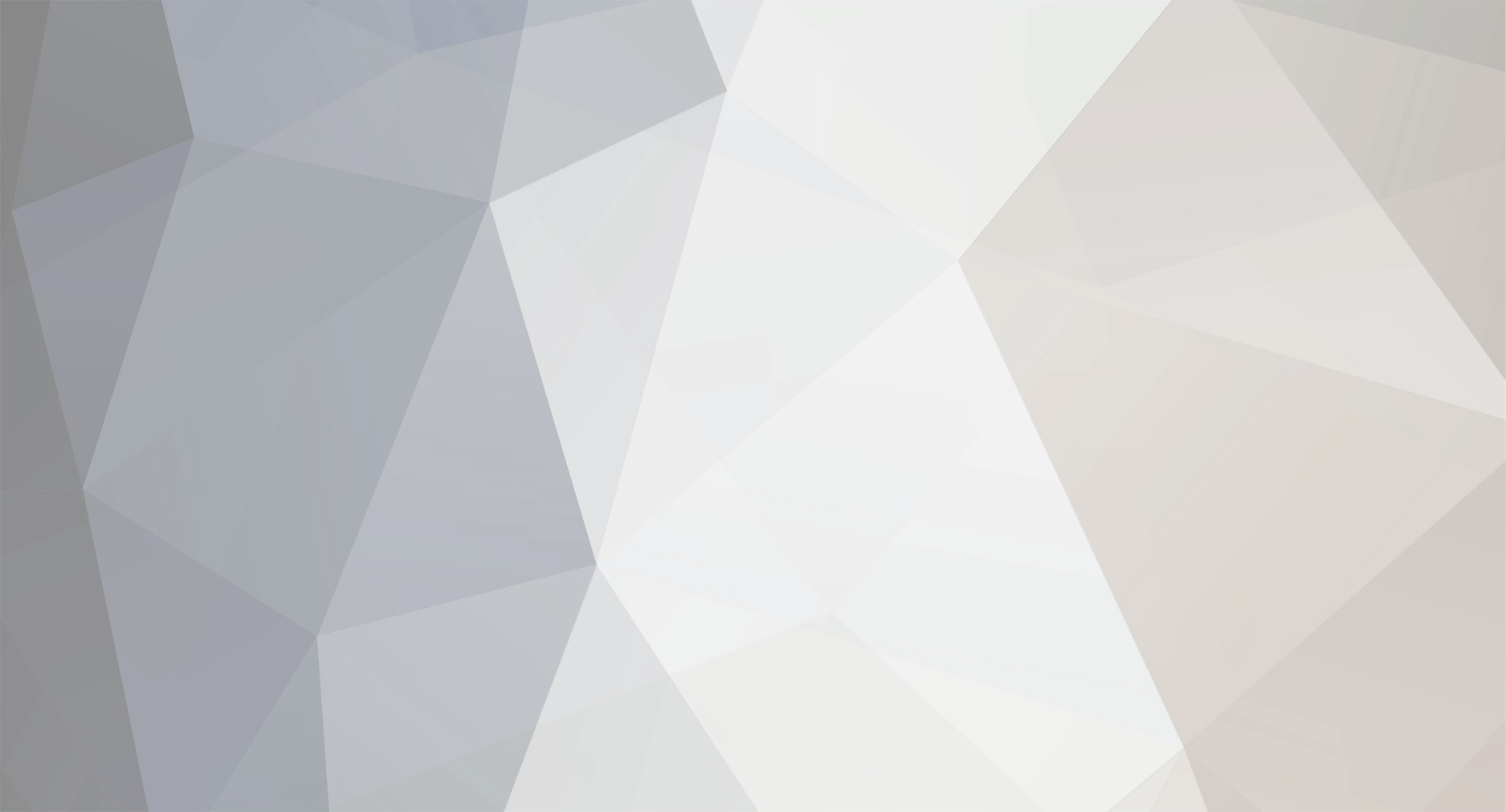
Big_Bad_E
Members-
Posts
312 -
Joined
-
Last visited
-
Days Won
1
Everything posted by Big_Bad_E
-
I am trying to simulate a player clicking to pick up and put down items in a ChestGUI (A macro for crafting certain items in a custom crafting table). I found the following method: PlayerControllerMP#windowClick(int windowId, int slotId, int mouseButtonClicked, int mode, EntityPlayer playerIn); I found a few things with it: - windowID is the Id of a Container, which doesn't work with the bottom Gui of a ChestGUI (A InventoryPlayer). - mouseButtonClicked is 0 for left click, 1 for right click. - Mode is either 0, 1, 3, or 5. 1 is used for picking up item, 0, is used for putting it down, 3 and 5 are used for dragging. Now my problem: - The top IInventory (InventoryPlayer) has no ID. - The bottom IInventory (ContainerLocalMenu) has no ID. It extends InventoryBasic but that has nothing.
-
I am in 1.14.2, thanks for telling me this. Yes, I know the code is stupid, but what gets passed to me is a PlayerEntity, and I need a ServerPlayerEntity for NetworkHooks#openGui(). I just realized I forgot !world.isRemote when I did that, my problem is fixed now by casting player to ServerPlayerEntity server-side.
-
I just need some clarification with opening a container to the player. I am trying to make a custom Crafting Table, I registered all my custom recipes in a custom Registry, but now I need to open the Container. Currently I am calling this: @Override public boolean onBlockActivated(BlockState state, World worldIn, BlockPos pos, PlayerEntity player, Hand handIn, BlockRayTraceResult hit) { BlockPos playerPos = player.getPosition(); double distance = pos.distanceSq(playerPos); if(player.isCreative() && distance <= 5 || !player.isCreative() && distance <= 3) { ServerPlayerEntity playerEntity = null; if (!worldIn.isRemote) { for (ServerPlayerEntity entity : worldIn.getServer().getPlayerList().getPlayers()) if (entity.getUniqueID().equals(player.getUniqueID())) { playerEntity = entity; break; } NetworkHooks.openGui(playerEntity, new CulinaryWorkbenchContainerProvider(pos)); } return false; } return true; } This goes to my CulinaryWorkbenchContainerProvider: public class CulinaryWorkbenchContainerProvider implements INamedContainerProvider { private BlockPos pos; public CulinaryWorkbenchContainerProvider(BlockPos pos) { this.pos = pos; } @Override public ITextComponent getDisplayName() { return ITextComponent.Serializer.fromJson("culinaryworkbench.name"); } @Nullable @Override public Container createMenu(int windowID, PlayerInventory playerInventory, PlayerEntity playerEntity) { return new CulinaryWorkbenchContainer(windowID, playerInventory, new PacketBuffer(Unpooled.buffer(8, 8)).writeBlockPos(pos)); } } And then my Container: public CulinaryWorkbenchContainer(int windowId, PlayerInventory playerInventory, PacketBuffer extraData) { super(ContainerType.CRAFTING, windowId); } Is this the correct usage? It seems that MinecraftServer#getPlayerList() is returning null, how else can I get the ServerPlayerEntity instance?
-
[1.12.1] Tell me how to create your item with a model .obj
Big_Bad_E replied to GreatWolf's topic in Modder Support
Ohh, yeah that makes more sense. Also, the scale should be 16x16. Any editor should show the xyz coordinates of where you have selected. -
Your right, that would only work for vanilla ores. I'd suggest looking at the ghost block's code. https://github.com/AbrarSyed/SecretRoomsMod-forge/tree/master/src/main/java/com/wynprice/secretroomsmod/render Classes of interest: https://github.com/AbrarSyed/SecretRoomsMod-forge/blob/master/src/main/java/com/wynprice/secretroomsmod/base/BaseFakeBlock.java https://github.com/AbrarSyed/SecretRoomsMod-forge/blob/master/src/main/java/com/wynprice/secretroomsmod/render/fakemodels/FakeBlockModel.java https://github.com/AbrarSyed/SecretRoomsMod-forge/blob/master/src/main/java/com/wynprice/secretroomsmod/base/interfaces/ISecretBlock.java Use this to get the IBakedModel from a BlockState: Minecraft.getMinecraft().getBlockRendererDispatcher().getModelForState(state); Getting it from a ResourceLocation: IBakedModel bakedModel; IModel model; try { model = ModelLoaderRegistry.getModel(resourceLocation); } catch (Exception e) { throw new RuntimeException(e); } bakedModel = model.bake(TRSRTransformation.identity(), DefaultVertexFormats.BLOCK, location -> Minecraft.getMinecraft().getTextureMapBlocks().getAtlasSprite(location.toString()));
-
I believe you can use a custom IStateMapper to set the blockstate of your block to the model of the vanilla/modded ore block.
-
[1.12.1] Tell me how to create your item with a model .obj
Big_Bad_E replied to GreatWolf's topic in Modder Support
The problem you have is software like blender makes large files. json models are smaller to make. If you want to reduce the size on an obj model: 1. Check the export quality on the software, Minecraft can have a low quality texture 2. Remove high-poly objects, you don't need a very detailed model. -
[1.12.2] Is there a tile entity equivelent for items?
Big_Bad_E replied to Mekelaina's topic in Modder Support
It depends on what you want your item to do. Capabilities are good for storing data, so it can be accessed outside of the class, or to show that it can do something. Most items are just used on click. What do you want your item to do? -
Forge not finding texture+blockstate, but shows the right path?
Big_Bad_E replied to Big_Bad_E's topic in Modder Support
I don't know where I saw to put it in postInit, fixed that! -
Because Intellij is annoying, and you never know. Makes it easier to debug I guess, also personal preference. Also, yes, never blindly copy paste code, and if you are stuck you can always get code from vanilla blocks (that is where that code is from anyways) I would suggest playing around in Java with other things, Forge is one of the hardest libraries out there. If you want to stick with Minecraft, Spigot is a lot easier. You could also mess around with Discord bots (https://github.com/DV8FromTheWorld/JDA/releases) or make games/software.
-
placer.getHorizontalFacing().getOpposite() That line is what "checks the player" (checks where they are facing). It seems a remap might of changed it, try using: PropertyEnum<EnumFacing> instead of PropertyDirection and set it to be: new PropertyEnum<EnumFacing>("facing", EnumFacing.class, Collections2.filter(Lists.newArrayList(EnumFacing.values()), EnumFacing.Plane.HORIZONTAL);
-
Sorry totally managed to misread that! I currently cannot test for 1.13.2 (gradle hates me :/), but the code should not change to my knowledge. If it does not work, what methods are missing/what is the error? I have read through the notes and have not seen any changes to IProperty or any non-deprecated methods.
-
I am trying to make a mod with a block similar to the enchanting table, but with some more enchants. I tried to copy the enchanting table, but am having some problems with textures. 1. The TESR for the book is not rendering 2. The blockstate is not found 3. The no textures are found 4. No models are found. I created a git for it, https://github.com/BigBadE/AdvancedEnchantments Here are the errors: On GUI open: It says it can't find advancedenchantments:textures/entity/simple_table_book.png but I have the png at the path assets/advancedenchantments/textures/entity/simple_table_book.png. Also it says it can't find a variant for minecraft:air#simple_table. Whenever I open the GUI for the first time, everything draws correctly except items, but then it instantly closes. After that it has the purple texture and instantly closes. (I have a static ResourceLocation for the texture) It seems the drawBackground method is called twice before it closes with the pink texture, but only once with the normal texture.
-
I understand what is going on, you iterate through all registered blocks, check if the modid is equal to yours, and if so create a new itemblock using it and set the registry name. I saw the code from another response of your and started using it cause it's a good idea (might be slow with a lot of mods though). Also, didn't check and see that there was an updated version of getValues() and that it was only there for legacy support.
-
If you want to make a block, just make a class extending block, set the registryname and unlocalizedname in there. Then make a class for registering them. add @EventBusSubscriber over the line saying class (classname) Then add this method: @SubscribeEvent public static void onBlockRegister(RegistryEvent.Register<Block> event) { event.getRegistry().registerAll(new YourBlock(), new YourOtherBlock()); } Then add this too to register the itemblocks (items you hold): @SubscribeEvent public static void onItemRegister(RegistryEvent.Register<Item> event) { for (Block block : ForgeRegistries.BLOCKS.getValues()) { if (block.getRegistryName().getResourceDomain().equals(MOD_ID)) { event.getRegistry().register(new ItemBlock(block).setRegistryName(block.getRegistryName()); } } If you want to get them just do /give @p modid:blockname. (Substitute MOD_ID and modid with your modid, blockname with the block name)
-
How to do rotation: 1. Store the direction Add this to your block class: public static final PropertyDirection FACING = BlockHorizontal.FACING; This just stores the direction. 2. Tell forge that direction is part of the state You need to tell forge direction is part of the state, so it can load it from the blockstate. Add this to your block's code: setDefaultState(getDefaultState().withProperty(FACING, EnumFacing.NORTH) (Add it to the constructor) 3. Set the state when the block is placed. You have to set the state when the block is placed. Add this to your block class: @Override @Nonnull public IBlockState getStateForPlacement(@Nullable World world, @Nullable BlockPos pos, @Nullable EnumFacing facing, float hitX, float hitY, float hitZ, int meta, @Nullable EntityLivingBase placer, EnumHand hand) { assert placer != null; return this.getDefaultState().withProperty(FACING, placer.getHorizontalFacing().getOpposite()); } The code placer.getHorizontalFacing().getOpposite() Just gets the horizontal facing direction (where you are looking along the x and z axis) then gets the opposite so the block faces you. 4. Add it to your blockstate This is an example of a blockstate with facing (works in 1.12.2): { "variants": { "facing=north": { "model": "modid:model" }, "facing=west": { "model": "modid:model", "y": 90 }, "facing=south": { "model": "modid:model", "y": 180 }, "facing=east": { "model": "modid:model", "y": 270 } } } (replace modid with your modid, model with the model resource location)
-
Don't use ID, you can just have a static instance of the Block in your registry class.
-
When I try to add HWYLA to the build.gradle so that my mod integrates with it, but gradle cannot find it and no libraries are imported. Here is my build.gradle: buildscript { repositories { jcenter() maven { url = "http://files.minecraftforge.net/maven" } } dependencies { classpath 'net.minecraftforge.gradle:ForgeGradle:2.3-SNAPSHOT' } } apply plugin: 'net.minecraftforge.gradle.forge' //Only edit below this line, the above code adds and enables the necessary things for Forge to be setup. version = "1.0" group = "bigbade.automechanica" // http://maven.apache.org/guides/mini/guide-naming-conventions.html archivesBaseName = "automechanica" sourceCompatibility = targetCompatibility = '1.8' // Need this here so eclipse task generates correctly. compileJava { sourceCompatibility = targetCompatibility = '1.8' } minecraft { version = "1.12.2-14.23.5.2768" runDir = "run" // the mappings can be changed at any time, and must be in the following format. // snapshot_YYYYMMDD snapshot are built nightly. // stable_# stables are built at the discretion of the MCP team. // Use non-default mappings at your own risk. they may not always work. // simply re-run your setup task after changing the mappings to update your workspace. mappings = "snapshot_20171003" // makeObfSourceJar = false // an Srg named sources jar is made by default. uncomment this to disable. } repositories { maven {url "http://tehnut.info/maven"} } dependencies { // you may put jars on which you depend on in ./libs // or you may define them like so.. //compile "some.group:artifact:version:classifier" //compile "some.group:artifact:version" // real examples //compile 'com.mod-buildcraft:buildcraft:6.0.8:dev' // adds buildcraft to the dev env //compile 'com.googlecode.efficient-java-matrix-library:ejml:0.24' // adds ejml to the dev env // the 'provided' configuration is for optional dependencies that exist at compile-time but might not at runtime. //provided 'com.mod-buildcraft:buildcraft:6.0.8:dev' // the deobf configurations: 'deobfCompile' and 'deobfProvided' are the same as the normal compile and provided, // except that these dependencies get remapped to your current MCP mappings //deobfCompile 'com.mod-buildcraft:buildcraft:6.0.8:dev' //deobfProvided 'com.mod-buildcraft:buildcraft:6.0.8:dev' // for more info... // http://www.gradle.org/docs/current/userguide/artifact_dependencies_tutorial.html // http://www.gradle.org/docs/current/userguide/dependency_management.html deobfCompile 'mcp.mobius.waila:Hwyla:1.8.26-B41_1.12.2' } processResources { // this will ensure that this task is redone when the versions change. inputs.property "version", project.version inputs.property "mcversion", project.minecraft.version // replace stuff in mcmod.info, nothing else from(sourceSets.main.resources.srcDirs) { include 'mcmod.info' // replace version and mcversion expand 'version':project.version, 'mcversion':project.minecraft.version } // copy everything else except the mcmod.info from(sourceSets.main.resources.srcDirs) { exclude 'mcmod.info' } }
-
You have to call player.openGUI(INSTANCE (Main mod instance), id (0 if it is the first GUI your mod has), other args...). Then you have to make a GUI handler like this: public class GuiHandler implements IGuiHandler { @Nullable @Override public Object getServerGuiElement(int ID, EntityPlayer player, World world, int x, int y, int z) { if(ID == 0) return new YourContainer(); return null; } @Nullable @Override public Object getClientGuiElement(int ID, EntityPlayer player, World world, int x, int y, int z) { if(ID == 0) return new YourGuiScreen(YourContainer()); return null; } } Then register it in your load method: NetworkRegistry.INSTANCE.registerGuiHandler(INSTANCE(Main mod instance), new GuiHandler());
-
That was it, thanks!
-
Then how do I get the correct instance that update is being called on? world.getTileEntity is giving me the wrong one.
-
It seems there are two instances of the TileEntity in the same position. By printing "this", I see two different TE instances, one gotten through world.getTileEntity, and never has the update method called, and one that has update called, but is not returned by the getTileEntity method. I am guessing this is because one is being run on client and the other is server side. I have my proxy setup, but I have two questions: 1. Which side should is the update method called on, and which side is neighborChanged() in the class Block called in? 2. How should I set the side? (Through proxy or through the annotation?)
-
I just posted it to GitHub as that is easier I tried to mimic EnderIO's multiple TEs/blocks one class system. The only other things there is the Creative tab. Im an idiot, https://github.com/BigBadE/AutoMechanica/