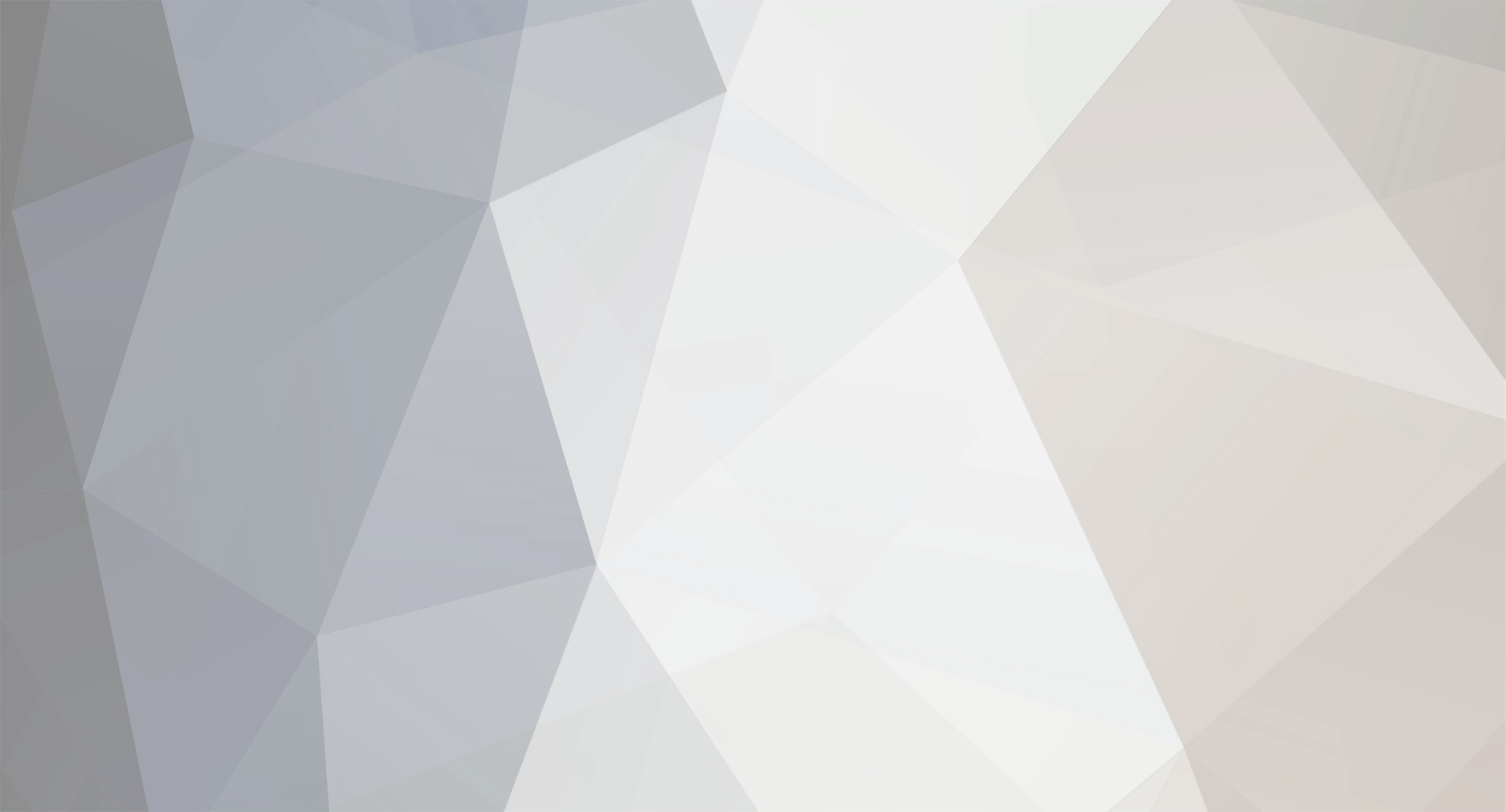
TheGreyGhost
Members-
Posts
3280 -
Joined
-
Last visited
-
Days Won
8
Everything posted by TheGreyGhost
-
Hi This project has an example of drinking https://github.com/TheGreyGhost/MinecraftByExample (see example 11) Item.getItemUseAction() is what you're looking for. -TGG
-
Hi By far the easiest way to track these down is to use your debugger. These links might help http://www.vogella.com/tutorials/EclipseDebugging/article.html http://www.terryanderson.ca/debugging/run.html I think it's well worth spending a couple of hours learning how to use your debugger properly because it will pay you back many times over, you'll wonder how you ever managed without it. Also a comment - it's bad style to name your object instances with a capital, it makes them look like classes. i.e. public static Item itemTest; // good public static Block BlockTest; // bad, should be Block blockTest -TGG
-
[1.7.10]Custom Rendered Block Questions
TheGreyGhost replied to Wyverndoes's topic in Modder Support
Actually you can place a fence on carpet, it just doesn't touch it (hovers above it). It's because each block in Minecraft is for a single block only. You can't overlap blocks. In order to place a fence directly on carpet, you would need to create a "fence on carpet" block. This link explains it a bit more. http://greyminecraftcoder.blogspot.co.at/2014/12/blocks-18.html -TGG -
[1.7.10] Entities won't show up when raytracing
TheGreyGhost replied to Eternaldoom's topic in Modder Support
It's because rayTraceBlocks only raytraces blocks, not entities. Look in EntityRenderer.getMouseOver() for the code which raytraces entities as well... -TGG -
[Forge 1.8] How to Render Blocks in Inventory?
TheGreyGhost replied to HassanS6000's topic in Modder Support
No worries, you're welcome -
Thanks, I'll take a look The project is at https://github.com/TheGreyGhost/MinecraftByExample -TGG
-
Howdy folks have any of you coded up a custom entity I could steal to use for an example code project? Just the basic stuff like - registering - spawning (spawn frequency in biomes) - simple AI - simple datawatcher - base attributes (health, damage, etc) Never coded an entity myself and although it would be fun to learn it'd be a lot faster if I had a good base to start from.... The example project is for 1.8 but I figure 1.7 entities will probably work just the same. -TGG
-
[Forge 1.8] How to Render Blocks in Inventory?
TheGreyGhost replied to HassanS6000's topic in Modder Support
Hi You should register your items and blocks in FMLPreInitializationEvent , not FMLInitializationEvent. The getItemModelMesher() stuff needs to come in FMLInitializationEvent, after you have registered the blocks and items. Also just something I noticed - instances of your classes should always start with a lower case, otherwise it looks like they are classes, which is pretty confusing for anyone who reads your code For example SpartanBoots = new HaloArmor(HaloArmor, bootID, 3).setUnlocalizedName("SpartanBoots"); // should be spartanBoots This resource is really good for learning how to format your code to make it easy for others to understand http://google-styleguide.googlecode.com/svn/trunk/javaguide.html -TGG -
Render Model face closest player function(s)
TheGreyGhost replied to Durand1w's topic in Modder Support
Hi I think the code is so simple (short) you needn't bother looking too hard. Just copy it out of the entity facing code which makes (eg) the wolf look at you. Your code just needs to provide it with the relative [x,y,z] of the nearest player which I think you have figured out already? You need the yaw and the pitch. EntityLookHelper.onUpdateLook() does the calculations like this, i.e. double d0 = this.posX - this.entity.posX; double d1 = this.posY - (this.entity.posY + (double)this.entity.getEyeHeight()); double d2 = this.posZ - this.entity.posZ; double d3 = (double)MathHelper.sqrt_double(d0 * d0 + d2 * d2); float f = (float)(Math.atan2(d2, d0) * 180.0D / Math.PI) - 90.0F; float f1 = (float)(-(Math.atan2(d1, d3) * 180.0D / Math.PI)); this.entity.rotationPitch = this.updateRotation(this.entity.rotationPitch, f1, this.deltaLookPitch); this.entity.rotationYawHead = this.updateRotation(this.entity.rotationYawHead, f, this.deltaLookYaw); -TGG -
[1.7.10] Approximating a rotated bounding box for an entity.
TheGreyGhost replied to Blargerist's topic in Modder Support
Hi Well if you want your sand to slide off other blocks, you don't need to use multiple bounding boxes at all. Just put some custom "sliding collision" logic in the entity update method, to replace the vanilla update code in your entity. eg get the collision boxes of the nearby objects and use your custom non-axis-aligned collision detection to figure out which way the entity should move. If you want other blocks to slide off your custom block, then that's a lot harder, but I don't think you need that? -TGG -
Problem with lighting for rendered item in TESR
TheGreyGhost replied to McJty's topic in Modder Support
Hi My guess is the "item lighting" since the top face is dark even when the side faces are bright, although it does look like you've done the right things with enabling & disabling it. I've had similar issues before and the only way I managed to track them down was trial and error enabling/disabling various settings - eg if you disable item lighting and the inconsistency goes away, you at least know it has something to do with that. You could also try drawing using the tessellator instead of the vanilla item, to see whether the vanilla is somehow messing with it. Some thoughts on stuff that has caused me similar problems before- - item lighting on/off - world "brightness" setting - changing settings on the tessellator vs using GL11.enable - rescale normals (used for item lighting) I have a tool which was some use to me in tracking stuff down - it dumps the state of the enable flags, can also be used for the other opengl settings such as lighting https://github.com/TheGreyGhost/MinecraftByExample/blob/master/src/main/java/minecraftbyexample/usefultools/OpenGLdebugging.java dumpAllIsEnabled() works; I'm not sure about the rest Good luck with it -TGG -
[1.7.10] Approximating a rotated bounding box for an entity.
TheGreyGhost replied to Blargerist's topic in Modder Support
Hi I'm not sure what you mean by "partially slides through blocks". What are you trying to achieve, exactly? I think that Axis Aligned Bounding Box might not actually have anything to do with it. AABB is used for detecting collisions with other entities or with the scenery, I don't think it affects the entity render. -TGG -
[Forge 1.8] How to Render Blocks in Inventory?
TheGreyGhost replied to HassanS6000's topic in Modder Support
Hi What are your symptoms? i.e. what does the item look like in your inventory, and what error message(s) do you get in the console? -TGG -
[SOLVED] Performance Bottleneck with Multithreading
TheGreyGhost replied to FlameAtronach93's topic in Modder Support
Hi I reckon adding extra threads is only an advantage if your performance is bound by the CPU. If you're constrained by memory or disk access then the extra threads probably won't help and in fact if they're blocking the server thread they will make things worse. You might benefit from a good profiling/system monitoring tool. Even something like SysInternal's Process Explorer will be a good start. If the server starts falling behind on its tick execution for more than a half a second, the player will probably notice it, i.e. it's a problem, otherwise not. If the server run() method is getting at least some idle time each tick then it's ok. An average above 50ms is of course unacceptable. You want to have some spare time per tick for when something happens, but guaranteed the player can always make your server lag (eg blowing up a stack of TNT). If you want to maximise the time per tick, instead of creating a new thread for your 'background' tasks, you could instead put them into a server tick event and keep track of the server load using System.nanoTime(). i.e. as long as the tick spacing remains stable, slowly increase the amount of time your background tasks use per tick. But if your tick event starts arriving later than 50 ms intevals, throttle back your background tasks or skip them entirely until the server catches up again. The Server tick event has pre and post events which should give you enough information to tell how long each tick is taking. -TGG -
Fire an event after a specific number of server ticks?
TheGreyGhost replied to ianc1215's topic in Modder Support
This guy's tutorial on Events is very helpful I think. http://www.wuppy29.com/minecraft/modding-tutorials/wuppys-minecraft-forge-modding-tutorials-for-1-7-events/ Events are very straightforward once you've written your first handler -TGG -
I've successfully used RenderWorldLastEvent and then used the tessellator to draw a wire frame or similar. You just need to draw relative to the player's position i.e. EntityPlayer.getPosition(partialTick); So - if the player is at x = 23.5, and the chunk you want to render is from x = 64 to 80, then your wireframe renders from x = 40.5 to 56.5 You will need to understand how to register Forge Events and how to use the Tessellator. Do you know how to use those? -TGG
-
Fire an event after a specific number of server ticks?
TheGreyGhost replied to ianc1215's topic in Modder Support
Hi If you're running a tick event anyway (ServerTickEvent - have you used Forge (FML) events before?) I would just add a counter. eg tickEvent(ServerTickEvent event) { tickCount++; int ticksElapsedSinceTrigger = tickCount - triggerTickCount; if (ticksElapsedSinceTrigger >= EVENT_DELAY) { // write ye texte file } } I have successfully written text files to the minecraft game directory and saves directory. Don't know if you can escape outside those. Probably yes, I imagine. -TGG -
[1.6.4][SOLVED!] How to get a players instance (SERVER SIDE)
TheGreyGhost replied to pokeyletsplays's topic in Modder Support
Hi The fact that it works client side makes me think that this.getUser() is null or is the wrong username on the server side. Have you checked using System.out.println() or a breakpoint? -TGG -
Hi Yep. It's about 32000. -TGG
-
How do I increase the reach of the player?
TheGreyGhost replied to memcallen's topic in Modder Support
Dude, you could call that method ten thousand times per tick and the user wouldn't notice. -TGG -
[Forge 1.8] Mod Not Working!!! Need HELP!!!!!!!!
TheGreyGhost replied to xenoxue's topic in Modder Support
when you say "export", do you mean gradlew build You need to use gradlew build to export your mod to a jar. It will appear in the build/libs folder. -TGG -
Hi Look at vanilla Entity subclasses. They all do this. http://greyminecraftcoder.blogspot.com.au/2013/10/the-most-important-minecraft-classes_9.html (diagram at bottom) -TGG
-
[Forge 1.8] How to Render Blocks in Inventory?
TheGreyGhost replied to HassanS6000's topic in Modder Support
Hi This site has some info on Block Rendering http://greyminecraftcoder.blogspot.com.au/p/list-of-topics.html (See the four topics on Blocks and BLock rendering) and the one on items http://greyminecraftcoder.blogspot.com.au/2014/12/item-rendering-18.html There is also an example project showing blocks and items here- https://github.com/TheGreyGhost/MinecraftByExample (see example 1) https://github.com/TheGreyGhost/MinecraftByExample/tree/master/src/main/java/minecraftbyexample/mbe01_block_simple The short answers are- yes, you need a JSON file for the item. No, your items doesn't need its own texture, your item can use the block texture. And you also need to do Item itemBlockSimple = GameRegistry.findItem("minecraftbyexample", "mbe01_block_simple"); ModelResourceLocation itemModelResourceLocation = new ModelResourceLocation("minecraftbyexample:mbe01_block_simple", "inventory"); final int DEFAULT_ITEM_SUBTYPE = 0; Minecraft.getMinecraft().getRenderItem().getItemModelMesher().register(itemBlockSimple, DEFAULT_ITEM_SUBTYPE, itemModelResourceLocation); -TGG -
You have to make sure your object is rendered after the water, if your object is closer than the water. For water that works fine, but if you (say) look through ice at your object, it will look mighty strange. Likewise if you look through one of your objects to see a second one of your objects I don't think in 1.7.10 that this can be properly solved without a big effort. In 1.8, transparent blocks are depth-sorted so it should work better! -TGG
-
[1.8][SOLVED] Problem with rendering of smaller blocks
TheGreyGhost replied to peter1745's topic in Modder Support
no worries