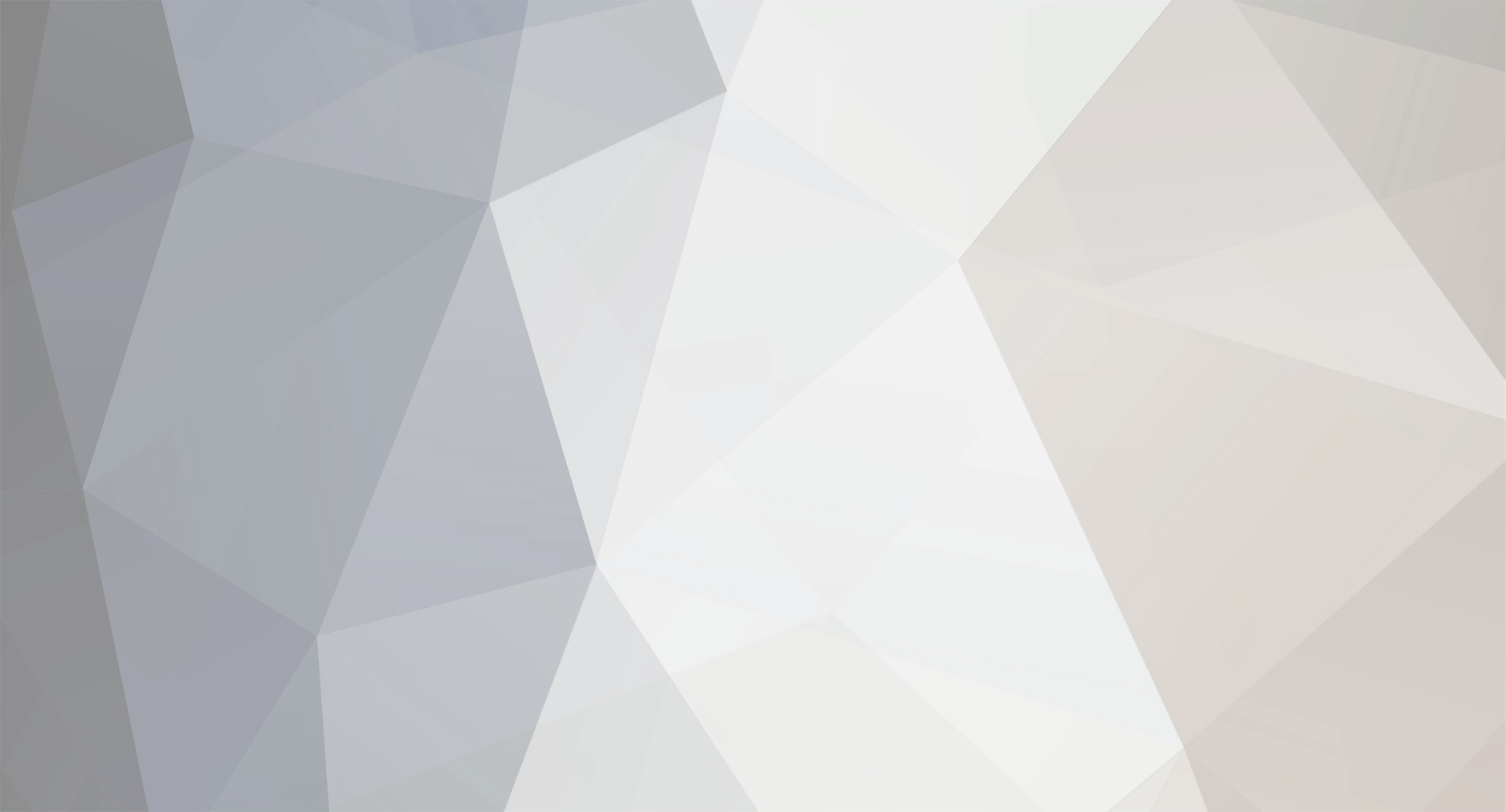
TheGreyGhost
Members-
Posts
3280 -
Joined
-
Last visited
-
Days Won
8
Everything posted by TheGreyGhost
-
[Solved] [1.8] Block#setLightLevel not working
TheGreyGhost replied to LordMastodon's topic in Modder Support
Hi Here's an example furnace that works like that (single block instead of two like vanilla) https://github.com/TheGreyGhost/MinecraftByExample/blob/master/src/main/java/minecraftbyexample/mbe31_inventory_furnace/Notes.txt The key bits are- in the TileEntity.update(): // when the number of burning slots changes, we need to force the block to re-render, otherwise the change in // state will not be visible. Likewise, we need to force a lighting recalculation. // The block update (for renderer) is only required on client side, but the lighting is required on both, since // the client needs it for rendering and the server needs it for crop growth etc int numberBurning = numberOfBurningFuelSlots(); if (cachedNumberOfBurningSlots != numberBurning) { cachedNumberOfBurningSlots = numberBurning; if (worldObj.isRemote) { worldObj.markBlockForUpdate(pos); } worldObj.checkLightFor(EnumSkyBlock.BLOCK, pos); } and in your block @Override public int getLightValue(IBlockAccess world, BlockPos pos) { int lightValue = 0; IBlockState blockState = getActualState(getDefaultState(), world, pos); int burningSides = (Integer)blockState.getValue(BURNING_SIDES_COUNT); if (burningSides == 0) { lightValue = 0; } else { // linearly interpolate the light value depending on how many slots are burning lightValue = ONE_SIDE_LIGHT_VALUE + (int)((FOUR_SIDE_LIGHT_VALUE - ONE_SIDE_LIGHT_VALUE) / (4.0 - 1.0) * burningSides); } lightValue = MathHelper.clamp_int(lightValue, 0, FOUR_SIDE_LIGHT_VALUE); return lightValue; } -TGG -
[1.8] Changing order of block registration messes up old world saves
TheGreyGhost replied to Tyron's topic in Modder Support
Hi I had this problem as well, it was caused by Forge doing some remapping of IDs from the save world but it wasn't 100% correctly implemented. I wasted nearly half a day figuring out what was going wrong. I thought it had been fixed, but perhaps not. So far it's never been an issue because I make a new saved game every time I change/add items. That doesn't help much if you've already released the mod though I would suggest you raise an issue at the forge github and provide links to your source and the saved game. Or even better, it would raise your chances of a successful bugfix if you made a very simple test case and submitted that. Or best of all, if you debug the source and pinpoint where the bug is (easier said than done) -TGG -
Hi Yes. In BlockLeaves and derived classes, check out: @SideOnly(Side.CLIENT) public int getBlockColor() { return ColorizerFoliage.getFoliageColor(0.5D, 1.0D); } @SideOnly(Side.CLIENT) public int getRenderColor(IBlockState state) { return ColorizerFoliage.getFoliageColorBasic(); } @SideOnly(Side.CLIENT) public int colorMultiplier(IBlockAccess worldIn, BlockPos pos, int renderPass) { return BiomeColorHelper.getFoliageColorAtPos(worldIn, pos); } - TGG
-
Hi Block animation is best done in one of two ways - For block texture animation (like lava) - use an animated texture. For others like chest - use a TileEntitySpecialRenderer. Some info here http://greyminecraftcoder.blogspot.com.au/2015/01/tileentity.html A working example here (MBE21) https://github.com/TheGreyGhost/MinecraftByExample You can't use JSON models to animate a block unless you rewrite the block every tick. -TGG
-
Hi One of my mods does this http://www.planetminecraft.com/mod/build-faster--four-tools-to-make-your-creative-life-easier/ There's quite a bit involved, not just NBT. You may get useful clues from here https://github.com/TheGreyGhost/SpeedyTools/tree/master/src/main/java/speedytools/serverside/worldmanipulation see WorldFragment.writeToWorldAsynchronous_do() Also - 1.8. has an inbuilt command to copy a range of blocks. Your code could just call that instead. -TGG
-
Hi Something easy to try first: try using all lower case for your SwordAttack1. The problem appears to be with your sounds.json not being found, or not registering the SwordAttack1 properly You could try: breakpoint in SoundManager.playSound() and inspect the registry in sndHandler.getSound to see if your sound was registered or not breakpoint in SoundHandler.onResourceManagerReload() to see what happens when it tries to load your sounds.json -TGG
-
Hi I've had this problem twice before- one time, my toBytes() packet code was overwriting the discriminator. (The buffer passed to toBytes() has the discriminator at position 0 and you're supposed to append to it.) another time, it was caused by a thread synchronisation bug (race condition) when sending to dimension or allNearbyPlayers. https://github.com/MinecraftForge/MinecraftForge/issues/1908 Try changing this line VintageCraft.packetPipeline.sendToDimension( new MechanicalNetworkNBTPacket(nbt, networkId), event.world.provider.getDimensionId() ); i.e. copy the code out of the forge sendToDimension to find the list of players and send them all a message one by one for example // StartupCommon.simpleNetworkWrapper.sendToDimension(msg, sendingPlayer.dimension); // DO NOT USE sendToDimension, it is buggy // as of build 1419 - see https://github.com/MinecraftForge/MinecraftForge/issues/1908 int dimension = sendingPlayer.dimension; MinecraftServer minecraftServer = sendingPlayer.mcServer; for (EntityPlayerMP player : (List<EntityPlayerMP>)minecraftServer.getConfigurationManager().playerEntityList) { TargetEffectMessageToClient msg = new TargetEffectMessageToClient(message.getTargetCoordinates()); // must generate a fresh message for every player! if (dimension == player.dimension) { StartupCommon.simpleNetworkWrapper.sendTo(msg, player); } } -TGG
-
[1.7.10] [SOLVED] Weird NoSuchMethodError with compiled mod.
TheGreyGhost replied to Elix_x's topic in Modder Support
HI 100% for sure using Reflection to get this field once per tick will not be noticeable. You could do it a million times per tick and the user probably still wouldn't notice. Just a comment - if you haven't specifically designed your mod to work with DedicatedServer, then it's a pretty good bet a whole pile of other things will break. If one of your classes has any mention of a vanilla client-side class, the classloader for your classes will often cause a crash even if the code that uses the client-side class is never ever executed. > You can use player.isUsingItem() without any issues, and if that's true, use player.getHeldItem() to get the item being used. No need for Reflection here. Didn't think of that... it's probably safe to assume they're always the same... probably... -TGG -
[1.7.10] [SOLVED] Weird NoSuchMethodError with compiled mod.
TheGreyGhost replied to Elix_x's topic in Modder Support
Why is it so? It's illogical... The Dedicated Server doesn't have a lot of the client-side code because it's not necessary if there's no local client. I guess none of the dedicated server code needs to access itemInUse from outside EntityPlayer, so they never added a getter on that side. Anyhow as Choonster said you can either use Reflection, an Access Transformer, or alternatively PlayerUseItemEvent and isUsingItem(). If you just want to access a private field it's pretty easy, eg @SideOnly(Side.CLIENT) public class KeyBindingInterceptor { private static final Field pressTimeField = ReflectionHelper.findField(KeyBinding.class, "pressTime", "field_151474_i"); // unobfuscated name, and obfuscatedname //... int value = 0; try { value = pressTimeField.getInt(keyBinding, 0); } catch (Exception e) { throw Throwables.propagate(e); } } -TGG -
Render a tileentity to imageFile?[1.8]
TheGreyGhost replied to ItsAMysteriousYT's topic in Modder Support
Yes. But it will need a fair bit of knowledge on OpenGL. The basic idea is to set up a framebuffer and render to that instead of to the screen, then save it to an image file. An example class which renders to a framebuffer is here https://gist.github.com/TheGreyGhost/96983a0cd47c8c2f294d The bits you're interested in are updateTextures(). The hardest bit is positioning & rotating your TileEntity so it is the correct size within the frame. The Minecraft screenshot code ScreenShotHelper is also very useful to look at. I should warn you it will be pretty hard going if you haven't done much OpenGL before. -TGG -
Projecting a 3D vector from object space into screen space
TheGreyGhost replied to 504185787's topic in Modder Support
Hi Render.renderLivingLabel() might give some inspiration on how you can add text on or near an entity. -TGG -
[1.8] [SOLVED] Blockstates json Wildcards?
TheGreyGhost replied to jeffryfisher's topic in Modder Support
Hi You can use a custom state mapper for your block properties to ignore some of them http://www.minecraftforge.net/forum/index.php/topic,28997.msg149446.html#msg149446 After re-reading your post, I don't think that will help with decoupling skin textures from the blockstates, though. You could consider either implementing an ISmartBlockModel with custom block loader to substitute the texture at loading (https://github.com/TheGreyGhost/MinecraftByExample MBE04, MBE05) or perhaps the forge blockstates can do this (I'm not familiar with those). -TGG -
Projecting a 3D vector from object space into screen space
TheGreyGhost replied to 504185787's topic in Modder Support
Hi The GLStateManager is buggy, don't use it. It doesn't dirty its cache properly with the push and pop, and it wouldn't surprise me if it's the cause of your stack underflow. With any luck that might solve the other issues too. -TGG -
[1.7.10] TESR/OpenGL Transparency Sorting Issues
TheGreyGhost replied to Izzy Axel's topic in Modder Support
It can be done. But it is an awful set of hacks and I reckon it's almost certainly better if you change your model so that it's not so visually annoying. -TGG -
[SOLVED] Particle Rendering - not facing the player
TheGreyGhost replied to yolp900's topic in Modder Support
Hi Vanilla particles always rotate the quad to directly face the player. An excerpt from a custom EntityFX I wrote (see below) might help make it clearer what's happening. Also this link to information about the tessellator. http://greyminecraftcoder.blogspot.co.at/2014/12/the-tessellator-and-worldrenderer-18.html -TGG /** * Render the EntityFX onto the screen. * The EntityFX is rendered as a two-dimensional object (Quad) in the world (three-dimensional coordinates). * The corners of the quad are chosen so that the EntityFX is drawn directly facing the viewer (or in other words, * so that the quad is always directly face-on to the screen.) * In order to manage this, it needs to know two direction vectors: * 1) the 3D vector direction corresponding to left-right on the viewer's screen (edgeLRdirection) * 2) the 3D vector direction corresponding to up-down on the viewer's screen (edgeURdirection) * These two vectors are calculated by the caller. * For example, the top right corner of the quad on the viewer's screen is equal to the centre point of the quad (x,y,z) * plus the edgeLRdirection vector multiplied by half the quad's width, plus the edgeURdirection vector multiplied * by half the quad's height. * NB edgeLRdirectionY is not provided because it's always 0, i.e. the top of the viewer's screen is always directly * up so moving left-right on the viewer's screen doesn't affect the y coordinate position in the world * @param worldRenderer * @param entity * @param partialTick * @param edgeLRdirectionX edgeLRdirection[XYZ] is the vector direction pointing left-right on the player's screen * @param edgeUDdirectionY edgeUDdirection[XYZ] is the vector direction pointing up-down on the player's screen * @param edgeLRdirectionZ edgeLRdirection[XYZ] is the vector direction pointing left-right on the player's screen * @param edgeUDdirectionX edgeUDdirection[XYZ] is the vector direction pointing up-down on the player's screen * @param edgeUDdirectionZ edgeUDdirection[XYZ] is the vector direction pointing up-down on the player's screen */ @Override public void func_180434_a(WorldRenderer worldRenderer, Entity entity, float partialTick, float edgeLRdirectionX, float edgeUDdirectionY, float edgeLRdirectionZ, float edgeUDdirectionX, float edgeUDdirectionZ) { double minU = this.particleIcon.getMinU(); double maxU = this.particleIcon.getMaxU(); double minV = this.particleIcon.getMinV(); double maxV = this.particleIcon.getMaxV(); RotatingQuad tex = new RotatingQuad(minU, minV, maxU, maxV); // RotatingQuad is just a helper that swaps the four texture corner points around, so the quad face can be rotated or flipped easily. You can ignore it. Random random = new Random(); if (random.nextBoolean()) { tex.mirrorLR(); } tex.rotate90(random.nextInt(4)); double scale = 0.1F * this.particleScale; final double scaleLR = scale; final double scaleUD = scale; double x = this.prevPosX + (this.posX - this.prevPosX) * partialTick - interpPosX; double y = this.prevPosY + (this.posY - this.prevPosY) * partialTick - interpPosY + this.height / 2.0F; // centre of rendering is now y midpt not ymin double z = this.prevPosZ + (this.posZ - this.prevPosZ) * partialTick - interpPosZ; worldRenderer.setColorRGBA_F(this.particleRed, this.particleGreen, this.particleBlue, this.particleAlpha); worldRenderer.addVertexWithUV(x - edgeLRdirectionX * scaleLR - edgeUDdirectionX * scaleUD, y - edgeUDdirectionY * scaleUD, z - edgeLRdirectionZ * scaleLR - edgeUDdirectionZ * scaleUD, tex.getU(0), tex.getV(0)); worldRenderer.addVertexWithUV(x - edgeLRdirectionX * scaleLR + edgeUDdirectionX * scaleUD, y + edgeUDdirectionY * scaleUD, z - edgeLRdirectionZ * scaleLR + edgeUDdirectionZ * scaleUD, tex.getU(1), tex.getV(1)); worldRenderer.addVertexWithUV(x + edgeLRdirectionX * scaleLR + edgeUDdirectionX * scaleUD, y + edgeUDdirectionY * scaleUD, z + edgeLRdirectionZ * scaleLR + edgeUDdirectionZ * scaleUD, tex.getU(2), tex.getV(2)); worldRenderer.addVertexWithUV(x + edgeLRdirectionX * scaleLR - edgeUDdirectionX * scaleUD, y - edgeUDdirectionY * scaleUD, z + edgeLRdirectionZ * scaleLR - edgeUDdirectionZ * scaleUD, tex.getU(3), tex.getV(3)); } -
[1.7.10] TESR/OpenGL Transparency Sorting Issues
TheGreyGhost replied to Izzy Axel's topic in Modder Support
Hi If your transparent pane is rendered first, it writes to the depth buffer, and then anything behind the pane that is rendered later (eg the second model, with the red liquid in your screenshot) is behind the glass, so it gets culled (doesn't render at all). It all depends which model renders first. It will help if you turn off writing to the depth buffer when drawing your transparent glass glDepthMask(false) and if you only render the panes which face the player (turn on back face culling). The only robust way to fix it is to render all your transparent parts last, sorted in depth order with the other transparent objects in the scene. This is a right pain in the butt. -TGG -
[1.8] Custom Furnace, some bugs....that i am spinning in circles!
TheGreyGhost replied to Vladan899's topic in Modder Support
Hi I haven't looked at your code in any detail, but I think you have a slot numbering problem. Keeping your slot numbering right is difficult, you need to pay careful attention to it. This tutorial has a working example that might give you some clues. https://github.com/TheGreyGhost/MinecraftByExample (see MBE31) -TGG -
Hi THere are a couple of other posts on this forum covering this (making up an item sword model from separate model pieces); ernio in particular has cracked it MBE15 in this tutorial project shows something similar - i.e. using ISmartItemModel https://github.com/TheGreyGhost/MinecraftByExample -TGG
-
Hi Some things to try - a) Are you sure you are registering your items in preInit and then the mesher in Init? Try setting breakpoints at the registration and tracing in to make sure the object is not null, the names are what you think they are. b) You could try some of the ideas on this page http://greyminecraftcoder.blogspot.com.au/2015/05/common-mistakes-with-filenames.html - especially checking the build folder build/classes/main/assets/minecraftbyexample intellij problem Apart from that, I don't see any obvious problem either. I've sometimes had success in searching the forgeSrc lib for the error message, putting a breakpoint at that location, and then inspecting the registry to see what the problem is. As a last resort, this tutorial project has working examples of items and blocks, so you could use that as a base for troubleshooting. https://github.com/TheGreyGhost/MinecraftByExample -TGG -TGG
-
[1.7.10] Portal Block Texture [SOLVED]
TheGreyGhost replied to HalestormXV's topic in Modder Support
Hi I looked in your code for the obvious problems and they look ok; I suggest you create a test world with a single CelStone next to a single BlockModPortal, put a breakpoint into CelStone.shouldSideBeRendered() and step out into vanilla to see what's going on. -TGG -
Hi One of the three items you use on TinyTools.java:81 is null. Also you should register recipes in init (FMLInitializationEvent) not in preinit (FMLPreInitializationEvent) This link has some info on how to debug this kind of error. http://www.terryanderson.ca/debugging/run.html -TGG