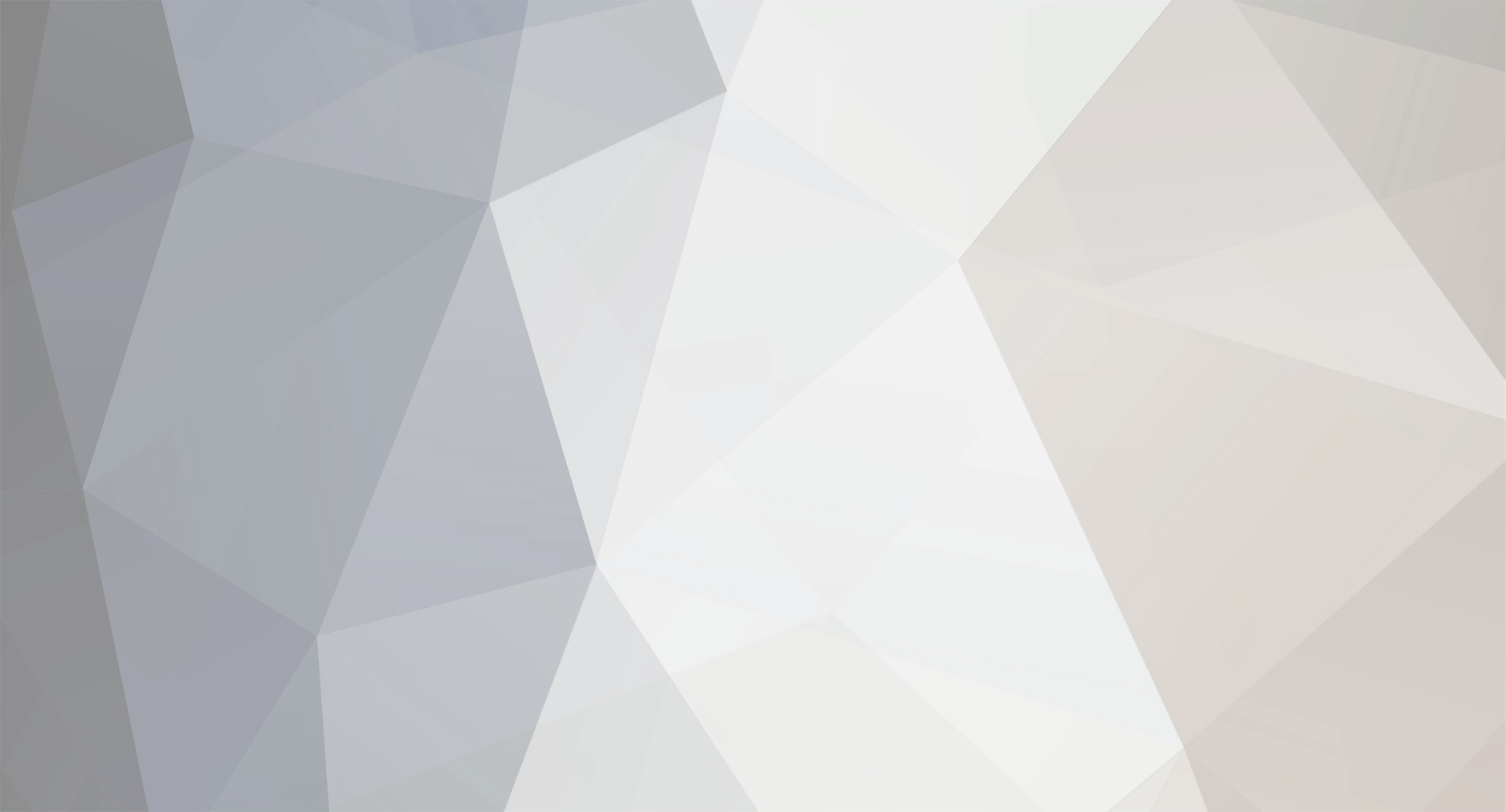
darthvader45
Members-
Posts
121 -
Joined
-
Last visited
Everything posted by darthvader45
-
You have to check for it. If you want to e.g. only drop things for mules, check first that the entity in the event is a horse and then check that it is also a mule. This is a forum, not a chat room. I cannot seem to get the type of horse needed. How would I call the variable that determines if the horse is a regular horse, a mule, or a donkey?
-
How would this deal with mobs that use multiple variations, such as horses, which have the variations donkey and mule(both stuck in the EntityHorse class as values of the variable i)? Edit: Excuse me, Jabelar, you still there?
-
I'm working for a team creating the Realistic Animal Products and Drops Mod (link: http://www.minecraftforum.net/forums/mapping-and-modding/minecraft-mods/wip-mods/2389704-realistic-animal-products-and-drops-mod) One item is hide, which has different colors depending on the animal it drops from (pig, sheep, cow, horse, donkey, or mule), and another is pelt, which drops from ocelots, cats, and wolves. How do I access the different mobs to determine the type of hide dropped? Edit: Solved by searching the net for adding Wither skelly drops. Turns out the solution shown there works for EntityHorse as well. I definitely learned something new today. Note to self: Find a similar problem and see if solution works with current issue.
-
I'll double-check the number of sub-types it has and make sure it's only 3 everywhere it's mentioned. Whenever I make a mod, I tend to register all my blocks as having an ItemBlock if they are supposed to. Should I register my ItemBlock in my ModItems.java file? Edit: Changed the ItemBlock to only have 3 variations, and I don't get any ArrayIndexOutOfBounds exceptions, yet I still don't see the other variations at all. I did register it as having an ItemBlock, just like your example showed.
-
Well, the fact I don't have metadata variants of my blocks in-game(which is what I want) is the problem I'm having currently. I fixed the original registration problem.
-
I don't have any other blocks registering(in other words, only one variation of smoky quartz). I'd like it to be like the vanilla quartz and have chiseled and pillar variants. The stair and slab I'll handle afterwards.
-
Ok, here's what I have for my blocks(made sure to call the init() method from ModBlocks in my main class): BlockSmokyQuartz.java: package net.lastdragonborn.smokyquartz.blocks; import java.util.List; import net.minecraft.block.Block; import net.minecraft.block.material.Material; import net.minecraft.creativetab.CreativeTabs; import net.minecraft.item.ItemStack; import cpw.mods.fml.relauncher.Side; import cpw.mods.fml.relauncher.SideOnly; public class BlockSmokyQuartz extends Block { public BlockSmokyQuartz (Material material) { super(material); this.setUnlocalizedName("smokyQuartz"); setCreativeTab(CreativeTabs.tabBlock); setStepSound(Block.soundTypeStone); } private String setUnlocalizedName(String string) { // TODO Auto-generated method stub return "smokyQuartz"; } @Override public int damageDropped (int metadata) { return metadata; } @SideOnly(Side.CLIENT) public void getSubBlocks(int unknown, CreativeTabs tab, List subItems) { for (int ix = 0; ix < 16; ix++) { subItems.add(new ItemStack(this, 1, ix)); } } } ItemBlockSmokyQuartz.java: package net.lastdragonborn.smokyquartz.items; import net.minecraft.block.Block; import net.minecraft.item.ItemBlock; import net.minecraft.item.ItemStack; public class ItemBlockSmokyQuartz extends ItemBlock { private final static String[] subNames = { "default", "chiseled", "lines" }; public ItemBlockSmokyQuartz(Block id) { super(id); setHasSubtypes(true); } @Override public int getMetadata (int damageValue) { return damageValue; } @Override public String getUnlocalizedName(ItemStack itemstack) { return getUnlocalizedName() + "." + subNames[itemstack.getItemDamage()]; } }
-
Alrighty, then. Here's what I have so far: BlockSmokyQuartz.java: package net.lastdragonborn.smokyquartz.blocks; import java.util.List; import net.lastdragonborn.smokyquartz.proxy.CommonProxy; import net.minecraft.block.Block; import net.minecraft.block.material.Material; import net.minecraft.client.renderer.texture.IIconRegister; import net.minecraft.creativetab.CreativeTabs; import net.minecraft.item.Item; import net.minecraft.item.ItemStack; import net.minecraft.util.IIcon; import cpw.mods.fml.relauncher.Side; import cpw.mods.fml.relauncher.SideOnly; public class BlockSmokyQuartz extends Block { @SideOnly(Side.CLIENT) private IIcon[] texture; public BlockSmokyQuartz (Material material) { super(material); setCreativeTab(CreativeTabs.tabBlock); } @Override @SideOnly(Side.CLIENT) public void registerBlockIcons(IIconRegister par1IconRegister){ texture = new IIcon[16]; for(int i = 0; i < 16; i++) texture[i] = par1IconRegister.registerIcon("sokobanMod:BlockConcreteLamp" + i); } @Override @SideOnly(Side.CLIENT) public void getSubBlocks(Item par1, CreativeTabs par2CreativeTabs, List par3List) { for(int var4 = 0; var4 < 16; ++var4) { par3List.add(new ItemStack(ModBlocks.smokyQuartz, 1, var4)); } } @Override @SideOnly(Side.CLIENT) public IIcon getIcon(int side, int meta){ return texture[meta]; } @Override public int damageDropped(int meta){ return meta; } } ItemBlockSmokyQuartz.java: package net.lastdragonborn.smokyquartz.items; import net.minecraft.block.Block; import net.minecraft.item.ItemBlock; import net.minecraft.item.ItemStack; public class ItemBlockSmokyQuartz extends ItemBlock { private String[] names = {"Block of", "Column", "Chiseled"}; public ItemBlockSmokyQuartz(Block block1, Block block2) { super(block1); setHasSubtypes(true); } @Override public String getItemStackDisplayName(ItemStack is){ return names[is.getItemDamage()] + "Smoky Quartz"; } @Override public int getMetadata(int meta){ return meta; } } Now my block isn't getting registered. What could be the problem?
-
Ok, I'm creating an ItemBlock file. Should it extend ItemBlockWithMetadata?
-
Okay, I've created a custom quartz block and have begun creation of a custom quartz slab and item. However, I have not been able to give the blocks a unique unlocalized name (i.e. they all have the name tile.smokyquartz.name) and also cannot seem to get them to be metadata. BlockSmokyQuartz.java: package net.lastdragonborn.smokyquartz.blocks; import java.util.List; import cpw.mods.fml.relauncher.Side; import cpw.mods.fml.relauncher.SideOnly; import net.lastdragonborn.smokyquartz.SmokyQuartz; import net.minecraft.block.Block; import net.minecraft.block.material.Material; import net.minecraft.client.renderer.texture.IIconRegister; import net.minecraft.creativetab.CreativeTabs; import net.minecraft.item.Item; import net.minecraft.item.ItemStack; import net.minecraft.util.IIcon; import net.minecraft.world.World; public class BlockSmokyQuartz extends Block { public static final String[] field_150191_a = new String[] {"default", "chiseled", "lines"}; private static final String[] field_150189_b = new String[] {"side", "chiseled", "lines", null, null}; @SideOnly(Side.CLIENT) private IIcon[] field_150192_M; @SideOnly(Side.CLIENT) private IIcon field_150193_N; @SideOnly(Side.CLIENT) private IIcon field_150194_O; @SideOnly(Side.CLIENT) private IIcon field_150190_P; @SideOnly(Side.CLIENT) private IIcon field_150188_Q; private static final String __OBFID = "CL_00000292"; public BlockSmokyQuartz(Material material) { super(material = Material.rock); setCreativeTab(CreativeTabs.tabBlock); } @SideOnly(Side.CLIENT) public IIcon getIcon(int p_149691_1_, int p_149691_2_) { if (p_149691_2_ != 2 && p_149691_2_ != 3 && p_149691_2_ != 4) { if (p_149691_1_ != 1 && (p_149691_1_ != 0 || p_149691_2_ != 1)) { if (p_149691_1_ == 0) { return this.field_150188_Q; } else { if (p_149691_2_ < 0 || p_149691_2_ >= this.field_150192_M.length) { p_149691_2_ = 0; } return this.field_150192_M[p_149691_2_]; } } else { return p_149691_2_ == 1 ? this.field_150193_N : this.field_150190_P; } } else { return p_149691_2_ == 2 && (p_149691_1_ == 1 || p_149691_1_ == 0) ? this.field_150194_O : (p_149691_2_ == 3 && (p_149691_1_ == 5 || p_149691_1_ == 4) ? this.field_150194_O : (p_149691_2_ == 4 && (p_149691_1_ == 2 || p_149691_1_ == 3) ? this.field_150194_O : this.field_150192_M[p_149691_2_])); } } /** * Called when a block is placed using its ItemBlock. Args: World, X, Y, Z, side, hitX, hitY, hitZ, block metadata */ public int onBlockPlaced(World p_149660_1_, int p_149660_2_, int p_149660_3_, int p_149660_4_, int p_149660_5_, float p_149660_6_, float p_149660_7_, float p_149660_8_, int p_149660_9_) { if (p_149660_9_ == 2) { switch (p_149660_5_) { case 0: case 1: p_149660_9_ = 2; break; case 2: case 3: p_149660_9_ = 4; break; case 4: case 5: p_149660_9_ = 3; } } return p_149660_9_; } /** * Determines the damage on the item the block drops. Used in cloth and wood. */ public int damageDropped(int p_149692_1_) { return p_149692_1_ != 3 && p_149692_1_ != 4 ? p_149692_1_ : 2; } /** * Returns an item stack containing a single instance of the current block type. 'i' is the block's subtype/damage * and is ignored for blocks which do not support subtypes. Blocks which cannot be harvested should return null. */ protected ItemStack createStackedBlock(int p_149644_1_) { return p_149644_1_ != 3 && p_149644_1_ != 4 ? super.createStackedBlock(p_149644_1_) : new ItemStack(Item.getItemFromBlock(this), 1, 2); } /** * The type of render function that is called for this block */ public int getRenderType() { return 39; } /** * returns a list of blocks with the same ID, but different meta (eg: wood returns 4 blocks) */ @SideOnly(Side.CLIENT) public void getSubBlocks(Item p_149666_1_, CreativeTabs p_149666_2_, List p_149666_3_) { p_149666_3_.add(new ItemStack(p_149666_1_, 1, 0)); p_149666_3_.add(new ItemStack(p_149666_1_, 1, 1)); p_149666_3_.add(new ItemStack(p_149666_1_, 1, 2)); } @SideOnly(Side.CLIENT) public void registerBlockIcons(IIconRegister p_149651_1_) { this.field_150192_M = new IIcon[field_150189_b.length]; for (int i = 0; i < this.field_150192_M.length; ++i) { if (field_150189_b[i] == null) { this.field_150192_M[i] = this.field_150192_M[i - 1]; } else { this.field_150192_M[i] = p_149651_1_.registerIcon(SmokyQuartz.MODID + ":" + "smoky_quartz_block" + "_" + field_150189_b[i]); } } this.field_150190_P = p_149651_1_.registerIcon(SmokyQuartz.MODID + ":" + "smoky_quartz_block" + "_" + "top"); this.field_150193_N = p_149651_1_.registerIcon(SmokyQuartz.MODID + ":" + "smoky_quartz_block" + "_" + "chiseled_top"); this.field_150194_O = p_149651_1_.registerIcon(SmokyQuartz.MODID + ":" + "smoky_quartz_block" + "_" + "lines_top"); this.field_150188_Q = p_149651_1_.registerIcon(SmokyQuartz.MODID + ":" + "smoky_quartz_block" + "_" + "bottom"); } }
-
When Syxes and I had the mod set up in the dev environment, the textures worked and had the correct names matching the code. Yet, whenever the mod is built, the names revert back to older names that don't work. Why is this?
-
How to setup a config file in 1.7.10?
darthvader45 replied to darthvader45's topic in Modder Support
Ok, so now I'm having trouble with the WeatherTickHandler and PlayersSleepingHandler. Basically, the weather handler makes all the biomes colder, meaning snow in every biome. The sleeping handler sets it so that when the player sleeps and has stockings placed, they get filled with a present(also, if a christmas tree is built(aka the base has been placed down), presents are underneath.) -
I decided since the owner of ChristmasCraft(aka newthead on the Minecraft Forums) has been away from his mod for more than a year, I would update his mod to 1.7.10 from 1.6.4 and give credit as well as posting a link back to his thread. However, it seems that the Configuration class no longer has a method for accepting blocks/items(getBlock and getItem, respectively). How can I create customizable ids in a config file in 1.7?
-
Ok, so I have a new weapon called ItemXBow. When it's right-clicked, I had it spawn a new projectile called EntityXBowBolt. However, it continues to render as a small cube, even though I set its render class to be RenderXBowBolt and used a custom model(ModelXBowBolt). EntityXBowBolt: ModelXBowBolt.java: RenderXBowBolt.java:
-
I saw a method mentioned in a similar topic to mine mentioning EntityRenderer.updateFogColor. Do I create a custom renderer class that extends it and override that method?
-
Mod textures not working after build with Gradle
darthvader45 replied to darthvader45's topic in ForgeGradle
Yeah, just figured that out. D'oh! *facepalm* I sent a message to my modmaking partner, Syxes. Might want to help him with coding, as he's just getting into the nitty gritty of forge modding. -
Ok, so my textures work perfectly when I test it through Eclipse. However, when I build the jar and then subsequently test it, the textures for some blocks and items just didn't work. The code referenced the textures correctly, yet the textures didn't work. I'll check through the console to see if there's any errors or missing references.
-
I have been working with Forge(successfully) for several weeks. Today it just wouldn't build a jar or even setup the decomp Workspace. It keeps giving me an error saying that my JAVA_HOME variable(which didn't exist before and Forge setup perfectly w/o previously) was invalid. Java jdk path: C:/Program Files/jdk1.7_72/ Why is this happening? Changing it to the above path doesn't work. Do I really need to do a factory reset of my computer or a restart? I really don't want to get that drastic.
-
Running Forge 1.7.10-10.13.2.1230 in Eclipse on Windows 7 64 bit. Immediately fails to even launch Minecraft with this error log in the console. I can run 1217 without any problems, though. Edit: Why has nobody responded to this? I desperately need help here. Without a fix, I cannot develop mods for this version of Forge. Exception in thread "main" [17:26:58] [main/ERROR]: Unable to launch java.lang.reflect.InvocationTargetException at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.7.0_67] at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) ~[?:1.7.0_67] at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) ~[?:1.7.0_67] at java.lang.reflect.Method.invoke(Unknown Source) ~[?:1.7.0_67] at net.minecraft.launchwrapper.Launch.launch(Launch.java:135) [launchwrapper-1.11.jar:?] at net.minecraft.launchwrapper.Launch.main(Launch.java:28) [launchwrapper-1.11.jar:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.7.0_67] at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) ~[?:1.7.0_67] at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) ~[?:1.7.0_67] at java.lang.reflect.Method.invoke(Unknown Source) ~[?:1.7.0_67] at GradleStart.bounce(GradleStart.java:107) [start/:?] at GradleStart.startClient(GradleStart.java:100) [start/:?] at GradleStart.main(GradleStart.java:55) [start/:?] Caused by: java.lang.UnsatisfiedLinkError: no lwjgl in java.library.path at java.lang.ClassLoader.loadLibrary(Unknown Source) ~[?:1.7.0_67] at java.lang.Runtime.loadLibrary0(Unknown Source) ~[?:1.7.0_67] at java.lang.System.loadLibrary(Unknown Source) ~[?:1.7.0_67] at org.lwjgl.Sys$1.run(Sys.java:73) ~[lwjgl-2.9.1.jar:?] at java.security.AccessController.doPrivileged(Native Method) ~[?:1.7.0_67] at org.lwjgl.Sys.doLoadLibrary(Sys.java:66) ~[lwjgl-2.9.1.jar:?] at org.lwjgl.Sys.loadLibrary(Sys.java:95) ~[lwjgl-2.9.1.jar:?] at org.lwjgl.Sys.<clinit>(Sys.java:112) ~[lwjgl-2.9.1.jar:?] at net.minecraft.client.Minecraft.getSystemTime(Minecraft.java:2660) ~[Minecraft.class:?] at net.minecraft.client.main.Main.main(SourceFile:72) ~[Main.class:?] ... 13 more [17:26:58] [main/INFO]: [java.lang.Throwable$WrappedPrintStream:println:-1]: java.lang.ArrayIndexOutOfBoundsException: 0 [17:26:58] [main/INFO]: [java.lang.Throwable$WrappedPrintStream:println:-1]: at GradleStart.main(GradleStart.java:59)
-
I keep getting this error no matter what I do. My ItemMudBucket.java(my custom bucket, meant to place my custom fluid on right click): package com.caske2000.carnivores.items; import java.util.List; import net.minecraft.block.Block; import net.minecraft.creativetab.CreativeTabs; import net.minecraft.entity.player.EntityPlayer; import net.minecraft.init.Blocks; import net.minecraft.init.Items; import net.minecraft.item.ItemBucket; import net.minecraft.item.ItemStack; import net.minecraft.world.World; import net.minecraftforge.fluids.Fluid; import net.minecraftforge.fluids.FluidContainerRegistry; import org.apache.commons.lang3.StringUtils; import com.caske2000.carnivores.handler.BucketHandler; import com.caske2000.carnivores.reference.Reference; import cpw.mods.fml.common.registry.GameRegistry; import cpw.mods.fml.relauncher.Side; import cpw.mods.fml.relauncher.SideOnly; public class ItemMudBucket extends ItemBucket{ public static ItemMudBucket create(Fluid fluid) { ItemMudBucket b = new ItemMudBucket(fluid.getBlock() != null ? fluid.getBlock() : Blocks.air, fluid.getName()); b.init(); FluidContainerRegistry.registerFluidContainer(fluid, new ItemStack(b), new ItemStack(Items.bucket)); BucketHandler.INSTANCE.registerFluid(fluid.getBlock(), b); return b; } private String fluidName = "mud"; protected ItemMudBucket(Block block, String fluidName) { super(block); this.fluidName = fluidName; setCreativeTab(CreativeTabs.tabMisc); setContainerItem(Items.bucket); setUnlocalizedName("bucket_mud"); setTextureName(Reference.MODID + ":" + "bucket_mud"); } protected void init() { GameRegistry.registerItem(this, "bucket" + StringUtils.capitalize(fluidName)); } } Error report: java.lang.NullPointerException at net.minecraft.world.chunk.storage.ExtendedBlockStorage.func_150818_a(ExtendedBlockStorage.java:96) ~[ExtendedBlockStorage.class:?] at net.minecraft.world.chunk.Chunk.func_150807_a(Chunk.java:667) ~[Chunk.class:?] at net.minecraft.world.World.setBlock(World.java:515) ~[World.class:?] at net.minecraft.item.ItemBucket.tryPlaceContainedLiquid(ItemBucket.java:210) ~[itemBucket.class:?] at net.minecraft.item.ItemBucket.onItemRightClick(ItemBucket.java:142) ~[itemBucket.class:?] at net.minecraft.item.ItemStack.useItemRightClick(ItemStack.java:167) ~[itemStack.class:?] at net.minecraft.client.multiplayer.PlayerControllerMP.sendUseItem(PlayerControllerMP.java:434) ~[PlayerControllerMP.class:?] at net.minecraft.client.Minecraft.func_147121_ag(Minecraft.java:1557) ~[Minecraft.class:?] at net.minecraft.client.Minecraft.runTick(Minecraft.java:2044) ~[Minecraft.class:?] at net.minecraft.client.Minecraft.runGameLoop(Minecraft.java:1039) ~[Minecraft.class:?] at net.minecraft.client.Minecraft.run(Minecraft.java:961) [Minecraft.class:?] at net.minecraft.client.main.Main.main(Main.java:164) [Main.class:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.7.0_67] at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) ~[?:1.7.0_67] at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) ~[?:1.7.0_67] at java.lang.reflect.Method.invoke(Unknown Source) ~[?:1.7.0_67] at net.minecraft.launchwrapper.Launch.launch(Launch.java:135) [launchwrapper-1.11.jar:?] at net.minecraft.launchwrapper.Launch.main(Launch.java:28) [launchwrapper-1.11.jar:?] at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method) ~[?:1.7.0_67] at sun.reflect.NativeMethodAccessorImpl.invoke(Unknown Source) ~[?:1.7.0_67] at sun.reflect.DelegatingMethodAccessorImpl.invoke(Unknown Source) ~[?:1.7.0_67] at java.lang.reflect.Method.invoke(Unknown Source) ~[?:1.7.0_67] at GradleStart.bounce(GradleStart.java:107) [start/:?] at GradleStart.startClient(GradleStart.java:100) [start/:?] at GradleStart.main(GradleStart.java:55) [start/:?]
-
Alright, I looked at a similar question and used Mecblader's sample code. This got it to work facing north. Updated code is in OP. Edit: Did a bit more fiddling, and got it to rotate the model properly. However, the collision boxes are only the same when looking at the front of the block. How do I fix that?
-
Okay, all I want is to make my AquariumGlass block(it's like a glass pane, except it renders on the edge of the block its sitting on) face the player when placed in any of the four cardinal directions. I've looked at several similar threads and tested the code, and still have no luck. I've even tried adding and modifying the Furnace rotation code, but have not been able to get it to work. BlockAquariumGlass: package com.aquariumglass.blocks; import net.minecraft.block.Block; import net.minecraft.block.BlockContainer; import net.minecraft.block.material.Material; import net.minecraft.entity.EntityLivingBase; import net.minecraft.item.ItemStack; import net.minecraft.tileentity.TileEntity; import net.minecraft.util.MathHelper; import net.minecraft.world.World; import com.aquariumglass.AquariumGlass; import com.aquariumglass.tileentity.TileEntityAquariumGlass; public class BlockAquariumGlass extends BlockContainer { public BlockAquariumGlass(Material material) { super(material); material = Material.glass; this.setHardness(2.0F); this.setResistance(5.0F); this.setCreativeTab(AquariumGlass.aquariumTab); } public int getRenderType(){ return -1; } public boolean isOpaqueCube(){ return false; } public boolean renderAsNormalBlock(){ return false; } @Override public TileEntity createNewTileEntity(World world, int int1) { // TODO Auto-generated method stub return new TileEntityAquariumGlass(); } public void onBlockAdded(World world, int x, int y, int z) { super.onBlockAdded(world, x, y, z); this.setDefaultDirection(world, x, y, z); } public void setDefaultDirection(World world, int x, int y, int z){ if(!world.isRemote){ Block b1 = world.getBlock(x, y, z - 1); Block b2 = world.getBlock(x, y, z + 1); Block b3 = world.getBlock(x - 1, y, z); Block b4 = world.getBlock(x + 1, y, z); byte b0 = 3; if (b1.func_149730_j() && !b2.func_149730_j()) { b0 = 3; } if (b2.func_149730_j() && !b1.func_149730_j()) { b0 = 2; } if (b3.func_149730_j() && !b4.func_149730_j()) { b0 = 5; } if (b4.func_149730_j() && !b3.func_149730_j()) { b0 = 4; } world.setBlockMetadataWithNotify(x, y, z, b0, 2); } } public void onBlockPlacedBy(World world, int x, int y, int z, EntityLivingBase entityplayer, ItemStack itemstack){ int l = MathHelper.floor_double((double)(entityplayer.rotationYaw * 4.0F / 360F) + 0.5D) & 3; if(l == 0){ world.setBlockMetadataWithNotify(x, y, z, 2, 2); } if(l == 1){ world.setBlockMetadataWithNotify(x, y, z, 5, 2); } if(l == 2){ world.setBlockMetadataWithNotify(x, y, z, 3, 2); } if(l == 3){ world.setBlockMetadataWithNotify(x, y, z, 4, 2); } } } RenderAquariumGlass: package com.aquariumglass.renderer; import net.minecraft.client.renderer.tileentity.TileEntitySpecialRenderer; import net.minecraft.tileentity.TileEntity; import net.minecraft.util.ResourceLocation; import net.minecraft.world.World; import org.lwjgl.opengl.GL11; import com.aquariumglass.AquariumGlass; import com.aquariumglass.blocks.BlockAquariumGlass; import com.aquariumglass.models.ModelAquariumGlass; public class RenderAquariumGlass extends TileEntitySpecialRenderer { private static final ResourceLocation texture = new ResourceLocation(AquariumGlass.modid + ":" + "textures/blocks/aquarGlass.png"); private ModelAquariumGlass model; public RenderAquariumGlass(){ this.model = new ModelAquariumGlass(); } @Override public void renderTileEntityAt(TileEntity tileentity, double x, double y, double z, float f) { GL11.glPushMatrix(); GL11.glTranslatef((float) x + 0.5F, (float) y + 1.5F, (float) z + 0.5F); GL11.glRotatef(180, 0F, 0F, 1F); int i = tileentity.getBlockMetadata(); short short1 = 0; if (i == 2) { short1 = 360; AquariumGlass.aquariumGlass.setBlockBounds(0F, 0F, 0F, 1F, 1F, 0.125F); } if (i == 3) { short1 = 180; AquariumGlass.aquariumGlass.setBlockBounds(0F, 0F, .875F, 1F, 1F, 1F); } if (i == 4) { short1 = -90; AquariumGlass.aquariumGlass.setBlockBounds(0F, 0F, 0F, .125F, 1F, 1F); } if (i == 5) { short1 = 90; AquariumGlass.aquariumGlass.setBlockBounds(.875F, 0F , 0F, 1F, 1F, 1F); } GL11.glRotatef((float)short1, 0.0F, 1.0F, 0.0F); this.bindTexture(texture); GL11.glPushMatrix(); this.model.renderModel(0.0625F); GL11.glPopMatrix(); GL11.glPopMatrix(); } }
-
Mobs dropping custom item with chance based on config file
darthvader45 replied to darthvader45's topic in Modder Support
I'll test. Thanks for the example code. Edit: Ok, now MrCrayfish sets it so that if the rarity is set to 0 in the config file, they always drop, but if it's not equal to zero, then the chance of coins dropping is one out of whatever the rarity is set to(i.e. rarity for copperToken is 8, it will be 1/8 chance of copperToken dropping). -
Mobs dropping custom item with chance based on config file
darthvader45 replied to darthvader45's topic in Modder Support
Where in the file would I add these snippets of code? -
Mobs dropping custom item with chance based on config file
darthvader45 replied to darthvader45's topic in Modder Support
I'm running it in debug and still cannot see any sign of what's going wrong in the console.