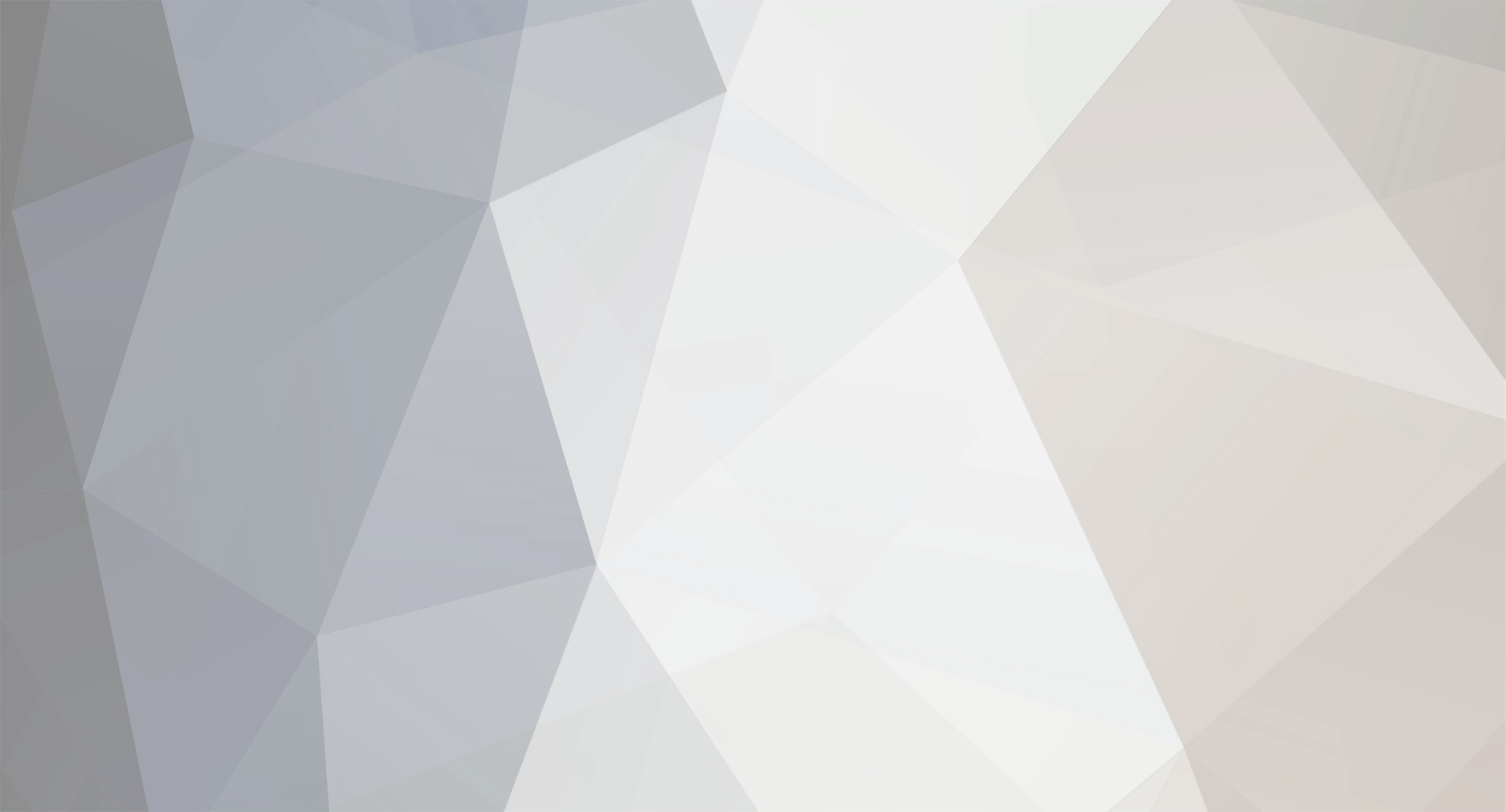
Toma™
Members-
Posts
80 -
Joined
-
Last visited
-
Days Won
1
Toma™ last won the day on November 13 2018
Toma™ had the most liked content!
Recent Profile Visitors
The recent visitors block is disabled and is not being shown to other users.
Toma™'s Achievements

Stone Miner (3/8)
8
Reputation
-
Not exactly that, I most likely worded that poorly. I have already implemented different codec mappings using the #dispatch functions, this question is more about codec creation for one value. I'll try to explain my current code in greater detail. I currently have one registry of objects which are called RewardTransformerType and it's class looks like this public final class RewardTransformerType<V, T extends RewardTransformer<V>> implements IdentifierHolder, Predicate<Class<?>> { public static final Codec<RewardTransformer<?>> CODEC = QuestingRegistries.REWARD_TRANSFORMERS.dispatch("type", RewardTransformer::getType, type -> type.codec); private final ResourceLocation identifier; private final Codec<T> codec; private final Class<V> type; public RewardTransformerType(ResourceLocation identifier, Codec<T> codec, Class<V> type) { this.identifier = identifier; this.codec = codec; this.type = type; } @Override public ResourceLocation getIdentifier() { return identifier; } @Override public boolean test(Class<?> aClass) { return aClass.equals(this.type); } } And one type implementation which looks like this public class CountByAttributeTransformer implements RewardTransformer<Integer> { public static final Codec<CountByAttributeTransformer> CODEC = RecordCodecBuilder.create(instance -> instance.group( OutputModifier.CODEC.fieldOf("modifier").forGetter(t -> t.modifier) ).apply(instance, CountByAttributeTransformer::new)); private final OutputModifier modifier; public CountByAttributeTransformer(OutputModifier modifier) { this.modifier = modifier; } @Override public Integer adjust(Integer originalValue, Player player, Quest quest) { return PlayerData.get(player).map(data -> { IAttributeProvider provider = data.getAttributes(); return (int) Math.round(this.modifier.getModifiedValue(provider, originalValue)); }).orElse(originalValue); } @Override public RewardTransformerType<?, ?> getType() { return QuestRegistry.COUNT_BY_ATTRIBUTE_TRANSFORMER; } } As you can see, currently the Codec for CountByAttributeTransformer class uses codec from OutputModifier class, which looks like this public static final Codec<OutputModifier> CODEC = RecordCodecBuilder.create(instance -> instance.group( ResourceLocation.CODEC.flatXmap(location -> { IAttributeId id = Attribs.find(location); return id == null ? DataResult.error("Unknown attribute " + location) : DataResult.success(id); }, attributeId -> attributeId == null ? DataResult.error("Attribute is null") : DataResult.success(attributeId.getId())) .fieldOf("attribute").forGetter(t -> t.attributeId), ResourceLocation.CODEC.flatXmap(location -> { IModifierOp op = AttributeOps.find(location); return op == null ? DataResult.error("Unknown operation " + location) : DataResult.success(op); }, operation -> operation == null ? DataResult.error("Operation is null") : DataResult.success(operation.getId())) .fieldOf("operation").forGetter(t -> t.operator) ).apply(instance, OutputModifier::new)); My problem with this is that when I want to serialize/deserialize it, result looks like this { "type": "namespace:id", "modifier": { "attribute": "namespace:id", "operation": "namespace:id" } } and I would like to get this result instead { "type": "namespace:id", "attribute": "namespace:id", "operation": "namespace:id" } So my question is how to adjust the codec in CountByAttribute class so I can get the wanted result (if it is even possible). I have also tried using this codec, but that was resulting in "Not an JSON object" error, so thats wrong approach too public static final Codec<CountByAttributeTransformer> CODEC = OutputModifier.CODEC.xmap(CountByAttributeTransformer::new, t -> t.modifier); Is there a way to get to the second result (without creating duplicate codec for OutputModifier class)?
-
Hello, I recently started implementation of Codec based serialization for my data objects and ran into some minor issues. I have some external utility class holding few parameters which is used by one data object (single parameter). The data object codecs are loaded via registry lookup and this extra parameter is mapped by extra key, which I would like to remove (even though I think that won't be possible as it could possibly cause issues when multiple objects are inlined, but in my case it's just one which would work). I have checked several documentations about codecs, but I have not found anything useful (is there any documentation by Mojang? I found only the DFU repository, which is for version 1.x, while MC is already on 5.x). I'll share code snippets which should explain the situation better than I do.. So I have some common class like this for example public class MyObj { public static final Codec<MyObj> CODEC = RecordCodecBuilder.create(i -> i.group( Codec.INT.fieldOf("i").forGetter(MyObj::i), Codec.INT.fieldOf("j").forGetter(MyObj::j) ).apply(i, MyObj::new)); } which I want to be loaded from format like this { "type": "namespace:id", "i": 1, "j": 2 } The actual codec inside the following class is mapped via Codec#dispatch method. My current implementation has it like this: public class DataObj { public static final Codec<DataObj> CODEC = MyObj.CODEC.fieldOf("key").xmap(DataObj::new, t -> t.myObj).codec(); private final MyObj myObj; private DataObj(MyObj myObj) { this.myObj = myObj; } } which expects to be in another Map object like this { "type": "namespace:id", "key": { "i": 1, "j": 2 } } Is there any way to inline it without the key parameter?
-
wait neverming, turns out the fps drop issue is caused by shader loading every render tick because I messed up some code...
-
Hello guys, what would be the best way to apply blur effect on player's screen? So far I have tried loading the vanilla blur shader in RenderTickEvent, but the fps went down like crazy, so I'm looking for better alternatives. Am I even supposed to load shaders in RenderTick event?
-
[1.12.2] Render player's view as zoomed in image on screen
Toma™ replied to Toma™'s topic in Modder Support
Looks like framebuffer texture is not the way to go. I have found method in EntityRenderer called renderWorld. Looks like that could be what I'm looking for, but from quick observation I can see it sets the OpenGL viewport to minecraft display width and height, so I need to find a way, how to compress it into smaller rectangle. Anyone knows? -
Hello everyone, I'm trying to render player's view (basically everything on screen) into screen overlay (using RenderGameOverlayEvent). But I cannot get my head around it. I have tried using FrameBuffer texture, but it didn't work out really well. As you can see here. Does anyone have any ideas how I would be able to achieve that? Current code, don't think it's going to actually help @SubscribeEvent public static void renderOverlay(RenderGameOverlayEvent.Pre event) { if (event.getType() == RenderGameOverlayEvent.ElementType.ALL) { Minecraft mc = Minecraft.getMinecraft(); Tessellator tessellator = Tessellator.getInstance(); GlStateManager.bindTexture(mc.getFramebuffer().framebufferTexture); BufferBuilder builder = tessellator.getBuffer(); builder.begin(7, DefaultVertexFormats.POSITION_TEX); builder.pos(x, y + h, 0).tex(0, 0).endVertex(); builder.pos(x + w, y + h, 0).tex(1, 0).endVertex(); builder.pos(x + w, y, 0).tex(1, 1).endVertex(); builder.pos(x, y, 0).tex(0, 1).endVertex(); tessellator.draw(); } } edit: I know I have no zoom in code here, for now I'm mostly interested in getting it to work normally
-
Hi there, I'm trying to change player hitbox. I just don't know where should I do it. I have tried to modify the bounding box inside the LivingUpdateEvent, however that works only until the original player hitbox collides with anything (and I have changed the hitbox on both sides). I see that there's method in EntityPlayer called updateSize which always resets the hitbox to the default one. Any ideas? EDIT: SOLVED Managed to solve it by subscribing to PlayerTickEvent and changing it there on both tick phases using the Entity#setBoundingBox method
-
There's TileEntitySpecialRenderer#isGlobalRenderer method. Minecraft uses that for rendering beacons, maybe it could help you with your issue with the rendering outside frustrum
-
Also to answer your next question - Depends on the side where code is executed, if it's server side it's going to take data from config stored on server. However someone has to confirm that, because I'm not sure and that's how I believe it works
-
Make sure to actually register the event handler. Either you can annotate the class with @EventBusSubscriber and let it register itself automatically (but your event method has to be declared as static) or register to to MinecraftForge.EVENT_BUS
-
If you want to use only overlay, and not actual GUI then you can use the RenderGameOverlayEvent, either Pre or Post. And be careful about it, because it fires for multiple elements. And for the toggling, what about toggling one boolean? While loop is pretty bad idea
-
[SOLVED][1.12.2] Issues with rendering color shape in world
Toma™ replied to Toma™'s topic in Modder Support
Nevermind, I just forgot to to call disableTexture2D function. That solved it. -
I'm trying to render shape in world similar to mc's world border, but I'm getting some weird issues with colors. When I rotate in specific angle, the color turns black and otherwise it's just plain blue. I cannot figure this out at all, and I have no idea what I'm doing wrong. Anyway, I'd be very happy if someone offered help. So there's the render code: And some images for reference:
-
There's also LivingKnockbackEvent
-
Create new class which extends WorldType and call it's constructor during one event (preInitialization/initialization/..) - That will register it. Then you can override some methods like getChunkGenerator if you want to change the world gen
- 1 reply
-
- 2
-