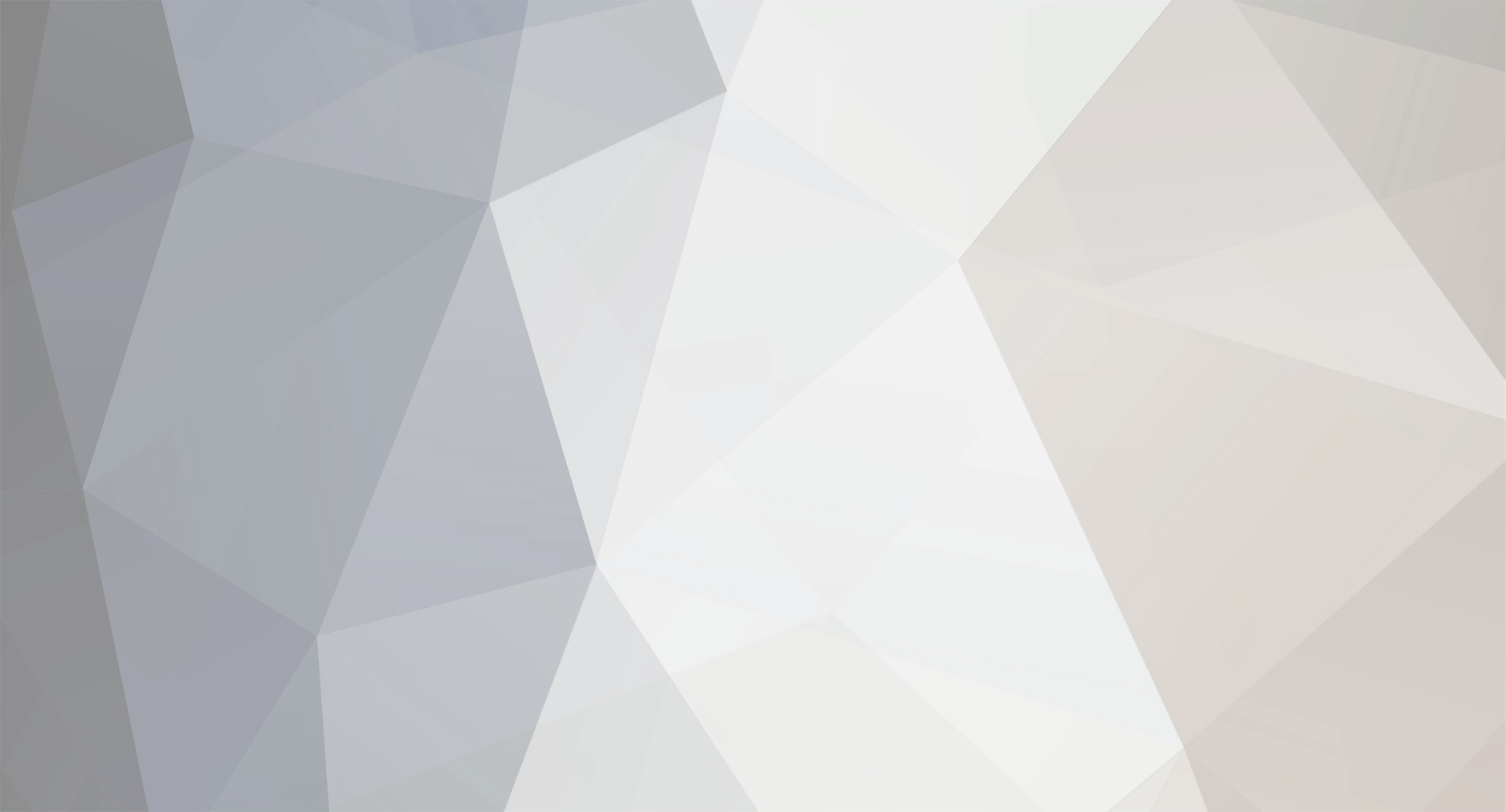
TheGreyGhost
Members-
Posts
3280 -
Joined
-
Last visited
-
Days Won
8
Everything posted by TheGreyGhost
-
[1.7.10] TileEntityRenderer Problems [Solved]
TheGreyGhost replied to mangoose's topic in Modder Support
Hi I don't know what's causing that symptom, it's quite odd. Vanilla does cull TESR based on where the player is looking, if you haven't overridden that, it might be culling your renderer as soon as you move your head. /** Return an appropriate bounding box enclosing the TESR * This method is used to control whether the TESR should be rendered or not, depending on where the player is looking. * The default is the AABB for the parent block, which might be too small if the TESR renders outside the borders of the * parent block. * If you get the boundary too small, the TESR may disappear when you aren't looking directly at it. * @return an appropriately size AABB for the TileEntity */ @SideOnly(Side.CLIENT) @Override public AxisAlignedBB getRenderBoundingBox() { // if your render should always be performed regardless of where the player is looking, use infinite AxisAlignedBB infiniteExample = INFINITE_EXTENT_AABB; // our gem will stay above the block, up to 1 block higher, so our bounding box is from [x,y,z] to [x+1, y+2, z+1] AxisAlignedBB aabb = new AxisAlignedBB(getPos(), getPos().add(1, 2, 1)); return aabb; } In any case, you can find the caller of your TESR by setting a breakpoint in renderTileEntityAt and then using your debugger to step out of the method into the caller. -TGG -
[1.8] [RESOLVED] return blockPos of clicked spot- even if it is air
TheGreyGhost replied to DJD's topic in Modder Support
Hi I've had to do this a couple of times. I essentially just copied the vanilla code and modified it slightly https://gist.github.com/TheGreyGhost/db79ee859f15a9c238c3 The hardest part is to get vanilla to ignore the player's right click when you want the air block and not the solid block a bit further away. I had to use a hack to do that (overwriting the KeyBinding instances in GameSettings.keyBindAttack with my own wrapper class that captures clicks and stops them from being passed to vanilla), you could also use ASM+Reflection. -TGG -
You only need ISmartItemModel if you want to customise your item's appearance based on the item state (for example - change the appearance if your item is damaged - since the damage information is stored in an ItemStack). To summarise - if you want to customise the block appearance when placed, you need to implement ISmartBlockModel. It sounds like you've got this bit working. If you also want to customise the block appearance when in inventory or tossed or in your hand, you need to implement ISmartItemModel as well - but the trick here is that you can't use the same information to customise the appearance. ISmartBlockModel gets to look at the IBlockState but there is no such thing for an item you're holding. Items use the ItemStack 'damage' field and (if present) NBT information. Could you describe a bit more what you're trying to do? i.e. how should the Item appearance be customised? -TGG
-
Ah... your code triggers a ghost of a memory... let me check... Try this: Tessellator tess = Tessellator.instance; tess.startDrawing(7); //swap order tess.setBrightness(15728880); public void startDrawing(int p_78371_1_) { if (this.isDrawing) { throw new IllegalStateException("Already tesselating!"); } else { this.isDrawing = true; this.reset(); this.drawMode = p_78371_1_; this.hasNormals = false; this.hasColor = false; this.hasTexture = false; this.hasBrightness = false; this.isColorDisabled = false; } } -TGG
-
Hi If your item is a ISmartItemModel then handleItemState() is good. Otherwise, I've used this spot a lot: ItemModelMesher.getItemModel() getActualState is useful for blocks because there are so many other blocks in addition to yours that you get hundreds of nuisance breaks. But with items you just put yours in the hotbar and it's the only one that reaches the breakpoint, so it's not an issue. -TGG
-
Hi Draco You could try calling GL11.glColor4f(colour.R, colour.G, colour.B, colour.A); instead of tess.setColorOpaque_F(1F, 0F, 0F); That fixed a similar problem I had once before. -TGG
-
Holy smokes dude! If the bit shifting is causing you that much grief, perhaps try this class instead- Java.awt.Color Color myColour = new Color(red, green, blue); Gui.drawRect(drawX, drawY, rectX2, rectY2, myColour.getRGB()); -TGG
-
Hi I'd suggest 1) Subscribe to the RenderGameOverlayEvent.Pre event 2) Use the Tessellator in GL11.GL_LINE_STRIP to draw the edges of your box I've posted some similar code from one of my mods which might help you with the key bits, there are a couple of aspects that are hard to figure out from scratch. https://gist.github.com/TheGreyGhost/035513e206b784591128 -TGG
-
[SOLVED] [1.8] Some problems with custom chest
TheGreyGhost replied to JimiIT92's topic in Modder Support
Hi For your item, just use a json model file for a normal item (see the MinecraftByExample for some examples of just a simple item). Your current json builtin/entity says "use the TileEntitySpecialRenderer for this item" which defaults to the vanilla chest it seems. Your missing texture when you smash the block - you can generate them yourself using Block.addDestroyEffects(), or alternative you can add a "particle" texture to your model json. If you want to trace through in the code to see where it is getting your blocks' destruction texture from, you can put a breakpoint into Block.addDestroyEffects() or alternatively EffectRenderer.func_180533_a() -TGG -
Hi Keen Re particles - I think that will be tricky because the particles render after all the blocks are finished. You would need to draw the particles before the transparent glass, i.e as part of your tessellator instead of as EntityFX particles. It can be done, but it's a bit tricky (basically - your tessellator would keep track of the EntityFX, move them & render them, etc, instead of spawning them as you normally would.) -TGG
-
[SOLVED] [1.8] Some problems with custom chest
TheGreyGhost replied to JimiIT92's topic in Modder Support
Hi This tutorial project has some examples of block models https://github.com/TheGreyGhost/MinecraftByExample Some more background info on block models here http://greyminecraftcoder.blogspot.com.au/p/list-of-topics.html and troubleshooting those missing texture problems here http://greyminecraftcoder.blogspot.com.au/2015/03/troubleshooting-block-and-item-rendering.html -TGG -
Using IExtendedEntityProperties for custom (non-simple) data types
TheGreyGhost replied to DJD's topic in Modder Support
Hi FYI This example class might be useful background info if you haven't used NBT before https://github.com/TheGreyGhost/MinecraftByExample/blob/master/src/main/java/minecraftbyexample/mbe20_tileentity_data/TileEntityData.java (see writeToNBT() and readFromNBT() ) -TGG -
Hi I'm having trouble seeing what's wrong in your images But I suggest to troubleshoot by 1) Render just the solid parts, including jarInside, without rendering the glass Your floor might not be rendering if the floor is facing the wrong way (or backface culling is on), or your floor exactly overlaps one of the others Once that works; 2) Turn on your alpha blending and render the glass as well, after the solid parts and jarInside. -TGG
-
There is a way, but the Forge Code Purity Police censor it out every time it gets mentioned on this forum A google on TileEntityItemStackRenderer.instance may show it up. -TGG
-
Hi ISmartBlockModel just lets you generate a texture using code, instead of having to specify it in a block model file. Honestly, if you just need an on/off light on your block, and it's always in the same place on the block, you should just make two block textures, one for on, the other for off, and switch between them using the IBlockState, it's the most straightforward way. If you want your light to blink or be animated, use a TESR. You really don't need ISmartBlockModel unless you're trying to do fancy stuff like count the number of nearby blocks or put the light in different locations depending on what's connected to your machine, or you've got lots of lights that work independently. That tutorial project has examples of ordinary blocks, blocks with variants (coloured signposts for example), also the more advanced ones that use ISmartBlockModel and such. -TGG
-
Hi From memory, the block.rotateBlock() was a Forge addition and it only works rotating the block in place (i.e. a block which is already placed into the world). You could use that if you're willing to place the block then rotate it. -TGG
-
Hi What's your concern with manipulating FACING? That's for sure the most portable and robust way to do it. Back in the bad old days of 1.7.10 you had to know the metadata encoding of every block... You might find this class useful https://gist.github.com/TheGreyGhost/d3e89af8f4121bd63acc -TGG
-
Hi You could use the resource manager to open your file as a stream, you don't need to worry about absolute paths at all then, just give it your domain eg from SoundHandler: final SoundList.SoundEntry soundentry = (SoundList.SoundEntry)iterator.next(); String s = soundentry.getSoundEntryName(); ResourceLocation resourcelocation1 = new ResourceLocation(s); final String s1 = s.contains(":") ? resourcelocation1.getResourceDomain() : p_147693_1_.getResourceDomain(); Object object; switch (SoundHandler.SwitchType.field_148765_a[soundentry.getSoundEntryType().ordinal()]) { case 1: ResourceLocation resourcelocation2 = new ResourceLocation(s1, "sounds/" + resourcelocation1.getResourcePath() + ".ogg"); InputStream inputstream = null; try { inputstream = this.mcResourceManager.getResource(resourcelocation2).getInputStream(); } catch (FileNotFoundException filenotfoundexception) { logger.warn("File {} does not exist, cannot add it to event {}", new Object[] {resourcelocation2, p_147693_1_}); continue; } catch (IOException ioexception) { logger.warn("Could not load sound file " + resourcelocation2 + ", cannot add it to event " + p_147693_1_, ioexception); continue; } finally { IOUtils.closeQuietly(inputstream); } -TGG
-
Hi Where do you want the data to be saved? in the saved game folder? If so- CommonProxy:: /** * Obtains the folder that world save backups should be stored in. * For Integrated Server, this is the saves folder * For Dedicated Server, a new 'backupsaves' folder is created in the same folder that contains the world save directory * @return the folder where backup saves should be created */ public abstract Path getOrCreateSaveBackupsFolder() throws IOException; ClientProxy:: @Override public Path getOrCreateSaveBackupsFolder() throws IOException { return new File(Minecraft.getMinecraft().mcDataDir, "saves").toPath(); } DedicatedServerProxy:: @Override public Path getOrCreateSaveBackupsFolder() throws IOException { Path universeFolder = FMLServerHandler.instance().getSavesDirectory().toPath(); Path backupsFolder = universeFolder.resolve(SpeedyToolsOptions.nameForSavesBackupFolder()); if (!Files.exists(backupsFolder)) { Files.createDirectory(backupsFolder); } return backupsFolder; } -TGG
-
> I really don't want to have textures for on and off, I want my code to be unique. Any ideas? I don't understand what you mean there, but anyway the easiest method is probably to use a TileEntitySpecialRenderer. You draw the underlying block with a Block Model, then use the TESR to draw your pixels on the top. a working example is in this tutorial project https://github.com/TheGreyGhost/MinecraftByExample MBE21 -TGG
-
Awesomeness -TGG
-
Hi Yes that basic idea should work. You need to be a bit clever about it though. When you find a cable, you need to check that you didn't find it before. Otherwise you end up with an infinite loop. This is a pretty standard flood fill algorithm and a bit of google will probably find you some efficient code you can base yours on. -TGG
-
[1.8] Craft a certain item with any sword/pickaxe/etc
TheGreyGhost replied to SuperSaltyGamer's topic in Modder Support
Hi Yes it's possible. You can add your own complicated recipes (eg like Fireworks recipes) by implementing IRecipe and registering it using GameRegistry.addRecipe(myRecipe implements IRecipe); -TGG -
Hi What you're trying to find is the plane which is normal (perpendicular) to the line of vision from the player and which also includes the laser beam. This is a bit like holding a ruler so that the flat face of the ruler is directly facing you so you can read the numbers along the long edge. You know the vector direction that the long edge of the ruler is facing in, that's the laser beam start and end points, but in order to draw the ruler properly you need to calculate the vector direction of the short edge of the ruler. (The thickness of the ruler is ignored) So you have three points: player eye position at P [xp, yp, zp] start of beam at S [xs, ys, zs] end of beam at E [xe, ye, ze] You need to create the two vectors PS and SE PS = [xs-xp, ys-yp, zs-zp] SE = [xe-xs, ye-ys, ze-zs] then find the cross product of PS and SE (Vec3 class has a crossproduct function) and normalise it (also a method of Vec3) this gives you a unit length vector that is perpendicular to both the line of sight from the player as well as the laser beam itself. If you call this vector say "acrossBeam" you draw a quad with the four points: S plus half beam width * acrossBeam S minus half beam width * acrossBeam E plus half beam width * acrossBeam E minus half beam width * acrossBeam The larger your value of half beam width, the wider the laser beam will appear. How much sense this makes to you probably depends on whether you've done much vector math before... a bit of google will help if necessary -TGG
-
Tile Entity storing and obtaining nbt data [solved]?
TheGreyGhost replied to Atijaf's topic in Modder Support
Hi As a matter of good code design I think your block should ask your TileEntity for the IBlockState value. It shouldn't try to retrieve the NBT information and then parse out what it needs itself. Apart from that your approach seems roughly right. If it's not working, trying putting some breakpoints or System.out.println in the various methods. -TGG