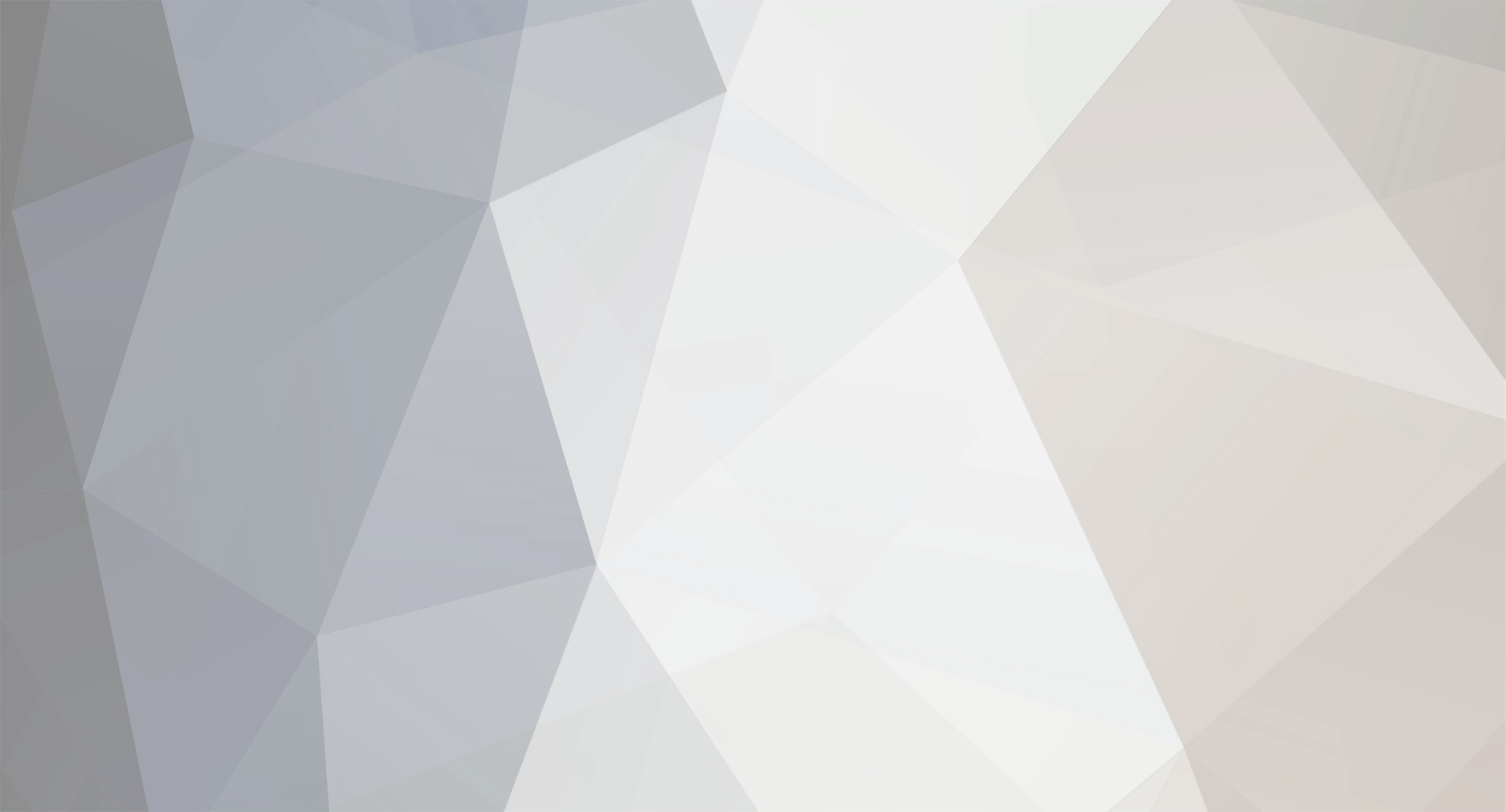
scientistknight1
Members-
Posts
37 -
Joined
-
Last visited
-
Days Won
3
Everything posted by scientistknight1
-
DataGen: Help with multiple items for a recipe.
scientistknight1 replied to DroidCrafter23's topic in Modder Support
Just add another .requires after the first one. You can even specify how many should be needed. In your case, that would look like: ShapelessRecipeBuilder.shapeless(RecipeCategory.MISC, ModItems.LEMON_JUICE.get(), 4) .requires(ModItems.LEMON.get()) .requires(Items.GLASS_BOTTLE, 4) .save(pWriter); -
All I know is what I've told you; I can't help more without inspecting the entirety of your code, and I think you'd probably learn the most from working this problem out yourself at this point. If you're still confused, try finding some other tutorials for modding; if you don't understand what the code is doing, try looking for Java tutorials. I hope this helps.
-
To add the item to the creative mode tab, you'll need to reference the item you registered in ModItems ( ModItems.LEMON_JUICE.get() ). The ModDrinks class is only ever used when creating your item.
-
To ensure that the entity drops resources in the usual way, try using the .kill() method instead of .setHealth(int); that should work better. Try making your class extend SwordItem to use all the standard sword infrastructure. Just make sure to add super calls at the top of all methods you override if you want to keep the existing behavior as well.
-
It looks like you're only setting the health if the thing you are hitting is a player.
-
It sounds like you accidentally have two items that are both named "orange". Ensure that you give items unique names in the string when you register them. That's one of the more annoying errors to track down if you don't know what's causing it, though.
-
If I remember correctly, Jump Boost uses an attribute modifier. It doesn't have its own class.
-
Item Equip animation plays when updating nbt
scientistknight1 replied to TheTrueSCP's topic in Modder Support
The method to override is shouldCauseReequipAnimation. -
Try using the build task to compile it into a runnable mod, if that's what you want. If you just want to copy the entire mod into a zipped folder, you can do that in your computer's file explorer. If you're on Windows, just go to the directory you're using and zip up the whole folder. Name it whatever you want.
-
I'm trying to make a campfire, and idk why, Minecraft's failing
scientistknight1 replied to Red4X's topic in Modder Support
Glad I could help. -
I'm trying to make a campfire, and idk why, Minecraft's failing
scientistknight1 replied to Red4X's topic in Modder Support
Ah; you can't just copy the properties, you need to use the same block class as the soul campfire. -
Unfortunately, you probably won't get any help with your problem here, since this is the Forge forums. As it says in the FAQ that you presumably read before posting, "We only support Forge here. We cannot help you with Fabric, Spigot, etc..." Forge and Fabric are completely different and run by different people, so it's unlikely (to say the least) that anyone here will be willing or able to assist with a Fabric problem. I'd recommend joining the Fabric Discord Server that's linked on the Fabric website. They might be able to help you there. I'd also recommend notifying the developers of whatever mod you're using.
-
From what I've found, you can only manipulate the player's motion on the client side. It's extremely annoying. If you know how to use packets, you can use packets to transfer the information from the server. Take a look at my implementation on GitHub here if you want. You'd want something similar to the way I've done the PlayerAddVelocityPacket. Unfortunately, packets are rather complex; I'd recommend you watch a few tutorials on them before even trying to work with them. Feel free to use my code as a reference, but please give attribution if you plan to directly copy it.
-
I'm trying to make a campfire, and idk why, Minecraft's failing
scientistknight1 replied to Red4X's topic in Modder Support
Can you post your ModBlocks class where you've registered the block, as well as which version of Minecraft you are using? -
It looks like you're trying to pass the lemon juice item itself to the .food method in your first code snippet; that won't work. The parameter to the .food method needs to be a FoodProperties object, as the error says. The second way you're doing it there is much closer to how it should be. The problem there is that you're trying to access the LEMON_JUICE field of the vanilla FoodProperties class. This won't work because the vanilla food properties class does not have LEMON_JUICE information in it. You'll need to make your own ModFoods class, looking something like the vanilla Foods class. Here's an example: public class ModFoods { public static final FoodProperties LEMON_JUICE = (new FoodProperties.Builder()).nutrition(3).saturationMod(0.25F) .effect(() -> new MobEffectInstance(MobEffects.DAMAGE_RESISTANCE, 1500), 0.5f).build(); } Then, your completed item registration would be: public static final RegistryObject<Item> LEMON_JUICE = ITEMS.register("lemon_juice", () -> new Item(new Item.Properties().food(ModFoods.LEMON_JUICE))); Notice that I changed the FoodProperties to ModFoods, since that's the class where you'd be storing the food data.
-
[1.20.4] Packets: cannot create a SimpleChannel instance
scientistknight1 replied to LeeCrafts's topic in Modder Support
Here is a tutorial; this is what I used. 1.20 Minecraft Forge Modding Tutorial - Packets -
Corrected item registration code: (for the ModItems class) public static final RegistryObject<Item> LEMON_JUICE_BOTTLE = ITEMS.register("lemon_juice_bottle", () -> new HoneyBottleItem(new Item.Properties().stacksTo(1) .food((new FoodProperties.Builder()).nutrition(3).saturationMod(0.25F) .effect(() -> new MobEffectInstance(MobEffects.DAMAGE_RESISTANCE, 1500), 0.5f).build())));
-
Apologies for the late reply. You'll need to register the item in ModItems; if you're following those tutorials, that's the only place you should ever register items. Otherwise, the mod will fail to register them properly and you'll get all sorts of interesting errors. Looking back at the code snipped I posted, I think that actually has some errors. I'm adding a lemon juice bottle to my mod just to ensure that it works correctly, and I will reply when I have solved the problems.
-
I might have an idea why your original method was causing so much trouble. See this while loop? You're only incrementing the number of blocks you've corrupted if you find one that you can corrupt. What happens if you can't find any? The while loop will run forever (a long time). This could happen if, for instance, the feature generates inside a vein of blocks that aren't marked as STONE_ABERRANTABLE. There are two alternate strategies I'd recommend to fix this. First, you could simply increment numBlockCorrupted regardless of whether you've actually corrupted the block. This is the simplest and quickest way, and it should ensure that the loop runs no more than numBlocksToCorrupt times. Alternatively, you could add a "kill switch" that keeps track of how many times the loop runs, and then ends it after a certain limit of your choosing. That could look something like this: // Keeps track of how many blocks have been checked so far. int numBlocksChecked = 0; // Check up to twice as many blocks as you actually want to corrupt. // This is a good compromise between speed and actually getting the number of blocks // that you want to corrupt. int numBlocksToCheck = numBlocksToCorrupt * 2; // Modified the while loop condition to end after a certain number of blocks are checked. while (numBlocksCorrupted < numBlocksToCorrupt && numBlocksChecked < numBlocksToCheck) { // Generate a random position within the area, using the offset origin BlockPos randomPos = offsetOrigin.offset( ctx.random().nextInt(2 * areaSizeX + 1) - areaSizeX, // between -areaSize and areaSize ctx.random().nextInt(2 * areaSizeY + 1) - areaSizeY, ctx.random().nextInt(2 * areaSizeZ + 1) - areaSizeZ ); // If the block at the random position is in the IS_ORE_ABERRANTABLE tag, replace it if (world.getBlockState(randomPos).is(ModBlockTags.STONE_ABERRANTABLE)) { world.setBlock(randomPos, surroundingBlockState, 2); numBlocksCorrupted++; } // Increment the number of blocks that you've checked. numBlocksChecked++; } Let me know if you're still running into lag problems or are confused by my explanation.
-
I'm guessing that's so people can implement their own custom animated models? I really don't know though, since I've never had to use that at all. I just know that you can have more control over a block entity model than over a standard block model, as well as use animations. I'm glad I could help, though!
-
I am confused with this error message.
scientistknight1 replied to DroidCrafter23's topic in Modder Support
It sounds to me like you're trying to register an item or block in the wrong place. Check to make sure you're handling the registries in the right place. -
A glowing texture for a custom armor is a daunting task; you'll need to create a custom render layer for your armor, which is easier said than done, and also connect that layer to the player that should be wearing it. I really have no idea how to do that. I'd recommend looking into how other mods do custom armor, and working from there.