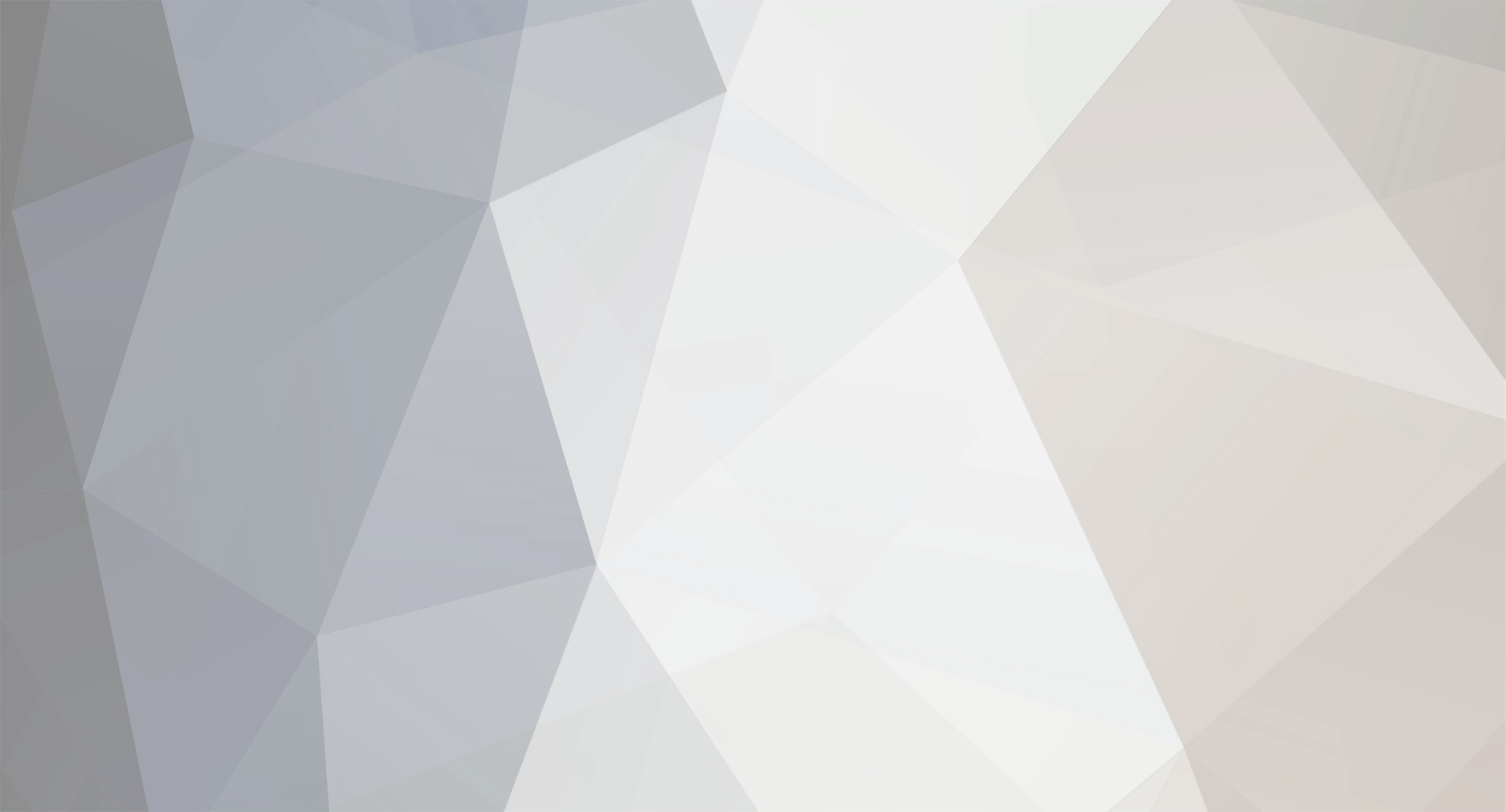
coolAlias
Members-
Posts
2805 -
Joined
-
Last visited
Everything posted by coolAlias
-
onPlayerLogin is on the server already, so you can't send a packet to the server from the server - you are already there! EDIT: Argh, ninja'd by diesieben07!!!
-
[SOLVED-ish][1.7.x]Why is last attacker for EntityCow always null?
coolAlias replied to jabelar's topic in Modder Support
You should be able just to use the EntityLivingBase#entityLivingToAttack field directly, well, through the getAITarget() method anyway (which simply returns that field). This field is set for every EntityLivingBase that doesn't completely override attackEntityFrom (i.e. by not calling 'super.attackEntityFrom'), so it should work even for passive mobs without you having to do any extra work. Should. With Minecraft, sometimes it can be difficult to know until you try -
Use one of the World#playSoundXXX methods and insert a valid sound file name (open Minecraft's 'sounds.json' file to find valid sound names). An example from inside an Entity sub-class that plays the xp orb sound: worldObj.playSoundAtEntity( this, // the entity at which to play the sound "random.orb", // sound file name from sounds.json 1.0F, // volume (randomize if you want) 0.5F * ((rand.nextFloat() - rand.nextFloat()) * 0.7F + 1.8F) // pitch that has lots some randomness );
-
Try using your editor's 'search and replace' functionality, if you aren't already using it - that should speed things up dramatically.
-
[SOLVED-ish][1.7.x]Why is last attacker for EntityCow always null?
coolAlias replied to jabelar's topic in Modder Support
For some reason that field is not used inside of EntityLivingBase, but may be elsewhere through the use of EntityLivingBase#setLastAttacker. However, the revenge target IS set, for whatever reason: Try using that field instead and see if you get better results. -
FOV is affected (slightly) by the player's current speed - if your screen was going crazy, then perhaps consider toning down your speed boost? I haven't looked at the setPlayerWalkingSpeed method, so I'm not sure how much that affects the player speed relative to something like sprinting, but in my own mod, I've created various items that in combination can will boost the player's speed by about as much as though they were sprinting x 3 or 4, and the player can sprint on top of that, which brings the player up to a pretty ridiculous speed yet without any extremely jarring zoom effect. If you go much faster than that, new chunks will have a very difficult time loading quickly enough and you will probably encounter other errors.
-
I mentioned earlier about SharedMonsterAttributes and AttributeModifiers, try using those instead of setPlayerWalkSpeed. Take a look at the sprinting mechanic as an example (specifically, see EntityLivingBase#setSprinting method and the related fields).
-
By SERVER side, you mean when !world.isRemote and not @SideOnly(Side.SERVER), right? The first would be the correct syntax. If that's not your problem, then is it possible the server is running an older version of Forge than your mod requires? What I mean is, perhaps the setWalkingSpeed method is still obfuscated on the server's version. Just a thought. An alternate solution, one that I use, is to apply a speed modifier based on SharedMonsterAttributes. You can do this either as a value you add directly to your armor in getItemAttributeModifiers, which would be applied even while holding the armor in hand, not just when worn, or by applying (and removing) the same attribute modifier via your tick handler to get the 'only when equipped' effect.
-
Barring a better solution: player.stepHeight += amount when you put it on, -= sameAmount when you take it off. EDIT: I forgot to mention that step height is handled only on the client, so be sure you set it on that side and not the server.
-
If you don't need any sort of Gui or other interaction with the player, you could just as well give your entity a List<ItemStack> field and shove whatever items it comes across into that... you might not even need to bother with an IInventory, it really depends on the exact details of how you want your entity to behave and interact with the rest of the Minecraft world.
-
There are two solutions: 1. Use a custom DamageSource in your call to attackEntityFrom and don't call attackEntityFrom from the event when event.source is an instanceof your CustomDamageSource 2. Like mentioned above, use damageEntity directly by creating a class 'DirtyEntityAccessor' and for its package declaration put 'package net.minecraft.entity' - this will give you access to protected methods in the Entity folder of MC, without actually changing any vanilla code, and the file/folder is still in your project, not in MC's folder. It's a neat trick I learned from diesieben07
-
[Solved][1.7.x]Confused myself about renderer registration
coolAlias replied to jabelar's topic in Modder Support
Ah, I hadn't thought about that - I guess it makes sense that DW must have some processes going on in the background to check if the data has changed, otherwise it wouldn't work Good point! -
Another option may be to simply add an InventoryBasic field to your entity, similar to how EntityPlayer has an InventoryPlayer field. Then you don't have to worry about the specifics of the inventory, but simply provide access to it and remember to save/load it from NBT. I'm not aware of any specific downsides to doing it this way, though I haven't done it myself.
-
[Solved][1.7.x]Confused myself about renderer registration
coolAlias replied to jabelar's topic in Modder Support
The additional spawn data is for the spawn entity packet sent to the client automatically by Forge (this is why you need to register all your mod entities, for this specific packet); thus, if you implemented that interface, you wouldn't have to do interact with the packet at all manually. Either way you do it, you still need to set the ItemStack you want before you spawn the entity, and then have a method to retrieve it (or make it a public field, but that's just bad practice ) -
[Solved][1.7.x]Confused myself about renderer registration
coolAlias replied to jabelar's topic in Modder Support
You could do as suggested above, or try something I've used which is to store the ItemStack in the entity's DataWatcher: // data type 5 is ItemStack, as seen in EntityItem's entityInit() dataWatcher.addObjectByDataType(DATAWATCHER_INDEX, 5); // then before you spawn your entity, update the DataWatcher with whatever ItemStack you want I did it that way because at the time I didn't know about IEntityAdditionalSpawnData, and it seems to get the job done just as well. I don't know if there is any advantage to one over the other, but perhaps some others have input in that regard. -
You know how you create the EntityArrow in the first place? EntityArrow arrow = new EntityArrow(world, player, charge); Well, once you've done that and before you spawn it into the world, you can alter the arrow.posX, arrow.posY, arrow.posZ slightly. Create two (or more) arrow entities, leave the first one unchanged, and change the others as you need. You might want to look into the player's lookVector or you will find your arrows look right only in one direction.
-
That sounds pretty thorough; did you make sure you printed out the information on the server side?
-
One reason I can think of is that then you are checking stuff every single time an entity dies, whereas putting the code in the Item class means you only check when that specific item hits an entity. Your solution would, of course, work fine, and I am guilty of over-using events myself, but ideally (imo) we should all use events only as a last resort when standard class methods are unable to provide the results we need.
-
[1.7.2] [SOLVED!] Arrow not rendering correctly while flying
coolAlias replied to Metalbot1's topic in Modder Support
Just to double-check with the OP and jabelar, above, you guys are registering your projectiles as mod entities, correct? Because that sends a lot of update information (position, motion, etc.) to the client if you do it correctly. This is what I use for all of my projectiles: // for arrow-types, I use a tracking frequency of '20', as that's what vanilla arrows use: EntityRegistry.registerModEntity(EntityArrowCustom.class, "arrowcustom", ++modEntityIndex, ZSSMain.instance, 64, 20, true); // all other throwables use a frequency of '10': EntityRegistry.registerModEntity(EntityBomb.class, "bomb", ++modEntityIndex, ZSSMain.instance, 64, 10, true); You don't have to have the exact same frequency, but it certainly seems to do the trick for me. Here is a full chart of vanilla entities and their associated values, put together I think by LockNLoad: https://docs.google.com/spreadsheet/pub?key=0Ap8gssssFFPAdFRXREZGSzZRY3k1WE8wcUE4S09xWXc&single=true&gid=0&output=html -
Whoa there, I'm talking about Minecraft Forge 'Events', not anything in Java; same goes for timing things - 99% of cases you don't want to use an actual Java Timer or sleep or any of that, but find methods that are called regularly (called a 'tick' in Minecraft), such as Entity#onUpdate, Item#onUpdate, etc. If you want to learn more, you should check the many tutorials on the wiki here, The Grey Ghost's excellent blog, or for more on Forge Events / some information about various ticking methods and events, you can see my tutorial on that.
-
[SOLVED][1.7.2] Packet data not received onMessage?
coolAlias replied to coolAlias's topic in Modder Support
Lol, you are totally right! I was thinking 'hey, it's in the same class, so the data will be there' wow. Derpety derp derp Thanks buddy! -
SOLUTION: Use the IMessageHandler#onMessage 'message' parameter to access the field, rather than trying to access the class field directly (it's a separate instance of the class when it processes the IMessageHandler vs the IMessage) - thanks jabelar! Hi again, I've run into an interesting problem - it seems that data stored in a message class is not available in the "onMessage" method. This is with Forge 10.12.2.1121 by the way. Here is the code for my message: And console output from that: To Bytes... writing From Bytes... reading WARNING: onMessage data is NULL!!! As you can see, the data is not null before it is written, nor is it null after it is read, but whatever happens in between being read and processing onMessage, the data disappears! In another message, I send a simple integer, and that one has the same problem. I thought it was working, but it was only because the value I sent was zero (the GUI index to open) - changing it to 10 failed to open a Gui. Any ideas what could be going wrong here? Thanks, coolAlias
-
How can you tell they are ignoring it? Did you isolate a single zombie and print out its attack times, or were there a bunch of zombies attacking you at once? If you dodge the attack (i.e. cancel), do you set the zombie's attack time manually? I haven't looked, but I suspect their attack time might not get set if the attack event is cancelled.
-
[SOLVED] Trying to make IMessageHandler more generic
coolAlias replied to coolAlias's topic in Modder Support
Sure, that can happen if it's a Server-Side class, but this isn't If you take another look, you'll see that the Minecraft.getMinecraft() is embedded inside of a method that only gets called when a message (packet) is being processed; this only ever happens on one side or the other (at a time - it could happen on either depending on how you register it), which is why I check the Side first. It's effectively the same as saying "if (world.isRemote) getClientStuff; else getServerStuff", which I'm sure you've seen happen in other methods, e.g. Item#onRightClick, in which you could either spawn an entity (server) or particles (client), neither of which will crash if executed on the correct side. I've definitely experienced what you are talking about though - it was with things like Render, Gui, KeyBindings and those kinds of classes. Basically, any time you are going to reference something that only exists on the client, you must segregate it somehow or you will run into the issue you described. The main problem is for an entire CLASS, such as a Render class that you stuck @SideOnly(Side.CLIENT) above because it's everywhere else in the code (I do that...), or a client-only field that is initialized in-line (i.e. public IIcon[] icons = new IIcon[3] <- crash), when you go to register that class, there is no segregation of sides done by default, so you must either check yourself (using something like FML's getEffectiveSide if you don't have a world object) or delegate to the Proxy classes (much cleaner and easier). Same idea for something like Minecraft.getMinecraft() - as long as you can segregate the call and ensure it happens on the client side, nothing averse will come of it. Anyway, my AbstractMessage class seems to be working just fine now, so I'm pretty happy with that I still need to test it in a multiplayer scenario, but I suspect it won't have any problems.