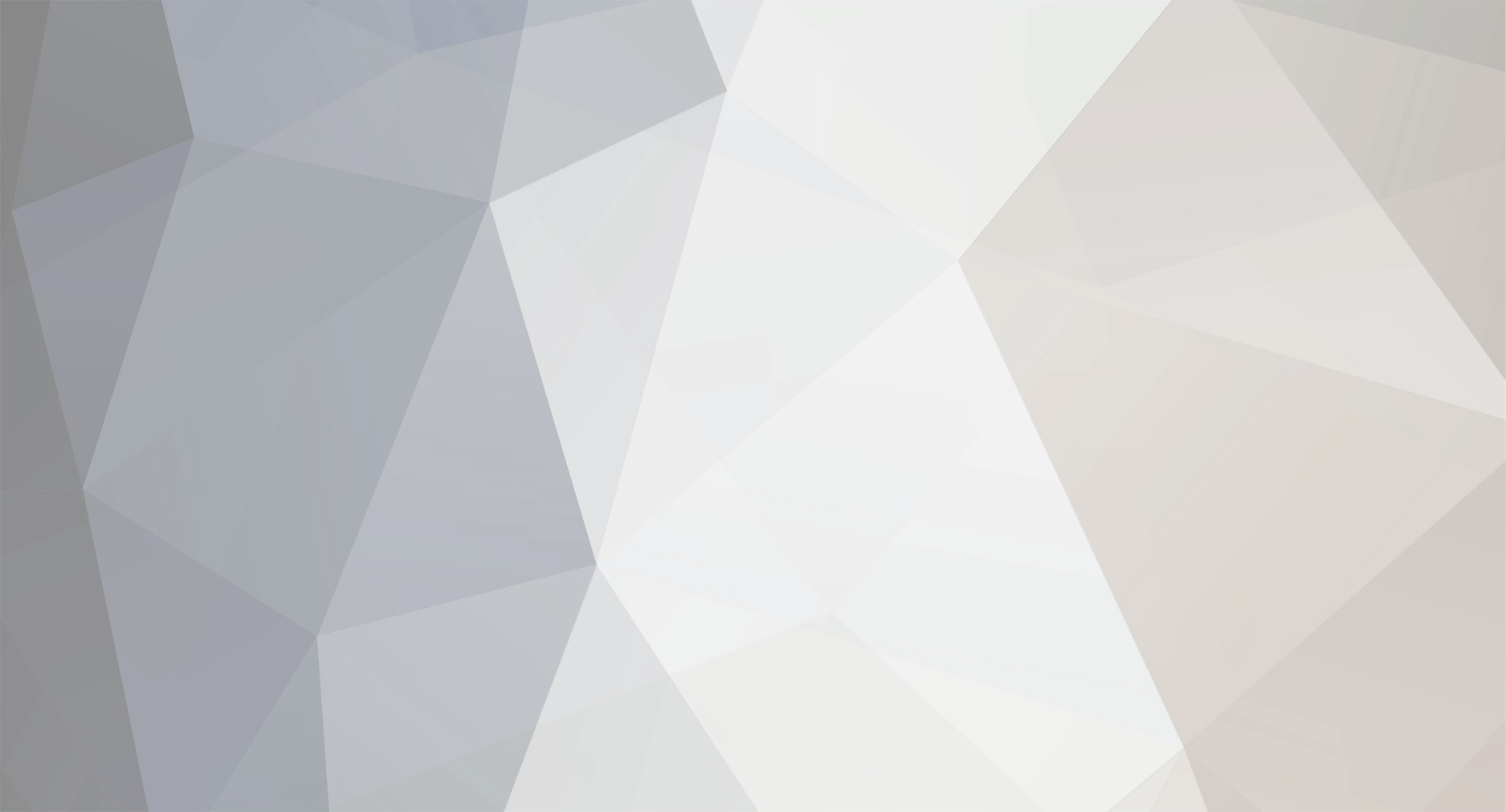
TheGreyGhost
Members-
Posts
3280 -
Joined
-
Last visited
-
Days Won
8
Everything posted by TheGreyGhost
-
Hi Beats me! Have you tried looking in EntityRenderer.orientCamera() to see if you can figure it out using breakpoints? I also notice an interesting setting this.mc.gameSettings.debugCamEnable which might get you the control you need? -TGG
-
[1.6.4] [SOLVED] Making Mob Only Follow Tempt By Owner
TheGreyGhost replied to saxon564's topic in Modder Support
Hi Perhaps you could create a new AI class from the existing one? eg if you want the pet to follow the closest person if it is the owner and is holding a diamond: class MyEntityAITempt extends EntityAITempt { @Override public boolean shouldExecute() { if (this.delayTemptCounter > 0) { --this.delayTemptCounter; return false; } else { this.temptingPlayer = this.temptedEntity.worldObj.getClosestPlayerToEntity(this.temptedEntity, 10.0D); /* get owner of a pet EntityTameable:: public EntityLivingBase func_130012_q() { return this.worldObj.getPlayerEntityByName(this.getOwnerName()); } */ if (this.temptingPlayer != this.func_130012_q()) return false; if (this.temptingPlayer == null) { return false; } else { ItemStack itemstack = this.temptingPlayer.getCurrentEquippedItem(); return itemstack == null ? false : itemstack.itemID == this.breedingFood; } } } I haven't tested this but I'm pretty sure it will work.... -TGG -
Hi Actually I'm not sure that the smelting time can be shortened in the vanilla furnace. The code below suggests to me that it's fixed at 10 seconds (200 ticks). I don't know why your code fragment causes Minecraft to crash when you place the block. How were you intending to use finishTime? -TGG TileEntityFurnace:: public void updateEntity() { boolean flag = this.furnaceBurnTime > 0; boolean flag1 = false; // snip if (this.isBurning() && this.canSmelt()) { ++this.furnaceCookTime; if (this.furnaceCookTime == 200) { this.furnaceCookTime = 0; this.smeltItem(); flag1 = true; } }
-
Hi The client/server side is pretty important to understand. These links might help... http://greyminecraftcoder.blogspot.com.au/2013/10/the-most-important-minecraft-classes.html http://greyminecraftcoder.blogspot.com.au/2013/10/client-server-communication-using.html -TGG
-
Hi Interesting. I tried it in my mod and it works fine. When I'm holding an item, left and right are swapped. When I'm not holding an item, left and right are normal. -TGG /** * User: The Grey Ghost * Date: 10/11/13 */ public class ConfusedMovementInput extends MovementInput { public ConfusedMovementInput(MovementInput interceptedMovementInput) { underlyingMovementInput = interceptedMovementInput; } @Override public void updatePlayerMoveState() { underlyingMovementInput.updatePlayerMoveState(); this.jump = underlyingMovementInput.jump; this.sneak = underlyingMovementInput.sneak; if (!confused) { this.moveStrafe = underlyingMovementInput.moveStrafe; this.moveForward = underlyingMovementInput.moveForward; } else { this.moveStrafe = -underlyingMovementInput.moveStrafe; //swap left and right this.moveForward = underlyingMovementInput.moveForward; } } public void setConfusion(boolean newConfused) { confused = newConfused; } protected MovementInput underlyingMovementInput; private boolean confused = false; } /** * User: The Grey Ghost * Date: 2/11/13 */ public class ClientTickHandler implements ITickHandler { public EnumSet<TickType> ticks() { return EnumSet.of(TickType.CLIENT); } public void tickStart(EnumSet<TickType> type, Object... tickData) { if (!type.contains(TickType.CLIENT)) return; EntityClientPlayerMP player = Minecraft.getMinecraft().thePlayer; if (player != null) { if (player.movementInput instanceof ConfusedMovementInput) { } else { SpeedyToolsMod.confusedMovementInput = new ConfusedMovementInput(player.movementInput); player.movementInput = SpeedyToolsMod.confusedMovementInput; } ItemStack heldItem = player.getHeldItem(); if (heldItem != null) { SpeedyToolsMod.confusedMovementInput.setConfusion(true); } else { SpeedyToolsMod.confusedMovementInput.setConfusion(false); } } } public void tickEnd(EnumSet<TickType> type, Object... tickData) { } } public String getLabel() { return "ClientTickHandler"; } } @Mod(modid="SpeedyToolsMod", name="Speedy Tools Mod", version="0.0.1") @NetworkMod(clientSideRequired=true, serverSideRequired=true, channels={"SpeedyTools"}, packetHandler = PacketHandler.class) public class SpeedyToolsMod { // The instance of your mod that Forge uses. @Mod.Instance("SpeedyToolsMod") public static speedytools.SpeedyToolsMod instance; public static ConfusedMovementInput confusedMovementInput; }
-
Hi You can just copy the entire project directory, with all the files including Minecraft and forge and all the rest, to a new directory, and then work in that directory. No need to download all the forge stuff again. To be honest, if you're not familiar with this then it's probably worth creating a couple of small practice projects ("Hello World" type stuff from a beginner's guide to Eclipse or something) to get you up to speed on how projects and directory structure works and how to get the most from the Eclipse development environment. You'll really be struggling otherwise. -TGG
-
Loading texture from external file into a blockIcon?
TheGreyGhost replied to Mazetar's topic in Modder Support
Hi I would be very surprised if you couldn't use GL11 to replace part of the texture sheet with a new texture (not that I know how to do it) - that might be a third option. Or a fourth - the TextureStitchEvent might also be a good place to look, you could search the hashMap for the texture you're interested in replacing, then overwrite it with a path to a different asset. Disclaimer: this is just guessing on my part. And last time I tried to delve into textures and resourcelocations it properly did my head in. -TGG -
:-) got there in the end, glad you solved the mystery -TGG
-
Crash on world load, java out of bounds exception
TheGreyGhost replied to Deco's topic in Modder Support
aiiiieeeeee that bites at least you fixed the problem :-) -TGG -
Hi This is some background info that might be useful... http://greyminecraftcoder.blogspot.com.au/2013/10/user-input.html EntityPlayerSP.movementInput uses the method MovementInputFromOptions.updatePlayerMoveState(), so if you create your own class ConfusedMovementInput extends MovementInputFromOptions, and store it in EntityPlayerSP.movementInput, you can turn confusion on or off eg public class ConfusedMovementInput extends MovementInputFromOptions { public ConfusedMovementInput(MovementInput interceptedMovementInput) { underlyingMovementInput = interceptedMovementInput; } @Override public void updatePlayerMoveState() { underlyingMovementInput.updatedPlayerMoveState(); this.jump = underlyingMovementInput.jump; this.sneak = underlyingMovementInput.sneak; if (!confused) { this.moveStrafe = underlyingMovementInput.moveStrafe; this.moveForward = underlyingMovementInput.moveForward; } else { // swap moveStrafe, moveForward, or make them negative, etc } } public void setConfusion(boolean newConfused) { confused = newConfused; } protected MovementInput underlyingMovementInput; private boolean confused = false; } And somewhere else in your code you execute ConfusedMovementInput myInput = new ConfusedMovementInput(entityPlayerSP.movementInput); entityPlayerSP.movementInput =myInput; and then myInput.setConfusion(true/false) to turn confusion on or off. You can access entityPlayerSP from Minecraft.getMinecraft().thePlayer I haven't tried to execute this code, but you get the idea? The mix of composition and inheritance is a bit clumsy because of the private GameSettings but it should still work. I have used something very similar for other keybindings (https://gist.github.com/TheGreyGhost/7033821) and it worked great. -TGG
-
Hi In 1.6.4, moveForward and moveStrafing are only used when you are riding (Packet27). They are used to transmit which movement keys the player is pressing. -TGG
-
Hi I don't know of a direct method. There is a Packet27PlayerInput - Transmit the current state of the player movement - but I think it's only used for riding at the moment. Two suggestions (which might or might not work depending on what you are trying to do and how responsive it needs to be)- Use the ITickHandler on the server to record the player's position every tick, compare it to the last position to see whether the position has changed much. But that might not work properly if the network is a bit laggy. Depending on what you need to know, it might be ok if you can smooth it out (say - over a second). Alternatively, you could add some code on the client side that sends custom packets to the server when the player moves (when the player is holding down a movement key) and when they stop moving (release all movement keys). -TGG
-
RenderGameOverlayEvent messes up the healthbar
TheGreyGhost replied to JavaPortals's topic in Modder Support
Hi I think you need to check for the Element Type and only render in the appropriate one. If that doesn't work, a wild guess: try @ForgeSubscribe(priority = EventPriority.NORMAL) public void onRender(RenderGameOverlayEvent event) { GL11.glPushMatrix(); this.drawString(this.getMinecraft().fontRenderer, "Test", 4, 4, 0xFFFFFF); GL11.glPopMatrix(); } -TGG -
Hi You could look in EntityAINearestAttackableTarget for inspiration. It does pretty much exactly what Mazetar described. and for homing it EntityAIMoveTowardsTarget -TGG
-
Probably not Possible - Getting a world reference
TheGreyGhost replied to Draco18s's topic in Modder Support
Hi During my browsings I have noticed something called FMLPacket which splits its data into packets of 32000 bytes and reassembles at the other end. That's all I know (didn't look further) but it might be of interest to you? -TGG -
Hi Well I'm stumped now. I can't see any problem in the code you've got here. Perhaps if you add a few diagnostic statements it might become clearer, eg see below. If you run it, equip and unequip, then paste the relevant bits of your console output to here, I'll have a look and see if there are any clues... -TGG if(playerNBT != null) { // etc } System.out.print( "lastSlots[0]:" + (this.lastSlots[0] == null) ? "null" : this.lastSlots[0].itemID +", currentSlots[0]:" + (this.currentSlots[0] == null) ? "null" : this.currentSlots[0]); for(int i = 0;i < 7;i++) { ItemStack itemStack1 = this.lastSlots[i]; ItemStack itemStack2 = this.currentSlots[i]; if(!ItemStack.areItemStacksEqual(itemStack1, itemStack2)) { if (i == 0) System.out.print("not equal "); if(itemStack2 != null && itemStack2.getItem() instanceof IAccessory) { if (i == 0) System.out.print(" equip"); IAccessory accessory = (IAccessory)this.currentSlots[i].getItem(); accessory.equipped(player); } if(itemStack1 != null && itemStack1.getItem() instanceof IAccessory) { if (i == 0) System.out.print(" unequip"); IAccessory accessory = (IAccessory)this.lastSlots[i].getItem(); accessory.unequipped(player); } } } System.out.println(" copied"); System.arraycopy(this.currentSlots, 0, this.lastSlots, 0, this.lastSlots.length);
-
Wow that is very strange, that stub is very simple. I might give it a go on my PCs here. Does it play on both clients regardless of which client you originate the sound from? (i.e. the one with the integrated server, and the one without)? Perhaps you could try putting a breakpoint on one of the clients just before the sound (pause the client thread only, not server thread), then step into the vanilla code line by line and see when the other client goes baa. Might give a clue. -TGG
-
Hi Have you tried putting a breakpoint in the rendering code to see what is happening? BlockDoor uses a special renderer (renderType = 7) and since you're deriving from BlockDoor, it will probably be calling the same renderer. Under some conditions the door doesn't render at all (if the adjacent block doesn't have the same ID). To be honest I don't understand exactly what it's doing there, but it might be causing your problem. RenderBlocks.renderBlockDoor(): public boolean renderBlockDoor(Block par1Block, int par2, int par3, int par4) { Tessellator tessellator = Tessellator.instance; // add breakpoint here int l = this.blockAccess.getBlockMetadata(par2, par3, par4); if ((l & != 0) { if (this.blockAccess.getBlockId(par2, par3 - 1, par4) != par1Block.blockID) { return false; } } else if (this.blockAccess.getBlockId(par2, par3 + 1, par4) != par1Block.blockID) { return false; } -TGG
-
Crash on world load, java out of bounds exception
TheGreyGhost replied to Deco's topic in Modder Support
Hi Not sure I understand - how did you manage to do this, if you didn't use a potion effect (eg .setPotionEffect)? It's possible you did something during development that has applied a weird potionID to your player. Every time the world reloads, it tries to use this saved potionID which causes the out of bounds. Probably easiest to create a new world. The problem will probably go away (unless something else in your code introduces it again). -TGG -
Hi I think the problem is the same type as the one we just solved if (this.lastSlots[i] != this.currentSlots[i]) i.e. this statement compares if the two references refer to the same objects, not if the contents of the objects are identical. And since you are filling currentSlots with new ItemStacks every tick.... ItemStack itemStack = ItemStack.loadItemStackFromNBT(nbt); this.currentSlots[i] = itemStack; Two ItemStacks can be compared for equality using ItemStack.areItemStacksEqual (checks if itemID, stackSize, damage, and stackTagCompound are all equal). Or you can just compare this.lastSlots.itemID. Depends on what you mean by "unequip" - eg if your item gets damaged by using it, does this count as an unequip? -TGG
-
Probably not Possible - Getting a world reference
TheGreyGhost replied to Draco18s's topic in Modder Support
keen. Let us know how it goes? -TGG -
Hi What I mean is - 1) Navigate through the vanilla code to the FolderResourcePack class. 2) Find the makeFullPath method 3) add this statement to the method: System.out.println("FolderResourcePack.makeFullPath:" + this.basePath + " , " + par1Str); // add this 4) Repeat for FileResoucePack.makeFullPath When you run minecraft, it should print a couple of helpful messages to the log. If your FolderResourcePack.makeFullPath doesn't look like this (it changed recently), paste the method in to show us which one you've got -TGG
-
Probably not Possible - Getting a world reference
TheGreyGhost replied to Draco18s's topic in Modder Support
Teleport them to a safe place in the new world, then change their rendering viewpoint to correspond to the portal location? -TGG -
Making a block orient towards the play on block place
TheGreyGhost replied to DJFergy's topic in Modder Support
Hi I think there are a couple of concepts in here that might help you http://greyminecraftcoder.blogspot.com.au/2013/07/rendering-standard-blocks-cubes.html So based on your current getIcon code, you need to set your metadata to match the side that the grill is on. i.e. 0 for bottom, 1 for top, 5 for east, etc. This line int rotation = MathHelper.floor_double((double)(entity.rotationYaw * 4F / 360F) * (double)(entity.rotationPitch * 1F / 180F)) & 3; will give you something completely different. I'd suggest you change it to match the algorithm in my earlier post. To be honest I don't know offhand what the 0 pitch value is, but it should be easy enough for you to figure it out :-) -TGG -
Hi I think this is probably the problem here: this.lastSlots = this.currentSlots; Although it looks like this copies currentSlots into lastSlots, it doesn't. It makes lastSlots refer to the same array as currentSlots does, i.e. they are both the same object. So your comparisons will always be true because you're comparing the same object against itself. You need to copy the elements of the array, eg for (int i = 0;i < 7;i++) { this.lastSlots[i] = this.currentSlots[i]; } or System.arraycopy(this.currentSlots, 0, this.lastSlots, 0, this.lastSlots.length); -TGG