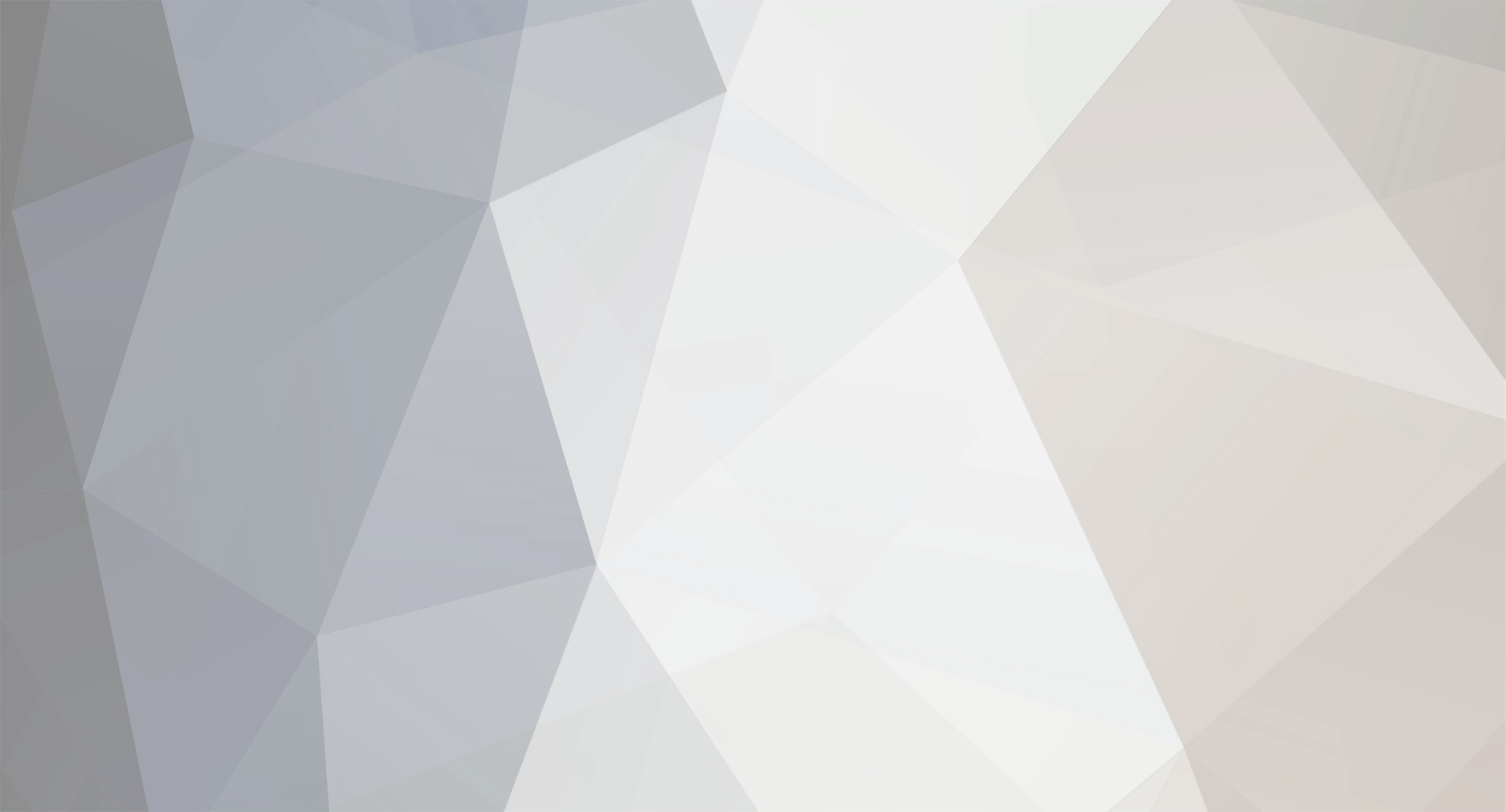
unassigned
Members-
Posts
103 -
Joined
-
Last visited
-
Days Won
1
Everything posted by unassigned
-
You probably are going to want a TileEntity (that extends ITickable - to allow your tile to update every tick) for this block. To check for air below a block, you are going to want to use World#getBlockState and check the position below the block (invoke BlockPos#down to offset the tile's pos down one). Something like: @Override public void update() { if(!this.world.isRemote) { BlockPos downPos = this.getPos().down(); if(world.getBlockState(downPos) == Blocks.AIR.getDefaultState()) { //set a bool to output redstone. } } } Then, in your block class, when you go to set your weak power, you grab the TE from World#getTileEntity and check whether it should be outputting power or not.
-
[1.12.2] Drawing Cube Through TileEntitySpecialRenderer
unassigned replied to unassigned's topic in Modder Support
Okay, thanks for this valuable information. I can now properly draw a square, and I assume I must draw six of them to draw a cube? (Implying that there is no other 'shortcut'). If so, I think I have it down, but I'm getting some weird culling issues and I do not know how I would fix. I tried uploading a gif to demonstrate it, but I guess they're not supported, so here are 2 images of this issue: Here is what it should look like: And here is what it looks like from a small distance: Here is the code I have, I draw each square separately, which creates for some messy code, but I wanted to visualize each squares vertices. Tessellator tessy = Tessellator.getInstance(); BufferBuilder buf = tessy.getBuffer(); GlStateManager.pushMatrix(); GlStateManager.disableCull(); GlStateManager.disableLighting(); GlStateManager.alphaFunc(GL11.GL_ALWAYS, 0); GlStateManager.translate(x+.5f, y+.5f, z+.5f); buf.begin(GL11.GL_QUADS, DefaultVertexFormats.POSITION); //south side [pos z] [parent x] buf.pos(x-0.5f, y+0.5f, z+0.5f).endVertex(); buf.pos(x-0.5f, y-0.5f, z+0.5f).endVertex(); buf.pos(x+0.5f, y-0.5f, z+0.5f).endVertex(); buf.pos(x+0.5f, y+0.5f, z+0.5f).endVertex(); //north side [neg z] [parent x] buf.pos(x-0.5f, y+0.5f, z-0.5f).endVertex(); buf.pos(x-0.5f, y-0.5f, z-0.5f).endVertex(); buf.pos(x+0.5f, y-0.5f, z-0.5f).endVertex(); buf.pos(x+0.5f, y+0.5f, z-0.5f).endVertex(); //east side [pos x] [parent z] buf.pos(x+0.5f, y+0.5f, z-0.5f).endVertex(); buf.pos(x+0.5f, y-0.5f, z-0.5f).endVertex(); buf.pos(x+0.5f, y-0.5f, z+0.5f).endVertex(); buf.pos(x+0.5f, y+0.5f, z+0.5f).endVertex(); //west side [neg x] [parent z] buf.pos(x-0.5f, y-0.5f, z+0.5f).endVertex(); buf.pos(x-0.5f, y-0.5f, z-0.5f).endVertex(); buf.pos(x-0.5f, y+0.5f, z-0.5f).endVertex(); buf.pos(x-0.5f, y+0.5f, z+0.5f).endVertex(); //top [pos y] [parent x & y] buf.pos(x+0.5f, y+0.5f, z-0.5f).endVertex(); buf.pos(x+0.5f, y+0.5f, z+0.5f).endVertex(); buf.pos(x-0.5f, y+0.5f, z+0.5f).endVertex(); buf.pos(x-0.5f, y+0.5f, z-0.5f).endVertex(); //bottom [neg y] [parent x & y] buf.pos(x+0.5f, y-0.5f, z-0.5f).endVertex(); buf.pos(x+0.5f, y-0.5f, z+0.5f).endVertex(); buf.pos(x-0.5f, y-0.5f, z+0.5f).endVertex(); buf.pos(x-0.5f, y-0.5f, z-0.5f).endVertex(); tessy.draw(); GlStateManager.enableLighting(); GlStateManager.enableCull(); GlStateManager.disableAlpha(); GlStateManager.popMatrix(); Thanks! - edit - I cannot get rid of this video player thing. I guess this happened when I tried to upload the gif. cullingerror.mp4 -
Hello, I have a TESR and I'd like to draw a cube (eventual a sphere, thought I'd start easy) at the location of the TE. I have very limited knowledge of opengl and how minecraft handles it through GlStateManager. I know how to do very basic things, but when it comes down to drawing vertices, I am lost. Here is what I have so far, as well as what I think should go where: Tessellator tessy = Tessellator.getInstance(); BufferBuilder buf = tessy.getBuffer(); GlStateManager.pushMatrix(); //translate matrix to te pos //set color / rot //begin drawing //set vertices to bufferbuilder //draw tessellator GlStateManager.popMatrix(); Any pointers would be nice. Thanks.
-
[1.12.2] Custom Furnace doesn't work [Solved]
unassigned replied to sunsigne's topic in Modder Support
You say there is a crash, not a logical error. You should really step through your code and see what is/not running under certain conditions. See what happens when you put in fuel and an item to smelt, see what variables are changing. Also, do you have a github for this project? I'd take a closer look at it if I could run it through my ide and debug it myself. Yes. Thanks for catching that. -
[1.12.2] Custom Furnace doesn't work [Solved]
unassigned replied to sunsigne's topic in Modder Support
Please use the coding text format when posting code like this, its a pain to read otherwise. extends BlockContainer I do not think you should be extended BlockContainer, just reimplement hasTileEntity and createNewTileEntity. The reasoning is that BlockContainer contains some useless vanilla code. Probably won't fix your problem. ---- Edit ---- isn't this intended behavior? You shouldn't be able to put things in the output slot, and the fuel slot should be restricted to fuels? Please post the crash if you'd like more help other than the thing I said and please reformat your post. I really can't be bothered trying to read plaintext code and digging for a possibly small issue. -
Make your modid more unique, 'bo' is not a good modid! I believe when you are setting RegistryNames like that; you need to include your modid. There is a function for this. this.setRegistryName(yourmodid, name); Make sure your modid is accessed from a global field - to make changing your modid less of a pain. IHasModel is bad! Never use this. Every item/block needs a model and by implementing this useless interface you are basically saying there is a case where I don't want it to have a model. Which there isn't. Many new modders make this mistake as trying to follow someone else's bad coding practices. Remove the interface and use the ModelRegistryEvent which will access your items and register them to the ModelLoader. Also, the console should be throwing an error if you are registering things correctly. Post this error if you'd like more help. If there is no error, post back with your registration code.
-
Hello, I have some rendering I'd like to do in some chunks. Basically, I have a capability attached to the world (more specifically each chunk), that can hold a certain value, once a value threshold is hit, I'd like to do some type of rendering. However, I'm having issues getting the chunks from the client. I'm currently using: Iterator<Chunk> loadedChunks = event.world.getPersistentChunkIterable(((WorldServer) event.world).getPlayerChunkMap().getChunkIterator()); which I access through WorldServer. Is there any way to get the chunks? Thanks.
-
[1.12.2] Compatibility with other mods [Closed]
unassigned replied to Legenes's topic in Modder Support
I know DeepResonace has support for both TheOneProbe and HWYLA, you can see how he implements them here. You can also reference his build.gradle to install the APIs into your environment, you can see this here, and here for the dependencies. If you'd like more functionality, both have open source APIs you can view. You can see HWYLA here, and TOP here. -
Whatever is here: at kr.guebeul.hertz.util.handler.EventHandler.playerevent(EventHandler.java:148) is null. I'm guessing, its either the player's capability or the entity itself. Figure out what it is, and check if its null.
-
We still need the crash log. Currently, we see nothing that will tell us what is wrong. Once the game crashes, copy the text (located in the console tab, that you screenshot), and paste it here. Then we can help you.
-
How to writeToNBT for more advanced objects?
unassigned replied to MrDireball's topic in Modder Support
Yeah, just remember ItemStacks should never be null. Instead, if you want an ItemStack to be empty, use ItemStack.EMPTY. What are you trying to achieve with this block? What it seems like you want to add the player's hand stack to its inventory. Instead of implementing your own inventory system, try exposing the IItemHandler capability. This will give your TE an inventory, and will most likely solve many of your problems. Not to mention, the capability will provide a lot better compat with other mods that access inventories. You shouldn't be creating an inventory from an ItemStack field. Here is an example of what I'm saying. (You should also reference the official forge documentation) First, create the handler itself (This will be a field will be in your TE): public final ItemStackHandler inv = new ItemStackHandler(1); The constructor to ItemStackHandler is how many slots the inventory should have. Now, you need to expose the capability for forge to access. @Override public boolean hasCapability(Capability<?> capability, @Nullable EnumFacing facing) { if(capability == CapabilityItemHandler.ITEM_HANDLER_CAPABILITY){ return true; //this is also where you'd check the facing, incase you only want to insert on certain sides. } return super.hasCapability(capability, facing); } @Nullable @Override public <T> T getCapability(Capability<T> capability, @Nullable EnumFacing facing) { if(capability == CapabilityItemHandler.ITEM_HANDLER_CAPABILITY){ //this will check to see if the capability being 'get' is, in fact, the ItemHandler return (T) this.inv; //this will be the inventory field that you have attached to your TE. } return super.getCapability(capability, facing); } To save the data of the inventory, use ItemStackHandler#serializeNBT and ItemStackHandler#deserializeNBT. From the block class, you need to get the capability (using TileEntity#getCapability) and use ItemStackHandler#insertItem to put the item into the TE's inventory. -
How to writeToNBT for more advanced objects?
unassigned replied to MrDireball's topic in Modder Support
You can use NBTagCompound#getCompoundTag instead of NBTTagCompound#getTag Aswell, are you sure that the itemStack field is not null? Are you assigning it anywhere? -
How to writeToNBT for more advanced objects?
unassigned replied to MrDireball's topic in Modder Support
What? Why are you creating a file? Just create a new NBTTagCompound (NBTTagCompound itemTag = new NBTTagCompound), write the data to that tag, then add that tag to your TEs compound tag (in writeToNBT) (via NBTTagCompound#setTag). Then access it when you go to read it via NBTagCompound#getCompoundTag and create a new ItemStack off this. Here is an example: @Override public NBTTagCompound writeToNBT(NBTTagCompound compound) { super.writeToNBT(compound); NBTTagCompound itemData = new NBTTagCompound(); // This will store the item data seperate from the TE's data ItemStack stack = new ItemStack(Items.DIAMOND); // You might not need to create a new item. stack.writeToNBT(itemData); //writes the Item's NBT to the 'itemData'. compound.setTag("ItemData", itemData); //writes the itemData tag to the TEs compound. This will be attached to the TE. return compound; } @Override public void readFromNBT(NBTTagCompound compound) { super.readFromNBT(compound); NBTTagCompound itemData = compound.getCompoundTag("ItemData"); //this will get the compound ItemStack stack = new ItemStack(itemData); //this will read the itemData and turn it into what it was. //From here, the stack will be loaded back into the TE and can be accessed again. } -
What isn't simply working? Are the particles not spawning?
-
How to writeToNBT for more advanced objects?
unassigned replied to MrDireball's topic in Modder Support
NBTTagCompound dataCompound = new NBTTagCompound(); ItemStack newStack = new ItemStack(Items.DIAMOND); newStack.writeToNBT(dataCompound); //writes newStack data to dataCompound if(dataCompound.hasKey("id")) { ItemStack fromCompoundStack = new ItemStack(dataCompound); } //if the dataCompound has a ItemStack data, make a new stack from that data. -
How to writeToNBT for more advanced objects?
unassigned replied to MrDireball's topic in Modder Support
If you'd like to save an item to nbt, you'd need to call ItemStack#writeToNBT, which will write a tag to the nbt under the key "id". If you'd like to store multiple items, you need to create an NBTTagList and write all items to that. -
Cutout Block Still Culling Adjacent Block Faces
unassigned replied to Niroque's topic in Modder Support
I think you need to override Block#isNormalCube. -
What do you mean broke it? Does it just not spawn, or something else? Also, listing the classes for us to look at will greatly help.
-
[1.12.2] Can't find IItemHandler isItemValid
unassigned replied to cptcorndog's topic in Modder Support
You should make a custom ItemStackHandler that extends ItemStackHandler in which you overwrite insertItem and extractItem, and check if the itemstack is valid. I have a small example of what I mean here: protected boolean canInsert(ItemStack stack, int slot) { return true; } protected boolean canExtract(ItemStack stack, int slot, int amount) { return true; } @Override public boolean isItemValid(int slot, @Nonnull ItemStack stack) { return this.canInsert(stack, slot); } @Nonnull @Override public ItemStack insertItem(int slot, @Nonnull ItemStack stack, boolean simulate) { if (this.canInsert(stack, slot)) { return super.insertItem(slot, stack, simulate); } else { return stack; } } @Nonnull @Override public ItemStack extractItem(int slot, int amount, boolean simulate) { if (this.canExtract(this.getStackInSlot(slot), slot, amount)) { return super.extractItem(slot, amount, simulate); } else { return ItemStack.EMPTY; } } But this isn't necessarily needed, as you should be able to modify isItemValid when instantiating the ItemStackHandler: public final ItemStackHandler inv = new ItemStackHandler(1) { @Override public boolean isItemValid(int slot, @Nonnull ItemStack stack) { return super.isItemValid(slot, stack); //check stack. } }; Also, make sure you are exposing the ItemHandler capability, or your inventory will not work properly. -
Maybe I do not entirely understand you, but you want to change the Tile's ID, without losing the data from another world? I don't think there is any way to do this. If you change the ID, the game doesn't recognize the TE in the world, which will make it not load it into game. Why do you care about the data attached to this block so much? I'm guessing you are in a testing environment, so changes like this will break existing TEs, but you can just put down the block with the new TE id and it should work the same.
-
We can't help you if you don't provide us with code. Saying it's a logical error doesn't tell us or show us anything.
-
Hello. I have an item that has an nbt stored of an item that was used in crafting, however, as all the items are the same, there is no actual identifier other than the tooltip (and nbt stored to the item). So I was wondering if I could overlay that item used in crafting on top of the item itself, as in if it used a diamond in crafting, it would overlay the diamond on top of the icon in the inventory. This would prevent me from making hundreds of textures for the item, as well as give way better mod compatibility. I'm just not sure how to go around doing this. Thanks.
-
[1.12.2] Getting all Ingots from vanilla / other mods.
unassigned replied to unassigned's topic in Modder Support
Yeah, I will look into doing that. Now I need to create these subtypes, however, I've stumped myself with how to do this. As this items' subtypes depends on other items being loaded, I'm stuck. I want to make it so that the item stores what type (either via nbt or capabilities), and have different crafting recipes. Could anyone give me pointers on how to do this? Thanks. -
[1.12.2] Getting all Ingots from vanilla / other mods.
unassigned replied to unassigned's topic in Modder Support
public NonNullList<ItemStack> getAcceptableCatalystTypes() { NonNullList<ItemStack> returnList = new NonNullList<>(); for(String oreName : OreDictionary.getOreNames()) { if(oreName.contains("ingot")){ for(ItemStack ingot : OreDictionary.getOres(oreName)){ returnList.add(ingot); } } } for(String stringLoc : VoidConfig.general.resourceCatalystTypes){ ResourceLocation location = new ResourceLocation(stringLoc); if(ForgeRegistries.ITEMS.containsKey(location)){ returnList.add(new ItemStack(ForgeRegistries.ITEMS.getValue(location))); }else if(ForgeRegistries.BLOCKS.containsKey(location)){ returnList.add(new ItemStack(ForgeRegistries.BLOCKS.getValue(location))); } } } Here is what I have so far. I don't really know if the config bit is entirely correct, as this was my first time accessing the ForgeRegistries values. I will be testing and seeing what happens. Thanks for the info. -
[1.12.2] Getting all Ingots from vanilla / other mods.
unassigned replied to unassigned's topic in Modder Support
Yeah, I see in the documentation that common prefixes, like ingot, can be found. However, I'm not seeing anything to get the items from these prefixes. Would I use something like OreDictionary#doesOreNameExist and check for each item I want to be a subtype? Also, I have a quick question with subtypes, is it possible to assign different names for each type, depending on a value that is stored upon the item (ex. type)?