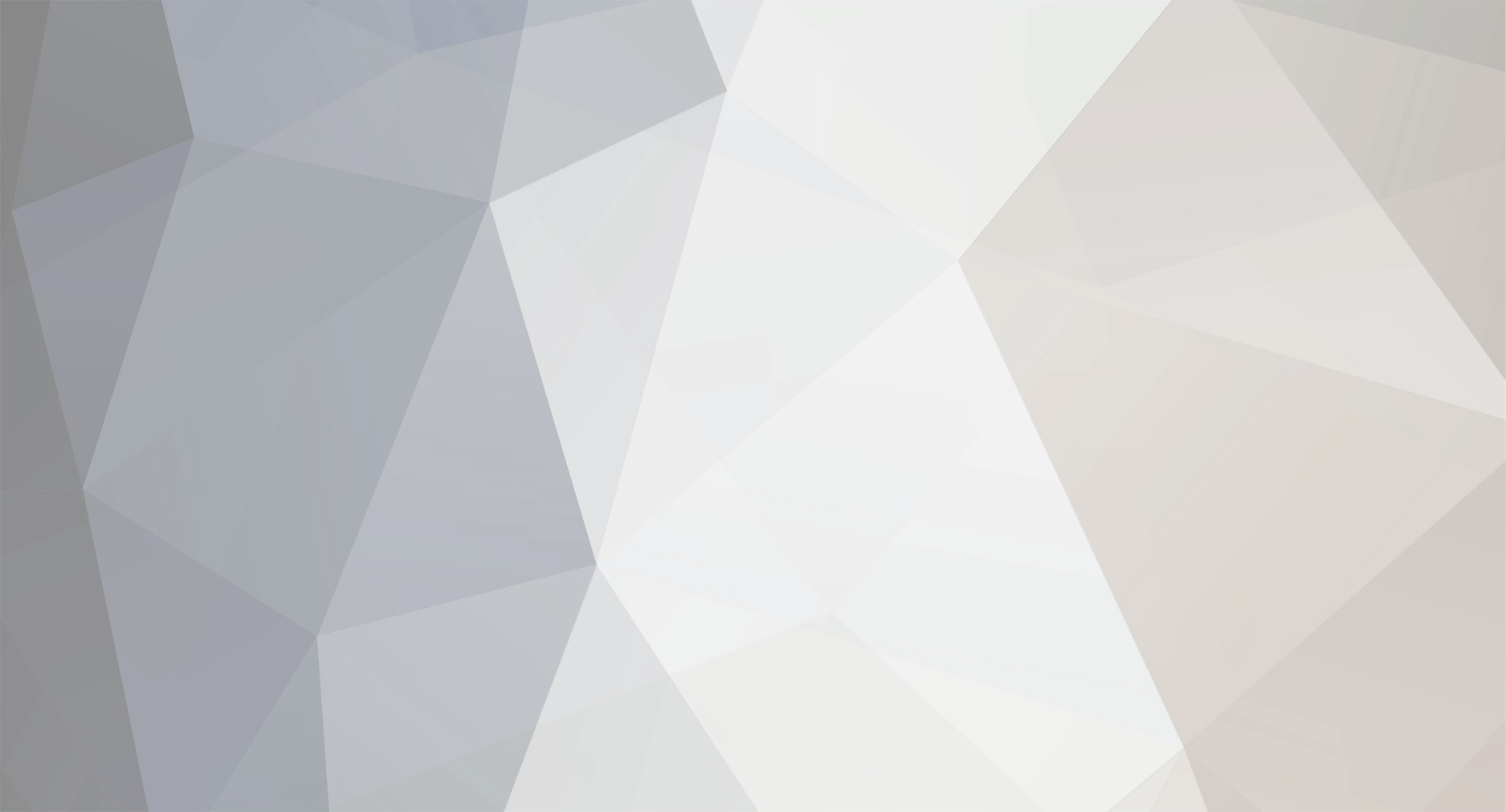
clowcadia
-
Posts
458 -
Joined
-
Last visited
Posts posted by clowcadia
-
-
5 hours ago, diesieben07 said:
You synchronize the value into the gui using the IContainerListener interface. You can see an example of how to use it in ContainerFurance.
This is because your entity doesn't save the value to NBT correctly (probably). Once you have implemented the gui-syncing using the method I described above and this is still an issue, post your full entity class.
Its only when the value is static for stomach.
-
Ok so i have a livingEntity that has a stomach value, and a container with a gui. when i inset food in one of the container slots eat consumes the item and increases the stomach value. what i am trying to do is display the value in the gui as it consumes the food. the problem i ran into is that the value display does not increase in the gui unless i re-open the gui. i was able to make the gui update its value with the stomach value as static but the problem is when i reload the world that value resets to 0 where it normally stays the same due to read/write to NBT
-
Any ideas?
-
I want to use packets to be able to use its data for updatable information in my gui from entityLiving
-
I am not very sure how to do this, I know tileEntity does have this methods that we can overide but a living entity does not seam to have so
-
How do i use packets with entityLiving?
-
Ok thank you.
Basic
package com.clowcadia.test.npc; import com.clowcadia.test.GuiHandler; import com.clowcadia.test.TestModHandler; import com.clowcadia.test.utils.Utils; import net.minecraft.block.ITileEntityProvider; import net.minecraft.entity.EntityLiving; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.init.Items; import net.minecraft.item.ItemStack; import net.minecraft.nbt.NBTTagCompound; import net.minecraft.network.NetworkManager; import net.minecraft.network.play.server.SPacketUpdateTileEntity; import net.minecraft.tileentity.TileEntity; import net.minecraft.util.EnumFacing; import net.minecraft.util.EnumHand; import net.minecraft.world.World; import net.minecraftforge.common.capabilities.Capability; import net.minecraftforge.common.capabilities.ICapabilityProvider; import net.minecraftforge.items.CapabilityItemHandler; import net.minecraftforge.items.ItemStackHandler; import scala.reflect.internal.Trees.This; public class Basic extends EntityLiving implements ICapabilityProvider{ public static Basic instance; private final ItemStackHandler handler; private String tagStomach = "Stomach"; private int stomach; private int stomachCap = 800; public Basic(World world) { super(world); this.handler = new ItemStackHandler(9); this.stomach = 0; //Utils.getLogger().info("basic constructor" +this.stomach); } public int getStomach(){ return stomach; } @Override public void readEntityFromNBT(NBTTagCompound compound) { this.handler.deserializeNBT(compound.getCompoundTag("ItemStackHandler")); this.stomach = compound.getInteger("Stomach"); if(compound.hasKey(tagStomach)) Utils.getLogger().info("Reading Stomach"); super.readEntityFromNBT(compound); } @Override public void writeEntityToNBT(NBTTagCompound compound) { super.writeEntityToNBT(compound); compound.setTag("ItemStackHandler", this.handler.serializeNBT()); compound.setInteger("Stomach", this.stomach); if(compound.hasKey(tagStomach)) Utils.getLogger().info("Writing Stomach"); } @Override public <T> T getCapability(Capability<T> capability, EnumFacing facing) { if(capability == CapabilityItemHandler.ITEM_HANDLER_CAPABILITY) return (T) this.handler; return super.getCapability(capability, facing); } @Override public boolean hasCapability(Capability<?> capability, EnumFacing facing) { if(capability == CapabilityItemHandler.ITEM_HANDLER_CAPABILITY) return true; return super.hasCapability(capability, facing); } @Override public void onEntityUpdate() { ItemStack foodStack = handler.getStackInSlot(0); if (this.world != null){ if(!this.world.isRemote){ Utils.getLogger().info("Stomach: "+stomach); if(foodStack.getUnlocalizedName().equals(Items.APPLE.getUnlocalizedName()) && stomach != stomachCap){ foodStack.splitStack(1); stomach += 40; } } } super.onEntityUpdate(); } @Override public boolean processInteract(EntityPlayer player, EnumHand hand) { if (!this.world.isRemote) { int basicID = this.getEntityId(); //for inputing into x/y/z in the opne gui to pass the entety id System.out.println("Player has interacted with the mob"); player.openGui(TestModHandler.instance, GuiHandler.BASIC, this.world, basicID,0, 0); } return true; } }
Gui Handler
package com.clowcadia.test; import java.security.PublicKey; import com.clowcadia.test.containers.ContainerBasic; import com.clowcadia.test.gui.GuiBasic; import com.clowcadia.test.npc.Basic; import net.minecraft.entity.Entity; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.util.datafix.fixes.EntityId; import net.minecraft.util.math.BlockPos; import net.minecraft.util.math.RayTraceResult; import net.minecraft.world.World; import net.minecraftforge.fml.common.network.IGuiHandler; public class GuiHandler implements IGuiHandler{ public static final int BASIC = 0; @Override public Object getServerGuiElement(int ID, EntityPlayer player, World world, int x, int y, int z) { if(ID==BASIC){ Entity basic = world.getEntityByID(x); return new ContainerBasic(player.inventory,basic); } return null; } @Override public Object getClientGuiElement(int ID, EntityPlayer player, World world, int x, int y, int z) { if(ID==BASIC){ Entity basic = world.getEntityByID(x); return new GuiBasic(player.inventory, basic); } return null; } }
GuiBasic
package com.clowcadia.test.gui; import java.util.ArrayList; import java.util.List; import com.clowcadia.test.TestModHandler; import com.clowcadia.test.containers.ContainerBasic; import com.clowcadia.test.npc.Basic; import com.clowcadia.tutorial3.Reference; import net.minecraft.client.gui.inventory.GuiContainer; import net.minecraft.client.renderer.GlStateManager; import net.minecraft.client.resources.I18n; import net.minecraft.entity.Entity; import net.minecraft.inventory.Container; import net.minecraft.inventory.IInventory; import net.minecraft.item.ItemStack; import net.minecraft.util.ResourceLocation; import net.minecraft.util.math.RayTraceResult; import net.minecraft.util.text.TextFormatting; import net.minecraft.world.World; import net.minecraftforge.common.capabilities.ICapabilityProvider; import net.minecraftforge.items.CapabilityItemHandler; import scala.reflect.internal.Trees.This; public class GuiBasic extends GuiContainer{ private IInventory playerInv; private Entity entity; private String stomachDisplay; public GuiBasic(IInventory playerInv, Entity entity) { super(new ContainerBasic(playerInv, entity)); this.xSize=176; this.ySize=166; this.playerInv = playerInv; this.entity = entity; } @Override protected void drawGuiContainerBackgroundLayer(float partialTicks, int mouseX, int mouseY) { GlStateManager.color(1.0F, 1.0F, 1.0F,1.0F); this.mc.getTextureManager().bindTexture(new ResourceLocation(TestModHandler.modId, "textures/gui/container/basic.png")); this.drawTexturedModalRect(this.guiLeft, this.guiTop, 0, 0, this.xSize,this.ySize); } /** * Draws the text that is an overlay, i.e where it says Block Breaker in the gui on the top */ @Override protected void drawGuiContainerForegroundLayer(int mouseX, int mouseY) { String s = I18n.format("container.basic"); //Gets the formatted name for the block breaker from the language file this.mc.fontRendererObj.drawString(s, this.xSize / 2 - this.mc.fontRendererObj.getStringWidth(s) / 2, 6, 4210752); //Draws the block breaker name in the center on the top of the gui this.mc.fontRendererObj.drawString(this.playerInv.getDisplayName().getFormattedText(), 8, 72, 4210752); //The player's inventory name this.mc.fontRendererObj.drawString(((Basic) entity).getStomach()+"", this.xSize / 2 - this.mc.fontRendererObj.getStringWidth(stomachDisplay) / 2, 20, 4210752); int actualMouseX = mouseX - ((this.width - this.xSize) / 2); int actualMouseY = mouseY - ((this.height - this.ySize) / 2); } }
-
8 hours ago, Jay Avery said:
If your entity data is being reset when the world is saved/loaded, that's not a problem with GUIs and Containers - it's to do with the entity itself (probably something to do with NBT). If that's still a problem, it's probably best to post a new thread about that specific topic to get more help.
The data in NBT seams to reset only when it is on static where the value of stomach variable actually adjust in gui properly. Static shows correct gui change but resets the value always on restart thats the issue. other wise NBT runs correctly but the value does not change with in gui or just shows the initial value of creation of entity 0, weather the getStomach method is return stomach; or return this.stomach;
-
29 minutes ago, Leomelonseeds said:
Huh??
Just do what you did befire.
its just an empty string, i am not worried about the centering so much as the output. what i did before does not refresh like it does on static value unfortunantly. but if i use a static value each time i exit and enter the game it starts the value over rather then reading from nbt
-
1 minute ago, Leomelonseeds said:
stomachDisplay = Basic.getStomach();
Remeber this line?
I stopped using it as a shorthand and just used the code instead (Basic.getStomach)
-
2 hours ago, Jay Avery said:
If only we can attack a video here
https://www.youtube.com/watch?v=Hn-enjcgV1o
Lol no homo, just thought it be funny
-
3 minutes ago, Leomelonseeds said:
Try replacing that with Entity basic (I doubt that will do anything)
That is saying to go back to entity rather then basic, i am assuming i should revert to my privious configurations?
-
private final ItemStackHandler handler;
it doesnt not recognize this
as the item stack in slot 0 is air even if i insert it
-
It no longer registers the fact that there are apples in the slot, i am assuming also it does not recognize the stomach values
-
package com.clowcadia.test.npc; import com.clowcadia.test.GuiHandler; import com.clowcadia.test.TestModHandler; import com.clowcadia.test.utils.Utils; import net.minecraft.block.ITileEntityProvider; import net.minecraft.entity.EntityLiving; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.init.Items; import net.minecraft.item.ItemStack; import net.minecraft.nbt.NBTTagCompound; import net.minecraft.network.NetworkManager; import net.minecraft.network.play.server.SPacketUpdateTileEntity; import net.minecraft.tileentity.TileEntity; import net.minecraft.util.EnumFacing; import net.minecraft.util.EnumHand; import net.minecraft.world.World; import net.minecraftforge.common.capabilities.Capability; import net.minecraftforge.common.capabilities.ICapabilityProvider; import net.minecraftforge.items.CapabilityItemHandler; import net.minecraftforge.items.ItemStackHandler; import scala.reflect.internal.Trees.This; public class Basic extends EntityLiving implements ICapabilityProvider{ public static Basic instance; private final ItemStackHandler handler; private String tagStomach = "Stomach"; private int stomach; private int stomachCap = 800; public Basic(World world) { super(world); this.handler = new ItemStackHandler(9); this.stomach = 0; //Utils.getLogger().info("basic constructor" +this.stomach); } public int getStomach(){ return stomach; } @Override public void readEntityFromNBT(NBTTagCompound compound) { this.handler.deserializeNBT(compound.getCompoundTag("ItemStackHandler")); this.stomach = compound.getInteger("Stomach"); if(compound.hasKey(tagStomach)) Utils.getLogger().info("Reading Stomach"); super.readEntityFromNBT(compound); } @Override public void writeEntityToNBT(NBTTagCompound compound) { super.writeEntityToNBT(compound); compound.setTag("ItemStackHandler", this.handler.serializeNBT()); compound.setInteger("Stomach", this.stomach); if(compound.hasKey(tagStomach)) Utils.getLogger().info("Writing Stomach"); } @Override public <T> T getCapability(Capability<T> capability, EnumFacing facing) { if(capability == CapabilityItemHandler.ITEM_HANDLER_CAPABILITY) return (T) this.handler; return super.getCapability(capability, facing); } @Override public boolean hasCapability(Capability<?> capability, EnumFacing facing) { if(capability == CapabilityItemHandler.ITEM_HANDLER_CAPABILITY) return true; return super.hasCapability(capability, facing); } @Override public void onEntityUpdate() { ItemStack foodStack = handler.getStackInSlot(0); if (this.world != null){ if(!this.world.isRemote){ if(foodStack.getUnlocalizedName().equals(Items.APPLE.getUnlocalizedName()) && stomach != stomachCap){ Utils.getLogger().info("Stomach: "+foodStack.getUnlocalizedName()); foodStack.splitStack(1); stomach += 40; } } } super.onEntityUpdate(); } @Override public boolean processInteract(EntityPlayer player, EnumHand hand) { if (!this.world.isRemote) { int basicID = this.getEntityId(); //for inputing into x/y/z in the opne gui to pass the entety id System.out.println("Player has interacted with the mob"); player.openGui(TestModHandler.instance, GuiHandler.BASIC, this.world, basicID,0, 0); } return true; } }
package com.clowcadia.test; import java.security.PublicKey; import com.clowcadia.test.containers.ContainerBasic; import com.clowcadia.test.gui.GuiBasic; import com.clowcadia.test.npc.Basic; import net.minecraft.entity.Entity; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.util.datafix.fixes.EntityId; import net.minecraft.util.math.BlockPos; import net.minecraft.util.math.RayTraceResult; import net.minecraft.world.World; import net.minecraftforge.fml.common.network.IGuiHandler; public class GuiHandler implements IGuiHandler{ public static final int BASIC = 0; @Override public Object getServerGuiElement(int ID, EntityPlayer player, World world, int x, int y, int z) { if(ID==BASIC){ Basic basic = new Basic(world); return new ContainerBasic(player.inventory,basic); } return null; } @Override public Object getClientGuiElement(int ID, EntityPlayer player, World world, int x, int y, int z) { if(ID==BASIC){ //Entity entity = world.getEntityByID(x); Basic basic = new Basic(world); return new GuiBasic(player.inventory, basic);//entity, } return null; } }
package com.clowcadia.test.gui; import java.util.ArrayList; import java.util.List; import com.clowcadia.test.TestModHandler; import com.clowcadia.test.containers.ContainerBasic; import com.clowcadia.test.npc.Basic; import com.clowcadia.tutorial3.Reference; import net.minecraft.client.gui.inventory.GuiContainer; import net.minecraft.client.renderer.GlStateManager; import net.minecraft.client.resources.I18n; import net.minecraft.entity.Entity; import net.minecraft.inventory.Container; import net.minecraft.inventory.IInventory; import net.minecraft.item.ItemStack; import net.minecraft.util.ResourceLocation; import net.minecraft.util.math.RayTraceResult; import net.minecraft.util.text.TextFormatting; import net.minecraft.world.World; import net.minecraftforge.common.capabilities.ICapabilityProvider; import net.minecraftforge.items.CapabilityItemHandler; import scala.reflect.internal.Trees.This; public class GuiBasic extends GuiContainer{ private IInventory playerInv; private Entity entity; private String stomachDisplay; private World world; private Basic basic = new Basic(world); public GuiBasic(IInventory playerInv, Basic basic) {//Entity entity, super(new ContainerBasic(playerInv, basic)); this.xSize=176; this.ySize=166; this.playerInv = playerInv; this.basic = basic; } @Override protected void drawGuiContainerBackgroundLayer(float partialTicks, int mouseX, int mouseY) { GlStateManager.color(1.0F, 1.0F, 1.0F,1.0F); this.mc.getTextureManager().bindTexture(new ResourceLocation(TestModHandler.modId, "textures/gui/container/basic.png")); this.drawTexturedModalRect(this.guiLeft, this.guiTop, 0, 0, this.xSize,this.ySize); } /** * Draws the text that is an overlay, i.e where it says Block Breaker in the gui on the top */ @Override protected void drawGuiContainerForegroundLayer(int mouseX, int mouseY) { String s = I18n.format("container.basic"); //Gets the formatted name for the block breaker from the language file this.mc.fontRendererObj.drawString(s, this.xSize / 2 - this.mc.fontRendererObj.getStringWidth(s) / 2, 6, 4210752); //Draws the block breaker name in the center on the top of the gui this.mc.fontRendererObj.drawString(this.playerInv.getDisplayName().getFormattedText(), 8, 72, 4210752); //The player's inventory name this.mc.fontRendererObj.drawString(basic.getStomach()+"", this.xSize / 2 - this.mc.fontRendererObj.getStringWidth(stomachDisplay) / 2, 20, 4210752); int actualMouseX = mouseX - ((this.width - this.xSize) / 2); int actualMouseY = mouseY - ((this.height - this.ySize) / 2); } }
package com.clowcadia.test.containers; import com.clowcadia.test.npc.Basic; import com.clowcadia.test.utils.Utils; import net.minecraft.entity.Entity; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.inventory.Container; import net.minecraft.inventory.IInventory; import net.minecraft.inventory.Slot; import net.minecraft.world.World; import net.minecraftforge.common.capabilities.ICapabilityProvider; import net.minecraftforge.items.CapabilityItemHandler; import net.minecraftforge.items.IItemHandler; import net.minecraftforge.items.SlotItemHandler; public class ContainerBasic extends Container{ //public Entity entity; public Basic basic; public ContainerBasic(IInventory playerInv, Basic basic) { this.basic = basic; IItemHandler handler = basic.getCapability(CapabilityItemHandler.ITEM_HANDLER_CAPABILITY, null); //Gets the inventory from our tile entity //Our tile entity slots this.addSlotToContainer(new SlotItemHandler(handler, 0, 8,8)); //The player's inventory slots int xPos = 8; //The x position of the top left player inventory slot on our texture int yPos = 84; //The y position of the top left player inventory slot on our texture //Player slots for (int y = 0; y < 3; ++y) { for (int x = 0; x < 9; ++x) { this.addSlotToContainer(new Slot(playerInv, x + y * 9 + 9, xPos + x * 18, yPos + y * 18)); } } for (int x = 0; x < 9; ++x) { this.addSlotToContainer(new Slot(playerInv, x, xPos + x * 18, yPos + 58)); } Utils.getLogger().info("Finish ContainerBasic"); } @Override public boolean canInteractWith(EntityPlayer player) { return !player.isSpectator(); } }
-
No errors, but i lost functionality in consuming items
-
?? Please explain more literally, that way if i need to learn some java i can look up the right material.
-
because i cant get the method that getStomach with out a cast or the actual class instance i believe
-
package com.clowcadia.test.npc; import com.clowcadia.test.GuiHandler; import com.clowcadia.test.TestModHandler; import com.clowcadia.test.utils.Utils; import net.minecraft.block.ITileEntityProvider; import net.minecraft.entity.EntityLiving; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.init.Items; import net.minecraft.item.ItemStack; import net.minecraft.nbt.NBTTagCompound; import net.minecraft.network.NetworkManager; import net.minecraft.network.play.server.SPacketUpdateTileEntity; import net.minecraft.tileentity.TileEntity; import net.minecraft.util.EnumFacing; import net.minecraft.util.EnumHand; import net.minecraft.world.World; import net.minecraftforge.common.capabilities.Capability; import net.minecraftforge.common.capabilities.ICapabilityProvider; import net.minecraftforge.items.CapabilityItemHandler; import net.minecraftforge.items.ItemStackHandler; import scala.reflect.internal.Trees.This; public class Basic extends EntityLiving implements ICapabilityProvider{ public static Basic instance; private final ItemStackHandler handler; private String tagStomach = "Stomach"; private int stomach; private int stomachCap = 800; public Basic(World world) { super(world); this.handler = new ItemStackHandler(9); this.stomach = 0; //Utils.getLogger().info("basic constructor" +this.stomach); } public int getStomach(){ return stomach; } @Override public void readEntityFromNBT(NBTTagCompound compound) { this.handler.deserializeNBT(compound.getCompoundTag("ItemStackHandler")); this.stomach = compound.getInteger("Stomach"); if(compound.hasKey(tagStomach)) Utils.getLogger().info("Reading Stomach"); super.readEntityFromNBT(compound); } @Override public void writeEntityToNBT(NBTTagCompound compound) { super.writeEntityToNBT(compound); compound.setTag("ItemStackHandler", this.handler.serializeNBT()); compound.setInteger("Stomach", this.stomach); if(compound.hasKey(tagStomach)) Utils.getLogger().info("Writing Stomach"); } @Override public <T> T getCapability(Capability<T> capability, EnumFacing facing) { if(capability == CapabilityItemHandler.ITEM_HANDLER_CAPABILITY) return (T) this.handler; return super.getCapability(capability, facing); } @Override public boolean hasCapability(Capability<?> capability, EnumFacing facing) { if(capability == CapabilityItemHandler.ITEM_HANDLER_CAPABILITY) return true; return super.hasCapability(capability, facing); } @Override public void onEntityUpdate() { ItemStack foodStack = handler.getStackInSlot(0); if (this.world != null){ if(!this.world.isRemote){ if(foodStack.getUnlocalizedName().equals(Items.APPLE.getUnlocalizedName()) && stomach != stomachCap){ Utils.getLogger().info("Stomach: "+foodStack.getUnlocalizedName()); foodStack.splitStack(1); stomach += 40; } } } super.onEntityUpdate(); } @Override public boolean processInteract(EntityPlayer player, EnumHand hand) { if (!this.world.isRemote) { int basicID = this.getEntityId(); //for inputing into x/y/z in the opne gui to pass the entety id System.out.println("Player has interacted with the mob"); player.openGui(TestModHandler.instance, GuiHandler.BASIC, this.world, basicID,0, 0); } return true; } }
-
Basic is my entity
-
package com.clowcadia.test; import java.security.PublicKey; import com.clowcadia.test.containers.ContainerBasic; import com.clowcadia.test.gui.GuiBasic; import com.clowcadia.test.npc.Basic; import net.minecraft.entity.Entity; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.util.datafix.fixes.EntityId; import net.minecraft.util.math.BlockPos; import net.minecraft.util.math.RayTraceResult; import net.minecraft.world.World; import net.minecraftforge.fml.common.network.IGuiHandler; public class GuiHandler implements IGuiHandler{ public static final int BASIC = 0; @Override public Object getServerGuiElement(int ID, EntityPlayer player, World world, int x, int y, int z) { if(ID==BASIC){ Entity basic = world.getEntityByID(x); return new ContainerBasic(player.inventory,basic); } return null; } @Override public Object getClientGuiElement(int ID, EntityPlayer player, World world, int x, int y, int z) { if(ID==BASIC){ Entity entity = world.getEntityByID(x); Basic basic = new Basic(world); return new GuiBasic(player.inventory, entity,basic); } return null; } }
package com.clowcadia.test.gui; import java.util.ArrayList; import java.util.List; import com.clowcadia.test.TestModHandler; import com.clowcadia.test.containers.ContainerBasic; import com.clowcadia.test.npc.Basic; import com.clowcadia.tutorial3.Reference; import net.minecraft.client.gui.inventory.GuiContainer; import net.minecraft.client.renderer.GlStateManager; import net.minecraft.client.resources.I18n; import net.minecraft.entity.Entity; import net.minecraft.inventory.Container; import net.minecraft.inventory.IInventory; import net.minecraft.item.ItemStack; import net.minecraft.util.ResourceLocation; import net.minecraft.util.math.RayTraceResult; import net.minecraft.util.text.TextFormatting; import net.minecraft.world.World; import net.minecraftforge.common.capabilities.ICapabilityProvider; import net.minecraftforge.items.CapabilityItemHandler; import scala.reflect.internal.Trees.This; public class GuiBasic extends GuiContainer{ private IInventory playerInv; private Entity entity; private String stomachDisplay; private World world; private Basic basic = new Basic(world); public GuiBasic(IInventory playerInv, Entity entity, Basic basic) { super(new ContainerBasic(playerInv, entity)); this.xSize=176; this.ySize=166; this.playerInv = playerInv; this.basic = basic; } @Override protected void drawGuiContainerBackgroundLayer(float partialTicks, int mouseX, int mouseY) { GlStateManager.color(1.0F, 1.0F, 1.0F,1.0F); this.mc.getTextureManager().bindTexture(new ResourceLocation(TestModHandler.modId, "textures/gui/container/basic.png")); this.drawTexturedModalRect(this.guiLeft, this.guiTop, 0, 0, this.xSize,this.ySize); } /** * Draws the text that is an overlay, i.e where it says Block Breaker in the gui on the top */ @Override protected void drawGuiContainerForegroundLayer(int mouseX, int mouseY) { String s = I18n.format("container.basic"); //Gets the formatted name for the block breaker from the language file this.mc.fontRendererObj.drawString(s, this.xSize / 2 - this.mc.fontRendererObj.getStringWidth(s) / 2, 6, 4210752); //Draws the block breaker name in the center on the top of the gui this.mc.fontRendererObj.drawString(this.playerInv.getDisplayName().getFormattedText(), 8, 72, 4210752); //The player's inventory name this.mc.fontRendererObj.drawString(basic.getStomach()+"", this.xSize / 2 - this.mc.fontRendererObj.getStringWidth(stomachDisplay) / 2, 20, 4210752); int actualMouseX = mouseX - ((this.width - this.xSize) / 2); int actualMouseY = mouseY - ((this.height - this.ySize) / 2); } }
-
its not working still value is 0 when it has been adjusted
-
edited last post with out the instance suggestion
-
package com.clowcadia.test.gui; import java.util.ArrayList; import java.util.List; import com.clowcadia.test.TestModHandler; import com.clowcadia.test.containers.ContainerBasic; import com.clowcadia.test.npc.Basic; import com.clowcadia.tutorial3.Reference; import net.minecraft.client.gui.inventory.GuiContainer; import net.minecraft.client.renderer.GlStateManager; import net.minecraft.client.resources.I18n; import net.minecraft.entity.Entity; import net.minecraft.inventory.Container; import net.minecraft.inventory.IInventory; import net.minecraft.item.ItemStack; import net.minecraft.util.ResourceLocation; import net.minecraft.util.math.RayTraceResult; import net.minecraft.util.text.TextFormatting; import net.minecraft.world.World; import net.minecraftforge.common.capabilities.ICapabilityProvider; import net.minecraftforge.items.CapabilityItemHandler; public class GuiBasic extends GuiContainer{ private IInventory playerInv; private Entity entity; private String stomachDisplay; private GuiBasic basic= new Basic public GuiBasic(IInventory playerInv, Entity entity) { super(new ContainerBasic(playerInv, entity)); this.xSize=176; this.ySize=166; this.playerInv = playerInv; this.entity = entity; } @Override protected void drawGuiContainerBackgroundLayer(float partialTicks, int mouseX, int mouseY) { GlStateManager.color(1.0F, 1.0F, 1.0F,1.0F); this.mc.getTextureManager().bindTexture(new ResourceLocation(TestModHandler.modId, "textures/gui/container/basic.png")); this.drawTexturedModalRect(this.guiLeft, this.guiTop, 0, 0, this.xSize,this.ySize); } /** * Draws the text that is an overlay, i.e where it says Block Breaker in the gui on the top */ @Override protected void drawGuiContainerForegroundLayer(int mouseX, int mouseY) { String s = I18n.format("container.basic"); //Gets the formatted name for the block breaker from the language file this.mc.fontRendererObj.drawString(s, this.xSize / 2 - this.mc.fontRendererObj.getStringWidth(s) / 2, 6, 4210752); //Draws the block breaker name in the center on the top of the gui this.mc.fontRendererObj.drawString(this.playerInv.getDisplayName().getFormattedText(), 8, 72, 4210752); //The player's inventory name this.mc.fontRendererObj.drawString(((Basic) this.entity).getStomach()+"", this.xSize / 2 - this.mc.fontRendererObj.getStringWidth(stomachDisplay) / 2, 20, 4210752); int actualMouseX = mouseX - ((this.width - this.xSize) / 2); int actualMouseY = mouseY - ((this.height - this.ySize) / 2); } }
Creating Packets in EntityLiving
in Modder Support
Posted