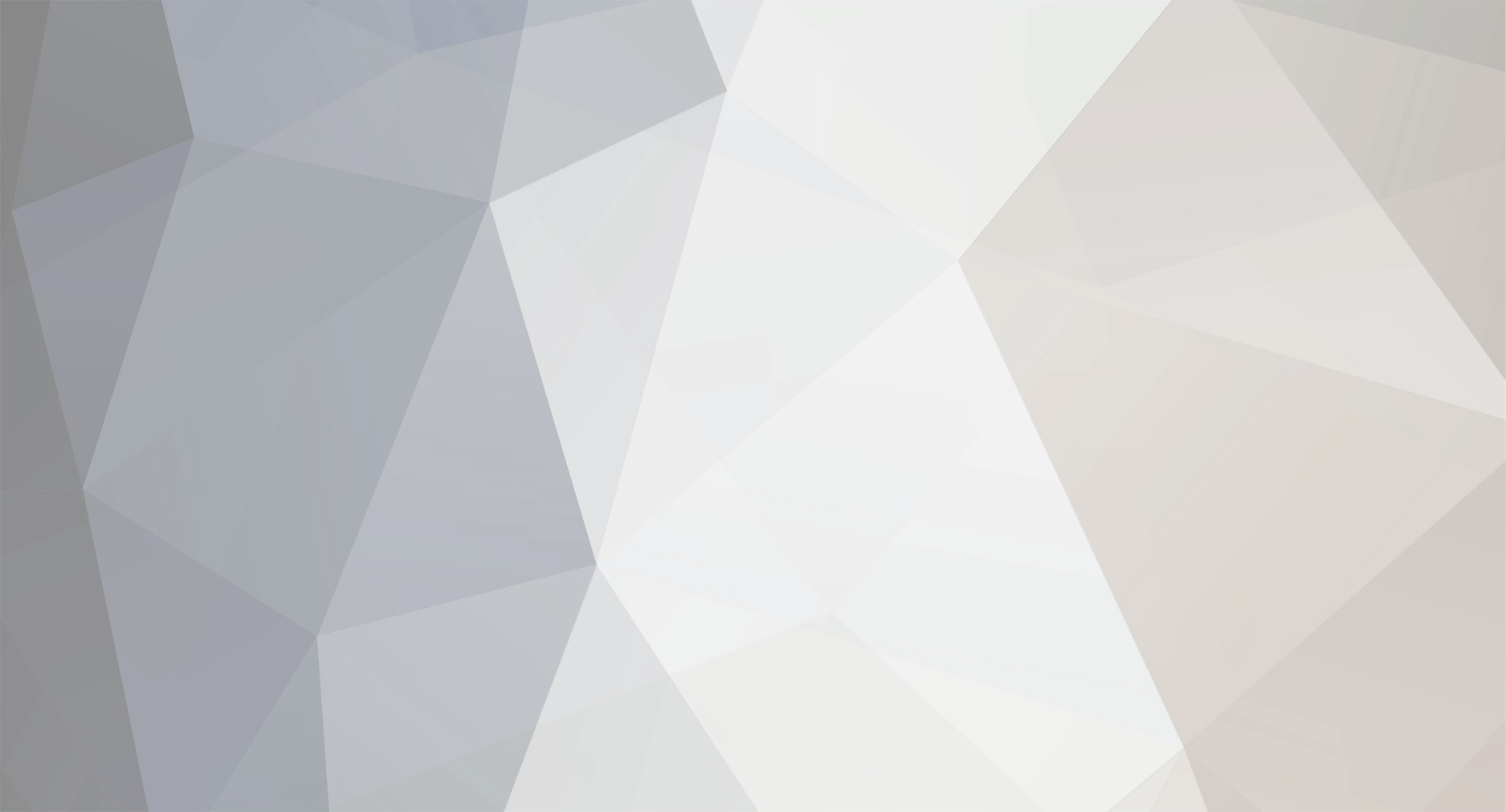
coolAlias
-
Posts
2805 -
Joined
-
Last visited
Posts posted by coolAlias
-
-
It's not package.info but package-info.java.
Oops, so it is! Maybe I should get some reading glasses
-
Depends - is your 'evolution stage' or whatever stored in DataWatcher?
If so, the client already knows about it and you can just set the bounding box on both sides.
If not, then you'll need to tell the client what stage the entity is at so it can set the bounding box.
One way to do that is custom packets; another is to use worldObj.setEntityState(this, some_byte_flag) which automatically sends a packet for you and that packet calls your entity's handleHealthUpdate(some_byte_flag) method. This is the method wolves and other animals use to spawn hearts when in love, villagers use to spawn various particles, and even golems use this to initiate their attack animation.
So you could define some byte flags, e.g. FIRST_STAGE = 5, FINAL_STAGE = 6, and send those via the entity state. Just take a look at every class that your entity inherits from to make sure you are not duplicating any byte flags. Won't cause any critical bugs if you don't (unlike DataWatcher indices), but it's better to check.
-
Only import what you need for your code to compile.
You may also want to include a package-info.java file within any packages that contain API files - this is a specially formatted file that Forge / FML uses to detect packages that should be loaded first, so they are available to all mods which may need them. As such, it is important not to put any non-API files in such packages.
This is all the package-info.java file should contain:
@API(owner = "yourmodid", provides = "YourModAPI", apiVersion = "1.0") package yourmod.path.to.your.api.package; import net.minecraftforge.fml.common.API;
-
Um, you should really look at the vanilla code that sets up the initial entity bounding box. The last 3 parameters are the max X/Y/Z, but you have them at 0, almost like your box is somehow inverted into the 4th dimension or something.
Point is, read vanilla code for examples.
-
Oh, I bet they switch, but unless you inform the client side as well, you won't see any update in the visible bounding box.
-
What about this
this.setEntityBoundingBox(new AxisAlignedBB(x1, y1, z1, x2, y2, z2));
Ok, yes, that technically gives it a rectangle with different x and z, but it won't rotate, which is what I was trying to get at but didn't explain.
You end up with a long rectangular bounding box, but when your entity turns, it's now mostly outside of the bounding box because the entity turns, but the box does not, make sense?
-
Alrighty, I fixed it after doing some research on what I was doing wrong. And I have I think my final question, how do I extend the entity bounding box on the Z axis?
There is no way (in Minecraft, without heroic coding skills) to specifically extend the bounding box along one axis only, as bounding boxes are defined by only 2 variables: height, and width, centered around the entity.
The width is used for both the x and z axis, making a square, with the height being the y axis turning it into a rectangle. Nothing you can do to make x and z different.
-
Use a TileEntity.
-
There will not and can not ever really be a tutorial on making an API, because an API is just code, and is always specific to whatever it is designed for.
There is nothing special about 'making an API' - it's simply a way for other programs / mods to interact with yours, in whatever way you specify.
So if I want to allow other mods to add their items as weapons in my mod, I create a 'registerWeapon(Item item)' method that specifies 'if you call this method with your Item, it will for all intents and purposes be considered a weapon in my mod', whatever that is supposed to mean.
That's all it is, which is why there are no (or few) tutorials.
-
Here is some information about how others might go about using your API, whether shipped separately or included in your mod.
I don't think there is actually a need anymore to create a specially deobfuscated version - you should be able to simply drop the compiled .jar into your /eclipse/libs or /eclipse/mods folder, drop the source code next to it (so it's not in YOUR src folder), and then link the .jar to the .src from within your IDE.
If you run gradlew eclipse, it should even automatically add the dependency to your project's build path; if not, you can include it manually by configuring the build path and adding it, which lets you (or another modder) access the API class files from within your own project.
Also, I think Jabelar's point is worth emphasizing: use your API in your own code. If you are not using your own API, chances are either your mod design has some major issues, or your API is not worth using. Obviously this is not always the case, but it very often is.
-
So if I should use "item.getUnlocalizedName().substring(5)" to get the unlocalized name, then what should I do? Just type in the name myself?
I have no idea - I frequently use getUnlocalizedName just like that to avoid typos and having to edit multiple locations in my code if I ever change a name.
@Lex (or anyone else who may know) - what is so egregiously incorrect about using the unlocalized name in place of hard-coding a texture or other name? I, too, am interested in what a better alternative would be, as well as what makes it "wrong".
With the paucity of any sort of usage guidelines, we modders are mostly left to come up with our own solutions, many of which may indeed have issues of which we are unaware due to our lack of understanding all of the internal workings of Forge and Minecraft.
With that in mind, please help educate instead of simply stating something is wrong.
-
Casing matters, and first and final warning Jedispencer DO NOT USE UNLOCALIZED NAME like that.
It's kind of a shame you edited his post, as now no one else can see what NOT to do.
@OP Look in your console for warning / error messages related to the texture path - more often than not, it tells you exactly what it looked for and couldn't find, and you can then go to that folder and see if your texture is there and named exactly the same.
As others have said, upper / lower case matters, with the convention being that texture names (and all your .json blockstates, models, etc.) should be ALL lower-case.
-
There are lots of examples, hints, guides, and troubleshooting steps that have been put together by TGG.
Not a bad place to start your foray into 1.8 if you're feeling lost.
-
Wait, I can use the same renderer for multiple models?
That's what I've been trying to tell you this whole time. Pass or create as many models as you want in your renderer constructor, then in the actual doRender method, switch to whatever model you want based on the entity's current state.
E.g.
public class YourRenderClass extends RenderLiving { private final ModelBase model1, model2, model3; public YourRenderClass(whatever arguments you want) { super(whatever arguments are needed); model1 = arg1; // assign from the arguments model2 = new ModelWhatever(); // or create new ones directly } public void doRender(EntityLiving entity, other arguments) { int state = ((YourEntity) entity).getState(); if (state == 1) { this.mainModel = model1; } else { this.mainModel = model2. } super.doRender(args); }
That's all there is to it, at its most fundamental level.
-
And is it possible to switch the mainModel by the entity state?
That is what he just said you could do, and I have said before.
It is really not difficult, but for some reason you just cannot seem to understand the words that we write. Is it because English is not your native language? Is it because you do not understand Java? A combination of the two? Something else altogether?
Whatever it is, please stop spamming post after post and actually READ the replies that you have already received. All of the information you need has already been provided - it is now up to you to read, understand, and use that information.
-
Sorry, that got lost amongst the many many code blocks and multiple times skimming through.
Anyway, TGG has a troubleshooting guide that may help you.
-
Do you not see this in your console?
[21:09:20] [Client thread/ERROR] [FML]: Model definition for location dmvp:red_sandstone_pillar#normal not found[21:09:20] [Client thread/ERROR] [FML]: Model definition for location dmvp:stonebrick_pillar#normal not found[21:09:20] [Client thread/ERROR] [FML]: Model definition for location dmvp:stonebrick_pillar_mossy#normal not found[21:09:20] [Client thread/ERROR] [FML]: Model definition for location dmvp:stonebrick_pillar_cracked#normal not found[21:09:20] [Client thread/ERROR] [FML]: Model definition for location dmvp:sandstone_pillar#normal not found
That is exactly the type of message I'm talking about. Your model .jsons are either in the wrong file, or you have them wrong in your blockstates .json.
-
stupid question? because I haven't figured it out yet.
It's not a stupid question, but it's one that you should easily be able to figure out with basic Java knowledge and basic understanding of Minecraft:
1. Your entity class ultimately inherits from Entity (duh)
2. All entities have a method (several, actually) that is called automatically every tick: #onUpdate, #onLivingUpdate, etc.
3. All classes can contain per-instance class fields
With that knowledge right there, you have what you need to create a timer - literally a decrementing or incrementing integer and one or two conditional statements.
If you're having trouble with something that basic (which you are), then I highly encourage you to take a break from modding just for a bit and actually study Java.
-
And did you read your console output? Because you have a ';' in your .json file which clearly isn't a valid texture name and would inform you of such in the console.
-
Note for others:
NEVER return "false" in all cases - it will cause client's world to not remove TE in given place, even when server removes it.
While it is NOT unsafe (something like ghost block) and will not cause errors (TE will get removed on world-reload), it might cause unwanted client actions (e.g particle spawning).
That's what the notes say, but all vanilla TileEntities return false by default, so why are modded TEs not automatically removed client-side when removed server-side, and what are we supposed to do about it in those cases where we must return false?
In fact, I just return false by default because 1. that's what vanilla does, and 2. 99% of the time that's the behavior I expect my TE to perform, i.e. not destroy itself and lose all data whenever the block changes state.
-
IExtendedBlockState, via Block#getExtendedState, is designed to address these sorts of issues. You can store as many block states as you need this way using a TileEntity.
-
In your blockstates.json file, you need to specify your modid with the resource location in the same format that you did in your other .json files, i.e. "model": "dmpv:stonebrick_pillar_top;" - note that 'dmpv:' is with the texture name, and not with 'model'.
In the future, all you need to do is look in your console output for errors of this nature - it will give you error messages explaining which texture / resource it could not find as well as other useful information.
-
Any clue where that list of (yeah) events are at?
So I can go there and browse and choose what I want?
In the Forge source. Learn to browse the packages, classes, and code - it is all there.
-
That's it - create a method 'public void whatever(EventYouWant)' and then register whatever class it's in to the correct bus. It's important that the event is the ONLY argument for the method.
[1.7.10]Spawning Particles
in Modder Support
Posted
Velocity simply means motion - i.e. the direction(s) in which the particle will be moving. Each is further modified (randomly) based on the particles type, so you can start with all zeroes and see what it looks like, then go from there.
Experimentation is key to getting particle motions looking right, and learning how they work.