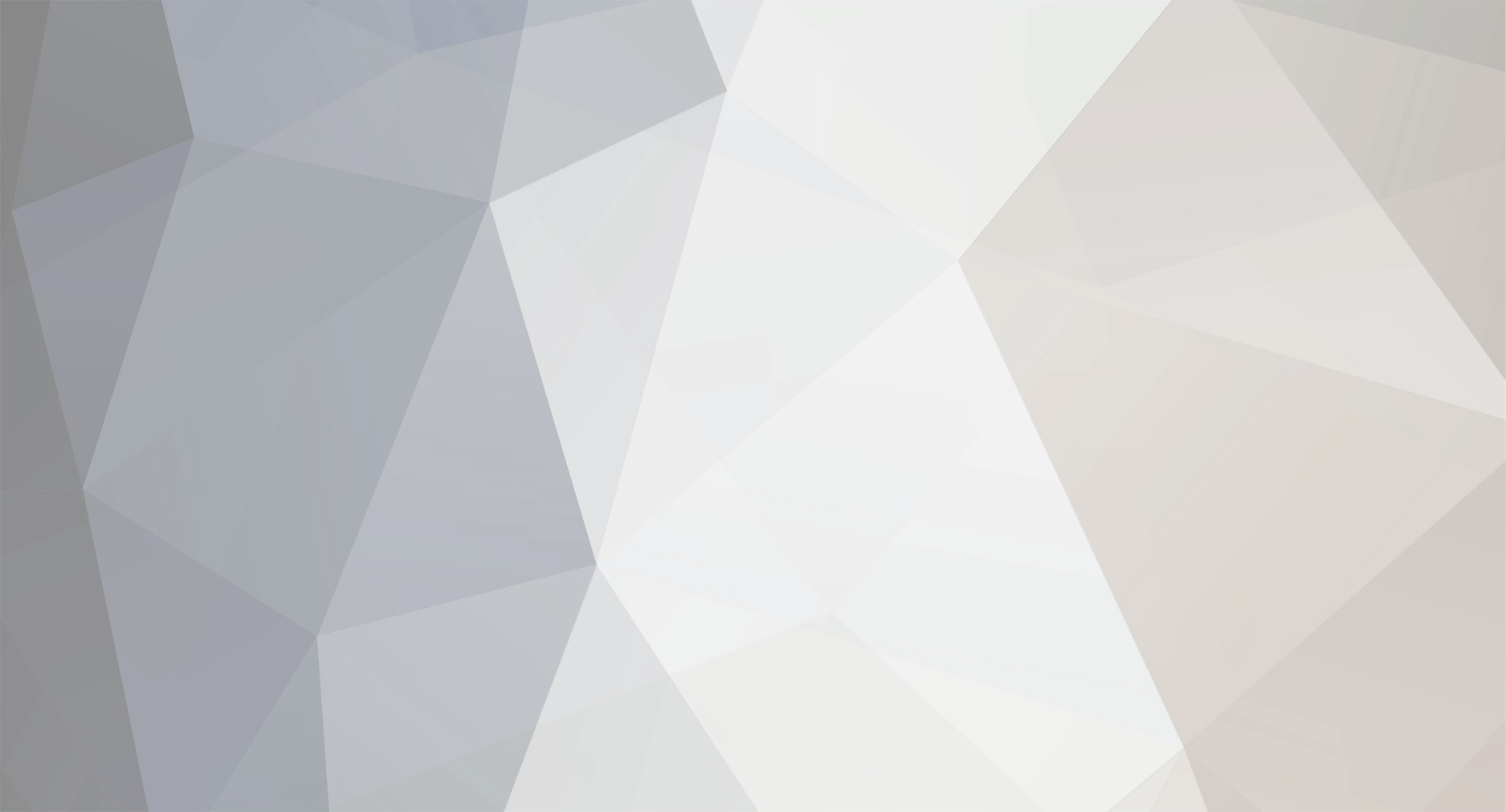
coolAlias
Members-
Posts
2805 -
Joined
-
Last visited
Everything posted by coolAlias
-
Minecraft 1.8.9 Eclipse Won't Run Server
coolAlias replied to Monstrous_Apple's topic in Modder Support
You would make a class named 'CommonProxy' in your com.MonstrousApple.mod package. It might be beneficial for you to use your favorite search engine to find some intro modding tutorials. -
You didn't follow the rabbit-hole far enough - eventually it should lead you to the ConfigurationManager or whatever the class is that you get from playerMp.mcServer.getConfigurationManager(), which has some nifty methods for interdimensional travel. I use it in 1.8.0, but it may well be named something different now.
-
The default behavior for non-vanilla TileEntities is to destroy any data it may have whenever the blockstate changes (this is the opposite behavior of vanilla TEs). If you want your data to persist across changes in block state, you can override the #shouldRefresh method in your TE: @Override public boolean shouldRefresh(World world, BlockPos pos, IBlockState oldState, IBlockState newState) { return (oldState.getBlock() != newState.getBlock()); }
-
Did you try looking at the vanilla code for the Nether or End portal and associated Teleporter? I bet those would have what you need.
-
Your problem is you are only opening the GUI on the client - Container-based interfaces need to be opened on the server. I.e. in your block class #onBlockActivated, change 'if (world.isRemote) {' to 'if (!world.isRemote) {' as the condition for opening the GUI.
-
[1.9] Getting the recipe of itemstacks that make up an item!
coolAlias replied to madcrazydrumma's topic in Modder Support
And the solution has, is, and probably always will be the same: loop through all the registered recipes and keep a Collection of any that match your criteria. You can do this once for all recipes you need during startup, or you could load each one on demand as it comes up. -
[1.9] Getting the recipe of itemstacks that make up an item!
coolAlias replied to madcrazydrumma's topic in Modder Support
Pretty sure that's the only way and if it's not, the other way is just a method that does it for you. Keep in mind that there may be more than one recipe to produce the same ItemStack (both shaped and not). -
Show the code you use to open the GUI as well as your IGuiHandler and Gui classes, preferably using a service such as pastebin, gist, etc. Probably wouldn't hurt to show your IInventory implementation either.
-
You add 5 slots per iteration of your for loops, and your for loops result in 27 iterations. 27 * 5 = 135 slots, plus the 8 you added for the hot bar. Move the 4 static (as in their position, not the Java keyword) slots outside of the loop: for (int x = 0; x < 9; x++) { for (int y = 0; y < 3; y++) { // this line covers the regular player inventory addSlotToContainer(new Slot(invPlayer, x + y * 9 + 9, 8 + 18 * x, 88 + 18 * y)); // and these 4 slots are added 27 times to the exact same location with a different id each time... //addSlotToContainer(new GenericSlot(decomp, nextId(), 80, 15)); //addSlotToContainer(new GenericSlot(decomp, nextId(), 60, 64)); //addSlotToContainer(new GenericSlot(decomp, nextId(), 80, 64)); //addSlotToContainer(new GenericSlot(decomp, nextId(), 100, 64)); } } // moved outside of the loop they will work just fine addSlotToContainer(new GenericSlot(decomp, nextId(), 80, 15)); addSlotToContainer(new GenericSlot(decomp, nextId(), 60, 64)); addSlotToContainer(new GenericSlot(decomp, nextId(), 80, 64)); addSlotToContainer(new GenericSlot(decomp, nextId(), 100, 64));
-
Register quartz pillar (EDIT: For structure)
coolAlias replied to Betterjakers's topic in Modder Support
That's practically identical to 1.7.10 as far as setting blocks. -
[1.8.9] WorldSavedData not executing readFromNBT [SOLVED]
coolAlias replied to Atijaf's topic in Modder Support
Yep, in your case (which is the typical case), you should use NBT.TAG_COMPOUND when retrieving your list. If your #readFromNBT is never getting called, is your static #get method ever called? How about #writeToNBT? Also, I'm a little dubious about your use of long as a storage method for potentially an entire chunk's worth of data... while it may work most of the time if you generally need to store only a few positions per chunk, it seems extremely fragile to me for very little benefit. Of course, I don't know the whole story, so maybe you will only ever have 1 or 2 positions per chunk and that's fine, but something to consider. -
Register quartz pillar (EDIT: For structure)
coolAlias replied to Betterjakers's topic in Modder Support
Okay, but why are you making a static ItemStack? That doesn't make sense either for registering a recipe, nor for placing a block. If you want to reuse it, that's fine, but just use a local variable inside the method rather than a class field. Anyway, you haven't mentioned Minecraft version, but here you go: Block block = block you want to use, e.g. Blocks.blockNetherQuartz World world and either BlockPos or x/y/z coordinates assumed from method // 1.7.10 world.setBlock(x, y, z, block, metadata, 2); // 1.8+ world.setBlockState(blockPos, block.getDefaultState().withProperty(someProperty), 2); -
How to add a mana setting to the player character?
coolAlias replied to crazygod2016's topic in Modder Support
Since you didn't specify Minecraft version: adding mana via IEEP tutorial for 1.6.4 through 1.8.0 can be found here. It helps people respond with more appropriate advice when you include the Minecraft version for which you are coding in your thread's title. -
[1.8.9] WorldSavedData not executing readFromNBT [SOLVED]
coolAlias replied to Atijaf's topic in Modder Support
How do you know it is not being called vs. simply failing? For example, you use NBT.TAG_LIST, but you are not storing a list of lists, you are storing a list of NBTTagCompounds, so you should be using that constant instead (the constant is the type of tag stored in the list, not the list itself). -
Register quartz pillar (EDIT: For structure)
coolAlias replied to Betterjakers's topic in Modder Support
Try Blocks.quartz_block - if that's not the block you want (which I don't think it is, but I don't recall), then open up the Blocks class or expand it in your package explorer pane and you can see all of its class fields and search until you find what you're looking for. -
[1.8.9] WorldSavedData not executing readFromNBT [SOLVED]
coolAlias replied to Atijaf's topic in Modder Support
In 1.8.0, I used: world.getPerWorldStorage().setData("unique_tag_name", nbttag); I'm not sure if 1.8.9 changed that to just World#setItemData, but it might be worth looking into. -
Setting motion client side would actually be a suitable approach, as that is where player motion is handled for the most part anyway. You don't have to simulate the key press itself to simulate the result of the key press That all said, the major issue is going to be path-finding. I suggest you take a look at the various pathing / move helper classes used in mob AI and consider using that as the basis for moving the player. This is not a trivial exercise, especially for a new coder, but at least Mojang has done most of the work for you. Another big issue is: what do you expect the player to be doing while you affect their motion? Will they still be in control, or is this supposed to be some kind of cinematic-like effect where the player sits back and watches as Steve/Alex gets moved around?
-
My suggestion: don't. Unless your blocks are all closely related, it is my opinion that your code is much better off with individual block classes and instances. But, if you really want to, there is a Block method (NOT the one in BlockContainer, but the one added by Forge) that returns a TileEntity and has the IBlockState / metadata as an argument, so you CAN return different TileEntity types based on that criteria. However, I believe you would be limited to the 16 values of IBlockState, as you wouldn't be able to use the 'actual' state at this point given that the TileEntity does not yet exist. As for rendering, #getActualState is perfectly fine, and you probably won't even need a TESR to do it - ISmartBlockModel (or whatever replaced it in 1.9) provides everything you need to render based on the extended block state.
-
[1.8 and 1.9] Having a few issues on 1.9 and 1.8
coolAlias replied to NovaViper's topic in Modder Support
I've found that NBT is read AFTER applyEntityAttributes(), so #isChild will always return false at that time. I got around it by adjusting those particular attributes as needed from the #readFromNBT method and/or when the entity first joins the world. -
[Solved] [1.8.9] Keybinds dont work when set to mouse buttons
coolAlias replied to Aulig's topic in Modder Support
You shouldn't be checking Mouse at all, just use your KeyBinding. The KeyBinding class doesn't care what button it is bound to, as long as the button is pressed, the KeyBinding will tell you that. if (Mymod.toggleTest.getKeyIsPressed()) { // might be #getIsKeyPressed or something, don't recall exactly // key binding was pressed, whether assigned to Keyboard, Mouse, GamePad, whatever } -
[1.8.9][SOLVED] Problems with PacketHandler and getXP
coolAlias replied to Mark136's topic in Modder Support
Note that event handlers are not usually implemented as static methods, at least in my experience. I don't know if that will break anything, but there is no reason to make the method static, and certainly do NOT use static ID - what the heck is that for? The BreakEvent GIVES you the block that was broken - use it. I'd also recommend making a lookup table for your xp charts, especially if you are going to include more block types or want a way for other mods to add their own blocks via some kind of API. As a brief and incomplete example: private static final HashMap<Block, Integer> xpChart = new HashMap<Block, Integer>(); public void setBlockXp(Block block, int xp) { xpChart.put(block, xp); } // then in your break event: @SubscribeEvent public void onBreak(BreakEvent event) { Integer xp = xpChart.get(event.block); // note that this may return NULL // etc. } -
Override Block#getDrops to create an ItemStack with the appropriate NBT for the existing TileEntity (the TE will still be intact at the time this method is called). Override Block#onBlockPlacedBy to read information from the ItemStack NBT and set it for the TileEntity (which has already been created for you by this point).
-
[Solved] [1.8.9] Keybinds dont work when set to mouse buttons
coolAlias replied to Aulig's topic in Modder Support
From my experience, KeyInputEvent does not fire at all for mouse buttons, so you have to also listen to MouseEvent (in which the real key code is equal to event.button - 100) and, if that key code is one of your key bindings, do your thing. An alternative is to not use either of those events but instead subscribe to the ClientTick event and iterate through all of your key bindings checking if any are pressed / released and handling them from there. This is the option most often advocated. -
[1.8.9] How can I create a gui with tabs?
coolAlias replied to TheCrafter4000's topic in Modder Support
Take a look at how the CreativeTabs work, as it sounds like exactly what you are trying to accomplish. -
[1.8 and 1.9] Having a few issues on 1.9 and 1.8
coolAlias replied to NovaViper's topic in Modder Support
Animations for a Block, an Entity, or something else? They are all somewhat different, although similar. To animate something, all you need are 'key frames', i.e. the important reference poses that you will be moving through. The more key frames you have, the better your animation will look, but you can't have more than one frame per render tick, so the length of your animation will limit that, and you usually don't need that many key frames. The minimum you can get away with is 2 key frames - the start pose and the end pose - as well as the number of ticks you want the action to take, and then each tick you interpolate between the end and start frames to get the current frame's angles etc. It's really simple in concept, but also can be quite complicated. BobMowzie has some good video tutorials on and . Even if you choose not to use the 3rd-party libraries that he does, you can still learn a lot about the concepts. Here is by Jabelar about animating, and below is an example of code I use for animating entities, using my own custom-built framework: Obviously there is a lot more that goes into it than just that, but it gives you an idea. You also have to consider that any of your Entity fields that you need to use to trigger / control the animation must be synced to the client. I typically use #setEntityState and #handleHealthUpdate to set animation flags, but you can use custom packets as well.