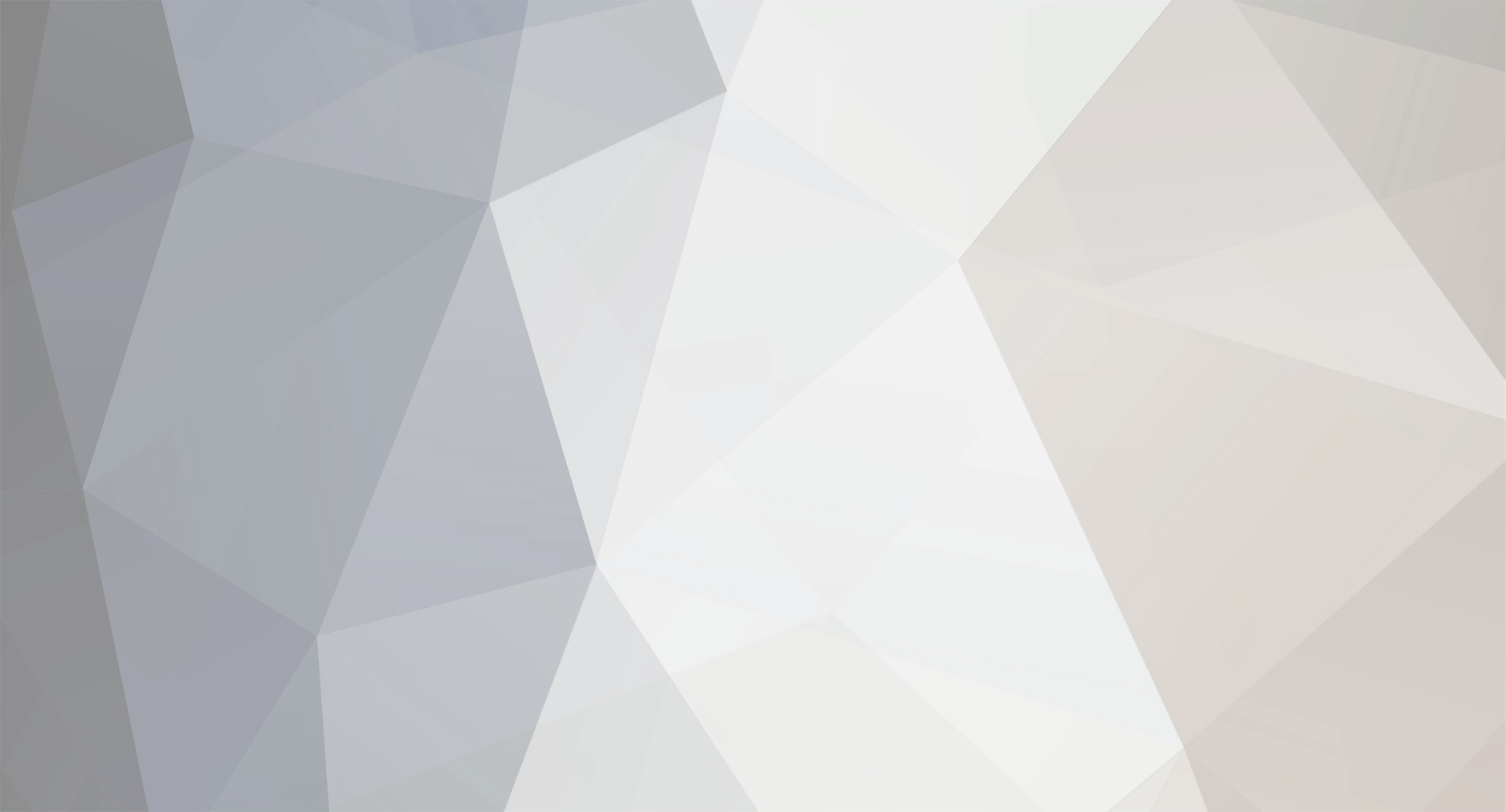
coolAlias
-
Posts
2805 -
Joined
-
Last visited
Posts posted by coolAlias
-
-
No, arrays are zero-indexed, and go up to but not including the size: int[] array = new int[16] has 16 elements, from 0 to 15. array[16] will give you an out of bounds exception.
-
Shouldn't you be using 16 instead of 15? Chunks are 16x16, using index 0 to 15, so it seems you are excluding a line of blocks on two sides of the chunk. Similarly, the y axis should use 256 instead of 255.
-
Isn't what's in that method required in 1.8?
I don't see what my item/block classes have to do with anything, but here:
Yes, the contents of the method are required, but you already have your 'render' method (poorly named, btw - it is not 'rendering' anything, it is registering resources to be used later in rendering), so why have that method if all it does is call another method? Just put the code in one method, and call that.
Your Block class especially is important because you may have had different IBlockStates, in which case your .json file would have been wrong.
Anyway, your .json files all look correct - TheGreyGhost's troubleshooting guide is quite comprehensive; if you can't find what you need in there, read it again. Seriously. It describes 99.99% of all texture troubles.
If that still doesn't help, update to the latest version of Forge, start Minecraft, and look again at the console output - there should most definitely be something in there describing the problem.
-
World#isDaytime() can tell you if it's night or day, and World#getWorldTime() gives you the current time in ticks, so divide that by 24000 and you've got the number of days spent in game.
Then there is World#getCurrentDate which gives you a java.util.Calendar object - this gives you the real date (as in from real life) and is used by certain mobs to do things on Halloween and such.
Finally, there is World#getMoonPhase which sort of gives you a rough idea of what time of the month it is, in game.
-
Well first of all, you need to make sure that you actually set the data BEFORE you spawn the entity. I notice only one of your constructors sets the metadata value - is that the one you are using when creating your entity?
Second, why are you messing around with the ByteBuf capacity and current index? Don't do that - just write what you need, and read back what you need in the same order.
public void writeSpawnData(ByteBuf buffer) { buffer.writeInt(this.metadata); } public void readSpawnData(ByteBuf buffer) { this.metadata = buffer.readInt(); }
That's it. You should never mess with the writer or reader index unless you really know what you're doing.
-
Is it a reloading animation? What will you do if the player switches to a different item, or no item at all?
If it is a reloading animation, I would tackle it a different way: store more information in the ItemStack's NBT tag about the current state of the gun, so that if it needs to be reloaded, any time the player switches to it it attempts to reload, which results in your 20 second animation, and if the player doesn't wait until the process finishes (i.e. switches to a different item), then the process must start again when the player switches back to the gun.
-
You need to show your Block and Item classes. And what's with the 'public static void rr()' method? Seems completely pointless.
-
Stop right there - why in the world do you have '@SideOnly(Side.CLIENT)' in your ChaosLabyrinth class?!?!?!?
Please read diesieben's excellent explanation.
-
If you only want to handle the player update tick and no other entities, you should use PlayerTickEvent (on the FML bus) rather than LivingUpdateEvent - the latter is fired for every single living entity and is overkill just to update the player.
-
You can ONLY ever access client input (keyboard, mouse, etc.) on the CLIENT side - ANY attempt to do so on the server, i.e. when the world is not remote, will crash your game.
This includes things like inline initializations:
@SideOnly(Side.CLIENT) public KeyBinding reloadKey = new KeyBinding("Reload", keyReload, "key.categories.fallout");
It doesn't matter that you put @SideOnly there, because that can only remove the reference, but you also try to assign it and that part is not removed, thus it crashes.
You CAN, however, put the @SideOnly annotation above your entire KeyBinding class, and that's what you should do, as well as make sure you only ever reference it on the client side.
-
Edit: Nevermind, after wondering why there was no chunkY in the event it finally dawned on me that chunks actually take up the whole Y and aren't 16 blocks like x and z.
At least until Cubic Chunks is implemented
[ like that will ever happen... but it would be great]
You can see an example of WorldSavedData here, but it is far more complicated than you would need. All you are doing is setting a single value 'generated' or not.
This is how I use the class during generation - you can see I'm using an instance of a class that ensures each time a structure is generated, it calls my WorldSavedData class to update the NBT and marks the tag as dirty so it gets saved the next time the world saves.
-
You're just generating a structure, not replacing an entire chunk, right? All you have to do is use setBlockState on the positions you want to change.
For the saved data class, you literally create a class that extends WorldSavedData. Then you create an instance of that class somewhere and use that to check if the structure has generated or not, and when you do generate it, you tell that class that it has generated.
-
You can easily spawn it once per world: create a class implementing WorldSavedData, this will let you save whether your structure has generated or not.
Subscribe to any of the generation events, such as PopulateChunkEvent.Post, to generate your structure during world generation.
-
Your issue is that you are not actually passing in the custom player inventory, only the vanilla inventory.
Your code should look like this:
return new ContainerCustomPlayer(player, player.inventory, ExtendedPlayer.get(player).inventory);
Note the last parameter is the custom inventory, which you need in your Container class to add the custom inventory slots:
// add one slot to the container for every slot in your custom inventory: addSlotToContainer(new SlotCustom(inventoryCustom, 0, 80, );
-
Loops by themselves won't make it that much better, though Java can optimize them pretty well.
If you are going to be setting the exact same IBlockState dozens of times, you could make a reference to it instead of recomputing it every time:
IBlockState chaos = ChaosBlock.blockChaosUnbreakable.getDefaultState(); world.setBlockState(x, y, z, chaos);
Again, that won't really save you too much computation time unless you are doing very abnormal things in your Block#getDefaultState().
How many blocks are you setting? You should easily be able to do several thousand without much lag, but if it is in the tens of thousands, you may just have to accept that it will be laggy.
How are you calling the generation code? Perhaps that has something to do with it.
Another thing to note: you should NOT be using Block.getBlockById when generating structures - use the actual Block reference.
-
Some users have reported crashes when a creeper explodes next to one of my blocks, which has the following code:
@Override public float getExplosionResistance(World world, BlockPos pos, Entity entity, Explosion explosion) { return (((Boolean) world.getBlockState(pos).getValue(UNBREAKABLE)).booleanValue() ? BlockWeight.getMaxResistance() : getExplosionResistance(entity)); }
Now, this method is called from Explosion, which calls Entity#getExplosionResistance using the IBlockState found at the original position, yet the Entity method inexplicably adds the entity's eye height to the block position before calling the original blockstate's block's method:
// Entity#getExplosionResistance: return blockStateIn.getBlock().getExplosionResistance(worldIn, pos.add(0, getEyeHeight(), 0), this, explosionIn);
Of course I know how to fix it for my own blocks, but my question is, why the heck would it be coded this way in the first place?
If a Block method is called with a BlockPos or an IBlockState parameter, I've come to expect that the block at that position / state is the one being called. Is this just another muck-up by Mojang, or are should we code expecting ANY Block method to be passed block states not corresponding to the block? That seems like a lot of lame 'if (state.getBlock() != this) return' statements that will need to be added if that is the case.
-
It really depends on the algorithm you use to decide how to place those blocks. Simply setting blockstates, even across 4 chunks, should not cause the game to freeze, but if you are doing lots of preprocessing to determine whether the block can be set at that location, or your for loops are written poorly, then you can easily case the game to freeze.
Another consideration is lighting: if you are generating a structure high in the sky, each setBlockState will cause the chunk to recalculate light unless you tell it not to - there was another thread about this in the last couple weeks that you may find interesting. I'll link to it later if I can find it.
At any rate, you should post your code on pastebin so we can see what you have attempted.
-
That looks like it should be saving and loading correctly - are you providing a proper server-side gui element, i.e. a Container, when opening your gui? Is that container using the player's custom inventory field?
Show your IGuiHandler code as well as your Container constructor.
-
To do that, I believe I need the door's BlockPos. How do I get the door's BlockPos from within the onNeighbourBlockChange method?
The BlockPos given in the method parameter is the position of the current block, not the neighbor. I suggest you take a look at the BlockDoor code and see how they handle it.
-
To shoot on right-click, you will need to use Events, specifically the MouseEvent and KeyInputEvent (to handle keyUseItem in the case that it is bound to a key other than the mouse).
Both of those events are CLIENT only, meaning you will have to send a packet to the server letting it know that the player right-clicked; from there, perform all necessary checks (e.g. is player riding mech? can he shoot this tick? etc.) and spawn the bullet based on decisions you make in response to Ernio's reply.
Just be aware that if you have never used Events or Packets before, it can be a daunting task as it seems far more complex at first than it actually is. Once you get the hang of them, they really are quite simple.
I followed the event tutorial, but what event should I use? I can't use the interact event because that would shoot a bullet every time I mounted the mob.
I have now put the events needed in bold. Please read more carefully in the future.
-
First you need to know which way your door is facing - BlockDoor has a property for this that you can get by accessing your block's state. Once you know the door's facing, you can use BlockPos#offset(facing.getOpposite()) to get the block behind the door and check if that is the one giving it power.
Keep in mind that the state storing the door's facing is in the block comprising the lower half of the door.
-
To shoot on right-click, you will need to use Events, specifically the MouseEvent and KeyInputEvent (to handle keyUseItem in the case that it is bound to a key other than the mouse).
Both of those events are CLIENT only, meaning you will have to send a packet to the server letting it know that the player right-clicked; from there, perform all necessary checks (e.g. is player riding mech? can he shoot this tick? etc.) and spawn the bullet based on decisions you make in response to Ernio's reply.
Just be aware that if you have never used Events or Packets before, it can be a daunting task as it seems far more complex at first than it actually is. Once you get the hang of them, they really are quite simple.
-
I like to name mine 'RenderNothing'
@SideOnly(Side.CLIENT) public class RenderNothing extends Render { public RenderNothing() {} @Override public void doRender(Entity entity, double x, double y, double z, float yaw, float partialTick) {} @Override protected ResourceLocation getEntityTexture(Entity entity) { return null; } }
Register this renderer instead of RenderItemSnowball and voilá, nothing renders
-
Break your problem down into smaller problems, and describe it in more detail.
Do you want the player to shoot the bullet, or the mech?
Can the mech shoot on its own, or does it only fire when controlled by a player?
Do you really want the player to have to be holding a certain item to shoot while riding the mech, or would you rather it just happens when the player right clicks like they entered some commands on the mech's control panel?
Always explain EXACTLY what you want to happen and how you want it to happen so people can help you more effectively.
[1.7.10][1.8]Save a block to be called later
in Modder Support
Posted
This isn't a good idea for several reasons:
1. Blocks (and Items) cannot reliably be reproduced from the ID alone, meaning that if you only store the Block (or Item), you are potentially missing a lot of other information such as the block state (metadata or ItemStack damage), tile entity information (or ItemStack NBT), etc.
2. If you store it in a static field, it is not per-player but per-world, which is fine if that's your intention.
3. What's the point of storing this information? Will you need to save this information somewhere?
But, if you really want to do what you described, then you can convert from an item to a block using the static Block method #getBlockFromItem, and vice versa using Item#getItemFromBlock.