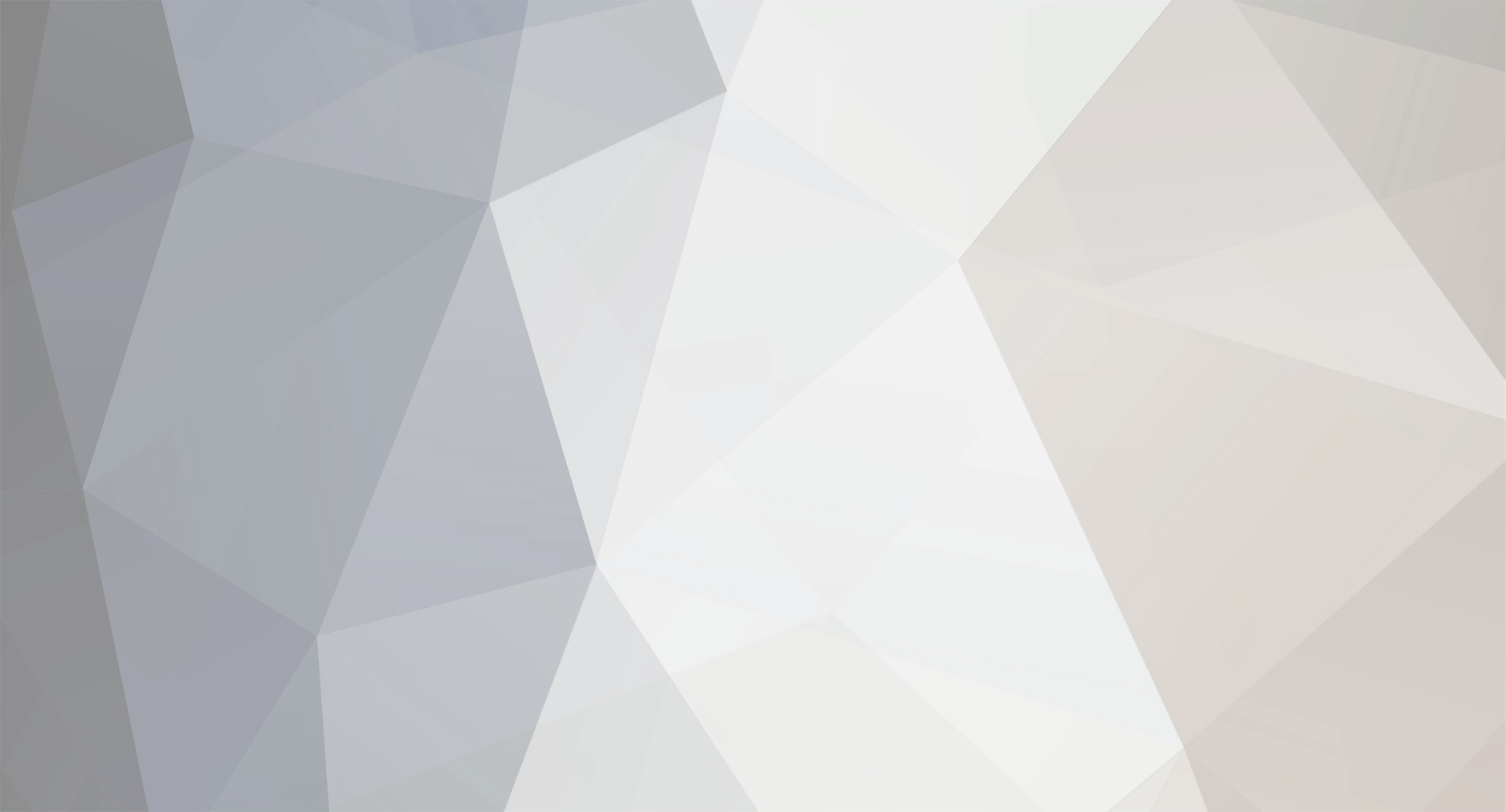
robustus
-
Posts
151 -
Joined
-
Last visited
Posts posted by robustus
-
-
DimensionManager.registerProviderType(dimension, WorldProviderShrine.class, false); DimensionManager.registerDimension(dimension, dimension);
Have you tried switching this to true instead of false? You are setting the dimension to not stay loaded so I am not 100% that is the issue but I would give it a try. Nether and Overworld are set to true.
-
The first step to do this is using metadata. I created a similar block, like a fence block, you need to check each block next to it set every scenario to a metadata, for example, I set up booleans representing north, south, east, west, up. Do a check on each spot, if there is a pipe north of the block set boolean to true, if not set it to false. Do this for all the directions and then you will have to set a metadata for each scenario. For example if(!south && !north && !east && !west) { set metdata to 0. that would mean the block has no surrounding pipes, then you would have to do each possible outcome as a metdata. If only pipe to the east, that would be one metadata, if pipe is to the east and west another seperate metata. Then in the TileEntityRender you get the blocks metadata and based on the metadata you render the proper model.
-
like SanAndreasP said, you do not want to name the class the same thing as the class you are extending.
Try
public class TileEntityA extends TileEntity { public int var = 1; }
then
public class SubTileEntity extends TileEntityA {
Think of it as a hierarchy, TileEntity -> TileEntityA -> SubTileEntity you cannot grab a variable that is declared in TileEntityA from the base Tile Entity, but you can grab it from SubTileEntity because it extends TileEntityA. Hope that makes sense, I'm still learning java myself.
-
The vanilla grass block does it, I havent understood the code yet, but it is in a giant block of code in renderStandardBlockWithAmbientOcclusion in renderblocks. search for fancygrass conditions are met when the grass block is rendered and it rendered the side grass over the dirt block to color it based on the biome.
-
I'll let you in on a little trick I have been working on. Essentially it is basically a terrain block that mimics other blocks. For example you create a stone block that can be generated under the 256 ID. You can then use conditionals based on the biome to change the texture and drop blocks, so theoretically you could make one stone block that uses a different texture based on conditions like height or biome. So you could have 100 diff biomes, use the one stone "mimic" block that will change texture based on the biome it spawns in and have the stone drop your higher ID stone block when mined. As far as I know you are not limited to the amount if conditional statements, and I havent tested out performance for something as crazy as 100 biomes, but so far on the smaller scale im working on I havent run into any problems.
-
biome decorator is not what generates the various biomes, it just decorates them.... I would assume you would need the custom chunkprovider, custom worldprovider, and then you would need to modify the GenLayer and stuff like that, clone the overworld regular field and start tweaking from there.
-
What exactly are you looking to replace? The only thing I can't figure out is how to override the WorldProvider for the Overworld, if anyone can put some input on how to do that, that would be great.
-
I've been messing with DimensionManager registerProviderType and registerDimension to name a new WorldProvider class to use for the OverWorld but have been unsuccessful, have even tried to unregisterDimension the overworld and then rename new provider and register but no dice. Anyone have any idea how to accomplish this? (I might add I am still using 1.4.7 if this method changed much since then i apologize, just have been too lazy to upgrade yet.)
-
I've been messing with DimensionManager registerProviderType and registerDimension to name a new WorldProvider class to use for the OverWorld but have been unsuccessful, have even tried to unregisterDimension the overworld and then rename new provider and register but no dice. Anyone have any idea how to accomplish this? (I might add I am still using 1.4.7 if this method changed much since then i apologize, just have been too lazy to upgrade yet.)
-
its in the chunk provider you should be able to do a search on how to do it
-
The block ID #'s need to be lower than 256 (255 and below) to work in the biome generation, that's usually the first mistake to check.
-
Hopefully this is an ok place to post this, if not I apologize. I've been working on a mod for a little bit now and have decided to look for a texture artist to team up. Im not ready to divulge what the mod involves to the public, but lets say its kind of an expansion mod. Hit me up with a private message if you're interested and Ill give more details. I'm a laid back easy going guy so the only requirement aside from being a decent texture artist is that you are not a prima donna or hard to deal with.
Thanks.
-
Your filler and top blocks for the biomes need to have block ID's less than 256 because terrain generation uses byte not integer.
-
Just guessing here but looks like you place down the Prehistoric stone in the gennerateterrain function, and also looks like you are placing it into the replaceblocksforbiome. Try replacing the replaceblocksforbiome with the normal default code, then only replacing the default stone with your stone and see if the lag disappears.
-
Right, Java starts at 0, my bad
-
The block you put in filler or top block has to be an ID below 256 or else you will crash when generating
Could you elaborate? What is your source for saying this, as to me this sounds wrong I would like to know why it wouldnt handle block ids above 256?
As previous poster stated the filler and top blocks are Byte variables not integers, and the highest number block ID is 256 that will work with the terrain gen.
-
The block you put in filler or top block has to be an ID below 256 or else you will crash when generating
-
I think you need to have the register blocks before you add the name and set the harvest level
-
Ok I had a look a little deeper for you, the creating a child entity is in Entity Animal (procreate). Basically there is an age timer that is set that counts down which you can see in EntityAgeable. Once the value goes into the negative it is no longer considered a child. There's probably a few approaches for you to add another stage, but you could probably set up your own timer with multiple stages.
Prob a quick root if you're not looking to do a lot of extra coding you can set the adult entity to be a completely new entity that's child state is your medium state or something like that.
-
I havent looked at the code yet but it seems to me that the code that changed baby wolf to grown wolf would be the code to do it, you would have baby entity coded to change into mid size entity which also is timed to change to adult entity
-
is there any kind of clever line of code that could be used to avoid having to rename and edit every single file of a mod to fix this?
-
Ive seen this code in my browsing located in forge.event.terraingen/worldtypeevent.class .. not sure if this is it but looks like it
public static class BiomeSize extends WorldTypeEvent { public final byte originalSize; public byte newSize; public BiomeSize(WorldType worldType, byte original) { super(worldType); originalSize = original; newSize = original; } }
-
Not sure how I should go about it, is there a hook to update the model from neighborchange or do i have to have a separate model for each state?
-
Ive been messing around with custom model block and just had a question. Basically the model is like a fence block where it will render differently if certain conditions are met. Im wondering the best place to put these conditions. I've gotten it to work but Im just paranoid that I put it in the wrong place, because it seems like I may have put the conditions in a place where each block checks the conditions every second, but I want it only to check when the blocks surrounding it change.
I placed the conditions in the renderModelAt function within my TileEntityRenderer and it passes those conditions to the model and renders. Obviously though the conditions will only change the model when an adjacent block is changed so I wouldn't need to do these checks when that happens, but I am having a hunch that the check is happening continuously though I could be wrong. Hope I explained that properly. If anyone could give some advice that would be great.
Dimension Generation Questions
in Modder Support
Posted
What you need to do is create a biome like you normally would in the overworld, and then use the removeBiome from Forge's GameRegistry class so that it does not spawn in the overworld. This won't remove the biome completely, just remove it from the list of biomes to spawn in the Overworld. Biomes are added to dimensions in the dimensions WorldChunkProvider:
And also some are added in GenLayer like beach, river, mushroom biome, you can see by looking at GenLayerShore i believe on how some of that works.