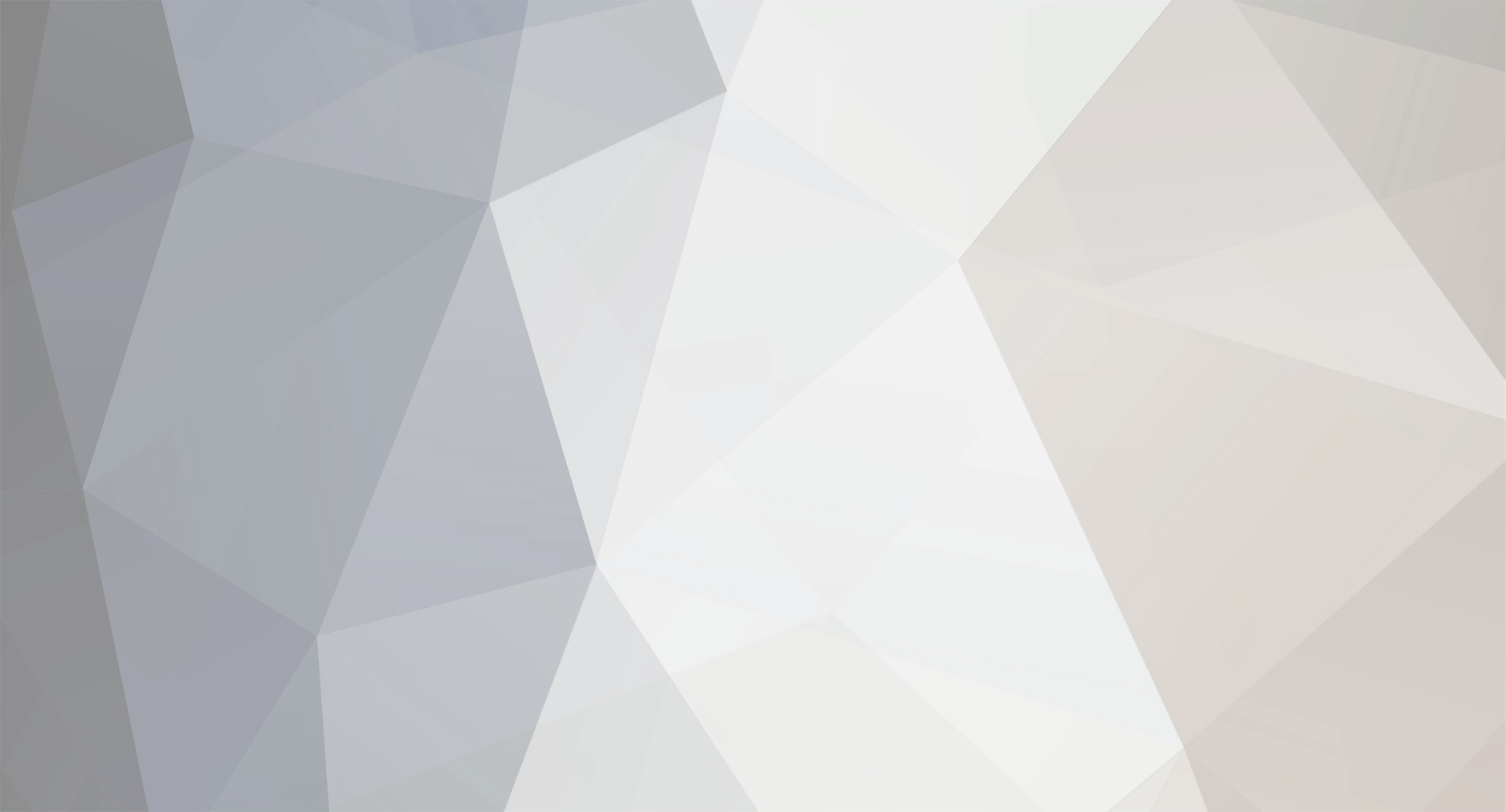
TheyCallMeDanger
Members-
Posts
73 -
Joined
-
Last visited
Everything posted by TheyCallMeDanger
-
/** * For some reason, the bounds actually have to be SMALLER (as I interpret the code) for the entity to hit it. * The parameters aren't quite what I would expect them to be. */ public AxisAlignedBB getCollisionBoundingBoxFromPool(World par1World, int par2, int par3, int par4) { float f = 0.0125f; return AxisAlignedBB.getAABBPool().getAABB((double)(((float)par2) + f), (double)par3, (double)(((float)par4) + f), (double)((float)(par2 + 1) - f), (double)((float)(par3 + 1)), (double)((float)(par4 + 1) - f)); } This works for me. I have a block called lava foam. It detects when you hit the block and bounces you back from whence you came. This routine sets the bounds of the block a little inside the actual block itself, so that mc detects when an entity actually enters the block, and thus triggers public void onEntityCollidedWithBlock(World par1World, int par2, int par3, int par4, Entity par5Entity) properly. It's strange, and you would think it would work the other way around, but this is what works... Basically, the args are minx, miny, minz, maxx, maxy, maxz, but all are set INSIDE the actual block. In this case I only care about x/z collisions, and leave y alone as the full block bounds.
-
MySparkFish = (new ItemSparkFish(BaseBlockID + OreSpawnConstants.SparkFishBlockID, 1, 0.2F, false).setUnlocalizedName("sparkfish")); public class ItemSparkFish extends ItemFood { public ItemSparkFish(int par1, int par2, float par3, boolean par4) { super(par1, par2, par3, par4); this.setAlwaysEdible(); } public void onFoodEaten(ItemStack par1ItemStack, World par2World, EntityPlayer par3EntityPlayer) { super.onFoodEaten(par1ItemStack, par2World, par3EntityPlayer); if (!par2World.isRemote) { par3EntityPlayer.addPotionEffect(new PotionEffect(Potion.fireResistance.id, 100, 0)); } } @Override @SideOnly(Side.CLIENT) public void registerIcons(IconRegister iconRegister) { this.itemIcon = iconRegister.registerIcon("OreSpawn:" + (this.getUnlocalizedName().substring(5))); } } Please note that "OreSpawn" is the name of my mod, and you should replace it with yours. The unlocalized name, "sparkfish", is actually short for the icon filename, sparkfish.png. There is a good beginner tutorial for 1.6.2 hidden here somewhere on the forge wiki. It is invaluable for a newbie. I suggest you search around until you find it!
-
Just realized that y'all had put out a new release, 804. Tried my original mod (OreSpawn on Planetminecraft) build from 789 on it, and t ran. Downloaded the sources for 804. Rebuilt it all. And it ran again! Nice. Very nice. One small problem though, was that when I tried to install the older 789 version, it seems that some files are missing on the file server. Kept getting HTTP error 403. But 804 downloaded, installed, compiled, and ran everything without a hitch. Give yourselves a pat on the back.
-
I've got a mountable, rideable, entity (actually a few) that used to work in 1.5.2 that now no longer function. I originally copied and modified EntityBoat, and it worked well. I did that again, but to no avail. It won't move. It mounts and unmounts. It even turns. It just won't go. The boat uses the entityRiddenBy.moveForward field. When I do, it's always zero. I printed it out, and on the client it is non-zero... But the control side is on the server, and there, it never gets set. I've tried every server motion variable from both the riding and mounted entities, and get nothing. I even tried setting a datawatcher in the client and reading it in the server. Unfortunately, it appears datawatchers only go one way, server to client. Anyone find a way to get mounted entities to work? Is there a 1.6.2 example out there (besides the boat) that I can look at? How do I get player motion in the server from the client? Help? Clues? Hints?
-
How to change weapons damage in Forge 1.6 without Enums
TheyCallMeDanger replied to tlr38usd's topic in Modder Support
OOOOOOoooooooooooooooooooo...................... You have no idea the can of worms you've just opened!!!! Lol! Thanks! -
My entities won't move with AI. I'm stumped...
TheyCallMeDanger replied to TheyCallMeDanger's topic in Modder Support
I found that you are now required to add more move code: /** * Called to update the entity's position/logic. */ public void onUpdate() { //RESET SPEED BECAUSE SOMEONE CLEARS IT EVERY ITERATION -- BUG!!!! this.func_110148_a(SharedMonsterAttributes.field_111263_d).func_111128_a(this.moveSpeed); //Movespeed super.onUpdate(); } Where this.moveSpeed is private float moveSpeed = 0.35f; //Whatever speed you want. The EntityAI*() routines are now RELATIVE speeds. Instead of passing in a speed like they used to, they now take a multiplier. Greater than 1 for faster (IE 1.25d), or less than one for slower (IE 0.75d). -
Yep. That worked. Odd thing is, it appears to be the ONLY way to get things to move. Somehow, all my entities reset their move speed, so: /** * Called to update the entity's position/logic. */ public void onUpdate() { this.func_110148_a(SharedMonsterAttributes.field_111263_d).func_111128_a(this.moveSpeed); //Movespeed super.onUpdate(); } Is required!
-
New Texture Directory For Minecraft Forge 1.6.1
TheyCallMeDanger replied to XxxXEclipse7XxxX's topic in Modder Support
Because they're 'unnamed', which implies that we shouldn't use them. I try to stick to functions that have been renamed into something resembling what they do. In some cases that's not possible, of course, like func_110143_aJ(), which should be getEntityHealth(). -
1.6.2 Custom leaves Decay Help (solved)!!
TheyCallMeDanger replied to fernandodx1000's topic in Modder Support
Your block/log may just need a canSustainLeaves() call (returns true!)... If that's not it, you can try my code, its much simpler! But you'll still need to add canSustainLeaves() returning true! /** * Ticks the block randomly for leaves. * This checks to see if there is wood nearby. */ public void updateTick(World par1World, int par2, int par3, int par4, Random par5Random) { int var7 = 2; //Basic leaf distance check int var12, var13, var14; int bid; int totaldist = 0; if (!par1World.isRemote) { for (var12 = -var7; var12 <= var7; ++var12) { for (var13 = -var7; var13 <= 0; ++var13) //Only check down, because leaves only grow on top of wood { for (var14 = -var7; var14 <= var7; ++var14) { totaldist = Math.abs(var12) + Math.abs(var13) + Math.abs(var14); //Make roundish corners, not square! if(totaldist <= 3){ //Furthest a leaf is allowed to be from a block of wood! bid = par1World.getBlockId(par2 + var12, par3 + var13, par4 + var14); Block block = Block.blocksList[bid]; if (block != null && block.canSustainLeaves(par1World, par2 + var12, par3 + var13, par4 + var14)) { //We are a valid leaf block. See if there is air underneath. Maybe we will drop an item! bid = par1World.getBlockId(par2, par3 - 1, par4); if(bid == 0) { if (par1World.rand.nextInt(20) == 0) { this.dropBlockAsItemWithChance(par1World, par2, par3 - 1, par4, 0, 0.0F, 0); } } return; //Found wood. Ours or not, who cares. Attached or not, who cares. Just nearby is all that counts. } } } } } this.removeLeaves(par1World, par2, par3, par4); } } private void removeLeaves(World par1World, int par2, int par3, int par4) { this.dropBlockAsItem(par1World, par2, par3, par4, 0, 0); par1World.setBlock(par2, par3, par4, 0,0,2); } -
So, I figured out on my own that entities need to make yet another initialization call. For example: protected void func_110147_ax() { super.func_110147_ax(); this.func_110148_a(SharedMonsterAttributes.field_111267_a).func_111128_a(this.getMaxHealth()); //Health this.func_110148_a(SharedMonsterAttributes.field_111263_d).func_111128_a(this.moveSpeed); //Movespeed this.func_110148_a(SharedMonsterAttributes.field_111264_e).func_111128_a(12.0D); //Attack Strength } I think I pretty much nailed what each of the fields are, with 111263_d being move speed. The EntityAI functions are getting called, and I traced waaaaay down into them to verify that yes indeed a valid path is returned and set. The problem, I found, is that when it gets back up to onLivingUpdate in EntityLivingBase the motionX and motionZ fields are 0. Hence, no movement. What am I missing to make my entities move? This all worked just fine in 1.5. It looked like all I had to do was add the func_110147_ax() routine. Yes, I figured out the rendering. They all now render and just stand there looking around. But they won't move... Help?
-
New Texture Directory For Minecraft Forge 1.6.1
TheyCallMeDanger replied to XxxXEclipse7XxxX's topic in Modder Support
Oh! Oh! I can answer this one now! Here's the hidden secret block tutorial that helped me figure it out... http://www.minecraftforge.net/wiki/Basic_Modding There appear to be a number of key elements here: 1) Everything is initialized in the preInit() function, which now has a qualifier (or whatever you call it) of @EventHandler. Look at the wiki main mod example. 2) Blocks/items are generally declared like MyFireFish = (new ItemFireFish(BaseBlockID + OreSpawnConstants.FireFishBlockID, 4, 0.6F, false).setUnlocalizedName("firefish")); The .setUnlocalizedName part is critical. It MUST be the name of your png file, without the .png, and nothing in front. 3) In your item/block class, you now need to add the following magic incantation: @Override @SideOnly(Side.CLIENT) public void registerIcons(IconRegister iconRegister) { this.itemIcon = iconRegister.registerIcon("OreSpawn:" + (this.getUnlocalizedName().substring(5))); } Except that instead of "OreSpawn" use your mod name (case doesn't matter here). Why substring(5)? I don't know. I don't care. It works. Yes, you need the ":" after your mod name too. And lastly... The directory that firefish.png ends up ....forge/mcp/src/minecraft/assets/orespawn/textures/items/firefish.png The important things to notice in the above, is that you need to create this directory tree exactly as is, from "assets" on down, except that you use your mod name (all lowercase) instead of "orespawn", which is my mod. Refer to the wiki page referenced above. It was small, simple, and easy to understand. Now, If I can just figure out why my entities won't move... sigh... Hope this helped! -
STILL won't find my textures. Arrrg!
TheyCallMeDanger replied to TheyCallMeDanger's topic in Support & Bug Reports
Oh geez. Nevermind. You've been no help at all. I found a nice little tutorial here: http://www.minecraftforge.net/wiki/Basic_Blocks That finally showed me the problem. Instead of making things EASIER for modders, you've gone and made it HARDER. setUnlocalizedName() used to do everything you needed. Now you need to add registerIcons() to every single block, AND still use setUnlocalizedName(). Would it be too much to ask for you to make life SIMPLER and EASIER on modders, rather than spitting all over us? We are, after all, the reason you exist. Quit jacking us around and changing the interfaces and requirements every release. You are SUPPOSED to provide a simple, clean, consistent, easy to use, interface. You do not. Fail. I've seen a lot of code in my 30+ years. Forge has not been a pleasant experience. -
Mob does not render, Texture not loading now [1.6.1]
TheyCallMeDanger replied to Beelzaboss's topic in Modder Support
Thank you for a nice clear answer. I'll be trying this too. -
STILL won't find my textures. Arrrg!
TheyCallMeDanger replied to TheyCallMeDanger's topic in Support & Bug Reports
Does it make any difference if the top of my source tree is at mcp/src/minecraft/danger/orespawn vs mcp/src/minecraft/orespawn? In other words, one level down? -
STILL won't find my textures. Arrrg!
TheyCallMeDanger replied to TheyCallMeDanger's topic in Support & Bug Reports
Doesn't work. I've tried ...forge/mcp/src/minecraft/assets/orespawn/textures/blocks/{myblocks}.png Looking at the files there right now. I register my block icons thusly, in the preInit() function: MyBlockUraniumBlock = (new BlockUranium(BaseBlockID + OreSpawnConstants.BlockUraniumBlockID).setUnlocalizedName("blockuranium")); This is supposed to automatically register the block. Yes, I know, blocks with multiple textures use the registerIcon() function. I've placed files all over god-knows-where in the directory tree, and it still refuses to find them. I'm looking at forge/mcp/src/minecraft/assets/orespawn/textures/blocks/blockuranium.png right now. I see it. Forge doesn't. "OreSpawn" is the name of my mod, so I assume that's what you mean by "domain". It ain't working. -
mcp8.03, forge 775. No matter where I put my textures, it won't find them. The error messages are of no help either. What's wrong with this thing? For example, an error message of: ...Using missing texture, unable to load: minecraft:textures/blocks/antnest_side.png But I go to .../forge/mcp/src/minecraft/textures/blocks, and there it is! antnest_side.png I've tried placing these files in every directory imaginable. In Minecraft:/textures Minecraft:/assets/textures Minecraft:/orespawn/assets/textures Minecraft:/danger/orespawn/textures Minecraft:/danger/orespawn/assets/textures and on and on. WHERE DO THEY GO? The name of my mod is "OreSpawn". I have yet to read a definitive post that actually works. Anyone?
-
1.6.1 Blocks not loading textures.
TheyCallMeDanger replied to KeeganDeathman's topic in Modder Support
Blocks and textures are broken. Waiting for forge to fix them... -
New Texture Directory For Minecraft Forge 1.6.1
TheyCallMeDanger replied to XxxXEclipse7XxxX's topic in Modder Support
Unfortunately, your github is not set up in a way that those of us NOT using a Pahimar setup can actually use it. I agree. It's all broken, and has to go back to the way it was in 1.5.2, where the "textures" directory can sit right next to the top source directory along with paulscodes and ibxm and friends. There is no reason for forge to be moving things around on us. They are supposed to be SHIELDING us from the underlying changes, not exacerbating them. Hello? Forge? Please put textures, sounds and such back the way it was. It was working. Stop messing with it. -
Could someone actually explain this, please. For example, it can't find my critter textures with the error message: "minecraft:/danger/orespawn/butterfly3.png" But when I go there. There it is! What the heck? If my mod name is MYMOD. And the top level source directory (cpw. ibxm, net, paulscode) is: /workspace/forge/mcp/src/minecraft. I know my sources go in /workspace/forge/mcp/src/minecraft/MYMOD. My critter textures used to be happy there too. Now they are not. Where do my critter textures go??? (full path please) My "textures" (block, items) directory used to be happy there too. Now it is not. Where does it go??? (full path please!) What about sounds??? Did they move too? (full path please!) And where does armor go now??? (again, full path please!) PLEASE give me/us/everyone full paths for this example.
-
System.out.printf stopped working....?!?
TheyCallMeDanger replied to TheyCallMeDanger's topic in Modder Support
Ah yes... They did. They redirected stdout and stderr. Awfully nice of them. Comment out lines 190 and 191 in FMLRelaunchLog.java: //System.setOut(new PrintStream(new LoggingOutStream(stdOut), true)); //System.setErr(new PrintStream(new LoggingOutStream(stdErr), true)); System.out.printf() works fine again. Yes, I know this is not what they want me to do, but since I can't find anything on how to set up logging properly as they intended... It even appears that they tried to automatically initialize logging into logfiles under each mod name. Doesn't appear to work though, at least not for me. Dear Forge: Could ya'll put the default outputs back so that mods with uninitialized logging can still get the standard System.out.printf()? That would be sweet... Thanks! -
System.out.printf stopped working....?!?
TheyCallMeDanger replied to TheyCallMeDanger's topic in Modder Support
I love how your signature says "Odds are good I've been programming since before you were born. Yeah. I'm OLD school." yet you think System.out.printf() not working is somehow forge's problem? Just tested the following code in my mod System.out.printf( "%-15s %10s %n", "Exam_Name", "Exam_Grade"); System.out.printf( "%-15s %10s %n", "English", "A"); System.out.printf( "%-15s %10s %n", "French", "F"); System.out.printf( "%-15s %10s %n", "Science", "C"); System.out.printf( "%-15s %10s %n", "Geography", "FAIL");[/code] and my output was 2013-03-22 05:53:26 [iNFO] [sTDOUT] Exam_Name Exam_Grade 2013-03-22 05:53:26 [iNFO] [sTDOUT] English A 2013-03-22 05:53:26 [iNFO] [sTDOUT] French F 2013-03-22 05:53:26 [iNFO] [sTDOUT] Science C 2013-03-22 05:53:26 [iNFO] [sTDOUT] Geography FAIL You must have either done something wrong as you have posted no code I don't know that. or you found a bug in the Java SDK thus your on the wrong forums. Interesting... Your printf output was redirected through the forge logging mechanism. I'll poke around through the logging functions. Apparently they redirected STDOUT. Probably a logging option I can turn on/off somewhere. In any event. No, your java printf did not work. It did not come out through the normal console output mechanism, which is what it used to do... Thanks for the hint!