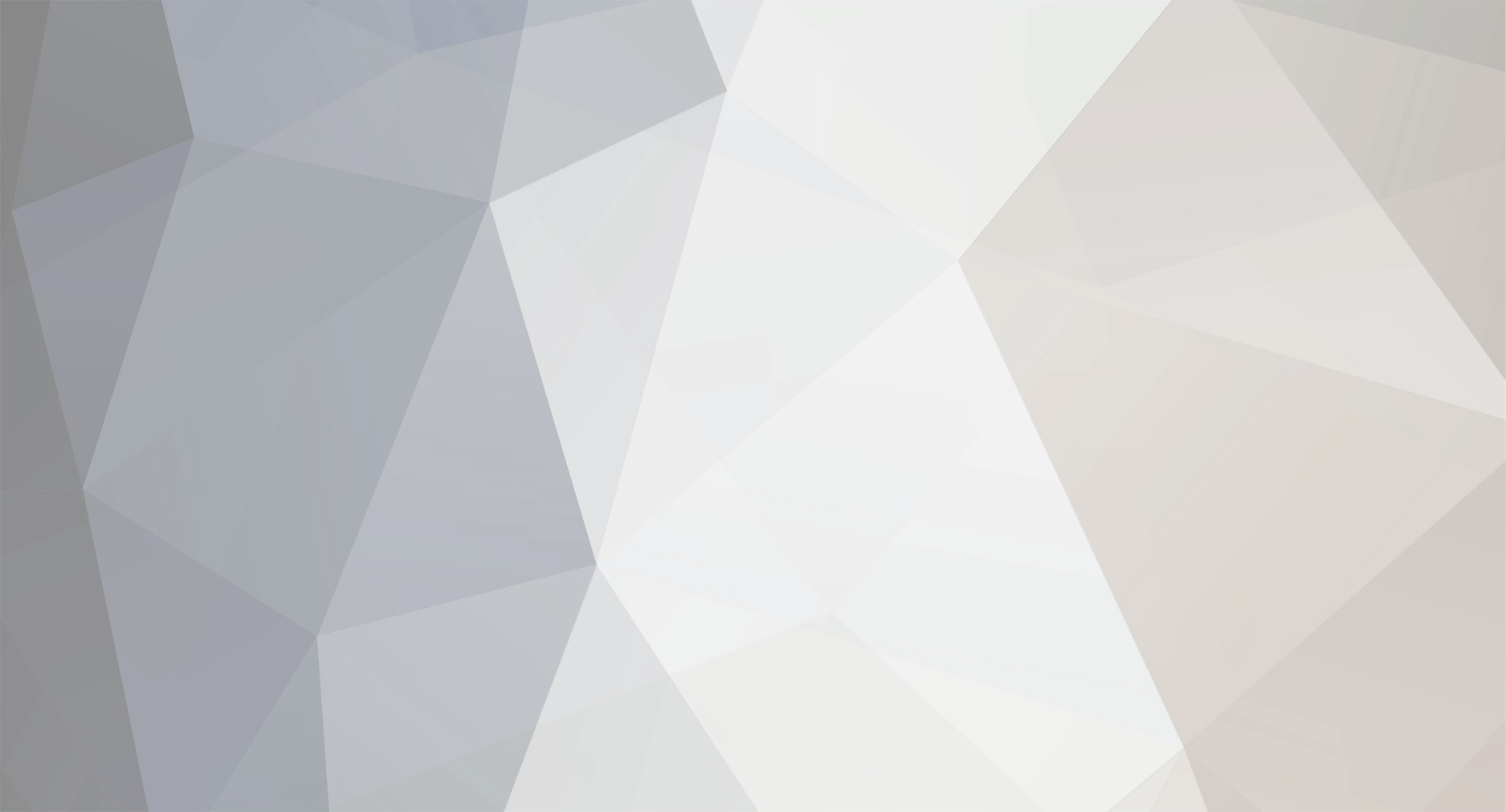
Guff
Members-
Posts
159 -
Joined
-
Last visited
-
Days Won
1
Everything posted by Guff
-
ITickHandler.
-
Detecting if the character has a specific armor equiped
Guff replied to thib92's topic in Modder Support
If you are using eclipse, right click in the file, go to source, select Override/Implement methods, go to the Item head, and select onArmorTickUpdate() and it will override it for you in your file. Then you add your code there. -
Detecting if the character has a specific armor equiped
Guff replied to thib92's topic in Modder Support
Well, I suppose you could override onArmorTickUpdate() in your item class, and then put the code in there, and it will work for all of the armor pieces as long as one of them is equipped. -
Detecting if the character has a specific armor equiped
Guff replied to thib92's topic in Modder Support
Specifically, what sequence of events do you want to happen that will lead to the point of having to see if the player has armor equipped? -
Detecting if the character has a specific armor equiped
Guff replied to thib92's topic in Modder Support
Assuming you are doing this with a blockActivated() or itemRightClick(), you can use the player parameter to do this: int id = player.inventory.armorInSlot(slot).itemID; if(id == myItem.itemID) { //do stuff } Vanilla code. -
You're welcome.
-
Use a Tile Entity, since they are updated every tick. Block's randomDisplayTick() is not ideal since it is just that; random.
-
Assuming you have the block's coordinates i, j, k: AxisAlignedBB aabb = AxisAlignedBB.getBoundingBoxFromPool(i - 5, j - 5, k - 5, i + 5, j + 5, k + 5); Then just use the same thing I showed you before.
-
In order to use NBT, you'll need tile entities. However, let's start with the item. In order to stop the chat from showing twice, every time you send chat to the player, you should surround it with: if(FMLCommonHandler.instance().getEffectiveSide() == Side.CLIENT) An important thing to remember for both Items and Blocks is that they are static objects - changing one will change all of them. If you change the mode for one player, it will also change it for other players, as the item with the ID 'x' will all be the same. Tile entities are how blocks become independent of being completely static - you can have multiple furnaces running out of sync with each other because they use tile entities. Items work the same way with ItemStacks. ItemStacks are all unique objects. For example, you could have two stacks of 32 wheat in your inventory, but they are not the same stack. Both tile entities and item stacks use NBT, so this is extremely helpful. When changing mods, change the mode using NBT on the item stack. As such, you should pass the ItemStack parameter to activateTool and changeMode, and then using NBT: public void changeMode(EntityPlayer player, ItemStack stack) { NBTTagCompound nbt = stack.getTagCompound(); if (nbt == null) { nbt = new NBTTagCompound(); stack.setTagCompound(nbt); } int mode = nbt.getInteger("mode"); if (mode < modes.length) { mode++; } else { mode = 0; // Use zero as your base instead of one } nbt.setInteger("mode", mode); if (FMLCommonHandler.instance().getEffectiveSide() == Side.CLIENT) { player.sendChatToPlayer(ChatColours.LightAqua + "Mode: " + modes[mode]); } } From that, you should be able to figure out how to change activateTool() and add the ItemStack parameter. Remember, everything that should not be shared between items should be set to the ItemStack, and everything that should not be shared between blocks should be set to the TileEntity. When you set up said tile entity, you will need to super-override readFromNBT() and writeToNBT(), and you will need to add the fields: private int teleportX; private int teleportY; private int teleportZ; Those will need to be written to and read from the passed NBT in the two methods you need to super-override, like this: public void writeToNBT(NBTTagCompound nbt) { super.writeToNBT(nbt); nbt.setInteger("teleportX", this.teleportX); nbt.setInteger("teleportY", this.teleportY); nbt.setInteger("teleportZ", this.teleportZ); } A similar thing is done with read from, but instead of setting, you will get: this.someVariable = nbt.getInteger("variableKey"); Hopefully this helps! If you need more, just ask.
-
Getting all instances of a block to do something?
Guff replied to googoo1876's topic in Modder Support
One way to do the transmission is to basically use nested for-loops for the x, y, and z directions for when the transmitter is updating (assuming you're using a tile entity, if not, you might be able to use randomDisplayTick()), and then run through every block: if(world.getBlockId(i + i1, j + j1, k + k1) == radio.blockID) where i, j, and k are the coords of the transmitter and i1, j1, and k1 are the offsets in the for-loops (-range <= i1 < range). Then under the if-statement, you can do something with the radio's tile entity (assuming you have one) like this: ((TileEntityRadio)world.getBlockTileEntity(i + i1, j + j1, k + k1)).playRadio(); and then your code for the radio's range will go in the entity file under play radio, to basically play a sound, song, etc at that coordinate using the Tile Entity's worldObj and coords, at a specific loudness to cover the radio's range. I'm sure you can figure out the things like whether or not a radio is on or not. Also, sorry if this confused you, I think you had something else in mind but I find probably the easiest way to do it. -
Getting all instances of a block to do something?
Guff replied to googoo1876's topic in Modder Support
What, specifically, are you trying to do? -
Getting all instances of a block to do something?
Guff replied to googoo1876's topic in Modder Support
If you mean every block in the world, the only way I could think it could be done would be to literally check every block in the world (the stored blocks array) and check if each one has the specific ID, and if it does, add it to the list. It'd be best to check a small range (maybe -64 to 64 in x, y, z directions) and see which of those blocks, if any, are the block ID you're looking for, and then add them. Doing literally every block in a world could cause extreme lag running through nested loops that many times. -
Replace: private static Item breadslice = new BreadSlice(5000).setUnlocalizedName("breadslice").setMaxStackSize(64).setCreativeTab(CreativeTabs.tabFood).func_111206_d("Sandwich:slicebread"); with private static Item breadslice; Move all of this to the method receiving FMLPreInitializationEvent: proxy.registerRenderers(); breadslice = new BreadSlice(5000); languageRegisters(); craftingRecipes(); Replace: breadslice = new BreadSlice(5000); with breadslice = new BreadSlice(5000).setUnlocalizedName("breadslice").setMaxStackSize(64).setCreativeTab(CreativeTabs.tabFood).func_111206_d("Sandwich:slicebread"); Annotate your methods that are receiving FML events with this: @EventHandler
-
To find all of the hostile mobs within 5 blocks, List<Entity> entities = world.getEntitiesWithinAABBExcludingEntity(player, player.boundingBox.expand(5.0D, 5.0D, 5.0D)); and then run through those and see which ones are hostile via instanceof. Then, if they are, entity.setDead().
-
Using a value from the player NBT as a string in a GUI
Guff replied to Flenix's topic in Modder Support
The Gui is Client-side and all he wants to do is display the value to the player, and as far as I can tell he wants nothing to do with sending it to the server and doing anything with it there. -
There is a way around, but look at the way he's modding. He's extending BaseMod, using mod_, using the load() method. These are all things from the ModLoader days, and he's asking for help on the Forge forums. The best way around is to get yourself up-to-date with Minecraft Forge, specifically since you're asking for help on these forums. Among hundreds of other things, it allows the addition of tool materials without the need to edit any classes. As for your problem, you didn't add an enum called Synthesis_IRON in EnumToolMaterial, so naturally it will give you an error.
-
Good, glad I could help.
-
Using a value from the player NBT as a string in a GUI
Guff replied to Flenix's topic in Modder Support
If I may, this may clean up your file a bit as well as help you with your question, without packets: //If you're not using the world and coord params, you can remove them public GuiATM (InventoryPlayer inventoryPlayer, TileEntityATMEntity tileEntity, World world, int x, int y, int z) { super(new ContainerATM(inventoryPlayer, tileEntity)); this.xSize = 176; this.ySize = 198; this.player = inventoryPlayer.player; } //these are inherited, no need to make new ones. // protected int xSize = 176; // protected int ySize = 198; private EntityPlayer player; @Override protected void drawGuiContainerForegroundLayer(int param1, int param2) { fontRenderer.drawString("ATM", 8, -8, 4210752); fontRenderer.drawString("Balance", 8, 9, 4210752); fontRenderer.drawString("Safe", 132, -11, 4210752); fontRenderer.drawString("Deposit", 124, -3, 4210752); fontRenderer.drawString("Withdraw", 8, 29, 4210752); fontRenderer.drawString("Deposit", 42, 68, 4210752); String playerBalance = this.player.getEntityData().getInteger("balanceKey") + ""; //playerBalance shouldn't be static. fontRenderer.drawString("$" + TileEntityATMBlock.playerBalance, 58, 9, 1237000); fontRenderer.drawString("345.67", 58, 29, 1237000); fontRenderer.drawString(StatCollector.translateToLocal("container.inventory"), 8, ySize - 111, 4210752); } @Override protected void drawGuiContainerBackgroundLayer(float par1, int par2, int par3) { GL11.glColor4f(1.0F, 1.0F, 1.0F, 1.0F); this.mc.func_110434_K().func_110577_a(texture); int x = (width - xSize) / 2; int y = (height - ySize) / 2; this.drawTexturedModalRect(x, y, 0, 0, xSize, ySize); } Obviously balanceKey is probably not where you actually stored the player balance, and I don't even know if you stored the balance inside of the player's NBT. If you did, however, that's how you would get it, but just changing the key to your key for balance. Then you can draw whatever string you want to by appending that, I know you know what you're doing. Since every Gui that comes up is a unique instance of the type, this will work even when two different people are using the block at the same time. -
ItemStack can't be extended, it is final. However, getting an integer shouldn't throw NPE as it will always either return a value if it has one or 0 if it does not. Look at the getInteger(String) method in NBTTagCompound and you'll see that it always returns something. The NPE is coming from one of two things (most likely the second) - The stack is null or it's tag compound is null. ItemStack tag compounds are protected against NPE, so first do this: NBTTagCompound nbt = stack.getTagCompound(); if(nbt == null) { nbt = new NBTTagCompound(); stack.setTagCompound(nbt); } A specific example of what you're trying to do with the NBT would be helpful, that way I can tell you where to set the int value for retrieval later.
-
Any method where you have an ItemStack parameter, you can assign data to it/get data from it using stack.getTagCompound().
-
You used for loops and if statements?
-
You shouldn't need to use 27k world.setBlock invocations. You can use if statements, for loops, etc. and it will cut down drastically on that amount. I implemented a world-gen feature for my mod called Building Blocks that uses a new file to organize the blocks into a layer-by-layer format and reads them back later when the world is generating. I wouldn't say necessarily to use Building Blocks, but if you can't figure out how to use schematics (I didn't even want to bother), it might be a good alternative.
-
In your ItemBlock, override: @Override public String getUnlocalizedName(ItemStack stack) { } Make it return whatever you need it to based on the stack's properties, specifically using stack.getItemDamage(). Then use LanguageRegistry to register names to the blocks: LanguageRegistry.addName(new ItemStack(block, 1, meta), "A Name");
-
It depends on how you're trying to check for an existing item. I think the best way would be to use it's unlocalized name, since IDs can be changed in config files which will result in the item being incorrect. Your method should be something like the following: public static boolean itemExists(String name) { for(Item item : Item.itemsList) { if(item != null) { if(item.getUnlocalizedName() == name) { return true; } } } return false; } This will check all of the available items, and if the item's unloc'd name is equal to the passed String, it will tell that the item exists and break out of the method. For example, if you want to check if the Item called diamond exists, you'd do this: itemExists("item.diamond") and it will return true once it reaches the first Item that has that. For blocks, you'll need a new method that runs through the blocks list instead of items list, and instead of "item.name" it is "tile.name". I'm sure you can figure it out from what I've already given you. You'll need to know the unlocalized names of the mod items you're looking for, but this should help you.