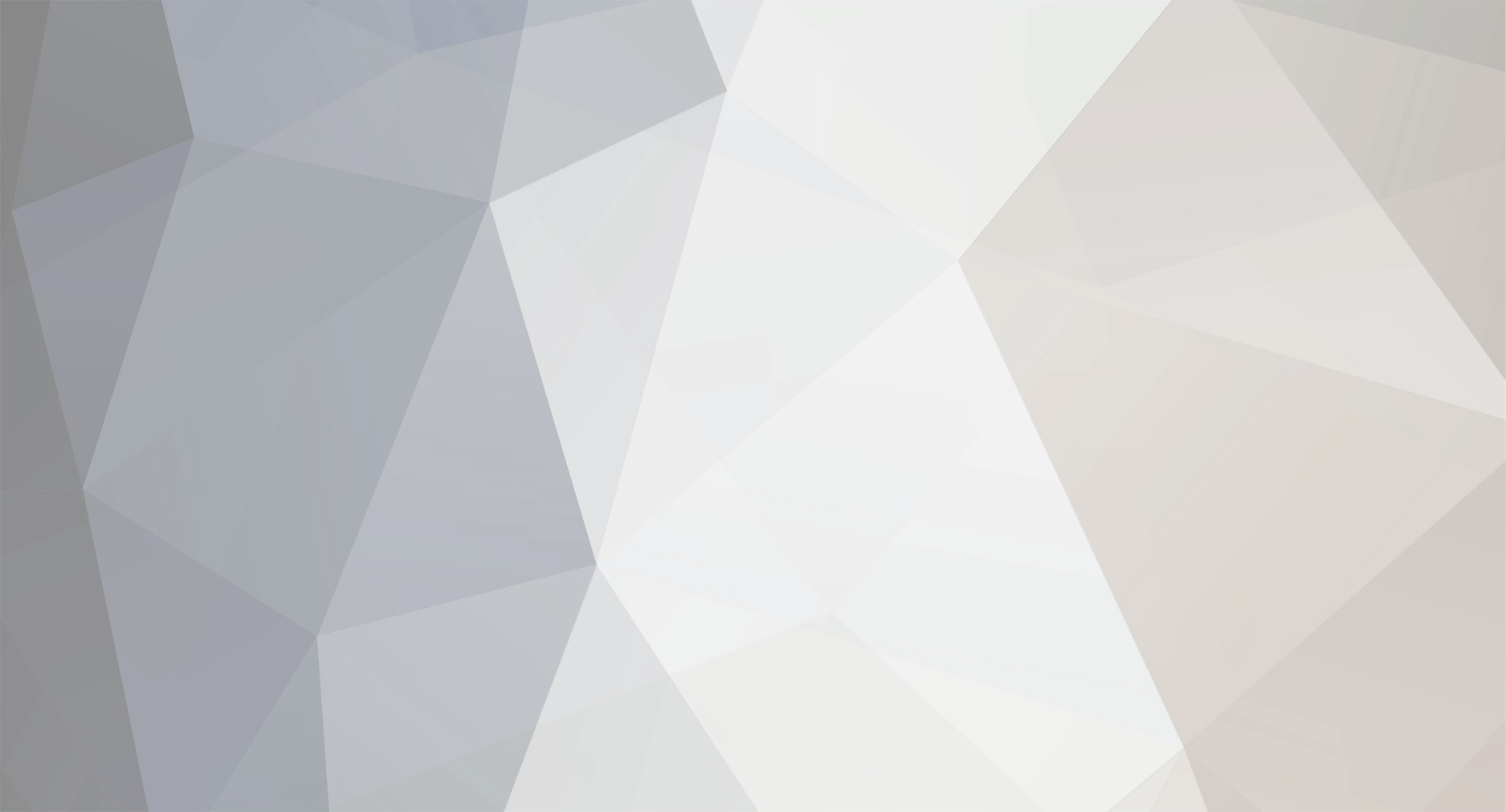
coolAlias
Members-
Posts
2805 -
Joined
-
Last visited
Everything posted by coolAlias
-
Perhaps try adding '@Override' annotation above your addInformation method?
-
Oh, sorry about that - it's just an int for how far you want to check, like 64 blocks or whatever max distance your item will work at.
-
The error log says it all: can't cast NBTTagFloat as NBTTagInt. Do you see: float xp = itemStack.stackTagCompound.getInteger("stored");
-
How To Add Kill Counter To A Custom Tool
coolAlias replied to fierymelon300's topic in Modder Support
addInformation is a method belonging to the Item class, so you need to implement/override it in your custom Item class. In the method, you will need to check if the Item NBT tag is null and if so, create a new tag first, otherwise you will crash your game when you mouse over your Item in the Creative Tab. -
How about the 'onUpdate' method from Item? It is called automatically for every ItemStack containing your Item each tick, so long as you override this method in your Item class. Maps use it to update while being held, but it's called so long as the item is in your inventory, not necessarily held. These are the parameters: public void onUpdate(ItemStack itemstack, World world, Entity entity, int par4, boolean isCurrentItem) Sorry I'm not sure what 'par4' is, I think ticks in use but I may be wrong.
-
Don't forget to click the 'Thank You' button for posts that help you.
-
You can try using 'getMovingObjectPositionFromPlayer' method to return a MovingObjectPosition, but that will only get you a tile if it is within reach distance. Otherwise, you will need to use vector's from the player's look position: Vec3 vec3 = player.getPosition(1.0F); Vec3 vec3a = player.getLook(1.0F); Vec3 vec3b = vec3.addVector(vec3a.xCoord * distance, vec3a.yCoord * distance, vec3a.zCoord * distance); MovingObjectPosition movingobjectposition = world.clip(vec3, vec3b); There may be a better/simpler way than this, but this worked for my purposes. The MovingObjectPosition will contain information on the tile hit, if any. Be sure to null-check.
-
onCreated is only called when the Item is crafted or smelted, while addInformation is called when you mouse over the item, such as in a Creative Tab, at which point it hasn't been created yet. You need to check if the NBT tag is null and create a new one if so in any method that may need to access it.
-
Can I use Entity.isCollidedHorizontally server side?
coolAlias replied to coolAlias's topic in Modder Support
Perhaps, but I've used event.entity.fallDistance = 0 with other checks and it works properly for both client and server, whereas here, I'm able to climb up and down but I still take damage on fall. This suggests that isCollidedHorizontally is only true on the client side. Printing output to the console within the 'if' statement proves this to be true: event.entity.fallDistance = 0.0F; if (!event.entity.worldObj.isRemote) System.out.println("[EVENT] Server side: On update fall distance: " + event.entity.fallDistance); else System.out.println("[EVENT] Client side: On update fall distance: " + event.entity.fallDistance); Only the client side prints. -
[solved] RenderPlayerEvent.SetArmorModel help
coolAlias replied to SenpaiSubaraki's topic in Modder Support
Not sure which version of Forge you're using, but if you're using at least 871 it looks like they've added some hooks into RenderBiped to allow for setting custom armor models: modelbiped = ForgeHooksClient.getArmorModel(par1EntityLiving, itemstack, par2, modelbiped); this.setRenderPassModel(modelbiped); Which links to: public static ModelBiped getArmorModel(EntityLivingBase entityLiving, ItemStack itemStack, int slotID, ModelBiped _default) { ModelBiped modelbiped = itemStack.getItem().getArmorModel(entityLiving, itemStack, slotID); return modelbiped == null ? _default : modelbiped; } Looks like you can now return a custom model from your ItemArmor class. From there your custom render for your armor model should be called, judging by the next line. -
Hi, I've been using isCollidedHorizontally to add ladder/spider-like behavior onLivingUpdate, but the entity takes damage when coming down even if I set fallDistance to 0. I tried sending packets to the client only to discover that the code was only being called client side. // in LivingUpdateEvent, no preceding 'if' statements if (event.entity.isCollidedHorizontally) { if (event.entity.isSneaking()) { event.entity.motionY = -0.1F; } else { event.entity.motionY = 0.1F; } // This part doesn't work; player still takes damage when coming down even when sneaking event.entity.fallDistance = 0.0F; } As you can see, it's very simple code that allows the player to climb up and down walls. I checked out Entity's moveEntity method since that's where isCollidedHorizontally is set, but didn't see anything there to suggest it is client-side only; however, it's obviously only called client side. Is there a way to avoid updating the server from a client packet but still get it to work?
-
Yes, you did: while(!(world.getBlockId(x, y, z) == Block.grass.blockID)) {--y;} There's nothing in there to stop the while loop other than hitting a grass block, but if you never encounter a grass block, it will keep going forever. Add '&& y > 2' or something like that if you want to stop that low (like in a superflat world) or '&& y > 62' or something for around ocean level.
-
It printed '0' for getBlockId(x, y+1, z) ? Try at (x, y, z) and see what that block is. If it is also air, then that would be your problem. Just experiment a little on your own with the values, printing out block id s at each one, trying with your canBlockStay check and without it, etc. You'll find the problem much more easily that way than waiting for someone else to try to figure it out from snippets of code.
-
[1.5.2] How to make an item that has an inventory?
coolAlias replied to AskHow1248's topic in Modder Support
That error is your own. Use Keyboard.isKeyDown(Keyboard.KEY_K), like I did in the tutorial, and it should work fine. If it gives you an error, then you've done something wrong. What is the error it gave you? It won't be multiplayer compatible - for that, see the note at the top of the tutorial. -
Then the problem is either the block at (x, y+1, z) is not an air block or your custom Block's canBlockStay method is incorrect. Print out the block id at those coordinates (before the if statement) to see what it is. I'm guessing it's grass or some such. If that's the case, you can try something like this: if (world.isAirBlock(x, y+1, z) || (Block.blocksList[world.getBlockId(x, y+1, z)] != null && !Block.blocksList[world.getBlockId(x, y+1, z)].blockMaterial.blocksMovement())) It checks if the block allows movement and if so, still let's you place your block. What's your block's canBlockStay method look like?
-
You are still assigning y to a random int after you've found the ground, meaning you are probably trying to generate your block underground or in the air. Also, you failed to assign coordinates before checking for ground, meaning the while loop is pretty much useless, as it isn't looking at the coordinates you want to place the block. Also this: (!world.provider.hasNoSky || j1 < 127) should all be handled in your heart block's canBlockStay method. One final note is that when you find the ground, that's what you've found. Try generating your block at y+1 to set the block ABOVE ground to your flower. Try this code: @Override public void generate(Random random, int chunkX, int chunkZ, World world, IChunkProvider chunkGenerator, IChunkProvider chunkProvider) { for(int l = 0; l < 64; ++l) { int x = chunkX + random.nextInt(16); int y = 128; int z = chunkZ + random.nextInt(16); while(!world.doesBlockHaveSolidTopSurface(x, y, z) && y > 128) { --y; } if(!world.doesBlockHaveSolidTopSurface(x, y, z)) { continue; } if (world.isAirBlock(x, y+1, z) && Block.blocksList[blockHelper.heartFlower.blockID].canBlockStay(world, x, y+1, z)) { world.setBlock(x, y+1, z, BlockHelper.heartFlower.blockID, 0, 2); } } } If it still doesn't work, put some println in there and see if it is being called like you think.
-
[1.5.2] How to make an item that has an inventory?
coolAlias replied to AskHow1248's topic in Modder Support
Thanks, I already knew that. That's why I explicitly told you it was for 1.6.2. I didn't give you exact code to copy, I gave you information on how to implement your idea conceptually with a fully functional example in 1.6.2. You'll have to work backwards from that to make it 1.5.2 compatible. What about it doesn't work? What errors are you getting? Is there any output in the console that might help you? Posting this kind of error information as well as your code will get you help. Simply stating that it 'doesn't work' will not get you anywhere. -
The way you're doing it, you'll want to place the while loop within the for loop, and when you check if there was not a solid surface, instead of 'return' you'll want to use 'continue'. Chunk coordinates x and z define the start position of the chunk, not any actual coordinates within it; when you get the coordinates, you don't need to add and then subtract random ints, just add nextInt(16) and you'll be fine. y doesn't need to be random at all, just set it back to 128 each iteration. Are your flowers generating at all? If not, maybe you forgot to register your IWorldGenerator in the main mod class.
-
It only has x/z because it gets an entire chunk, which is 16x16 blocks area 256 blocks tall, so all of the y coordinates are included. Try setting y to a high value such as 128 and then use a while loop to find the ground level, then add your block at that position: // Check for solid surface only above around ocean level while (!world.doesBlockHaveSolidTopSurface(x, y, z) && y > 62) { --y; } // If you didn't find a solid surface, return; check if your flower can stay at this point if (!world.doesBlockHaveSolidTopSurface(x, y, z)) { return; } // generate your flower I'd recommend checking out Wuppy's tutorial series, specifically 1.3.2 because he covers lots of world gen stuff there that is still relevant. This one in particular applies to your situtation, though it generates ore: http://wuppy29.blogspot.nl/2012/08/modding-ore-generation.html
-
Override the following method: /** * Velocity of entity; default value 1.5F for ThrowableEntity */ @Override protected float func_70182_d() { return 1.5F; // set to speed you want } The method name may or may not have changed, depending on Forge version, but you can find it in ThrowableEntity.
-
[1.5.2] How to make an item that has an inventory?
coolAlias replied to AskHow1248's topic in Modder Support
This tutorial adds an inventory to an item: http://www.minecraftforum.net/topic/1949352-creating-an-item-that-stores-an-inventory/ It's for 1.6.2, but most of the concepts should be the same. Basically you need to store the inventory in the itemstack's NBT. There's a good tutorial specifically about Item NBT on the Forge wiki: http://www.minecraftforge.net/wiki/Item_nbt -
[SOLVED] Synchronizing ItemStack NBT during onItemUse method
coolAlias replied to coolAlias's topic in Modder Support
Solved it. Don't know why I didn't think of this earlier, but I can just send the chat message from the server upon receipt of the packet. Now the client side doesn't have to handle any of the NBT data at all. Thanks for the clarifications, and sorry for troubling you. I was indeed making it too complicated. -
[SOLVED] Synchronizing ItemStack NBT during onItemUse method
coolAlias replied to coolAlias's topic in Modder Support
I was only trying to think of a way to minimize traffic. Guess it's not a big deal, though. Yes, but it won't be synced quickly enough to display the correct values immediately, which is why I did what I did above. As you can tell, I'm no expert in Java, and I couldn't think of an easy way to display the information immediately without changing the client-side NBT. As I mentioned, it's only used for the display, not any actual calculations that affect the world, player or anything else. I only send the key pressed to the server, not the client side NBT data. Is this still bad form? What would you suggest, then, to display the information? -
[SOLVED] Synchronizing ItemStack NBT during onItemUse method
coolAlias replied to coolAlias's topic in Modder Support
The way I have it set up now is to send packets to the server with the key pressed information, but I also directly edit the NBT client side for display purposes only. When the NBT is actually needed for processing, it only uses the NBT stored on the server. Would this be considered acceptable, or should I not edit the NBT at all client side without requesting the data from the server? I'll show you what I mean: Then in the Item onItemUse, I only get NBT data from the server: (And sorry about spamming the tutorials - I thought they were relevant to the topics, though some were a few days old they didn't look resolved yet, except for one... my bad.) -
[SOLVED] Synchronizing ItemStack NBT during onItemUse method
coolAlias replied to coolAlias's topic in Modder Support
Heh, yeah I know. I was just trying to figure a way around sending so many packets, but I guess there's no help for it in this situation. I was really hoping to be able to just send one packet with all the information, but since the keyboard is handled client side, that's impossible (as far as I know) without editing NBT from there, which isn't good practice. Thanks for the reply anyway.