.ySize=166; this.playerInv = playerInv; this.entity = entity; } @Override protected void drawGuiContainerBackgroundLayer(float partialTicks, int mouseX, int mouseY) { GlStateManager.color(1.0F, 1.0F, 1.0F,1.0F); this.mc.getTextureManager().bindTexture(new ResourceLocation(TestModHandler.modId, "textures/gui/container/basic.png")); this.drawTexturedModalRect(this.guiLeft, this.guiTop, 0, 0, this.xSize,this.ySize); } /** * Draws the text that is an overlay, i.e where it says Block Breaker in the gui on the top */ @Override protected void drawGuiContainerForegroundLayer(int mouseX, int mouseY) { String s = I18n.format("container.basic"); //Gets the formatted name for the block breaker from the language file this.mc.fontRendererObj.drawString(s, this.xSize / 2 - this.mc.fontRendererObj.getStringWidth(s) / 2, 6, 4210752); //Draws the block breaker name in the center on the top of the gui this.mc.fontRendererObj.drawString(this.playerInv.getDisplayName().getFormattedText(), 8, 72, 4210752); //The player's inventory name this.mc.fontRendererObj.drawString(this.stomach+"", this.xSize / 2 - this.mc.fontRendererObj.getStringWidth("") / 2, 20, 4210752); int actualMouseX = mouseX - ((this.width - this.xSize) / 2); int actualMouseY = mouseY - ((this.height - this.ySize) / 2); } }
-
package com.clowcadia.test.gui; import java.util.ArrayList; import java.util.List; import com.clowcadia.test.TestModHandler; import com.clowcadia.test.containers.ContainerBasic; import com.clowcadia.test.npc.Basic; import com.clowcadia.tutorial3.Reference; import net.minecraft.client.gui.inventory.GuiContainer; import net.minecraft.client.renderer.GlStateManager; import net.minecraft.client.resources.I18n; import net.minecraft.entity.Entity; import net.minecraft.inventory.Container; import net.minecraft.inventory.IInventory; import net.minecraft.item.ItemStack; import net.minecraft.util.ResourceLocation; import net.minecraft.util.math.RayTraceResult; import net.minecraft.util.text.TextFormatting; import net.minecraft.world.World; import net.minecraftforge.common.capabilities.ICapabilityProvider; import net.minecraftforge.items.CapabilityItemHandler; import scala.reflect.internal.Trees.This; public class GuiBasic extends GuiContainer{ private IInventory playerInv; private Entity entity; private String stomachDisplay; public GuiBasic(IInventory playerInv, Entity entity) { super(new ContainerBasic(playerInv, entity)); this.xSize=176; this.ySize=166; this.playerInv = playerInv; this.entity = entity; } @Override protected void drawGuiContainerBackgroundLayer(float partialTicks, int mouseX, int mouseY) { GlStateManager.color(1.0F, 1.0F, 1.0F,1.0F); this.mc.getTextureManager().bindTexture(new ResourceLocation(TestModHandler.modId, "textures/gui/container/basic.png")); this.drawTexturedModalRect(this.guiLeft, this.guiTop, 0, 0, this.xSize,this.ySize); } /** * Draws the text that is an overlay, i.e where it says Block Breaker in the gui on the top */ @Override protected void drawGuiContainerForegroundLayer(int mouseX, int mouseY) { String s = I18n.format("container.basic"); //Gets the formatted name for the block breaker from the language file this.mc.fontRendererObj.drawString(s, this.xSize / 2 - this.mc.fontRendererObj.getStringWidth(s) / 2, 6, 4210752); //Draws the block breaker name in the center on the top of the gui this.mc.fontRendererObj.drawString(this.playerInv.getDisplayName().getFormattedText(), 8, 72, 4210752); //The player's inventory name this.mc.fontRendererObj.drawString(((Basic) entity).getStomach()+"", this.xSize / 2 - this.mc.fontRendererObj.getStringWidth(stomachDisplay) / 2, 20, 4210752); int actualMouseX = mouseX - ((this.width - this.xSize) / 2); int actualMouseY = mouseY - ((this.height - this.ySize) / 2); } }
package com.clowcadia.test.containers; import com.clowcadia.test.npc.Basic; import com.clowcadia.test.utils.Utils; import net.minecraft.entity.Entity; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.inventory.Container; import net.minecraft.inventory.IInventory; import net.minecraft.inventory.Slot; import net.minecraft.world.World; import net.minecraftforge.common.capabilities.ICapabilityProvider; import net.minecraftforge.items.CapabilityItemHandler; import net.minecraftforge.items.IItemHandler; import net.minecraftforge.items.SlotItemHandler; public class ContainerBasic extends Container{ public Entity entity; public ContainerBasic(IInventory playerInv, Entity entity) { this.entity = entity; IItemHandler handler = entity.getCapability(CapabilityItemHandler.ITEM_HANDLER_CAPABILITY, null); //Gets the inventory from our tile entity //Our tile entity slots this.addSlotToContainer(new SlotItemHandler(handler, 0, 8,8)); //The player's inventory slots int xPos = 8; //The x position of the top left player inventory slot on our texture int yPos = 84; //The y position of the top left player inventory slot on our texture //Player slots for (int y = 0; y < 3; ++y) { for (int x = 0; x < 9; ++x) { this.addSlotToContainer(new Slot(playerInv, x + y * 9 + 9, xPos + x * 18, yPos + y * 18)); } } for (int x = 0; x < 9; ++x) { this.addSlotToContainer(new Slot(playerInv, x, xPos + x * 18, yPos + 58)); } Utils.getLogger().info("Finish ContainerBasic"); } @Override public boolean canInteractWith(EntityPlayer player) { return !player.isSpectator(); } }
static variable, and that instance isnt used i just put it there for previous testing or trying to make this work
Rendering texture issue
in Modder Support
Posted
Changing the model to ModelBiped() is the exact same deal