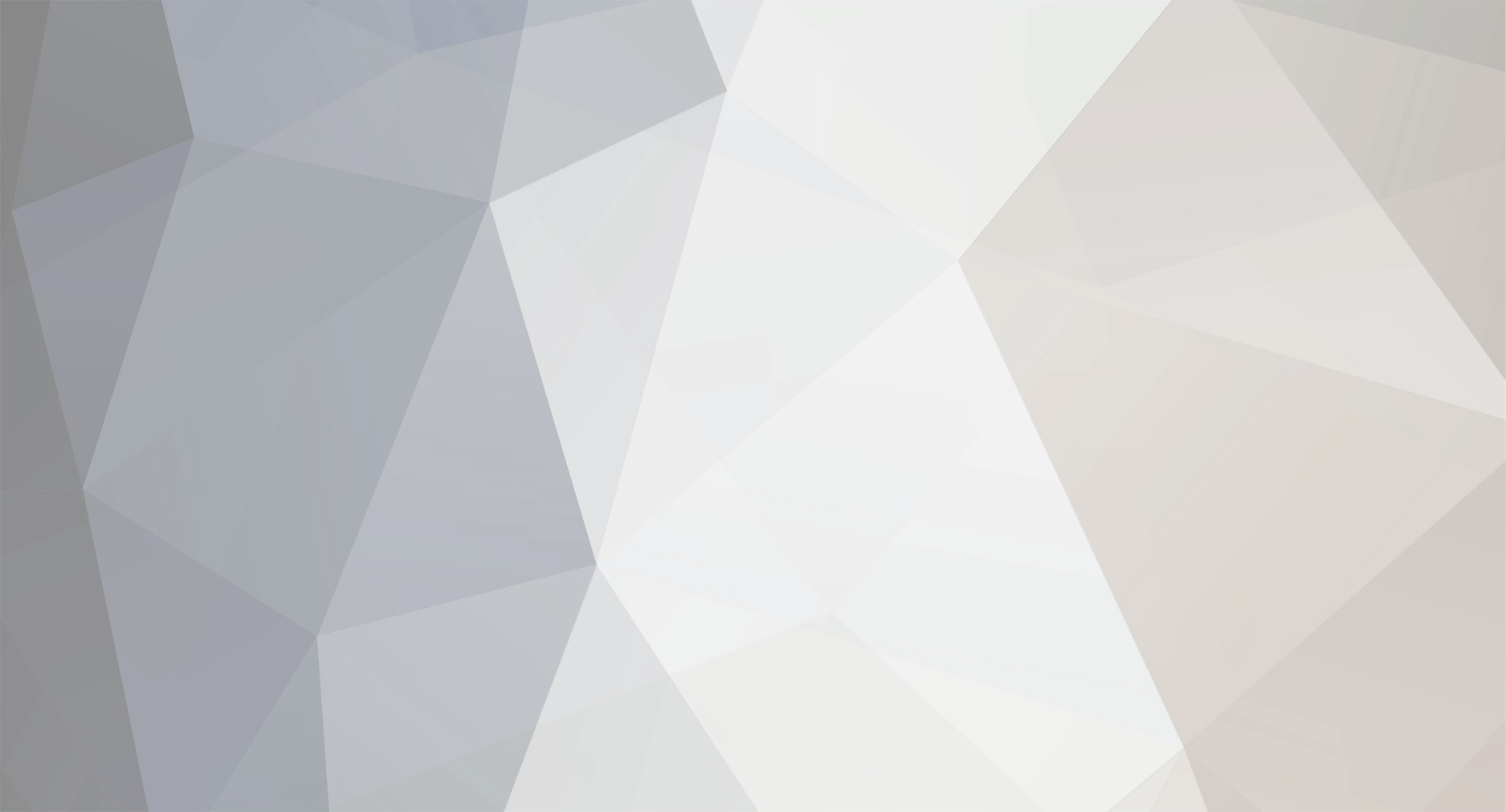
ILuvYouCompanionCube
Members-
Posts
112 -
Joined
-
Last visited
Everything posted by ILuvYouCompanionCube
-
I know very little about dragon eggs, so I'm not sure what you want. You need it to make it unmovable by pistons, blocking pistons from extending? edit: if that's all you want, why would you need to add a method to the class? Anyway, if all you need to do is make the block unmovable by pistons, just add this code somewhere in your mod (I'm thinking the PreInitializationEvent, but the InitializationEvent or some other may work as well) Block dragonEggBlock = Block.dragonEgg; try { Material dragonEggMaterial = dragonEggBlock.blockMaterial; Class materialClass = Material.class; Field mobilityFlagField = materialClass.getDeclaredField("mobilityFlag"); mobilityFlagField.setAccessible(true); mobilityFlagField.set(dragonEggMaterial, 2); mobilityFlagField.setAccessible(false); } catch (Exception e) { System.err.println("An Exception was thrown while making Dragon Eggs unmovable: " + e.getClass() + "\n[[" + e.getMessage() + "]]"); e.printStackTrace(); } And if any exception is thrown let me know. Note that if there's any other thing in the game that has the same Material as the DragonEggBlock (which is Material.dragonEgg), this other thing will also be unmovable. If that's not desirable, we need to rethink our strategy. If you need to make the block unbreakable (like bedrock) it will also be unmovable by pistons but it's a lot easier to do. Ignore all the code above, and just invoke this method: Block.dragonEgg.setBlockUnbreakable(); And finally, if you also need it to be undestructible by blasts, you also need this: //I'll set it's blast resistance to the same of bedrock's, which is an exageration, but it doesn't make any difference, as long as it's a big number Block.dragonEgg.setResistance(6000000.0F); Although it was not clear to me what exactly did you want, I hope I gave you the information you needed. edit2: there was an unecessary line on the code for changing the mobility flag. I spotted it and removed it. edit3: of course, any mods that expect dragon eggs to have their vanilla behaviour will be incompatible with yours. If thats a concern, you should subclass dragon egg and make your own version where you can change whatever you want, but leaving the original dragon eggs where they should be and behaving as they should.
-
You mean there is a good reason why I can't override static methods? (If that's it, I know there is one. It does't even make sense from a OOP point of view, and it would also break the virtual machine since static methods are invoked differently with a different OPcode). But if you mean there is a good reason why I can do this in java, please let me know: Integer aNumber; aNumber.parseInt("1000"); Calling a static method on an instance is confusing. People would assume aNumber has the value 1000 now, but it doesn't, The static method doesn't even know aNumber exists.
-
Open Vanilla GUI w/ item right click?
ILuvYouCompanionCube replied to GravityWolf's topic in Modder Support
yeah, I think showing the GUI is one thing. But actually making it work involves some more coding. Containers and inventories and etc. I wish we had an up-to-date tutorial on that subject. -
The icons are overriden with the ones I want on my class (which are the same, but slightly tinged golden), but as you can see in the code I posted, renderBlockHopperMetadata calls a static method of BlockHopper, which uses the static instance of BlockHopper owned by the Block class (Block.blockHopper). If I ovewrite the textures of BlockHopper, my Hopper will work, but the vanilla Hopper will look just like mine. I don't know what is a ISBRH. Could you explain that?
-
the method that renders the hopper is in another class called net.minecraft.client.renderer.RenderBlocks. And that same class has a method (named renderBlockHopperMetadata) which is called for rendering the hopper. That method in renderBlockHopperMetadata "asks" the hopper for it's icons, but the method it invokes is static (so I can't override it), and it invokes it like this: Icon icon1 = BlockHopper.getHopperIcon("hopper_inside"); getHopperIcon does the following: @SideOnly(Side.CLIENT) public static Icon getHopperIcon(String par0Str) { return par0Str.equals("hopper_outside") ? Block.hopperBlock.hopperIcon : (par0Str.equals("hopper_inside") ? Block.hopperBlock.hopperInsideIcon : null); } so my derived class is left out... And I know nothing of the other thing you said oO GL11 what
-
Open Vanilla GUI w/ item right click?
ILuvYouCompanionCube replied to GravityWolf's topic in Modder Support
navigating through some the minecraft classes I've found out what was wrong. you need to damage the ItemStack, so a method called tryUseItem will return true. This method is in a class called net.minecraft.item.ItemInWorldManager. If it returns false it is as if you failed on using the item. So, you have to set the item maxDamage (using the method setMaxDamage of the Item, maybe in the constructor), which is the maximum number of times you can use the item. If you don't set this, it will be zero, and tryUseItem will return false even earlier. The item will break eventually, but it's just a matter of setting a very very large number if you don't want it to break soon. (Like Integer.MAX_VALUE if you want it to last reeeally long) This is what you have to do: public *nameOfYourConstructor*(*parameter list of your constructor*) { super(*parameter list of superclass constructor*); *whatever else your constructor does* setMaxDamage(*whatever int you want*); } @Override public ItemStack onItemRightClick(ItemStack par1ItemStack, World world, EntityPlayer player) { if(world.isRemote) { player.displayGUIWorkbench((int)player.posX, (int)player.posY, (int)player.posZ); setDamage(par1ItemStack, par1ItemStack.getItemDamage() + 1); } return par1ItemStack; } -
Open Vanilla GUI w/ item right click?
ILuvYouCompanionCube replied to GravityWolf's topic in Modder Support
Are you using onItemUse or onItemRightClick ? use both just to be safe, if it works, undo one of them to figure which one worked -
I have a block that looks exactly like a hopper except for the textures. How do I make my hopper show the correct textures? I managed to make it show the _top and _outside textures using reflection (my textures are just like the ones from the original hopper, but with different colour), but from what I could understand, _inside texture is referenced only inside a method of the RenderBlocks class called renderBlockHopperMetadata, exactly at this line: Icon icon1 = BlockHopper.getHopperIcon("hopper_inside"); And that's my problem. That line is invoking a static method. For obvious reasons I can't override a static method (even though I think java should allow that since it's the only language I know where you can access a static member using an instance, like: SomeClass instance = new SomeClass(); instance.SomeStaticMethod(); //this line is one of the top 10 reasons of why java sucks IMO.) and here's the BlockHopper static method: @SideOnly(Side.CLIENT) public static Icon getHopperIcon(String par0Str) { return par0Str.equals("hopper_outside") ? Block.hopperBlock.hopperIcon : (par0Str.equals("hopper_inside") ? Block.hopperBlock.hopperInsideIcon : null); } What can I do? Basically, all I need to do is change the color of the hopper, nothing more, like tinge it a little. Is there a way to do that? Thanks
-
Open Vanilla GUI w/ item right click?
ILuvYouCompanionCube replied to GravityWolf's topic in Modder Support
try taking off the if(!world.isRemote) I don't think client EntityPlayers can open GUIs -
Crafting recipe error when using custom items
ILuvYouCompanionCube replied to sinsiliuxs120's topic in Modder Support
check whether you are defining all the letters in your recipe, each letter is an ingredient. And check if all the items/blocks in the recipe are registered -
you're welcome
-
If I recalll corectly, you can't trust item IDs the way you're doing inside your switch, The IDs for items are changed internally. Try replacing the constants inside the switch for your items' field itemID instead (i.e. make the cases in your switch block be flintshovel.itemID and flintaxe.itemID).
-
TileEntity unbinds from block so NBTTag is worthless
ILuvYouCompanionCube replied to jh62's topic in Modder Support
By the way, talking about tile entities and such, the tutorial on containers and GUIs is marked as outdated. The time has come for a hero to step in and update it so we, the common folk, can go on learning stuff. -
TileEntity unbinds from block so NBTTag is worthless
ILuvYouCompanionCube replied to jh62's topic in Modder Support
oh ok. That's weird haha -
TileEntity unbinds from block so NBTTag is worthless
ILuvYouCompanionCube replied to jh62's topic in Modder Support
Just a thing. Does the super class of your block implements ITileEntityProvider? You should always annotate overriden methods with @Override, so people reading your code can make better sense of it. (And also, because the compiler will complain if you add @Override to a method that doesn't exist on your super class or any of your implemented interfaces or if the method is private. This way you can check if everything works as you expect or if you're just creating a new method that minecraft can't ever know about) -
Thanks for your help Mew. I quit for now. Maybe I'll need to try something like this again someday, but I'm very tired. I have modding surges these days, then I spend hours modding and playing minecraft, then I rest from anything minecraft related for days lol. (well I actually rest from everything, minecraft related or not) Anyway thanks a lot. Good luck on your coddings
-
The tutorial tells me to create a package inside Minecraft\common folder but I can't. I can create packages in Minecraft\src only. I created the package in src and dragged it to common but then it was no longer a package , just a folder. And recompile.bat doesn't bother with it. Is there something wrong with my eclipse setup? Will it work normally if I create the package in src instead? EDIT: I tried creating the package in src. Recompiled and then reobfuscated. But the tutorial tells me to put my mod in a coremods folder. My minecraft installation has no such folder. Is this tutorial outdated by any chance? By the way, when I run it I get the expected IllegalAccessError caused by me trying to set the value of a final field
-
Minecraft 1.6.2, Forge version 1.6.2-9.10.0.804 I don't suppose this is a usual problem. I need to make a tiny change to the Block class at runtime that won't break any other mod because it's such an insignificant change (I only want to make Block.blockMaterial not final). But by the time my mod gets the first chance to run anything (even during the preInit event) the Block class has already been loaded and Java doesn't alow class reloading. The exception message is this: java.lang.LinkageError: loader (instance of net/minecraft/launchwrapper/LaunchClassLoader): attempted duplicate class definition for name: "net/minecraft/block/Block" I can't even find that package net/minecraft/launchwrapper, but I noticed some Forge classes use that LaunchClassLoader class and import it. Even if I've found it, I wouldn't know what to do... Is there any way to execute code before the Block class is loaded OR is there some other thing I can do to reflect on Block and change it's byte code just a tiny bit, even though it's already loaded? It would be monstruous to manually strip that field off that final modifier, because I'd have to make some kind of installer to replace the old Block class by my altered one... or something.