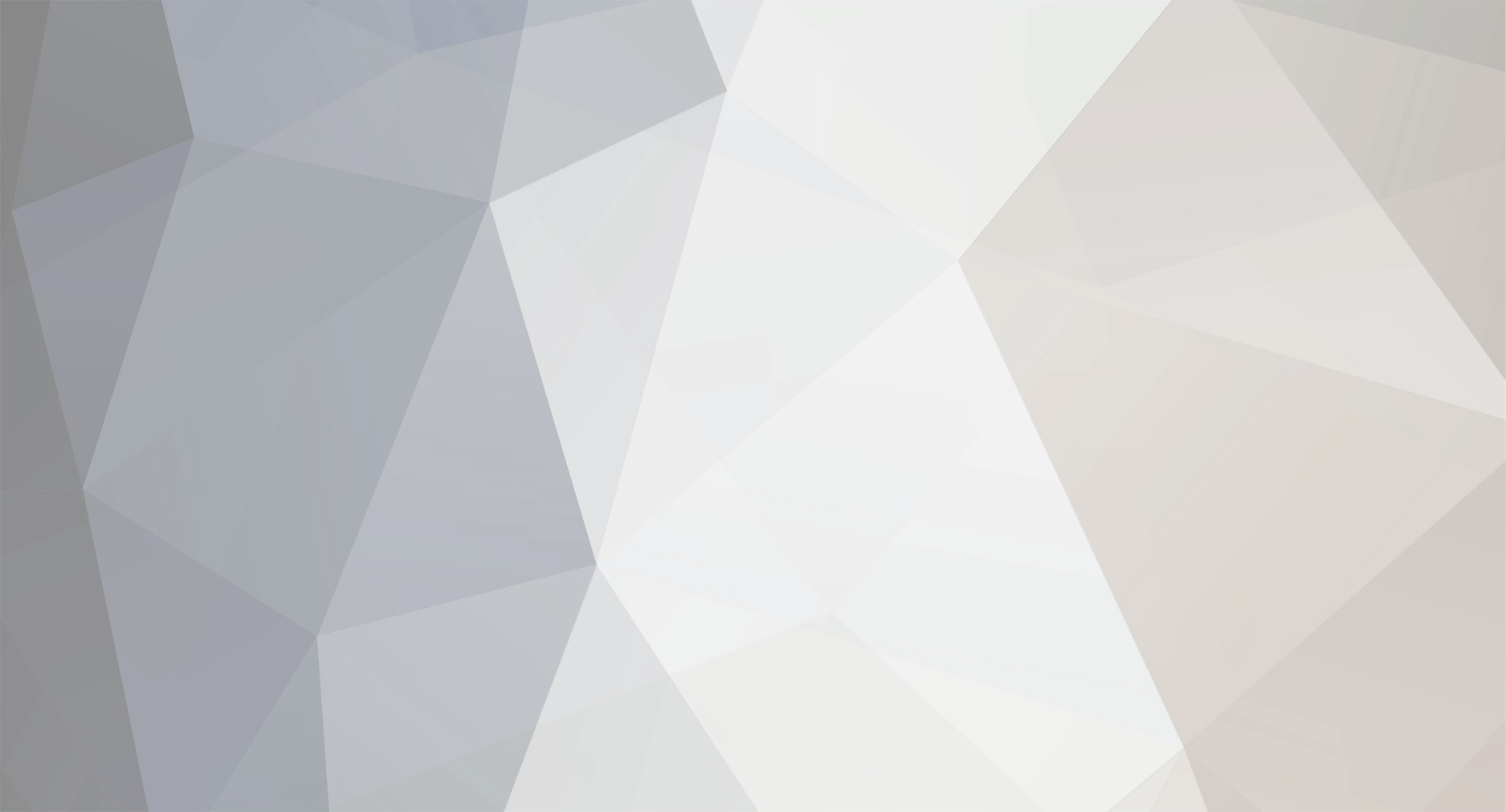
charsmud
-
Posts
89 -
Joined
-
Last visited
Posts posted by charsmud
-
-
What do you mean by coordinates to your nameplate? Do you mean the location of the image relative to the mob?
-
What are the X, Y, and Z coordinates? Are those coordinates in the world?
-
It happens with I load a world. I tried changing the line to this:
File beginningOfWorld = new File(minecraft.getMinecraftDir() + "/mods/TimeMod/present/" + FMLServerHandler.instance().getServer().getWorldName());
But I got the same crash.
-
I know that ms is null, but I don't know why ms is null. It should be passed in through the Server Ticker, but for some reason, it is null.
-
This is line 129:
File beginningOfWorld = new File(minecraft.getMinecraftDir() + "/mods/TimeMod/present/" + ms.getWorldName());
I'm fairly confident it's because ms is null, but I have no idea how to fix it. I tried if(ms != null) but that just crashed.
-
I am trying to move my client ticker over to a server ticker, as all my code was only being executed by the client. However, I am getting a nullPointerException on a MinecraftServer variable inside of my server ticker. Here's the code and error:
package timeTraveler.ticker; import java.io.BufferedReader; import java.io.File; import java.io.FileReader; import java.io.IOException; import java.util.EnumSet; import timeTraveler.core.TimeTraveler; import timeTraveler.entities.EntityPlayerPast; import timeTraveler.gui.GuiTimeTravel; import timeTraveler.mechanics.CopyFile; import timeTraveler.mechanics.EntityMechanics; import timeTraveler.mechanics.PastMechanics; import net.minecraft.client.Minecraft; import net.minecraft.client.entity.EntityPlayerSP; import net.minecraft.entity.Entity; import net.minecraft.entity.EntityList; import net.minecraft.entity.EntityLiving; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.pathfinding.PathEntity; import net.minecraft.potion.Potion; import net.minecraft.server.MinecraftServer; import cpw.mods.fml.client.FMLClientHandler; import cpw.mods.fml.common.ITickHandler; import cpw.mods.fml.common.TickType; import cpw.mods.fml.server.FMLServerHandler; public class ServerTickHandler implements ITickHandler { public int ctr; public int ct; public int count; public static int paradoxLevel; public static int seconds = 10; public static int minutes = 1; public int invisPotTime = 0; public int sneakTime = 0; private int timeNumber = 1; /*int prevSheep; int prevPig; int prevCow; int prevChick; int prevSqu; int prevWol; int prevOce; int prevBat; int prevGol; int prevMoo; int prevVil; int prevEnd; int prevZpi; int prevBla; int prevCsp; int prevCre; int prevGha; int prevMag; int prevSil; int prevSke; int prevSli; int prevSpi; int prevWit; int prevZom;*/ String text; CopyFile copyFile = new CopyFile(); public boolean hasRun = false; public boolean hasInitMobs = false; private boolean nextSet = true; private boolean isInPast; private void onServerTick(MinecraftServer server, Minecraft mc) { if(FMLServerHandler.instance().getServer() != null || mc.theWorld != null) { System.out.println(""); ctr++; ct++; PastMechanics mechanics = new PastMechanics(); EntityMechanics entityMechanics = new EntityMechanics(); text = "Time Remaining: " + minutes + " Minute, " + seconds + " Seconds"; TimeTraveler.vars.setPastText(text); TimeTraveler.vars.setParadoxLevel(paradoxLevel); System.out.println(TimeTraveler.vars.getPastText()); System.out.println(TimeTraveler.vars.getParadoxLevel()); System.out.println(text); System.out.println(paradoxLevel); isInPast = GuiTimeTravel.isInPast; if(!isInPast) { if(mc.thePlayer.isJumping) { } if(mc.thePlayer.isSneaking()) { } } if(ct == 20) { if(!isInPast) { mechanics.addEntityData(TimeTraveler.vars.getEntiyLocData()); ct = 0; } } if(ctr == 20 * 60) { if(!isInPast) { mechanics.saveTime(server, mc, copyFile); //mechanics.addPlayerLoc(mc.getIntegratedServer(), mc, "stop"); //mechanics.addPlayerLoc(mc.getIntegratedServer(), mc, "newtime"); mechanics.saveEntityData(TimeTraveler.vars.getEntiyLocData(), server); } ctr = 0; } if(!hasRun) { hasRun = true; mechanics.firstTime(server, mc); } if(isInPast) { mc.theWorld.getGameRules().setOrCreateGameRule("doMobSpawning", "false"); File allEntityData = new File(mc.getMinecraftDir() + "/mods/TimeMod/past/EntityLocations/" + server.getWorldName() + "/" + TimeTraveler.vars.getPastTime() + ".epd"); try { BufferedReader reader = new BufferedReader(new FileReader(allEntityData)); String line; while (((line = reader.readLine()) != null) && nextSet) { if(line.equals("====================")) { nextSet = false; } else { String[] entityData = line.split(","); String entityName = entityData[0]; int entityX = Integer.parseInt(entityData[1]); int entityY = Integer.parseInt(entityData[2]); int entityZ = Integer.parseInt(entityData[3]); //System.out.println(entityName + " " + entityX + " " + entityY + " " + entityZ); Entity pastEntity = EntityList.createEntityByName(entityName, mc.thePlayer.worldObj); if(pastEntity != null) { PathEntity path = ((EntityLiving)pastEntity).getNavigator().getPath(); if(pastEntity != null) { pastEntity.posX = (double)entityX; pastEntity.posY = (double)entityY; pastEntity.posZ = (double)entityZ; System.out.println(pastEntity); mc.thePlayer.worldObj.spawnEntityInWorld(pastEntity); } } else { EntityPlayerPast pastPlayer = new EntityPlayerPast(mc.thePlayer.worldObj); pastPlayer.posX = (double)entityX; pastPlayer.posY = (double)entityY; pastPlayer.posZ = (double)entityZ; System.out.println(pastPlayer); mc.thePlayer.worldObj.spawnEntityInWorld(pastPlayer); } //path = ((EntityLiving)pastEntity).getNavigator().getPathToXYZ((double)entityX, (double)entityY, (double)entityZ); //((EntityLiving)pastEntity).getNavigator().setPath(path, 1.0F); //((EntityLiving)pastEntity).getNavigator().tryMoveToXYZ((double)entityX, (double)entityY, (double)entityZ, 0.5F); } System.out.println(nextSet); } reader.close(); } catch (IOException e) { e.printStackTrace(); } count++; if(paradoxLevel < 0) { paradoxLevel = 0; } if(paradoxLevel <= 128) { EntityPlayerSP eps = mc.thePlayer; if(eps.isPotionActive(Potion.invisibility)) { invisPotTime++; if(invisPotTime == 10) { paradoxLevel = paradoxLevel - 5; invisPotTime = 0; } } if(eps.isEating()) { paradoxLevel++; eps.experienceTotal = eps.experienceTotal + 2; } if(eps.isSneaking()) { sneakTime++; if(sneakTime == 30) { paradoxLevel = paradoxLevel - 2; sneakTime = 0; } } /*WorldClient w = mc.theWorld; //Passive Mobs EntitySheep es = new EntitySheep(w); EntityPig ep = new EntityPig(w); EntityCow ec = new EntityCow(w); EntityChicken eck = new EntityChicken(w); EntitySquid esq = new EntitySquid(w); EntityWolf ew = new EntityWolf(w); EntityOcelot eo = new EntityOcelot(w); EntityBat eb = new EntityBat(w); EntityIronGolem ei = new EntityIronGolem(w); EntityMooshroom em = new EntityMooshroom(w); EntityVillager ev = new EntityVillager(w); EntityEnderman ee = new EntityEnderman(w); EntityPigZombie ezp = new EntityPigZombie(w); EntityBlaze ebl = new EntityBlaze(w); EntityCaveSpider ecs = new EntityCaveSpider(w); EntityCreeper ecr = new EntityCreeper(w); EntityGhast eg = new EntityGhast(w); EntityMagmaCube emc = new EntityMagmaCube(w); EntitySilverfish esi = new EntitySilverfish(w); EntitySkeleton esk = new EntitySkeleton(w); EntitySlime esl = new EntitySlime(w); EntitySpider esp = new EntitySpider(w); EntityWitch ewi = new EntityWitch(w); EntityZombie ez = new EntityZombie(w); if(!hasInitMobs) { prevSheep = w.countEntities(es.getClass()); prevPig = w.countEntities(ep.getClass()); prevCow = w.countEntities(ec.getClass()); prevChick = w.countEntities(eck.getClass()); prevSqu = w.countEntities(esq.getClass()); prevWol = w.countEntities(ew.getClass()); prevOce = w.countEntities(eo.getClass()); prevBat = w.countEntities(eb.getClass()); prevGol = w.countEntities(ei.getClass()); prevMoo = w.countEntities(ei.getClass()); prevVil = w.countEntities(ev.getClass()); prevEnd = w.countEntities(ee.getClass()); prevZpi = w.countEntities(ezp.getClass()); prevBla = w.countEntities(ebl.getClass()); prevCsp = w.countEntities(ecs.getClass()); prevCre = w.countEntities(ecr.getClass()); prevGha = w.countEntities(eg.getClass()); prevMag = w.countEntities(emc.getClass()); prevSil = w.countEntities(esi.getClass()); prevSke = w.countEntities(esk.getClass()); prevSli = w.countEntities(esl.getClass()); prevSpi = w.countEntities(esp.getClass()); prevWit = w.countEntities(ewi.getClass()); prevZom = w.countEntities(ez.getClass()); hasRun = true; } if(prevSheep > w.countEntities(es.getClass())) { paradoxLevel = paradoxLevel + 4; prevSheep = w.countEntities(es.getClass()); } if(prevPig > w.countEntities(ep.getClass())) { paradoxLevel = paradoxLevel + 8; prevPig = w.countEntities(ep.getClass()); } if(prevCow > w.countEntities(ec.getClass())) { paradoxLevel = paradoxLevel + 12; prevCow = w.countEntities(ec.getClass()); } if(prevChick > w.countEntities(eck.getClass())) { paradoxLevel = paradoxLevel + 7; prevChick = w.countEntities(eck.getClass()); } if(prevSqu > w.countEntities(esq.getClass())) { paradoxLevel = paradoxLevel + 2; prevSqu = w.countEntities(esq.getClass()); } if(prevWol > w.countEntities(ew.getClass())) { paradoxLevel = paradoxLevel + 14; prevWol = w.countEntities(ew.getClass()); } if(prevOce > w.countEntities(eo.getClass())){ paradoxLevel = paradoxLevel + 20; prevOce = w.countEntities(eo.getClass()); } if(prevBat > w.countEntities(eb.getClass())) { paradoxLevel++; prevBat = w.countEntities(eo.getClass()); } if(prevMoo > w.countEntities(em.getClass())) { paradoxLevel = paradoxLevel + 14; prevMoo = w.countEntities(em.getClass()); } if(prevGol > w.countEntities(ei.getClass())) { paradoxLevel = paradoxLevel + 78; prevBat = w.countEntities(ei.getClass()); } if(prevVil > w.countEntities(ev.getClass())) { paradoxLevel = paradoxLevel + 55; prevVil = w.countEntities(ev.getClass()); } if(prevEnd > w.countEntities(ee.getClass())) { paradoxLevel = paradoxLevel + 25; prevEnd = w.countEntities(ee.getClass()); } if(prevZpi > w.countEntities(ezp.getClass())) { paradoxLevel = paradoxLevel + 19; prevZpi = w.countEntities(ezp.getClass()); } if(prevBla > w.countEntities(ebl.getClass())) { paradoxLevel = paradoxLevel + 33; prevBla = w.countEntities(ebl.getClass()); } if(prevCsp > w.countEntities(ecs.getClass())) { paradoxLevel = paradoxLevel + 29; prevCsp = w.countEntities(ecs.getClass()); } if(prevCre > w.countEntities(ecr.getClass())) { paradoxLevel = paradoxLevel + 69; prevCre = w.countEntities(ecr.getClass()); } if(prevGha > w.countEntities(eg.getClass())) { paradoxLevel = paradoxLevel + 56; prevGha = w.countEntities(eg.getClass()); } if(prevMag > w.countEntities(emc.getClass())) { paradoxLevel = paradoxLevel + 10; prevMag = w.countEntities(emc.getClass()); } if(prevSil > w.countEntities(esi.getClass())) { paradoxLevel = paradoxLevel + 4; prevSil = w.countEntities(esi.getClass()); } if(prevSke > w.countEntities(esk.getClass())) { paradoxLevel = paradoxLevel + 34; prevSke = w.countEntities(esk.getClass()); } if(prevSli > w.countEntities(esl.getClass())) { paradoxLevel = paradoxLevel + 10; prevSli = w.countEntities(esl.getClass()); } if(prevSpi > w.countEntities(esp.getClass())) { paradoxLevel = paradoxLevel + 27; prevSpi = w.countEntities(esp.getClass()); } if(prevWit > w.countEntities(ewi.getClass())) { paradoxLevel = paradoxLevel + 49; prevWit = w.countEntities(ewi.getClass()); } if(prevZom > w.countEntities(ez.getClass())) { paradoxLevel = paradoxLevel + 20; prevZom = w.countEntities(ez.getClass()); } if(prevSheep < w.countEntities(es.getClass())) { prevSheep = w.countEntities(es.getClass()); } if(prevPig < w.countEntities(ep.getClass())) { prevPig = w.countEntities(ep.getClass()); } if(prevCow < w.countEntities(ec.getClass())) { prevCow = w.countEntities(ec.getClass()); } if(prevChick < w.countEntities(eck.getClass())) { prevChick = w.countEntities(eck.getClass()); } if(prevSqu < w.countEntities(esq.getClass())) { prevSqu = w.countEntities(esq.getClass()); } if(prevWol < w.countEntities(ew.getClass())) { prevWol = w.countEntities(ew.getClass()); } if(prevOce < w.countEntities(eo.getClass())) { prevOce = w.countEntities(eo.getClass()); } if(prevBat < w.countEntities(eb.getClass())) { prevBat = w.countEntities(eb.getClass()); } if(prevGol < w.countEntities(ei.getClass())) { prevGol = w.countEntities(ei.getClass()); } if(prevMoo < w.countEntities(em.getClass())) { prevMoo = w.countEntities(em.getClass()); } if(prevVil < w.countEntities(ev.getClass())) { prevVil = w.countEntities(ev.getClass()); } if(prevEnd < w.countEntities(ee.getClass())) { prevEnd = w.countEntities(ee.getClass()); } if(prevZpi < w.countEntities(ezp.getClass())) { prevZpi = w.countEntities(ezp.getClass()); } if(prevBla < w.countEntities(ebl.getClass())) { prevBla = w.countEntities(ebl.getClass()); } if(prevCsp < w.countEntities(ecs.getClass())) { prevCsp = w.countEntities(ecs.getClass()); } if(prevCre < w.countEntities(ecr.getClass())) { prevCre = w.countEntities(ecr.getClass()); } if(prevGha < w.countEntities(eg.getClass())) { prevGha = w.countEntities(eg.getClass()); } if(prevMag < w.countEntities(emc.getClass())) { prevMag = w.countEntities(emc.getClass()); } if(prevSil < w.countEntities(esi.getClass())) { prevSil = w.countEntities(esi.getClass()); } if(prevSke < w.countEntities(esk.getClass())) { prevSke = w.countEntities(esk.getClass()); } if(prevSli < w.countEntities(esl.getClass())) { prevSli = w.countEntities(esl.getClass()); } if(prevSpi < w.countEntities(esp.getClass())) { prevSpi = w.countEntities(esp.getClass()); } if(prevWit < w.countEntities(ewi.getClass())) { prevWit = w.countEntities(ewi.getClass()); } if(prevZom < w.countEntities(ez.getClass())) { prevZom = w.countEntities(ez.getClass()); }*/ } else { mechanics.returnToPresent(mc, paradoxLevel, server); } } if(isInPast) { if(count == 20) { seconds--; count = 0; } if(seconds == 0) { minutes--; seconds = 60; } if(minutes == 0) { text = "Time Remaining: " + seconds + " Seconds"; } if(minutes <= 0 && seconds <= 1) { mechanics.outOfTime(mc, server, text); } } } } @Override public void tickStart(EnumSet<TickType> type, Object... tickData) { if (type.equals(EnumSet.of(TickType.PLAYER))) { onServerTick(FMLServerHandler.instance().getServer(), FMLClientHandler.instance().getClient()); } } @Override public EnumSet<TickType> ticks() { return EnumSet.of(TickType.PLAYER, TickType.SERVER); } @Override public void tickEnd(EnumSet<TickType> type, Object... tickData) { } @Override public String getLabel() { return null; } }
package timeTraveler.core; import java.io.File; import java.sql.Connection; import java.sql.DriverManager; import java.sql.ResultSet; import java.sql.SQLException; import java.sql.Statement; import net.minecraft.block.Block; import net.minecraft.block.material.Material; import net.minecraft.entity.Entity; import net.minecraft.entity.EntityEggInfo; import net.minecraft.entity.EntityList; import net.minecraft.entity.EnumCreatureType; import net.minecraft.item.Item; import net.minecraft.item.ItemStack; import net.minecraft.item.crafting.FurnaceRecipes; import net.minecraft.src.ModLoader; import net.minecraft.world.biome.BiomeGenBase; import timeTraveler.blocks.BlockParadoxCondenser; import timeTraveler.blocks.BlockTimeTraveler; import timeTraveler.blocks.Collision; import timeTraveler.blocks.ParadoxExtractor; import timeTraveler.entities.EntityParadoxHunter; import timeTraveler.entities.EntityPlayerPast; import timeTraveler.gui.GuiHandler; import timeTraveler.items.BottledParadox; import timeTraveler.items.CondensedParadox; import timeTraveler.items.EmptyBottle; import timeTraveler.items.ItemParadoximer; import timeTraveler.mechanics.FutureTravelMechanics; import timeTraveler.network.TimeTravelerPacketHandler; import timeTraveler.proxies.CommonProxy; import timeTraveler.structures.StructureGenerator; import timeTraveler.ticker.ServerTickHandler; import timeTraveler.ticker.TickerClient; import timeTraveler.tileentity.TileEntityCollision; import timeTraveler.tileentity.TileEntityExtractor; import cpw.mods.fml.client.FMLClientHandler; import cpw.mods.fml.common.Mod; import cpw.mods.fml.common.Mod.Init; import cpw.mods.fml.common.Mod.Instance; import cpw.mods.fml.common.Mod.PreInit; import cpw.mods.fml.common.Mod.ServerStarted; import cpw.mods.fml.common.Mod.ServerStarting; import cpw.mods.fml.common.SidedProxy; import cpw.mods.fml.common.event.FMLInitializationEvent; import cpw.mods.fml.common.event.FMLPreInitializationEvent; import cpw.mods.fml.common.event.FMLServerStartedEvent; import cpw.mods.fml.common.event.FMLServerStartingEvent; import cpw.mods.fml.common.network.NetworkMod; import cpw.mods.fml.common.network.NetworkMod.SidedPacketHandler; import cpw.mods.fml.common.network.NetworkRegistry; import cpw.mods.fml.common.registry.EntityRegistry; import cpw.mods.fml.common.registry.GameRegistry; import cpw.mods.fml.common.registry.LanguageRegistry; import cpw.mods.fml.common.registry.TickRegistry; import cpw.mods.fml.relauncher.Side; @Mod(modid = "Charsmud_TimeTraveler", name = "Time Traveler", version = "0.1") @NetworkMod(clientSideRequired = true, serverSideRequired = false, serverPacketHandlerSpec = @SidedPacketHandler (channels = {"futuretravel", "paradoxgui"}, packetHandler = TimeTravelerPacketHandler.class)) /** * Main laucher for TimeTraveler * @author Charsmud * */ public class TimeTraveler { @SidedProxy(clientSide = "timeTraveler.proxies.ClientProxy", serverSide = "timeTraveler.proxies.CommonProxy") public static CommonProxy proxy; @Instance public static TimeTraveler instance = new TimeTraveler(); public static Block travelTime; public static Block paradoxCondenser; public static Block paradoxExtractor; public static Block collisionBlock; public static Item paradoximer; public static Item bottledParadox; public static Item condensedParadox; public static Item emptyBottle; public static final String modid = "Charsmud_TimeTraveler"; FutureTravelMechanics ftm; private GuiHandler guihandler; static int startEntityId = 300; public static UnchangingVars vars = new UnchangingVars(); /** * Initializes DeveloperCapes * @param event */ @PreInit public void preInit(FMLPreInitializationEvent event) { proxy.initCapes(); } /** * Initiates mod, registers block and item for use. Generates the necessary folders. */ @Init public void load(FMLInitializationEvent event) { proxy.registerRenderThings(); initialize(); registerBlocks(); addNames(); addRecipes(); addSmelt(); mkDirs(); registerEntities(); //sqlLite(); //villageStuff(); GameRegistry.registerTileEntity(TileEntityCollision.class, "collide"); GameRegistry.registerTileEntity(TileEntityExtractor.class, "extractor"); TickRegistry.registerTickHandler(new TickerClient(), Side.CLIENT); GameRegistry.registerWorldGenerator(new StructureGenerator()); NetworkRegistry.instance().registerGuiHandler(this, guihandler); ModLoader.registerEntityID(EntityPlayerPast.class, "PlayerPast", 100);//registers the mobs name and id // ModLoader.addSpawn(EntityPlayerPast.class, 25, 25, 25, EnumCreatureType.creature); } @ServerStarting public void onServerStart(FMLServerStartingEvent event) { TickRegistry.registerTickHandler(new ServerTickHandler(), Side.SERVER); } public static int getUniqueEntityId() { do { startEntityId++; } while (EntityList.getStringFromID(startEntityId) != null); return startEntityId; } public static void registerEntityEgg(Class<? extends Entity> entity, int primaryColor, int secondaryColor) { int id = getUniqueEntityId(); EntityList.IDtoClassMapping.put(id, entity); EntityList.entityEggs.put(id, new EntityEggInfo(id, primaryColor, secondaryColor)); } /** * Initializes Variables */ public void initialize() { paradoximer = new ItemParadoximer(2330).setUnlocalizedName("ItemParadoximer"); bottledParadox = new BottledParadox(2331).setUnlocalizedName("BottledParadox"); condensedParadox = new CondensedParadox(2332).setUnlocalizedName("CondensedParadox"); emptyBottle = new EmptyBottle(2333).setUnlocalizedName("emptyBottle"); travelTime = new BlockTimeTraveler(255).setUnlocalizedName("BlockTimeTraveler"); paradoxCondenser = new BlockParadoxCondenser(254, true).setUnlocalizedName("BlockParadoxCondenser"); paradoxExtractor = new ParadoxExtractor(253, true).setUnlocalizedName("ParadoxExtractor"); collisionBlock = new Collision(252, Material.air).setUnlocalizedName("collisionBlock"); ftm = new FutureTravelMechanics(); guihandler = new GuiHandler(); } /** * Registers Blocks */ public void registerBlocks() { GameRegistry.registerBlock(travelTime, "travelTime"); GameRegistry.registerBlock(paradoxCondenser, "paradoxCondenser"); GameRegistry.registerBlock(paradoxExtractor, "paradoxExtractor"); GameRegistry.registerBlock(collisionBlock, "collisionBlock"); } /** * Adds Names */ public void addNames() { LanguageRegistry.addName(travelTime, "Paradox Cube"); LanguageRegistry.addName(paradoximer, "Paradoximer"); LanguageRegistry.addName(paradoxCondenser, "Paradox Condenser"); LanguageRegistry.addName(bottledParadox, "Bottled Paradox"); LanguageRegistry.addName(condensedParadox, "Condensed Paradox"); LanguageRegistry.addName(paradoxExtractor, "Paradox Extractor"); LanguageRegistry.addName(emptyBottle, "Empty Bottle"); } /** * Adds Recipes */ public void addRecipes() { /*GameRegistry.addRecipe(new ItemStack(emptyBottle, 1), new Object[] { " o ", "x x", " x ", Character.valueOf('x'), Block.glass.blockID, Character.valueOf('o'), Item.clay.itemID });*/ } /** * Adds Smelting Recipes */ public void addSmelt() { FurnaceRecipes.smelting().addSmelting(Item.glassBottle.itemID, new ItemStack(TimeTraveler.emptyBottle), 0.2F); } /** * Makes the Directories needed */ public void mkDirs() { File pastCreation = new File(FMLClientHandler.instance().getClient().getMinecraftDir() + "/mods/TimeMod/past"); File presentCreation = new File(FMLClientHandler.instance().getClient().getMinecraftDir() + "/mods/TimeMod/present"); File futureCreation = new File(FMLClientHandler.instance().getClient().getMinecraftDir() + "/mods/TimeMod/future"); pastCreation.mkdirs(); presentCreation.mkdirs(); futureCreation.mkdirs(); } /** * Registers Entities */ public void registerEntities() { EntityRegistry.registerModEntity(EntityParadoxHunter.class, "ParadoxHunter", 1, this, 80, 1, true); EntityRegistry.addSpawn(EntityParadoxHunter.class, 10, 2, 4, EnumCreatureType.creature, BiomeGenBase.beach, BiomeGenBase.extremeHills, BiomeGenBase.extremeHillsEdge, BiomeGenBase.forest, BiomeGenBase.forestHills, BiomeGenBase.jungle, BiomeGenBase.jungleHills, BiomeGenBase.mushroomIsland, BiomeGenBase.mushroomIslandShore, BiomeGenBase.ocean, BiomeGenBase.plains, BiomeGenBase.river, BiomeGenBase.swampland); LanguageRegistry.instance().addStringLocalization("entity.Charsmud_TimeTraveler.ParadoxHunter.name", "Paradox Hunter"); registerEntityEgg(EntityParadoxHunter.class, 0xffffff, 0x000000); } public void sqlLite() { try { Class.forName("org.sqlite.JDBC"); Connection connection = null; try { // create a database connection connection = DriverManager.getConnection("jdbc:sqlite:" + FMLClientHandler.instance().getClient().getMinecraftDir() + "/mods/TimeMod/database.db"); Statement statement = connection.createStatement(); statement.setQueryTimeout(30); // set timeout to 30 sec. //statement.executeUpdate("drop table if exists person"); statement.executeUpdate("create table MobData (name string, x int, y int, z int)"); statement.executeUpdate("insert into MobData values('Test', 1, 2, 3)"); statement.executeUpdate("insert into MobData values('Test 2', 4, 5, 6)"); ResultSet rs = statement.executeQuery("select * from MobData"); while (rs.next()) { // read the result set System.out.println("name = " + rs.getString("name")); System.out.println("x = " + rs.getInt("x")); System.out.println("x = " + rs.getInt("y")); System.out.println("x = " + rs.getInt("z")); } } catch (SQLException e) { // if the error message is "out of memory", // it probably means no database file is found System.err.println(e.getMessage()); } finally { try { if (connection != null) { connection.close(); } } catch (SQLException e) { // connection close failed. System.err.println(e); } } } catch(Exception e) { System.err.println(e); } } }
package timeTraveler.mechanics; import java.io.BufferedWriter; import java.io.File; import java.io.FileWriter; import java.io.IOException; import java.util.List; import net.minecraft.client.Minecraft; import net.minecraft.client.gui.GuiButton; import net.minecraft.client.gui.GuiIngame; import net.minecraft.client.gui.GuiMainMenu; import net.minecraft.client.gui.ScaledResolution; import net.minecraft.client.multiplayer.WorldClient; import net.minecraft.entity.EntityLiving; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.server.MinecraftServer; import net.minecraft.stats.StatList; import net.minecraft.world.WorldSettings; import net.minecraft.world.storage.WorldInfo; import org.lwjgl.opengl.GL11; import cpw.mods.fml.client.FMLClientHandler; import timeTraveler.core.TimeTraveler; import timeTraveler.gui.GuiTimeTravel; import timeTraveler.ticker.ServerTickHandler; import timeTraveler.ticker.TickerClient; /** * Contains information about the new Mechanics for the past * @author Charsmud * */ public class PastMechanics { /** * Updates Paradox Bar and Renders * @param minecraft * @param amtOfParadox */ public void updateParadoxBar(Minecraft minecraft, int amtOfParadox) { ScaledResolution var5 = new ScaledResolution(minecraft.gameSettings, minecraft.displayWidth, minecraft.displayHeight); int var6 = var5.getScaledWidth(); int var7 = var5.getScaledHeight(); int var8 = var7 / var7 + 25; GuiIngame gig = new GuiIngame(minecraft); GL11.glBindTexture(GL11.GL_TEXTURE_2D, minecraft.renderEngine.getTexture("/timeTraveler/textureMap/newGUIElements.png")); gig.drawTexturedModalRect(var6 / 2 - 200, var8, 0, 0, 128, ; gig.drawTexturedModalRect(var6 / 2 - 200, var8, 0, 8, amtOfParadox, ; } /** * adds an EntityLiving's name and coordinates to a List. * @param par1EntityLiving * @param par2List */ public void addEntityData(List<String> par2List) { List<EntityLiving> allEntities = FMLClientHandler.instance().getClient().theWorld.loadedEntityList; for(int i = 0; i < allEntities.size(); i++) { if(allEntities.get(i) instanceof EntityLiving) { String entityName = allEntities.get(i).getEntityName(); int xCoord = (int) allEntities.get(i).posX; int yCoord = (int) allEntities.get(i).posY; int zCoord = (int) allEntities.get(i).posZ; par2List.add(entityName + "," + xCoord + "," + yCoord + "," + zCoord); } } par2List.add("===================="); } /** * Saves the list input as a .epd file (entity position data). * Also clears the list once it's done writing to the file. * @param par1List */ public void saveEntityData(List<String> par1List, MinecraftServer par2MinecraftServer) { FileWriter fstream; try { File init = new File(FMLClientHandler.instance().getClient().getMinecraftDir() + "\\mods\\TimeMod\\past\\EntityLocations\\" + par2MinecraftServer.getWorldName()); if(!init.exists()) { init.mkdirs(); } int fNumbers = new File(FMLClientHandler.instance().getClient().getMinecraftDir(), "\\mods\\TimeMod\\past\\EntityLocations\\" + par2MinecraftServer.getWorldName()).listFiles().length + 1; String time = "Time "; time = time.concat(String.format("%03d",fNumbers)); fstream = new FileWriter(FMLClientHandler.instance().getClient().getMinecraftDir() + "\\mods\\TimeMod\\past\\EntityLocations\\" + par2MinecraftServer.getWorldName() + "\\" + time + ".epd"); BufferedWriter out = new BufferedWriter(fstream); for(int i = 0; i < par1List.size(); i++) { out.write(par1List.get(i)); out.newLine(); } out.flush(); out.close(); par1List.clear(); } catch (IOException e) { e.printStackTrace(); } } /** * Saves the first timezone when world is created * @param ms */ public void firstTime(MinecraftServer ms, Minecraft minecraft) { if(ClientMethods.isSinglePlayer()) { CopyFile cf = new CopyFile(); File beginningOfWorld = new File(minecraft.getMinecraftDir() + "/mods/TimeMod/present/" + ms.getWorldName()); if(beginningOfWorld.length() == 0) { File initWorldGen = new File(minecraft.getMinecraftDir() + "/mods/TimeMod/past/" + ms.getWorldName()); initWorldGen.mkdirs(); File fi = new File(minecraft.getMinecraftDir() + "/saves/" + ms.getWorldName() + "/region"); File moveTo = new File(minecraft.getMinecraftDir() + "/mods/TimeMod/past/" + ms.getWorldName() + "/Time 001"); try { cf.copyDirectory(fi, moveTo); } catch(IOException ex) { ex.printStackTrace(); } } } } /** * Creates a Past Timezone * @param ms * @param minecraft * @param cf * */ public void saveTime(MinecraftServer ms, Minecraft minecraft, CopyFile cf) { if(ClientMethods.isSinglePlayer()) { WorldInfo we = minecraft.theWorld.getWorldInfo(); File fil = new File(minecraft.getMinecraftDir(), "mods/TimeMod/past/" + ms.getWorldName()); if(!fil.exists()) { fil.mkdir(); } int counter = new File(minecraft.getMinecraftDir(), "mods/TimeMod/past/" + ms.getWorldName()).listFiles().length + 1; //int counter = counterstart - 1; try { WorldInfo worldinfo = minecraft.theWorld.getWorldInfo(); File fi = new File(minecraft.getMinecraftDir() + "\\saves\\" + ms.getWorldName() + "\\region"); File f2 = new File (minecraft.getMinecraftDir() + "\\mods\\TimeMod\\past\\" + ms.getWorldName()); f2.mkdir(); String fname = minecraft.getMinecraftDir() + "\\mods\\TimeMod\\past\\" + ms.getWorldName() + "\\Time "; //counter = counter + 1; fname = fname.concat(String.format("%03d",counter)); File directoryToMoveTo = new File(fname); cf.copyDirectory(fi, directoryToMoveTo); System.out.println("Created a time!"); //File destination = new File(Minecraft.getMinecraftDir(), "mods/TimeMod/past"); //File zipped = new File(Minecraft.getMinecraftDir(), "mods/TimeMod/past/w1.zip"); // copyfiletime.unzip(zipped, destination); } catch(Exception ex) { ex.printStackTrace(); } } } /** * Return the player to the present from past. * @param minecraft * @param paradoxLevel * @param ms * @param minutes * @param seconds */ public void returnToPresent(Minecraft minecraft, int paradoxLevel, MinecraftServer ms) { if(ClientMethods.isSinglePlayer()) { String worldName = ms.getWorldName(); String folderName = ms.getFolderName(); WorldClient worldClient = minecraft.theWorld; GuiTimeTravel gtt = new GuiTimeTravel(); EntityPlayer ep = minecraft.thePlayer; ep.setDead(); paradoxLevel = 0; minecraft.statFileWriter.readStat(StatList.leaveGameStat, 1); minecraft.theWorld.sendQuittingDisconnectingPacket(); minecraft.loadWorld((WorldClient)null); //minecraft.displayGuiScreen(new GuiMainMenu()); File present = new File(minecraft.getMinecraftDir() + "/mods/TimeMod/present/" + ms.getWorldName()); File worldFileDest = GuiTimeTravel.staticsource; File worldFile = new File(minecraft.getMinecraftDir() + "/saves/" + ms.getWorldName() + "/region"); System.out.println(present); System.out.println(worldFileDest); System.out.println(worldFile); try { if (minecraft.getSaveLoader().canLoadWorld(worldName)) { Thread.sleep(3000); CopyFile.moveMultipleFiles(worldFile, worldFileDest); Thread.sleep(2000); CopyFile.moveMultipleFiles(present, worldFile); gtt.isInPast = false; ServerTickHandler.minutes = 1; ServerTickHandler.seconds = 10; paradoxLevel = 0; minecraft.launchIntegratedServer(folderName, worldName, (WorldSettings)null); } else { System.out.println("COULD NOT LOAD WORLD! "); } } catch (Exception ex) { ex.printStackTrace(); } } } /** * Draws the time left button * @param minecraft * @param text */ public void drawTimeTicker(Minecraft minecraft, String text) { GuiButton button = new GuiButton(0,0,5, text); button.drawButton(minecraft,0,0); } /** * Player ran out of time in the past, sends player to present * @param minecraft * @param ms * @param minutes * @param seconds * @param text */ public void outOfTime(Minecraft minecraft, MinecraftServer ms, String text) { if(ClientMethods.isSinglePlayer()) { GuiTimeTravel gtt = new GuiTimeTravel(); WorldClient worldClient = minecraft.theWorld; String worldName = ms.getWorldName(); String folderName = ms.getFolderName(); minecraft.theWorld.sendQuittingDisconnectingPacket(); minecraft.loadWorld((WorldClient)null); minecraft.displayGuiScreen(new GuiMainMenu()); File present = new File(minecraft.getMinecraftDir() + "/mods/TimeMod/present/" + ms.getWorldName()); File worldFileDest = GuiTimeTravel.staticsource; File worldFile = new File(minecraft.getMinecraftDir() + "/saves/" + ms.getWorldName() + "/region"); try { if (minecraft.getSaveLoader().canLoadWorld(worldName)) { Thread.sleep(3000); CopyFile.moveMultipleFiles(worldFile, worldFileDest); Thread.sleep(1000); CopyFile.moveMultipleFiles(present, worldFile); gtt.isInPast = false; ServerTickHandler.minutes = 1; ServerTickHandler.seconds = 10; minecraft.launchIntegratedServer(folderName, worldName, (WorldSettings)null); //minecraft.loadWorld(worldClient); } } catch (Exception ex) { ex.printStackTrace(); } } } }
And the error report:
2013-08-02 12:32:53 [iNFO] [ForgeModLoader] Forge Mod Loader version 5.2.12.712 for Minecraft 1.5.2 loading 2013-08-02 12:32:53 [iNFO] [ForgeModLoader] Java is Java HotSpot(TM) 64-Bit Server VM, version 1.7.0_09, running on Windows 7:amd64:6.1, installed at C:\Users\Will\Desktop\eclipse-jee-juno-SR2-win32-x86_64\eclipse\jre 2013-08-02 12:32:53 [iNFO] [ForgeModLoader] Managed to load a deobfuscated Minecraft name- we are in a deobfuscated environment. Skipping runtime deobfuscation 2013-08-02 12:32:56 [iNFO] [sTDOUT] 229 recipes 2013-08-02 12:32:56 [iNFO] [sTDOUT] 27 achievements 2013-08-02 12:32:56 [iNFO] [Minecraft-Client] Setting user: Player748 2013-08-02 12:32:56 [iNFO] [sTDOUT] (Session ID is -) 2013-08-02 12:32:56 [iNFO] [sTDERR] Client asked for parameter: server 2013-08-02 12:32:56 [iNFO] [Minecraft-Client] LWJGL Version: 2.4.2 2013-08-02 12:32:56 [iNFO] [MinecraftForge] Attempting early MinecraftForge initialization 2013-08-02 12:32:56 [iNFO] [sTDOUT] MinecraftForge v7.8.0.712 Initialized 2013-08-02 12:32:56 [iNFO] [ForgeModLoader] MinecraftForge v7.8.0.712 Initialized 2013-08-02 12:32:56 [iNFO] [sTDOUT] Replaced 85 ore recipies 2013-08-02 12:32:57 [iNFO] [MinecraftForge] Completed early MinecraftForge initialization 2013-08-02 12:32:57 [iNFO] [ForgeModLoader] Reading custom logging properties from C:\Users\Will\Desktop\minecraftforge-src-1.5.2-7.8.0.712 - TimeTraveler\forge\mcp\jars\config\logging.properties 2013-08-02 12:32:57 [OFF] [ForgeModLoader] Logging level for ForgeModLoader logging is set to ALL 2013-08-02 12:32:57 [iNFO] [ForgeModLoader] Searching C:\Users\Will\Desktop\minecraftforge-src-1.5.2-7.8.0.712 - TimeTraveler\forge\mcp\jars\mods for mods 2013-08-02 12:32:58 [iNFO] [ForgeModLoader] Forge Mod Loader has identified 4 mods to load 2013-08-02 12:32:58 [iNFO] [mcp] Activating mod mcp 2013-08-02 12:32:58 [iNFO] [FML] Activating mod FML 2013-08-02 12:32:58 [iNFO] [Forge] Activating mod Forge 2013-08-02 12:32:58 [iNFO] [Charsmud_TimeTraveler] Activating mod Charsmud_TimeTraveler 2013-08-02 12:32:58 [iNFO] [ForgeModLoader] Registering Forge Packet Handler 2013-08-02 12:32:58 [iNFO] [ForgeModLoader] Succeeded registering Forge Packet Handler 2013-08-02 12:32:58 [iNFO] [ForgeModLoader] Configured a dormant chunk cache size of 0 2013-08-02 12:32:59 [iNFO] [sTDOUT] https://dl.dropboxusercontent.com/u/85284082/cape.txt 2013-08-02 12:32:59 [iNFO] [sTDOUT] https://dl.dropboxusercontent.com/u/85284082/TimeTravelerCapeC.png 2013-08-02 12:32:59 [iNFO] [sTDOUT] https://dl.dropboxusercontent.com/u/85284082/TimeTravelerCapeA.png 2013-08-02 12:32:59 [iNFO] [sTDOUT] https://dl.dropboxusercontent.com/u/85284082/TimeTravelerCapeB.png 2013-08-02 12:32:59 [iNFO] [sTDOUT] https://dl.dropboxusercontent.com/u/85284082/TimeTravelerCapeD.png 2013-08-02 12:32:59 [iNFO] [sTDOUT] https://dl.dropboxusercontent.com/u/85284082/TimeTravelerCapeE.png 2013-08-02 12:33:00 [iNFO] [sTDOUT] 2013-08-02 12:33:00 [iNFO] [sTDOUT] Starting up SoundSystem... 2013-08-02 12:33:00 [iNFO] [sTDOUT] Initializing LWJGL OpenAL 2013-08-02 12:33:00 [iNFO] [sTDOUT] (The LWJGL binding of OpenAL. For more information, see http://www.lwjgl.org) 2013-08-02 12:33:00 [iNFO] [sTDOUT] OpenAL initialized. 2013-08-02 12:33:00 [iNFO] [sTDOUT] 2013-08-02 12:33:01 [iNFO] [ForgeModLoader] Forge Mod Loader has detected an older LWJGL version, new advanced texture animation features are disabled 2013-08-02 12:33:01 [iNFO] [ForgeModLoader] Not using advanced OpenGL 4.3 advanced capability for animations : OpenGL 4.3 is not available 2013-08-02 12:33:01 [iNFO] [Minecraft-Client] Found animation info for: textures/blocks/lava_flow.txt 2013-08-02 12:33:01 [iNFO] [Minecraft-Client] Found animation info for: textures/blocks/water_flow.txt 2013-08-02 12:33:01 [iNFO] [Minecraft-Client] Found animation info for: textures/blocks/fire_0.txt 2013-08-02 12:33:01 [iNFO] [Minecraft-Client] Found animation info for: textures/blocks/fire_1.txt 2013-08-02 12:33:01 [iNFO] [Minecraft-Client] Found animation info for: textures/blocks/lava.txt 2013-08-02 12:33:01 [iNFO] [Minecraft-Client] Found animation info for: textures/blocks/portal.txt 2013-08-02 12:33:01 [iNFO] [Minecraft-Client] Found animation info for: textures/blocks/water.txt 2013-08-02 12:33:02 [iNFO] [Minecraft-Client] Found animation info for: textures/items/clock.txt 2013-08-02 12:33:02 [iNFO] [Minecraft-Client] Found animation info for: textures/items/compass.txt 2013-08-02 12:33:02 [iNFO] [ForgeModLoader] Forge Mod Loader has successfully loaded 4 mods 2013-08-02 12:33:03 [iNFO] [Minecraft-Client] Found animation info for: textures/blocks/lava_flow.txt 2013-08-02 12:33:03 [iNFO] [Minecraft-Client] Found animation info for: textures/blocks/water_flow.txt 2013-08-02 12:33:03 [iNFO] [Minecraft-Client] Found animation info for: textures/blocks/BLockParadoxCondenserFront.txt 2013-08-02 12:33:03 [iNFO] [Minecraft-Client] Found animation info for: textures/blocks/BlockParadoxCondenserBack.txt 2013-08-02 12:33:03 [iNFO] [Minecraft-Client] Found animation info for: textures/blocks/BlockParadoxCondenserBot.txt 2013-08-02 12:33:03 [iNFO] [Minecraft-Client] Found animation info for: textures/blocks/BlockParadoxCondenserSide.txt 2013-08-02 12:33:03 [iNFO] [Minecraft-Client] Found animation info for: textures/blocks/fire_0.txt 2013-08-02 12:33:03 [iNFO] [Minecraft-Client] Found animation info for: textures/blocks/fire_1.txt 2013-08-02 12:33:03 [iNFO] [Minecraft-Client] Found animation info for: textures/blocks/lava.txt 2013-08-02 12:33:03 [iNFO] [Minecraft-Client] Found animation info for: textures/blocks/portal.txt 2013-08-02 12:33:03 [iNFO] [Minecraft-Client] Found animation info for: textures/blocks/water.txt 2013-08-02 12:33:04 [iNFO] [Minecraft-Client] Found animation info for: textures/items/clock.txt 2013-08-02 12:33:04 [iNFO] [Minecraft-Client] Found animation info for: textures/items/compass.txt 2013-08-02 12:33:07 [iNFO] [Minecraft-Server] Starting integrated minecraft server version 1.5.2 2013-08-02 12:33:07 [iNFO] [Minecraft-Server] Generating keypair 2013-08-02 12:33:07 [iNFO] [ForgeModLoader] Loading dimension 0 (ang) (net.minecraft.server.integrated.IntegratedServer@5e0948f1) 2013-08-02 12:33:07 [iNFO] [ForgeModLoader] Loading dimension 1 (ang) (net.minecraft.server.integrated.IntegratedServer@5e0948f1) 2013-08-02 12:33:07 [iNFO] [ForgeModLoader] Loading dimension -1 (ang) (net.minecraft.server.integrated.IntegratedServer@5e0948f1) 2013-08-02 12:33:07 [iNFO] [Minecraft-Server] Preparing start region for level 0 2013-08-02 12:33:08 [iNFO] [sTDOUT] loading single player 2013-08-02 12:33:08 [iNFO] [Minecraft-Server] Player748[/127.0.0.1:0] logged in with entity id 280 at (-279.69999998807907, 71.0, 219.30000001192093) 2013-08-02 12:33:08 [iNFO] [MinecraftForge] Attempting early MinecraftForge initialization 2013-08-02 12:33:08 [iNFO] [sTDOUT] MinecraftForge v7.8.0.712 Initialized 2013-08-02 12:33:08 [iNFO] [ForgeModLoader] MinecraftForge v7.8.0.712 Initialized 2013-08-02 12:33:08 [iNFO] [MinecraftForge] Completed early MinecraftForge initialization 2013-08-02 12:33:08 [iNFO] [sTDOUT] 2013-08-02 12:33:08 [iNFO] [sTDOUT] Time Remaining: 1 Minute, 10 Seconds 2013-08-02 12:33:08 [iNFO] [sTDOUT] 0 2013-08-02 12:33:08 [iNFO] [sTDOUT] Time Remaining: 1 Minute, 10 Seconds 2013-08-02 12:33:08 [iNFO] [sTDOUT] 0 2013-08-02 12:33:08 [iNFO] [sTDERR] net.minecraft.util.ReportedException: Ticking player 2013-08-02 12:33:08 [iNFO] [sTDERR] at net.minecraft.network.NetworkListenThread.networkTick(NetworkListenThread.java:60) 2013-08-02 12:33:08 [iNFO] [sTDERR] at net.minecraft.server.integrated.IntegratedServerListenThread.networkTick(IntegratedServerListenThread.java:109) 2013-08-02 12:33:08 [iNFO] [sTDERR] at net.minecraft.server.MinecraftServer.updateTimeLightAndEntities(MinecraftServer.java:675) 2013-08-02 12:33:08 [iNFO] [sTDERR] at net.minecraft.server.MinecraftServer.tick(MinecraftServer.java:571) 2013-08-02 12:33:08 [iNFO] [sTDERR] at net.minecraft.server.integrated.IntegratedServer.tick(IntegratedServer.java:127) 2013-08-02 12:33:08 [iNFO] [sTDERR] at net.minecraft.server.MinecraftServer.run(MinecraftServer.java:469) 2013-08-02 12:33:08 [iNFO] [sTDERR] at net.minecraft.server.ThreadMinecraftServer.run(ThreadMinecraftServer.java:16) 2013-08-02 12:33:08 [iNFO] [sTDERR] Caused by: java.lang.NullPointerException 2013-08-02 12:33:08 [iNFO] [sTDERR] at timeTraveler.mechanics.PastMechanics.firstTime(PastMechanics.java:129) 2013-08-02 12:33:08 [iNFO] [sTDERR] at timeTraveler.ticker.ServerTickHandler.onServerTick(ServerTickHandler.java:140) 2013-08-02 12:33:08 [iNFO] [sTDERR] at timeTraveler.ticker.ServerTickHandler.tickStart(ServerTickHandler.java:549) 2013-08-02 12:33:08 [iNFO] [sTDERR] at cpw.mods.fml.common.SingleIntervalHandler.tickStart(SingleIntervalHandler.java:28) 2013-08-02 12:33:08 [iNFO] [sTDERR] at cpw.mods.fml.common.FMLCommonHandler.tickStart(FMLCommonHandler.java:122) 2013-08-02 12:33:08 [iNFO] [sTDERR] at cpw.mods.fml.common.FMLCommonHandler.onPlayerPreTick(FMLCommonHandler.java:383) 2013-08-02 12:33:08 [iNFO] [sTDERR] at net.minecraft.entity.player.EntityPlayer.onUpdate(EntityPlayer.java:279) 2013-08-02 12:33:08 [iNFO] [sTDERR] at net.minecraft.entity.player.EntityPlayerMP.onUpdateEntity(EntityPlayerMP.java:326) 2013-08-02 12:33:08 [iNFO] [sTDERR] at net.minecraft.network.NetServerHandler.handleFlying(NetServerHandler.java:308) 2013-08-02 12:33:08 [iNFO] [sTDERR] at net.minecraft.network.packet.Packet10Flying.processPacket(Packet10Flying.java:51) 2013-08-02 12:33:08 [iNFO] [sTDERR] at net.minecraft.network.MemoryConnection.processReadPackets(MemoryConnection.java:89) 2013-08-02 12:33:08 [iNFO] [sTDERR] at net.minecraft.network.NetServerHandler.networkTick(NetServerHandler.java:134) 2013-08-02 12:33:08 [iNFO] [sTDERR] at net.minecraft.network.NetworkListenThread.networkTick(NetworkListenThread.java:53) 2013-08-02 12:33:08 [iNFO] [sTDERR] ... 6 more 2013-08-02 12:33:08 [sEVERE] [Minecraft-Server] Encountered an unexpected exception ReportedException net.minecraft.util.ReportedException: Ticking player at net.minecraft.network.NetworkListenThread.networkTick(NetworkListenThread.java:60) at net.minecraft.server.integrated.IntegratedServerListenThread.networkTick(IntegratedServerListenThread.java:109) at net.minecraft.server.MinecraftServer.updateTimeLightAndEntities(MinecraftServer.java:675) at net.minecraft.server.MinecraftServer.tick(MinecraftServer.java:571) at net.minecraft.server.integrated.IntegratedServer.tick(IntegratedServer.java:127) at net.minecraft.server.MinecraftServer.run(MinecraftServer.java:469) at net.minecraft.server.ThreadMinecraftServer.run(ThreadMinecraftServer.java:16) Caused by: java.lang.NullPointerException at timeTraveler.mechanics.PastMechanics.firstTime(PastMechanics.java:129) at timeTraveler.ticker.ServerTickHandler.onServerTick(ServerTickHandler.java:140) at timeTraveler.ticker.ServerTickHandler.tickStart(ServerTickHandler.java:549) at cpw.mods.fml.common.SingleIntervalHandler.tickStart(SingleIntervalHandler.java:28) at cpw.mods.fml.common.FMLCommonHandler.tickStart(FMLCommonHandler.java:122) at cpw.mods.fml.common.FMLCommonHandler.onPlayerPreTick(FMLCommonHandler.java:383) at net.minecraft.entity.player.EntityPlayer.onUpdate(EntityPlayer.java:279) at net.minecraft.entity.player.EntityPlayerMP.onUpdateEntity(EntityPlayerMP.java:326) at net.minecraft.network.NetServerHandler.handleFlying(NetServerHandler.java:308) at net.minecraft.network.packet.Packet10Flying.processPacket(Packet10Flying.java:51) at net.minecraft.network.MemoryConnection.processReadPackets(MemoryConnection.java:89) at net.minecraft.network.NetServerHandler.networkTick(NetServerHandler.java:134) at net.minecraft.network.NetworkListenThread.networkTick(NetworkListenThread.java:53) ... 6 more 2013-08-02 12:33:08 [sEVERE] [Minecraft-Server] This crash report has been saved to: C:\Users\Will\Desktop\minecraftforge-src-1.5.2-7.8.0.712 - TimeTraveler\forge\mcp\jars\.\crash-reports\crash-2013-08-02_12.33.08-server.txt 2013-08-02 12:33:08 [iNFO] [Minecraft-Server] Stopping server 2013-08-02 12:33:08 [iNFO] [Minecraft-Server] Saving players 2013-08-02 12:33:08 [iNFO] [Minecraft-Server] Saving worlds 2013-08-02 12:33:08 [iNFO] [Minecraft-Server] Saving chunks for level 'ang'/Overworld 2013-08-02 12:33:08 [iNFO] [Minecraft-Server] Saving chunks for level 'ang'/Nether 2013-08-02 12:33:08 [iNFO] [Minecraft-Server] Saving chunks for level 'ang'/The End 2013-08-02 12:33:09 [iNFO] [ForgeModLoader] Unloading dimension 0 2013-08-02 12:33:09 [iNFO] [ForgeModLoader] Unloading dimension -1 2013-08-02 12:33:09 [iNFO] [ForgeModLoader] Unloading dimension 1 2013-08-02 12:33:09 [sEVERE] [ForgeModLoader] Fatal errors were detected during the transition from SERVER_STARTED to SERVER_STOPPED. Loading cannot continue 2013-08-02 12:33:09 [sEVERE] [ForgeModLoader] mcp{7.44} [Minecraft Coder Pack] (minecraft.jar) Unloaded->Constructed->Pre-initialized->Initialized->Post-initialized->Available->Available->Available->Available->Available FML{5.2.12.712} [Forge Mod Loader] (coremods) Unloaded->Constructed->Pre-initialized->Initialized->Post-initialized->Available->Available->Available->Available->Available Forge{7.8.0.712} [Minecraft Forge] (coremods) Unloaded->Constructed->Pre-initialized->Initialized->Post-initialized->Available->Available->Available->Available->Available Charsmud_TimeTraveler{0.1} [Time Traveler] (bin) Unloaded->Constructed->Pre-initialized->Initialized->Post-initialized->Available->Available->Available->Available->Available 2013-08-02 12:33:09 [sEVERE] [ForgeModLoader] The ForgeModLoader state engine has become corrupted. Probably, a state was missed by and invalid modification to a base classForgeModLoader depends on. This is a critical error and not recoverable. Investigate any modifications to base classes outside ofForgeModLoader, especially Optifine, to see if there are fixes available. 2013-08-02 12:33:09 [iNFO] [sTDERR] Exception in thread "Server thread" java.lang.RuntimeException: The ForgeModLoader state engine is invalid 2013-08-02 12:33:09 [iNFO] [sTDERR] at cpw.mods.fml.common.LoadController.transition(LoadController.java:134) 2013-08-02 12:33:09 [iNFO] [sTDERR] at cpw.mods.fml.common.Loader.serverStopped(Loader.java:800) 2013-08-02 12:33:09 [iNFO] [sTDERR] at cpw.mods.fml.common.FMLCommonHandler.handleServerStopped(FMLCommonHandler.java:468) 2013-08-02 12:33:09 [iNFO] [sTDERR] at net.minecraft.server.MinecraftServer.run(MinecraftServer.java:531) 2013-08-02 12:33:09 [iNFO] [sTDERR] at net.minecraft.server.ThreadMinecraftServer.run(ThreadMinecraftServer.java:16) 2013-08-02 12:33:18 [iNFO] [Minecraft-Client] Stopping! 2013-08-02 12:33:19 [iNFO] [sTDOUT] 2013-08-02 12:33:19 [iNFO] [sTDOUT] SoundSystem shutting down... 2013-08-02 12:33:19 [iNFO] [sTDOUT] Author: Paul Lamb, www.paulscode.com 2013-08-02 12:33:19 [iNFO] [sTDOUT]
Any ideas?
-
You can't spawn mobs on the client.
How would I fix this? I have tried both WorldClient and World to no avail.... do I need a separate ticker for the server?
-
Hello! I am trying to spawn some mobs inside of my TickerClient. I have spawned entities using items before, but for some reason, I cannot get the mobs to spawn! At first, I thought it was because I was using WorldClient and not World, but even after switching to World instead of WorldClient, it still didn't work. Here's the related code:
private void onTickInGame(Minecraft mc) { mc.theWorld.getGameRules().setOrCreateGameRule("doMobSpawning", "false"); File allEntityData = new File(mc.getMinecraftDir() + "/mods/TimeMod/past/EntityLocations/" + FMLClientHandler.instance().getServer().getWorldName() + "/" + TimeTraveler.vars.getPastTime() + ".epd"); try { BufferedReader reader = new BufferedReader(new FileReader(allEntityData)); String line; while (((line = reader.readLine()) != null) && nextSet) { if(line.equals("====================")) { nextSet = false; } else { String[] entityData = line.split(","); String entityName = entityData[0]; int entityX = Integer.parseInt(entityData[1]); int entityY = Integer.parseInt(entityData[2]); int entityZ = Integer.parseInt(entityData[3]); //System.out.println(entityName + " " + entityX + " " + entityY + " " + entityZ); Entity pastEntity = EntityList.createEntityByName(entityName, mc.thePlayer.worldObj); if(pastEntity != null) { PathEntity path = ((EntityLiving)pastEntity).getNavigator().getPath(); if(pastEntity != null) { pastEntity.posX = (double)entityX; pastEntity.posY = (double)entityY; pastEntity.posZ = (double)entityZ; System.out.println(pastEntity); mc.thePlayer.worldObj.spawnEntityInWorld(pastEntity); } } else { EntityPlayerPast pastPlayer = new EntityPlayerPast(mc.thePlayer.worldObj); pastPlayer.posX = (double)entityX; pastPlayer.posY = (double)entityY; pastPlayer.posZ = (double)entityZ; System.out.println(pastPlayer); mc.thePlayer.worldObj.spawnEntityInWorld(pastPlayer); } //path = ((EntityLiving)pastEntity).getNavigator().getPathToXYZ((double)entityX, (double)entityY, (double)entityZ); //((EntityLiving)pastEntity).getNavigator().setPath(path, 1.0F); //((EntityLiving)pastEntity).getNavigator().tryMoveToXYZ((double)entityX, (double)entityY, (double)entityZ, 0.5F); } System.out.println(nextSet); } reader.close(); } catch (IOException e) { e.printStackTrace(); } }
-
RenderLivingEvent doesn't seem to be what I want, or I might just not be understanding it. I found this method inside of RenderLiving:
/** * Draws the debug or playername text above a living */ protected void renderLivingLabel(EntityLiving par1EntityLiving, String par2Str, double par3, double par5, double par7, int par9) { double d3 = par1EntityLiving.getDistanceSqToEntity(this.renderManager.livingPlayer); if (d3 <= (double)(par9 * par9)) { FontRenderer fontrenderer = this.getFontRendererFromRenderManager(); float f = 1.6F; float f1 = 0.016666668F * f; GL11.glPushMatrix(); GL11.glTranslatef((float)par3 + 0.0F, (float)par5 + par1EntityLiving.height + 0.5F, (float)par7); GL11.glNormal3f(0.0F, 1.0F, 0.0F); GL11.glRotatef(-this.renderManager.playerViewY, 0.0F, 1.0F, 0.0F); GL11.glRotatef(this.renderManager.playerViewX, 1.0F, 0.0F, 0.0F); GL11.glScalef(-f1, -f1, f1); GL11.glDisable(GL11.GL_LIGHTING); GL11.glDepthMask(false); GL11.glDisable(GL11.GL_DEPTH_TEST); GL11.glEnable(GL11.GL_BLEND); GL11.glBlendFunc(GL11.GL_SRC_ALPHA, GL11.GL_ONE_MINUS_SRC_ALPHA); Tessellator tessellator = Tessellator.instance; byte b0 = 0; if (par2Str.equals("deadmau5")) { b0 = -10; } GL11.glDisable(GL11.GL_TEXTURE_2D); tessellator.startDrawingQuads(); int j = fontrenderer.getStringWidth(par2Str) / 2; tessellator.setColorRGBA_F(0.0F, 0.0F, 0.0F, 0.25F); tessellator.addVertex((double)(-j - 1), (double)(-1 + b0), 0.0D); tessellator.addVertex((double)(-j - 1), (double)(8 + b0), 0.0D); tessellator.addVertex((double)(j + 1), (double)(8 + b0), 0.0D); tessellator.addVertex((double)(j + 1), (double)(-1 + b0), 0.0D); tessellator.draw(); GL11.glEnable(GL11.GL_TEXTURE_2D); fontrenderer.drawString(par2Str, -fontrenderer.getStringWidth(par2Str) / 2, b0, 553648127); GL11.glEnable(GL11.GL_DEPTH_TEST); GL11.glDepthMask(true); fontrenderer.drawString(par2Str, -fontrenderer.getStringWidth(par2Str) / 2, b0, -1); GL11.glEnable(GL11.GL_LIGHTING); GL11.glDisable(GL11.GL_BLEND); GL11.glColor4f(1.0F, 1.0F, 1.0F, 1.0F); GL11.glPopMatrix(); } }
This seems to be how names are rendered above a mob. Is this correct, and how can I adapt it to work with images?
-
Hello! I need to be able to display text or an image over a mob. Is there a way to do this? Text most likely won't be as much of an issue, but I have absolutely no idea of how to render an image over a mob like a nameplate.
-
Hm.... I've managed to get it to print inside of the method, meaning I was able to register the new packet. However, the stuff does not expand anymore!
Here's my changed code:
package timeTraveler.core; import java.io.ByteArrayInputStream; import java.io.DataInputStream; import java.io.File; import java.io.IOException; import net.minecraft.block.Block; import net.minecraft.client.Minecraft; import net.minecraft.item.Item; import net.minecraft.item.ItemStack; import net.minecraft.network.INetworkManager; import net.minecraft.network.packet.Packet250CustomPayload; import net.minecraft.server.MinecraftServer; import net.minecraft.src.ModLoader; import net.minecraft.world.World; import net.minecraftforge.common.DimensionManager; import net.minecraftforge.common.MinecraftForge; import timeTraveler.blocks.BlockTimeTraveler; import timeTraveler.entities.EntityPlayerPast; import timeTraveler.items.ItemParadoximer; import timeTraveler.mechanics.FutureTravelMechanics; import timeTraveler.proxies.CommonProxy; import timeTraveler.structures.StructureGenerator; import timeTraveler.ticker.TickerClient; import cpw.mods.fml.client.FMLClientHandler; import cpw.mods.fml.common.Mod; import cpw.mods.fml.common.Mod.Init; import cpw.mods.fml.common.Mod.PreInit; import cpw.mods.fml.common.Mod.ServerStarted; import cpw.mods.fml.common.SidedProxy; import cpw.mods.fml.common.event.FMLInitializationEvent; import cpw.mods.fml.common.event.FMLPreInitializationEvent; import cpw.mods.fml.common.event.FMLServerStartedEvent; import cpw.mods.fml.common.network.IPacketHandler; import cpw.mods.fml.common.network.NetworkMod; import cpw.mods.fml.common.network.NetworkRegistry; import cpw.mods.fml.common.network.Player; import cpw.mods.fml.common.registry.GameRegistry; import cpw.mods.fml.common.registry.LanguageRegistry; import cpw.mods.fml.common.registry.TickRegistry; import cpw.mods.fml.relauncher.Side; @Mod(modid = "Charsmud_TimeTraveler", name = "Time Traveler", version = "0.1") @NetworkMod(clientSideRequired = true, serverSideRequired = false) /** * Main laucher for TimeTraveler * @author Charsmud * */ public class TimeTraveler { @SidedProxy(clientSide = "timeTraveler.proxies.ClientProxy", serverSide = "timeTraveler.proxies.CommonProxy") public static CommonProxy proxy; public static Block travelTime; public static Item paradoximer; public static final String modid = "Charsmud_TimeTraveler"; FutureTravelMechanics ftm; /** * Initializes DeveloperCapes * @param event */ @PreInit public void preInit(FMLPreInitializationEvent event) { proxy.initCapes(); } /** * Initiates mod, registers block and item for use. Generates the necessary folders. */ @Init public void load(FMLInitializationEvent event) { proxy.registerRenderThings(); TickRegistry.registerTickHandler(new TickerClient(), Side.CLIENT); Minecraft m = FMLClientHandler.instance().getClient(); MinecraftServer ms = m.getIntegratedServer(); mkDirs(); paradoximer = new ItemParadoximer(2330).setUnlocalizedName("ItemParadoximer"); travelTime = new BlockTimeTraveler(255).setUnlocalizedName("BlockTimeTraveler"); GameRegistry.registerBlock(travelTime, "travelTime"); LanguageRegistry.addName(travelTime, "Paradox Cube"); LanguageRegistry.addName(paradoximer, "Paradoximer"); GameRegistry.registerWorldGenerator(new StructureGenerator()); GameRegistry.addRecipe(new ItemStack(travelTime, 13), new Object[] { // "x", Character.valueOf('x'), Block.dirt }); GameRegistry.addRecipe(new ItemStack(paradoximer, 13), new Object[] { "x", "s", Character.valueOf('x'), Block.wood, Character.valueOf('s'), Block.dirt }); ModLoader.registerEntityID(EntityPlayerPast.class, "PlayerPast", 100);//registers the mobs name and id // ModLoader.addSpawn(EntityPlayerPast.class, 25, 25, 25, EnumCreatureType.creature); ftm = new FutureTravelMechanics(); //MinecraftForge.EVENT_BUS.register(new EventHookContainer()); registerPackets(); } /** * Makes the Directories needed */ public void mkDirs() { File pastCreation = new File(FMLClientHandler.instance().getClient().getMinecraftDir() + "/mods/TimeMod/past"); pastCreation.mkdirs(); File presentCreation = new File(FMLClientHandler.instance().getClient().getMinecraftDir() + "/mods/TimeMod/present"); presentCreation.mkdirs(); File futureCreation = new File(FMLClientHandler.instance().getClient().getMinecraftDir() + "/mods/TimeMod/future"); } public void registerPackets() { NetworkRegistry.instance().registerChannel(new IPacketHandler() { @Override public void onPacketData(INetworkManager manager, Packet250CustomPayload packet, Player player) { DataInputStream datainputstream = new DataInputStream(new ByteArrayInputStream(packet.data)); try { System.out.println("A"); int run = datainputstream.readInt(); World world = DimensionManager.getWorld(0); if (world != null) { System.out.println("B"); for (int i = 0; i < run; i++) { ftm.expandOres(world, 1, 1, 1, 1, 1, 1, 1); ftm.expandForests(world, 2); } } } catch (IOException ioexception) { ioexception.printStackTrace(); } } }, "futuretravel", Side.SERVER); } }
package timeTraveler.mechanics; import java.util.Iterator; import java.util.Random; import net.minecraft.block.Block; import net.minecraft.block.BlockSapling; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.world.ChunkCoordIntPair; import net.minecraft.world.World; import net.minecraft.world.chunk.Chunk; import cpw.mods.fml.client.FMLClientHandler; /** * Contains information about the future mechanics * @author Charsmud * */ public class FutureTravelMechanics { EntityPlayer ep; World world; /** * Constructor */ public FutureTravelMechanics() { ep = FMLClientHandler.instance().getClient().thePlayer; world = FMLClientHandler.instance().getClient().theWorld; } /** * Main expanding ores method * @param world * @param coal * @param diamond * @param emerald * @param gold * @param iron * @param lapis * @param redstone */ public void expandOres(World world, int coal, int diamond, int emerald, int gold, int iron, int lapis, int redstone) { Iterator<ChunkCoordIntPair> iterator = world.activeChunkSet.iterator(); System.out.println("EXPANDORES"); while(iterator.hasNext()) { ChunkCoordIntPair coords = iterator.next(); //Chunk currentScanningChunk = world.getChunkFromBlockCoords((int)ep.posX, (int) ep.posZ); Chunk currentScanningChunk = world.getChunkFromChunkCoords(coords.chunkXPos, coords.chunkZPos); expandRedstone(world, currentScanningChunk, redstone); expandDiamond(world, currentScanningChunk, diamond); expandCoal(world, currentScanningChunk, coal); expandEmerald(world, currentScanningChunk, emerald); expandGold(world, currentScanningChunk, gold); expandIron(world, currentScanningChunk, iron); expandLapis(world, currentScanningChunk, lapis); /*ISaveHandler save = world.getSaveHandler(); IChunkLoader saver = save.getChunkLoader(world.provider); try { System.out.println(world); System.out.println(currentScanningChunk); saver.saveChunk(world, currentScanningChunk); } catch(MinecraftException ex) { ex.printStackTrace(); System.out.println("FAILED TO SAVE MINE"); } catch(IOException ex) { ex.printStackTrace(); System.out.println("FAILED TO SAVE IO"); }*/ } } /** * Main expanding forests method * @param world * @param size */ public void expandForests(World world, int size) { Iterator<ChunkCoordIntPair> iterator = world.activeChunkSet.iterator(); System.out.println("EXPANDFORESTS"); while(iterator.hasNext()) { ChunkCoordIntPair coords = iterator.next(); Chunk currentScanningChunk = world.getChunkFromChunkCoords(coords.chunkXPos, coords.chunkZPos); expandForest(world, currentScanningChunk, size); /* ISaveHandler save = world.getSaveHandler(); IChunkLoader saver = save.getChunkLoader(world.provider); try { saver.saveChunk(world, currentScanningChunk); } catch(MinecraftException ex) { ex.printStackTrace(); System.out.println("FAILED TO SAVE MINE"); } catch(IOException ex) { ex.printStackTrace(); System.out.println("FAILED TO SAVE IO"); }*/ } } //BELOW ARE HELPER METHODS /** * Coal ore expansion helper method * @param world * @param currentScanningChunk * @param size */ public void expandCoal(World world, Chunk currentScanningChunk, int size) { for(int i = 0; i < size; i++) { for(int x = 0; x < 15; x++) { for(int y = 0; y < 255; y++) { for(int z = 0; z < 15; z++) { if(world.blockExists(x, y, z)) { if(currentScanningChunk.getBlockID(x, y, z) == Block.oreCoal.blockID) { Random rand = new Random(); int expandX = rand.nextInt(3)-1; int expandY = rand.nextInt(3)-1; int expandZ = rand.nextInt(3)-1; System.out.println(x*16 + " " + y + " " + z*16); if(currentScanningChunk.getBlockID(Math.abs(x + expandX), Math.abs(y + expandY), Math.abs(z + expandZ)) != 0) { currentScanningChunk.setBlockIDWithMetadata(Math.abs(x + expandX), Math.abs(y + expandY), Math.abs(z + expandZ), Block.oreCoal.blockID, 0); } } } } } } } } /** * Diamond ore expansion helper method * @param world * @param currentScanningChunk * @param size */ public void expandDiamond(World world, Chunk currentScanningChunk, int size) { for(int i = 0; i < size; i++) { for(int x = 0; x < 15; x++) { for(int y = 0; y < 255; y++) { for(int z = 0; z < 15; z++) { if(world.blockExists(x, y, z)) { if(currentScanningChunk.getBlockID(x, y, z) == Block.oreDiamond.blockID) { Random rand = new Random(); int expandX = rand.nextInt(3)-1; int expandY = rand.nextInt(3)-1; int expandZ = rand.nextInt(3)-1; System.out.println(x*16 + " " + y + " " + z*16); if(currentScanningChunk.getBlockID(Math.abs(x + expandX), Math.abs(y + expandY), Math.abs(z + expandZ)) != 0) { currentScanningChunk.setBlockIDWithMetadata(Math.abs(x + expandX), Math.abs(y + expandY), Math.abs(z + expandZ), Block.oreDiamond.blockID, 0); } } } } } } } } /** * Emerald ore expansion helper method * @param world * @param currentScanningChunk * @param size */ public void expandEmerald(World world, Chunk currentScanningChunk, int size) { for(int i = 0; i < size; i++) { for(int x = 0; x < 15; x++) { for(int y = 0; y < 255; y++) { for(int z = 0; z < 15; z++) { if(world.blockExists(x, y, z)) { if(currentScanningChunk.getBlockID(x, y, z) == Block.oreEmerald.blockID) { Random rand = new Random(); int expandX = rand.nextInt(3) - 1; int expandY = rand.nextInt(3)-1; int expandZ = rand.nextInt(3)-1; System.out.println(x*16 + " " + y + " " + z*16); if(currentScanningChunk.getBlockID(Math.abs(x + expandX), Math.abs(y + expandY), Math.abs(z + expandZ)) != 0) { currentScanningChunk.setBlockIDWithMetadata(Math.abs(x + expandX), Math.abs(y + expandY), Math.abs(z + expandZ), Block.oreEmerald.blockID, 0); } } } } } } } } /** * Gold ore expansion helper method * @param world * @param currentScanningChunk * @param size */ public void expandGold(World world, Chunk currentScanningChunk, int size) { for(int i = 0; i < size; i++) { for(int x = 0; x < 15; x++) { for(int y = 0; y < 255; y++) { for(int z = 0; z < 15; z++) { if(world.blockExists(x, y, z)) { if(currentScanningChunk.getBlockID(x, y, z) == Block.oreGold.blockID) { Random rand = new Random(); int expandX = rand.nextInt(3)-1; int expandY = rand.nextInt(3)-1; int expandZ = rand.nextInt(3)-1; System.out.println(x*16 + " " + y + " " + z*16); if(currentScanningChunk.getBlockID(Math.abs(x + expandX), Math.abs(y + expandY), Math.abs(z + expandZ)) != 0) { currentScanningChunk.setBlockIDWithMetadata(Math.abs(x + expandX), Math.abs(y + expandY), Math.abs(z + expandZ), Block.oreGold.blockID, 0); } } } } } } } } /** * Iron ore expansion helper method * @param world * @param currentScanningChunk * @param size */ public void expandIron(World world, Chunk currentScanningChunk, int size) { for(int i = 0; i < size; i++) { for(int x = 0; x < 15; x++) { for(int y = 0; y < 255; y++) { for(int z = 0; z < 15; z++) { if(world.blockExists(x, y, z)) { if(currentScanningChunk.getBlockID(x, y, z) == Block.oreIron.blockID) { Random rand = new Random(); int expandX = rand.nextInt(3)-1; int expandY = rand.nextInt(3)-1; int expandZ = rand.nextInt(3)-1; System.out.println(x*16 + " " + y + " " + z*16); if(currentScanningChunk.getBlockID(Math.abs(x + expandX), Math.abs(y + expandY), Math.abs(z + expandZ)) != 0) { currentScanningChunk.setBlockIDWithMetadata(Math.abs(x + expandX), Math.abs(y + expandY), Math.abs(z + expandZ), Block.oreIron.blockID, 0); } } } } } } } } /** * Lapis ore expansion helper method * @param world * @param currentScanningChunk * @param size */ public void expandLapis(World world, Chunk currentScanningChunk, int size) { for(int i = 0; i < size; i++) { for(int x = 0; x < 15; x++) { for(int y = 0; y < 255; y++) { for(int z = 0; z < 15; z++) { if(world.blockExists(x, y, z)) { if(currentScanningChunk.getBlockID(x, y, z) == Block.oreLapis.blockID) { Random rand = new Random(); int expandX = rand.nextInt(3)-1; int expandY = rand.nextInt(3)-1; int expandZ = rand.nextInt(3)-1; System.out.println(x*16 + " " + y + " " + z*16); if(currentScanningChunk.getBlockID(Math.abs(x + expandX), Math.abs(y + expandY), Math.abs(z + expandZ)) != 0) { currentScanningChunk.setBlockIDWithMetadata(Math.abs(x + expandX), Math.abs(y + expandY), Math.abs(z + expandZ), Block.oreLapis.blockID, 0); } } } } } } } } /** * Redstone ore expansion helper method * @param world * @param currentScanningChunk * @param size */ public void expandRedstone(World world, Chunk currentScanningChunk, int size) { for(int i = 0; i < size; i++) { for(int x = 0; x < 15; x++) { for(int y = 0; y < 255; y++) { for(int z = 0; z < 15; z++) { if(world.blockExists(x, y, z)) { if(currentScanningChunk.getBlockID(x, y, z) == Block.oreRedstone.blockID) { Random rand = new Random(); int expandX = rand.nextInt(3)-1; int expandY = rand.nextInt(3)-1; int expandZ = rand.nextInt(3)-1; System.out.println(x*16 + " " + y + " " + z*16); if(currentScanningChunk.getBlockID(Math.abs(x + expandX), Math.abs(y + expandY), Math.abs(z + expandZ)) != 0) { currentScanningChunk.setBlockIDWithMetadata(Math.abs(x + expandX), Math.abs(y + expandY), Math.abs(z + expandZ), Block.oreRedstone.blockID, 0); } } if(currentScanningChunk.getBlockID(x, y, z) == Block.oreRedstoneGlowing.blockID) { Random rand = new Random(); int expandX = rand.nextInt(3)-1; int expandY = rand.nextInt(3)-1; int expandZ = rand.nextInt(3)-1; System.out.println(x*16 + " " + y + " " + z*16); if(currentScanningChunk.getBlockID(Math.abs(x + expandX), Math.abs(y + expandY), Math.abs(z + expandZ)) != 0) { currentScanningChunk.setBlockIDWithMetadata(Math.abs(x + expandX), Math.abs(y + expandY), Math.abs(z + expandZ), Block.oreRedstone.blockID, 0); } } } } } } } } /** * Forest expansion helper method * @param world * @param currentScanningChunk * @param size */ public void expandForest(World world, Chunk currentScanningChunk, int size) { for(int i = 0; i < size; i++) { for(int x = 0; x < 15; x++) { for(int y = 0; y < 250; y++) { for(int z = 0; z < 15; z++) { if(world.blockExists(x, y, z)) { if(currentScanningChunk.getBlockID(x, y, z) == Block.leaves.blockID) { Random rand = new Random(); int expandX = rand.nextInt(5) - 5; int expandY = rand.nextInt(5) - 5; int expandZ = rand.nextInt(5) - 5; System.out.println(expandY); if(currentScanningChunk.getBlockID(Math.abs(x + expandX), Math.abs(y + expandY), Math.abs(z + expandZ)) == Block.grass.blockID) { //if(currentScanningChunk.canBlockSeeTheSky(Math.abs(x + expandX), Math.abs(y + expandY), Math.abs(z + expandZ))) //{ currentScanningChunk.setBlockIDWithMetadata(Math.abs(x + expandX), Math.abs(y + expandY + 1), Math.abs(z + expandZ), Block.sapling.blockID, 0); //} ((BlockSapling)Block.sapling).growTree(world, Math.abs(x + expandX), Math.abs(y + expandY + 1), Math.abs(z + expandZ), rand); } } } } } } } } }
This works (as in the prints print), but nothing actually happens.
-
Yea, that would be it... How would I register it as an event handler? I've never actually done much with event handlers, so I'm sort of a beginner at that.
-
Hello! I have been trying to use the @ServerStarted annotation, but for some reason, it is not working. Here's my main class and the related classes:
package timeTraveler.core; import java.io.ByteArrayInputStream; import java.io.DataInputStream; import java.io.File; import java.io.IOException; import net.minecraft.block.Block; import net.minecraft.client.Minecraft; import net.minecraft.item.Item; import net.minecraft.item.ItemStack; import net.minecraft.network.INetworkManager; import net.minecraft.network.packet.Packet250CustomPayload; import net.minecraft.server.MinecraftServer; import net.minecraft.src.ModLoader; import net.minecraft.world.World; import net.minecraftforge.common.DimensionManager; import timeTraveler.blocks.BlockTimeTraveler; import timeTraveler.entities.EntityPlayerPast; import timeTraveler.items.ItemParadoximer; import timeTraveler.mechanics.FutureTravelMechanics; import timeTraveler.proxies.CommonProxy; import timeTraveler.structures.StructureGenerator; import timeTraveler.ticker.TickerClient; import cpw.mods.fml.client.FMLClientHandler; import cpw.mods.fml.common.Mod; import cpw.mods.fml.common.Mod.Init; import cpw.mods.fml.common.Mod.PreInit; import cpw.mods.fml.common.Mod.ServerStarted; import cpw.mods.fml.common.SidedProxy; import cpw.mods.fml.common.event.FMLInitializationEvent; import cpw.mods.fml.common.event.FMLPreInitializationEvent; import cpw.mods.fml.common.event.FMLServerStartedEvent; import cpw.mods.fml.common.network.IPacketHandler; import cpw.mods.fml.common.network.NetworkMod; import cpw.mods.fml.common.network.NetworkRegistry; import cpw.mods.fml.common.network.Player; import cpw.mods.fml.common.registry.GameRegistry; import cpw.mods.fml.common.registry.LanguageRegistry; import cpw.mods.fml.common.registry.TickRegistry; import cpw.mods.fml.relauncher.Side; @Mod(modid = "Charsmud_TimeTraveler", name = "Time Traveler", version = "0.1") @NetworkMod(clientSideRequired = true, serverSideRequired = false) /** * Main laucher for TimeTraveler * @author Charsmud * */ public class TimeTraveler { @SidedProxy(clientSide = "timeTraveler.proxies.ClientProxy", serverSide = "timeTraveler.proxies.CommonProxy") public static CommonProxy proxy; public static Block travelTime; public static Item paradoximer; public static final String modid = "Charsmud_TimeTraveler"; FutureTravelMechanics ftm; /** * Initializes DeveloperCapes * @param event */ @PreInit public void preInit(FMLPreInitializationEvent event) { proxy.initCapes(); } /** * Initiates mod, registers block and item for use. Generates the necessary folders. */ @Init public void load(FMLInitializationEvent event) { proxy.registerRenderThings(); TickRegistry.registerTickHandler(new TickerClient(), Side.CLIENT); Minecraft m = FMLClientHandler.instance().getClient(); MinecraftServer ms = m.getIntegratedServer(); mkDirs(); paradoximer = new ItemParadoximer(2330).setUnlocalizedName("ItemParadoximer"); travelTime = new BlockTimeTraveler(255).setUnlocalizedName("BlockTimeTraveler"); GameRegistry.registerBlock(travelTime, "travelTime"); LanguageRegistry.addName(travelTime, "Paradox Cube"); LanguageRegistry.addName(paradoximer, "Paradoximer"); GameRegistry.registerWorldGenerator(new StructureGenerator()); GameRegistry.addRecipe(new ItemStack(travelTime, 13), new Object[] { // "x", Character.valueOf('x'), Block.dirt }); GameRegistry.addRecipe(new ItemStack(paradoximer, 13), new Object[] { "x", "s", Character.valueOf('x'), Block.wood, Character.valueOf('s'), Block.dirt }); ModLoader.registerEntityID(EntityPlayerPast.class, "PlayerPast", 100);//registers the mobs name and id // ModLoader.addSpawn(EntityPlayerPast.class, 25, 25, 25, EnumCreatureType.creature); ftm = new FutureTravelMechanics(); } /** * Makes the Directories needed */ public void mkDirs() { File pastCreation = new File(FMLClientHandler.instance().getClient().getMinecraftDir() + "/mods/TimeMod/past"); pastCreation.mkdirs(); File presentCreation = new File(FMLClientHandler.instance().getClient().getMinecraftDir() + "/mods/TimeMod/present"); presentCreation.mkdirs(); File futureCreation = new File(FMLClientHandler.instance().getClient().getMinecraftDir() + "/mods/TimeMod/future"); } /** * Runs when server starts. Contains information about new packets and data about what to do with them. * @param event */ @ServerStarted public void onServerStarted(FMLServerStartedEvent event) { System.out.println("Z"); NetworkRegistry.instance().registerChannel(new IPacketHandler() { @Override public void onPacketData(INetworkManager manager, Packet250CustomPayload packet, Player player) { DataInputStream datainputstream = new DataInputStream(new ByteArrayInputStream(packet.data)); try { System.out.println("A"); int run = datainputstream.readInt(); World world = DimensionManager.getWorld(0); if (world != null) { System.out.println("B"); for (int i = 0; i < run; i++) { ftm.expandOres(world, 1, 1, 1, 1, 1, 1, 1); ftm.expandForests(world, 2); } } } catch (IOException ioexception) { ioexception.printStackTrace(); } } }, "futuretravel", Side.SERVER); } }
package timeTraveler.gui; // import java.io.ByteArrayOutputStream; import java.io.DataOutputStream; import net.minecraft.client.gui.GuiButton; import net.minecraft.client.gui.GuiScreen; import net.minecraft.client.gui.GuiTextField; import net.minecraft.client.multiplayer.WorldClient; import net.minecraft.network.packet.Packet250CustomPayload; import net.minecraft.util.StringTranslate; import org.lwjgl.input.Keyboard; import timeTraveler.mechanics.FutureTravelMechanics; import cpw.mods.fml.client.FMLClientHandler; import cpw.mods.fml.common.network.PacketDispatcher; /** * GUI for the paradoximer * @author Charsmud * */ public class GuiFutureTravel extends GuiScreen{ private GuiScreen parentGuiScreen; private GuiTextField theGuiTextField; private final String yearsIntoFuture; public GuiFutureTravel(GuiScreen par1GuiScreen, String par2Str) { this.parentGuiScreen = par1GuiScreen; this.yearsIntoFuture = par2Str; } /** * Called from the main game loop to update the screen. */ public void updateScreen() { this.theGuiTextField.updateCursorCounter(); } /** * Adds the buttons (and other controls) to the screen in question. */ public void initGui() { StringTranslate var1 = StringTranslate.getInstance(); Keyboard.enableRepeatEvents(true); this.buttonList.clear(); this.buttonList.add(new GuiButton(0, this.width / 2 - 100, this.height / 4 + 96 + 12, var1.translateKey("Travel Into the Future!"))); this.buttonList.add(new GuiButton(1, this.width / 2 - 100, this.height / 4 + 120 + 12, var1.translateKey("gui.cancel"))); this.theGuiTextField = new GuiTextField(this.fontRenderer, this.width / 2 - 100, 60, 200, 20); this.theGuiTextField.setFocused(true); this.theGuiTextField.setText(yearsIntoFuture); } /** * Called when the screen is unloaded. Used to disable keyboard repeat events */ public void onGuiClosed() { Keyboard.enableRepeatEvents(false); } /** * Fired when a control is clicked. This is the equivalent of ActionListener.actionPerformed(ActionEvent e). */ protected void actionPerformed(GuiButton par1GuiButton) { if (par1GuiButton.enabled) { if (par1GuiButton.id == 1) { this.mc.displayGuiScreen(null); } else if (par1GuiButton.id == 0) { if(theGuiTextField.getText() != "") { int run = Integer.parseInt(theGuiTextField.getText()); FutureTravelMechanics ftm = new FutureTravelMechanics(); WorldClient world = FMLClientHandler.instance().getClient().theWorld; System.out.println(run); /*for (int i = 0; i < run; i++) { ftm.expandOres(world, 1, 1, 1, 1, 1, 1, 1); ftm.expandForests(world, 2); }*/ ByteArrayOutputStream bytearrayoutputstream = new ByteArrayOutputStream(); DataOutputStream dataoutputstream = new DataOutputStream(bytearrayoutputstream); try { System.out.println(dataoutputstream + " "); dataoutputstream.writeInt(run); System.out.println(" :)"); PacketDispatcher.sendPacketToServer(new Packet250CustomPayload("futuretravel", bytearrayoutputstream.toByteArray())); System.out.println(" :) "); } catch (Exception exception) { exception.printStackTrace(); } } } } } /** * Fired when a key is typed. This is the equivalent of KeyListener.keyTyped(KeyEvent e). */ protected void keyTyped(char par1, int par2) { Character c = par1; if(c.isDigit(c)) { this.theGuiTextField.textboxKeyTyped(par1, par2); } ((GuiButton)this.buttonList.get(0)).enabled = this.theGuiTextField.getText().trim().length() > 0; if (par1 == 13) { this.actionPerformed((GuiButton)this.buttonList.get(0)); } } /** * Called when the mouse is clicked. */ protected void mouseClicked(int par1, int par2, int par3) { super.mouseClicked(par1, par2, par3); this.theGuiTextField.mouseClicked(par1, par2, par3); } /** * Draws the screen and all the components in it. */ public void drawScreen(int par1, int par2, float par3) { StringTranslate var4 = StringTranslate.getInstance(); this.drawDefaultBackground(); this.drawCenteredString(this.fontRenderer, var4.translateKey("Future Travel"), this.width / 2, this.height / 4 - 60 + 20, 16777215); this.drawString(this.fontRenderer, var4.translateKey("Years"), this.width / 2 - 100, 47, 10526880); this.theGuiTextField.drawTextBox(); super.drawScreen(par1, par2, par3); } }
Any ideas as to why this isn't working?
Render Nameplate or image above mob
in Modder Support
Posted
OK, thanks.
I'll see if I can get this to work.