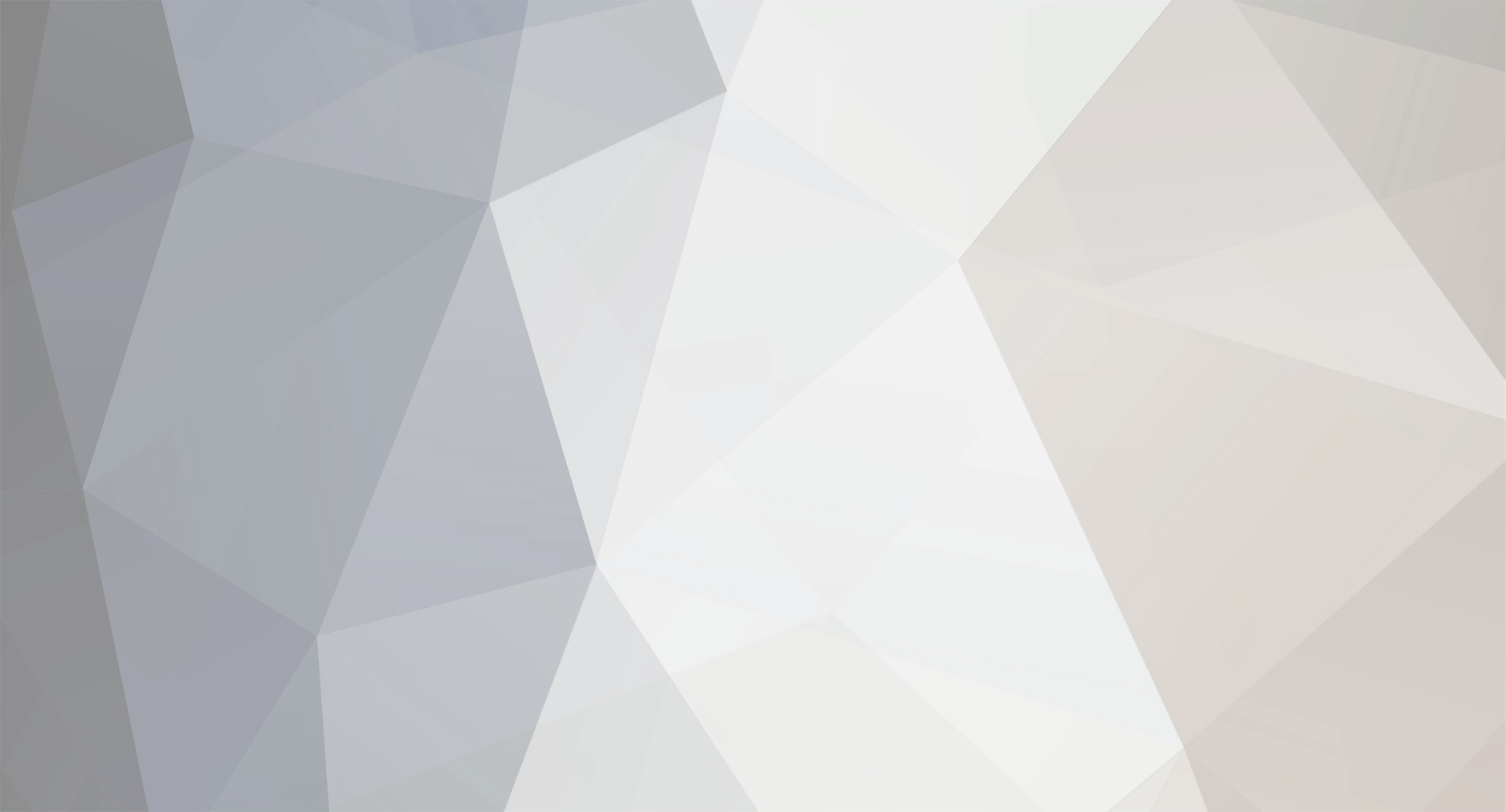
TheGreyGhost
Members-
Posts
3280 -
Joined
-
Last visited
-
Days Won
8
Everything posted by TheGreyGhost
-
[1.10.2] Rotate block-model by small degrees -without- TESR?
TheGreyGhost replied to Matryoshika's topic in Modder Support
Hi Yeah that is a problem if you don't use lighting. The vanilla blocks use a manual lighting method for faces which point east, north, etc. Vanilla items use a couple of openGL light sources. So you could try turning on the item lighting which might make your edges show up better. Alternatively you could change some of the quad textures to be a bit brighter or duller. A bit more info here... http://greyminecraftcoder.blogspot.com/2014/12/lighting-18.html I've used that before and it can work really well; the block model part blends nicely with the scenery and the animated bit is easy to render separately. I've put an example of something like that in this tutorial project: https://github.com/TheGreyGhost/MinecraftByExample (see MBE21) -TGG -
Hi You might find this tutorial project useful https://github.com/TheGreyGhost/MinecraftByExample mbe35 has examples of how to create custom recipes -TGG
-
[1.10.2] Rotate block-model by small degrees -without- TESR?
TheGreyGhost replied to Matryoshika's topic in Modder Support
Hi Block models will never work for that type of animation because they aren't updated every frame. They are compiled once into a render list or vertex buffer and not refreshed unless the blocks are modified (created, destroyed, blockstate changed, etc). You can animate the texture but that's it. I think a TESR is your best option. If it's causing a massive slowdown it's almost certainly because you've implemented it inefficiently, or perhaps your model is too complicated (too many quads). If you compile your quads to a vertex buffer, cache it, and draw that in your TESR, it will be just as fast as drawing block models, maybe faster. -TGG -
[SOLVED] Dynamic blocks models and complex underlying block states
TheGreyGhost replied to desht's topic in Modder Support
Try adding this to your block class: // used by the renderer to control lighting and visibility of other blocks. // set to false because this block doesn't fill the entire 1x1x1 space @Override public boolean isOpaqueCube(IBlockState iBlockState) { return false; } // used by the renderer to control lighting and visibility of other blocks, also by // (eg) wall or fence to control whether the fence joins itself to this block // set to false because this block doesn't fill the entire 1x1x1 space @Override public boolean isFullCube(IBlockState iBlockState) { return false; } -TGG -
[SOLVED] Dynamic blocks models and complex underlying block states
TheGreyGhost replied to desht's topic in Modder Support
Hi You're right, the StateMapperBase collapses all of your variants into just one ModelResourceLocation so none of the other variant models are registered, which is why you get a missing whenever you try to retrieve the baked model. So either - 1) your getQuads has to select the correct model from its own register, based on the blockState; or 2) You can get rid of the StateMapperBase completely, which means all your block variants will be registered; your ModelBakeEventHandler will then need to find and replace all of the variant models with your CustomBakedModel. Since your camo property is an IUnlistedProperty, it won't generate extra entries. (2) is likely to be the simplest method I think. -TGG -
Hi I think you should remove the .png from your filename. Have you seen this tutorial project yet? https://github.com/TheGreyGhost/MinecraftByExample mbe50 has a simple working example of what you need. Also, I have a recollection that getAtlasSprite won't retrieve the texture until after the textures are stitched, so your getAtlasSprite immediately after registerSprite is too soon (i.e. it's not testing what you think it's testing). -TGG
-
[1.10.2] StackOverflowError on Biome Decoration
TheGreyGhost replied to LogicTechCorp's topic in Modder Support
Hi It looks to me like some of your generators/decorators are causing an infinite loop, for example at nex.world.gen.feature.WorldGenThornBush.generate(WorldGenThornBush.java:35) i.e. you ask for a blockstate, which cause vanilla to generate a region, which calls your generator, which asks for a blockstate, which causes vanilla to generate another region, which calls your generator, etc I've never worked with generator/decorators before but for sure you are either calling methods you're not supposed to, or you're calling them with the wrong coordinates. -TGG -
[1.10][SOLVED]Problems with entity render
TheGreyGhost replied to Norzeteus's topic in Modder Support
Hi Any classes that get loaded on the dedicated server must not contain any mention of client-side classes at all. It doesn't matter if that code is never executed on the server. So for example EntityBeetle.createEntity(EntityMobBeetle.class, new RenderBeetle(null), "Beetle"); must not be in your Mainclass. Put it into a server-side-only proxy instead. See here for more background info. http://greyminecraftcoder.blogspot.com.au/2013/11/how-forge-starts-up-your-code.html -TGG -
[1.10.2] Block is loading but not showing up
TheGreyGhost replied to trentmartin2's topic in Modder Support
Hi YOu might find this tutorial project useful https://github.com/TheGreyGhost/MinecraftByExample Look at mbe01. -TGG -
[1.10] [SOLVED] Accessing stone variants
TheGreyGhost replied to swordkorn's topic in Modder Support
Hi @SwordKorn you can do it the way you were trying, it's just a bit more involved. ItemStack heldItem = entityPlayer.getHeldItemMainhand(); IBlockState iBlockStateAndesite = Blocks.STONE.getDefaultState().withProperty(BlockStone.VARIANT, BlockStone.EnumType.ANDESITE); Item itemStone = Item.getItemFromBlock(Blocks.STONE); ItemStack itemStackAndesite = new ItemStack(itemStone, Blocks.STONE.damageDropped(iBlockStateAndesite)); // see Block.getItem() boolean heldItemIsAndesite = ItemStack.areItemsEqual(heldItem, itemStackAndesite); I think Koward's suggestion is probably clearer. -TGG -
[1.8.9] making blocks place based on orientation
TheGreyGhost replied to Dark_but_Good's topic in Modder Support
Hi You might find this example project useful, it has a block you can orient in four directions. https://github.com/TheGreyGhost/MinecraftByExample see MBE03 In particular have a look at BlockVariants.onBlockPlaced(), getStateFromMeta(), and getMetaFromState() -TGG -
Hi I don't see an obvious problem; have you tried placing breakpoints in your getUpdatePacket() to see if it's being called properly You could try comparing your code to this example project https://github.com/TheGreyGhost/MinecraftByExample MBE30 and MBE31 are two working examples which sound similar to what you're doing. -TGG
-
Hi Do you have a json for your item in assets/yourmodid/models/item? You might find this example project useful to get the structure of the resources correct https://github.com/TheGreyGhost/MinecraftByExample/tree/1-8final The mbe01 and mbe10 are probably the closest to what you're doing. -TGG
-
Hi You could use reflection and access modifiers to make the BufferedImage public. It adds a bit of extra maintenance because you need to know both the obfuscated and the deobfuscated name of the variable, but it's pretty straightforward. There's an example here: https://github.com/TheGreyGhost/SpeedyTools/blob/V3-0-0/src/main/java/speedytools/clientside/userinput/KeyBindingInterceptor.java in particular this bit private static final Field keybindArrayField = ReflectionHelper.findField(KeyBinding.class, "keybindArray", "field_74516_a"); The syntax will be slightly different because your field is non-static, but you seem to have enough of a clue to figure it out An alternative which I've also used before is to render the skin into a framebuffer and then use that framebuffer as a texture. It's a lot more complicated, but in case you're interested the code is here https://github.com/TheGreyGhost/SpeedyTools/blob/V3-0-0/src/main/java/speedytools/clientside/selections/SelectionBlockTextures.java (I used it to capture "flat" textures from rendered block models) -TGG
-
Hi In that case, try using registerBlock with null in place of itemClass, and then register your item separately, like in the code you posted. -TGG
-
Hi registerBlock also registers an ItemBlock automatically, corresponding to the block. public static Block registerBlock(Block block, String name) { return registerBlock(block, ItemBlock.class, name); } If you want to use your own ItemBLock, use this method instead /** * Register a block with the world, with the specified item class and block name * * @param block The block to register * @param itemclass The item type to register with it : null registers a block without associated item. * @param name The mod-unique name to register it as, will get prefixed by your modid. */ public static Block registerBlock(Block block, Class<? extends ItemBlock> itemclass, String name) { return registerBlock(block, itemclass, name, new Object[] {}); } -TGG
-
[1.10.2] Held ItemBlock from TESR turns dark
TheGreyGhost replied to Matryoshika's topic in Modder Support
Hi For sure this has something to do with a lighting setting (eg the 'multitexturing' brightness lighting ) Is it your item that's dark, or does your TESR cause something else to go dark? If the second, you could try GL11.glPushAttrib(GL11.GL_ENABLE_BIT); If the first, you could try OpenGlHelper.setLightmapTextureCoords(OpenGlHelper.lightmapTexUnit, SKY_LIGHT_VALUE * 16.0F, BLOCK_LIGHT_VALUE * 16.0F); before rendering your item some clues here: https://github.com/TheGreyGhost/MinecraftByExample/blob/master/src/main/java/minecraftbyexample/mbe21_tileentityspecialrenderer/TileEntitySpecialRendererMBE21.java This link has some further info http://greyminecraftcoder.blogspot.com.au/2014/12/lighting-18.html It's 1.8 but the concepts are the same -TGG -
Tesselator API Documentation and/or Tutorials
TheGreyGhost replied to Mattizin's topic in Modder Support
Hi You might find this example project useful https://github.com/TheGreyGhost/MinecraftByExample See MBE21 and especially https://github.com/TheGreyGhost/MinecraftByExample/blob/master/src/main/java/minecraftbyexample/mbe21_tileentityspecialrenderer/TileEntitySpecialRendererMBE21.java Also this link http://greyminecraftcoder.blogspot.com.au/2014/12/the-tessellator-and-worldrenderer-18.html It's for 1.8 but the concepts are the same. -TGG -
[1.10.2] [Solved] Custom EntityHanging doesn't render on a server
TheGreyGhost replied to Palaster's topic in Modder Support
Hi My guess is that you've messed up the registration of your entity on the server. Perhaps you have registered in the client proxy instead of the common proxy? If you spawn your entity and look at it, what do you see in single player vs multiplayer (on the F3 debug screen, I mean)? -TGG -
Hi Look in ProjectileHelper. forwardsRaycast() does this for you. -TGG
-
Hi In general when implementing a TileEntity which saves information you need to implement at least six methods The readFromNBT and the writeToNBT you do already you also need getUpdatePacket(), getUpdateTag(), onDataPacket(), and handleUpdateTag() // getUpdatePacket() and onDataPacket() are used for one-at-a-time TileEntity updates // getUpdateTag() and handleUpdateTag() are used by vanilla to collate together into a single chunk update packet See this example project for some clues https://github.com/TheGreyGhost/MinecraftByExample/blob/master/src/main/java/minecraftbyexample/mbe20_tileentity_data/TileEntityData.java'>https://github.com/TheGreyGhost/MinecraftByExample/blob/master/src/main/java/minecraftbyexample/mbe20_tileentity_data/TileEntityData.java By the way, saving the "Capability" has nothing to do with saving your private fields (your items), as far as I can tell from your code. You also seem to be using a number of interfaces I don't recognise, are they experimental, eg IInventoryOnwerIinv is it really spelled "Onwer"? This example project also has other examples of similar items - a small chest and a furnace - which are similar. https://github.com/TheGreyGhost/MinecraftByExample/blob/master see mbe30 and mbe31 -TGG -TGG
-
Hi Perhaps your blockstates json is not correct. You could check your getActualState by putting a breakpoint in there or alternatively System.out.println("state:" + actualState); @Override public IBlockState getActualState(IBlockState state, IBlockAccess worldIn, BlockPos pos) { actualState = state.withProperty(MID, isBottom((World) worldIn, pos)); return actualState }
-
[1.10.02] Overlay item texture on block texture
TheGreyGhost replied to TrekkieCub314's topic in Modder Support
Hi This doesn't look right @Override public NBTTagCompound getUpdateTag() { NBTTagCompound tag = super.getUpdateTag(); readInvFromNBT(tag); /*( tag.setInteger("x", this.pos.getX()); tag.setInteger("y", this.pos.getY()); tag.setInteger("z", this.pos.getZ()); */ return super.getUpdateTag(); // <--- should probably be return tag; } -TGG -
Hi Translucent rendering is always a problem because the appearance of your model will change depending on which order the faces are drawn in (and which angle you're looking at the model from). I think that's probably what is happening to you. Usually, you should draw the opaque faces first, then the translucent faces second, and I don't think the block model guarantees that. But the same problem applies to translucent faces as well, i.e. if you look through one translucent face and see a second translucent face. In order to get it right you usually have to depth-sort the faces. This link explains it a bit better https://www.opengl.org/wiki/Transparency_Sorting I've implemented a jar similar to yours before (admittedly before 1.10.2), and I used a TileEntity to do it. The opaque parts I drew with a block model, then in the TileEntitySpecialRenderer, I draw the transparent faces in depth-sorted order. It worked but was pretty complicated and you would have to understand quite a bit about how depth buffering, culling, and depth-sorting work. Cutout_mipped is something different and will make your translucent panes go non-translucent. BlockRenderLayer.TRANSLUCENT is correct but is not enough. If you find a simpler way please let me know..! -TGG
-
[1.10.2] Redstone and Block States.
TheGreyGhost replied to GrandMasterRubix's topic in Modder Support
Hi You might find this example project useful https://github.com/TheGreyGhost/MinecraftByExample see mbe03 There are also redstone power examples (mbe06) but not converted for 10.1.2 quite yet. In the meantime, this link might help (for 1.8 but still the same for 1.10) http://greyminecraftcoder.blogspot.com.au/2015/11/redstone.html -TGG