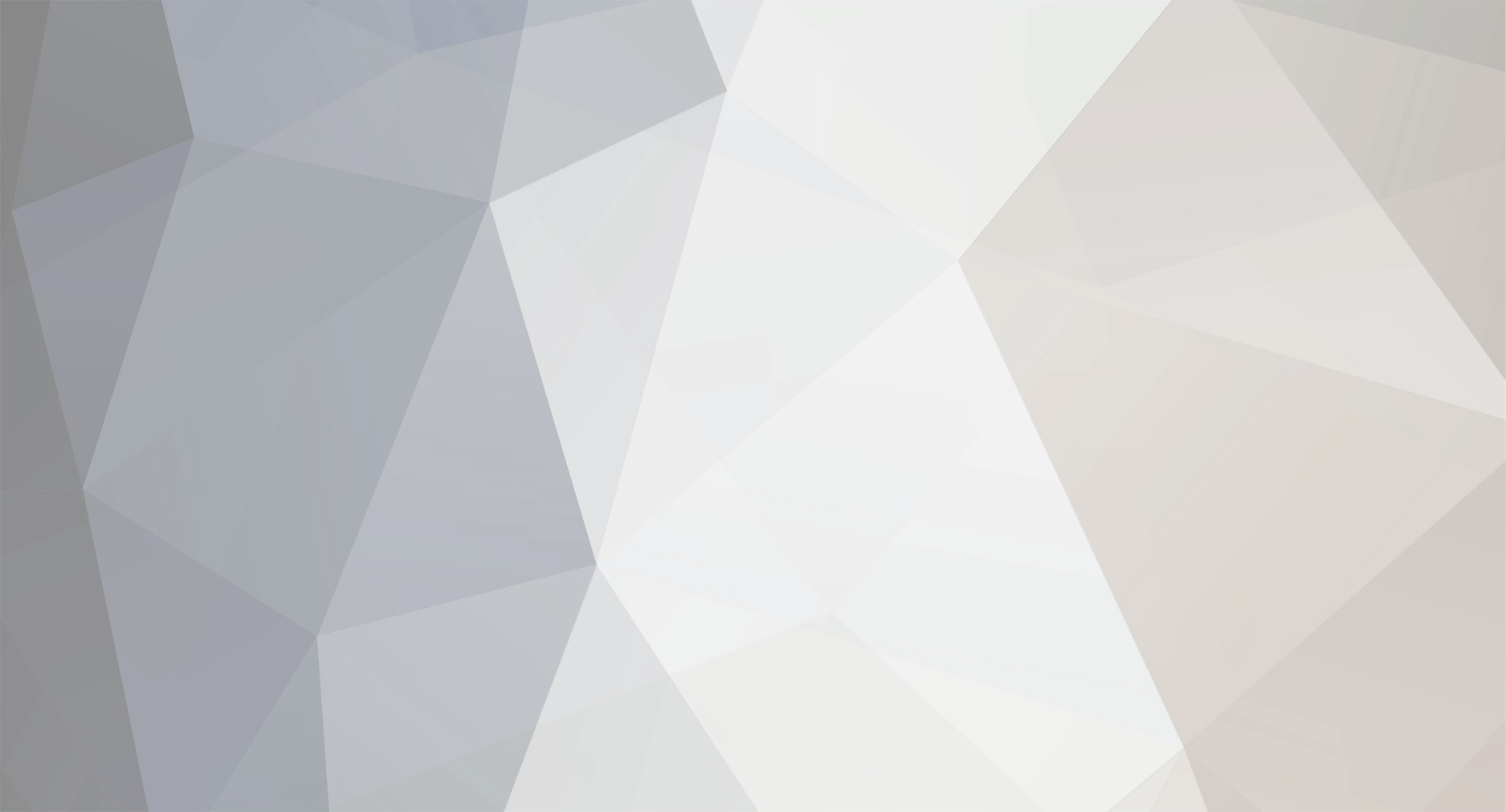
coolAlias
Members-
Posts
2805 -
Joined
-
Last visited
Everything posted by coolAlias
-
[1.8][Solved] Event Handlers, adding chicken tempt item
coolAlias replied to Cleverpanda's topic in Modder Support
Yes, that's exactly right - AI tasks typically (always? I can't think of any counterexamples) only process on the server, so only add it there. -
If using 1.9+, you should be using the Capability system instead of interacting with ItemStack NBT directly. The reason you need to use NBT / Capabilities is indeed that unlike TileEntities but similar to Blocks, Items do not have unique instances so you cannot store any mutable data in the class.
-
No, super#onUpdate goes in the overridden #onUpdate method in your Entity class. You need to call it because the method does TONS of stuff in the super classes, and by not calling it you are preventing your Entity from working properly. Note also that the super method automatically calls #onEntityUpdate - why are you overriding that method instead of just putting your logic in #onUpdate (before or after calling super)?
-
How Do I Dispense A Custom Throwable Entity? (1.10.2)
coolAlias replied to gurujive's topic in Modder Support
Already showed you - you just have to check and see if it still works in 1.10.2. If it doesn't, I bet BlockDispenser will have something similar, or you can use your IDE to find where other dispenser behaviors are registered. BlockDispenser.dispenseBehaviorRegistry.putObject(yourItem, new YourCustomDispenserBehavior()); BUT, you can't do that until you have non-abstract versions of your classes. I still don't see where you register your projectile entity? Am I correct in assuming that e.g. EntityFireOrb extends your abstract EntityOrb class? Note: I'm not going to download random files from you, sorry. Post code using Pastebin, Gist, or create an online repository such as with GitHub. -
Renderers should only be registered in the ClientProxy - you mentioned you did so in your CommonProxy. What version of Minecraft are you modding for? Show your entity registration code for v2. You should at least be calling super#onEntityUpdate if not also super#onUpdate. Lots of important things happen there...
-
How Do I Dispense A Custom Throwable Entity? (1.10.2)
coolAlias replied to gurujive's topic in Modder Support
Well you are making all of your classes abstract... do you know what that means? That means you can't actually instantiate them, i.e. you can't use them the way you seem to think you can, unless somewhere else you are actually making a concrete class implementation? Show your concrete class implementations for the Entity AND the dispenser behavior. Show where you register your Entity class. Show where your register your dispenser behavior. Show where you register your Entity's Render factory. All of those things must be done or it won't work at all - this has nothing to do with 1.10.2 vs. 1.8 or other versions - you have do do all of those things regardless of version, they're just done slightly differently. -
How Do I Dispense A Custom Throwable Entity? (1.10.2)
coolAlias replied to gurujive's topic in Modder Support
You need to actually make a real projectile class, i.e. not an abstract one. Easiest way is to extend EntityThrowable and override #onImpact to do what you want; remember to register the entity and entity renderer classes. Then in your dispenser behavior implementation, create a new YourThrowableEntity instance and spawn it. And yes, you have to register your dispenser behavior - the following was in 1.8, probably something similar these days: BlockDispenser.dispenseBehaviorRegistry.putObject(yourItem, new YourCustomDispenserBehavior()); -
You can undo the effects of being pushed by water by making a method to reverse everything done in the handleMaterialAcceleration method (I think that's what it's called anyway) and call your reversal method each applicable tick. The only way I've found to improve walking speed while walking underwater is to check server side if the player is in water and send a packet to the client; on the client, check if player#onGround is true and multiply their motion values by about 1.25 (maybe a little less). If this is going to be a constant effect for all players, then you could probably forgo the packet and just give them all that bonus when #onGround and in water directly on the client side.
-
[1.7.10] [SOLVED] Eclipse crashes when I run my mod
coolAlias replied to 10forever's topic in Modder Support
Yeah, you can remove new Object[]{} and just keep the parameters, e.g.: GameRegistry.addRecipe(new ItemStack(itemTable), "WWW"," W "," W ",'W', Blocks.planks); -
[SOLVED][1.10.2] Is Inventory full & drop the item.
coolAlias replied to Oniro's topic in Modder Support
You didn't quite follow what diesieben said: you need to drop the returned stack, if any: // toDrop is whatever remains of PotatoStack after trying to add it to the inventory ItemStack toDrop = ItemHandlerHelper.insertItem(item, PotatoStack, false); if (toDrop != null) { // if it's not null, drop it playerIn.dropItem(toDrop, false); } -
[1.7.10] [SOLVED] Eclipse crashes when I run my mod
coolAlias replied to 10forever's topic in Modder Support
It's way easier to provide assistance if you post the code mentioned in the crash report, i.e. at tntdiamond.RegidMod.RegidMod.init(RegidMod.java:145) line 145 of that class. Another helpful thing is to post your entire class using gist or pastebin with line numbers - that way we can easily find the problem. -
[SOLVED][1.10.2] Is Inventory full & drop the item.
coolAlias replied to Oniro's topic in Modder Support
In previous versions, you could do this: if (!player.inventory.addItemStackToInventory(stack)) { player.dropPlayerItemWithRandomChoice(stack, false); } I'd bet there are the same or similar methods still. -
Teleporting into an unloaded chunk? 1.8
coolAlias replied to Captain_Admiral's topic in Modder Support
Thanks It's gotten pretty outdated by now since my computer turned into a toaster about 5-6 months ago, but I'm hoping to get back into it when I scrounge up enough for a new one. -
If you have multiple fields that need to be synced to the client (e.g. mana + some other thing to display on the HUD), I'd create one packet for each of them to send when they change individually, and one packet that syncs all of them for when the player joins the world. This way you aren't sending a bunch of extra data that hasn't changed when you don't need to. Of course if you only have 2 integers or some such it won't make much of a difference, but the network traffic can start to add up as the amount of data you need to send becomes more and more.
-
Minecraft.getMinecraft().thePlayer - but really you should make a proxy method that fetches the player entity given the current context / side, and your ClientProxy would return the above.
-
That's because you call #readInt() twice, but #writeInt() only once. They must be exactly the same. Removing your System.out or swapping it to after you read and using the actual field instead of another call to #readInt() will fix it.
-
In other words, as I suspected, you have to send a packet - you can't just change mana values on the client. This is true whether you use Capabilities or not. Key press to activate some magic skill -> send packet to server saying 'hey this key was pressed' -> server decides what to do e.g. consume mana and activate skill -> server then notifies client of whatever it needs to know e.g. new current mana amount so mana bar can display properly.
-
Teleporting into an unloaded chunk? 1.8
coolAlias replied to Captain_Admiral's topic in Modder Support
This was my attempt at forcing nearby client players to recognize that a new entity has popped into existence near them, but it doesn't seem to work 100% of the time. However, if the entity doesn't render right away, it does after a few seconds. As for entities teleporting into unloaded chunks, you will have to first load the chunk, teleport to it, and then mark the chunk as needing to save to disk. You should be able to do this even if there aren't any players in that chunk. -
Show where you are consuming mana from, e.g. an Item class or something.
-
Sounds to me like you are only consuming mana client-side, so it appears to work via the mana bar, but the server side values are not actually changing and those are the ones that get saved and loaded via NBT. This is relevant whether you use the outdated IEEP or update to use the newer Capability system.
-
It's one of those things that I've yet to see an elegant and problem-free solution for. You could try adding one or more boolean flags per player and attempt to figure out what the value of #allowFlying should be for other mods, then delay setting it to false until the next tick to make sure you don't do so preemptively. The logic can get kind of messy, but it should be doable.
-
Teleporting into an unloaded chunk? 1.8
coolAlias replied to Captain_Admiral's topic in Modder Support
First of all, why do you send a packet to the client? If you set the player's position on the server, the client will be notified automatically. See here, but you'll need to update some of the method naameters probably if you are 1.9 or up. 1.9 or up. Also note that the packet I send is just to play a sound client side, nothing else. -
Isn't that what Ernio showed via PlayerEvent.Clone?