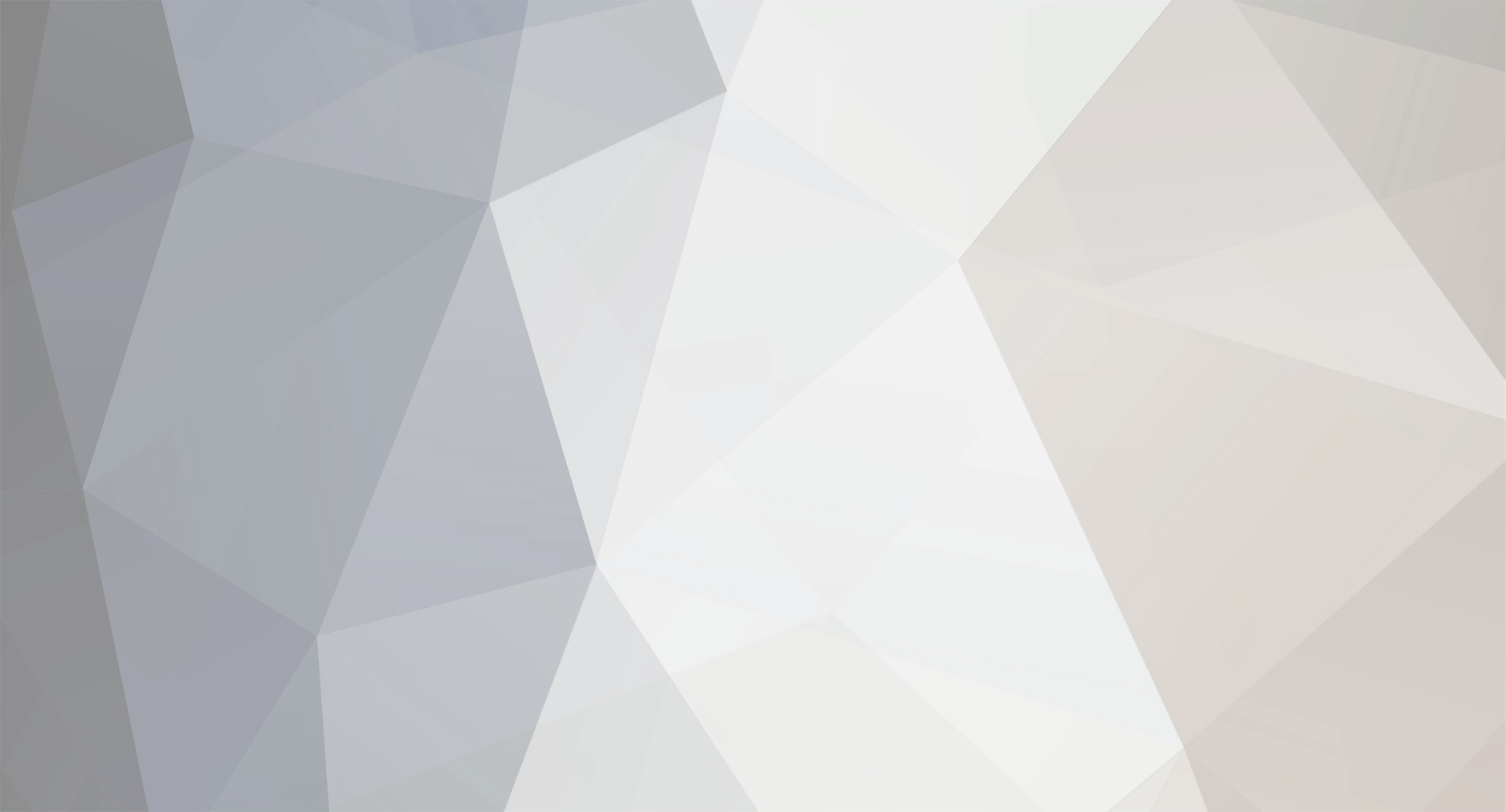
robustus
-
Posts
151 -
Joined
-
Last visited
Posts posted by robustus
-
-
It's in my last post
-
I believe it is in the WorldProvider
-
usually the errors will give you the line number of the code that is encountering the problem, can you post the code in World and ChunkProvider that is giving the issue? Also you may be able to achieve this without creating an entire new BiomeGenBase. If you have skype you can drop me a line and I will try to work through it with you, my skype name is robustushax.
-
public int getBiomeGrassColor() { return 0x9ab219; }
add this function to your biome class
-
You are going to have to copy every thing the overworld uses for multiple biomes and then customize it for yourself, that means custom chunkprovider, genlayer, biomecache etc etc everything, it is no easy feat not something someone can just passively suggest on how you accomplish it.
-
flowing liquid block needs to be one ID lower than the still block (or vice versa, I forget, search for liquid on the forum)
-
you need basic java knowledge to atleast get going on modding, its tough for people to help you out if you cannot figure out that int a cannot be 5 and 10 at the same time
-
Sorry, I still don't quite follow. Which lines am I putting in which classes? Any chance you can show me an example? (psuedocode is fine)
Im not home at the moment to go into more detail, but you can look at BiomeGenBase at all the code related to the biome decorator, copy it into your biome and change it to your biome decorator. I personally dont use a biome decorator, I put all the decorating in the biome file itself. Ill post some code later when I get time if you havent figured it out by then.
-
/** * Decorates the world. Calls code that was formerly (pre-1. in ChunkProviderGenerate.populate */ public void decorate(World par1World, Random par2Random, int par3, int par4) { if (this.currentWorld != null) { throw new RuntimeException("Already decorating!!"); } else { this.currentWorld = par1World; this.randomGenerator = par2Random; this.chunk_X = par3; this.chunk_Z = par4; this.decorate(); this.currentWorld = null; this.randomGenerator = null; } }
This is the code that you need to add in your biome. the decorate function is this.decorate. you need to call your custom decorator instead
How exactly do I call my custom decorator though? I don't see anywhere that... "asks" for it?
public YourCustomBiomeDecorator thebiomedecorator;
YourCustomBiomeDecorator thebiomedecorator = new YourCustomBiomeDecorator() thebiomedecorator.decorate();
-
public static final Block arturoBlock = new BlockArturoBlock(500, 0).setUnlocalizedName("ArturoBlock");
-
/** * Decorates the world. Calls code that was formerly (pre-1. in ChunkProviderGenerate.populate */ public void decorate(World par1World, Random par2Random, int par3, int par4) { if (this.currentWorld != null) { throw new RuntimeException("Already decorating!!"); } else { this.currentWorld = par1World; this.randomGenerator = par2Random; this.chunk_X = par3; this.chunk_Z = par4; this.decorate(); this.currentWorld = null; this.randomGenerator = null; } }
This is the code that you need to add in your biome. the decorate function is this.decorate. you need to call your custom decorator instead
-
I see, I think the problem is you are registering the biome before you register the blocks. register the blocks first.
-
What about the block ID's
this.topBlock = (byte) Remula.akatoeGrass.blockID; //This is the line referenced in the stacktrace this.fillerBlock = (byte) Remula.akatoeDirt.blockID;
these blocks need to be under 256 , if you put a block in here that is above 256 u will get a crash/error
-
1) Yes you need the custom WorldChunkProvider to put your biome in the array, and any blocks that you are going to use to spawn in terrain generation have to have a block ID under 256.
-
Add this to your still block to get the liquid to flow when placed in world.
public void onBlockAdded(World par1World, int par2, int par3, int par4) { if (par1World.getBlockId(par2, par3, par4) == this.blockID) { this.setNotStationary(par1World, par2, par3, par4); } }
-
I've been looking at this stuff also, and as someone who has really only learned java by modding minecraft, so maybe you will have more luck figuring it out, but i would take a look at GenLayerShore that seems to be a little of what you are looking to do, basically seems to specify the edges of the biome as a different biome.
-
make flowing liquid id 501, and the still block id 502 or modify/override this code in the still block. its prob just easier to change the block ID to avoid confusion.
/** * Changes the block ID to that of an updating fluid. */ private void setNotStationary(World par1World, int par2, int par3, int par4) { int l = par1World.getBlockMetadata(par2, par3, par4); par1World.setBlock(par2, par3, par4, this.blockID - 1, l, 2); par1World.scheduleBlockUpdate(par2, par3, par4, this.blockID - 1, this.tickRate(par1World)); }
as you can see by default they have the still block subtracting 1 from the ID for flowing.
-
no, just add your mod before it like mymod.BiomeName
public static ArrayList<BiomeGenBase> allowedBiomes = new ArrayList<BiomeGenBase>(Arrays.asList(forest, plains, taiga, taigaHills, forestHills, jungle. jungleHills, mymod.BiomeName)); -
Just register it like a normal block
-
did you put your biome in the array of allowed biomes? It still didn't work?
-
You need to create a flowing block and a still block, optimally the flowing block is one id below the still block, but I think you can change the code somewhere if you cant do that. then for your flowing block class use
extends BlockFluid implements ILiquid
then for the stationary block class use
extends BlockStationary implements ILiquid
-
I'd recommend getting rid of alot of those functions like get respawnmessage and renderstars etc just for testing purposes and go with the bare minimum. That or try to add @Override to as many of them as you can. I'm also pretty sure you don't need to re-add the function WorldProvider getProviderForDimension
-
yeah, it appears the GameRegistry.addBiome serves the purpose of adding the biome to array of biomes for the Overworld. Either that line with your biomes instead of the default ones, or if you look at WorldProviderHell
/** * creates a new world chunk manager for WorldProvider */ public void registerWorldChunkManager() { this.worldChunkMgr = new WorldChunkManagerHell(BiomeGenBase.hell, 1.0F, 0.0F); this.isHellWorld = true; this.hasNoSky = true; this.dimensionId = -1; }
You can do it here
-
True it doesn't, but someone had once advised me that checking neighboring blocks in the render call is a drain on resources, but what do I know
Changing the size of the grassbuffer
in Modder Support
Posted
I'm just looking to tinker with the grass colorizer between biomes and increase the blending zone, I am looking in ColorizerGrass and it looks like it is fed an array of blocks but am not sure where to look to modify the amount of blocks the blending occurs over.
Here is the default ColorizerGrass just so you can see