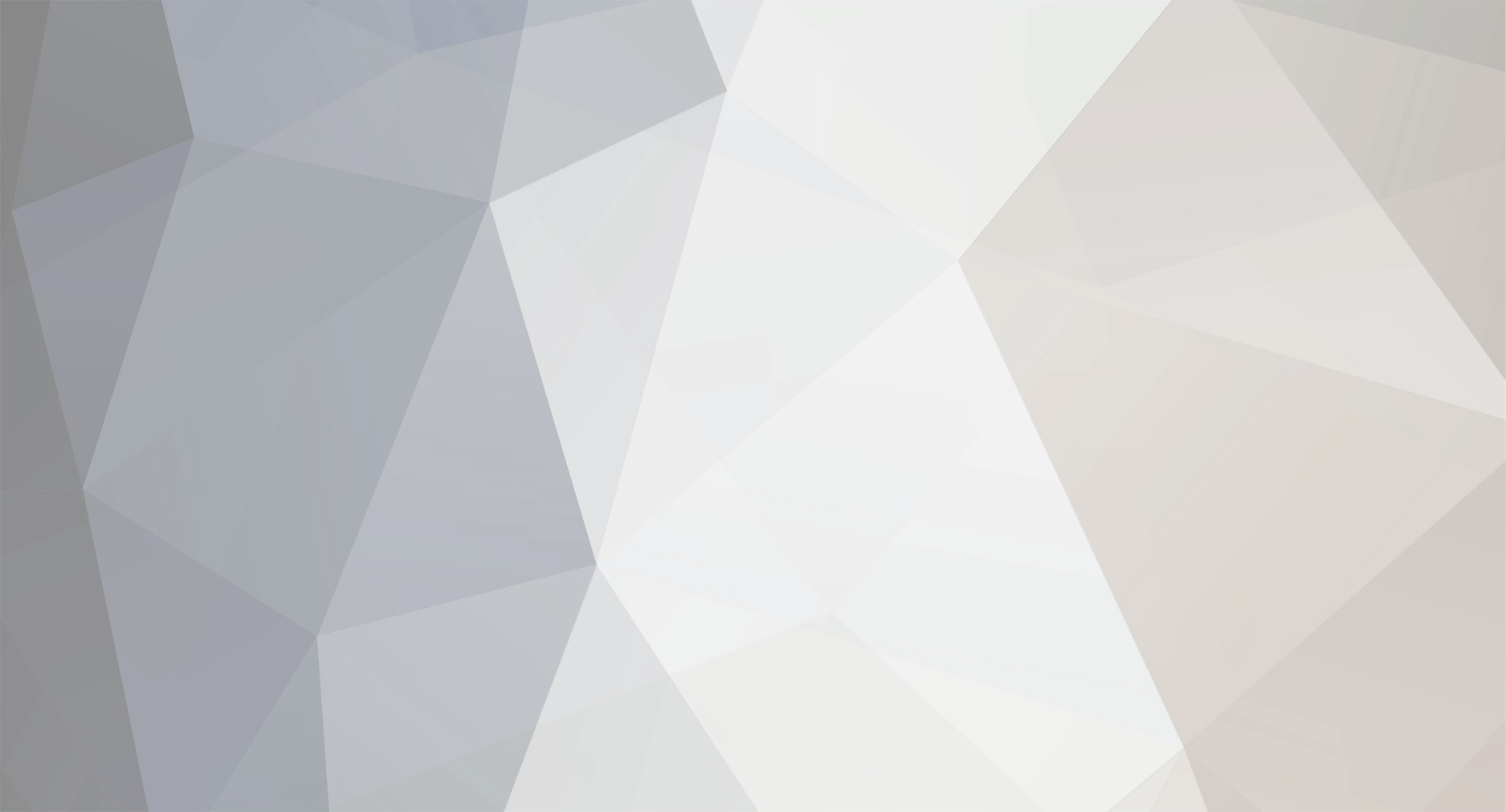
TheGreyGhost
Members-
Posts
3280 -
Joined
-
Last visited
-
Days Won
8
Everything posted by TheGreyGhost
-
Hi This link might help a bit http://greyminecraftcoder.blogspot.co.at/2014/12/blocks-18.html in particular this bit "The same method Block.getActualState() is also used by blocks which change appearance depending on their neighbours - for example BlockFence, BlockRedstoneWire. Block.getActualState() is generally called just before a render, to make sure the block has the correct appearance." -TGG
-
[1.8] Tile Entity check if entity is on top of block
TheGreyGhost replied to Brickfix's topic in Modder Support
The logic looks roughly right. Have you tried debugging it using breakpoints or System.out.println, eg System.out.println("Found entities count:"+list.size()): etc -TGG -
[1.7.10]How would I get the delta x,y, and z from two entites
TheGreyGhost replied to starwarsmace's topic in Modder Support
Hi You are on the right track with vector calculation If you just want the motion to be in a straight line, then it's pretty simple- 1) calculate the motion vector = PositionOfB - PositionOfA 2) normalise the vector to convert it to length 1 regardless of the distance between B and A 3) motionX = vectorX * speed motionY = vectorY * speed motionZ = vectorZ * speed The *0.98 stuff is to slow the projectile down once you have fired it, air resistance as Draco said. It has no effect on the direction. Gravity is a different story, that will make your motion curve downwards, but I guess you aren't worried about that? -TGG -
Hi Try these, taken from EntityLightningBolt this.worldObj.playSoundEffect(this.posX, this.posY, this.posZ, "ambient.weather.thunder", 10000.0F, 0.8F + this.rand.nextFloat() * 0.2F); this.worldObj.playSoundEffect(this.posX, this.posY, this.posZ, "random.explode", 2.0F, 0.5F + this.rand.nextFloat() * 0.2F); You will need to do it on the server, not the client. Playing the sound through World on the client does nothing. You can play a sound locally using other methods eg Minecraft.getMinecraft().getSoundHandler().playSound(sound); but then nobody else on the server will be able to hear it. -TGG
-
Saving a Reference to an Entity (Save/Load Persistence)
TheGreyGhost replied to Draco18s's topic in Modder Support
Hi I think you could try three things - One is to "leash" your minecart similar to what DieSieben suggested, not sure if that works for non-EntityLiving. Second is to replace the vanilla Minecart Entity with a MyCapturableMinecart extends EntityMinecart to make it responsible for maintaining a link to the "capturing" tileentity, or thirdly your TileEntity could despawn the Minecart while it is captured and respawn once it's finished. -TGG > I literally have no way of getting an entity from the World by anything other than incremental spawn ID PS forgot to mention- do you already know about persistent EntityID ? i.e. getPersistentID() or getUniqueID() instead of getEntityId() -
[1.7.10] Help with getting raw texture data
TheGreyGhost replied to SuperKael's topic in Modder Support
Yes, but that large texture sheet isn't referenced by anything anywhere. Well, it probably is, but it's buried so deep that I have never been able to find it. The texture is stored in OpenGL, not as an integer array any more. The texture index is stored in TextureMap.mapTextureObjects and is accessed using this.mc.getTextureManager().bindTexture(TextureMap.locationBlocksTexture); See for example SimpleTexture.loadTexture() and TextureMap.loadTextureAtlas() (for the stitched-together block textures) Neither will do much good if we can't save the data back into the texture sheet. This is very easy to do with DynamicTexture, for example this snippet which stores custom texture info for all six faces for a list of custom blocks public class SelectionBlockTextures { public SelectionBlockTextures(TextureManager i_textureManager) { textureManager = i_textureManager; final int BLOCK_COUNT = 1; final int NUMBER_OF_FACES_PER_BLOCK = 6; final int U_TEXELS_PER_FACE = 16; final int V_TEXELS_PER_FACE = 16; int textureWidthTexels = BLOCK_COUNT * U_TEXELS_PER_FACE; int textureHeightTexels = NUMBER_OF_FACES_PER_BLOCK * V_TEXELS_PER_FACE; TEXELHEIGHTPERFACE = 1.0 / NUMBER_OF_FACES_PER_BLOCK; TEXELWIDTHPERBLOCK = 1.0 / BLOCK_COUNT; blockTextures = new DynamicTexture(textureWidthTexels, textureHeightTexels); textureResourceLocation = textureManager.getDynamicTextureLocation("SelectionBlockTextures", blockTextures); // just for now, initialise texture to all white // todo make texturing properly int [] rawTexture = blockTextures.getTextureData(); for (int i = 0; i < rawTexture.length; ++i) { rawTexture[i] = Color.WHITE.getRGB(); } blockTextures.updateDynamicTexture(); } public void bindTexture() { textureManager.bindTexture(textureResourceLocation); } public SBTIcon getSBTIcon(IBlockState iBlockState, EnumFacing whichFace) { double umin = 0.0; double vmin = 0.0; return new SBTIcon(umin, umin + TEXELWIDTHPERBLOCK, vmin, vmin + TEXELHEIGHTPERFACE); } private final DynamicTexture blockTextures; private final ResourceLocation textureResourceLocation; private final TextureManager textureManager; private final double TEXELWIDTHPERBLOCK; private final double TEXELHEIGHTPERFACE; public class SBTIcon { public SBTIcon(double i_umin, double i_umax, double i_vmin, double i_vmax) { umin = i_umin; umax = i_umax; vmin = i_vmin; vmax = i_vmax; } public double getMinU() { return umin; } public double getMaxU() { return umax; } public double getMinV() { return vmin; } public double getMaxV() { return vmax; } private double umin; private double umax; private double vmin; private double vmax; } } -TGG -
[1.7.10] Help with getting raw texture data
TheGreyGhost replied to SuperKael's topic in Modder Support
The raw textures are inserted into a single texture sheet during initialisation, and after that the Icon just tells the coordinates of the rectangular part of the large texture sheet that corresponds to that item. For example the pickaxe might occupy the rectangle from [u=0.3, v = 0.1] to [u=0.4, v=0.2] So I think there are two ways you can go - either extract the texture name from the Item's model and reload it from the file, or use OpenGL to render the relevant part of the texture sheet into a FrameBufferObject and read it back out. I've never done it myself but a quick google shows up keywords like PixelBufferObject, glReadPixels that seem suitable. It will be tricky unless you know a bit about OpenGL, I think. -TGG -
[1.8] How to render a solidified texture?
TheGreyGhost replied to Bedrock_Miner's topic in Modder Support
Hi The "builtin/generated" items are generated using this code ItemModelGenerator.makeItemModel() and especially func_178394_a() the thickness comes from here which creates the two large flat faces: arraylist.add(new BlockPart(new Vector3f(0.0F, 0.0F, 7.5F), new Vector3f(16.0F, 16.0F, 8.5F), hashmap, (BlockPartRotation)null, true)); (8.5F - 7.5F = 1 texel wide) and then func_178397_a() for all the thin "sideways" faces that make up the edges I think you would need to use this code to generate your own block model for your item, probably using ISmartItemModel and perhaps ICustomModelLoader http://www.minecraftforge.net/forum/index.php/topic,28714.msg147632.html#msg147632 I think it will be fairly tricky... -TGG -
Hi This example project has a working example of an item with NBT https://github.com/TheGreyGhost/MinecraftByExample see MBE12. The interesting stuff is in ItemNBTAnimate.java in onItemRightClick() and onItemUseFinish() -TGG
-
Minecraft.getMinecraft().gameSettings.thirdPersonView -TGG
-
You can probably hack that by using Item.getModel, where you return a different model depending on whether the current view is first person or third person. Custom rendering like the map can probably be done the same way, perhaps using ISmartItemModel. Maps are rendered as a "special case" in ItemRenderer.renderItemInFirstPerson() - see if (this.itemToRender.getItem() == Items.filled_map) { this.func_178097_a(entityplayersp, f3, f1, f2); } It probably won't be much help to you... -TGG
-
Fence Textures don't work if texture pack is on.
TheGreyGhost replied to MacDue's topic in Modder Support
Based on that error message, your fence model needs to define the texture that should be used for #side, not just #texture Perhaps the texture pack models are different to the vanilla models -TGG -
Hi Your orge_crops.json has an error in it JsonSyntaxException: com.google.gson.stream.MalformedJsonException: Unterminated object at line 4 column 10 Which is supposedly in this file { "variants": { "age=0": { "model": "obockmod:orge_crops_0" }, "age=1": { "model": "obockmod:orge_crops_1" }, etc although I have to admit I don't see it. Are you sure this is the actual file? In the correct directory? Try renaming or deleting it and seeing if the error stays the same. There might also be strange characters in there - try pasting it into notepad (not notepad++) to get rid of odd characters, then save it as a .json You could also try copying and pasting it into here http://jsonlint.com/ (I tried your listing and it seemed fine) -TGG
-
Hi What are the symptoms now? What error messages do you get in the console? Cheers TGG
-
Hi Try this troubleshooting guide http://greyminecraftcoder.blogspot.com.au/2015/03/troubleshooting-block-and-item-rendering.html -TGG
-
Hi I'm not sure there's an easy way to do this; although EntityLightningBolt is an "Entity", it isn't treated the same as other entities. It gets "spawned" into its own list of weather effects. The code is in WorldServer:: this.theProfiler.endStartSection("thunder"); int i1; BlockPos blockpos; if (this.provider.canDoLightning(chunk) && this.rand.nextInt(100000) == 0 && this.isRaining() && this.isThundering()) { this.updateLCG = this.updateLCG * 3 + 1013904223; i1 = this.updateLCG >> 2; blockpos = this.func_175736_a(new BlockPos(k + (i1 & 15), 0, l + (i1 >> 8 & 15))); if (this.func_175727_C(blockpos)) { this.addWeatherEffect(new EntityLightningBolt(this, (double)blockpos.getX(), (double)blockpos.getY(), (double)blockpos.getZ())); } } There is also client-side code (see S2CPacketSpawnGlobalEntity.processPacket() but I don't think it will help either. I think you will probably need to use ASM+Reflection to insert your own hook in there. That's a pretty advanced technique. -TGG
-
[1.8] Why the hell don't door blockstates work at all?!
TheGreyGhost replied to MacDue's topic in Modder Support
Dude, look closer It's looking for this facing=west,half=lower,hinge=left,open=false,powered=false and your blockstates json has this facing=east,half=lower,hinge=left,open=false protected BlockState createBlockState() { return new BlockState(this, new IProperty[] {HALF, FACING, OPEN, HINGE, POWERED}); } -TGG -
There are a lot of folks on Mac who still can't even use Java 7. Something about the launcher being incompatible. With any luck that will change soon (or has already? there was a new launcher released recently?) -TGG
-
[SOLVED] Trouble with Blockstates and a Torch-like block
TheGreyGhost replied to TheRedMezek's topic in Modder Support
Hi This troubleshooting guide might help http://greyminecraftcoder.blogspot.com.au/2015/03/troubleshooting-block-and-item-rendering.html and perhaps this background information on states http://greyminecraftcoder.blogspot.co.at/2014/12/blocks-18.html Also this example mod (MBE03) https://github.com/TheGreyGhost/MinecraftByExample For vanilla see BlockTorch. you are probably missing protected BlockState createBlockState() { return new BlockState(this, new IProperty[] {FACING}); } ? -TGG -
Makes sense to me And from what I've seen, it would make you the second person in the history of modding to use automated testing on their mod I can tell you from painful experience of two version upgrades that regression testing in particular is very very important. -TGG
-
[1.7.10] Ticking Memory Connection-- packet problem?
TheGreyGhost replied to Athire's topic in Modder Support
Hi The error has nothing to do with packet handling. public mageSpellList mageList; public unholySpellList necroList; public holySpellList holyList; //Stores id's to send via packets public int[] mageIDs =new int[mageList.listOfSpells.length]; // NPE here These links might help http://frequal.com/java/DebuggingNullPointerExceptions.html http://www.terryanderson.ca/debugging/run.html -TGG -
[1.8] Block Does Not Have Texture When Placed In World
TheGreyGhost replied to LogicTechCorp's topic in Modder Support
Hi Yes there is a way. I think this link might help you understand blockstates and block rendering better. http://greyminecraftcoder.blogspot.com.au/p/list-of-topics.html (Three topics under 'block rendering'). This example project has a block with variants with different model files https://github.com/TheGreyGhost/MinecraftByExample (see MBE03) -TGG -
[1.8] Block Does Not Have Texture When Placed In World
TheGreyGhost replied to LogicTechCorp's topic in Modder Support
Hi Show test code and console message for your simple item? -TGG -
[1.8] Block Does Not Have Texture When Placed In World
TheGreyGhost replied to LogicTechCorp's topic in Modder Support
Looks to me like you're registering your blocks with dud names like ore.raw.alt stone.brick.mossy Try getting rid of the dots - comment out your complicated registration stuff and just hand write a couple of simple text names. If that works, you've found your problem. -TGG