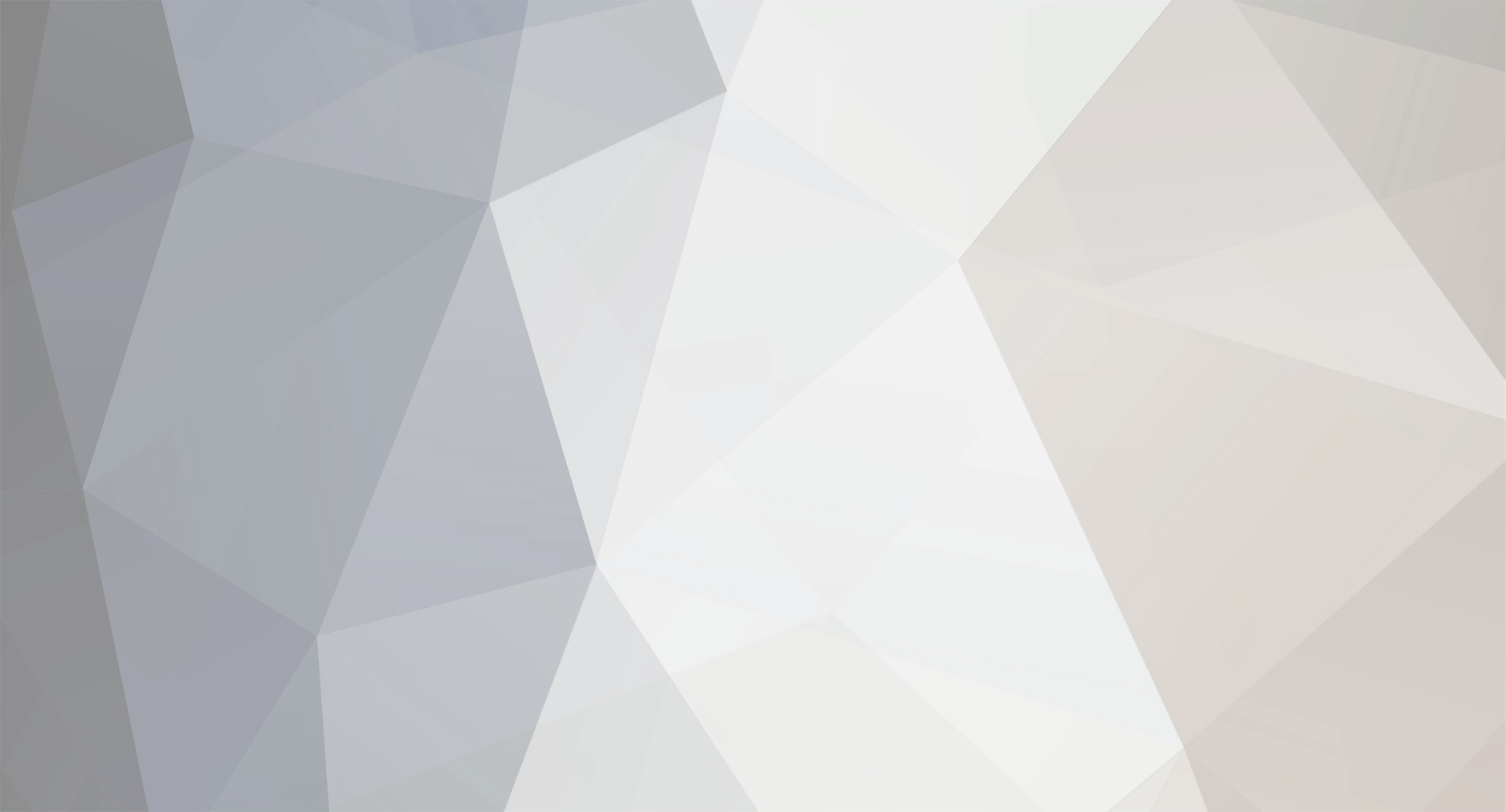
coolAlias
-
Posts
2805 -
Joined
-
Last visited
Posts posted by coolAlias
-
-
You have a return statement inside your for loop, so of course it ends after the first shearable entity. Put return statements last unless you actually want to return right away.
If the return value is important, store it in a variable:
boolean sheared = false; // start with false for (a : b) { if (a is shearable) { sheared = true; } } return sheared; // if even 1 sheep was sheared, this will be true
-
You've got your code backwards:
for (int i = 0; i < shearList.size(); i++) { // recall that 'entity' is passed in the method parameters... but don't you want to check the entity from the list? if (entity instanceof IShearable) { IShearable target = (IShearable)shearList.get(i);
Should be:
for (int i = 0; i < shearList.size(); i++) { Entity target = shearList.get(i); if (target instanceof IShearable) { IShearable shearable = (IShearable) target;
Note that you can also retrieve a list of just IShearable entities directly:
List<IShearable> shearList = player.worldObj.getEntitiesWithinAABB(IShearable.class, player.boundingBox.expand(6.0F, 6.0F, 6.0F)); for (IShearable shearable : shearList) { // now you've already got an IShearable instance }
-
Just to note that other mods may also be listening for the jump event and applying their own modifiers (e.g. jump boost), and their listeners may or may not come before yours. If they come after, you might find that the player is still able to jump, albeit probably not as high as they would have without your event handler.
You can set a Priority level for your event handler; HIGHer priorities are handled first, and LOWer priorities handled last.
@SubscribeEvent(priority=EventPriority.LOWEST) public void onJump(LivingJumpEvent event) { // this handler will now happen after all other non-LOWEST priority handlers }
Generally, you want to leave your priority level alone, but if you disabling player jumping is integral to your mod, you can consider using LOW or LOWEST priority so that your handler has the last say.
-
My first thought would be to subscribe to LivingJumpEvent and, if the jumping entity is a player, set their motionY value to zero.
-
It sounds to me like the center block shouldn't be doing anything at all when neighbor blocks change - it is the one controlling all the other blocks. In fact, I wouldn't do anything for any of the blocks in that method, but instead toggle all the blocks together as one when any of the blocks is toggled.
E.g.
#onBlockActivated { this.toggleDoor(); // this is the method that should actually toggle the door state, right? } #toggleDoor { openState = !block.openState; // get the opposite 'open' state from the current one for (all 9 blocks in the door) { world.setBlockState(block, openState); // set them to have the same exact state: open or closed } }
-
Glad you got it working! Don't forget to mark the thread "solved".
What's with so many people saying this lately?
"Your mod isn't working, I have some kind of crash here" and then the crash report says "invalid block ID".
Keep in mind that the only way you'll ever run out of block IDs is if you have so many mods installed that you have over 4096 blocks... that's a TON of blocks.
While there are certainly some mods that could benefit from consolidating some of their blocks into one, this will only buy you a few more blocks before you run out again. There will always be a limit. I don't think the gain is generally worth it in terms of code maintainability when the blocks being consolidated are completely unrelated. I'd much rather have readable code and a few less mods than the other way around, but I often seem to be a minority in such matters
-
Usually sub-blocks do not include separate tile entities, but things like wool with different colors, or logs, etc. I'm not saying it's impossible as I don't know that to be a fact, but I haven't ever seen it done and it seems like a bad idea, in my opinion.
Saving a block ID is not worth the headache and fugly code caused by trying to have more than one TileEntity in a single Block.
But anyway, opinions aside, it seems like the client-side version of the TileEntity is the wrong class, at least temporarily, causing your game to crash when it first tries to access the icon. If you did an instanceof check before doing any casting, as others have suggested, you could prevent the crash, allowing you to figure out if things work out after that.
E.g.
@Override public IIcon getIcon(IBlockAccess world, int x, int y, int z, int side) { int meta = world.getBlockMetadata(x, y, z); TileEntity te = world.getTileEntity(x, y, z); if (meta > 11) { if (!(te instanceof TileEntityFissionPort)) { // log error - incorrect TileEntity for this metadata // return base block icon } // return icons based on meta and TileEntityFissionPort } else if (meta > 7) { // same as above for other TileEntity } else { // non-TE based icons }
-
I got the same issue too, I followed the tutorial exactly as it said, and it I'm getting errors saying that the MCP mapping is meant for 1.8.8, not 1.8.9 and it also just immediately ends instead of running like it was with 1.8
That's because each MCP mapping is for a specific version of Minecraft - you can't mix and match. The latest MCP mapping (i.e. current date) will probably always be for the latest Forge/Minecraft version, e.g. 1.8.9. If you are wanting to use an older Forge/Minecraft version, just keep turning back the date in your build.gradle's mappings setting until you get one that works.
mappings = "snapshot_20150617" // 1.8 mapping, specified by date rather than a specific MCP release mappings = "stable_20" // specifically released stable 1.8.9 mapping, but you could change to 'snapshot_date' for more recent ones if you want
-
You can't have multiple TileEntities created for a single Block, and I'm pretty sure you can't register multiple tile entity classes to a single Block class (or rather, doing so wouldn't do what you want, as later registrations override the initial registration).
Besides, trying to splice a Block into two different TileEntities based on metadata is ridiculous - whatever conditional functionality you want to achieve can be done in a single TileEntity class, e.g.
// any TileEntity method: if (blockMeta < 7) { // perform duty 1 } else { // perform duty 2 }
However, if the TileEntity's functionality is really different, you probably should consider making 2 different blocks.
-
Can you see a way to override a method so that the final stage has a chance to roll around to the 1st stage?
I would assume the term 'spoil', even in Minecraft terms, doesn't mean returning to seedling stage, but I could be wrong
Crops generally use a metadata-based growing system with the block's age (i.e. metadata, typically using the first 3 of the 4 available bits) incrementing randomly during the block's update tick.
I'm not aware of any global notification that occurs for metadata / state changes, so without introducing your own via ASM or using an existing API that does so as Draco suggested, I don't think it would be possible.
EDIT: Actually, there is the Forge block snapshot system that was added somewhat recently (recently being within the last year or so...). That might be the hook you are looking for.
-
In your block's model JSON, inherit from block orientable and set the front texture to the one you want to face you in the inventory screen:
{ "parent": "minecraft:block/orientable", "textures": { "particle" : "your_mod_id:blocks/block_main", "top": "your_mod_id:blocks/block_top", "side": "your_mod_id:blocks/block_side", "front": "your_mod_id:blocks/block_face" } }
Furthermore, your SOUTH facing in your blockstates file should be the one with no rotation applied:
{ "variants": { "facing=north": { "model": "your_mod_id:your_block_model", "y": 180 }, "facing=south": { "model": "your_mod_id:your_block_model" }, "facing=west": { "model": "your_mod_id:your_block_model", "y": 90 }, "facing=east": { "model": "your_mod_id:your_block_model", "y": 270 } } }
EDIT: I should also mention that the default value for the blockstate's facing is NORTH.
-
Real tests > postulation
Good to know it worked, though I find it somewhat surprising.
-
Sort of, but I was thinking more along the lines of each gas block placed has a chance of spawning a wisp block above/near it, and then the wisp block would remain there until the gas block is 'broken' or a block is set in its (or the wisp's) place.
The wisp TileEntity would be responsible for determining whether or not to render the wisp; when not rendered, it would be invisible / appear the same as air, even though the wisp block is technically still there. I don't see any reason to set and destroy the block over and over - only when you actually want it to be permanently gone should you destroy it, imo.
-
After "this." model is not something that shows up.
That's because you didn't make a class field to store it in... which you would then need to initialize during class construction. If you don't know how to add and initialize class members, please consider reviewing basic Java.
-
You can't just spawn a TileEntity anywhere you want - it is part of the Block, and must be at the same position. You can spawn particles and whatever else anywhere you like, though.
TileEntities take a lot more processing power than regular Blocks and should be used only for blocks that truly need them, so I highly suggest making the wisp its own separate Block+TileEntity so that your swamp gas can be a regular (i.e. non-TileEntity) block. Users of your mod will thank you.
-
Okay, well your render class isn't doing anything. You have to actually tell the model to render, i.e. override #doRender and call 'this.model.render(args...)'.
-
Well, you could use a TileEntity, then, for the wisp, which would do whatever fancy rendering you need in place and also have the ability to affect things on the server via its update tick.
In that case, however, you don't want to really spawn and despawn the TileEntity - your Wisp would be an actual Block that changes state, e.g. one state that is visible and one that is invisible (like air). You should allow other blocks to be placed in this space, just like air.
-
Then show your updated code, especially your render class.
-
1. You don't need an entire class to register an entity - it takes ONE line...
2. Don't use global entity IDs - those are obsolete
3. Where did you get the values '14' and '1' from? The first is tracking range, and the second is update frequency, and neither of those values are anything close to any other projectile. I suggest you use the values '64' and '10' to start with.
EntityRegistry.registerModEntity(EntityBlast.class, "Blast", 1, PinesMod.modInstance, 64, 10, true);
That line can go in your main mod's preInit method and is all you need.
4. I also suggest you extend EntityThrowable instead of Entity, as it takes care of a lot of the work for you.
-
Is it purely for visual effect? Will it ever have to move more than 1-2 blocks' distance? If Yes and No, then make a custom particle (which is a special type of Entity) and spawn that from the Block#randomDisplayTick method.
Whether that will work or not really depends on how, exactly, you want the 'wisp' to behave in game, e.g. whether it can be interacted with or not.
-
Show how you register the entity class (should be EntityRegistry#registerModEntity(...)).
-
You could use the Block#randomDisplayTick (or whatever it's named nowadays) to spawn gas-like particles for your swamp gas block, but as Draco pointed out, neither a Block nor a TileEntity is appropriate for an actual, moving entity such as a Wisp.
-
UUID#fromString and UUID#toString? Or, using long values instead:
// Writing to NBT: compound.setLong("YourUUIDMost", uuid.getMostSignificantBits()); compound.setLong("YourUUIDLeast", uuid.getLeastSignificantBits()); // Recreating from NBT: UUID uuid = new UUID(compound.getLong("YourUUIDMost"), compound.getLong("YourUUIDLeast"));
-
One possible reason for the 'quirks', as you describe it, is that TileEntities also store their BlockPos in NBT, so while calling those methods on the TE is a handy way to transfer data, it also means you may be transferring some data you shouldn't be.
You can probably get around that easily by re-setting the block position after reading the TileEntity from NBT, but there could be other things going on, too.
Also, you probably don't need to mark the block for an update unless you have data in your TileEntity that is required for rendering.
[1.7.10] Potion effect isn't working on armor
in Modder Support
Posted
In other words, you have to apply the potion effect for at least 10 ticks, and preferably not every single tick.
Also note that many potions apply their effect every n ticks where n goes down as the potion level increases, so Regen I may be every 50 ticks, Regen II every 25 ticks, and Regen III every 10 ticks. I didn't pull those numbers from the code, but you can suss out the actual values by looking in the potion classes and calculating them based on the fun formula there. Hope you know how bit operators work
If you don't want to allow the Regen potion to have a long duration but still be effective, you could consider foregoing potion effects altogether and just healing the player directly every so often in the armor tick. E.g.: