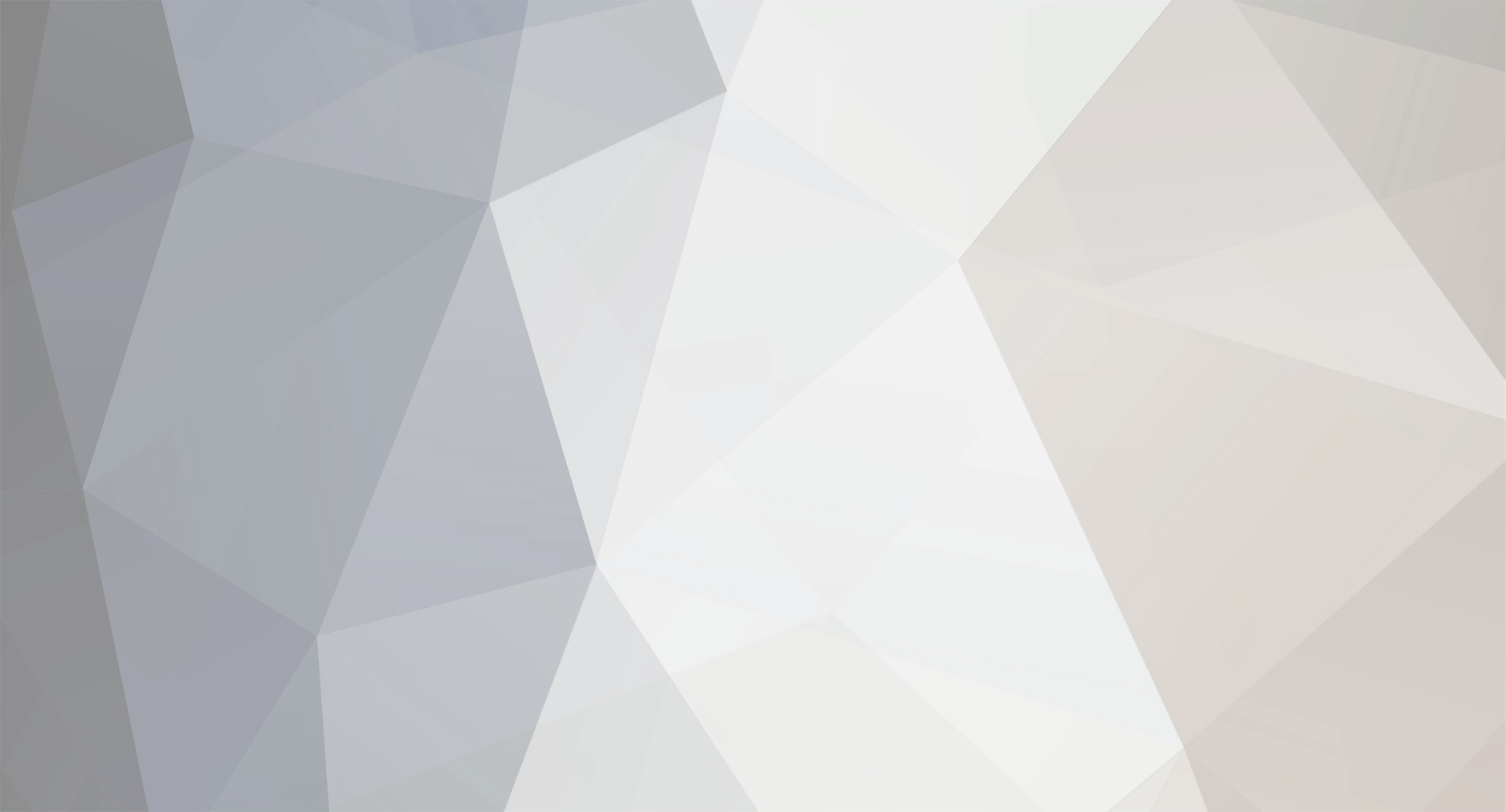
coolAlias
-
Posts
2805 -
Joined
-
Last visited
Posts posted by coolAlias
-
-
Don't have access to the code right now, but you may want to take a look at the other methods in the BlockLadder class, specifically the two #onEntityCollidedWithBlock methods. I believe that is where motionY is added the climbing entity, but if not, then you can use your IDE's search function to find the locations of BlockLadder and Blocks.ladder (or whatever it is called) in the code and inspect those to find out how ladders function.
-
Do you have any mods installed, and are you using any modded blocks in your structure? The trouble with modded blocks is that they do not have set IDs - the integer IDs can and will be different between different worlds, so they are no longer a valid storage format without some sort of converter (e.g. a file storing the map of block IDs to block registry names used to create the structure).
-
No, but since you're passing '0', chances are you are getting a non-null but incorrect ItemStack in that slot in your IGuiHandler, so you either need to pass player.inventory.currentItem OR actually do a real check in your IGuiHandler (which would be safer anyway) - note that you can and should do both.
// in IGuiHandler ItemStack stack = player.inventory.getStackInSlot(x); if (stack != null && stack.getItem() instanceof YourBackPackItem) { // okay to return GUI or Container element } else { // do nothing - invalid item }
-
Thanks. Unfortunately I don't know how to trigger the crash (it happens randomly quite occasionally), but I will try this.
It happens when a non-player causes blocks to drop, such as water flowing over crops or a creeper exploding (maybe the creeper counts as a harvester? it's been months since I've looked at the code).
-
Show your Item code.
-
The typical way to go about it is to check if the player can see the sky or not.
The problem with that is how do you differentiate being under a tree or in a house vs. in a cave? So you have to decide how would you even define a 'cave'? Should it be mostly stone blocks? What about dirt? Sandstone? Mineshafts?
Once you decide that, you can get fancier and check surrounding blocks, but that will slow your algorithm down exponentially with the search radius. To make it faster, you could only check the first non-air block above the player and see if it's in your whitelist of acceptable 'cave' blocks, but then you still get false positives if a player makes a house of stone, for example.
Anyway, there isn't any surefire way that I know of to say with 100% certainty a player is in a cave, so you have to figure out what is an acceptable level of error for your purposes.
-
The shift-click ghost issue is one I have noticed occurs when you limit the stack size of your inventory slot to 1 - is this perhaps the case with your inventory?
If so, the issue isn't your code, it's Mojang's, but you can fix it by overriding #mergeItemStack in your Container class and using the code found in that link. That implementation I wrote myself almost 2 years ago now, but I've seen others do it slightly differently since then that you may want to look at also, or if you want a challenge you can avoid looking at my or anyone else's code and see if you can't figure out what's wrong with Mojang's implementation on your own
-
If you read the docs for HarvestDropsEvent, you'll see that it specifically states that 'event.harvester' can be NULL, so you need to check for that.
-
Within a given loot table, there is not any way of which I am aware to affect what is chosen - thus the use of different loot tables when fishing.
It might be possible, however, to change the weights of the items in the table before selecting an item, but unless you made a copy of the table to do so (possibly slow) or make sure to change it back (possibly affects other loot using the same table or even potentially risks ConcurrentModificationException), then it's probably not advisable.
-
Where do you register it? Show that entire class, not just the single line.
Also, are you still testing on a server with the mod only on your client? Because this very likely isn't going to work if you don't have the mod on the server, too.
-
That's the same error, but caused because when you right click, you aren't sending a packet and thus not sending the slot index (well, hopefully you're sending '0').
You can fix the issue by sending player.inventory.currentItem, or you can also do a check for the player's held item in the IGuiHandler code and use that if it is correct, or you can pass a 'flag' such as -1 as the slot index when right-clicked which you can check for to know that you need to use the held item instead of the inventory slot.
-
Are you using your main mod class as the event handler? Probably not the best of ideas, but if that's really what you want, use the mod instance that should already exist,e.g. Main.instance.
That all said, we can't help you if all you say is 'it doesn't work' and don't show us any other code. Show us the entire class that contains your mis-named LivingHurtEvent handler, as well as the entire class containing your registration of that event handler.
And again, keep in mind that LivingHurtEvent only fires on the SERVER except under very specific circumstances, so if you are trying to make a client-side only mod, you are probably going to have to come up with a different solution.
-
That's strange that it requires a copy to be made, but that still doesn't negate the fact that you are reducing a stack of unknown size to a size of 1 for no apparent reason.
Why not just return the copy directly?
@Override public ItemStack getContainerItem(ItemStack itemStack) { return itemStack.copy(); }
-
If I recall from my own testing, LivingHurtEvent only fires on the server UNLESS the hurt entity is a player. So you will not be able to do what you want with a client-side only mod.
-
You're passing the slot index so that you can retrieve the ItemStack from the inventory rather than using player.getHeldItem(), since you're not requiring the item to be held.
So replace 'player.getHeldItem()' with:
ItemStack stack = player.inventory.getStackInSlot(x); // where 'x' is a method parameter passed via IGuiHandler // maybe check again if the stack is correct, just to be safe // then pass it into your inventory constructor when returning the GUI or Container, e.g.: return new GuiCelestialKeyPouch((ContainerCelestialKeypouch) new ContainerCelestialKeypouch(player, player.inventory, new InventoryKeyPouch(stack)));
-
I don't recognize BlockHelper as a Forge class, but I don't have the code to check right now - are you sure it wasn't from a 3rd party library or something? Show the imports for your ore gen class from 1.8.
-
It looks like somehow you have created an invalid ItemStack. Show the code that is removing the item.
Also, why are you sending a packet every update tick? The Container can sync variables for you via a few methods such as #detectAndSendChanges - that's how the furnace burn time is updated in the client-side GUI, for example. It's better because it only sends packets when needed, e.g. when displaying the information in the GUI. Of course, if you need temperature for rendering the block or something, then yeah, you'll want to send that packet.
-
Might I recommend you ask this on StackOverflow or some Java support forum (or search 'accessing MySQL from Java' on Google)? It's not really related to Minecraft or Forge.
That said, you are opening up a whole can of worms as far as code complexity goes. What are you trying to accomplish by involving a database? Why would you allow clients to write to it? This sounds like the wrong approach to me.
-
Okay, so your problem is that your IGuiHandler code expects the player to be holding the backpack, but you are opening it from a key press so that is not necessarily true. The player might not be holding anything - i.e. NULL - but still have the backpack somewhere in their inventory.
So you have 2 options:
1. Restrict opening of the backpack to when it is in the player's hand by checking that condition when receiving the packet server side. This makes it pointless to use a key press, however, as the player may as well just right-click use the item and open the GUI from there.
2. Loop through the player's inventory again to find the backpack ItemStack instance so you can use it to create the inventory. To avoid looping, you could pass the inventory slot index of the backpack from your packet:
for (i = 0; i < player.inventory.size(); ++i) { if (inventory[i] is backpack item) { player.openGui(MainRegistry.modInstance, message.id, player.worldObj, i, 0, 0); } }
In your IGuiHandler, 'x' will now contain the slot index, so you can easily retrieve the ItemStack you need.
-
It looks like you are passing NULL rather than a valid ItemStack to your IInventory constructor. Show your IGuiHandler class.
Also, you don't need to pass the player's position when opening a GUI unless you need them for some reason; those last 3 parameters are usually used for Block-based GUIs to pass the BlockPos coordinates.
As for checking things on the server side, that's what you do when you handle your packet - recall it was sent from the client to the server and you should know or be able to check which side you are on when you receive it.
Then, just loop through the player's inventory and check each slot for your special inventory item. If you find it, go ahead and open the inventory, otherwise don't. Simple as that.
-
Why aren't you using the SimpleNetworkWrapper?
-
Where did you get your 'player' instance from you use in your condition? You should use the one provided by the Event parameter, like you did when trying to add the item to the inventory.
-
Have a BOOLEAN property for BURNING - check out any of the vanilla toggleable blocks such as doors, levers, pressure plates, etc. All of them have some sort of boolean property. Do the same.
-
While I don't know the answer off-hand, I do know that there have been many threads about this exact topic here that you could probably find the answer in if you searched.
There is some kind of trick to getting a painting to render correctly that isn't really obvious as it is not handled the same way that most other rendering is in Minecraft.
EDIT: Here is a thread with a potential solution.
[1.7.10] Calculations Server Side Only?
in Modder Support
Posted
Your previous code was this:
Well, all you know is that the stack is not null - what if it's a stack of chicken meat? Or dirt? It could be anything, but your inventory constructor is expecting a specific class of Items, specifically YourBackpackItem, and if it gets anything else, you are probably going to crash.