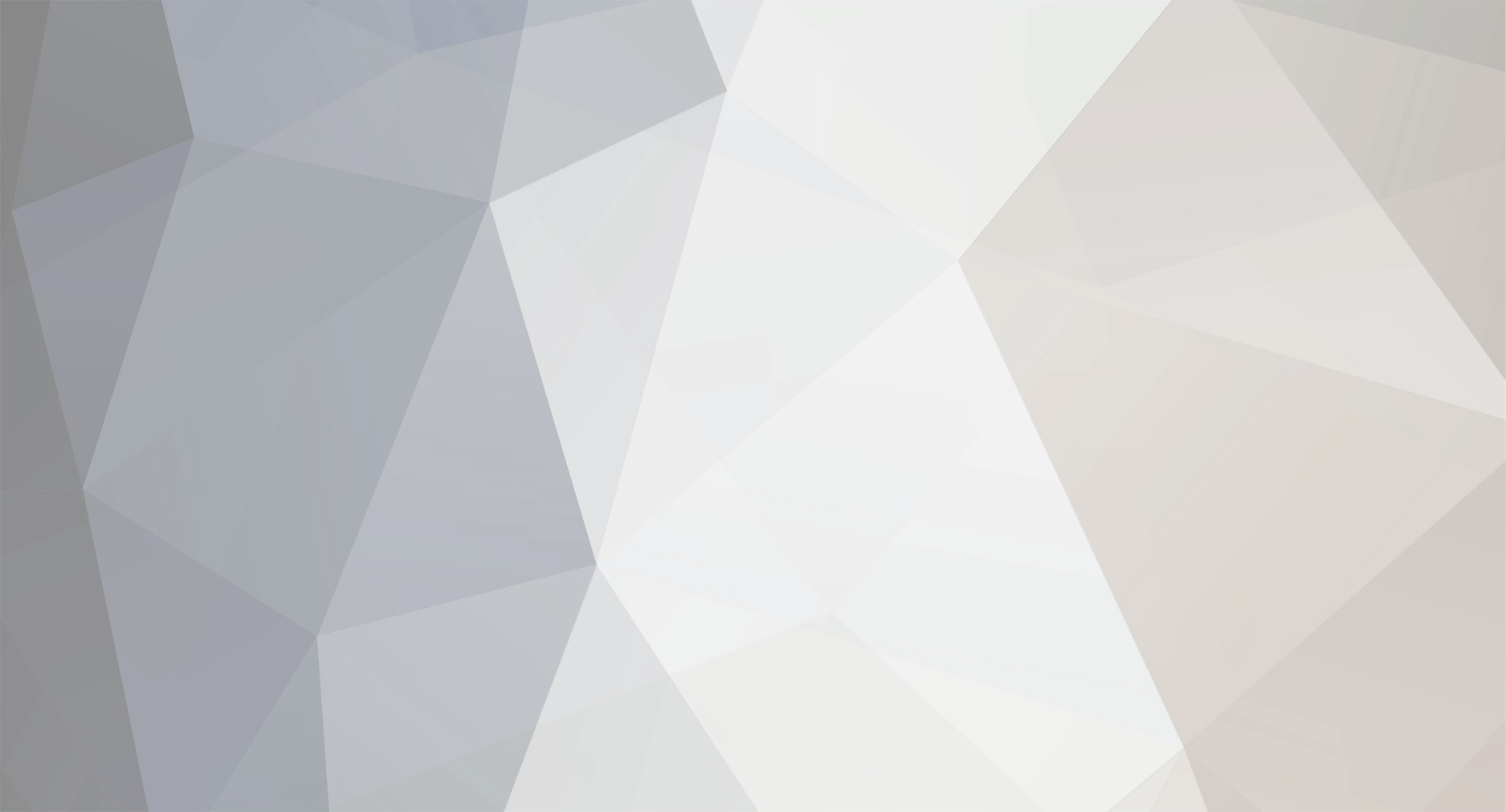
ShetiPhian
Members-
Posts
198 -
Joined
-
Last visited
Everything posted by ShetiPhian
-
[still unsolved]where to place texture
ShetiPhian replied to buster_the_dog's topic in Modder Support
if this is 1.6 you need to change "mods" to "assets" and "slenderChicken" must be lowercase "slenderchicken" MCP Location /mcp/src/minecraft/assets/slenderchicken/textures/entity/slenderChicken.png Zip/Jar Location /assets/slenderchicken/textures/entity/slenderChicken.png EDIT: redmen800 has a nice guide up, http://www.minecraftforum.net/topic/1878002-161forgeupdating-to-161-texturesinitialization/ -
How can I assign a static int to my item, then read from it later?
ShetiPhian replied to Flenix's topic in Modder Support
You could store all the values in a map, public static Map<Item, Integer> itemValue = new HashMap<Item, Integer>(); add item/value pair itemValue.put(theItem, thevalue); get value of an item if (itemValue.containsKey(theItem)) { value = itemValue.get(theItem); } That would also allow you to use item your mod doesn't add. If it's only for your own items, you could add it directly to your items class and read from it later. public class ItemValued extends Item { public static itemValue = 200; ...... Retrieved later if (item instanceof ItemValued) { value = ((ItemValued)item).itemValue; } -
[1.6.1] Block that creates 'larger block'
ShetiPhian replied to KeeganDeathman's topic in Modder Support
you missed a line in your onBlockActivated ((TileEntityTowerFloor)tile).shouldRender = true; As for rendering right away, did you set shouldRender in your tile entity to false? What about checking shouldRender in your rendering code? -
That code allows your blazePowder to replace the original, so you should be able to eat it. You'll need to do similar code you make your other replacements work also. But I overlooked "add item subtext" Now I'm assuming your trying to add more info to the tooltip. In which case you current setup has problems. Your replacement is to ItemFood, which allows you to eat it, but you need to use your class and have it extend ItemFood/ItemFoodSeed before you do anything else. New Class : ItemEatWeirdly public class ItemEatWeirdly extends ItemFood { String[] subText; public ItemEatWeirdly(int id, int foodValue, float saturation, boolean wolfEat, String...subText) { super(id, foodValue, saturation, wolfEat); this.subText = subText; setCreativeTab(CreativeTabs.tabBrewing); setAlwaysEdible(); } public ItemEatWeirdly setEatEffect(int potionId, int potionDuration, int potionAmplifier, float potionProbability) { setPotionEffect(potionId, potionDuration, potionAmplifier, potionProbability); return this; } public ItemEatWeirdly setBrewEffect(String brewEffect) { setPotionEffect(brewEffect); return this; } public ItemEatWeirdly setNameAndIcon(String name, String icon) { setUnlocalizedName(name); func_111206_d(icon); return this; } @Override public void addInformation(ItemStack itemStack, EntityPlayer entityPlayer, List list, boolean par4) { if (this.subText != null && this.subText.length > 0) { for (String text : this.subText){ list.add(text); } } } } New Class : ItemEatWeirdlySeed public class ItemEatWeirdlySeed extends ItemSeedFood { String[] subText; public ItemEatWeirdlySeed(int id, int foodValue, float saturation, int cropId, int soilId, String...subText) { super(id, foodValue, saturation, cropId, soilId); this.subText = subText; setCreativeTab(CreativeTabs.tabBrewing); setAlwaysEdible(); } public ItemEatWeirdlySeed setEatEffect(int potionId, int potionDuration, int potionAmplifier, float potionProbability) { setPotionEffect(potionId, potionDuration, potionAmplifier, potionProbability); return this; } public ItemEatWeirdlySeed setBrewEffect(String brewEffect) { setPotionEffect(brewEffect); return this; } public ItemEatWeirdlySeed setNameAndIcon(String name, String icon) { setUnlocalizedName(name); func_111206_d(icon); return this; } @Override public void addInformation(ItemStack itemStack, EntityPlayer entityPlayer, List list, boolean par4) { if (this.subText != null && this.subText.length > 0) { for (String text : this.subText){ list.add(text); } } } } In you main class, @EventHandler // this is for 1.6 if your on 1.5 use @PreInit public void preInit(FMLPreInitializationEvent event) { int replaceId; replaceId = Item.blazePowder.itemID; Item.itemsList[replaceId] = new ItemEatWeirdly(replaceId - 256, 2, 0.3F, true, "SubText", "another line").setNameAndIcon("blazePowder", "blaze_powder").setBrewEffect(PotionHelper.blazePowderEffect).setEatEffect(Potion.damageBoost.id,10, 0, 1.0F); replaceId = Item.netherStalkSeeds.itemID; Item.itemsList[replaceId] = new ItemEatWeirdlySeed(replaceId - 256, 2, 0.3F, Block.netherStalk.blockID, Block.slowSand.blockID, "SubText", "another line", "you can keep adding strings here").setNameAndIcon("netherStalkSeeds", "nether_wart").setBrewEffect("+4").setCreativeTab(CreativeTabs.tabMaterials); } the string used for the icon is found in .func_111206_d(" something here ");
-
Is this the best way to disable the 'moved too quickly' check?
ShetiPhian replied to jayemen's topic in Modder Support
Though it not much help if your using a mod by another coder, having the server move the player gets around this issue. when I first attempted moving the player I did it client side and kept getting the moved wrongly error. Now server side I'm using entityPlayer.setPositionAndUpdate(placeX + .5, placeY, placeZ + .5); to move the player. -
[1.6.1] Block that creates 'larger block'
ShetiPhian replied to KeeganDeathman's topic in Modder Support
Replying here instead just in case someone else runs across this needing the same answers. To your block class add (your block will need to extend BlockContainer or implement ITileEntityProvider before its available) @Override public TileEntity createNewTileEntity(World world) { return new TileEntityLargeBlock(); //Rename to your tile entity } This will create the tile entity as soon as the block is in the world, but you didn't want it to render until your right clicked so in your tile entity add public boolean shouldRender = false; Back to your block class @Override public boolean onBlockActivated(World world, int x, int y, int z, EntityPlayer entityPlayer, int face, float posX, float posY, float posZ) { TileEntity tile = world.getBlockTileEntity(x, y, z); // Once again rename to your tile entity. if (!(tile instanceof TileEntityLargeBlock)) { return false; // If for some reason your block does not have the correct tileentity you don't want it to create the false blocks, as nothing will hide them. } // run any pre-generation code you have here. eg. making sure there is space for the false blocks. ((TileEntityLargeBlock)tile).shouldRender = true; // create your false blocks here as they are now hidden return true; } The last thing to do is in your renderer, simply check if shouldRender is true before rendering, if not leave without rendering anything. You can change the other code I gave you too, @Override public AxisAlignedBB getCollisionBoundingBoxFromPool(World world, int x, int y, int z) { TileEntity tile = world.getBlockTileEntity(x, y, z); // Once again rename to your tile entity. if (tile instanceof TileEntityLargeBlock && ((TileEntityLargeBlock)tile).shouldRender) { return AxisAlignedBB.getBoundingBox(x - 1, y - 1, z - 1, x + 1, y + 1, z + 1); } return super.getCollisionBoundingBoxFromPool(world, x, y, z); } -
[1.6.1] Block that creates 'larger block'
ShetiPhian replied to KeeganDeathman's topic in Modder Support
If your model is based of the centre block, adding this to your blocks class should do the trick. @Override public AxisAlignedBB getCollisionBoundingBoxFromPool(World world, int x, int y, int z) { return AxisAlignedBB.getBoundingBox(x - 1, y - 1, z - 1, x + 1, y + 1, z + 1); } -
Initializing game java.lang.NullPointerException [1.6.1]
ShetiPhian replied to Beelzaboss's topic in Modder Support
Its out of order, you need to define the part before you can change it If you switch l22 and l23 around the order will be correct, you could also delete l22 because it is repeated on l27 -
I've just started playing with them myself. Here is what I've changed from the default Blender export options uncheck "Write Materials" generates an unneeded file check "Triangulate Faces" the importer wouldn't accept any of my models without this, even if I pre-applied a triangulate modifier uncheck "Objects as OBJ Objects" the importer rejects the lines this adds The the other change I do is check "Selection Only" but that only needed if you have parts you don't want exported
-
Never edit base classes diesieben07 was referring to doing this: Item.itemsList[item.blazePowder.itemID] = <yourItem>
-
do you have a mcmod.info file? [ { "modid": "", "name": "", "description": "", "version": "", "mcversion": "", "url": "", "updateUrl": "", "authors": [ "" ], "credits": "", "logoFile": "", "screenshots": [], "parent":"", "dependencies": [] } ] Is the zip structure correct? <yourzip>/mcmod.info <yourzip>/assets/%modname%/textures/ <yourzip>/%modpackage%/<mod files> <= I figure this is correct as it works unziped
-
[1.6] Missing getServerData() from Minecraft.java
ShetiPhian replied to maxpowa's topic in Modder Support
There are a few places I needed to do something similar, I first try for the obfuscated name and if that fails check for the "normal" name I've been looking up the obfuscated names in these files. /mcp/config/fields.csv /mcp/config/methods.csv In this case it looks like field_71422_O is currentServerData you can also go to the mcp irc channel and by whispering the bot get the data, that way is much easier when there is a few that are similar in name (and the csv lacks a description) as the bot provides more info. -
Textures and LanguageRegistry in preInit instead of Init
ShetiPhian replied to sorash67's topic in Modder Support
I had a bit of a texture problem but have solved it. Here is what I've done, it might be useful for others Always use the newest Forge, at the time of writing this #762 is what I'm using. ------------- @PreInit @Init @PostInit All become @EventHandler ------------- Your blocks and items must be registered during FMLPreInitializationEvent otherwise registerIcons is not called and causes a null icon crash when item is viewed. According to CPW, this is where they should be anyway. (Mod.EventHandler Javadoc) [Reformatted for forums] ------------- NOTE: In my Eclipse I have a source folder for my textures. they are not in the mcp folder, so I can not tell you if it works. 1.5 texture location: mods.ModName.textures.blocks mods.ModName.textures.items 1.6 texture location: assets.modname.textures.blocks assets.modname.textures.items Those MUST be in lower case (this is what got me) ------------- Last change: In your blocks and items, if you used capitals in your registerIcons you need to get rid of them. iconRegister.registerIcon("ModName:block1"); ==> iconRegister.registerIcon("modname:block1"); -
Question about EntityPlayer.getItemInUse() being Side.CLIENT
ShetiPhian replied to ShetiPhian's topic in General Discussion
I was using the server side instance of the player (checking in LivingUpdateEvent) but getItemInUse is locked to the client (even though unlocked it works on the server) PlayerInteractEvent works better, it doesn't need getItemInUse and the check doesn't happen as often. -
What ever you make it. If you make a new item "myDyes" and set white to meta 0 and blue to meta 1 OreDictionary.registerOre("dyeWhite", new ItemStack(myDyes.shiftedIndex, 1, 0)); OreDictionary.registerOre("dyeBlue", new ItemStack(myDyes.shiftedIndex, 1, 1));
-
Question about EntityPlayer.getItemInUse() being Side.CLIENT
ShetiPhian replied to ShetiPhian's topic in General Discussion
I'm checking if the item is a food, and stopping the player from eating. EDIT: Found a workaround Using PlayerInteractEvent, I check for right click events, get the current item, see if its a food, cancel the event accordingly. It also solves the problem of the cake blocks avoiding my previous checks. stops the block from being used but players still get the food value. -
Is there a reason EntityPlayer.getItemInUse() is flagged @SideOnly(Side.CLIENT) ? Removing @SideOnly(Side.CLIENT) doesn't appear to cause any problems (very limited testing) Testing on both the built-in LAN server and stand alone dedicated server (through Eclipse) returned the correct item every time. If it has to stay client side only, does anyone know of a server side equivalent?
-
If you want the resulting item to be bone meal / lapis Just set up a standard crafting with the result being ItemStack(Item.dyePowder, 1, 15) / ItemStack(Item.dyePowder, 1, 4) But if you want the result to be a white / blue dye alternative Create your item and recipe like normal, then register your item to the ore dictionary OreDictionary.registerOre("dyeWhite", new ItemStack(YOUR_ITEM_ID, 1, WHITE_META)); OreDictionary.registerOre("dyeBlue", new ItemStack(YOUR_ITEM_ID, 1, BLUE_META)); Any mod that checks the ore dictionary for dye can use your item. http://www.minecraftforge.net/wiki/Ore_dictionary http://www.minecraftforge.net/wiki/How_to_use_the_ore_dictionary
-
Currently not drawing with gui's open, but would prefer to have it working like the default one. (Always drawn, darkened when gui's are open, covered up by other gui's elements instead of being on top) The only two ways I can think to do that are: 1 - forge hook after the ingame gui is drawn, before any guiscreen gets drawn 2 - tell minecraft to use an EntityRenderer I create instead of the original (not something I'd do for just a hud though)
-
I have my HUD in the tick handler now, but I need to stop drawing it when other interfaces are open due to my HUD elements appearing top level (setting the z level has no effect) What I had in mind was more visually pleasing but not worth performance loss, thus my question about it having a negative effect on the event bus.
-
Other mods modifying stackSize directly screws up my mod
ShetiPhian replied to copygirl's topic in General Discussion
I'm now wondering if this is the reason my chests don't seem to work correctly with automation. I've been looking things over but just couldn't seem to find the problem. Is your issue the same as mine? The tile entity of each chest is storing its own items, Breaking a chest drops all of the items stored in the tile entity. GUI shows inventory of all joined chests. Player can shift click items in and out of any slot, extra items will spill over into the next joined inventory. But when connected to BuildCraft pipes only the tile entity the pipe connects to is ever accessed. -
How to make a crafting-recipe WITH Item, you won't loose
ShetiPhian replied to elterPro's topic in Modder Support
I have a note on this.... ok here it is, Now I haven't tested it myself but I found it here a while back. -
Take a closer look at the events I gave you, one will do what you want. It provides the player and block. It also gets called the entire time the block is being broken. As for the held item goes, once you have the player getting that is very easy. Oh and running others down results in less people willing to help, and if done enough no one will help with future problems.
-
Use the newest version, 1.25 to 1.3 required mods to be rewritten, so unless you like doing things twice there is no point in back dating. you'll want to use the event system net.minecraftforge.event.entity.player.AttackEntityEvent; net.minecraftforge.event.entity.player.PlayerEvent.BreakSpeed; net.minecraftforge.event.entity.player.PlayerEvent.HarvestCheck; AttackEntityEvent will allow you to edit how much damage a weapon does. HarvestCheck returns true if the current tool can harvest the block and false otherwise BreakSpeed sets how fast a harvest-able block can be broken with the current tool AtomicStryker has an Events HowTo: http://www.minecraftforum.net/topic/1419836-forge-4x-events-howto/ Other helpful links: http://www.minecraftforge.net/wiki/Tutorials http://www.minecraftforge.net/wiki/Tutorials/Upgrading_To_Forge_for_1.3.1
-
[PROBLEM] 3 Forge Issues, Any help would be greatly appreciated!
ShetiPhian replied to KingAtomx's topic in Modder Support
MinecraftForge.setBlockHarvestLevel Is your mcmod.info in the root of your zip? The formatting might be off slightly (I forgot a comma once )