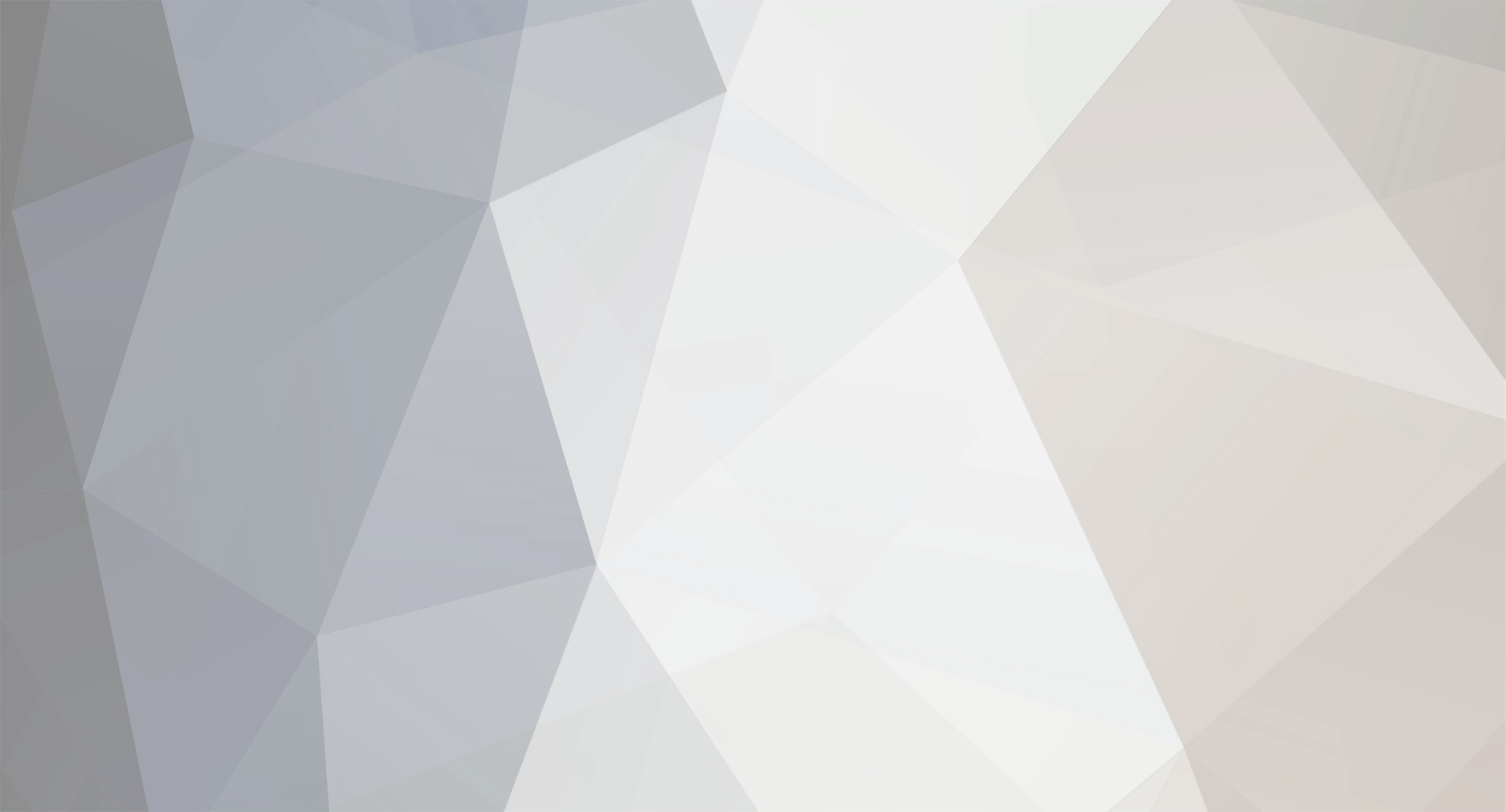
Big_Bad_E
Members-
Posts
312 -
Joined
-
Last visited
-
Days Won
1
Everything posted by Big_Bad_E
-
Custom Furnace GUI displays incorrect fuel left [1.12.2]
Big_Bad_E replied to thedarkcolour's topic in Modder Support
Always check your values before assuming your code is wrong! -
Custom Furnace GUI displays incorrect fuel left [1.12.2]
Big_Bad_E replied to thedarkcolour's topic in Modder Support
Use outprints to check of the burnlength is the right value. Send a spoiler with the outprints of k. -
Replace it with EnumFacing.getHorizontal(meta);
-
Replace EnumFacing.fromAngle(player.rotationYaw); with placer.getHorizontalFacing().getOpposite();
-
You have it set so there is up or down values, but just replace it with a horizontal value. remove that and add this: @Override @Nonnull public IBlockState getStateForPlacement(@Nullable World world, @Nullable BlockPos pos, @Nullable EnumFacing facing, float hitX, float hitY, float hitZ, int meta, @Nullable EntityLivingBase placer, EnumHand hand) { assert placer != null; return this.getDefaultState().withProperty(FACING, placer.getHorizontalFacing().getOpposite()); }
-
I am trying to keep a hashmap in my TileEntity of all the neighbors which have the power capability. I can get and store the neighbors, but for some reason every tick the hashmap clears. Code: Update method: @Override public void update() { if (lastConsume == 0) { if (fuel > 0 && power < max-production) { lastConsume = type.isFuel(getStack()); power += production; ITEMHANDLER.extractItem(0, 1, false); fuel -= 1; onConsume(); markDirty(); } else { getWorld().setBlockState(pos, getWorld().getBlockState(getPos()).cycleProperty(MachineBlock.LIT)); lastConsume -= 1; markDirty(); } } else if (lastConsume == -1) { if (fuel > 0 && power < max-production) { power += production; lastConsume = type.isFuel(getStack()); getWorld().setBlockState(pos, getWorld().getBlockState(getPos()).cycleProperty(MachineBlock.LIT)); ITEMHANDLER.extractItem(0, 1, false); fuel -= 1; onConsume(); markDirty(); } } else if(power < max-production){ lastConsume -= 1; power += production; } if(power > exportSpeed) { if (power > exportSpeed*neighbors.size()) { System.out.println(neighbors.size()); for(EnumFacing face : neighbors.keySet()) System.out.println("Power at " + face.getName()); for (IEnergyStorage storage : neighbors.values()) { power -= storage.receiveEnergy(exportSpeed, false); } } else { for (IEnergyStorage storage : neighbors.values()) { power -= storage.receiveEnergy(exportSpeed, false); if (power < exportSpeed) return; } } } } Neighbor finding method (Called in the block neighborUpdated method): public void onNeighborUpdated(BlockPos pos, World world, EnumFacing face, BlockPos fromPos) { if(world.getBlockState(fromPos).getBlock() == Blocks.AIR) { System.out.println("Removing neighbor!"); neighbors.remove(face); } TileEntity neighbor = world.getTileEntity(fromPos); if(neighbor == null) return; if(neighbor.hasCapability(CapabilityEnergy.ENERGY, face)) { System.out.println("Added neighbor at " + face.getName()); neighbors.put(face, neighbor.getCapability(CapabilityEnergy.ENERGY, face)); System.out.println(neighbors.size()); } } Removing neighbor! is not printed unless the block is removed, but every tick the hashmap is cleared. Whenever I add a neighbor, the hashmap length is the correct length. So basically: In update(), the hashmap is the empty, in onNeighborUpdated(), the hashmap is correct.
-
Bumping, after looking through EnderIO it seems that it uses a pathing system, so energy is never stored on the cables. I found the generators in the code, but can't find how it pushes power. (Do the pipes do that automatically?).
-
How would I push energy to modded cables/machines? I know using: te.hasCapability(CapabilityEnergy.ENERGY, side) I can check if it can receive energy, but I cannot push to it as I do not know how I would. Current 1.12.2 energy types that I know of (and would like to be able to push to): Tesla RF FE I know how to push to FE, I can just check if it implements IEnergyStorage and call the push method, but what about RF and Tesla? I would also like it to work with EnderIO, but I have no idea how to add support for EnderIO either because the conduits don't have any energy methods or implement any capability interfaces.
-
Suprised Intellij didn’t tell me that, thanks for the response.
-
Well thanks anyways!
-
I am trying to make the block model change depending on the IProperty, and the initial value is set correctly, but when I call IBlockState#cycleValue(property), the model (and value) is not changed. I tried using TileEntity#markDirty() and overriding markDirty() and explicit calling it after I cycle the value, but the value doesn't change. Current code to change values: @Override public void update() { if (lastConsume == 0) { System.out.println("Consuming coal"); if (coal.getCount() > 0) { lastConsume = 400; power += 5; coal.setCount(coal.getCount()-1); markDirty(); } else { System.out.println("Out of coal!"); getWorld().getBlockState(getPos()).cycleProperty(CoalGeneratorBlock.LIT); lastConsume -= 1; markDirty(); } } else if(lastConsume == -1) { if(coal.getCount() > 0) { power += 5; lastConsume = 400; System.out.println(getWorld().getBlockState(getPos()).getValue(CoalGeneratorBlock.LIT) + " consume"); getWorld().getBlockState(getPos()).cycleProperty(CoalGeneratorBlock.LIT); System.out.println(getWorld().getBlockState(getPos()).getValue(CoalGeneratorBlock.LIT) + " consume"); coal.setCount(coal.getCount()-1); markDirty(); } } else { lastConsume -= 1; power += 20; } } I added markDirty() after it did not work normally. Current markDirty method: @Override public void markDirty() { System.out.println("Dirty!"); world.markChunkDirty(pos, this); world.updateComparatorOutputLevel(pos, this.getBlockType()); System.out.println(getWorld().getBlockState(getPos()).getValue(CoalGeneratorBlock.LIT) + " dirty"); } The code outprints "false consume" twice whenever lastConsume == -1, when it should print "false consume" then "true consume". Next it prints "Dirty!", then prints "false dirty".
-
The block's registry name was fine, I accidentally tried to set the model in blockstates to be /blocks/coal_generator and forgot the modid, same with my textures. Errors are fixed now!
-
Did not notice that somehow, thought it was a different mistake cause first time using IProperty, fixing it now. Thanks! Still getting errors, updated post.
-
So I am trying to make a block with two properties, EnumDirection and a Boolean for activated or not. When I start forge I get these errors: Current code (I think I implemented BooleanProperty and EnumDirection decently correctly, but maybe not.) Block: public class CoalGeneratorBlock extends Block { private static final PropertyDirection FACING = BlockHorizontal.FACING; public static final PropertyBool LIT = PropertyBool.create("lit"); public CoalGeneratorBlock() { super(Material.ROCK, MapColor.GRAY); setDefaultState(this.blockState.getBaseState().withProperty(FACING, EnumFacing.NORTH)); setRegistryName("coal_generator"); setUnlocalizedName("coal_generator"); setCreativeTab(AutoMechanica.TAB); } @Override public boolean hasTileEntity(IBlockState state) { return true; } @Override public TileEntity createTileEntity(World world, IBlockState state) { return new CoalGeneratorTE(world); } @Override public boolean onBlockActivated(World worldIn, BlockPos pos, IBlockState state, EntityPlayer playerIn, EnumHand hand, EnumFacing facing, float hitX, float hitY, float hitZ) { ItemStack inhand = playerIn.getHeldItemMainhand(); if(inhand.getItem() == Items.COAL) { ItemStack coal = ((CoalGeneratorTE) Objects.requireNonNull(worldIn.getTileEntity(pos))).coal; coal.setCount(coal.getCount()+1); inhand.setCount(inhand.getCount()); } return false; } @Override public IBlockState getStateForPlacement(World world, BlockPos pos, EnumFacing facing, float hitX, float hitY, float hitZ, int meta, EntityLivingBase placer, EnumHand hand) { return this.getDefaultState().withProperty(FACING, placer.getHorizontalFacing().getOpposite()); } @Override public BlockStateContainer createBlockState() { return new BlockStateContainer(this, LIT, FACING); } @Override public int getMetaFromState(IBlockState state) { int facingbits = state.getValue(FACING).getHorizontalIndex(); int litbits = state.getValue(LIT) ? 0 : 1 << 2; return facingbits | litbits; } @Override public IBlockState getStateFromMeta(int meta) { return getDefaultState().withProperty(LIT, meta >> 2 == 1).withProperty(FACING, EnumFacing.getHorizontal(meta)); } @Override public IBlockState getStateForPlacement(World worldIn, BlockPos pos, EnumFacing blockFaceClickedOn, float hitX, float hitY, float hitZ, int meta, EntityLivingBase placer) { EnumFacing enumfacing = (placer == null) ? EnumFacing.NORTH : EnumFacing.fromAngle(placer.rotationYaw); return this.getDefaultState().withProperty(FACING, enumfacing).withProperty(LIT, false); } } BlockState: { "variants": { "facing=north,lit=true": { "model": "coal_generator_lit" }, "facing=west,lit=true": { "model": "coal_generator_lit", "y": 90 }, "facing=south,lit=true": { "model": "coal_generator_lit", "y": 180 }, "facing=east,lit=true": { "model": "coal_generator_lit", "y": 270 }, "facing=north,lit=false": { "model": "coal_generator" }, "facing=west,lit=false": { "model": "coal_generator", "y": 90 }, "facing=south,lit=false": { "model": "coal_generator", "y": 180 }, "facing=east,lit=false": { "model": "coal_generator", "y": 270 } } } coal_generator_lit: { "parent": "block/cube_all", "textures": { "all": "blocks/coal_generator_lit" } } coal_generator: { "parent": "block/cube_all", "textures": { "all": "blocks/coal_generator" } } The block has a ton of errors when I load it and doesn't show up in game. No other errors.
-
That is what I am doing, most the code is from the ItemRenderer class. The rest is from the renderItem() method.
-
That was an accident, I fixed it. Still no change.
-
Oops, forgot to update my code. The code: public void draw(Minecraft mc) { InventoryPlayer inventory = mc.thePlayer.inventory; for (ItemStack item : inventory.mainInventory) { if(item == null) continue; if (item.getItem().getUnlocalizedName().contains("potion")) { IBakedModel model = mc.getRenderItem().getItemModelMesher().getItemModel(item); mc.getTextureManager().bindTexture(TextureMap.locationBlocksTexture); mc.getTextureManager().getTexture(TextureMap.locationBlocksTexture).setBlurMipmap(false, false); boolean flag = model.isGui3d(); if (!flag) { GlStateManager.scale(2.0F, 2.0F, 2.0F); } GlStateManager.color(1.0F, 1.0F, 1.0F, 1.0F); GlStateManager.enableRescaleNormal(); GlStateManager.alphaFunc(516, 0.1F); GlStateManager.enableBlend(); GlStateManager.tryBlendFuncSeparate(770, 771, 1, 0); GlStateManager.pushMatrix(); model = net.minecraftforge.client.ForgeHooksClient.handleCameraTransforms(model, ItemCameraTransforms.TransformType.GUI); mc.getRenderItem().renderItem(item, model); GlStateManager.cullFace(1029); GlStateManager.disableRescaleNormal(); GlStateManager.disableBlend(); mc.getTextureManager().bindTexture(TextureMap.locationBlocksTexture); mc.getTextureManager().getTexture(TextureMap.locationBlocksTexture).restoreLastBlurMipmap(); GlStateManager.popMatrix(); drawCenteredString(mc.fontRendererObj, "test", (int) (mc.displayWidth*position.x), (int) (mc.displayHeight*position.y), Integer.parseInt("FFAA00", 16)); } } } Currently, nothing is drawn to the screen except the word "Test" with no color. No errors are in console and for some reason the Player inventory is 2x bigger (The armor bar and a bit of the paler are the only things on screen). Another attempt: This code replaces mc.getRenderItem().renderItem(item, model): Tessellator tessellator = Tessellator.getInstance(); WorldRenderer worldrenderer = tessellator.getWorldRenderer(); worldrenderer.begin(7, DefaultVertexFormats.ITEM); for (EnumFacing enumfacing : EnumFacing.values()) { this.renderQuads(worldrenderer, model.getFaceQuads(enumfacing), -1, item); } this.renderQuads(worldrenderer, model.getGeneralQuads(), -1, item); tessellator.draw(); Test is drawn in orange, but no item is drawn. Player inventory is normal scale again. (Also, no GlErrors from GL11.glGetErrors())
-
Gradlew said there are no deamons running. Refresh dependencies again gives me this error: Restarting, got the original error again. Refreshed dependencies, got this error:
-
Using Intellij: When I import build.gradle in Forge, I check the boxes for auto-import and use custom gradle 'wrapper' configuration, I get this error on the project loading: Java Compiler: java.lang.IllegalStateException: ProjectScopeServices has been closed. When I check my dependencies I only see: Could not find: net.minecraftforge:forge:1.13.2-25.0.92_mapped_snapshot_20180921-1.13. I tried running gradlew refresh-dependencies and restarting the project but my project doesn't find the forge library.
-
Okay, I have updated that, but why does OpenGL not find a matrix, event though I pushed a matrix?
-
Where is the Item instance for a potion?
-
Ok I figured out how to get the item model: IBakedModel model = mc.getRenderItem().getItemModelMesher().getItemModel(item); and I render it like this: IBakedModel model = mc.getRenderItem().getItemModelMesher().getItemModel(item); mc.getTextureManager().bindTexture(TextureMap.locationBlocksTexture); mc.getTextureManager().getTexture(TextureMap.locationBlocksTexture).setBlurMipmap(false, false); boolean flag = model.isGui3d(); if (!flag) { GlStateManager.scale(2.0F, 2.0F, 2.0F); } GlStateManager.color(1.0F, 1.0F, 1.0F, 1.0F); GlStateManager.enableRescaleNormal(); GlStateManager.alphaFunc(516, 0.1F); GlStateManager.enableBlend(); GlStateManager.tryBlendFuncSeparate(770, 771, 1, 0); GlStateManager.pushMatrix(); // TODO: check if negative scale is a thing model = net.minecraftforge.client.ForgeHooksClient.handleCameraTransforms(model, ItemCameraTransforms.TransformType.GUI); mc.getRenderItem().renderItem(item, model); GlStateManager.cullFace(1029); GlStateManager.popMatrix(); GlStateManager.disableRescaleNormal(); GlStateManager.disableBlend(); mc.getTextureManager().bindTexture(TextureMap.locationBlocksTexture); mc.getTextureManager().getTexture(TextureMap.locationBlocksTexture).restoreLastBlurMipmap(); GlStateManager.popMatrix(); I am checking if it is a potion by getting the unlocalized name and checking if it contains potion. Thanks for pointing out the ItemRenderer class.
-
I am trying to get the texture of a Potion (with the colored liquid) from the ItemStack. I cannot find where the texture for the ItemStack is located, if it is in the ItemStack object I cannot find a method to find it. Also I do need to check if the item is a potion, and the Potion class does not extend Item, I can't find the Item class for potions. The Potion item seems to load a potion texture, and color it in with a color specified in the Potion object. So my question is: Where is the texture for an ItemStack located/what method can I call to get it (Do I need reflection)? How do I check if an ItemStack is a potion? Since this is being drawn to a GUI if there is a method to bind the ItemStack's texture that would also be a great solution.
-
Last time I did this gradle threw an error, I think because the folder was in use. Also how do I refresh the gradle project, I got a new hard drive and now all the dependencies are missing from my mods.
-
Forge just doesn't see my mod at all. How I set it up: copy the files: gradlew.bat, gradlew, gradle, src, build.gradle into my mod folder Run gradlew setupDecompWorkspace Open the build.gradle as a project (I have a plugin to open it) Close Intellij Run gradlew genIntellijRuns Open Intellij Set the classpath to .main on both configurations My main class: @Mod(modid = "automechanica", name = "Automechanica", version = "1.0.0") public class Main { @Mod.EventHandler public void preInit(FMLPreInitializationEvent event) { } @Mod.EventHandler public void init(FMLInitializationEvent event) { } @Mod.EventHandler public void postInit(FMLPostInitializationEvent event) { } } mcmod.info: [ { "modid": "automechanica", "name": "AutoMechanica", "description": "Automate your Minecraft world to your needs!", "version": "${version}", "mcversion": "${mcversion}", "url": "", "updateUrl": "", "authorList": ["Big_Bad_E"], "credits": "Forge", "logoFile": "", "screenshots": [], "dependencies": [] } ] pack.mcmeta: { "pack": { "description": "Automechanica resources", "pack_format": 3; } } When I press run and let Minecraft run, I see the following mods: Minecraft Minecraft Coder Pack Forge Mod Loader Minecraft Forge I have made mods and they have loaded, but for some reason this mod is not loading. Intellij version: Intellij IDEA 2018.3.3 (Community Version) Console output if it is needed (I don't see anything out of the ordinary): Thanks in advance for any help, I appreciate it. Update: Now I get this error when I open my project: "4:54 PM Unsupported Modules Detected: Compilation is not supported for following modules: AutoMechanica. Unfortunately you can't have non-Gradle Java modules and Android-Gradle modules in one project."