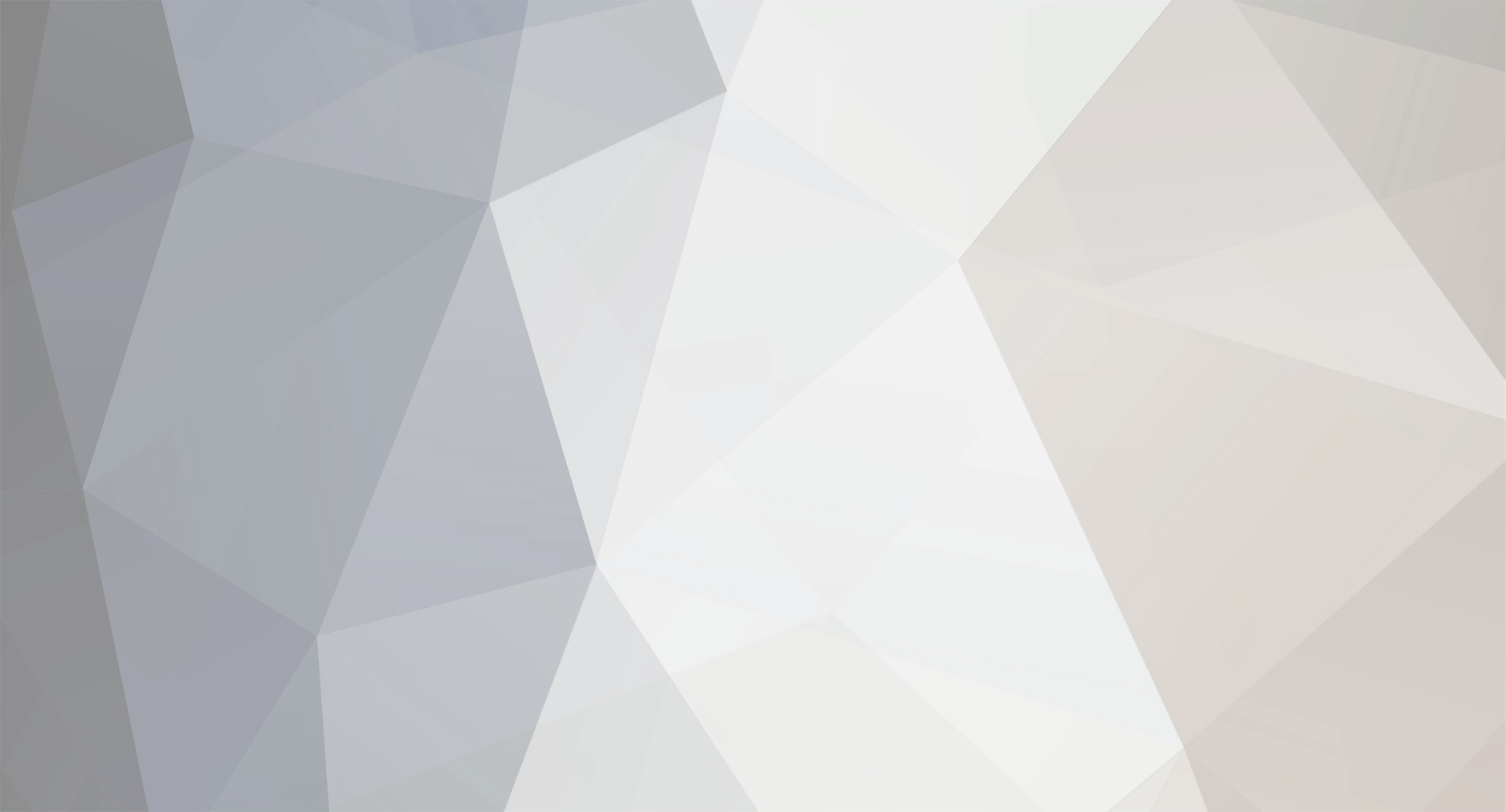
TheGreyGhost
Members-
Posts
3280 -
Joined
-
Last visited
-
Days Won
8
Everything posted by TheGreyGhost
-
[1.8][SOLVED] Problem with rendering of smaller blocks
TheGreyGhost replied to peter1745's topic in Modder Support
hi Have you overridden Block.isOpaqueCube and .isFullCube? // used by the renderer to control lighting and visibility of other blocks. // set to false because this block doesn't fill the entire 1x1x1 space @Override public boolean isOpaqueCube() { return false; } // used by the renderer to control lighting and visibility of other blocks, also by // (eg) wall or fence to control whether the fence joins itself to this block // set to false because this block doesn't fill the entire 1x1x1 space @Override public boolean isFullCube() { return false; } -TGG -
[1.8] Trying to make a particle with a custom texture
TheGreyGhost replied to The_SlayerMC's topic in Modder Support
If you solve it, pls give me some snippets so I can add it to my example code project? -TGG -
Aiiieeee {screams of agony as minecraft crashes randomly yet again with a strange error message} that should be if (worldObj.isRemote) Slip of the keys I guess? you must not access Minecraft from the server side. If you have access to a world or even better a worldclient, for a vanilla particle you can also use eg worldClient.spawnParticle("particlenamehere", spawnXpos, spawnYpos, spawnZpos, 0, 0, 0); -TGG
-
Hi These links might help http://greyminecraftcoder.blogspot.com.au/2015/01/tileentity.html and http://greyminecraftcoder.blogspot.com.au/2015/01/gui-containers.html This link has an example code project which is for 1.8 but is very similar to 1.7 see examples 20 and 30 https://github.com/TheGreyGhost/MinecraftByExample -TGG
-
Hi The RenderGameOverlayEvent.Pre is your friend. /** * Draw the custom crosshairs if reqd * Otherwise, cancel the event so that the normal crosshair is drawn. * @param event */ @SubscribeEvent public void renderOverlayPre(RenderGameOverlayEvent.Pre event) { if (event.type == RenderGameOverlayEvent.ElementType.CROSSHAIRS) { ClientSide.speedyToolRenderers.render(renderGameOverlayCrosshairsEvent, event.partialTicks); event.setCanceled(renderGameOverlayCrosshairsEvent.isCanceled()); } return; } You will also need to know - - how to subscribe your eventhandler to the event bus, http://www.wuppy29.com/minecraft/modding-tutorials/wuppys-minecraft-forge-modding-tutorials-for-1-7-events and how to use OpenGL to draw your custom cursor directly to the screen. (This is trickier. The fragment below might give you some clues. Let us know if you need more guidance.) -TGG ScaledResolution scaledResolution = event.resolution; int width = scaledResolution.getScaledWidth(); int height = scaledResolution.getScaledHeight(); final float Z_LEVEL_FROM_GUI_IN_GAME_FORGE = -90.0F; // taken from GuiInGameForge.renderCrossHairs final double CROSSHAIR_ICON_WIDTH = 88; final double CROSSHAIR_ICON_HEIGHT = 88; final double CROSSHAIR_ICON_RADIUS = 40; final double CROSSHAIR_X_OFFSET = -CROSSHAIR_ICON_WIDTH / 2.0; final double CROSSHAIR_Y_OFFSET = -CROSSHAIR_ICON_HEIGHT / 2.0; try { GL11.glPushAttrib(GL11.GL_ENABLE_BIT); GL11.glEnable(GL11.GL_BLEND); GL11.glBlendFunc(GL11.GL_SRC_ALPHA, GL11.GL_ONE_MINUS_SRC_ALPHA); GL11.glColor3d(lineColour.R * ringColourIntensity, lineColour.G * ringColourIntensity, lineColour.B * ringColourIntensity); Minecraft.getMinecraft().renderEngine.bindTexture(ringTexture); GL11.glPushMatrix(); GL11.glTranslatef(width / 2, height / 2, Z_LEVEL_FROM_GUI_IN_GAME_FORGE); drawTexturedRectangle(CROSSHAIR_X_OFFSET * starSize * ringSize, CROSSHAIR_Y_OFFSET * starSize * ringSize, Z_LEVEL_FROM_GUI_IN_GAME_FORGE, CROSSHAIR_ICON_WIDTH * starSize * ringSize, CROSSHAIR_ICON_HEIGHT * starSize * ringSize); GL11.glPopMatrix(); GL11.glColor3d(lineColour.R * starColourIntensity, lineColour.G * starColourIntensity, lineColour.B * starColourIntensity); Minecraft.getMinecraft().renderEngine.bindTexture(octoStarTexture); GL11.glPushMatrix(); GL11.glTranslatef(width / 2, height / 2, Z_LEVEL_FROM_GUI_IN_GAME_FORGE); GL11.glRotated( (clockwiseRotation ? degreesOfRotation : - degreesOfRotation), 0, 0, 1.0F); drawTexturedRectangle(CROSSHAIR_X_OFFSET * starSize, CROSSHAIR_Y_OFFSET * starSize, Z_LEVEL_FROM_GUI_IN_GAME_FORGE, CROSSHAIR_ICON_WIDTH * starSize, CROSSHAIR_ICON_HEIGHT * starSize); GL11.glPopMatrix(); final float ARC_LINE_WIDTH = 4.0F; if (drawTaskCompletionRing) { double progressBarIntensity = RING_COLOUR_MAX_INTENSITY; GL11.glColor3d(lineColour.R * progressBarIntensity, lineColour.G * progressBarIntensity, lineColour.B * progressBarIntensity); GL11.glDisable(GL11.GL_TEXTURE_2D); GL11.glPushMatrix(); GL11.glLineWidth(ARC_LINE_WIDTH); GL11.glTranslatef(width / 2, height / 2, Z_LEVEL_FROM_GUI_IN_GAME_FORGE); drawArc(CROSSHAIR_ICON_RADIUS * ringSize, 0.0, clockwiseRotation ? taskCompletionRingAngle : 360.0 - taskCompletionRingAngle, 0.0 ); GL11.glPopMatrix(); } } finally { GL11.glPopAttrib(); } and /** * Draws a textured rectangle at the given z-value, using the entire texture. Args: x, y, z, width, height */ private void drawTexturedRectangle(double x, double y, double z, double width, double height) { double ICON_MIN_U = 0.0; double ICON_MAX_U = 1.0; double ICON_MIN_V = 0.0; double ICON_MAX_V = 1.0; Tessellator tessellator = Tessellator.instance; tessellator.startDrawingQuads(); tessellator.addVertexWithUV( x + 0, y + height, z, ICON_MIN_U, ICON_MAX_V); tessellator.addVertexWithUV(x + width, y + height, z, ICON_MAX_U, ICON_MAX_V); tessellator.addVertexWithUV(x + width, y + 0, z, ICON_MAX_U, ICON_MIN_V); tessellator.addVertexWithUV( x + 0, y + 0, z, ICON_MIN_U, ICON_MIN_V); tessellator.draw(); }
-
Hi This link might help you understand blockstates, model files, and textures a bit more. http://greyminecraftcoder.blogspot.com.au/p/list-of-topics.html (see the four topics on ~blocks) The example project here also has a couple of examples on how to set up block models properly (examples 1 - 3) https://github.com/TheGreyGhost/MinecraftByExample -TGG
-
Hi This example project shows you what the assets folder structure should look like in your project. https://github.com/TheGreyGhost/MinecraftByExample -TGG
-
Ah. I just went to look for the vanilla chest model - there isn't one. It's drawn using a different rendertype so it has no blockmodel like most other blocks do. The TileEntitySpecialRenderer renders it instead, so the lid animation can be done properly. --> if you want a block texture for the chest you'll need to make them yourself. You can find the texture sheet in ("textures/entity/chest/normal.png"); -TGG
-
Thanks guys. I recompiled for V6 and a whole stack of code to do with Path needs rewriting, which is just a bit too much work for little return @SanAndreasP - thx for link. After a bit more googling to understand it, I found another similar one which goes into a little bit more detail http://gaming.stackexchange.com/questions/178178/making-minecraft-run-with-java-8-on-os-x-10-10 The second one (from "Teilo") looks simpler... -TGG
-
A trap to watch out for - in 1.8, your IMessageHandler now runs in a separate thread (i.e. not in the client or server thread). If you try to access vanilla objects in your handler, it will cause concurrency bugs (i.e. crashes, corruption and general weirdness). This link talks a bit more about how to modify for 1.8 http://greyminecraftcoder.blogspot.com.au/2015/01/thread-safety-with-network-messages.html -TGG
-
Ah. The problem appears to be that your blockstates is wrong. It doesn't have the facing variants that it expects to see - you have given your block four facings but the blockstates file doesn't have any of them. Did you look in the links I sent earlier? You need a blockstates file something like { "variants": { "facing=north": { "model": "minecraftbyexample:mbe03_block_variants_model_red"}, "facing=east": { "model": "minecraftbyexample:mbe03_block_variants_model_red", "y": 90 }, "facing=south": { "model": "minecraftbyexample:mbe03_block_variants_model_red", "y": 180 }, "facing=west": { "model": "minecraftbyexample:mbe03_block_variants_model_red", "y": 270 }, } } -TGG
-
Hi all One of the users of a mod I made can't use it because I wrote it using the SDK for 7 and he only has 6 installed. He says he has the latest version installed. Does Minecraft 1.7.10 for Mac only work on Java 6? -TGG
-
Yep The gradle build command creates a jar in build\libs. If you zip up the classes, they still have all the deobfuscated names (like "wood"), and when you try to run it as a mod, none of the names match up. Hence the error message you got. But the jar in build\libs has all your code converted to use the original ("obfusctated") vanilla names (like "field_151575_d"). Try it and see... -TGG
-
[1.8] (SOLVED) make particle render from further away
TheGreyGhost replied to sigurd4's topic in Modder Support
What particles are you talking about? If you are spawning them using World.spawnParticle(): @SideOnly(Side.CLIENT) public void spawnParticle(EnumParticleTypes particleType, boolean p_175682_2_, double p_175682_3_, double p_175682_5_, double p_175682_7_, double p_175682_9_, double p_175682_11_, double p_175682_13_, int ... p_175682_15_) { this.spawnParticle(particleType.getParticleID(), particleType.func_179344_e() | p_175682_2_, p_175682_3_, p_175682_5_, p_175682_7_, p_175682_9_, p_175682_11_, p_175682_13_, p_175682_15_); } The second parameter is a boolean, if you set it to true, it should spawn the effect regardless of how far away the player is. (From RenderGlobal.spawnEntityFX(): private EntityFX spawnEntityFX(int p_174974_1_, boolean p_174974_2_, double p_174974_3_, double p_174974_5_, double p_174974_7_, double p_174974_9_, double p_174974_11_, double p_174974_13_, int ... p_174974_15_) { //.... if (p_174974_2_) { // if force render return this.mc.effectRenderer.spawnEffectParticle(p_174974_1_, p_174974_3_, p_174974_5_, p_174974_7_, p_174974_9_, p_174974_11_, p_174974_13_, p_174974_15_); } else { // check distance d6 = delta X, d7 = delta y, d8 = delta z -> if distance is more than 16 (sq distance > 16*16 = 256), don't render double d9 = 16.0D; return d6 * d6 + d7 * d7 + d8 * d8 > 256.0D ? null : (k > 1 ? null : this.mc.effectRenderer.spawnEffectParticle(p_174974_1_, p_174974_3_, p_174974_5_, p_174974_7_, p_174974_9_, p_174974_11_, p_174974_13_, p_174974_15_)); } -TGG -
Hi Well it sure looks like a reobfuscation problem to me... your mod should use "field_151575_d" not "wood". Just to confirm - you used gradlew build, and then you used the jar you found in build\libs? If so - I don't have any idea, sorry! Your code looks perfectly normal to me. You could try a fresh install of forge, it might perhaps help. -TGG
-
[1.6.4][SOLVED] My Custom Grass Textures
TheGreyGhost replied to jkorn2324swagg's topic in Modder Support
Hi what are the symptoms? eg compiler error messages, what do you see vs what you expect to see, any related error messages in the console? -TGG -
[1.8] Is it possible to "Mix" textures dynamicly at runtime?
TheGreyGhost replied to techstack's topic in Modder Support
Hi Just use a texture pack? Alternatively, it's probably possible for you to gain access to the block texture sheet and overwrite it with the new texture (TextureStitchEvent.Post, perhaps?) Never tried it myself. -TGG -
Hi Is this the name of your chest? If so, the problem appears to be that you haven't registered the models properly. [19:30:40] [Client thread/WARN]: Unable to load variant: facing=east from primevalforest:lockedChest#facing=east Show your block code and registration code? You could try looking this example project for clues on how to properly register a block with variants https://github.com/TheGreyGhost/MinecraftByExample - see example 3 in particular This link is very helpful on learning how to use the debugger, you'll wonder how you ever managed with out it... http://www.vogella.com/tutorials/EclipseDebugging/article.html -TGG
-
Hi You might find this example project useful to see how to name your textures, where to place them, and how to register them properly. https://github.com/TheGreyGhost/MinecraftByExample See example 1. -TGG
-
[1.8][Solved] Block Texture/Render Bug
TheGreyGhost replied to Powerman913717's topic in Modder Support
Hi Your pictures didn't work Perhaps post on imgur and add the link? Also pls post code? -TGG -
[1.6.4][SOLVED] My Custom Grass Textures
TheGreyGhost replied to jkorn2324swagg's topic in Modder Support
It might help understand why registerIcons won't work if you add @Override before it @Override public void registerIcons(IconRegister par1IconRegister) Adding @Override for methods you expect to inherit from the base class is a really good way for the compiler to tell you "er, that isn't going to do what you think it will do" I forget offhand what the method you're looking for is actually called, you will for sure find it if you have a look at BlockGrass. Most of the time, I find that's the fastest way to see how to do something, i.e. think of a vanilla item or block or command that does something similar to what I want, and then look at that vanilla code for clues. -TGG -
minecraft 1.8 block (pillar like blocks)
TheGreyGhost replied to ShadowBeastGod's topic in Modder Support
Hi You might find this link useful, it talks about the block model system in quite a bit of detail; see the four topics under "Blocks" http://greyminecraftcoder.blogspot.com.au/p/list-of-topics.html There is also an example project that you can download and compile to see how it works. Example 1 does exactly what you need. https://github.com/TheGreyGhost/MinecraftByExample -TGG -
Hi If you don't want some of the EntityArrow behaviour and you need some of the other methods such as getGravityVelocity and onImpact, then override onUpdate and provide only the behaviour you want? Alternatively perhaps something like EntitySnowball might provide some clues - you could try deriving from that instead of EntityArrow. It really depends on your specific requirements for your projectile... -TGG