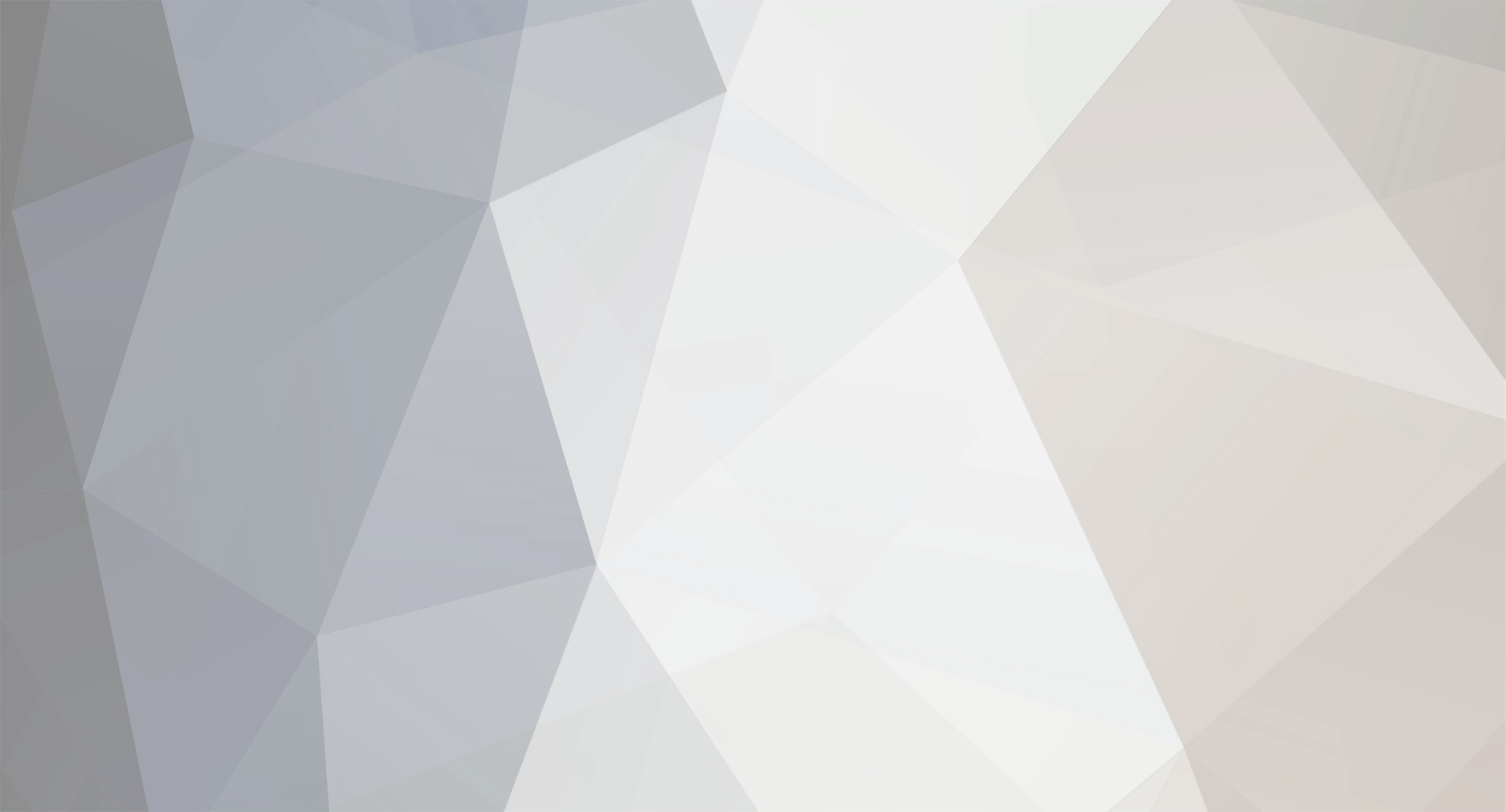
coolAlias
Members-
Posts
2805 -
Joined
-
Last visited
Everything posted by coolAlias
-
Whenever asking for help with code, you should always post the code you are using - otherwise we cannot help you beyond vague guesses at what you may be doing incorrectly.
-
To add a tooltip to your own item classes, you can override the addInformation method and add Strings to the List given. To dynamically add tooltips to vanilla items, you need to use the Forge ItemTooltipEvent (see how to use Forge Events). You could probably use the RenderBlockOverlayEvent to render your in-game display above certain blocks, though I have never personally used it so I don't know what it does exactly beyond what it's documentation says.
-
Also, if you bothered to read the event that you are using or check the type of 'item', you'll see it is an EntityItem, not an Item. Change your code accordingly.
-
Set player.isFlying to false as well.
-
Requesting assistance with packet implementation.(SOLVED)
coolAlias replied to Cyan's topic in Modder Support
Can you just show us whatever your TestScreen class is? Is it an Entity? Is that where the setNickname method resides? It's really very simple: 1. Send the entity ID and the name (CHECK - provided you got the correct entity ID) 2. In onMessage, get the instance of your entity from the world, which we told you how to do 3. Cast the entity to your custom class and call its setNickname method Much of this is just plain old basic Java; step 2 is: Entity entity = ctx.getServerHandler().playerEntity.worldObj.getEntityByID(message.entityid); -
He's asking why do you need to send a packet from the client requesting information? What exactly are you trying to accomplish? Typically, the server already knows what's going on and can send information directly to the client when needed, such as when a GUI is opened, meaning you are making your code too complicated. What information do you need, where do you need it, and when do you need it?
-
No, don't use @SideOnly; in the method parameters for onItemRightClick, there is a 'World' object, named 'world' -> use that to check side: if (world.isRemote) { // you are on the client right now } // if (!world.isRemote) means you are on the server For NBT tags, they are stored in the ItemStack already, not something you add to your class. Use them like this: // 'stack' is the ItemStack from the method parameters, and this goes inside the method // always check if the stack has a tag compound before you try to use it: if (!stack.hasTagCompound()) { stack.setTagCompound(new NBTTagCompound()); } if (!world.isRemote) { // on the server // there are lots of different 'set' methods for NBTTagCompounds // each tag is stored with a String key, such as 'isGlowing': boolean isGlowing = stack.getTagCompound().getBoolean("isGlowing"); // now store the opposite: stack.getTagCompound().setBoolean("isGlowing", !isGlowing); } } Then in your hasEffect(ItemStack, int) method, you can return 'stack.hasTagCompound() && stack.getTagCompound().getBoolean("isGlowing")'
-
Requesting assistance with packet implementation.(SOLVED)
coolAlias replied to Cyan's topic in Modder Support
What kind of Entity is it? If it's a TileEntity, send the x/y/zCoord in the message, and you can then get the tile entity from the player's world object; if it's an Entity entity, send the entity.getEntityId() in the message, and then use the player's world object again to getEntityByID(id). -
Ah... sorry larsgerrits, I didn't see the addition of code to the OP @OP You need to store it in the ItemStack NBT, like largerrits mentioned, and make sure you only do it once per right click, and do it on the server - NBT should sync automatically for ItemStacks.
-
Caused by: java.lang.ClassNotFoundException: net.minecraft.client.Minecraft Caused by: java.lang.RuntimeException: Attempted to load class atv for invalid side SERVER This is a problem with the mods you are using, not Forge - bring it up with the mod author(s).
-
Roboman, the method is fine in your Item class. larsgerrits, 'hasEffect' is not a class field, it's a class method, otherwise you would be right, at least in the case of wanting to have a unique value for each stack, rather than per item. It sounds like the OP just wants every Item of this type to have the glow.
-
[1.7.10][Solved] Packet not called or not sending correctly?
coolAlias replied to robertcars's topic in Modder Support
You should use SimpleNetworkWrapper. If you insist on the old code, it looks like you didn't register your packet and initialize + post-initialize the pipeline. -
If you looked in Item, you'd see it: "public boolean hasEffect(ItemStack par1ItemStack, int pass)"
-
Have you ever looked at any of the vanilla mob classes? Most of them have an EntityAITasks field named 'tasks', and you will see in the class constructor all of the tasks that get added such as 'tasks.addTask(0, new EntityAISwimming(this));', where the number is the priority of execution (low number is high priority) and the second argument is an instance of the AI task that you want. Spend some time looking through vanilla code and you will find the answers, and if you don't understand it, then spend some more time reading the code and look up as much Java as you need to figure out what's going on.
-
Look at the vanilla AI classes - some of those have path-finding algorithms in them; or look at EntitySquid to see how they are caused to move somewhat randomly, or EntityBat, which also has an interesting path-finding algorithm which may be more suitable for a fish than the squid's motion. Then, either create a custom AI class and add it as a task for your fish (preferable), or add the movement code directly into the update tick like bats and squid (not as preferable, but still works).
-
Good work finding that out, though adding your own discriminators into the map has some huge potential for conflicting with not only other mods that may think of doing the same thing, but also vanilla if they ever decide to use those values for their packets. I would still recommend using SimpleNetworkWrapper and the ByteBufUtils for your packet needs, as the implementation is probably pretty identical in terms of lines of code and simplicity, but ensures compatibility. Anyway, that's still neat that you figured out how to register your own packets to the vanilla system - you could do that with any packet, I would guess, not just sub-classes of vanilla ones.
-
[SOLVED][1.6.4][TileEntity][GUI] Updating Power Bar
coolAlias replied to pokeyletsplays's topic in Modder Support
Look at ContainerFurnace - you need to detect and send changes, update the progress bar, etc., all in your Container class. -
NullPointerException when spawning entity
coolAlias replied to UntouchedWagons's topic in Modder Support
No, it has to do with the entity's spawn packet, which you would get by registering the entity to the game registry using registerModEntity, but why the heck are you trying to spawn a FakePlayer? I don't think that this is what it was designed for... -
entity.getEntityId(), but what kind of info are you trying to get, and why do you need to send a packet from the client to the server? If it's your entity, you should either implement IEntityAdditionalSpawnData to get the extra data to the client when the entity spawns, or use DataWatcher or packets to keep whatever fields you are watching up-to-date on the client.
-
[1.7.10] Issue with a furnace like block
coolAlias replied to The_SlayerMC's topic in Modder Support
Don't return a new instance of the tile entity! You should be sending the instance retrieved from the world: TileEntity te = world.getTileEntity(x, y, z), and check if it's an instanceof your TE class before casting it and passing it to the Container's constructor. -
This tutorial should get you started setting up your workspace to work with the API, but your best bet for actual implementation specifications is to check out the API documentation, assuming you are confident with Java; if not, then your first step should be to learn as much Java as you can.
-
(SOLVED) Get side of block that player is looking at
coolAlias replied to wnx's topic in Modder Support
If you have a MovingObjectPosition object 'mop', mop.sideHit gives you the side in that format. -
private String getOwnerName() { // TODO Auto-generated method stub return null; } private void setOwner(String s) { // TODO Auto-generated method stub } Erm... you really need to read up on some basic Java tutorials. When we say 'override the methods inherited from the IOwnable interface', that code there is not how it's done, not to mention you STILL did not implement any code in there - returning null and an empty method is not usually considered a viable implementation. Anyway, it is a moot point - the two methods are already implemented by EntityTameable, so you shouldn't need to do anything other than extend that class. My advice to you would be to start over and use EntityWolf as an example - you will see all the code you have copied from EntityTameable is not necessary, because you inherit all that functionality already. EntityWolf doesn't bother with the owner at all, as far as those two methods and save/load NBT are concerned.
-
[1.6.4] Why world.isRemote is always true?
coolAlias replied to BlackCrafer666's topic in Modder Support
Looks to me like you made 'shot' both a class field in your Item class and probably 'static' on top of that. Oops on both counts. You should store the number of shots fired in the ItemStack's NBT and your problem with isRemote will probably fix itself. Is that piece of code the only place you use the 'shot' variable? -
Or simply use ByteBufUtils.writeTag(buffer) and ByteBufUtils.readTag(buffer)...