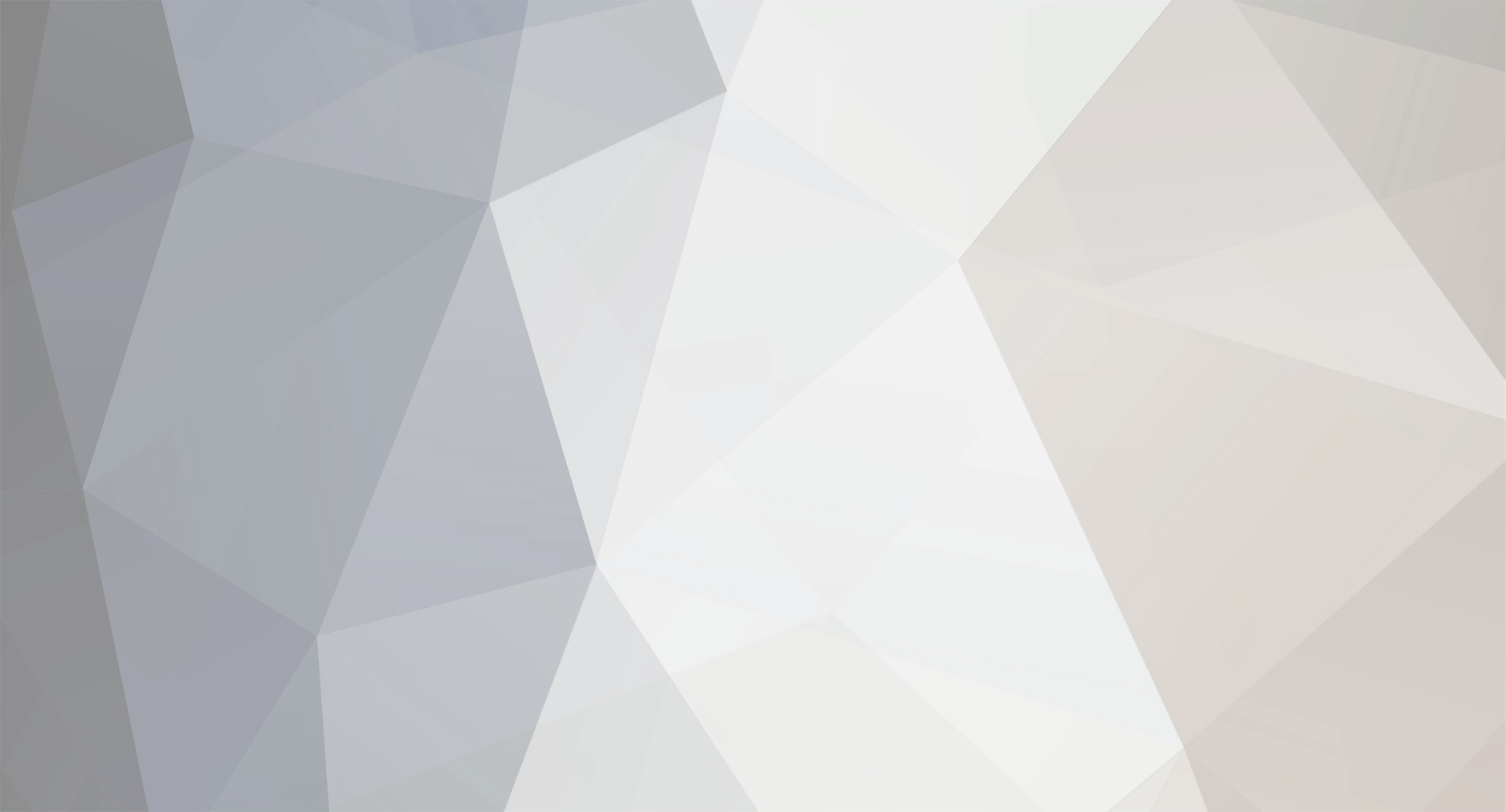
TheGreyGhost
Members-
Posts
3280 -
Joined
-
Last visited
-
Days Won
8
Everything posted by TheGreyGhost
-
Hi Unfortunately there is no easier way to do it, but I wouldn't worry about optimising it, if you're only drawing a few faces it will take no time at all even if your code is very inefficient. Because the faces are parallel to the x, y, or z axes, sorting is actually very easy. For example - consider the two faces on the y axis - the top face at y[top] and the bottom face at y[bottom] Look at the player's y. If y > y[top], draw the bottom face first If y < y[bottom], draw the top face first. Otherwise, it doesn't matter. Similarly with the x and z faces. Once you have figured out which face is furthest for each axis, draw them in this order x furthest, y furthest, z furthest then x closest, y closest, z closest As easy as that You're right that the z buffer will not help you, and GL_ALPHA_TEST is no use to you either. That's only for textures with pixels that are either fully opaque or fully transparent. -TGG
-
Ye gods man! Where did @Instance come from? It's used by forge to find a place to store your mod instance. I really doubt you need it here! -TGG
-
Hi You might find this link about block mining interesting http://greyminecraftcoder.blogspot.ch/2015/01/mining-blocks-with-tools.html and http://greyminecraftcoder.blogspot.ch/2015/01/summary-of-logic-flow-when-mining-blocks.html -TGG
-
[1.8] How do we use ISmartItemModel to create dynamic models?
TheGreyGhost replied to TrashCaster's topic in Modder Support
Ah. I think I've misunderstood what you want. I reckon your thought about getItemModel() in the ItemMesher is right, i.e.: If your ItemStack has an NBT with (say) two ItemStacks "A" and "B" in it, you would get the vanilla IBakedModels for those items using IBakedModel ibakedmodel = Minecraft.getMinecraft().getRenderItem().getItemModelMesher().getItemModel(itemStackA); and same for B. The only trick is - if the Camera Transforms aren't the same for both items, you will have to choose one of the two... -TGG -
[1.8] Custom walls displaying duplicate creative tab items *SOLVED*
TheGreyGhost replied to csb123's topic in Modder Support
You're welcome -
[1.7.10] Tile Entity Owned Resources and shouldRefresh
TheGreyGhost replied to Bystander's topic in Modder Support
> The reality is the block is only orphaned/removed on the client side, I don't think this is true. The server unloads the chunk with TileEntities when the player is a certain distance away, so you would get the same problem. The inventory is saved in NBT format on the server side, which is why TileEntities don't lose information when they're unloaded. Which you can't do for your object reference unless you use some sort of key, which comes back to using a registry. -TGG PS see here for a bit of background info - which you may know already, but just to be sure http://greyminecraftcoder.blogspot.com.au/2015/01/tileentity.html -
Do you mean you can see the outline of a block (cube) but not the actual model shape? Or can you see the outline of the model shape but it's transparent? If the first one - go to near x=0, z = 0 and try placing your block way up in the sky. It might be rendering in the wrong x,y,z location, and that will give you a chance to see where it is actually rendering. That sheep is very distracting, by the way. -TGG
-
[1.8] Custom walls displaying duplicate creative tab items *SOLVED*
TheGreyGhost replied to csb123's topic in Modder Support
Some ideas: Do you still get two duplicates if you search for your item on the search tab? What if you set the tab to a vanilla creative tab instead of your custom? Do your blocks have multiple metadata? What does your getSubItems() (or Block.getSubBlocks() ) look like? You could try adding a breakpoint into ItemModelMesher.getItemModel() if (ibakedmodel == null) { ibakedmodel = this.modelManager.getMissingModel(); } - this is where it silently substitutes the missing model if it can't find the one it's looking for. This will tell you what stack it's looking for, and with any luck why it's not finding it. -TGG -
[1.7.10] Help needed with understanding EntityArrowObject
TheGreyGhost replied to SSslimer's topic in Modder Support
Well then - try it and see Put a system.out.println({position}) in the arrow's tick update (don't forget that it will run on both client and server), and you will be able to calculate the distance per tick very easily. -TGG -
Hi This link goes into quite some detail on it http://greyminecraftcoder.blogspot.com.au/p/list-of-topics.html (See the Blocks link and the Item link). This example project shows how to make 3D blocks and corresponding items. 3D items are done in exactly the same way, i.e. you use a Block Model file with an "elements" section. https://github.com/TheGreyGhost/MinecraftByExample -TGG
-
[1.7.10] Tile Entity Owned Resources and shouldRefresh
TheGreyGhost replied to Bystander's topic in Modder Support
I don't think there is a robust way of controlling the resource lifetime, this is more a Java problem than a minecraft problem. You'll probably have a similar issue when the chunk unloads and the TileEntity is orphaned. When a TileEntity is (re)created, it's just an empty shell until something reloads its contents by calling readFromNBT. You could perhaps make your ticked looping sound responsible for checking if the TileEntity exists, rather than the other way around. Alternatively, as you said, you could have a sound registry that you service at regular intervals using a tick handler, along with the tile entity coordinates for each looping sound. (This is what I would try first personally). -TGG -
[1.7.10] Help needed with understanding EntityArrowObject
TheGreyGhost replied to SSslimer's topic in Modder Support
Hi Based on the code in setThrowableHeading(), it appears to be the initial speed of the arrow, probably in metres per tick. -TGG -
[1.8] Custom walls displaying duplicate creative tab items *SOLVED*
TheGreyGhost replied to csb123's topic in Modder Support
Hi Do you see any error messages about missing texture or missing model in the console? If you cursor over the models with missing texture, what name does it say? -TGG -
for sure you would need to add some extra "error" detection to the basic idea. Probably the best is a hybrid. Four times per second the client sends a packet. Each packet says "fire a burst for 250s" or similar. A bit of experimentation on a laggy server will show the best strategy from the player's point of view.. -TGG
-
I suggest: 1) On the client side, detect when the player is holding down the fire button. You can do this by overriding Item.onEntitySwing (return true to stop further vanilla processing). 2) Send a packet to the server to start firing the gun. 3) On the server, you have a tick handler which is called 20 times per second. 4) When the "start firing" packet arrives at the server, fire a bullet every x ticks, where x depends on your desired firing rate. Push the player backwards too. 5) When the client side detects that the player isn't holding down the fire button any more, send a "stop firing" packet to the server. You'll probably need to tweak the logic a bit to get it how you want, but the basic idea should work. -TGG
-
Hi Some info on TE that might be useful. For 1.8 but almost identical for 1.7 http://greyminecraftcoder.blogspot.com.au/2015/01/tileentity.html https://github.com/TheGreyGhost/MinecraftByExample/tree/master/src/main/java/minecraftbyexample/mbe20_tileentity_data The block can use world.getTileEntity(x,y,z) to get its corresponding TileEntity. Don't forget to check for null in case it hasn't been initialised yet. -TGG
-
[1.7.10] Opening Achievement Page causes Crash...
TheGreyGhost replied to xXxKanemanxXx's topic in Modder Support
Hi One of your achievements appears to be null. A breakpoint should very quickly show you which one. http://www.vogella.com/tutorials/EclipseDebugging/article.html -TGG -
[1.8]Where can I get the saves directory for the current world?
TheGreyGhost replied to Mecblader's topic in Modder Support
Not to forget, the saved game is different on an integrated server than on the dedicated server. I used this in 1.7.10. It's probably similar in 1.8. In my proxy classes: DedicatedServer:: /** * Obtains the folder that world save backups should be stored in. * For Integrated Server, this is the saves folder * For Dedicated Server, a new 'backupsaves' folder is created in the same folder that contains the world save directory * * @return the folder where backup saves should be created */ @Override public Path getOrCreateSaveBackupsFolder() throws IOException { Path universeFolder = FMLServerHandler.instance().getSavesDirectory().toPath(); Path backupsFolder = universeFolder.resolve(SpeedyToolsOptions.nameForSavesBackupFolder()); if (!Files.exists(backupsFolder)) { Files.createDirectory(backupsFolder); } return backupsFolder; } IntegratedServer (ClientProxy):: /** * Obtains the folder that world save backups should be stored in. * For Integrated Server, this is the saves folder * For Dedicated Server, a new 'backupsaves' folder is created in the same folder that contains the world save directory * * @return the folder where backup saves should be created */ @Override public Path getOrCreateSaveBackupsFolder() throws IOException { return new File(Minecraft.getMinecraft().mcDataDir, "saves").toPath(); } -TGG -
Hi I moved them to a new topic... http://www.minecraftforge.net/forum/index.php/topic,27413.0.html pls start a new post when you have a new question otherwise we wind up with ridiculously long reply chains and the subject has nothing to do with the question any more... -TGG
-
[1.7.10] Commenting on the JSON (for Configuration)
TheGreyGhost replied to Abastro's topic in Modder Support
Comments aren't possible in JSON. The guy who designed it deliberately removed them to avoid folks misusing them for metadata. The best you can do is descriptive property names I think.. -TGG -
Vanilla's chest gets the break texture through a filthy kludge BlockModelShapes.getTexture(IBlockState state) if (block == Blocks.wall_sign || block == Blocks.standing_sign || block == Blocks.chest || block == Blocks.trapped_chest || block == Blocks.standing_banner || block == Blocks.wall_banner) { return this.modelManager.getTextureMap().getAtlasSprite("minecraft:blocks/planks_oak"); } //.. etc... You can't do that, so I think you need to make a block model for block/cube, give it a "particle": texture. leave the other sides blank (create an empty texture for them). -TGG
-
Problems upgrading to 1.8 - IBlockState
TheGreyGhost replied to BlazeAxtrius's topic in Modder Support
Hi Blaze You're digging deep enough now that we aren't going to be able to help you much more unless you get a thorough understanding of some of the basics of 1.8, including IBlockState. There are a few tutorials around (Wuppy's for example) that will help you get up to speed and make it a lot more frustrating for everyone -TGG -
It is happening because the rendering order of partially transparent objects is important. If you draw the nearest face first, it completely hides the furthest face due to depth culling. You need to draw the further face first, then the nearest face. -TGG
-
Any particular reason why not? They look equivalent to me in this case? The javadoc from hasTileEntity() looks like it was written many versions ago and is no longer true. * Called throughout the code as a replacement for block instanceof BlockContainer * Moving this to the Block base class allows for mods that wish to extend vanilla * blocks, and also want to have a tile entity on that block, may. The only reason now is to allow you to have TileEntity or not depending on the block metadata (block state)? -TGG