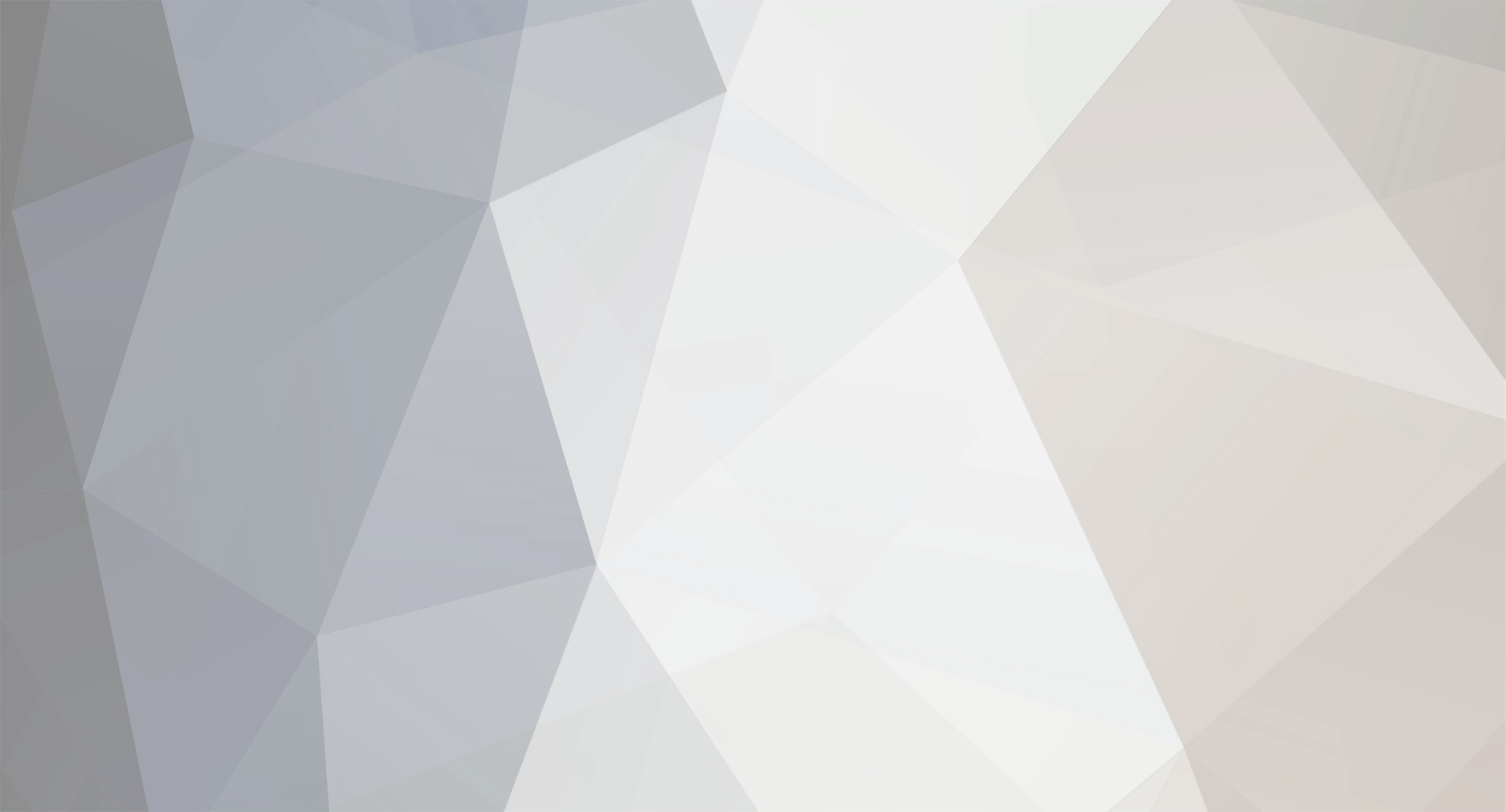
charsmud
-
Posts
89 -
Joined
-
Last visited
Posts posted by charsmud
-
-
I am attempting to slow down the speed of all entities within a specific range of the player. The method I am doing this is working, but is resulting in odd behavior (like arrows stopping suddenly in air and then rapidly updating their position, mobs visually oscillating, etc). Here is my current method:
List<Entity> entitiesToSlow = world.getEntitiesWithinAABB(Entity.class, AxisAlignedBB.getBoundingBox(player.posX - 5, player.posY - 5, player.posZ - 5, player.posX + 5, player.posY + 5, player.posZ + 5)); if(entitiesToSlow.size() > 0) { for(int i = 0; i < entitiesToSlow.size(); i++) { Entity e = entitiesToSlow.get(i); if(e instanceof EntityPlayer) { } else { double velx = (e.posX - e.prevPosX); double vely = (e.posY - e.prevPosY); double velz = (e.posZ - e.prevPosZ); if(velx != e.getEntityData().getDouble("slowX") || vely != e.getEntityData().getDouble("slowY") || velz != e.getEntityData().getDouble("slowZ")) { e.getEntityData().setBoolean("inSlowZone", false); } if(!e.getEntityData().getBoolean("inSlowZone") ) { double factor = 0.7D; e.setVelocity(velx * factor, vely * factor, velz * factor); e.getEntityData().setBoolean("inSlowZone", true); e.getEntityData().setDouble("slowX", velx * factor); e.getEntityData().setDouble("slowY", vely * factor); e.getEntityData().setDouble("slowZ", velz * factor); } } }
Is this the best way of slowing down the speed of an entity by a specific factor if it's within a specific area of the player?
-
Please read about how events work.
An event simply runs when certain conditions are met; in this case it's when a potion is brewed.
-
I am attempting to animate my armor model. The model displays, but nothing is animated; the model just follows along the player.
package timeTraveler.models; import net.minecraft.client.model.ModelBiped; import net.minecraft.client.model.ModelRenderer; import net.minecraft.entity.Entity; public class ModelSlowTimeArmor extends ModelBiped { //fields ModelRenderer belt; ModelRenderer crystal; ModelRenderer star3; ModelRenderer star2; ModelRenderer star4; ModelRenderer star5; ModelRenderer handleft; ModelRenderer torso; ModelRenderer lid4; ModelRenderer handright; ModelRenderer star; ModelRenderer star1; ModelRenderer button; ModelRenderer frontpiece4; ModelRenderer frontpiece3; ModelRenderer helmet1; ModelRenderer helmet4; ModelRenderer helmet2; ModelRenderer helmet5; ModelRenderer crystal7; ModelRenderer helmet; ModelRenderer crystal3; ModelRenderer crystal4; ModelRenderer helmet3; ModelRenderer Shape15; ModelRenderer crystal5; ModelRenderer boots3; ModelRenderer leg1; ModelRenderer boots2; ModelRenderer frontpiece1; ModelRenderer boots1; ModelRenderer boots; ModelRenderer leg; ModelRenderer tube; ModelRenderer shouldercap1; ModelRenderer crystal6; ModelRenderer crysta7; ModelRenderer crystal8; ModelRenderer crystal9; ModelRenderer crystal10; ModelRenderer frontpiece2; ModelRenderer crystal11; ModelRenderer crystal12; ModelRenderer jar4; ModelRenderer shouldercap; ModelRenderer jar; ModelRenderer jar1; ModelRenderer jar2; ModelRenderer jar3; ModelRenderer lid; ModelRenderer lid1; ModelRenderer lid2; ModelRenderer lid3; public ModelSlowTimeArmor(float f) { super (f); textureWidth = 64; textureHeight = 64; belt = new ModelRenderer(this, 55, 14); belt.addBox(-1F, 1F, 0F, 1, 10, 3); belt.setRotationPoint(-2F, 0F, 1.8F); belt.setTextureSize(64, 64); belt.mirror = true; setRotation(belt, 0F, 0F, -0.5235988F); this.bipedBody.addChild(belt); crystal = new ModelRenderer(this, 0, 27); crystal.addBox(0F, 0F, 0F, 1, 4, 1); crystal.setRotationPoint(10.8F, -1.5F, 1F); crystal.setTextureSize(64, 64); crystal.mirror = true; setRotation(crystal, 0F, 0F, 0.7853982F); this.bipedLeftArm.addChild(crystal); star3 = new ModelRenderer(this, 2, 19); star3.addBox(0F, 0F, 0F, 1, 2, 2); star3.setRotationPoint(8.5F, 8.7F, -1.4F); star3.setTextureSize(64, 64); star3.mirror = true; setRotation(star3, 0.7853982F, 0F, 0F); this.bipedLeftArm.addChild(star3); star2 = new ModelRenderer(this, 2, 19); star2.addBox(0F, 0F, 0F, 1, 2, 2); star2.setRotationPoint(8.5F, 7.7F, -1F); star2.setTextureSize(64, 64); star2.mirror = true; setRotation(star2, 0F, 0F, 0F); this.bipedLeftArm.addChild(star2); star4 = new ModelRenderer(this, 2, 19); star4.addBox(0F, 0F, 0F, 1, 2, 2); star4.setRotationPoint(-9.5F, 7.7F, -1F); star4.setTextureSize(64, 64); star4.mirror = true; setRotation(star4, 0F, 0F, 0F); this.bipedRightArm.addChild(star4); star5 = new ModelRenderer(this, 2, 19); star5.addBox(0F, 0F, 0F, 1, 2, 2); star5.setRotationPoint(-9.5F, 8.7F, -1.5F); star5.setTextureSize(64, 64); star5.mirror = true; setRotation(star5, 0.7853982F, 0F, 0F); this.bipedRightArm.addChild(star5); handleft = new ModelRenderer(this, 0, 47); handleft.addBox(0F, -2F, -2.5F, 5, 12, 5); handleft.setRotationPoint(4F, 1F, 0F); handleft.setTextureSize(64, 64); handleft.mirror = true; setRotation(handleft, 0F, 0F, 0F); this.bipedLeftArm.addChild(handleft); torso = new ModelRenderer(this, 0, 0); torso.addBox(-4F, 0F, -2.5F, 8, 12, 5); torso.setRotationPoint(0F, 0F, 0F); torso.setTextureSize(64, 64); torso.mirror = true; setRotation(torso, 0F, 0F, 0F); this.bipedBody.addChild(torso); lid4 = new ModelRenderer(this, 0, 20); lid4.addBox(0F, 1F, 0F, 1, 1, 1); lid4.setRotationPoint(1.5F, 0.7F, 3F); lid4.setTextureSize(64, 64); lid4.mirror = true; setRotation(lid4, 0F, 0F, 1.047198F); this.bipedBody.addChild(lid4); handright = new ModelRenderer(this, 0, 47); handright.addBox(-5F, -2F, -2.5F, 5, 12, 5); handright.setRotationPoint(-4F, 1F, 0F); handright.setTextureSize(64, 64); handright.mirror = true; setRotation(handright, 0F, 0F, 0F); this.bipedRightArm.addChild(handright); star = new ModelRenderer(this, 0, 19); star.addBox(1F, 0F, 0F, 3, 3, 1); star.setRotationPoint(-2.5F, 1.5F, -3F); star.setTextureSize(64, 64); star.mirror = true; setRotation(star, 0F, 0F, 0F); this.bipedBody.addChild(star); star1 = new ModelRenderer(this, 0, 19); star1.addBox(0F, 0F, 0F, 3, 3, 1); star1.setRotationPoint(0F, 1F, -3F); star1.setTextureSize(64, 64); star1.mirror = true; setRotation(star1, 0F, 0F, 0.7853982F); this.bipedBody.addChild(star1); button = new ModelRenderer(this, 0, 41); button.addBox(0F, 0F, 0F, 2, 2, 1); button.setRotationPoint(-1F, 2F, -3.2F); button.setTextureSize(64, 64); button.mirror = true; setRotation(button, 0F, 0F, 0F); this.bipedBody.addChild(button); frontpiece4 = new ModelRenderer(this, 34, 55); frontpiece4.addBox(-5F, 0F, 0F, 5, 2, 1); frontpiece4.setRotationPoint(5F, -9F, -5.2F); frontpiece4.setTextureSize(64, 64); frontpiece4.mirror = true; setRotation(frontpiece4, 0F, 0F, -0.2617994F); this.bipedHead.addChild(frontpiece4); frontpiece3 = new ModelRenderer(this, 34, 55); frontpiece3.addBox(0F, 0F, 0F, 5, 2, 1); frontpiece3.setRotationPoint(-5F, -9F, -5.2F); frontpiece3.setTextureSize(64, 64); frontpiece3.mirror = true; setRotation(frontpiece3, 0F, 0F, 0.2617994F); this.bipedHead.addChild(frontpiece3); helmet1 = new ModelRenderer(this, 27, 27); helmet1.addBox(0F, 0F, 0F, 1, 5, 9); helmet1.setRotationPoint(4F, -8F, -4F); helmet1.setTextureSize(64, 64); helmet1.mirror = true; setRotation(helmet1, 0F, 0F, 0F); this.bipedHead.addChild(helmet1); helmet4 = new ModelRenderer(this, 27, 27); helmet4.addBox(0F, 0F, 0F, 1, 5, 9); helmet4.setRotationPoint(-5F, -8F, -4F); helmet4.setTextureSize(64, 64); helmet4.mirror = true; setRotation(helmet4, 0F, 0F, 0F); this.bipedHead.addChild(helmet4); helmet2 = new ModelRenderer(this, 22, 52); helmet2.addBox(0F, 0F, 0F, 10, 2, 10); helmet2.setRotationPoint(-5F, -10F, -5F); helmet2.setTextureSize(64, 64); helmet2.mirror = true; setRotation(helmet2, 0F, 0F, 0F); this.bipedHead.addChild(helmet2); helmet5 = new ModelRenderer(this, 34, 0); helmet5.addBox(0F, 0F, 0F, 1, 2, 10); helmet5.setRotationPoint(-5F, -3F, -5F); helmet5.setTextureSize(64, 64); helmet5.mirror = true; setRotation(helmet5, 0F, 0F, 0F); this.bipedHead.addChild(helmet5); crystal7 = new ModelRenderer(this, 18, 19); crystal7.addBox(0F, 0F, 0F, 1, 1, 4); crystal7.setRotationPoint(3.5F, -11.5F, -7F); crystal7.setTextureSize(64, 64); crystal7.mirror = true; setRotation(crystal7, -0.7853982F, 0F, 0F); this.bipedHead.addChild(crystal7); helmet = new ModelRenderer(this, 34, 0); helmet.addBox(0F, 0F, 0F, 1, 2, 10); helmet.setRotationPoint(4F, -3F, -5F); helmet.setTextureSize(64, 64); helmet.mirror = true; setRotation(helmet, 0F, 0F, 0F); this.bipedHead.addChild(helmet); crystal3 = new ModelRenderer(this, 29, 19); crystal3.addBox(0F, 0F, 0F, 1, 4, 1); crystal3.setRotationPoint(3.5F, -14.8F, -7.7F); crystal3.setTextureSize(64, 64); crystal3.mirror = true; setRotation(crystal3, 0F, 0F, 0F); this.bipedHead.addChild(crystal3); crystal4 = new ModelRenderer(this, 18, 19); crystal4.addBox(0F, 0F, 0F, 1, 1, 4); crystal4.setRotationPoint(-4.5F, -11.5F, -7F); crystal4.setTextureSize(64, 64); crystal4.mirror = true; setRotation(crystal4, -0.7853982F, 0F, 0F); this.bipedHead.addChild(crystal4); helmet3 = new ModelRenderer(this, 22, 43); helmet3.addBox(0F, 0F, 0F, 8, 7, 1); helmet3.setRotationPoint(-4F, -8F, 4F); helmet3.setTextureSize(64, 64); helmet3.mirror = true; setRotation(helmet3, 0F, 0F, 0F); this.bipedHead.addChild(helmet3); Shape15 = new ModelRenderer(this, 28, 0); Shape15.addBox(0F, 0F, 0F, 1, 2, 1); Shape15.setRotationPoint(-4F, -3F, -5F); Shape15.setTextureSize(64, 64); Shape15.mirror = true; setRotation(Shape15, 0F, 0F, 0F); this.bipedHead.addChild(Shape15); crystal5 = new ModelRenderer(this, 29, 19); crystal5.addBox(0F, 0F, 0F, 1, 4, 1); crystal5.setRotationPoint(-4.5F, -14.8F, -7.7F); crystal5.setTextureSize(64, 64); crystal5.mirror = true; setRotation(crystal5, 0F, 0F, 0F); this.bipedHead.addChild(crystal5); boots3 = new ModelRenderer(this, 0, 0); boots3.addBox(0F, 0F, 0F, 6, 4, 6); boots3.setRotationPoint(0F, 20F, -3F); boots3.setTextureSize(64, 64); boots3.mirror = true; setRotation(boots3, 0F, 0F, 0F); this.bipedLeftLeg.addChild(boots3); leg1 = new ModelRenderer(this, 0, 0); leg1.addBox(-2.5F, 0F, -2.5F, 5, 8, 5); leg1.setRotationPoint(2.5F, 12F, 0F); leg1.setTextureSize(64, 64); leg1.mirror = true; setRotation(leg1, 0F, 0F, 0F); this.bipedLeftLeg.addChild(leg1); boots2 = new ModelRenderer(this, 0, 7); boots2.addBox(-3F, 0F, -3F, 6, 2, 3); boots2.setRotationPoint(3F, 22F, -3F); boots2.setTextureSize(64, 64); boots2.mirror = true; setRotation(boots2, 0F, 0F, 0F); this.bipedLeftLeg.addChild(boots2); frontpiece1 = new ModelRenderer(this, 28, 0); frontpiece1.addBox(0F, 0F, 0F, 1, 2, 1); frontpiece1.setRotationPoint(3F, -3F, -5F); frontpiece1.setTextureSize(64, 64); frontpiece1.mirror = true; setRotation(frontpiece1, 0F, 0F, 0F); this.bipedHead.addChild(frontpiece1); boots1 = new ModelRenderer(this, 0, 0); boots1.addBox(0F, 0F, 0F, 6, 4, 6); boots1.setRotationPoint(-6F, 20F, -3F); boots1.setTextureSize(64, 64); boots1.mirror = true; setRotation(boots1, 0F, 0F, 0F); this.bipedRightLeg.addChild(boots1); boots = new ModelRenderer(this, 0, 7); boots.addBox(-3F, 0F, -3F, 6, 2, 3); boots.setRotationPoint(-3F, 22F, -3F); boots.setTextureSize(64, 64); boots.mirror = true; setRotation(boots, 0F, 0F, 0F); this.bipedRightLeg.addChild(boots); leg = new ModelRenderer(this, 0, 0); leg.addBox(-2.5F, 0F, -2.5F, 5, 8, 5); leg.setRotationPoint(-2.5F, 12F, 0F); leg.setTextureSize(64, 64); leg.mirror = true; setRotation(leg, 0F, 0F, 0F); this.bipedRightLeg.addChild(leg); tube = new ModelRenderer(this, 12, 26); tube.addBox(0F, 0F, 0F, 1, 6, 1); tube.setRotationPoint(-1F, 4F, -2.8F); tube.setTextureSize(64, 64); tube.mirror = true; setRotation(tube, 0F, 0F, 0.2617994F); this.bipedBody.addChild(tube); shouldercap1 = new ModelRenderer(this, 0, 40); shouldercap1.addBox(0F, 0F, 0F, 2, 1, 2); shouldercap1.setRotationPoint(6F, -1.5F, -1F); shouldercap1.setTextureSize(64, 64); shouldercap1.mirror = true; setRotation(shouldercap1, 0F, 0F, 0F); this.bipedLeftArm.addChild(shouldercap1); crystal6 = new ModelRenderer(this, 0, 27); crystal6.addBox(0F, 0F, 0F, 1, 3, 1); crystal6.setRotationPoint(10.5F, -3.8F, -2F); crystal6.setTextureSize(64, 64); crystal6.mirror = true; setRotation(crystal6, 0F, 0F, 0F); this.bipedLeftArm.addChild(crystal6); crysta7 = new ModelRenderer(this, 0, 27); crysta7.addBox(-1F, 0F, 0F, 1, 4, 1); crysta7.setRotationPoint(-10.8F, -1.5F, -2F); crysta7.setTextureSize(64, 64); crysta7.mirror = true; setRotation(crysta7, 0F, 0F, -0.7853982F); this.bipedRightArm.addChild(crysta7); crystal8 = new ModelRenderer(this, 0, 27); crystal8.addBox(-1F, 0F, 0F, 1, 4, 1); crystal8.setRotationPoint(-10.8F, -1.5F, 1F); crystal8.setTextureSize(64, 64); crystal8.mirror = true; setRotation(crystal8, 0F, 0F, -0.7853982F); this.bipedRightArm.addChild(crystal8); crystal9 = new ModelRenderer(this, 0, 27); crystal9.addBox(-1F, 0F, 0F, 1, 3, 1); crystal9.setRotationPoint(-10.5F, -3.8F, -2F); crystal9.setTextureSize(64, 64); crystal9.mirror = true; setRotation(crystal9, 0F, 0F, 0F); this.bipedRightArm.addChild(crystal9); crystal10 = new ModelRenderer(this, 0, 27); crystal10.addBox(0F, 0F, 0F, 1, 4, 1); //crystal10.setRotationPoint(10.8F, -1.5F, -2F); crystal10.setRotationPoint(0F, 0F, 0F); crystal10.setTextureSize(64, 64); crystal10.mirror = true; setRotation(crystal10, 0F, 0F, 0.7853982F); //this.bipedLeftArm.addChild(crystal10); frontpiece2 = new ModelRenderer(this, 34, 55); frontpiece2.addBox(-1F, 0F, 0F, 2, 4, 1); frontpiece2.setRotationPoint(0F, -9F, -5F); frontpiece2.setTextureSize(64, 64); frontpiece2.mirror = true; setRotation(frontpiece2, -0.2268928F, 0F, 0F); this.bipedHead.addChild(frontpiece2); crystal11 = new ModelRenderer(this, 0, 27); crystal11.addBox(-1F, 0F, 0F, 1, 3, 1); crystal11.setRotationPoint(-10.5F, -3.8F, 1F); crystal11.setTextureSize(64, 64); crystal11.mirror = true; setRotation(crystal11, 0F, 0F, 0F); this.bipedRightArm.addChild(crystal11); crystal12 = new ModelRenderer(this, 0, 27); crystal12.addBox(0F, 0F, 0F, 1, 3, 1); crystal12.setRotationPoint(10.5F, -3.8F, 1F); crystal12.setTextureSize(64, 64); crystal12.mirror = true; setRotation(crystal12, 0F, 0F, 0F); this.bipedLeftArm.addChild(crystal12); jar4 = new ModelRenderer(this, 10, 39); jar4.addBox(0F, 0F, 0F, 2, 3, 2); jar4.setRotationPoint(-0.4F, 1F, 2.5F); jar4.setTextureSize(64, 64); jar4.mirror = true; setRotation(jar4, 0F, 0F, 1.047198F); this.bipedBody.addChild(jar4); shouldercap = new ModelRenderer(this, 0, 40); shouldercap.addBox(0F, 0F, 0F, 2, 1, 2); shouldercap.setRotationPoint(-8F, -1.5F, -1F); shouldercap.setTextureSize(64, 64); shouldercap.mirror = true; setRotation(shouldercap, 0F, 0F, 0F); this.bipedRightArm.addChild(shouldercap); jar = new ModelRenderer(this, 10, 39); jar.addBox(0F, 0F, 0F, 2, 3, 2); jar.setRotationPoint(-3F, 9F, -4.5F); jar.setTextureSize(64, 64); jar.mirror = true; setRotation(jar, 0F, 0F, 0F); this.bipedBody.addChild(jar); jar1 = new ModelRenderer(this, 10, 39); jar1.addBox(0F, 0F, 0F, 2, 3, 2); jar1.setRotationPoint(3F, 7F, 2.5F); jar1.setTextureSize(64, 64); jar1.mirror = true; setRotation(jar1, 0F, 0F, 1.047198F); this.bipedBody.addChild(jar1); jar2 = new ModelRenderer(this, 10, 39); jar2.addBox(0F, 0F, 0F, 2, 3, 2); jar2.setRotationPoint(1.9F, 5F, 2.5F); jar2.setTextureSize(64, 64); jar2.mirror = true; setRotation(jar2, 0F, 0F, 1.047198F); this.bipedBody.addChild(jar2); jar3 = new ModelRenderer(this, 10, 39); jar3.addBox(0F, 0F, 0F, 2, 3, 2); jar3.setRotationPoint(0.8F, 3F, 2.5F); jar3.setTextureSize(64, 64); jar3.mirror = true; setRotation(jar3, 0F, 0F, 1.047198F); this.bipedBody.addChild(jar3); lid = new ModelRenderer(this, 0, 20); lid.addBox(0F, 0F, 0F, 1, 1, 1); lid.setRotationPoint(-2.5F, 8.5F, -4F); lid.setTextureSize(64, 64); lid.mirror = true; setRotation(lid, 0F, 0F, 0F); this.bipedBody.addChild(lid); lid1 = new ModelRenderer(this, 0, 20); lid1.addBox(0F, 1F, 0F, 1, 1, 1); lid1.setRotationPoint(4.5F, 6.5F, 3F); lid1.setTextureSize(64, 64); lid1.mirror = true; setRotation(lid1, 0F, 0F, 1.047198F); this.bipedBody.addChild(lid1); lid2 = new ModelRenderer(this, 0, 20); lid2.addBox(0F, 1F, 0F, 1, 1, 1); lid2.setRotationPoint(3.5F, 4.5F, 3F); lid2.setTextureSize(64, 64); lid2.mirror = true; setRotation(lid2, 0F, 0F, 1.047198F); this.bipedBody.addChild(lid2); lid3 = new ModelRenderer(this, 0, 20); lid3.addBox(0F, 1F, 0F, 1, 1, 1); lid3.setRotationPoint(2.5F, 2.7F, 3F); lid3.setTextureSize(64, 64); lid3.mirror = true; setRotation(lid3, 0F, 0F, 1.047198F); this.bipedBody.addChild(lid3); } public void render(Entity entity, float f, float f1, float f2, float f3, float f4, float f5) { //super.render(entity, f, f1, f2, f3, f4, f5); super.setRotationAngles(f, f1, f2, f3, f4, f5, entity); belt.render(f5); crystal.render(f5); star3.render(f5); star2.render(f5); star4.render(f5); star5.render(f5); handleft.render(f5); torso.render(f5); lid4.render(f5); handright.render(f5); star.render(f5); star1.render(f5); button.render(f5); frontpiece4.render(f5); frontpiece3.render(f5); helmet1.render(f5); helmet4.render(f5); helmet2.render(f5); helmet5.render(f5); crystal7.render(f5); helmet.render(f5); crystal3.render(f5); crystal4.render(f5); helmet3.render(f5); Shape15.render(f5); crystal5.render(f5); boots3.render(f5); leg1.render(f5); boots2.render(f5); frontpiece1.render(f5); boots1.render(f5); boots.render(f5); leg.render(f5); tube.render(f5); shouldercap1.render(f5); crystal6.render(f5); crysta7.render(f5); crystal8.render(f5); crystal9.render(f5); crystal10.render(f5); frontpiece2.render(f5); crystal11.render(f5); crystal12.render(f5); jar4.render(f5); shouldercap.render(f5); jar.render(f5); jar1.render(f5); jar2.render(f5); jar3.render(f5); lid.render(f5); lid1.render(f5); lid2.render(f5); lid3.render(f5); } private void setRotation(ModelRenderer model, float x, float y, float z) { model.rotateAngleX = x; model.rotateAngleY = y; model.rotateAngleZ = z; } }
-
I don't think I was specific enough. I want to make a new potion effect; I know how to add it to the item, but I have no idea of how to actually make the effect (if that makes sense).
-
I am trying to create a new potion, but I haven't been able to find any relevant information besides a tutorial with very little information. Could anyone explain to me the steps of adding in a new potion with a brewing recipe?
-
I've updated the OP with the other two files.
-
I have created a custom mob, but for some reason the default ModelBiped animations (walking, arms swinging, etc) are not displaying. Here are the appropriate files:
package timeTraveler.render; import static net.minecraftforge.client.IItemRenderer.ItemRenderType.EQUIPPED; import static net.minecraftforge.client.IItemRenderer.ItemRendererHelper.BLOCK_3D; import net.minecraft.block.Block; import net.minecraft.client.Minecraft; import net.minecraft.client.entity.AbstractClientPlayer; import net.minecraft.client.model.ModelBiped; import net.minecraft.client.renderer.IImageBuffer; import net.minecraft.client.renderer.ImageBufferDownload; import net.minecraft.client.renderer.RenderBlocks; import net.minecraft.client.renderer.ThreadDownloadImageData; import net.minecraft.client.renderer.entity.RenderBiped; import net.minecraft.client.renderer.texture.TextureManager; import net.minecraft.client.renderer.texture.TextureObject; import net.minecraft.client.renderer.tileentity.TileEntitySkullRenderer; import net.minecraft.entity.Entity; import net.minecraft.entity.EntityLiving; import net.minecraft.entity.EntityLivingBase; import net.minecraft.item.EnumAction; import net.minecraft.item.Item; import net.minecraft.item.ItemBlock; import net.minecraft.item.ItemStack; import net.minecraft.util.ResourceLocation; import net.minecraft.util.StringUtils; import net.minecraftforge.client.IItemRenderer; import net.minecraftforge.client.MinecraftForgeClient; import org.lwjgl.opengl.GL11; import timeTraveler.entities.EntityPlayerPast; import cpw.mods.fml.relauncher.Side; import cpw.mods.fml.relauncher.SideOnly; @SideOnly(Side.CLIENT) public class RenderPastPlayer extends RenderBiped { public static final ResourceLocation locationStevePng = new ResourceLocation("textures/entity/steve.png"); public RenderPastPlayer(ModelBiped par1ModelBase, float par2) { super(par1ModelBase, par2); } //private static final ResourceLocation Your_Texture = new ResourceLocation("mocap","test.png"); //refers to:assets/yourmod/models/optionalfile/yourtexture.png /** * Called from doRenderLiving in RenderBiped. */ @Override protected void func_82420_a(EntityLiving par1EntityLiving, ItemStack par2ItemStack) { return; } @Override public void doRenderLiving(EntityLiving par1EntityLiving, double par2, double par4, double par6, float par8, float par9) { EntityPlayerPast par1EntityMocap = (EntityPlayerPast)par1EntityLiving; ItemStack itemstack = par1EntityLiving.getHeldItem(); this.field_82423_g.heldItemRight = this.field_82425_h.heldItemRight = this.modelBipedMain.heldItemRight = itemstack != null ? 1 : 0; if (itemstack != null && par1EntityMocap.isEating()) { EnumAction enumaction = itemstack.getItemUseAction(); if (enumaction == EnumAction.eat) { this.field_82423_g.heldItemRight = this.field_82425_h.heldItemRight = this.modelBipedMain.heldItemRight = 4; } if (enumaction == EnumAction.block) { this.field_82423_g.heldItemRight = this.field_82425_h.heldItemRight = this.modelBipedMain.heldItemRight = 3; } else if (enumaction == EnumAction.bow) { this.field_82423_g.aimedBow = this.field_82425_h.aimedBow = this.modelBipedMain.aimedBow = true; } } this.field_82423_g.isSneak = this.field_82425_h.isSneak = this.modelBipedMain.isSneak = par1EntityLiving.isSneaking(); super.doRenderLiving(par1EntityLiving, par2, par4, par6, par8, par9); } /** * Called from renderEquippedItems in RenderBiped. */ @Override protected void func_130005_c(EntityLiving par1EntityLiving, float par2) { EntityPlayerPast par1EntityMocap = (EntityPlayerPast)par1EntityLiving; ItemStack itemstack1 = par1EntityLiving.getHeldItem(); ItemStack helmetItem = par1EntityLiving.func_130225_q(3); float f1 = 1.0F; GL11.glColor3f(f1, f1, f1); /* * Render Skulls, etc. */ float f2; if (helmetItem != null) { GL11.glPushMatrix(); this.modelBipedMain.bipedHead.postRender(0.0625F); IItemRenderer customRenderer = MinecraftForgeClient.getItemRenderer(helmetItem, EQUIPPED); boolean is3D = (customRenderer != null && customRenderer.shouldUseRenderHelper(EQUIPPED, helmetItem, BLOCK_3D)); if (helmetItem.getItem() instanceof ItemBlock) { if (is3D || RenderBlocks.renderItemIn3d(Block.blocksList[helmetItem.itemID].getRenderType())) { f2 = 0.625F; GL11.glTranslatef(0.0F, -0.25F, 0.0F); GL11.glRotatef(90.0F, 0.0F, 1.0F, 0.0F); GL11.glScalef(f2, -f2, -f2); } this.renderManager.itemRenderer.renderItem(par1EntityLiving, helmetItem, 0); } else if (helmetItem.getItem().itemID == Item.skull.itemID) { f2 = 1.0625F; GL11.glScalef(f2, -f2, -f2); String s = ""; if (helmetItem.hasTagCompound() && helmetItem.getTagCompound().hasKey("SkullOwner")) { s = helmetItem.getTagCompound().getString("SkullOwner"); } TileEntitySkullRenderer.skullRenderer.func_82393_a(-0.5F, 0.0F, -0.5F, 1, 180.0F, helmetItem.getItemDamage(), s); } GL11.glPopMatrix(); } if (itemstack1 != null) { GL11.glPushMatrix(); this.modelBipedMain.bipedRightArm.postRender(0.0625F); GL11.glTranslatef(-0.0625F, 0.4375F, 0.0625F); EnumAction enumaction = null; if (par1EntityMocap.isEating()) { enumaction = itemstack1.getItemUseAction(); } float f11; IItemRenderer customRenderer = MinecraftForgeClient.getItemRenderer(itemstack1, EQUIPPED); boolean is3D = (customRenderer != null && customRenderer.shouldUseRenderHelper(EQUIPPED, itemstack1, BLOCK_3D)); boolean isBlock = itemstack1.itemID < Block.blocksList.length && itemstack1.getItemSpriteNumber() == 0; if (is3D || (isBlock && RenderBlocks.renderItemIn3d(Block.blocksList[itemstack1.itemID].getRenderType()))) { f11 = 0.5F; GL11.glTranslatef(0.0F, 0.1875F, -0.3125F); f11 *= 0.75F; GL11.glRotatef(20.0F, 1.0F, 0.0F, 0.0F); GL11.glRotatef(45.0F, 0.0F, 1.0F, 0.0F); GL11.glScalef(-f11, -f11, f11); } else if (itemstack1.itemID == Item.bow.itemID) { f11 = 0.625F; GL11.glTranslatef(0.0F, 0.125F, 0.3125F); GL11.glRotatef(-20.0F, 0.0F, 1.0F, 0.0F); GL11.glScalef(f11, -f11, f11); GL11.glRotatef(-100.0F, 1.0F, 0.0F, 0.0F); GL11.glRotatef(45.0F, 0.0F, 1.0F, 0.0F); } else if (Item.itemsList[itemstack1.itemID].isFull3D()) { f11 = 0.625F; if (Item.itemsList[itemstack1.itemID].shouldRotateAroundWhenRendering()) { GL11.glRotatef(180.0F, 0.0F, 0.0F, 1.0F); GL11.glTranslatef(0.0F, -0.125F, 0.0F); } if (par1EntityMocap.isEating() && enumaction == EnumAction.block) { GL11.glTranslatef(0.05F, 0.0F, -0.1F); GL11.glRotatef(-50.0F, 0.0F, 1.0F, 0.0F); GL11.glRotatef(-10.0F, 1.0F, 0.0F, 0.0F); GL11.glRotatef(-60.0F, 0.0F, 0.0F, 1.0F); } GL11.glTranslatef(0.0F, 0.1875F, 0.0F); GL11.glScalef(f11, -f11, f11); GL11.glRotatef(-100.0F, 1.0F, 0.0F, 0.0F); GL11.glRotatef(45.0F, 0.0F, 1.0F, 0.0F); } else { f11 = 0.375F; GL11.glTranslatef(0.25F, 0.1875F, -0.1875F); GL11.glScalef(f11, f11, f11); GL11.glRotatef(60.0F, 0.0F, 0.0F, 1.0F); GL11.glRotatef(-90.0F, 1.0F, 0.0F, 0.0F); GL11.glRotatef(20.0F, 0.0F, 0.0F, 1.0F); } float f12; float f13; float f6; int j; if (itemstack1.getItem().requiresMultipleRenderPasses()) { for (j = 0; j < itemstack1.getItem().getRenderPasses(itemstack1.getItemDamage()); ++j) { int k = itemstack1.getItem().getColorFromItemStack(itemstack1, j); f13 = (float)(k >> 16 & 255) / 255.0F; f12 = (float)(k >> 8 & 255) / 255.0F; f6 = (float)(k & 255) / 255.0F; GL11.glColor4f(f13, f12, f6, 1.0F); this.renderManager.itemRenderer.renderItem(par1EntityLiving, itemstack1, j); } } else { j = itemstack1.getItem().getColorFromItemStack(itemstack1, 0); float f14 = (float)(j >> 16 & 255) / 255.0F; f13 = (float)(j >> 8 & 255) / 255.0F; f12 = (float)(j & 255) / 255.0F; GL11.glColor4f(f14, f13, f12, 1.0F); this.renderManager.itemRenderer.renderItem(par1EntityLiving, itemstack1, 0); } GL11.glPopMatrix(); } } //@Override protected void renderModelTest(EntityLivingBase par1EntityLivingBase, float par2, float par3, float par4, float par5, float par6, float par7) { this.bindEntityTexture(par1EntityLivingBase); GL11.glPushMatrix(); GL11.glColor4f(1.0F, 1.0F, 1.0F, 0.35F); GL11.glDepthMask(false); GL11.glEnable(GL11.GL_BLEND); GL11.glBlendFunc(GL11.GL_SRC_ALPHA, GL11.GL_ONE_MINUS_SRC_ALPHA); GL11.glAlphaFunc(GL11.GL_GREATER, 0.003921569F); this.mainModel.render(par1EntityLivingBase, par2, par3, par4, par5, par6, par7); GL11.glDisable(GL11.GL_BLEND); GL11.glAlphaFunc(GL11.GL_GREATER, 0.1F); GL11.glPopMatrix(); GL11.glDepthMask(true); } }
package timeTraveler.entities; import java.util.ArrayList; import java.util.Collections; import java.util.List; import java.util.Random; import net.minecraft.entity.Entity; import net.minecraft.entity.EntityLiving; import net.minecraft.entity.item.EntityItem; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.entity.projectile.EntityArrow; import net.minecraft.item.ItemBlock; import net.minecraft.item.ItemStack; import net.minecraft.server.MinecraftServer; import net.minecraft.util.ChatMessageComponent; import net.minecraft.util.MathHelper; import net.minecraft.util.Vec3; import net.minecraft.world.World; import timeTraveler.core.TimeTraveler; import timeTraveler.pasttravel.PastAction; import timeTraveler.pasttravel.PastActionTypes; import cpw.mods.fml.client.FMLClientHandler; import cpw.mods.fml.server.FMLServerHandler; public class EntityPlayerPast extends EntityLiving { /** * A list of pending actions the Entity has to perform, injected * by the replay thread. */ private int itemInUseCount = 0; public List<PastAction> eventsList = Collections.synchronizedList(new ArrayList<PastAction>()); public EntityPlayerPast(World par1World) { super(par1World); this.tasks.taskEntries.clear(); this.targetTasks.taskEntries.clear(); } public void onLivingUpdate() { if (eventsList.size() > 0) { PastAction ma = eventsList.remove(0); processActions(ma); } this.updateArmSwingProgress(); if (this.newPosRotationIncrements > 0) { double d0 = this.posX + (this.newPosX - this.posX) / (double) this.newPosRotationIncrements; double d1 = this.posY + (this.newPosY - this.posY) / (double) this.newPosRotationIncrements; double d2 = this.posZ + (this.newPosZ - this.posZ) / (double) this.newPosRotationIncrements; double d3 = MathHelper.wrapAngleTo180_double(this.newRotationYaw - (double) this.rotationYaw); this.rotationYaw = (float) ((double) this.rotationYaw + d3 / (double) this.newPosRotationIncrements); this.rotationPitch = (float) ((double) this.rotationPitch + (this.newRotationPitch - (double) this.rotationPitch) / (double) this.newPosRotationIncrements); --this.newPosRotationIncrements; this.setPosition(d0, d1, d2); this.setRotation(this.rotationYaw, this.rotationPitch); } else if (!this.isClientWorld()) { this.motionX *= 0.98D; this.motionY *= 0.98D; this.motionZ *= 0.98D; } if (Math.abs(this.motionX) < 0.005D) { this.motionX = 0.0D; } if (Math.abs(this.motionY) < 0.005D) { this.motionY = 0.0D; } if (Math.abs(this.motionZ) < 0.005D) { this.motionZ = 0.0D; } if (!this.isClientWorld()) { this.rotationYawHead = this.rotationYaw; } this.prevLimbSwingAmount = this.limbSwingAmount; double d0 = this.posX - this.prevPosX; double d1 = this.posZ - this.prevPosZ; float f6 = MathHelper.sqrt_double(d0 * d0 + d1 * d1) * 4.0F; if (f6 > 1.0F) { f6 = 1.0F; } this.limbSwingAmount += (f6 - this.limbSwingAmount) * 0.4F; this.limbSwing += this.limbSwingAmount; } public boolean interact(EntityPlayer par1EntityPlayer) { //System.out.println(worldObj.isRemote); //System.out.println(getSkinSource()); //System.out.println(posY); return true; } protected boolean isAIEnabled() { return false; } /* public void onEntityUpdate() { super.onEntityUpdate(); if(isPlayerSeen(FMLClientHandler.instance().getClient().thePlayer)) { System.out.println("PLAYER SPOTTED!"); int paradox = TimeTraveler.vars.getParadoxAmt(); paradox = paradox + 3; TimeTraveler.vars.setParadoxAmt(paradox); } }*/ }
package timeTraveler.proxies; import net.minecraft.client.model.ModelBiped; import net.minecraftforge.client.MinecraftForgeClient; import timeTraveler.core.TimeTraveler; import timeTraveler.entities.EntityParadoxHunter; import timeTraveler.entities.EntityPlayerPast; import timeTraveler.models.ModelParadoxHunter; import timeTraveler.render.ItemCondenserRenderer; import timeTraveler.render.ItemTimeMachineRenderer; import timeTraveler.render.RenderCondenser; import timeTraveler.render.RenderExtractor; import timeTraveler.render.RenderParadoxHunter; import timeTraveler.render.RenderPastPlayer; import timeTraveler.render.RenderTimeMachine; import timeTraveler.tileentity.TileEntityExtractor; import timeTraveler.tileentity.TileEntityParadoxCondenser; import timeTraveler.tileentity.TileEntityTimeTravel; import com.jadarstudios.developercapes.DevCapes; import cpw.mods.fml.client.registry.ClientRegistry; import cpw.mods.fml.client.registry.RenderingRegistry; public class ClientProxy extends CommonProxy { public void registerRenderThings() { ClientRegistry.bindTileEntitySpecialRenderer(TileEntityExtractor.class, new RenderExtractor()); ClientRegistry.bindTileEntitySpecialRenderer(TileEntityParadoxCondenser.class, new RenderCondenser()); ClientRegistry.bindTileEntitySpecialRenderer(TileEntityTimeTravel.class, new RenderTimeMachine()); RenderingRegistry.registerEntityRenderingHandler(EntityParadoxHunter.class, new RenderParadoxHunter(new ModelParadoxHunter(), 0.3F)); RenderingRegistry.registerEntityRenderingHandler(EntityPlayerPast.class, new RenderPastPlayer(new ModelBiped(), 0.3F)); MinecraftForgeClient.registerItemRenderer(TimeTraveler.paradoxCondenser.blockID, new ItemCondenserRenderer()); MinecraftForgeClient.registerItemRenderer(TimeTraveler.timeTravel.blockID, new ItemTimeMachineRenderer()); } @Override public void initCapes() { // move this to dropbox DevCapes.getInstance().registerConfig("https://raw2.github.com/jadar/TimeTraveler/master/capes/capes.json", TimeTraveler.modid); } }
package timeTraveler.core; import java.io.File; import java.util.ArrayList; import java.util.Collections; import java.util.HashMap; import java.util.LinkedList; import java.util.List; import java.util.Map; import net.minecraft.block.Block; import net.minecraft.block.material.Material; import net.minecraft.command.ICommandSender; import net.minecraft.command.PlayerSelector; import net.minecraft.entity.Entity; import net.minecraft.entity.EntityEggInfo; import net.minecraft.entity.EntityList; import net.minecraft.entity.EnumCreatureType; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.entity.player.EntityPlayerMP; import net.minecraft.item.Item; import net.minecraft.item.ItemStack; import net.minecraft.item.crafting.FurnaceRecipes; import net.minecraft.world.biome.BiomeGenBase; import net.minecraftforge.common.DimensionManager; import net.minecraftforge.common.MinecraftForge; import timeTraveler.blocks.BlockParadoxCondenser; import timeTraveler.blocks.BlockTime; import timeTraveler.blocks.BlockTimeTraveler; import timeTraveler.blocks.Collision; import timeTraveler.blocks.ParadoxExtractor; import timeTraveler.entities.EntityChair; import timeTraveler.entities.EntityParadoxHunter; import timeTraveler.entities.EntityPlayerPast; import timeTraveler.futuretravel.FutureTravelMechanics; import timeTraveler.futuretravel.WorldProviderFuture; import timeTraveler.gui.GuiHandler; import timeTraveler.items.BottledParadox; import timeTraveler.items.CondensedParadox; import timeTraveler.items.EmptyBottle; import timeTraveler.items.ItemExpEnhance; import timeTraveler.items.ItemFlashback; import timeTraveler.items.ItemParadoximer; import timeTraveler.mechanics.TTEventHandler; import timeTraveler.network.TimeTravelerPacketHandler; import timeTraveler.pasttravel.PastAction; import timeTraveler.pasttravel.TimeTravelerPastRecorder; import timeTraveler.pasttravel.TimeTravelerPlayerTracker; import timeTraveler.proxies.CommonProxy; import timeTraveler.structures.StructureGenerator; import timeTraveler.ticker.TickerClient; import timeTraveler.tileentity.TileEntityCollision; import timeTraveler.tileentity.TileEntityExtractor; import timeTraveler.tileentity.TileEntityParadoxCondenser; import timeTraveler.tileentity.TileEntityTimeTravel; import cpw.mods.fml.client.FMLClientHandler; import cpw.mods.fml.common.FMLCommonHandler; import cpw.mods.fml.common.Mod; import cpw.mods.fml.common.Mod.EventHandler; import cpw.mods.fml.common.Mod.Instance; import cpw.mods.fml.common.SidedProxy; import cpw.mods.fml.common.event.FMLInitializationEvent; import cpw.mods.fml.common.network.NetworkMod; import cpw.mods.fml.common.network.NetworkMod.SidedPacketHandler; import cpw.mods.fml.common.network.NetworkRegistry; import cpw.mods.fml.common.registry.EntityRegistry; import cpw.mods.fml.common.registry.GameRegistry; import cpw.mods.fml.common.registry.LanguageRegistry; import cpw.mods.fml.common.registry.TickRegistry; import cpw.mods.fml.relauncher.Side; @Mod(modid = TimeTraveler.modid, name = "Time Traveler", version = "0.1") @NetworkMod(clientSideRequired = true, serverSideRequired = false, serverPacketHandlerSpec = @SidedPacketHandler (channels = {"futuretravel", "paradoxgui", "entityspawn"}, packetHandler = TimeTravelerPacketHandler.class)) /** * Main launcher for TimeTraveler * @author Charsmud6 * */ public class TimeTraveler { @SidedProxy(clientSide = "timeTraveler.proxies.ClientProxy", serverSide = "timeTraveler.proxies.CommonProxy") public static CommonProxy proxy; @Instance public static TimeTraveler instance = new TimeTraveler(); /** * Initiates mod, registers block and item for use. Generates the necessary folders. */ @EventHandler public void load(FMLInitializationEvent event) { EntityRegistry.registerGlobalEntityID(EntityPlayerPast.class, "PlayerPast", EntityRegistry.findGlobalUniqueEntityId(), 0x191919, 0x000000);//registers the mobs name and id EntityRegistry.registerGlobalEntityID(EntityChair.class, "Chiar", EntityRegistry.findGlobalUniqueEntityId()); proxy.registerRenderThings(); } /** * Registers Entities */ public void registerEntities() { EntityRegistry.registerModEntity(EntityParadoxHunter.class, "ParadoxHunter", 1, this, 80, 1, true); EntityRegistry.registerModEntity(EntityPlayerPast.class, "PastPlayer", 2, this, 80, 1, true); EntityRegistry.addSpawn(EntityParadoxHunter.class, 10, 2, 4, EnumCreatureType.creature, BiomeGenBase.beach, BiomeGenBase.extremeHills, BiomeGenBase.extremeHillsEdge, BiomeGenBase.forest, BiomeGenBase.forestHills, BiomeGenBase.jungle, BiomeGenBase.jungleHills, BiomeGenBase.mushroomIsland, BiomeGenBase.mushroomIslandShore, BiomeGenBase.ocean, BiomeGenBase.plains, BiomeGenBase.river, BiomeGenBase.swampland); LanguageRegistry.instance().addStringLocalization("entity.Charsmud_TimeTraveler.ParadoxHunter.name", "Paradox Hunter"); registerEntityEgg(EntityParadoxHunter.class, 0xffffff, 0x000000); } }
Note that I have trimmed the files to contain only the necessary code. Any ideas?
-
Thanks, I'll look into that! I might end up making a dummy dimension so I can do the file manipulation, but if I can't I'll just create a dynamic dimension.
-
I've attempted to implement that, but the world files appear to still be in use. Here's the modified code block:
if(future.exists()) { try { System.out.println("THIS FUTURE EXISTS, MOVING THE FUTURE IN"); //mc.displayGuiScreen(null); MinecraftServer allServers = MinecraftServer.getServer(); for (int i = 0; i < allServers.worldServers.length; ++i) { if (allServers.worldServers[i] != null) { WorldServer wServer = allServers.worldServers[i]; wServer.canNotSave = true; System.out.println(wServer.canNotSave); } } //mc.statFileWriter.readStat(StatList.leaveGameStat, 1); //mc.theWorld.sendQuittingDisconnectingPacket(); //ms.stopServer(); // mc.loadWorld((WorldClient)null); Thread.sleep(3000); CopyFile.moveMultipleFiles(future, worldFile); for(int i = 0; i < allServers.worldServers.length; ++i) { if(allServers.worldServers[i] != null) { WorldServer wServer = allServers.worldServers[i]; wServer.canNotSave = false; } } //FutureTravelMechanics.launchWorld(folderName, worldName, (WorldSettings)null); //ms.startServerThread(); //mc.launchIntegratedServer(folderName, worldName, (WorldSettings) null); } catch(Exception ex) { ex.printStackTrace(); } }
What I'm trying to achieve is load in a version of the world file that I had previously modified. However, because the world files are in use, they cannot be moved unless minecraft is not using them. Is it possible that Minecraft is still reading from the world file, and thus why the files aren't moving?
-
What I want the server-side to do is to exit the world, then reload it after I move around files in the world folder.
-
Thanks for the input! I also was thinking of doing this as well, but wanted to check here to make sure I'm not just over-looking something.
-
I am attempting to run mc.launchIntegratedServer, but I keep getting the following error:
2014-07-10 13:48:18 [iNFO] [sTDERR] java.lang.RuntimeException: No OpenGL context found in the current thread. 2014-07-10 13:48:18 [iNFO] [sTDERR] at org.lwjgl.opengl.GLContext.getCapabilities(GLContext.java:124) 2014-07-10 13:48:18 [iNFO] [sTDERR] at org.lwjgl.opengl.GL11.glClear(GL11.java:585) 2014-07-10 13:48:18 [iNFO] [sTDERR] at net.minecraft.client.gui.LoadingScreenRenderer.setLoadingProgress(LoadingScreenRenderer.java:81) 2014-07-10 13:48:18 [iNFO] [sTDERR] at net.minecraft.client.gui.LoadingScreenRenderer.resetProgresAndWorkingMessage(LoadingScreenRenderer.java:62) 2014-07-10 13:48:18 [iNFO] [sTDERR] at net.minecraft.client.Minecraft.loadWorld(Minecraft.java:2097) 2014-07-10 13:48:18 [iNFO] [sTDERR] at net.minecraft.client.Minecraft.loadWorld(Minecraft.java:2063) 2014-07-10 13:48:18 [iNFO] [sTDERR] at net.minecraft.client.Minecraft.launchIntegratedServer(Minecraft.java:1981) 2014-07-10 13:48:18 [iNFO] [sTDERR] at timeTraveler.mechanics.FutureTravelMechanics.launchWorld(FutureTravelMechanics.java:287) 2014-07-10 13:48:18 [iNFO] [sTDERR] at timeTraveler.network.TimeTravelerPacketHandler.onPacketData(TimeTravelerPacketHandler.java:88) 2014-07-10 13:48:18 [iNFO] [sTDERR] at cpw.mods.fml.common.network.NetworkRegistry.handlePacket(NetworkRegistry.java:255) 2014-07-10 13:48:18 [iNFO] [sTDERR] at cpw.mods.fml.common.network.NetworkRegistry.handleCustomPacket(NetworkRegistry.java:245) 2014-07-10 13:48:18 [iNFO] [sTDERR] at cpw.mods.fml.common.network.FMLNetworkHandler.handlePacket250Packet(FMLNetworkHandler.java:85) 2014-07-10 13:48:18 [iNFO] [sTDERR] at net.minecraft.network.NetServerHandler.handleCustomPayload(NetServerHandler.java:1130) 2014-07-10 13:48:18 [iNFO] [sTDERR] at net.minecraft.network.packet.Packet250CustomPayload.processPacket(Packet250CustomPayload.java:61) 2014-07-10 13:48:18 [iNFO] [sTDERR] at net.minecraft.network.MemoryConnection.processReadPackets(MemoryConnection.java:89) 2014-07-10 13:48:18 [iNFO] [sTDERR] at net.minecraft.network.NetServerHandler.networkTick(NetServerHandler.java:141) 2014-07-10 13:48:18 [iNFO] [sTDERR] at net.minecraft.network.NetworkListenThread.networkTick(NetworkListenThread.java:54) 2014-07-10 13:48:18 [iNFO] [sTDERR] at net.minecraft.server.integrated.IntegratedServerListenThread.networkTick(IntegratedServerListenThread.java:109) 2014-07-10 13:48:18 [iNFO] [sTDERR] at net.minecraft.server.MinecraftServer.updateTimeLightAndEntities(MinecraftServer.java:691) 2014-07-10 13:48:18 [iNFO] [sTDERR] at net.minecraft.server.MinecraftServer.tick(MinecraftServer.java:587) 2014-07-10 13:48:18 [iNFO] [sTDERR] at net.minecraft.server.integrated.IntegratedServer.tick(IntegratedServer.java:129) 2014-07-10 13:48:18 [iNFO] [sTDERR] at net.minecraft.server.MinecraftServer.run(MinecraftServer.java:484) 2014-07-10 13:48:18 [iNFO] [sTDERR] at net.minecraft.server.ThreadMinecraftServer.run(ThreadMinecraftServer.java:583)
This is the affected method:
public static void launchWorld(String folderName, String worldName, WorldSettings ws) { if(FMLClientHandler.instance().getClient().isSingleplayer()) { FMLClientHandler.instance().getClient().launchIntegratedServer(folderName, worldName, ws); } }
And here is where I am calling this method:
if(future.exists()) { try { System.out.println("THIS FUTURE EXISTS, MOVING THE FUTURE IN"); mc.displayGuiScreen(null); mc.statFileWriter.readStat(StatList.leaveGameStat, 1); mc.theWorld.sendQuittingDisconnectingPacket(); ms.stopServer(); // mc.loadWorld((WorldClient)null); Thread.sleep(3000); CopyFile.moveMultipleFiles(future, worldFile); FutureTravelMechanics.launchWorld(folderName, worldName, (WorldSettings)null); //ms.startServerThread(); //mc.launchIntegratedServer(folderName, worldName, (WorldSettings) null); } catch(Exception ex) { ex.printStackTrace(); } }
This is inside of a class that implements IPacketHandler. I'm guessing that this error is occuring because this is run on the server, which does not have an OpenGL instance. However, this code needs to run serverside; any suggestions?
-
Thanks for that great explanation! I should hopefully be able to get that implemented sometime soon!
-
That assumption won't work for me, but I'll look into the EntitySenses and canEntityBeSeen methods.
After experimentation, I've discovered that canEntityBeSeen does not use the direction that the entity is facing: It just raytraces from the entity to the target entity, and assumes it is seen. Is there a way to modify this to get it to work for my needs?
-
Hello. I am trying to detect whether a player is visible by an entity; I have fiddled with the Enderman code but to no avail, as that code only detects if the enderman is seen by the player. Is there a way to detect this?
-
Read up on it, and I managed to get it to work.
-
Thanks, I've managed to get a basic coremod that recognizes the class and method working. However, I cannot seem to figure out how to get the ASM part working. Here is my ClassTransformer:
package timeTraveler.core; import static org.objectweb.asm.Opcodes.FDIV; import static org.objectweb.asm.Opcodes.INVOKEVIRTUAL; import static org.objectweb.asm.tree.AbstractInsnNode.METHOD_INSN; import java.util.Iterator; import net.minecraft.launchwrapper.IClassTransformer; import org.objectweb.asm.ClassReader; import org.objectweb.asm.ClassWriter; import org.objectweb.asm.Label; import org.objectweb.asm.MethodVisitor; import org.objectweb.asm.tree.AbstractInsnNode; import org.objectweb.asm.tree.ClassNode; import org.objectweb.asm.tree.InsnList; import org.objectweb.asm.tree.MethodInsnNode; import org.objectweb.asm.tree.MethodNode; public class TTClassTransformer implements IClassTransformer { @Override public byte[] transform(String arg0, String arg1, byte[] arg2) { //System.out.println(arg0); if (arg0.equals("aqz")) { System.out.println("********* INSIDE OBFUSCATED BLOCK TRANSFORMER ABOUT TO PATCH: " + arg0); return patchClassASM(arg0, arg2, true); } if (arg0.equals("net.minecraft.block.Block")) { System.out.println("********* INSIDE BLOCK TRANSFORMER ABOUT TO PATCH: " + arg0); return patchClassASM(arg0, arg2, false); } return arg2; } public byte[] patchClassASM(String name, byte[] bytes, boolean obfuscated) { String targetMethodName = ""; if(obfuscated == true) { targetMethodName ="a"; System.out.println("******** Using obfuscated method"); } else { targetMethodName ="onBlockPlaced"; System.out.println("******** Using unobfuscated method"); } //set up ASM class manipulation stuff. Consult the ASM docs for details ClassNode classNode = new ClassNode(); ClassReader classReader = new ClassReader(bytes); classReader.accept(classNode, 0); //Now we loop over all of the methods declared inside the Block class until we get to the targetMethodName "onBlockPlaced" // find method to inject into @SuppressWarnings("unchecked") Iterator<MethodNode> methods = classNode.methods.iterator(); while(methods.hasNext()) { MethodNode m = methods.next(); System.out.println("********* Method Name: "+m.name + " Desc:" + m.desc); int fdiv_index = -1; //Check if this is onBlockPlaced and it's method signature is (Lnet/minecraft/world/World;IIIIFFFI)I if ((m.name.equals(targetMethodName) && m.desc.equals("(Lnet/minecraft/world/World;IIIIFFFI)I"))) { System.out.println("********* Inside target method!"); // find interesting instructions in method, there is a single FDIV instruction we use as target //System.out.println("m.instructions.size = " + m.instructions.size()); InsnList toInject = new InsnList(); //TODO: NEED TO CONVERT THIS TO JAVA CODE TO INJECT //LINENUMBER 87 L0 //NEW timeTraveler/mechanics/BlockPlaceEvent //DUP //ALOAD 0 //ILOAD 2 //ILOAD 3 //ILOAD 4 //INVOKESPECIAL timeTraveler/mechanics/BlockPlaceEvent.<init> (Lnet/minecraft/block/Block;III)V //ASTORE 5 //L1 //LINENUMBER 88 L1 //GETSTATIC net/minecraftforge/common/MinecraftForge.EVENT_BUS : Lnet/minecraftforge/event/EventBus; //ALOAD 5 //INVOKEVIRTUAL net/minecraftforge/event/EventBus.post (Lnet/minecraftforge/event/Event;)Z //POP //L2 /* //To add new instructions, such as calling a static method can be done like so: // make new instruction list InsnList toInject = new InsnList(); toInject.add(new VarInsnNode(ALOAD, 0)); toInject.add(new MethodInsnNode(INVOKESTATIC, "mod/culegooner/MyStaticClass", "myStaticMethod", "()V")); // inject new instruction list into method instruction list m.instructions.insert(targetNode, toInject); */ AbstractInsnNode currentNode = null; AbstractInsnNode targetNode = null; @SuppressWarnings("unchecked") Iterator<AbstractInsnNode> iter = m.instructions.iterator(); int index = -1; //Loop over the instruction set and find the instruction FDIV which does the division of 1/explosionSize while (iter.hasNext()) { index++; currentNode = iter.next(); System.out.println("********* index : " + index + " currentNode.getOpcode() = " + currentNode.getOpcode()); //Found it! save the index location of instruction FDIV and the node for this instruction if (currentNode.getOpcode() == FDIV) { targetNode = currentNode; fdiv_index = index; } } System.out.println("********* fdiv_index should be 336 -> " + fdiv_index); if (targetNode == null) { System.out.println("Did not find all necessary target nodes! ABANDON CLASS!"); return bytes; } if (fdiv_index == -1) { System.out.println("Did not find all necessary target nodes! ABANDON CLASS!"); return bytes; } /* //now we want the save nods that load the variable explosionSize and the division instruction: The line in Explosion.java that we want to modify is: var25.dropBlockAsItemWithChance(this.worldObj, var4, var5, var6, this.worldObj.getBlockMetadata(var4, var5, var6), 1.0F / this.explosionSize, 0); The code we are looking for is the following in bytecode: mv.visitInsn(FCONST_1); mv.visitVarInsn(ALOAD, 0); mv.visitFieldInsn(GETFIELD, "net/minecraft/src/Explosion", "explosionSize", "F"); mv.visitInsn(FDIV); mv.visitInsn(ICONST_0); mv.visitMethodInsn(INVOKEVIRTUAL, "net/minecraft/src/Block", "dropBlockAsItemWithChance", "(Lnet/minecraft/src/World;IIIIFI)V"); */ AbstractInsnNode remNode1 = m.instructions.get(fdiv_index-2); // mv.visitVarInsn(ALOAD, 0); AbstractInsnNode remNode2 = m.instructions.get(fdiv_index-1); // mv.visitFieldInsn(GETFIELD, "net/minecraft/src/Explosion", "explosionSize", "F"); AbstractInsnNode remNode3 = m.instructions.get(fdiv_index); // mv.visitInsn(FDIV); //This part is just to show how if the opcode we are looking for is an invokevirtual AbstractInsnNode invVirt = m.instructions.get(fdiv_index+2); if(invVirt.getOpcode() == INVOKEVIRTUAL) { if(invVirt.getType() == METHOD_INSN){ System.out.println("INVOKEVIRTUAL opcode is " + invVirt.getOpcode() + " METHOD_INSN type is " + invVirt.getType() ); MethodInsnNode testMethod = (MethodInsnNode)invVirt; //only do this cast if the getType match to a MethodInsnNode!! look at the javadoc for the other types! System.out.println("INVOKEVIRTUAL :" + testMethod.owner + " , " + testMethod.name + " , " + testMethod.desc ); } } //just remove these nodes from the instruction set, this will prevent the instruction FCONST_1 to be divided. m.instructions.remove(remNode1); m.instructions.remove(remNode2); m.instructions.remove(remNode3); //in this commented section, i'll just illustrate how to inject a call to a static method if your instruction is a little more advanced than just removing a couple of instruction: /* //To add new instructions, such as calling a static method can be done like so: // make new instruction list InsnList toInject = new InsnList(); toInject.add(new VarInsnNode(ALOAD, 0)); toInject.add(new MethodInsnNode(INVOKESTATIC, "mod/culegooner/MyStaticClass", "myStaticMethod", "()V")); // inject new instruction list into method instruction list m.instructions.insert(targetNode, toInject); */ System.out.println("Patching Complete!"); break; } } //ASM specific for cleaning up and returning the final bytes for JVM processing. ClassWriter writer = new ClassWriter(ClassWriter.COMPUTE_MAXS | ClassWriter.COMPUTE_FRAMES); classNode.accept(writer); return writer.toByteArray(); } }
Any ideas? (Note: I'm following a tutorial, but it doesn't explain the actual ASM part very well).
-
Hello. I am looking into using a coremod to inject code into a class. After digging around a bit, I saw the Tweak class under the cpw package. I have a few questions: What exactly does the cpw Tweak class do, and is it a possible way for me to inject code into a class using something in Forge (possibly the tweak class, if that is what it does)?
-
@GotoLink, that seems to be the thing I want to do: However, I cannot seem to find a method or variable that I can use to check if the xp orb is colliding with the player or not.
-
I looked at those methods, and I didn't see anything that would help. I need to essentially change the amount of xp an EntityXPOrb gives you based on certain conditions: any ideas?
-
Nothing is printed when I pick up an XP orb.
-
Hello! I am trying to do something when the player picks up an XP orb. I am using the following Event code for detecting XP orbs:
@ForgeSubscribe public void onPlayerEntityInteract(EntityItemPickupEvent event) { System.out.println(""); if(event.entity instanceof EntityXPOrb) { System.out.println(" :D"); int xpAmt = ((EntityXPOrb)event.entity).getXpValue(); int amtOfEnhance = 0; if(event.entityPlayer.inventory.hasItem(TimeTraveler.expEnhance.itemID)) { ItemStack[] items = event.entityPlayer.inventory.mainInventory; for(int i = 0; i < 35; i++) { if(items[i].getItem() instanceof ItemExpEnhance) { amtOfEnhance++; } } } System.out.println(amtOfEnhance); int newXpAmt = (amtOfEnhance * 2) * (xpAmt); event.entityPlayer.experience = event.entityPlayer.experience + newXpAmt; } } }
however, "
:D" never prints and amtOfEnhance does not print as well. Any idea?
-
I'm using the UUID to identify each mob individually, otherwise you have eight Zombies but no idea which is which. I do realize that EntityIDs and UUIDs are different things. I want to despawn all entities and prevent any from existing unless it has the specific UUID (which is why i need to set the UUID; The EntityHandler uses the UUID and MobType to determine whether or not to despawn the entity) that is contained in my data storage.
-
I need to change it because I need to identify a mob based off of it. I need to do this so I can only let certain mobs spawn, IE despawn all mobs and then spawn certain ones with certain UUIDs and EntityName so I can track each individual one.
Slowing the Speed of an Entity
in Modder Support
Posted
Adding a potion effect will not work for me, as I need this to affect ALL entities, not just living entities.