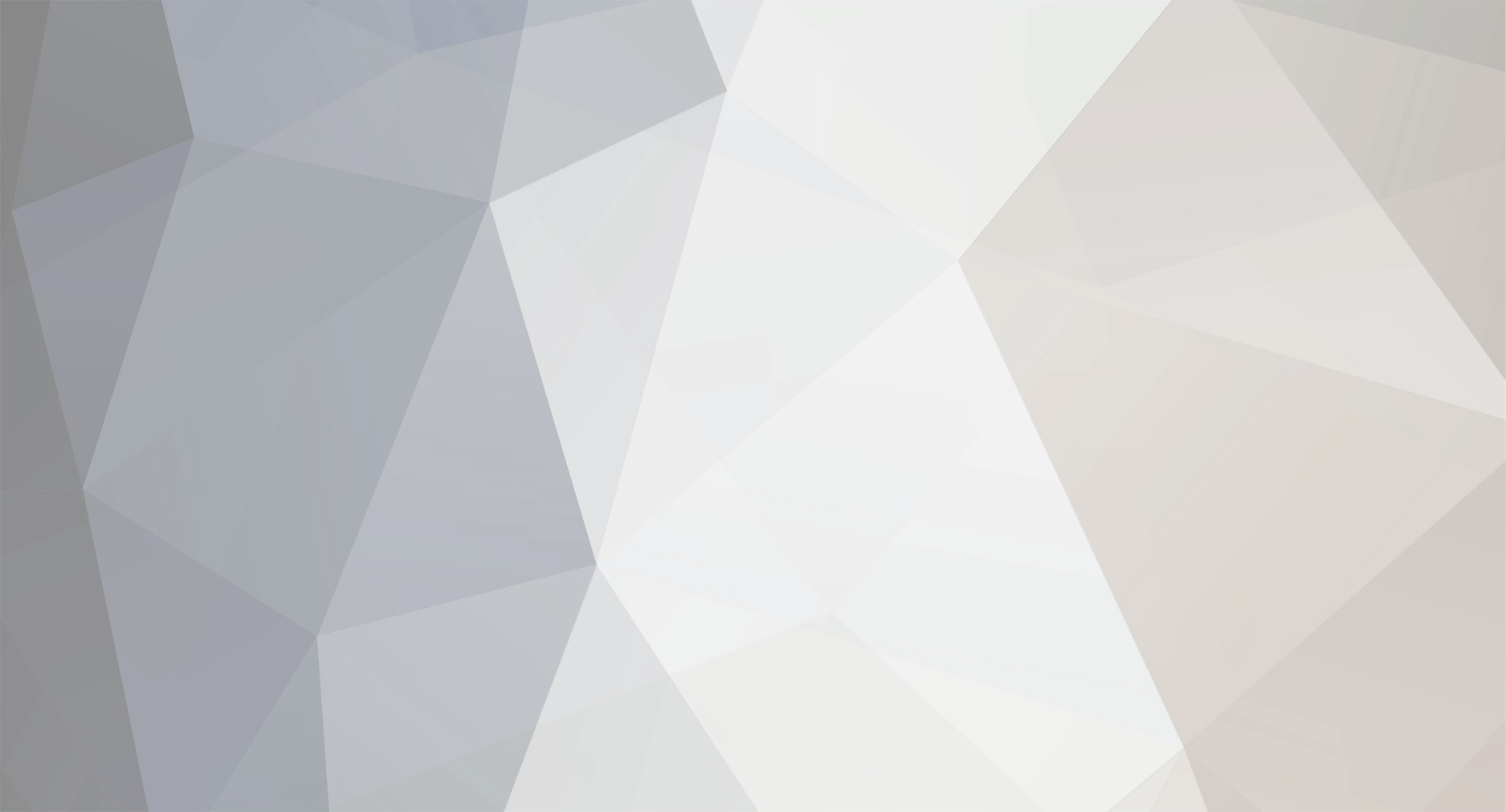
coolAlias
-
Posts
2805 -
Joined
-
Last visited
Posts posted by coolAlias
-
-
Blocks are singletons, so you cannot store any state information in the block class - if you change the color of one block, you've changed the color for ALL of those blocks. To have independent block information, you need to use metadata (if possible values are between 0 and 15) or a TileEntity. In your case, since you are storing color, you will want a TileEntity.
Then you can return the color value from the TE for the Block using getColorMultiplier:
@Override @SideOnly(Side.CLIENT) public int colorMultiplier(IBlockAccess world, BlockPos pos, int renderPass) { TileEntity te = world.getTileEntity(te); if (te instanceof YourTileEntity) { return ((YourTileEntity) te).getCustomColorMultiplier(renderPass); } return super.colorMultiplier(world, pos, renderPass); }
Doesn't have a side argument, but you can look around in the Block class and see if you can find any color methods that take both a BlockPos (to get the TileEntity) and the EnumFacing (for the side).
-
How, in the game, would a player specify the recipient of their waypoint? The answer will determine what kind of method you should use.
As for servers, I've seen some people still making client-side only mods, but most must be installed on both the server and the client to function properly. Again, the answer really depends on the specifics of your mod.
-
1. World#getBiomeGenForCoords(BlockPos)
2. That's the only way I know of.
3. All things are possible, but why would you do that? Are you planning to completely regenerate the chunk(s)?
-
Given the fact that when you were setting blocks client side you said that it resulted in the correct pattern, you could send a packet from the client to the server with the player's current client-side position information, then the server should be able to figure out what to do from there.
If that doesn't work, you could instead send the block position(s) that need to be changed, but it's not generally recommended to let the client dictate any sort of state, but if you really don't want a checkerboard pattern, it might be the only solution.
Also, when setting blocks, instead of using getStateFromMeta(0), you should use getDefaultState().
-
It mentions S3FPacketCustomPayload - are you sending any of those and, if so, can you show us the code for it?
EDIT: larsgerrits' answer is much more likely the cause.
-
There are several tutorials on that subject (use Google); surely they can help you more effectively than someone writing snippets of information post by post.
-
@diesieben Except he's not trying to get a field in the class, but additional data set by another mod. Whether the other mod uses IEEP, getEntityData(), or some other method of adding this data is unknown.
@OP You need to ask the mod author how they are adding the data, because that determines how you will retrieve it:
1. IEEP would be something like: ModsIeepClass.get(player).getSubspecies()
2. getEntityData() would be just as you have it in your code above, but you need to make sure you have the tag key exact - "Subspecies" and "subspecies" are not the same, for example
3. Somehow written to the entity's actual NBT compound, which is a non-trivial exercise and not very likely that the mod author took this route - if they did, you should ask them to use IEEP instead, but you can test by doing this:
NBTTagCompound tag = new NBTTagCompound(); entity.writeToNBT(tag); // This will print out all the entry keys in the entity's NBT tag: for (Object o : tag.getKeySet()) { System.out.println((String) o); } // Once you find which one you want, you can use it: int subspecies = tag.getInteger("Subspecies");
Again, that last method is VERY unlikely, and if that is indeed what the modder used, it's also very hacky, but that's how you would use it if that's the case.
-
1. EntityPlayer#getItemInUse can be null, and always will be in your case (can't be using an item while in a GUI)
2. ItemStack#getTagCompound can be null
3. You can NOT set NBT data on the client - you must send a packet to the server
-
In Java and probably every language with classes, you need an instance of the class to access a non-static class field, because the field is different for every single class (that's the whole point). E.g.
public class Example { public int value; public Example(int value) { this.value = value; } } Example a = new Example(1); Example b = new Example(2); System.out.println("A = " + a.value + " | B = " + b.value);
That's what I mean by 'you need an instance of the class' - both 'a' and 'b' are specific instances of Example, and thus each can have an independent value for 'this.value', whereas if you make 'value' static, the value will NOT be independent: 'a' and 'b' cannot have different values.
Don't you have a class field to store an instance of your inventory? That's what you need to use to access the contents of the inventory, because nothing else makes sense.
And that's why I've been so adamant that you spend some time learning about this stuff. These are not really Minecraft questions - they are Java questions.
-
Ahem... no.markBlockForUpdate causes Block#updateTick to be called the next tick
Er, I meant scheduleUpdate (which can schedule a number of ticks in the future, not just the next one)...
-
would it be a good idea to put my gui handler like you have it or keep it as a separate class
Simpler is better, in my opinion. I don't see any reason to make your IGuiHandler as complicated as you have unless you have a LOT of guis, and even then it might not be worth it.
If you consolidate it into one class, it's much easier for both you and us to quickly see what's going on, and then you can also put all of your gui IDs in there, getting rid of your GuiRefs class (or whatever it was called, don't remember).
But it's totally up to you - the way you have it set up is not technically wrong, just unnecessarily complex.
-
markBlockForUpdate scheduleUpdate causes Block#updateTick to be called some number of ticks in the future, and from there you can perform the same logic or even call the neighbor method with whatever block you want.
EDIT: markBlockForUpdate results in the TileEntity sending its description packet to all nearby client players.
Although now that I think about it, you can just call whatever method you want on your block directly from your TileEntity. And saying that, if your Block/TileEntity are implemented properly, it should already be giving off the correct redstone signal without you having to do anything... can you show where you set and return the redstone power?
As for the packet, you don't have to use ByteBufUtils for everything: ByteBuf can read and write most values directly.
read(ByteBuf buffer) { int i = buffer.readInt(); float f = buffer.readFloat(); // etc. }
-
You can send as much information as you want (within reason) in a packet, and it is better to send one with 2 fields than 2 with 1 field if both pieces of information are always sent at the same time.
You can force a block to update at any time by marking it:
worldObj.markBlockForUpdate(pos);
-
When in the world gen process is your 'place' method being called? I've generated lots of chests and none of them have had this problem (at least that I've found / heard so far).
Aside: why are you using world.getChunkFromChunkCoords to check the block at the position instead of the world method directly? I.e. world.getBlockState(pos).getBlock().
Speaking of block states, can you show your DBlock.placeChest method? Perhaps something is going awry in there?
-
That's what I've been trying to tell you - you GUI is CLIENT side, and the CLIENT side does NOT know about the values in your TileEntity by default.
You need to send a packet from your Gui to the server saying "the player pressed button X, now what?" and let the server process everything, since TileEntity data should only ever be set on the server side (setting it client side does nothing, in other words).
If the player can set the frequency as well, you need to send that data in the packet.
-
And where is that method called from? How do you know the frequency is always 0, are you printing it out?
-
Here's your problem right here:
Minecraft.getMinecraft().theWorld
Minecraft is CLIENT side only.
Also, you shouldn't try to store the loaded TileEntities in your class; instead, whenever you need them, get the list from the current world object (every TE has a worldObj, btw).
-
Okay, you are trying to access your IEEP data on the CLIENT side - have you sent the data you need to from the server to the client?
-
I'm pretty sure that all of the TileEntities in loadedTileEntitiesList should have their correct data as loaded from NBT, but I've never actually used it. Perhaps you are not saving / loading your TileEntity properly? Are you using the exact same string to load as you do to save?
It would be simpler if you had a Github repository or something that I could look at online, or post your TileEntity, Gui, and IMessage classes on pastebin.
-
Where are you calling the method from? Show code.
-
Maybe!
The problem is, that the frequency in this TileEntities loaded by "world.loadedTileEntityList" is always 0. Maybe it's 0 because the readFromNBT()-method won't be executed at the time. So this method is not a option I think. I really don't know how I could do this.
How are you setting the frequency in these TileEntities? You mentioned a GUI - are you sending packets to the server? If not, that's why you always get 0...
-
Is this not sufficient?
World#loadedTileEntityList
-
I see you cleaned up the rest of your code, so that's good, but did you remove the static modifier that diesieben pointed out yet?
-
Are you modding for 1.8? If so, modded TileEntity data is destroyed by default, rather than preserved, unless you override #shouldRefresh in your TileEntity class to return a sane value (i.e. return the same condition that causes vanilla TileEntities to refresh, rather than always true). See here for more information.
If the TileEntities are not your own and you are trying to 'tack on' information, then it would probably be easiest to store a list of controlled block coordinates / positions in YOUR TileEntity and let your TE save / load that data. So if your TE controls a block at 1/1/1 and 3/3/3, it saves those coordinates and when the game loads back up, it knows to check those blocks.
Be warned that this kind of system does not scale well - if you plan on having your TE control thousands of blocks at once, well, good luck.
[1.8] Sending packets
in Modder Support
Posted
You can send anything via packets, as underneath it is just a stream of bytes.
Look at the outline for the ByteBuf class to see the methods available to you and it should be pretty obvious how you can write other data types. I don't see how you're going to make this mod client-side only, though.