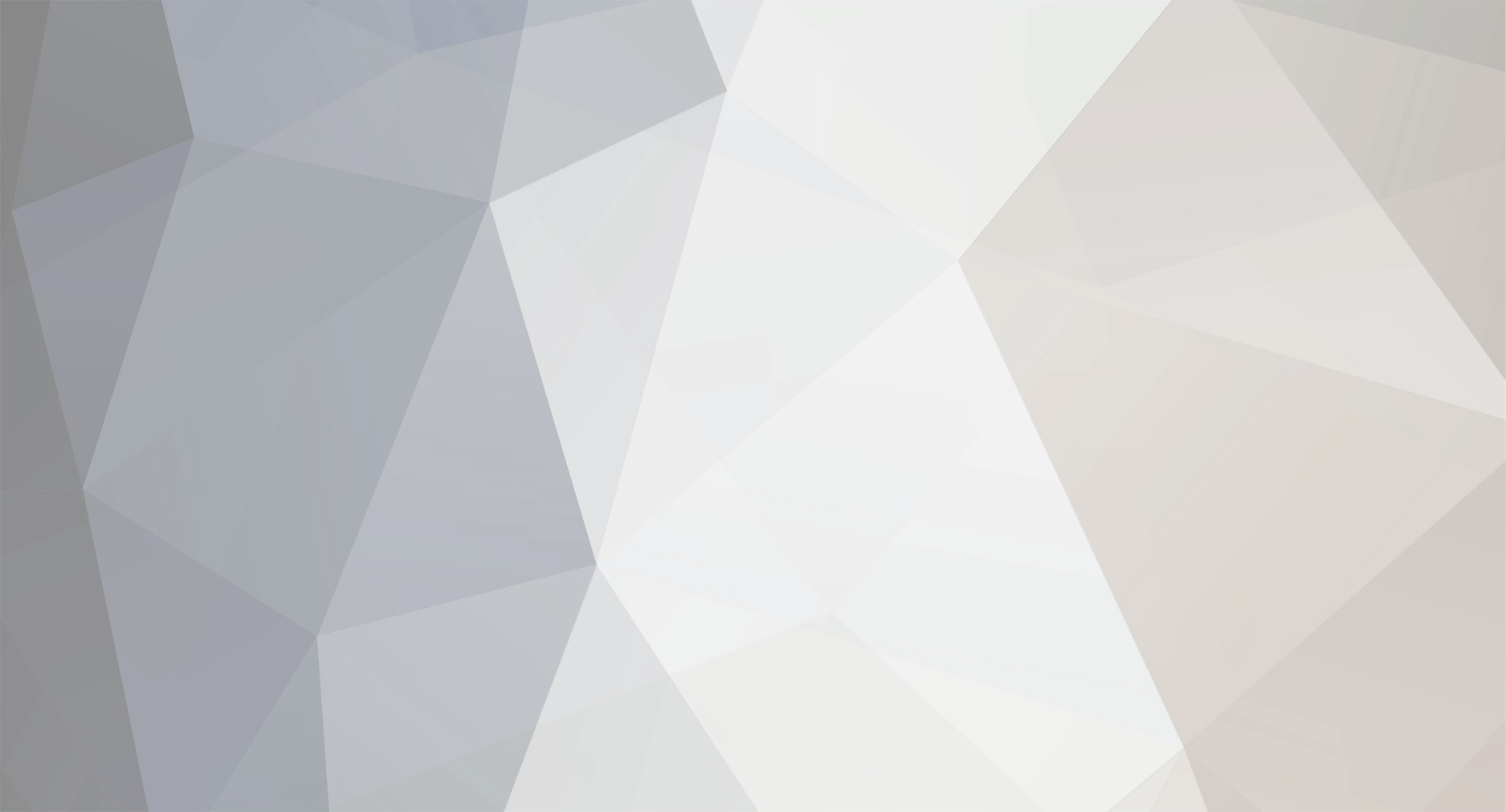
RANKSHANK
Members-
Posts
305 -
Joined
-
Last visited
Everything posted by RANKSHANK
-
How to access open gui on client from packet handler?
RANKSHANK replied to MarcinS's topic in Modder Support
just send it to the gui: //pseudo code public void onPacketData(DataInpuStream d){ if(!Minecraft.getMinecraft.inGameHasFocus && Minecraft.getMinecraft().currentScreen instanceof YourGuiScreen) ((YourGuiScreen)Minecraft.getMinecraft().currentScreen()).handlePacketData(d); } -
a drawn circle = static Tessellator tess = Tessellator.instance; /**because processing speed > ram*/ static double factor = Math.PI/2; /**the non math- mindeds' dragon*/ public static void circle(double radius){ tess.startDrawing(GL11.GL_TRIANGLE_STRIP); tess.addVertex(0,0,0); //100 is example, the bigger the factor, the rounder the sides, this will draw regular polygons too(hexagon, octagon, etc), when you figure out how to use it for(int j = 0; j <= 100; j++) tess.addVertex(radius*MathHelper.cos(j*factor), radius*MathHelper.sin(j*factor),0); tess.draw(); } or you could use parametrics for a traced circle double posX,posY; public void traceCircle(int time){ posX = Math.cos(time/180); posY = Math.sin(time/180); } which is a simplified version of what i used back in the pikmin mod for way points and whistling what you should try to draw is a teapot
-
a drawn circle = static Tessellator tess = Tessellator.instance; /**because processing speed > ram*/ static double factor = Math.PI/2; /**the non math- mindeds' dragon*/ public static void circle(double radius){ tess.startDrawing(GL11.GL_TRIANGLE_STRIP); tess.addVertex(0,0,0); //100 is example, the bigger the factor, the rounder the sides, this will draw regular polygons too(hexagon, octagon, etc), when you figure out how to use it for(int j = 0; j <= 100; j++) tess.addVertex(radius*MathHelper.cos(j*factor), radius*MathHelper.sin(j*factor),0); tess.draw(); } or you could use parametrics for a traced circle double posX,posY; public void traceCircle(int time){ posX = Math.cos(time/180); posY = Math.sin(time/180); } which is a simplified version of what i used back in the pikmin mod for way points and whistling what you should try to draw is a teapot
-
Crazy crafting recipe request - two outputs, 4 billion combinations
RANKSHANK replied to Reika's topic in Modder Support
I'm betting you're after Item.getContainerItemForItemStack(ItemStack). not sure if that's the exact name but you just return the corresponding itemstack -
first of all: ALWAYS CLOSE THE STREAM. ALWAYS. also you aren't specifying what to read, the Data(Output/Input)Streams comes with UTF handling. You could try to use that instead of reading fully. And you cannot throw a byte array into the stream and expect it to be received as a char array(String). If you want to handle raw byte arrays try using a byte buffer like so: //for reading byte[] b = data.read(); ByteBuffer.wrap(b).asCharBuffer().array().toString(); although you'd have to break it down to match the ByteBuffer formatting on input (UTF != charBuffer formatting). I'd just stick with DataOutputStream.writeUTF("yadda");
-
passing in a class and then instantiating later?
RANKSHANK replied to endershadow's topic in Modder Support
start with something like public Class<? extends BowType.class> type; public itemConstructor(int id, Class<? extends BowType.class> c){ super(i); type = c; } ; then depending on if the class constructor needs parameters public BowType bowInit(){ try{ //no parameters return type.newInstance(); } catch(Exception e){ e.printStackTrace(); } return null; } or public BowType bowInit(){ try{ return type.getConstructor(Parameter1.class, Parameter2.class).newInstance(parameter1Object, parameter2Object); } catch(Exception e){ e.printStackTrace(); } return null; } -
If that worked with server connections, that would definitely be a must have-
-
public class foo{ public static final String[] yadda = new String[3]; //Only called once static{ yadda[0] = "blah"; yadda[1] = "herp"; yadda[2] = "derp"; } //as opposed to this, which is called when the class is given a new Object instance { yadda[2] = "merp"; } } [code] argument is invalid. no method is needed to assign static variables. though the point on using initializers is a good one for this case, however it's not fun when it could just be recursed over or needs to be read externally, but that's a whole different question
-
[1.5.1] Consuming an item trough a Gui Button
RANKSHANK replied to SenpaiSubaraki's topic in Modder Support
The double consuming is probably a client side bug: the item is consumed and the packet to consume the item is sent- OR it is an internal server issue- either way make sure the method is all packet based -
you can't parse your own long? because it looks like forge offers string config types.
-
[unsolved]Installing mod gives java.lang.NoClassDefFoundError
RANKSHANK replied to Azurn's topic in Modder Support
you didn't add a stray @SideOnly right? -
there are a few issues, one of the forge mods has stated before (I think Lex because I remember a response laden with sarcasm) but the issue then becomes.... less than intelligent modders whom hard code thier ids or not use forge(dirty modloader junkies) messing up the ID hiearcy. then there is save handling, the changes values are bad, and not all chunks are loaded per session, so Thatcher mean the id coorelation would have to be saved per chunk, or load times would increase as all should be unloaded chunks are loaded, processed, and unloaded, and if a tile entity that holds a dynamically id'd item, how will you know to update that during processing. its a pain in the butt, but not much can be done about it... I don't personally like forges config system(7 separate mods in my environment) so I built an annotation based configuration reader/writer (if any forge dev is interested ill toss some links, otherwise can't be bothered wasting time with setting up for a PR), so how do you account for lazy programmers like me? you cant, try the KD resolver, or make a mod that installs your cofigs from in the mod zip/jar if they don't exist
-
If its not forge based nothing can be done about it
-
Allocate more memory to your JVM or add nogui to your launch parameters. This is not a forge issue
-
I ♥ Linux, beautiful OS, its the anti Mac with all the customizability, but I hope you know the jargon or are dual booting since common tongue is not spoken in Linux how toos anyways more info is needed, such as: do you have the correct version of python installed?
-
Random Render Brightness and Entities float away! Help! (Please :) )
RANKSHANK replied to Jamezo97's topic in Modder Support
First issue is minecrafts.... unique... lighting system at work. Not much you can do, you can seel some of its charcteristics when you rotate an item in a frame . The second issue needs some entity code posted. -
Dependant on OS: 1)recompile.bat / .sh 2)reobfuscate.bat /.sh
-
You will either have to: 1) rely on random ticks to update the block to a lit one, not precise 2) use a tile entity to keep track of time, heavier on processing 3) keep track of all loaded block coords and update via a scheduled tick handler. Or you could try using a scheduled tick handler to manipulate a boolean that sets the light value on Day/night rotations, however sleep will ruin the tick handler so you'll need to make a check for that...
-
And when the TE is unloaded? Better off storing and writing to a custom nbt file. Just use event a packets and commands to tell where and when to send it
-
an ability to not render the rider of a spesific entity
RANKSHANK replied to Ninjusk's topic in Suggestions
It wraps.... not replaces. Meaning you can wrap the wrapper...and if someone replaces the render before it those changes are kept. The problem is if some blundersome programmer replaces or wraps out of order (forced invisibility is a top priority, so should be done last) -
an ability to not render the rider of a spesific entity
RANKSHANK replied to Ninjusk's topic in Suggestions
Wrap the rendering instance to your need, I'm too lazy to research it but pseudo code: public class RenderWrapper extends Render{ private Render wrappedRenderer; public static List<Class<? extends Entity>> norend = new ArrayList(); static{ norend.add(yourEntity.class); } public RenderWrapper(Render r){ wrappedRender = r } public void doRender(Entity e, //Otros parameters){ if(e.isRiding && !norend.containse(e.entityRidden.getClass())) wrappedRender.doRender(e, other parameters); } } { Using client proxy of course public void postInit(FMLPostInitializationEvent e){ Rederplayer r = RenderingRegistry.renderList.get(playerrender); RenderingRegistry.renderList.remove(r); RenderingRegistry.registerEntityRender(EntityPlayer.class,new RenderWrapper(r);//super pseudo } No Asm reflection(I think), base edits. -
Pseudo code [ code] system.out.println(StatCollector.translateToLocal( Block.field_94337_cv.getUnlocalizedName()));
-
Then go look at the snowball renderer
-
Lex just locked a thread on exactly this. Use your searchbar. Or just look at the forum. 3 topics ago.
-
They say that to prevent people whom learn java from YouTube videos instead of textbooks from making really sloppy and slow programs. Not really though, its specific to dynamic reflection. Byte code is generated after about 15 reflective calls dependant on your runtimes threshhold. I suggest you read Bloch's efficient java before labeling poor programming form in methods you don't understand.