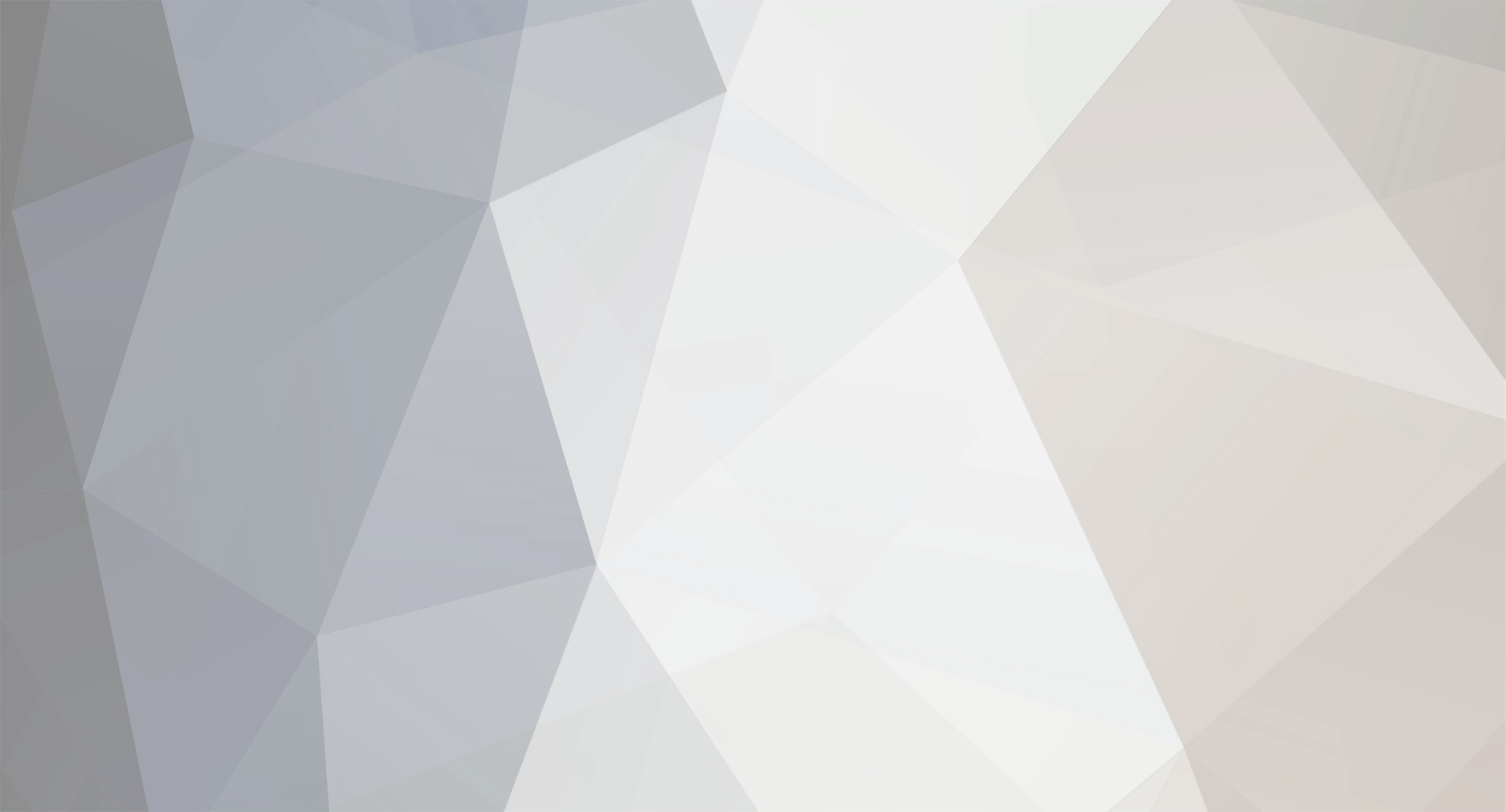
charsmud
-
Posts
89 -
Joined
-
Last visited
Posts posted by charsmud
-
-
Hello! I was messing around with 1.6.2 when I realized that mc.getMinecraftDir() is missing? What do I do now to get the directory?
I'm rather used to mc.getMinecraftDir, so that's all that I really know.
-
I tried iterating the world.loadedEntityList with this world var:
World world = mc.theWorld;
However, this did not actually despawn anything (probably because it's client side). Any ideas?
-
Hello! I need to do a few things:
1. Despawn all EntityLivings in the world.
2. Prevent any new EntityLivings from spawning in the world.
2a. Only allow entities to spawn if I instruct them to.
I have no idea where to start with this. I have dug around in the World code and the entity code, but to no avail. Any ideas?
-
It has to have some sort of modular expansion, as the villages are modular. I need to access whatever is used to modulate the village.
-
I don't think that's what I need.... I was thinking of just saving the mapping or whatever villages use when they generate for the modulation and then just referencing that whenever I need it, but I'm not entirely sure that this is something that you can do without editing base classes......
I might be able to do it with reflection whenever a village generates but I'm not entirely sure if this would work.
-
Hello! Is there a way that I can access a Village structure so I can create new buildings? I DO NOT mean adding new modded buildings to a village: I want to be able to essentially "expand" villages that have been generated. I've looked through the events, but I couldn't find one that pertains to my situation. Any ideas?
-
Figured it out!
After some experimentation, I figured out that this line was controlling the distance from the center:
GL11.glTranslated(0, 3, 0);//noted 2
I changed i to 0, -1, 0 and it was right above the head!
Thanks very much for all the help!
-
Doing that increased the radius that it oribits.
-
Hm, still didn't work.
I noticed that the drawing code is for the image laying down: what if it's changed so that it renders with the image facing forward?
-
I am translating then rotating. Here's the rotating + translating code:
GL11.glTranslated(diffX, diffY + 3, diffZ); //GL11.glTranslated(0, 3, 0);//move the whole thing 0.5 unit in the air GL11.glRotatef(-90, 1, 0, 0);//rotate around the y axis by 90 degree GL11.glRotatef(-player.rotationYaw, 0.0F, 0.0F, 1.0F);
And here's the whole file:
package village.mechanics; import net.minecraft.client.Minecraft; import net.minecraft.client.entity.EntityClientPlayerMP; import net.minecraft.client.renderer.Tessellator; import net.minecraft.client.renderer.entity.RenderManager; import net.minecraftforge.client.event.RenderLivingEvent; import org.lwjgl.opengl.GL11; /** * Contains information about the new Mechanics for the past * @author Charsmud * */ public class VillageMechanics { /** * Updates Paradox Bar and Renders * @param minecraft * @param amtOfParadox */ public void updateTradeBubble(Minecraft minecraft, RenderLivingEvent.Specials.Pre event) { System.out.println("EXECUTED FROM VILLAGEMECHANICS"); Tessellator tessellator = Tessellator.instance; EntityClientPlayerMP player = minecraft.thePlayer; double diffX = (event.entity.prevPosX + (event.entity.posX - event.entity.prevPosX)) - (player.prevPosX + (player.posX - player.prevPosX)); double diffY = (event.entity.prevPosY + (event.entity.posY - event.entity.prevPosY)) - (player.prevPosY + (player.posY - player.prevPosY)); double diffZ = (event.entity.prevPosZ + (event.entity.posZ - event.entity.prevPosZ)) - (player.prevPosZ + (player.posZ - player.prevPosZ)); GL11.glPushMatrix(); GL11.glTranslated(diffX, diffY + 3, diffZ); //GL11.glTranslated(0, 3, 0);//move the whole thing 0.5 unit in the air GL11.glRotatef(-90, 1, 0, 0);//rotate around the y axis by 90 degree GL11.glRotatef(-player.rotationYaw, 0.0F, 0.0F, 1.0F); minecraft.renderEngine.bindTexture("/village/images/bubble1.png"); tessellator.startDrawingQuads(); tessellator.addVertexWithUV(-1, 1, 1, 0, 0); tessellator.addVertexWithUV(-1, 1, -1, 0, 1); tessellator.addVertexWithUV(1, 1, -1, 1, 1); tessellator.addVertexWithUV(1, 1, 1, 1, 0); tessellator.addVertexWithUV(-1, 1, 1, 0, 0); tessellator.addVertexWithUV(1, 1, 1, 1, 0); tessellator.addVertexWithUV(1, 1, -1, 1, 1); tessellator.addVertexWithUV(-1, 1, -1, 0, 1); tessellator.draw(); GL11.glPopMatrix();//restore saved gl transform state } }
-
Sure. Here's a link to an imgur gallery:
Essentially, the image is rotating AROUND the villager and not around the center of the texture, so instead of spinning, it's going in a circle.
-
Thanks, that fixed the rotation problem! However, now the image rotates around the villager, instead of just spinning on it's center.... any idea how to fix that?
-
It worked, thanks! I just need to rotate the image 90 degrees on an axis, but I can figure that out.
Thanks for all the help! If I wanted to move the image up a bit, I would just edit the 2nd var in addVertexWithUV, right?
-
OK, so the image is now centered.
I changed the rotation to this:
GL11.glRotatef(-player.rotationYaw, 0.0F, 1.0F, 0.0F); GL11.glRotatef(player.rotationPitch, 1.0F, 0.0F, 0.0F);
And now it rotates wierdly.... I probably want cylindrical billboarding (that's just spinning in the center axis, right?
).
Off-Topic: let's not get into Direct3D vs OGL, that never ends well, and they both have their benefits.
-
Ok, I was able to translate it over to the Entity. However, I think my billboarding is messed up.... The texture is also not over the villager (I have NO idea how to fix this.
) The texture is still screwy like this as well:
package village.mechanics; import net.minecraft.client.Minecraft; import net.minecraft.client.entity.EntityClientPlayerMP; import net.minecraft.client.renderer.Tessellator; import net.minecraft.client.renderer.entity.RenderManager; import net.minecraftforge.client.event.RenderLivingEvent; import org.lwjgl.opengl.GL11; /** * Contains information about the new Mechanics for the past * @author Charsmud * */ public class VillageMechanics { /** * Updates Paradox Bar and Renders * @param minecraft * @param amtOfParadox */ public void updateTradeBubble(Minecraft minecraft, RenderLivingEvent.Specials.Pre event) { System.out.println("EXECUTED FROM VILLAGEMECHANICS"); Tessellator tessellator = Tessellator.instance; EntityClientPlayerMP player = minecraft.thePlayer; double diffX = (event.entity.prevPosX + (event.entity.posX - event.entity.prevPosX)) - (player.prevPosX + (player.posX - player.prevPosX)); double diffY = (event.entity.prevPosY + (event.entity.posY - event.entity.prevPosY)) - (player.prevPosY + (player.posY - player.prevPosY)); double diffZ = (event.entity.prevPosZ + (event.entity.posZ - event.entity.prevPosZ)) - (player.prevPosZ + (player.posZ - player.prevPosZ)); GL11.glPushMatrix();//save current gl transform state GL11.glTranslated(diffX, diffY, diffZ); GL11.glRotatef(-RenderManager.instance.playerViewY, 0.0F, 1.0F, 0.0F); GL11.glRotatef(RenderManager.instance.playerViewX, 1.0F, 0.0F, 0.0F); minecraft.renderEngine.bindTexture("/village/images/bubble1.png"); tessellator.startDrawingQuads(); tessellator.addVertexWithUV(1, 1, 0, 0, 0); tessellator.addVertexWithUV(1, 1, 0+2, 0, 1); tessellator.addVertexWithUV(1+1, 1+2, 0, 1, 1); tessellator.addVertexWithUV(1, 1+1, 0, 1, 0); tessellator.addVertexWithUV(1, 1, 0, 0, 0); tessellator.addVertexWithUV(1, 1+1, 0, 1, 0); tessellator.addVertexWithUV(1+1, 1+2, 0, 1, 1); tessellator.addVertexWithUV(1, 1, 0+2, 0, 1); tessellator.draw(); GL11.glPopMatrix();//restore saved gl transform state } }
OFF-TOPIC: I was going to learn OGL, but I decided with DirectX instead, as OGL was just a bunch of states that were enabled or disabled....
-
I replaced the RenderLivingEvent with RenderLivingEvent.Specials.Pre and it worked! However, it's over the player's head but not the villager (which I thought I prevented in my VillagerBubbleHandler):
package village.mechanics; import net.minecraft.entity.passive.EntityVillager; import net.minecraftforge.client.event.RenderLivingEvent; import net.minecraftforge.event.ForgeSubscribe; import cpw.mods.fml.client.FMLClientHandler; public class VillagerBubbleHandler { public VillagerBubbleHandler() { } @ForgeSubscribe public void onVillagerRender(RenderLivingEvent.Specials.Pre event ) { VillageMechanics mechanics = new VillageMechanics(); if(event.entity instanceof EntityVillager) { System.out.println("EXECUTED FROM VILLAGERBUBBLEHANDLER"); mechanics.updateTradeBubble(FMLClientHandler.instance().getClient()); } } }
Also, the image is distorted like this:
Any ideas?
-
I put prints in the @PreInit.
package village.core; import net.minecraftforge.client.event.RenderLivingEvent; import net.minecraftforge.common.MinecraftForge; import village.mechanics.VillagerBubbleHandler; import village.proxies.CommonProxy; import cpw.mods.fml.common.Mod; import cpw.mods.fml.common.Mod.Init; import cpw.mods.fml.common.Mod.Instance; import cpw.mods.fml.common.Mod.PreInit; import cpw.mods.fml.common.SidedProxy; import cpw.mods.fml.common.event.FMLInitializationEvent; import cpw.mods.fml.common.event.FMLPreInitializationEvent; import cpw.mods.fml.common.network.NetworkMod; import cpw.mods.fml.common.network.NetworkMod.SidedPacketHandler; import cpw.mods.fml.common.registry.TickRegistry; import cpw.mods.fml.relauncher.Side; @Mod(modid = "Charsmud_VillageUpgrades", name = "Village Upgrades", version = "1.0") @NetworkMod(clientSideRequired = true, serverSideRequired = false) /** * Main laucher for VillageUpgrades * @author Charsmud * */ public class VillageUpgrades { @SidedProxy(clientSide = "village.proxies.ClientProxy", serverSide = "village.proxies.CommonProxy") public static CommonProxy proxy; @Instance public static VillageUpgrades instance = new VillageUpgrades(); public static final String modid = "Charsmud_VillageUpgrades"; /** * Initiates mod, registers block and item for use. Generates the necessary folders. */ @PreInit public void preInit(FMLPreInitializationEvent event) { System.out.println("EXECUTED FRMO VILLAGEUPGRADES"); MinecraftForge.EVENT_BUS.register(new VillagerBubbleHandler()); System.out.println("EXECUTED FROM VILLAGEUPGRADES PRINT 2"); } @Init public void load(FMLInitializationEvent event) { proxy.registerRenderThings(); TickRegistry.registerTickHandler(new TickerClient(), Side.CLIENT); } }
Both print just fine. My CommonProxy and ClientProxy:
package village.proxies; public class CommonProxy { public void registerRenderThings() { } }
package village.proxies; public class ClientProxy extends CommonProxy { @Override public void registerRenderThings() { } }
I believe that it is initializing correctly, but I get this error on load, but the game still loads:
2013-08-07 10:41:39 [iNFO] [ForgeModLoader] Forge Mod Loader version 5.2.23.737 for Minecraft 1.5.2 loading 2013-08-07 10:41:39 [iNFO] [ForgeModLoader] Java is Java HotSpot(TM) 64-Bit Server VM, version 1.7.0_09, running on Windows 7:amd64:6.1, installed at C:\Users\Will\Desktop\eclipse-jee-juno-SR2-win32-x86_64\eclipse\jre 2013-08-07 10:41:39 [iNFO] [ForgeModLoader] Managed to load a deobfuscated Minecraft name- we are in a deobfuscated environment. Skipping runtime deobfuscation 2013-08-07 10:41:42 [iNFO] [sTDOUT] 229 recipes 2013-08-07 10:41:42 [iNFO] [sTDOUT] 27 achievements 2013-08-07 10:41:42 [iNFO] [Minecraft-Client] Setting user: Player659 2013-08-07 10:41:42 [iNFO] [sTDOUT] (Session ID is -) 2013-08-07 10:41:42 [iNFO] [sTDERR] Client asked for parameter: server 2013-08-07 10:41:42 [iNFO] [Minecraft-Client] LWJGL Version: 2.4.2 2013-08-07 10:41:43 [iNFO] [MinecraftForge] Attempting early MinecraftForge initialization 2013-08-07 10:41:43 [iNFO] [sTDOUT] MinecraftForge v7.8.1.737 Initialized 2013-08-07 10:41:43 [iNFO] [ForgeModLoader] MinecraftForge v7.8.1.737 Initialized 2013-08-07 10:41:43 [iNFO] [sTDOUT] Replaced 85 ore recipies 2013-08-07 10:41:43 [iNFO] [MinecraftForge] Completed early MinecraftForge initialization 2013-08-07 10:41:43 [iNFO] [ForgeModLoader] Reading custom logging properties from C:\Users\Will\Desktop\minecraftforge-src-1.5.2-7.8.1.737 - Villager\forge\mcp\jars\config\logging.properties 2013-08-07 10:41:43 [OFF] [ForgeModLoader] Logging level for ForgeModLoader logging is set to ALL 2013-08-07 10:41:43 [iNFO] [ForgeModLoader] Searching C:\Users\Will\Desktop\minecraftforge-src-1.5.2-7.8.1.737 - Villager\forge\mcp\jars\mods for mods 2013-08-07 10:41:44 [iNFO] [ForgeModLoader] Forge Mod Loader has identified 4 mods to load 2013-08-07 10:41:44 [iNFO] [mcp] Activating mod mcp 2013-08-07 10:41:44 [iNFO] [FML] Activating mod FML 2013-08-07 10:41:44 [iNFO] [Forge] Activating mod Forge 2013-08-07 10:41:44 [iNFO] [Charsmud_VillageUpgrades] Activating mod Charsmud_VillageUpgrades 2013-08-07 10:41:44 [iNFO] [ForgeModLoader] Registering Forge Packet Handler 2013-08-07 10:41:44 [iNFO] [ForgeModLoader] Succeeded registering Forge Packet Handler 2013-08-07 10:41:44 [iNFO] [ForgeModLoader] Configured a dormant chunk cache size of 0 2013-08-07 10:41:44 [iNFO] [sTDOUT] EXECUTED FRMO VILLAGEUPGRADES 2013-08-07 10:41:44 [iNFO] [sTDERR] java.lang.InstantiationException 2013-08-07 10:41:44 [iNFO] [sTDERR] at sun.reflect.InstantiationExceptionConstructorAccessorImpl.newInstance(Unknown Source) 2013-08-07 10:41:44 [iNFO] [sTDERR] at java.lang.reflect.Constructor.newInstance(Unknown Source) 2013-08-07 10:41:44 [iNFO] [sTDERR] at net.minecraftforge.event.EventBus.register(EventBus.java:76) 2013-08-07 10:41:44 [iNFO] [sTDERR] at net.minecraftforge.event.EventBus.register(EventBus.java:58) 2013-08-07 10:41:44 [iNFO] [sTDERR] at village.core.VillageUpgrades.preInit(VillageUpgrades.java:47) 2013-08-07 10:41:44 [iNFO] [sTDERR] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) 2013-08-07 10:41:44 [iNFO] [sTDERR] at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) 2013-08-07 10:41:44 [iNFO] [sTDERR] at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) 2013-08-07 10:41:44 [iNFO] [sTDERR] at java.lang.reflect.Method.invoke(Unknown Source) 2013-08-07 10:41:44 [iNFO] [sTDERR] at cpw.mods.fml.common.FMLModContainer.handleModStateEvent(FMLModContainer.java:494) 2013-08-07 10:41:44 [iNFO] [sTDERR] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) 2013-08-07 10:41:44 [iNFO] [sTDERR] at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) 2013-08-07 10:41:44 [iNFO] [sTDERR] at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) 2013-08-07 10:41:44 [iNFO] [sTDERR] at java.lang.reflect.Method.invoke(Unknown Source) 2013-08-07 10:41:44 [iNFO] [sTDERR] at com.google.common.eventbus.EventHandler.handleEvent(EventHandler.java:74) 2013-08-07 10:41:44 [iNFO] [sTDERR] at com.google.common.eventbus.SynchronizedEventHandler.handleEvent(SynchronizedEventHandler.java:45) 2013-08-07 10:41:44 [iNFO] [sTDERR] at com.google.common.eventbus.EventBus.dispatch(EventBus.java:314) 2013-08-07 10:41:44 [iNFO] [sTDERR] at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:296) 2013-08-07 10:41:44 [iNFO] [sTDERR] at com.google.common.eventbus.EventBus.post(EventBus.java:267) 2013-08-07 10:41:44 [iNFO] [sTDERR] at cpw.mods.fml.common.LoadController.sendEventToModContainer(LoadController.java:192) 2013-08-07 10:41:44 [iNFO] [sTDERR] at cpw.mods.fml.common.LoadController.propogateStateMessage(LoadController.java:172) 2013-08-07 10:41:44 [iNFO] [sTDERR] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) 2013-08-07 10:41:44 [iNFO] [sTDERR] at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) 2013-08-07 10:41:44 [iNFO] [sTDERR] at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) 2013-08-07 10:41:44 [iNFO] [sTDERR] at java.lang.reflect.Method.invoke(Unknown Source) 2013-08-07 10:41:44 [iNFO] [sTDERR] at com.google.common.eventbus.EventHandler.handleEvent(EventHandler.java:74) 2013-08-07 10:41:44 [iNFO] [sTDERR] at com.google.common.eventbus.SynchronizedEventHandler.handleEvent(SynchronizedEventHandler.java:45) 2013-08-07 10:41:44 [iNFO] [sTDERR] at com.google.common.eventbus.EventBus.dispatch(EventBus.java:314) 2013-08-07 10:41:44 [iNFO] [sTDERR] at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:296) 2013-08-07 10:41:44 [iNFO] [sTDERR] at com.google.common.eventbus.EventBus.post(EventBus.java:267) 2013-08-07 10:41:44 [iNFO] [sTDERR] at cpw.mods.fml.common.LoadController.distributeStateMessage(LoadController.java:103) 2013-08-07 10:41:44 [iNFO] [sTDERR] at cpw.mods.fml.common.Loader.loadMods(Loader.java:515) 2013-08-07 10:41:44 [iNFO] [sTDERR] at cpw.mods.fml.client.FMLClientHandler.beginMinecraftLoading(FMLClientHandler.java:163) 2013-08-07 10:41:44 [iNFO] [sTDERR] at net.minecraft.client.Minecraft.startGame(Minecraft.java:411) 2013-08-07 10:41:44 [iNFO] [sTDERR] at net.minecraft.client.MinecraftAppletImpl.startGame(MinecraftAppletImpl.java:44) 2013-08-07 10:41:44 [iNFO] [sTDERR] at net.minecraft.client.Minecraft.run(Minecraft.java:733) 2013-08-07 10:41:44 [iNFO] [sTDERR] at java.lang.Thread.run(Unknown Source) 2013-08-07 10:41:44 [iNFO] [sTDOUT] EXECUTED FROM VILLAGEUPGRADES PRINT 2 2013-08-07 10:41:45 [iNFO] [sTDOUT] 2013-08-07 10:41:45 [iNFO] [sTDOUT] Starting up SoundSystem... 2013-08-07 10:41:45 [iNFO] [sTDOUT] Initializing LWJGL OpenAL 2013-08-07 10:41:45 [iNFO] [sTDOUT] (The LWJGL binding of OpenAL. For more information, see http://www.lwjgl.org) 2013-08-07 10:41:45 [iNFO] [sTDOUT] OpenAL initialized. 2013-08-07 10:41:45 [iNFO] [sTDOUT] 2013-08-07 10:41:46 [iNFO] [ForgeModLoader] Forge Mod Loader has detected an older LWJGL version, new advanced texture animation features are disabled 2013-08-07 10:41:46 [iNFO] [ForgeModLoader] Not using advanced OpenGL 4.3 advanced capability for animations : OpenGL 4.3 is not available 2013-08-07 10:41:46 [iNFO] [Minecraft-Client] Found animation info for: textures/blocks/lava_flow.txt 2013-08-07 10:41:46 [iNFO] [Minecraft-Client] Found animation info for: textures/blocks/water_flow.txt 2013-08-07 10:41:46 [iNFO] [Minecraft-Client] Found animation info for: textures/blocks/fire_0.txt 2013-08-07 10:41:46 [iNFO] [Minecraft-Client] Found animation info for: textures/blocks/fire_1.txt 2013-08-07 10:41:46 [iNFO] [Minecraft-Client] Found animation info for: textures/blocks/lava.txt 2013-08-07 10:41:46 [iNFO] [Minecraft-Client] Found animation info for: textures/blocks/portal.txt 2013-08-07 10:41:46 [iNFO] [Minecraft-Client] Found animation info for: textures/blocks/water.txt 2013-08-07 10:41:46 [iNFO] [Minecraft-Client] Found animation info for: textures/items/clock.txt 2013-08-07 10:41:46 [iNFO] [Minecraft-Client] Found animation info for: textures/items/compass.txt 2013-08-07 10:41:46 [iNFO] [ForgeModLoader] Forge Mod Loader has successfully loaded 4 mods 2013-08-07 10:41:46 [iNFO] [Minecraft-Client] Found animation info for: textures/blocks/lava_flow.txt 2013-08-07 10:41:46 [iNFO] [Minecraft-Client] Found animation info for: textures/blocks/water_flow.txt 2013-08-07 10:41:46 [iNFO] [Minecraft-Client] Found animation info for: textures/blocks/fire_0.txt 2013-08-07 10:41:46 [iNFO] [Minecraft-Client] Found animation info for: textures/blocks/fire_1.txt 2013-08-07 10:41:46 [iNFO] [Minecraft-Client] Found animation info for: textures/blocks/lava.txt 2013-08-07 10:41:46 [iNFO] [Minecraft-Client] Found animation info for: textures/blocks/portal.txt 2013-08-07 10:41:46 [iNFO] [Minecraft-Client] Found animation info for: textures/blocks/water.txt 2013-08-07 10:41:47 [iNFO] [Minecraft-Client] Found animation info for: textures/items/clock.txt 2013-08-07 10:41:47 [iNFO] [Minecraft-Client] Found animation info for: textures/items/compass.txt 2013-08-07 10:42:11 [iNFO] [Minecraft-Server] Starting integrated minecraft server version 1.5.2 2013-08-07 10:42:11 [iNFO] [Minecraft-Server] Generating keypair 2013-08-07 10:42:11 [iNFO] [ForgeModLoader] Loading dimension 0 (New World) (net.minecraft.server.integrated.IntegratedServer@66bf349f) 2013-08-07 10:42:11 [iNFO] [ForgeModLoader] Loading dimension 1 (New World) (net.minecraft.server.integrated.IntegratedServer@66bf349f) 2013-08-07 10:42:11 [iNFO] [ForgeModLoader] Loading dimension -1 (New World) (net.minecraft.server.integrated.IntegratedServer@66bf349f) 2013-08-07 10:42:11 [iNFO] [Minecraft-Server] Preparing start region for level 0 2013-08-07 10:42:13 [iNFO] [sTDOUT] loading single player 2013-08-07 10:42:13 [iNFO] [Minecraft-Server] Player659[/127.0.0.1:0] logged in with entity id 346 at (-126.2266029604828, 70.0, 246.11333630926705) 2013-08-07 10:42:28 [iNFO] [Minecraft-Server] Saving and pausing game... 2013-08-07 10:42:28 [iNFO] [Minecraft-Server] Saving chunks for level 'New World'/Overworld 2013-08-07 10:42:28 [iNFO] [Minecraft-Server] Saving chunks for level 'New World'/Nether 2013-08-07 10:42:28 [iNFO] [Minecraft-Server] Saving chunks for level 'New World'/The End 2013-08-07 10:42:29 [iNFO] [Minecraft-Server] Stopping server 2013-08-07 10:42:29 [iNFO] [Minecraft-Server] Saving players 2013-08-07 10:42:29 [iNFO] [Minecraft-Server] Saving worlds 2013-08-07 10:42:29 [iNFO] [Minecraft-Server] Saving chunks for level 'New World'/Overworld 2013-08-07 10:42:29 [iNFO] [Minecraft-Server] Saving chunks for level 'New World'/Nether 2013-08-07 10:42:29 [iNFO] [Minecraft-Server] Saving chunks for level 'New World'/The End 2013-08-07 10:42:29 [iNFO] [ForgeModLoader] Unloading dimension 0 2013-08-07 10:42:29 [iNFO] [ForgeModLoader] Unloading dimension -1 2013-08-07 10:42:29 [iNFO] [ForgeModLoader] Unloading dimension 1 2013-08-07 10:42:31 [iNFO] [Minecraft-Client] Stopping! 2013-08-07 10:42:31 [iNFO] [sTDOUT] 2013-08-07 10:42:31 [iNFO] [sTDOUT] SoundSystem shutting down... 2013-08-07 10:42:31 [iNFO] [sTDOUT] Author: Paul Lamb, www.paulscode.com 2013-08-07 10:42:31 [iNFO] [sTDOUT]
-
OK, I added prints here:
public void updateTradeBubble(Minecraft minecraft) { System.out.println("EXECUTED FROM VILLAGEMECHANICS"); Tessellator tessellator = Tessellator.instance; minecraft.renderEngine.bindTexture("/village/images/bubble1.png"); tessellator.startDrawingQuads(); tessellator.addVertexWithUV(1, 1, 0, 0, 0); tessellator.addVertexWithUV(1, 1, 0+2, 0, 1); tessellator.addVertexWithUV(1+1, 1+2, 0, 1, 1); tessellator.addVertexWithUV(1, 1+1, 0, 1, 0); tessellator.addVertexWithUV(1, 1, 0, 0, 0); tessellator.addVertexWithUV(1, 1+1, 0, 1, 0); tessellator.addVertexWithUV(1+1, 1+2, 0, 1, 1); tessellator.addVertexWithUV(1, 1, 0+2, 0, 1); tessellator.draw(); }
public void onVillagerRender(RenderLivingEvent event) { VillageMechanics mechanics = new VillageMechanics(); if(event.entity instanceof EntityVillager) { System.out.println("EXECUTED FROM VILLAGERBUBBLEHANDLER"); mechanics.updateTradeBubble(FMLClientHandler.instance().getClient()); } }
None of those prints print.
I also added some in the main mod file, but those print just fine. I also edited the tesselator addVertexWithUVs... is this a more reasonable size?
-
Now I cannot get it to render at all!
Here are my files:
package village.mechanics; import net.minecraft.client.Minecraft; import net.minecraft.client.gui.GuiIngame; import net.minecraft.client.gui.ScaledResolution; import net.minecraft.client.renderer.Tessellator; import net.minecraft.client.renderer.entity.RenderManager; import org.lwjgl.opengl.GL11; /** * Contains information about the new Mechanics for the past * @author Charsmud * */ public class VillageMechanics { /** * Updates Paradox Bar and Renders * @param minecraft * @param amtOfParadox */ public void updateTradeBubble(Minecraft minecraft) { Tessellator tessellator = Tessellator.instance; minecraft.renderEngine.bindTexture("/village/images/speech.png"); tessellator.startDrawingQuads(); tessellator.addVertexWithUV(1, 1, 0, 0, 0); tessellator.addVertexWithUV(1, 1, 0+600, 0, 1); tessellator.addVertexWithUV(1+776, 1+600, 0, 1, 1); tessellator.addVertexWithUV(1, 1+776, 0, 1, 0); tessellator.draw(); } }
package village.mechanics; import cpw.mods.fml.client.FMLClientHandler; import net.minecraft.entity.passive.EntityVillager; import net.minecraftforge.client.event.RenderLivingEvent; import net.minecraftforge.event.Event; import net.minecraftforge.event.ForgeSubscribe; public class VillagerBubbleHandler { public VillagerBubbleHandler() { } @ForgeSubscribe public void onVillagerRender(RenderLivingEvent event) { VillageMechanics mechanics = new VillageMechanics(); if(event.entity instanceof EntityVillager) { mechanics.updateTradeBubble(FMLClientHandler.instance().getClient()); } } }
package village.core; import net.minecraftforge.client.event.RenderLivingEvent; import net.minecraftforge.common.MinecraftForge; import village.mechanics.VillagerBubbleHandler; import village.proxies.CommonProxy; import cpw.mods.fml.common.Mod; import cpw.mods.fml.common.Mod.Init; import cpw.mods.fml.common.Mod.Instance; import cpw.mods.fml.common.Mod.PreInit; import cpw.mods.fml.common.SidedProxy; import cpw.mods.fml.common.event.FMLInitializationEvent; import cpw.mods.fml.common.event.FMLPreInitializationEvent; import cpw.mods.fml.common.network.NetworkMod; import cpw.mods.fml.common.network.NetworkMod.SidedPacketHandler; import cpw.mods.fml.common.registry.TickRegistry; import cpw.mods.fml.relauncher.Side; @Mod(modid = "Charsmud_VillageUpgrades", name = "Village Upgrades", version = "1.0") @NetworkMod(clientSideRequired = true, serverSideRequired = false) /** * Main laucher for VillageUpgrades * @author Charsmud * */ public class VillageUpgrades { @SidedProxy(clientSide = "village.proxies.ClientProxy", serverSide = "village.proxies.CommonProxy") public static CommonProxy proxy; @Instance public static VillageUpgrades instance = new VillageUpgrades(); public static final String modid = "Charsmud_VillageUpgrades"; /** * Initiates mod, registers block and item for use. Generates the necessary folders. */ @PreInit public void preInit(FMLPreInitializationEvent event) { MinecraftForge.EVENT_BUS.register(new VillagerBubbleHandler()); } @Init public void load(FMLInitializationEvent event) { proxy.registerRenderThings(); TickRegistry.registerTickHandler(new TickerClient(), Side.CLIENT); } }
I have NO idea why it's not working when Its a copy-paste of what I had before. The image is in the right place.
-
I feel like I messed up somewhere.... now the image doesn't even render, even messed up...
-
Hm.... neither of those worked. Nothing now appears. Here's what I have edited:
package village.mechanics; import net.minecraft.client.Minecraft; import net.minecraft.client.renderer.Tessellator; import net.minecraft.client.renderer.entity.RenderManager; import net.minecraft.entity.player.EntityPlayer; import net.minecraftforge.client.event.RenderLivingEvent; import org.lwjgl.opengl.GL11; /** * Contains information about the new Mechanics for the past * @author Charsmud * */ public class VillageMechanics { /** * Updates Paradox Bar and Renders * @param minecraft * @param amtOfParadox */ public void updateTradeBubble(Minecraft minecraft, RenderLivingEvent event) { Tessellator tessellator = Tessellator.instance; GL11.glRotatef(-RenderManager.instance.playerViewY, 0.0F, 1.0F, 0.0F); GL11.glRotatef(RenderManager.instance.playerViewX, 1.0F, 0.0F, 0.0F); EntityPlayer localPlayer = Minecraft.getMinecraft().thePlayer; double x = event.entity.posX - localPlayer.posX; double y = event.entity.posY - localPlayer.posY; double z = event.entity.posZ - localPlayer.posZ; //double x = localPlayer.posX - event.entity.posX; //double y = localPlayer.posY - event.entity.posY; //double z = localPlayer.posZ - event.entity.posZ; GL11.glPushMatrix(); GL11.glTranslated(x, y, z); minecraft.renderEngine.bindTexture("/village/images/speech.png"); tessellator.startDrawingQuads(); tessellator.addVertexWithUV((double)1, (double)1, (double)0, (double)0, (double)0); tessellator.addVertexWithUV((double)1, (double)1, (double)0+600, (double)0, (double)1); tessellator.addVertexWithUV((double)1+776, (double)1+600, (double)0, (double)1, (double)1); tessellator.addVertexWithUV((double)1+776, (double)1, (double)0, (double)1, (double)0); tessellator.draw(); GL11.glPopMatrix(); } }
package village.mechanics; import cpw.mods.fml.client.FMLClientHandler; import net.minecraft.entity.passive.EntityVillager; import net.minecraftforge.client.event.RenderLivingEvent; import net.minecraftforge.event.Event; import net.minecraftforge.event.ForgeSubscribe; public class VillagerBubbleHandler extends Event { VillageMechanics mechanics; public VillagerBubbleHandler() { mechanics = new VillageMechanics(); } @ForgeSubscribe public void onVillagerRender(RenderLivingEvent event) { if(event.entity instanceof EntityVillager) { mechanics.updateTradeBubble(FMLClientHandler.instance().getClient(), event); } } }
I was going to learn OGL but then I settled with Direct3D instead.
Have any idea why it's not rendering?
-
Do you know how to do that? I'm guessing you get the x, y, and z coordinates of a mob but I'm not sure what to do from there....
I'm a bit of a noob at OpenGL, as I haven't really delved that deep into it.
-
Please give us your main mod file (the one with the @Mod annotation). The error is originating from in there, so we need that file.
-
OK, I have made progress.... however, it seems to be glitching out.
Here are the relevant files:
package village.mechanics; import cpw.mods.fml.client.FMLClientHandler; import net.minecraft.entity.passive.EntityVillager; import net.minecraftforge.client.event.RenderLivingEvent; import net.minecraftforge.event.Event; import net.minecraftforge.event.ForgeSubscribe; public class VillagerBubbleHandler extends Event { VillageMechanics mechanics; public VillagerBubbleHandler() { mechanics = new VillageMechanics(); } @ForgeSubscribe public void onVillagerRender(RenderLivingEvent event) { System.out.println(event.entity); if(event.entity instanceof EntityVillager) { mechanics.updateTradeBubble(FMLClientHandler.instance().getClient()); } } }
package village.mechanics; import net.minecraft.client.Minecraft; import net.minecraft.client.gui.GuiIngame; import net.minecraft.client.gui.ScaledResolution; import net.minecraft.client.renderer.Tessellator; import net.minecraft.client.renderer.entity.RenderManager; import org.lwjgl.opengl.GL11; /** * Contains information about the new Mechanics for the past * @author Charsmud * */ public class VillageMechanics { /** * Updates Paradox Bar and Renders * @param minecraft * @param amtOfParadox */ public void updateTradeBubble(Minecraft minecraft) { Tessellator tessellator = Tessellator.instance; minecraft.renderEngine.bindTexture("/village/images/speech.png"); tessellator.startDrawingQuads(); tessellator.addVertexWithUV((double)1, (double)1, (double)0, (double)0, (double)0); tessellator.addVertexWithUV((double)1, (double)1, (double)0+600, (double)0, (double)1); tessellator.addVertexWithUV((double)1+776, (double)1+600, (double)0, (double)1, (double)1); tessellator.addVertexWithUV((double)1+776, (double)1, (double)0, (double)1, (double)0); tessellator.draw(); } }
package village.core; import net.minecraftforge.client.event.RenderLivingEvent; import net.minecraftforge.common.MinecraftForge; import village.mechanics.VillagerBubbleHandler; import village.proxies.CommonProxy; import cpw.mods.fml.common.Mod; import cpw.mods.fml.common.Mod.Init; import cpw.mods.fml.common.Mod.Instance; import cpw.mods.fml.common.Mod.PreInit; import cpw.mods.fml.common.SidedProxy; import cpw.mods.fml.common.event.FMLInitializationEvent; import cpw.mods.fml.common.event.FMLPreInitializationEvent; import cpw.mods.fml.common.network.NetworkMod; import cpw.mods.fml.common.network.NetworkMod.SidedPacketHandler; import cpw.mods.fml.common.registry.TickRegistry; import cpw.mods.fml.relauncher.Side; @Mod(modid = "Charsmud_VillageUpgrades", name = "Village Upgrades", version = "1.0") @NetworkMod(clientSideRequired = true, serverSideRequired = false) /** * Main laucher for VillageUpgrades * @author Charsmud * */ public class VillageUpgrades { @SidedProxy(clientSide = "village.proxies.ClientProxy", serverSide = "village.proxies.CommonProxy") public static CommonProxy proxy; @Instance public static VillageUpgrades instance = new VillageUpgrades(); public static final String modid = "Charsmud_VillageUpgrades"; /** * Initiates mod, registers block and item for use. Generates the necessary folders. */ @Init public void load(FMLInitializationEvent event) { MinecraftForge.EVENT_BUS.register(new VillagerBubbleHandler()); proxy.registerRenderThings(); TickRegistry.registerTickHandler(new TickerClient(), Side.CLIENT); } }
I am also getting this error upon start, but the game runs fine:
2013-08-06 13:51:03 [iNFO] [ForgeModLoader] Forge Mod Loader version 5.2.23.737 for Minecraft 1.5.2 loading 2013-08-06 13:51:03 [iNFO] [ForgeModLoader] Java is Java HotSpot(TM) 64-Bit Server VM, version 1.7.0_09, running on Windows 7:amd64:6.1, installed at C:\Users\Will\Desktop\eclipse-jee-juno-SR2-win32-x86_64\eclipse\jre 2013-08-06 13:51:03 [iNFO] [ForgeModLoader] Managed to load a deobfuscated Minecraft name- we are in a deobfuscated environment. Skipping runtime deobfuscation 2013-08-06 13:51:05 [iNFO] [sTDOUT] 229 recipes 2013-08-06 13:51:05 [iNFO] [sTDOUT] 27 achievements 2013-08-06 13:51:06 [iNFO] [Minecraft-Client] Setting user: Player343 2013-08-06 13:51:06 [iNFO] [sTDOUT] (Session ID is -) 2013-08-06 13:51:06 [iNFO] [sTDERR] Client asked for parameter: server 2013-08-06 13:51:06 [iNFO] [Minecraft-Client] LWJGL Version: 2.4.2 2013-08-06 13:51:06 [iNFO] [MinecraftForge] Attempting early MinecraftForge initialization 2013-08-06 13:51:06 [iNFO] [sTDOUT] MinecraftForge v7.8.1.737 Initialized 2013-08-06 13:51:06 [iNFO] [ForgeModLoader] MinecraftForge v7.8.1.737 Initialized 2013-08-06 13:51:06 [iNFO] [sTDOUT] Replaced 85 ore recipies 2013-08-06 13:51:06 [iNFO] [MinecraftForge] Completed early MinecraftForge initialization 2013-08-06 13:51:06 [iNFO] [ForgeModLoader] Reading custom logging properties from C:\Users\Will\Desktop\minecraftforge-src-1.5.2-7.8.1.737 - Villager\forge\mcp\jars\config\logging.properties 2013-08-06 13:51:06 [OFF] [ForgeModLoader] Logging level for ForgeModLoader logging is set to ALL 2013-08-06 13:51:06 [iNFO] [ForgeModLoader] Searching C:\Users\Will\Desktop\minecraftforge-src-1.5.2-7.8.1.737 - Villager\forge\mcp\jars\mods for mods 2013-08-06 13:51:08 [iNFO] [ForgeModLoader] Forge Mod Loader has identified 4 mods to load 2013-08-06 13:51:08 [iNFO] [mcp] Activating mod mcp 2013-08-06 13:51:08 [iNFO] [FML] Activating mod FML 2013-08-06 13:51:08 [iNFO] [Forge] Activating mod Forge 2013-08-06 13:51:08 [iNFO] [Charsmud_VillageUpgrades] Activating mod Charsmud_VillageUpgrades 2013-08-06 13:51:08 [iNFO] [ForgeModLoader] Registering Forge Packet Handler 2013-08-06 13:51:08 [iNFO] [ForgeModLoader] Succeeded registering Forge Packet Handler 2013-08-06 13:51:08 [iNFO] [ForgeModLoader] Configured a dormant chunk cache size of 0 2013-08-06 13:51:08 [iNFO] [sTDOUT] 2013-08-06 13:51:08 [iNFO] [sTDOUT] Starting up SoundSystem... 2013-08-06 13:51:08 [iNFO] [sTDOUT] Initializing LWJGL OpenAL 2013-08-06 13:51:08 [iNFO] [sTDOUT] (The LWJGL binding of OpenAL. For more information, see http://www.lwjgl.org) 2013-08-06 13:51:09 [iNFO] [sTDOUT] OpenAL initialized. 2013-08-06 13:51:09 [iNFO] [sTDOUT] 2013-08-06 13:51:09 [iNFO] [ForgeModLoader] Forge Mod Loader has detected an older LWJGL version, new advanced texture animation features are disabled 2013-08-06 13:51:09 [iNFO] [ForgeModLoader] Not using advanced OpenGL 4.3 advanced capability for animations : OpenGL 4.3 is not available 2013-08-06 13:51:09 [iNFO] [Minecraft-Client] Found animation info for: textures/blocks/lava_flow.txt 2013-08-06 13:51:09 [iNFO] [Minecraft-Client] Found animation info for: textures/blocks/water_flow.txt 2013-08-06 13:51:09 [iNFO] [Minecraft-Client] Found animation info for: textures/blocks/fire_0.txt 2013-08-06 13:51:09 [iNFO] [Minecraft-Client] Found animation info for: textures/blocks/fire_1.txt 2013-08-06 13:51:09 [iNFO] [Minecraft-Client] Found animation info for: textures/blocks/lava.txt 2013-08-06 13:51:09 [iNFO] [Minecraft-Client] Found animation info for: textures/blocks/portal.txt 2013-08-06 13:51:09 [iNFO] [Minecraft-Client] Found animation info for: textures/blocks/water.txt 2013-08-06 13:51:10 [iNFO] [Minecraft-Client] Found animation info for: textures/items/clock.txt 2013-08-06 13:51:10 [iNFO] [Minecraft-Client] Found animation info for: textures/items/compass.txt 2013-08-06 13:51:10 [iNFO] [sTDERR] java.lang.InstantiationException 2013-08-06 13:51:10 [iNFO] [sTDERR] at sun.reflect.InstantiationExceptionConstructorAccessorImpl.newInstance(Unknown Source) 2013-08-06 13:51:10 [iNFO] [sTDERR] at java.lang.reflect.Constructor.newInstance(Unknown Source) 2013-08-06 13:51:10 [iNFO] [sTDERR] at net.minecraftforge.event.EventBus.register(EventBus.java:76) 2013-08-06 13:51:10 [iNFO] [sTDERR] at net.minecraftforge.event.EventBus.register(EventBus.java:58) 2013-08-06 13:51:10 [iNFO] [sTDERR] at village.core.VillageUpgrades.load(VillageUpgrades.java:46) 2013-08-06 13:51:10 [iNFO] [sTDERR] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) 2013-08-06 13:51:10 [iNFO] [sTDERR] at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) 2013-08-06 13:51:10 [iNFO] [sTDERR] at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) 2013-08-06 13:51:10 [iNFO] [sTDERR] at java.lang.reflect.Method.invoke(Unknown Source) 2013-08-06 13:51:10 [iNFO] [sTDERR] at cpw.mods.fml.common.FMLModContainer.handleModStateEvent(FMLModContainer.java:494) 2013-08-06 13:51:10 [iNFO] [sTDERR] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) 2013-08-06 13:51:10 [iNFO] [sTDERR] at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) 2013-08-06 13:51:10 [iNFO] [sTDERR] at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) 2013-08-06 13:51:10 [iNFO] [sTDERR] at java.lang.reflect.Method.invoke(Unknown Source) 2013-08-06 13:51:10 [iNFO] [sTDERR] at com.google.common.eventbus.EventHandler.handleEvent(EventHandler.java:74) 2013-08-06 13:51:10 [iNFO] [sTDERR] at com.google.common.eventbus.SynchronizedEventHandler.handleEvent(SynchronizedEventHandler.java:45) 2013-08-06 13:51:10 [iNFO] [sTDERR] at com.google.common.eventbus.EventBus.dispatch(EventBus.java:314) 2013-08-06 13:51:10 [iNFO] [sTDERR] at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:296) 2013-08-06 13:51:10 [iNFO] [sTDERR] at com.google.common.eventbus.EventBus.post(EventBus.java:267) 2013-08-06 13:51:10 [iNFO] [sTDERR] at cpw.mods.fml.common.LoadController.sendEventToModContainer(LoadController.java:192) 2013-08-06 13:51:10 [iNFO] [sTDERR] at cpw.mods.fml.common.LoadController.propogateStateMessage(LoadController.java:172) 2013-08-06 13:51:10 [iNFO] [sTDERR] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) 2013-08-06 13:51:10 [iNFO] [sTDERR] at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) 2013-08-06 13:51:10 [iNFO] [sTDERR] at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) 2013-08-06 13:51:10 [iNFO] [sTDERR] at java.lang.reflect.Method.invoke(Unknown Source) 2013-08-06 13:51:10 [iNFO] [sTDERR] at com.google.common.eventbus.EventHandler.handleEvent(EventHandler.java:74) 2013-08-06 13:51:10 [iNFO] [sTDERR] at com.google.common.eventbus.SynchronizedEventHandler.handleEvent(SynchronizedEventHandler.java:45) 2013-08-06 13:51:10 [iNFO] [sTDERR] at com.google.common.eventbus.EventBus.dispatch(EventBus.java:314) 2013-08-06 13:51:10 [iNFO] [sTDERR] at com.google.common.eventbus.EventBus.dispatchQueuedEvents(EventBus.java:296) 2013-08-06 13:51:10 [iNFO] [sTDERR] at com.google.common.eventbus.EventBus.post(EventBus.java:267) 2013-08-06 13:51:10 [iNFO] [sTDERR] at cpw.mods.fml.common.LoadController.distributeStateMessage(LoadController.java:103) 2013-08-06 13:51:10 [iNFO] [sTDERR] at cpw.mods.fml.common.Loader.initializeMods(Loader.java:691) 2013-08-06 13:51:10 [iNFO] [sTDERR] at cpw.mods.fml.client.FMLClientHandler.finishMinecraftLoading(FMLClientHandler.java:213) 2013-08-06 13:51:10 [iNFO] [sTDERR] at net.minecraft.client.Minecraft.startGame(Minecraft.java:448) 2013-08-06 13:51:10 [iNFO] [sTDERR] at net.minecraft.client.MinecraftAppletImpl.startGame(MinecraftAppletImpl.java:44) 2013-08-06 13:51:10 [iNFO] [sTDERR] at net.minecraft.client.Minecraft.run(Minecraft.java:733) 2013-08-06 13:51:10 [iNFO] [sTDERR] at java.lang.Thread.run(Unknown Source) 2013-08-06 13:51:10 [iNFO] [ForgeModLoader] Forge Mod Loader has successfully loaded 4 mods 2013-08-06 13:51:10 [iNFO] [Minecraft-Client] Found animation info for: textures/blocks/lava_flow.txt 2013-08-06 13:51:10 [iNFO] [Minecraft-Client] Found animation info for: textures/blocks/water_flow.txt 2013-08-06 13:51:10 [iNFO] [Minecraft-Client] Found animation info for: textures/blocks/fire_0.txt 2013-08-06 13:51:10 [iNFO] [Minecraft-Client] Found animation info for: textures/blocks/fire_1.txt 2013-08-06 13:51:10 [iNFO] [Minecraft-Client] Found animation info for: textures/blocks/lava.txt 2013-08-06 13:51:10 [iNFO] [Minecraft-Client] Found animation info for: textures/blocks/portal.txt 2013-08-06 13:51:10 [iNFO] [Minecraft-Client] Found animation info for: textures/blocks/water.txt 2013-08-06 13:51:10 [iNFO] [Minecraft-Client] Found animation info for: textures/items/clock.txt 2013-08-06 13:51:10 [iNFO] [Minecraft-Client] Found animation info for: textures/items/compass.txt 2013-08-06 13:51:12 [iNFO] [Minecraft-Server] Starting integrated minecraft server version 1.5.2 2013-08-06 13:51:12 [iNFO] [Minecraft-Server] Generating keypair 2013-08-06 13:51:13 [iNFO] [ForgeModLoader] Loading dimension 0 (ds) (net.minecraft.server.integrated.IntegratedServer@75d811e7) 2013-08-06 13:51:13 [iNFO] [ForgeModLoader] Loading dimension 1 (ds) (net.minecraft.server.integrated.IntegratedServer@75d811e7) 2013-08-06 13:51:13 [iNFO] [ForgeModLoader] Loading dimension -1 (ds) (net.minecraft.server.integrated.IntegratedServer@75d811e7) 2013-08-06 13:51:13 [iNFO] [Minecraft-Server] Preparing start region for level 0 2013-08-06 13:51:14 [iNFO] [sTDOUT] loading single player 2013-08-06 13:51:14 [iNFO] [Minecraft-Server] Player343[/127.0.0.1:0] logged in with entity id 403 at (-78.67326373669563, 64.0, 313.677763770806) 2013-08-06 13:51:23 [iNFO] [Minecraft-Server] Saving and pausing game... 2013-08-06 13:51:23 [iNFO] [Minecraft-Server] Saving chunks for level 'ds'/Overworld 2013-08-06 13:51:23 [iNFO] [Minecraft-Server] Saving chunks for level 'ds'/Nether 2013-08-06 13:51:23 [iNFO] [Minecraft-Server] Saving chunks for level 'ds'/The End 2013-08-06 13:51:24 [iNFO] [Minecraft-Server] Saving and pausing game... 2013-08-06 13:51:24 [iNFO] [Minecraft-Server] Saving chunks for level 'ds'/Overworld 2013-08-06 13:51:24 [iNFO] [Minecraft-Server] Saving chunks for level 'ds'/Nether 2013-08-06 13:51:24 [iNFO] [Minecraft-Server] Saving chunks for level 'ds'/The End 2013-08-06 13:51:25 [iNFO] [Minecraft-Server] Saving and pausing game... 2013-08-06 13:51:25 [iNFO] [Minecraft-Server] Saving chunks for level 'ds'/Overworld 2013-08-06 13:51:25 [iNFO] [Minecraft-Server] Saving chunks for level 'ds'/Nether 2013-08-06 13:51:25 [iNFO] [Minecraft-Server] Saving chunks for level 'ds'/The End 2013-08-06 13:51:28 [iNFO] [Minecraft-Server] Saving and pausing game... 2013-08-06 13:51:28 [iNFO] [Minecraft-Server] Saving chunks for level 'ds'/Overworld 2013-08-06 13:51:28 [iNFO] [Minecraft-Server] Saving chunks for level 'ds'/Nether 2013-08-06 13:51:28 [iNFO] [Minecraft-Server] Saving chunks for level 'ds'/The End 2013-08-06 13:52:05 [iNFO] [Minecraft-Client] [CHAT] Saved screenshot as 2013-08-06_13.52.05.png 2013-08-06 13:52:06 [iNFO] [Minecraft-Server] Saving and pausing game... 2013-08-06 13:52:06 [iNFO] [Minecraft-Server] Saving chunks for level 'ds'/Overworld 2013-08-06 13:52:06 [iNFO] [Minecraft-Server] Saving chunks for level 'ds'/Nether 2013-08-06 13:52:06 [iNFO] [Minecraft-Server] Saving chunks for level 'ds'/The End 2013-08-06 13:52:06 [iNFO] [Minecraft-Server] Stopping server 2013-08-06 13:52:06 [iNFO] [Minecraft-Server] Saving players 2013-08-06 13:52:06 [iNFO] [Minecraft-Server] Saving worlds 2013-08-06 13:52:06 [iNFO] [Minecraft-Server] Saving chunks for level 'ds'/Overworld 2013-08-06 13:52:06 [iNFO] [Minecraft-Server] Saving chunks for level 'ds'/Nether 2013-08-06 13:52:06 [iNFO] [Minecraft-Server] Saving chunks for level 'ds'/The End 2013-08-06 13:52:07 [iNFO] [ForgeModLoader] Unloading dimension 0 2013-08-06 13:52:07 [iNFO] [ForgeModLoader] Unloading dimension -1 2013-08-06 13:52:07 [iNFO] [ForgeModLoader] Unloading dimension 1 2013-08-06 13:52:08 [iNFO] [Minecraft-Client] Stopping! 2013-08-06 13:52:08 [iNFO] [sTDOUT] 2013-08-06 13:52:08 [iNFO] [sTDOUT] SoundSystem shutting down... 2013-08-06 13:52:08 [iNFO] [sTDOUT] Author: Paul Lamb, www.paulscode.com 2013-08-06 13:52:08 [iNFO] [sTDOUT]
I also tried using the nameplate rendering code directly from EntityLiving.java, but that just glitched out and did not have the results that I want. Any idea?
Change an entity's UUID
in Modder Support
Posted
Hello! I need to change an entity's UUID: I am able to retrieve the entity's UUID, but am unable to set it. I am currently in 1.6.2, and am hoping that 1.7.2 will hold the changes to UUIDs necessary to be able to do this, or is this already implemented without reflection?