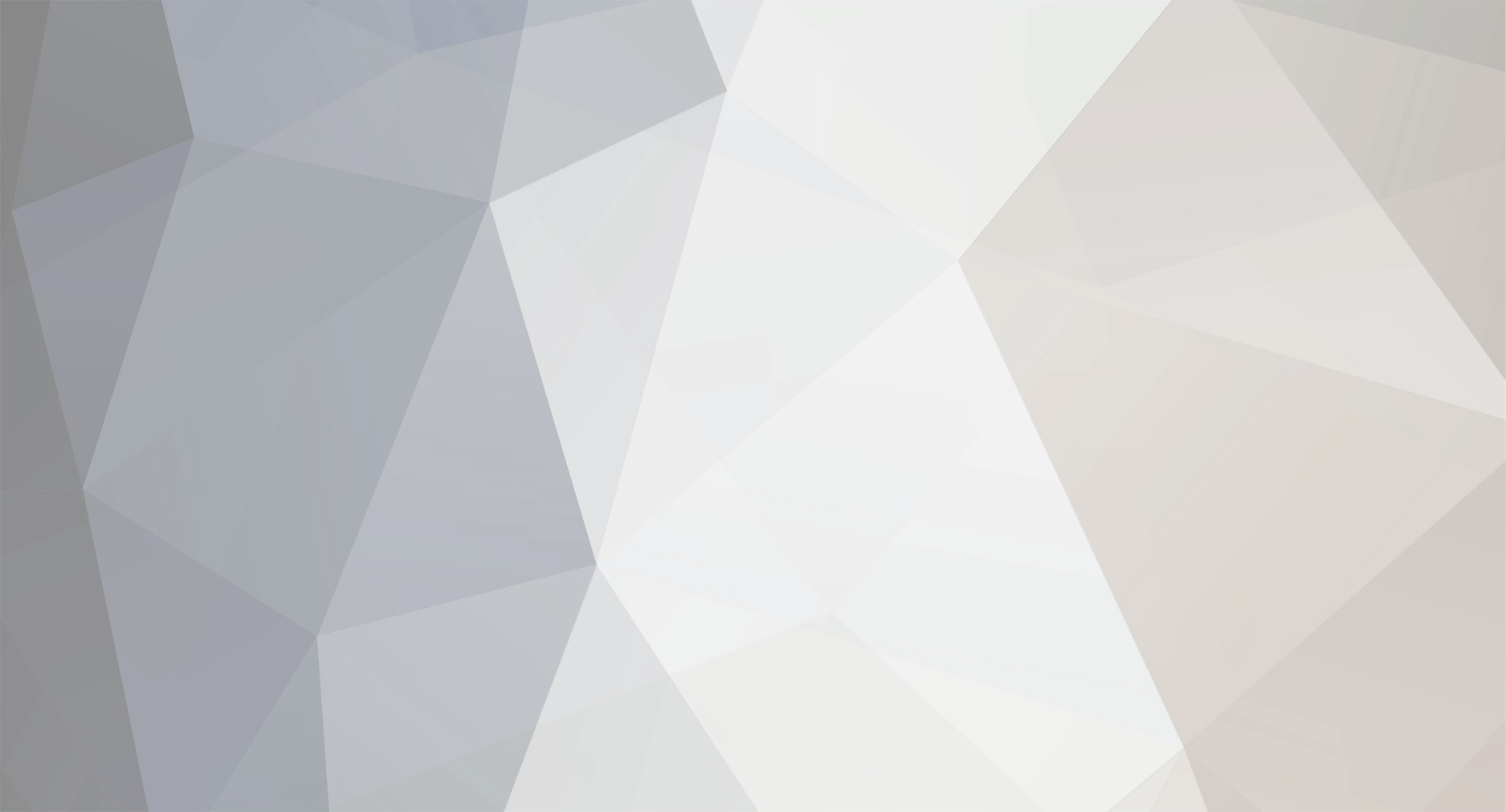
TheGreyGhost
Members-
Posts
3280 -
Joined
-
Last visited
-
Days Won
8
Everything posted by TheGreyGhost
-
This tutorial project might also help https://github.com/TheGreyGhost/MinecraftByExample (see MBE03) -TGG
-
Creating a config file in the world save folder
TheGreyGhost replied to Elrol_Arrowsend's topic in Modder Support
Well, if it helps, you can get the world save locations from here - it's different on a dedicated server and an integrated server, so I used a proxy method to get the right one. /** * Obtains the folder that world save backups should be stored in. * For Integrated Server, this is the saves folder * For Dedicated Server, a new 'backupsaves' folder is created in the same folder that contains the world save directory * @return the folder where backup saves should be created */ public abstract Path getOrCreateSaveBackupsFolder() throws IOException; in your proxy class ---> On an integrated server @Override public Path getOrCreateSaveBackupsFolder() throws IOException { return new File(Minecraft.getMinecraft().mcDataDir, "saves").toPath(); } On a dedicated server @Override public Path getOrCreateSaveBackupsFolder() throws IOException { Path universeFolder = FMLServerHandler.instance().getSavesDirectory().toPath(); Path backupsFolder = universeFolder.resolve(SpeedyToolsOptions.nameForSavesBackupFolder()); if (!Files.exists(backupsFolder)) { Files.createDirectory(backupsFolder); } return backupsFolder; } -TGG -
[1.8] Item textures not working outside of eclipse
TheGreyGhost replied to laci200270's topic in Modder Support
Make sure your upper case / lower case names match exactly. eg myItemTexture.png vs myitemtexture.png Windows will tolerate upper case/lower case mismatches, but jar files don't. -TGG -
[1.8] mcmod.info and assets not loading
TheGreyGhost replied to Chaka15205's topic in Modder Support
This link might help http://greyminecraftcoder.blogspot.com.au/2015/03/troubleshooting-block-and-item-rendering.html -TGG -
You are testing this mod in dedicated server, yeah? That section of code is being run in init, which is before the client and server threads start. Side.SERVER is the dedicated server, not server thread, that is different. http://greyminecraftcoder.blogspot.com.au/2013/11/how-forge-starts-up-your-code.html How are you testing this mod / where does the message appear? -TGG
-
[1.7.10] How to render a 3D line / rectangle.
TheGreyGhost replied to whizvox's topic in Modder Support
This link might help http://greyminecraftcoder.blogspot.com.au/2013/08/the-tessellator.html -TGG -
Hi Could you explain what the code is supposed to do, how it is supposed to work, and what the symptoms of the problem are? I'm having trouble understanding it. -TGG
-
If a packet class or handler is ever instantiated on the Server side, it must not contain any references to vanilla client code at all. For example this will cause your mod to crash on a dedicated server: if(ctx.side.isClient()) { EntityPlayer player = Minecraft.getMinecraft().thePlayer; Doesn't matter that you've hidden Minecraft inside a check for the Client side - the way the classloader works, it will try to look for Minecraft before executing any code at all. It doesn't find Minecraft class on the dedicated server, so it throws this error. Looks to me like something similar is happening for one of your classes with EntityClientPlayerMP, which is client-side only. -TGG
-
Not sure what you mean? Map colour is a property of the material the block is made of. For example: BlockLeaves has material = Material.leaves Material.leaves has MapColor.foliageColor MapColor.foliageColor is MapColor(7, 31744); you can also override Block:: public MapColor getMapColor(IBlockState state) 31744 is a number for the green, red, blue components of the colour; see here http://www.rapidtables.com/web/color/RGB_Color.htm normally it's written in hexadecimal; you can also use java.awt.Color to work with colour more easily package java.awt; /** * The <code>Color</code> class is used to encapsulate colors in the default * sRGB color space or colors in arbitrary color spaces identified by a * {@link ColorSpace}. Every color has an implicit alpha value of 1.0 or * an explicit one provided in the constructor. The alpha value * defines the transparency of a color and can be represented by * a float value in the range 0.0 - 1.0 or 0 - 255. * An alpha value of 1.0 or 255 means that the color is completely * opaque and an alpha value of 0 or 0.0 means that the color is * completely transparent. * When constructing a <code>Color</code> with an explicit alpha or * getting the color/alpha components of a <code>Color</code>, the color * components are never premultiplied by the alpha component. * <p> * The default color space for the Java 2D(tm) API is sRGB, a proposed * standard RGB color space. For further information on sRGB, * see <A href="http://www.w3.org/pub/WWW/Graphics/Color/sRGB.html"> * http://www.w3.org/pub/WWW/Graphics/Color/sRGB.html * </A>. * <p> * @version 10 Feb 1997 * @author Sami Shaio * @author Arthur van Hoff * @see ColorSpace * @see AlphaComposite */ public class Color implements Paint, java.io.Serializable { For example new MapColor(newID, Color.WHITE.getRGB()); or myCustomColour = new Color(red, green, blue); * Creates an opaque sRGB color with the specified red, green, * and blue values in the range (0 - 255). -TGG
-
Hi This link might be useful. http://greyminecraftcoder.blogspot.com.au/2013/10/user-input.html I did something similar for the Keyboard, I wound up overwriting the KeyBinding fields in GameSettings with a custom class derived from KeyBinding, eg GameSettings.keyBindAttack to prevent mouse clicks / attacking. -TGG
-
[1.7.10] [Solved] Quad rendering issues
TheGreyGhost replied to Guichaguri's topic in Modder Support
Hi This page talks a bit about the Tessellator and quads http://greyminecraftcoder.blogspot.com.au/2013/08/the-tessellator.html A couple of comments 1) If you're drawing a quad instead of a line, you don't need addVertexWithUV, use addVertex instead 2) If you want your line to be roughly the same thickness regardless of where the player is looking from, I think you'll need to choose one of two tricks either a) use some vector maths to draw the quad in a plane perpendicular to the viewer's eyes and figure out the width based on the distance to the player (this is not easy to do from scratch but ActiveRenderInfo.updateRenderInfo() has the necessary calculations and method calls to do the first one for you, you just need to figure out how to use it..), or b) draw a tube (rectangular prism) with four sides (figure out deltaX = endX - startX, deltaY = endY - startY, deltaZ = endZ - startZ, choose the biggest one (eg deltaY if the line is most vertical), then the four quads: south side [startX - half_line_width, startY, startZ+half_line_width] to [endX + half_line_width, endY, endZ + half_line_width] north side [startX - half_line_width, startY, startZ-half_line_width] to [endX + half_line_width, endY, endZ - half_line_width] west side [startX - half_line_width, startY, startZ - half_line_width] to [endX - half_line_width, endY, endZ + half_line_width] east side [startX + half_line_width, startY, startZ - half_line_width] to [endX + half_line_width, endY, endZ + half_line_width] This is a lot easier, but the line will change thickness a bit as you move around, and might look more like a square tube than a line, depending on how big it is. The width of the line will also vary depending how close the player is; you would need to change the line width proportional to the player's distance from the line. Depends what effect you're looking for really. -TGG -
Hi Yes there is, have a look at vanilla MovingSoundMinecartRiding and/or RenderGlobal.playRecord(). You can play the sound using something like ResourceLocation mySoundRes = new ResourceLocation("mymodid:mysound"); PositionedSoundRecord mySoundRes = PositionedSoundRecord.create(mySoundRes); Minecraft.getMinecraft().getSoundHandler().playSound(mySoundRes); put a sounds.json in resources/assets/mymodid/ { "mysound": {"category": "ambient", "sounds": ["mysoundfilename"]} } and your .ogg sound file in resources/assets/mymodid/sounds mysoundfilename.ogg It's a bit fiddly to get right.... the console often gives you a relevant error message. -TGG
-
[1.8][SOLVED] Armor texture shows incorrectly.
TheGreyGhost replied to SSslimer's topic in Modder Support
Hi Sorry dude, I can't make heads or tails of that code. I suggest you refactor it to make the names meaningful, then break it into pieces that you can understand. I think it will probably become obvious what is wrong once you have done that. It's not obvious to me right now, that's for sure. -TGG -
[SOLVED][1.8] Having trouble with texture on a custom block model
TheGreyGhost replied to jabelar's topic in Modder Support
Hi Jabelar This might give you some clues https://github.com/TheGreyGhost/MinecraftByExample/blob/master/src/main/resources/assets/minecraftbyexample/models/block/mbe02_block_partial_model.json -TGG -
[1.8][SOLVED] Help with dual custom texture rendering
TheGreyGhost replied to NovaViper's topic in Modder Support
Hi BY crikey your code is hard to read with all those p_177141_3_ in there, perhaps you should refactor it to make it more reader-friendly? I would suggest as a first step to add System.out.println() to your code to make sure the texture binding and rendering code is being executed in the correct order, when you expect it. If it works for one model but not the other, try to find the differences between the two. Try swapping the rendering order (eg do the collar before the dog) to see if the symptoms swap, that sort of thing. -TGG -
[1.8][SOLVED] Armor texture shows incorrectly.
TheGreyGhost replied to SSslimer's topic in Modder Support
Hi Pls provide pictures and relevant code? -TGG -
As to your second question about the sorting in a creative tab - yes, it's easy See this example for ideas https://github.com/TheGreyGhost/MinecraftByExample/tree/master/src/main/java/minecraftbyexample/mbe08_creative_tab -TGG
-
[1.7.10] Custom rendered block pushable by piston. [Abandoned]
TheGreyGhost replied to Alphafox_13's topic in Modder Support
Probably ITileEntityProvider. But I doubt there would be a noticeable difference. You could try it and see... -TGG -
This tutorial mod has an example of such recipes https://github.com/TheGreyGhost/MinecraftByExample/blob/master/src/main/java/minecraftbyexample/mbe35_recipes/StartupCommon.java with pictures http://greyminecraftcoder.blogspot.com.au/2015/02/recipes.html -TGG
-
[1.8, solved] ISmartBlockModel issue; item is textured, block is not
TheGreyGhost replied to Elyon's topic in Modder Support
You're back! Long time no post... This link might help with troubleshooting the block appearance http://greyminecraftcoder.blogspot.com.au/2015/03/troubleshooting-block-and-item-rendering.html This mod might help for the cubic metre problem: http://www.planetminecraft.com/mod/item-transform-helper---interactively-rotate-scale-translate/ The "Blocks" and four subtopics on this page might help http://greyminecraftcoder.blogspot.com.au/p/list-of-topics.html Also this tutorial post on the smart models http://www.minecraftforge.net/forum/index.php/topic,28714.0.html -TGG -
[1-8] Blockstates in the rendering files
TheGreyGhost replied to Bedrock_Miner's topic in Modder Support
Yes that's right. For modded blocks Forge always looks for the blockstates file with the same name as the block. re the alphabetic order: The lines in your blockstates can be in any order. The properties within a line, eg colour=red,facing=east vs facing=east,colour=red, are in some order that I don't know. Maybe alphabetical, maybe something else, never bothered to figure it out. If you get the order round the wrong way, the console error message will show you. If you don't ModelBakery.addVariantName, you will get missing model errors. It might seem redundant to have to use both ModelBakery and the Mesher, that's just how Vanilla does it... Yes I think you can exclude states, vanilla seems to do it, but I never figured out exactly how. To be honest, once I figured out that I could get the same effect by listing the 16 blockstates in one blockstates file, I didn't bother looking. If you work it out, let me know? -TGG -
[1.8] Trouble setting TileEntity data when block is placed
TheGreyGhost replied to Mystify's topic in Modder Support
Wow, that's nasty. Too subtle for me. There must be more to it than that, TileEntities which spontaneously lose their data doesn't sound right. Might have to add that to my example project as a warning. -TGG -
[1-8] Blockstates in the rendering files
TheGreyGhost replied to Bedrock_Miner's topic in Modder Support
Hi The way that MBE03 is coded, it has a line for every possible blockstate. But the four red blockstates all specify the same red model file, the four blue blockstates all specify the same blue model file, etc. This controls the way the block renders when you've placed it in the world. mbe03_block_variants.json:: { "variants": { "colour=red,facing=north": { "model": "minecraftbyexample:mbe03_block_variants_model_red"}, "colour=red,facing=east": { "model": "minecraftbyexample:mbe03_block_variants_model_red", "y": 90 }, "colour=red,facing=south": { "model": "minecraftbyexample:mbe03_block_variants_model_red", "y": 180 }, "colour=red,facing=west": { "model": "minecraftbyexample:mbe03_block_variants_model_red", "y": 270 }, "colour=green,facing=north": { "model": "minecraftbyexample:mbe03_block_variants_model_green" }, "colour=green,facing=east": { "model": "minecraftbyexample:mbe03_block_variants_model_green", "y": 90 }, "colour=green,facing=south": { "model": "minecraftbyexample:mbe03_block_variants_model_green", "y": 180 }, "colour=green,facing=west": { "model": "minecraftbyexample:mbe03_block_variants_model_green", "y": 270 }, "colour=yellow,facing=north": { "model": "minecraftbyexample:mbe03_block_variants_model_yellow" }, "colour=yellow,facing=east": { "model": "minecraftbyexample:mbe03_block_variants_model_yellow", "y": 90 }, "colour=yellow,facing=south": { "model": "minecraftbyexample:mbe03_block_variants_model_yellow", "y": 180 }, "colour=yellow,facing=west": { "model": "minecraftbyexample:mbe03_block_variants_model_yellow", "y": 270 }, "colour=blue,facing=north": { "model": "minecraftbyexample:mbe03_block_variants_model_blue" }, "colour=blue,facing=east": { "model": "minecraftbyexample:mbe03_block_variants_model_blue", "y": 90 }, "colour=blue,facing=south": { "model": "minecraftbyexample:mbe03_block_variants_model_blue", "y": 180 }, "colour=blue,facing=west": { "model": "minecraftbyexample:mbe03_block_variants_model_blue", "y": 270 } } } The stuff in the client proxy with ModelBakery.addVariantName(..) and Minecraft.getMinecraft().getRenderItem().getItemModelMesher().register(itemBlockVariants, BlockVariants.EnumColour.BLUE.getMetadata(), itemModelResourceLocation); and in the custom Block class Block.getSubBlocks() is used to pick some of the possible block states to appear in the inventory and as items. There are only four items, one per colour, because the different 'facing' directions are all the same when you hold them as an item. // create a list of the subBlocks available for this block, i.e. one for each colour // - used to populate items for the creative inventory // - the "metadata" value of the block is set to the colours metadata @Override @SideOnly(Side.CLIENT) public void getSubBlocks(Item itemIn, CreativeTabs tab, List list) { EnumColour[] allColours = EnumColour.values(); for (EnumColour colour : allColours) { list.add(new ItemStack(itemIn, 1, colour.getMetadata())); } } -TGG -
Textures Missing in Client but not Eclipse Executor
TheGreyGhost replied to gosaints70's topic in Modder Support
Hi If some of your textures are there but not all of them, it's probably a lower case/upper case mismatch problem. Windows ignores the case of files but jar files don't. I just make all my folder names and texture filenames lower case to be on the safe side. -TGG -
[1.7.10] Massive rendering glitches with Multiblock OBJ Model
TheGreyGhost replied to BenignBanana's topic in Modder Support
Hi The problem that minecraft 1.7.10 has with z-sorting is only for partially transparent textures (and it's fixed in 1.8 FYI). Your object has fully-solid-or-fully-transparent texels only, so it should render fine - the z-buffer in OpenGL does all the work for you, minecraft doesn't need to do any sorting at all. Your rendering code appears to turn on alpha blending, which is not needed, so perhaps it also turns off writing to the z-buffer. Try adding these lines to the start of your rendering: GL11.glEnable(GL11.GL_DEPTH_TEST); GL11.glDepthMask(true); GL11.glEnable(GL11.GL_ALPHA_TEST); GL11.glAlphaFunc(GL11.GL_GREATER, 0.1F); If that doesn't work, there is probably something wrong with your model. Round/spherical objects are always a problem. If you post a video where you walk around the model and look at it from different angles, it will help us diagnose the issue. -TGG PS what is this? wrapper.render(); Couldn't find wrapper anywhere.