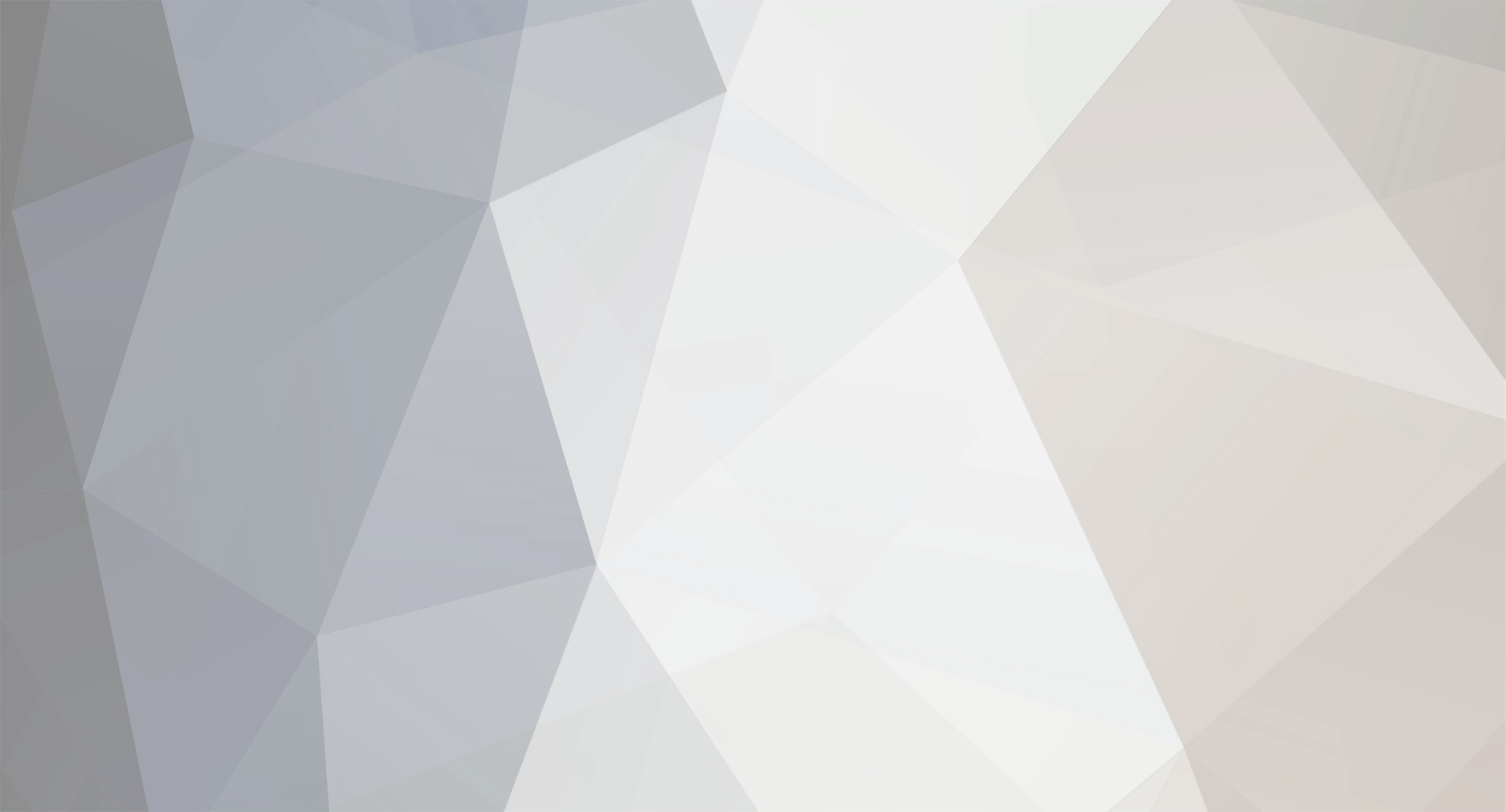
coolAlias
Members-
Posts
2805 -
Joined
-
Last visited
Everything posted by coolAlias
-
'instanceof BlockWall' means that yes, but not 'instanceof' - he can use 'instanceof CustomBlockWall' or whatever his Block class is and restrict the connections to his custom wall blocks instead of allowing all, if that's what he wants, but I assumed he would want them to connect to other walls as well (the logical thing to allow in most cases).
-
I don't see anything obviously wrong. If you put a System.out.println in both of your IGuiHandler methods, do you see output from those?
-
IBlockAccess is roughly equivalent to a World object. Instead of that mess of conditions, you could greatly simplify it: if (block instanceof BlockWall) { return true; // this should cover almost that entire wall of text } // this one: if ((block==TemBlocks.claywalls && meta ==0) || (block==TemBlocks.claywalls && meta ==1) || (block==TemBlocks.claywalls && meta ==2) || (block==TemBlocks.claywalls && meta ==3) || (block==TemBlocks.claywalls && meta ==4) || (block==TemBlocks.claywalls && meta ==5)){ return true; } // can be changed to: if (block==TemBlocks.claywalls && meta < 6) { return true; } // you'll want to check that one before the instanceof BlockWall check, however, or meta will be ignored // and this part: block != this // do you not want your wall blocks to be able to connect to themselves? Seems weird, but okay If it's not working as you expect, try something simpler at first: always return true. Does your block connect to everything? Good, at least you know that part is working, and now you can start introducing some logic into it one piece at a time. If it didn't work, then you can rule out your #canConnectWallTo implementation as the problem and begin looking elsewhere for issues.
-
Only thing I can see is you are sending the message twice - once when the key is pressed, and again when it is released. You should add ' && isDown' to your condition. You're not getting any error messages at all in your console log? Show your Container class, then, since that is the only other thing that has been added since it stopped working.
-
You may just be rendering it in the wrong place. Either put a break point in your render code and run the debugger, or put a System.out.println printing out the entity and HP in the render code and check your console output - you should be getting spammed with messages when looking at any entity.
-
Yes, it's from BlockWall. If you are not using it, you should override #createBlockState, #getStateFromMeta, and #getMetaFromState. However, since the default state + properties are usually set in the constructor, you should check BlockWall's constructor and make sure it is not setting that property; if it is, you are probably better off just extending Block instead of BlockWall, though I'm not sure how much of an impact that will have on your custom wall's compatibility with other walls. Another option would be to use a custom state mapper and ignore the variant state: ModelLoader.setCustomStateMapper(yourBlock, (new StateMap.Builder()).addPropertiesToIgnore(VARIANT).build());
-
Show your key handler and GUI classes. Are you by any chance using the same key to close the GUI?
-
That wouldn't work. For one, I assume 'block' is an instance of Block, which you are trying to test equality against an ItemStack. Totally different things. For another, ItemStack equality does not compare will with the '==' operator - that actually checks identity (Google it). If you want to test equality of a block + metadata, that is exactly what you do: // let's assume 'World world', 'Block block', int 'x', 'y', and 'z' are given as method parameters int meta = world.getBlockMetadata(x, y, z); if (block == TemBlocks.claywalls && meta == 0) { // there you go }
-
That's because your blockstate file does not include all of the block variants: hawm:wall_stonebrick#east=false,north=false,south=true,up=false,variant=cobblestone,west=true You can either use an UnlistedProperty for the stone type, or you need to include that variant in your blockstate file. I recommend using the Forge syntax - it will greatly reduce the number of files and lines you need to use.
-
That's not how the proxy system works - you make ONE reference to your CommonProxy class in your Main class, and all calls to proxies are run through that instance. FML will create the appropriate proxy type based on the environment at run time. This means that any method you want to call from your ClientProxy needs to exist in the CommonProxy class as well, usually with an empty implementation.
-
Crash log?
-
Ah, well if the texture doesn't already have an alpha layer, I'm not sure how to force it to render semi-transparently, sorry. Hopefully someone does, good luck!
-
-
Does it crash, or just not open? Show your IGuiHandler and if available, crash report.
-
[1.8] A question about performance and how to improve it.
coolAlias replied to Samalot's topic in Modder Support
Obviously, if you can avoid using a tick event, for example because the Item class already has #onUpdate which you can override to do things on a tick-by-tick basis, then avoid it. But if a tick event is the only way to make your mod work, then go for it - as long as you don't overdo it (avoid nested loops and recursive calls as much as possible) it has negligible impact on performance. And yes, you shouldn't be putting most of your Item code in event handlers rather than the Item class itself, but depending on what you are trying to do, this may be unavoidable. LivingHurtEvent is a good example - you can't use the Item AttributeModifiers since I assume the Item is not held or equipped while doing damage, so that event is really the only way you can apply your damage bonus. That's perfectly fine, especially since it sounds like you are setting up your logic to break out early and avoid processing when not necessary, which is the best you can ask for. Basically: events are already pretty optimized and, in comparison to everything else that is already going on in Minecraft, require negligible processing power UNLESS you do something stupid in there -
Sure: save a boolean value to your Config file. When you read the config, check if that value is false - if it is, generate the files, set the value to true, and save your config. Next time, the value will be read in as true and the files will not generate; in the future, you can always edit your config file and change the value back to false to re-generate the JSON files.
-
First, your texture needs to have an alpha layer - you can add these via GIMP or PhotoShop pretty easily. Then, when rendering, you need to enable the alpha layer: // first push attributes, like pushing the matrix, so you don't mess up anything // that renders after your block GlStateManager.pushAttrib(); // enable alpha and blend so you can render your alpha layer GlStateManager.enableAlpha(); GlStateManager.enableBlend(); // render your stuff here // finally, pop to return the attributes back to their original state GlStateManager.popAttrib();
-
You can use Forge's blockstate format to condense the number of JSONs required. What exactly is not working? Are you getting the purple and black texture, or is something else wrong? Check your console log output for texture errors.
-
I'm not sure why you can't drop it (might have to do with removing the ItemStack parameter), but to prevent it from being immediately picked up you need to set the pickup delay: entityitem.delayBeforeCanPickup = 40; You can see a working example (i.e. one that can be both dropped and picked up) here.
-
The problem is you are still using the client player in your message handler, despite being on the server side: EntityPlayer playerIn = Minecraft.getMinecraft().thePlayer; You should be getting the player via a call to your proxy: EntityPlayer player = Main.proxy.getPlayerEntity(ctx); // CommonProxy / ServerProxy implementation: public EntityPlayer getPlayerEntity(MessageContext ctx) { return ctx.getServerHandler().playerEntity; } // ClientProxy implementation: @Override public EntityPlayer getPlayerEntity(MessageContext ctx) { return (ctx.side.isClient() ? Minecraft.getMinecraft().thePlayer : super.getPlayerEntity(ctx)); }
-
[1.7.10]NullPointerException: Unexpected error
coolAlias replied to _Lightning_'s topic in Support & Bug Reports
It looks like one of the mods is creating an ItemStack that does not contain an Item, possibly in the context of a GUI or other client-side element since no one else crashes when they go to that chunk. Did you break any particular Block or use any particular GUI / Item right before the crash? -
[1.8.9] [SOLVED] PlayerTickEvent not applying damage when it should
coolAlias replied to Daeruin's topic in Modder Support
Player motion is unfortunately handled almost entirely on the client. What you can do is listen on the client for the player movement, then send a packet to the server to perform the damage. -
You won't see the source code in your mod directory - you find it using your IDE by looking in the referenced libraries. The actual location of the setupDecompWorkspace output varies but is usually found in a folder somewhere under your /%User%/ directory. You can find out by checking the path of the forgeSrc entry under your IDE's referenced libraries. EDIT: Sorry, didn't see your note about not using an IDE :\
-
[1.8.9] [SOLVED] PlayerTickEvent not applying damage when it should
coolAlias replied to Daeruin's topic in Modder Support
The player doesn't take any damage on the client because it's not supposed to - changes to data should only ever occur on the server, which then, if the data is important for the client to know about (e.g. for rendering GUIs), notifies the client of the current values. Since you are testing on single player, the client and server are not really separated - the integrated server runs in the same program instance as the client - so your event handler only has one instance shared between the two. When you increment the tick counter on either side, the increased value is also seen on the other side, so both sides are incrementing the same counter. You would not notice this behavior when running on a dedicated server. Anyway, to fix your issue, nest all of your event code in an 'if (!event.player.worldObj.isRemote)' statement - that will ensure your code only runs on the logical server side, which is what you want. -
[Solved] [1.9] IBlockState in BlockEvent.HarvestDropsEvent
coolAlias replied to Voidi's topic in Modder Support
Because the block was harvested, so the space it used to inhabit is now air. Use the event class fields to access the harvested block data.