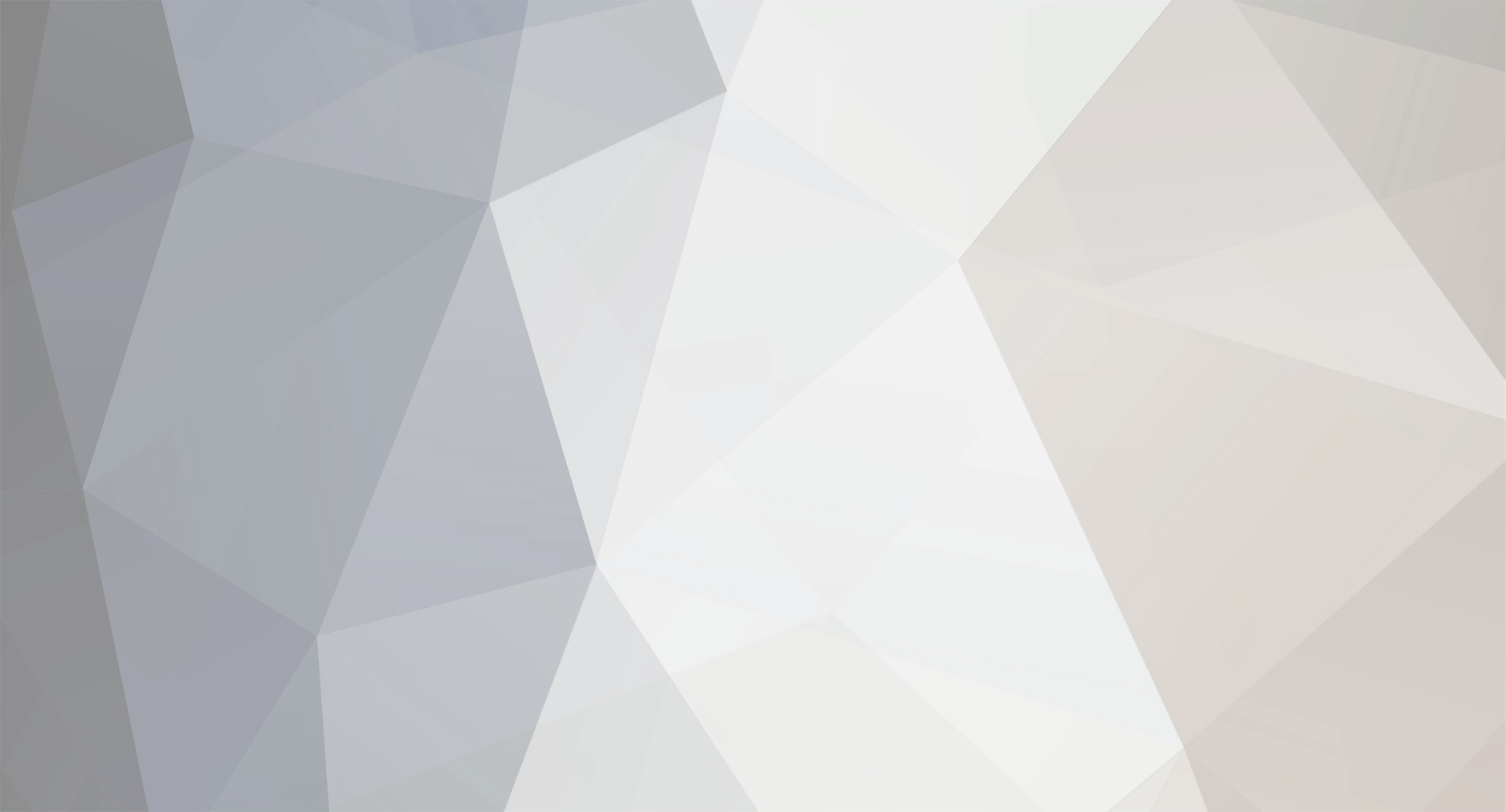
coolAlias
-
Posts
2805 -
Joined
-
Last visited
Posts posted by coolAlias
-
-
Your item is never setInUse, so the ticksInUse is always zero. Look again at ItemBow#onItemRightClick.
-
As SanAndreas mentioned, you MUST check if the entity is a player before proceeding:
if (entity instanceof EntityPlayer && !world.isRemote)
That won't fix your problem, but will prevent crashes later on.
What is going on in your super.onUpdate method? Because that call is pointless if it only ends up calling Item#onUpdate, which does nothing.
Finally, what exactly is the problem you are having? The potion effect is not getting applied at all, or you are not able to have the swing animation?
If you are not able to swing the shovel, can you post your entire shovel class again? It may also have something to do with applying the digSpeed potion specifically (wild guess, but worth a try) - maybe try using a different potion effect like regen that doesn't affect the shovel as its trying to dig.
-
Part of the problem, at least in the code you posted, is your logic is wrong. If thirdPersonView resolves to 'true', the view is 3rd person, not first.
However, as you've found, that will not work for inside the inventory as thirdPersonView can be false but in the inventory view it should be using 3rd person no matter what, and you are breaking that by returning models from there.
The solution is to use IPerspectiveAwareModel, so that your model class itself can handle it - #handlePerspective returns a pair of the model to use and the transformations required.
You can store references to multiple models easily in the class:
private final IBakedModel baseModel; private final IBakedModel emptyModel; public ModelItemBomb(IBakedModel baseModel) { bombModel = new ModelBomb(); this.baseModel = baseModel; ModelResourceLocation resource = new ModelResourceLocation(ModInfo.ID + ":empty", "inventory"); this.emptyModel = Minecraft.getMinecraft().getRenderItem().getItemModelMesher().getModelManager().getModel(resource); if (emptyModel == null) { ZSSMain.logger.warn("Failed to retrieve model for resource location: " + resource); } }
-
Edit: Congrats
I'll leave the rest of my comment anyway.
Well your first bit of code in your last post shouldn't even compile - you have an extra closing curly brace that ends the method before you return anything.
player.isSneaking() returns a boolean value - it doesn't actually do anything. The only use for it is to check if the player is sneaking or not, and for that it must be in an if statement.
In your last code snippet, what are you trying to do? The way it is written, the item is only consumed if the player is NOT sneaking, because if isSneaking returns true, the rest of the condition is ignored. You may want to review basic logic operators and their uses - especially the examples later on down that page may prove instructive.
-
onItemRightClick replaces whatever item was clicked with the stack that is returned - return the new stack that you want instead of the original one.
-
No, it doesn't cause that, but you should reference the item you want directly, rather than relying on ids which can vary from game to game. Item 373 could be something completely different when I load up Minecraft vs. when you do.
Anyway, your problem is that you have mixed up the item damage and the item stack size arguments in ItemStack - switch the potion id to the 3rd argument.
-
Does attackEntityFrom() work on the client-side?
Yes and no - you can use it, but it won't have any real effect. If you want to attack the entity for real (i.e. cause it to take damage), you must do so on the server.
-
You can't reference a client class in the server jar, so if your 'clientPlayer' reference in your main class extends EntityPlayerSP, right there is your problem - you have to put that kind of stuff in your ClientProxy and NEVER reference it directly unless you are in an @SideOnly(Side.CLIENT) class already.
-
how do you remove an item stack from an inventory?
How is that related to this topic?
-
You should NOT be using Item.getItemById, especially in this case since you clearly know exactly what item you want: Items.potionitem.
That aside, how do you know your potions are getting replaced? Did you put a breakpoint in / log all items generated, or did you just generate a few chests and look at their contents in game?
-
I'm doing this for what basically amounts to a helmet of anti-zombie laser vision, so I need to be able to do it from the armor tick method. Any suggestions?
If you only want to display some information (i.e. client side), the easiest way is to use Minecraft.getMinecraft().objectMouseOver, then check if the entityHit is not null and there you go.
Server side is a bit more complicated - ray trace only works for blocks, so you have to do some math using the player's look vector; you can pretty much copy the code from EntityRenderer#getMouseOver.
Are those ^^^ not suggestions?
-
If you only want to display some information (i.e. client side), the easiest way is to use Minecraft.getMinecraft().objectMouseOver, then check if the entityHit is not null and there you go.
Server side is a bit more complicated - ray trace only works for blocks, so you have to do some math using the player's look vector; you can pretty much copy the code from EntityRenderer#getMouseOver.
-
Show your code.
Methods you will need:
EntityLivingBase#getHealth()
EntityLivingBase#attackEntityFrom(DamageSource, float)
EntityPlayer#inventory#addItemStackToInventory(ItemStack)
-
That's because, contrary to all expectation, Entity#getBoundingBox returns NULL. Don't use it. Instead, use Entity#getEntityBoundingBox, which actually returns the entity's bounding box.
-
You misunderstood my statement. I said you "initiate" the spawn. That does not mean you use any world spawn method. I mean you use your own code to send a packet to all the clients.
This is the confusion -- the impetus for spawning particles should be on the server. The actual instance of the particles is only created on the clients.
There is no reason that particle spawning must be initiated on the server - it is one of the few cases where it is perfectly fine to do it only on the client. The times when you do initiate it on the server are typically when a. the trigger happens there, such as when a villager is struck, or b. you want the particles visible to all players and it is not run in a method that happens consistently client side.
Things like Block#randomDisplayTick or any update tick method that happens on the client (such as for spawning critical particles for the EntityArrow) are perfectly valid places to initiate particle spawning and need never go through the server.
-
Yes, it is possible. Take a look at TheGreyGhost's MinecraftByExample project - I'm pretty sure there is at least one example that renders multiple models.
-
You'll need to check every AI task that your entity has to make sure it does not affect its current rotation, as well as make sure that the rotation is the same on both server and client.
Your best bet it is probably to output the current rotationYaw each tick in different update methods (updateEntityActionState, etc.) and see where it is changing from.
-
There is always a way, but at some point you are going to need to discover these things on your own. The way you do that is by breaking the problem down into smaller parts:
1. When does the entity attack? When the AI determines it should attack
2. Okay, look at the attack AI that you currently have - ranged, okay, well that calls #attackEntityWithRangedAttack or whatever
3. Now you need to STOP it from attacking with a ranged attack once in a while, how can you do that? Maybe by returning early from the method mentioned in #2
4. How about melee attack? When do you want that to happen, when it collides with a player, like a zombie? So add that AI
5. Repeat from step 1 but substitute the melee AI in the questions and answers
With a little bit of Java knowledge and good logic / problem solving skills, you can do quite a lot, but you really need to practice your skills to improve, and by practice, I mean try to solve problems on your own, get frustrated, keep trying and trying until you either solve it or can't take it anymore, then try again.
Only after you have really put in a solid effort (measured in at least hours if not days) should you seek help.
-
I don't know about a .json cuboid specifically, but you can most certainly still bind textures - just look at any vanilla Entity render class.
-
Before attempting any kind of animating, it is very important that your model is correct. If any rotation points are off or pieces of the model are acting independently (i.e. not added as children of a parent part), then you are just making the task 1000x more difficult than it should be.
If your model is all set up correctly, then be aware that the axis of rotation is also affected by the model itself - if you have a box that is rotated naturally by some amount, the yaw/roll/pitch axes are relative to the box's rotation, not necessarily that of the entity.
-
I'm not sure what your MiscTools#areItemStacksEqual implementation is like (or why you would even need one when the ItemStack class itself already offers several ways to check equality), but if your headgear can be damaged, I highly doubt it will ever return true.
Not only that, but unless your item requires stack damage or NBT to determine equality, you are wasting a lot of effort.
// this monstrosity: MiscTools.areItemStacksEqual(new ItemStack(Minecraft.getMinecraft().thePlayer.getEquipmentInSlot(4).getItem()), new ItemStack(UnLogicII.crystal_eye_headband) // should be rewriten as: Minecraft.getMinecraft().thePlayer.getEquipmentInSlot(4).getItem() == UnLogicII.crystal_eye_headband
Also, why not store a reference to Minecraft, either in your class or locally in the method, so you don't have such long-winded statements?
Finally, this:
byte i = 0; if(i < 40){ i++; } else {
What are you expecting to happen there? You initialize i to zero EVERY SINGLE TIME the method is called, so i++ is completely pointless and your 'else' statement is never called.
-
I thought I would be clever and make a workaround, but for some reason it fails miserably:
@Override public float getExplosionResistance(World world, BlockPos pos, Entity entity, Explosion explosion) { IBlockState state = world.getBlockState(pos); if (state.getBlock() != this && entity != null) { // reverse the insanity caused by Mojang: state = world.getBlockState(pos.add(0, -entity.getEyeHeight(), 0)); } // Return resistance for block at the original position if still not the correct block: if (state.getBlock() != this) { return world.getBlockState(pos).getBlock().getExplosionResistance(world, pos, entity, explosion); } // now the state should be the correct block, but often still isn't! return ((SomeProperty) state.getProperty(SOMETHING)).someValue(); }
As mentioned, that does not always result in the correct block. Not only that, but even if it is the correct block, it is in no way guaranteed to be the correct state, or perhaps it is that the position added for the block in the Explosion class is affected and causes a different outcome than expected later.
Block#getExplosionResistance should only be called once per block position from Explosion#doExplosionA(), either directly or passed through Entity#getExplosionResistance, but you can see below that each position is called many times PER explosion, some even as many as ~10 or more times. Here is the log output from a single creeper explosion:
[spoier]
[21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-488, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-488, y=69, z=-271} is tile.air // HERE, for example, this block position is checked 3 times in a row (not sure why), and recall that this ONLY prints if MY block's // getExplosionResistance method was called, meaning that neither the initial nor the adjusted position was actually my block! [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-488, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-488, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-488, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-488, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-488, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-488, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-488, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-488, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-488, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-488, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-488, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-488, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-488, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-488, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-488, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-488, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-488, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-488, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-488, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-488, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-269} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-269} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-269} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-269} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-269} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-269} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-488, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-488, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-488, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-488, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-488, y=71, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-488, y=69, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-269} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-269} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-269} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-269} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-269} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-269} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-269} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-269} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-269} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-269} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-488, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-488, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-488, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-488, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-488, y=71, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-488, y=69, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-269} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-269} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-269} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-269} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-269} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-269} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-269} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-269} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-488, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-488, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-488, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-488, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-488, y=71, z=-272} is tile.chest_invisible [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-488, y=69, z=-272} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-488, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-488, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-488, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-488, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-488, y=71, z=-272} is tile.chest_invisible [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-488, y=69, z=-272} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-488, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-488, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-488, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-488, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-272} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-272} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-272} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-272} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-272} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-272} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-272} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-272} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-272} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-272} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-488, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-488, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-488, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-488, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-272} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-272} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-272} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-272} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-272} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-272} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-272} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-272} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-272} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-272} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-488, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-488, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-488, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-488, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-272} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-272} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-272} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-272} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-272} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-272} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-272} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-272} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-272} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-272} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-272} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-272} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-272} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-272} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-488, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-488, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-488, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-488, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-272} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-272} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-272} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-272} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-272} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-272} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-272} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-272} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-488, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-488, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-488, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-488, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-272} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-272} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-488, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-488, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-488, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-488, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-488, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-488, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-488, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-488, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-488, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-488, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-488, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-488, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-488, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-488, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-488, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-488, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-488, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-488, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-488, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-488, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-488, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-488, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-488, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-488, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-269} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-269} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-488, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-488, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-488, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-488, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-488, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-488, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-488, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-488, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-488, y=71, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-488, y=69, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-269} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-269} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-269} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-269} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-269} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-269} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-488, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-488, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-488, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-488, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-488, y=71, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-488, y=69, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-269} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-269} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-269} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-269} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-269} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-269} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-269} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-269} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-488, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-488, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-488, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-488, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-488, y=71, z=-272} is tile.chest_invisible [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-488, y=69, z=-272} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-488, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-488, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-488, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-488, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-488, y=71, z=-272} is tile.chest_invisible [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-488, y=69, z=-272} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-488, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-488, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-488, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-488, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-272} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-272} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-272} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-272} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-272} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-272} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-272} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-272} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-272} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-272} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-272} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-272} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-488, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-488, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-488, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-488, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-272} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-272} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-272} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-272} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-272} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-272} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-272} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-272} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-272} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-272} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-272} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-272} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-488, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-488, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-488, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-488, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-272} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-272} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-272} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-272} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-272} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-272} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-272} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-272} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-272} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-272} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-272} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-272} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-488, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-488, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-488, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-488, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-272} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-272} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-272} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-272} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-272} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-272} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-272} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-272} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-488, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-488, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-488, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-488, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-272} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-272} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-488, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-488, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-488, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-488, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-488, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-488, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-488, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-488, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-488, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-488, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-488, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-488, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-488, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-488, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-488, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-488, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-488, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-488, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-488, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-488, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-488, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-488, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-488, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-488, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-488, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-488, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-488, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-488, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-269} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-269} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-269} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-269} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-488, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-488, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-488, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-488, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-488, y=71, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-488, y=69, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-488, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-488, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-488, y=71, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-488, y=69, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-488, y=71, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-488, y=69, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-487, y=71, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-487, y=69, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-269} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-269} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-269} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-269} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-269} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-269} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=71, z=-269} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=69, z=-269} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-485, y=74, z=-272} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-485, y=72, z=-272} is tile.secret_stone [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-485, y=74, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-485, y=72, z=-271} is tile.secret_stone [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-485, y=74, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-485, y=72, z=-271} is tile.secret_stone [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-485, y=74, z=-269} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-485, y=72, z=-269} is tile.secret_stone [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-485, y=74, z=-272} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-485, y=72, z=-272} is tile.secret_stone [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-485, y=74, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-485, y=72, z=-271} is tile.secret_stone [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-485, y=74, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-485, y=72, z=-271} is tile.secret_stone [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-485, y=74, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-485, y=72, z=-270} is tile.secret_stone [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-485, y=74, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-485, y=72, z=-270} is tile.secret_stone [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-486, y=74, z=-273} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-486, y=72, z=-273} is tile.secret_stone [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-485, y=74, z=-272} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-485, y=72, z=-272} is tile.secret_stone [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-485, y=74, z=-271} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-485, y=72, z=-271} is tile.secret_stone [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-485, y=74, z=-270} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-485, y=72, z=-270} is tile.secret_stone [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-485, y=74, z=-269} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-485, y=72, z=-269} is tile.secret_stone [21:03:00] [server thread/WARN] [zeldaswordskills]: Wrong block! Block at BlockPos{x=-485, y=74, z=-269} is tile.air [21:03:00] [server thread/WARN] [zeldaswordskills]: Block at adjusted position BlockPos{x=-485, y=72, z=-269} is tile.secret_stone
I was pretty confident the above solution would work, but obviously it's not good enough (or even useful at all, really). I also tried using ceil() on the eye height to make sure it got full block positions with the same result as above; maybe I should try floor() instead.
Anyway, I've re-added the hack I used in 1.7.2 when a similar bug was present that caused my supposedly indestructible blocks to be destroyed by explosions, except in the current case it is specific to creature-based explosions such as the creeper; inanimate explosions such as TNT work properly.
Hack to prevent blocks from getting destroyed that shouldn't be (varies somewhat based on the specific block, but you get the idea):
@Override public void onBlockExploded(World world, BlockPos pos, Explosion explosion) { // only allow block to be exploded if it is not unbreakable if (!((Boolean) world.getBlockState(pos).getValue(UNBREAKABLE)).booleanValue()) { super.onBlockExploded(world, pos, explosion); } }
-
Don't tick - use a 'long' to save the next time it will become active, and when the player tries to interact with it, check if the current world time has surpassed the stored time and you're golden.
Unless, of course, you need your block to reset immediately when it next becomes available (visually, for example), then you will unfortunately need to tick, but you can still use the world time implementation so you don't have to increment / decrement a counter.
-
Your instructors couldn't, or wouldn't? If they really couldn't, that's pretty sad...
BlockFurnace uses a static method (which you can't override) that is called from TileEntityFurnace's onUpdate method - you have to override the TE method to call your own static method instead, which would then set the block state to YOUR active / inactive block, rather than the vanilla one.
There is a lot of stuff going on in those classes, though, so what I would recommend is to start off with a simple block in 1.8, get it rendering and all of that, then follow a 1.7.10 tutorial on furnaces to get it going as a furnace with custom recipes, as all of that is basically the same.
Problems with Crops
in Modder Support
Posted
It does seem dumb, but there is in fact a very good reason for it:
The block constructor is called before the item is initialized, so the 'reference' you pass to your constructor is 'null', which doesn't point to anything. Then later, when your item gets initialized to an actual object, the block has no way to know because the reference that you stored there is still 'null', whereas if you don't store the reference at all but use the actual item reference from your main class, that reference will always be correct when it is needed.
Kind of unintuitive, but from the computer's perspective, it is very logical.