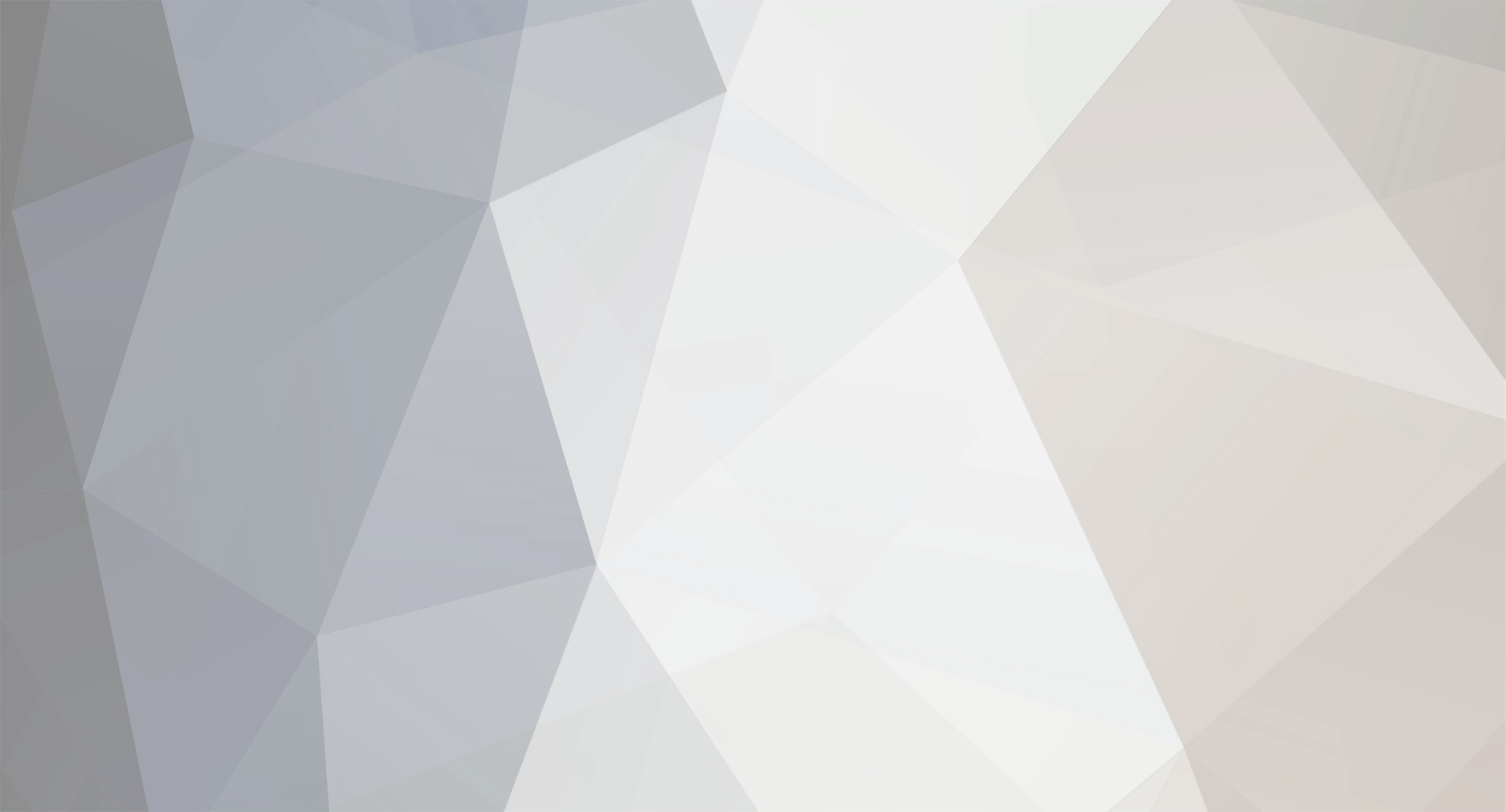
TheGreyGhost
Members-
Posts
3280 -
Joined
-
Last visited
-
Days Won
8
Everything posted by TheGreyGhost
-
Hi Hmmm I don't see anything obviously wrong with that. I've always registered my handlers in @EventHandler public void load(FMLInitializationEvent event) { } so it might be worth trying that. (Have no idea about ClientProxy.registerRenderers. Try a breakpoint there?). It's fine to have an argument for your RenderSpear constructor (see RenderManager eg this.entityRenderMap.put(EntityPig.class, new RenderPig(new ModelPig(), new ModelPig(0.5F), 0.7F) ); And no, the constructor doesn't call doRender. That happens in RenderManager.renderEntityWithPosYaw public void renderEntityWithPosYaw(Entity entityToRender, double x, double y, double z, float yaw, float partialTickTime) { Render render = null; try { render = this.getEntityRenderObject(entityToRender); // retrieves your custom renderer here if (render != null && this.renderEngine != null) { // snip try { render.doRender(entityToRender, x, y, z, yaw, partialTickTime); } catch (Throwable throwable1) { throw new ReportedException(CrashReport.makeCrashReport(throwable1, "Rendering entity in world")); } If you're feeling up to it, you could put a breakpoint on the getEntityRenderObject above, then trace into it and examine the entityRenderMap to see if your entity has been properly registered in it or not. Other useful spots for breakpoints: (1) RenderingRegistry.registerEntityRenderingHandler and (2) RenderingRegistry.loadEntityRenderers (copies your registered handlers into the vanilla rendering map.) Perhaps your code never makes it to (1). Or alternatively, it gets to (2) before (1) because your handler is registering too late -TGG
-
[SOLVED] [1.6.4] Custom Sounds Not Working
TheGreyGhost replied to saxon564's topic in Modder Support
"lay diamond" huh? Those poor chickens. Hate to think how you're going to record the sound for that :-) -
No worries :-)
-
Hi If it's not being called, that means you almost certainly haven't registered the renderer properly. Show us the part of your code where you do this? : RenderingRegistry.registerEntityRenderingHandler(NameOfYourEntityClass.class, new NameOfYourRenderer()); Once you have registered your handler, the vanilla code will call it whenever it needs to draw your Entity. You don't call it yourself (that's what "handler" means). -TGG PS /** * Renders the specified entity with the passed in position, yaw, and partialTickTime. Args: entity, x, y, z, yaw, * partialTickTime */ public void doRender(Entity entityToRender, double x, double y, double z, float yaw, float partialTickTime)
-
Hi My guess is that onItemRightClick is being called twice - once from server, once from client, so you are getting two chat messages. Should be easy enough to test? -TGG
-
Hi Have you tried putting a breakpoint or System.out.println("doRender"); in RenderSpear.doRender, to see if it's actually being called? -TGG
-
Alpha Blending with DynamicTexture (in a GUI)
TheGreyGhost replied to Draco18s's topic in Modder Support
Keen, glad it worked. only stumbled over it myself by accident when trying to figure out how block transparency works. -TGG -
Hi keen, that's a useful clue. The effect of GL11.glTranslate(0.0f, 0.0f, -1.0f) shows that there is almost certainly another part of the code which is drawing an opaque rectangle over the top of your alpha rectangle. So ideally it would be best to go find that and turn it off, rather than "fix" it with the translate. Perhaps you could trace through step by step with a debugger to see if another rect is drawn over the top of yours. Or alternatively you could look through the I don't have forge on this pc, perhaps there is an obvious method like "drawBackground" or something. Also - perhaps you need to "cancel" the event before returning from it, to stop the vanilla code from drawing the normal gui screen? -TGG
-
Hi That suggests to me that the reason your transparent rectangle is not transparent is because another part of the GUI is drawing over the top of your transparent rectangle. GL11.glLoadIdentity might be making it render somewhere offscreen. Instead of GL11.glLoadIdentity(), you could try a GL11.glTranslatef or GL11.glScalef to see whether you get both your alpha rectangle and something else on the screen too. Might be a useful clue. -TGG
-
Particles stop spawning! Server/Client desynch?
TheGreyGhost replied to Frepo's topic in Modder Support
Hi There's a bit more background about the Client-Server division here that might help http://greyminecraftcoder.blogspot.com/2013/10/the-most-important-minecraft-classes.html http://greyminecraftcoder.blogspot.com/2013/10/client-server-communication-using.html http://greyminecraftcoder.blogspot.com.au/2013/10/client-side-class-linkage-map.html http://greyminecraftcoder.blogspot.com/2013/10/server-side-class-linkage-map.html To be honest I also find it difficult to be sure when I have to do something on one side only. Some are obvious (such as rendering) but often it's not. I use vanilla code as a guideline, i.e. I find a block or item or whatever in the vanilla code that does something similar to what I want, and see whether they are bothering with isRemote. If I'm not sure about a particular method, I'll search for all usages of it in the code and look to see if they're called exclusively from one side or from both. -TGG -
hmmm yeah you're right I'm out of ideas then, sorry! :-| -TGG
-
Hi Try GL11.glEnable(GL11.GL_BLEND); at the start of your method. This turns on the alpha blending function. -TGG PS your English is fine, I understood you no problem :-)
-
Hi So - if you set your breakpoint in onEaten, what do you see in the stack trace? Should look something like this Server thread@2890, prio=5, in group 'main', status: 'RUNNING' at net.minecraft.item.ItemFood.onEaten(ItemFood.java:56) at net.minecraft.item.ItemStack.onFoodEaten(ItemStack.java:181) at net.minecraft.entity.player.EntityPlayer.onItemUseFinish(EntityPlayer.java:467) at net.minecraft.entity.player.EntityPlayerMP.onItemUseFinish(EntityPlayerMP.java:903) at net.minecraft.entity.player.EntityPlayer.onUpdate(EntityPlayer.java:296) at net.minecraft.entity.player.EntityPlayerMP.onUpdateEntity(EntityPlayerMP.java:324) at net.minecraft.network.NetServerHandler.handleFlying(NetServerHandler.java:301) at net.minecraft.network.packet.Packet10Flying.processPacket(Packet10Flying.java:51) at net.minecraft.network.MemoryConnection.processReadPackets(MemoryConnection.java:89) at net.minecraft.network.NetServerHandler.networkTick(NetServerHandler.java:138) at net.minecraft.network.NetworkListenThread.networkTick(NetworkListenThread.java:54) at net.minecraft.server.integrated.IntegratedServerListenThread.networkTick(IntegratedServerListenThread.java:109) at net.minecraft.server.MinecraftServer.updateTimeLightAndEntities(MinecraftServer.java:689) at net.minecraft.server.MinecraftServer.tick(MinecraftServer.java:585) at net.minecraft.server.integrated.IntegratedServer.tick(IntegratedServer.java:129) at net.minecraft.server.MinecraftServer.run(MinecraftServer.java:482) at net.minecraft.server.ThreadMinecraftServer.run(ThreadMinecraftServer.java:16) Then hit resume and it will break again immediately (assuming your code really is being called twice), then look at the stack trace again and see if it is being called from a different place. -TGG
-
Alpha Blending with DynamicTexture (in a GUI)
TheGreyGhost replied to Draco18s's topic in Modder Support
Hi Try GL11.glDisable(GL11.GL_ALPHA_TEST); The Alpha test threshold is set in a few places eg Minecraft.loadScreen() and Minecraft.startGame(); GL11.glAlphaFunc(GL11.GL_GREATER, 0.1F); (0.1F being 0.1 * 256 = 26) -TGG -
[1.6.4] [SOLVED] Making Mob Only Follow Tempt By Owner
TheGreyGhost replied to saxon564's topic in Modder Support
Hi Could you please show your chick entity class? If it derives from EntityTameable (like wolf or ocelot), then it will be available from your EntityCreature http://greyminecraftcoder.blogspot.com/2013/10/the-most-important-minecraft-classes_9.html Otherwise, you will need to add your own field to your chick entity class to keep track of the owner. Or alternatively, add it to the constructor for ChickAITempt public ChickAITempt(EntityCreature temptedEntityInit, EntityPlayer owner, double movementSpeedInit, int temptingItemIDInit, boolean scaredByPlayerMovementInit) { super(temptedEntityInit, movementSpeedInit, temptingItemIDInit, scaredByPlayerMovementInit); this.ownerName = .getCommandSenderName(); } private String ownerName = new String(); } etc Have a look at the code for EntityTameable - around setOwner, getOwner (etc). It uses a DataWatcher object to keep track of who the owning player is - otherwise it will "forget" every time you save the game. You will need to add these methods to your chick Class if you want the same "tame" functions. -TGG -
Hi 1) For a quick and dirty see http://www.minecraftforge.net/forum/index.php/topic,7403.msg37894.html#msg37894 - you could change the textures to completely transparent See also RenderGlobal.renderSky (you can hook into the skyrenderer... easiest way is to copy the renderSky code into your own IRenderHandler, register it, and tweak the code. 2) No idea, never done that :-( -TGG
-
Hi You've got the right idea I think, but the order of your flag in your code is wrong. you need to set it to true immediately BEFORE the call to travelToDimension, and then back to false immediately afterwards. Also, travelToDimension creates a new PlayerEntity in the new dimension and I think that the new entity might not be populated with NBT data before calling the event, so the flag is being reset to false every time. Instead, you could consider a static variable in your mod class to store the flag public static boolean teleporting; I'm pretty sure that the flow of the code will make it impossible for a second player to start teleporting before the first is finished. If this is a problem, you could use an array or set to store the currently-teleporting player names instead. -TGG
-
Particles stop spawning! Server/Client desynch?
TheGreyGhost replied to Frepo's topic in Modder Support
Hi A suggestion - try adding System.out.println("{log msg here}"); at key points in your code, eg @SideOnly(Side.CLIENT) public void randomDisplayTick(World par1World, int par2, int par3, int par4, Random par5Random) { TileEntityCampfire tileentity = (TileEntityCampfire)par1World.getBlockTileEntity(par2, par3, par4); System.out.println("randomDisplayTick"); if (tileentity != null && tileentity instanceof TileEntityCampfire) { System.out.println("tileentity correct"); tileentity.validate(); tileentity.generateCampfireFlames(par1World, par2, par3, par4, par5Random); } } That should quickly help you narrow down which part is not working as you expect - eg the randomDisplayTicks stop, your entity disappears, isBurning() becomes false, etc. -TGG -
[solved]Sync client and server Extended Properties
TheGreyGhost replied to Bedrock_Miner's topic in Modder Support
Hi Just something I noticed you said It's really important for you to understand the difference between object1 == object2 and object1.equals(object2) If you don't, it will cause you endless head damage in Java. http://www.javabeat.net/2013/02/what-is-difference-between-equals-and/# http://java67.blogspot.com.au/2012/11/difference-between-operator-and-equals-method-in.html -TGG -
[1.6.4] [SOLVED] Making Mob Only Follow Tempt By Owner
TheGreyGhost replied to saxon564's topic in Modder Support
Hi Try this constructor public ChickAITempt(EntityCreature temptedEntityInit, double movementSpeedInit, int temptingItemIDInit, boolean scaredByPlayerMovementInit) { super(temptedEntityInit, movementSpeedInit, temptingItemIDInit, scaredByPlayerMovementInit); } The function func_130012_q is already defined for a tameable entity. It might be called EntityTameable.getOwnerEntity in your version of Forge. No need to add it to your ChickAITempt -TGG -
Hi From the stack trace it looks like your PlayerEventHandler is being called in a recursive loop. i.e. your code fragment is being triggered in response to the EntityJoinWorldEvent, which calls the code fragment, which triggers the EntityJoinWorldEvent, which calls the code fragment.... Your code fragment is inside an event handler, yes? I'd suggest you either remove your code fragment to somewhere outside of the event handler, you split your event handler up (if it's catching multiple events) or you set a flag in the player or elsewhere to prevent recursively calling the player.travelToDimension(0). -TGG
-
no worries, you're welcome, it was fun to try and figure it out :-) -TGG
-
[SOLVED]Custom mob increased maximum movement speed?
TheGreyGhost replied to code4240's topic in Modder Support
Hi I don't know the answer, but have you tried inserting a breakpoint in a couple of key locations and seeing what happens/has happened to your settings? eg EntityLivingBase.moveEntityWithHeading EntityLivingBase.onLivingUpdate EntityMoveHelper.onUpdateMoveHelper Entity.moveEntity PathNavigate.onUpdateNavigation -TGG