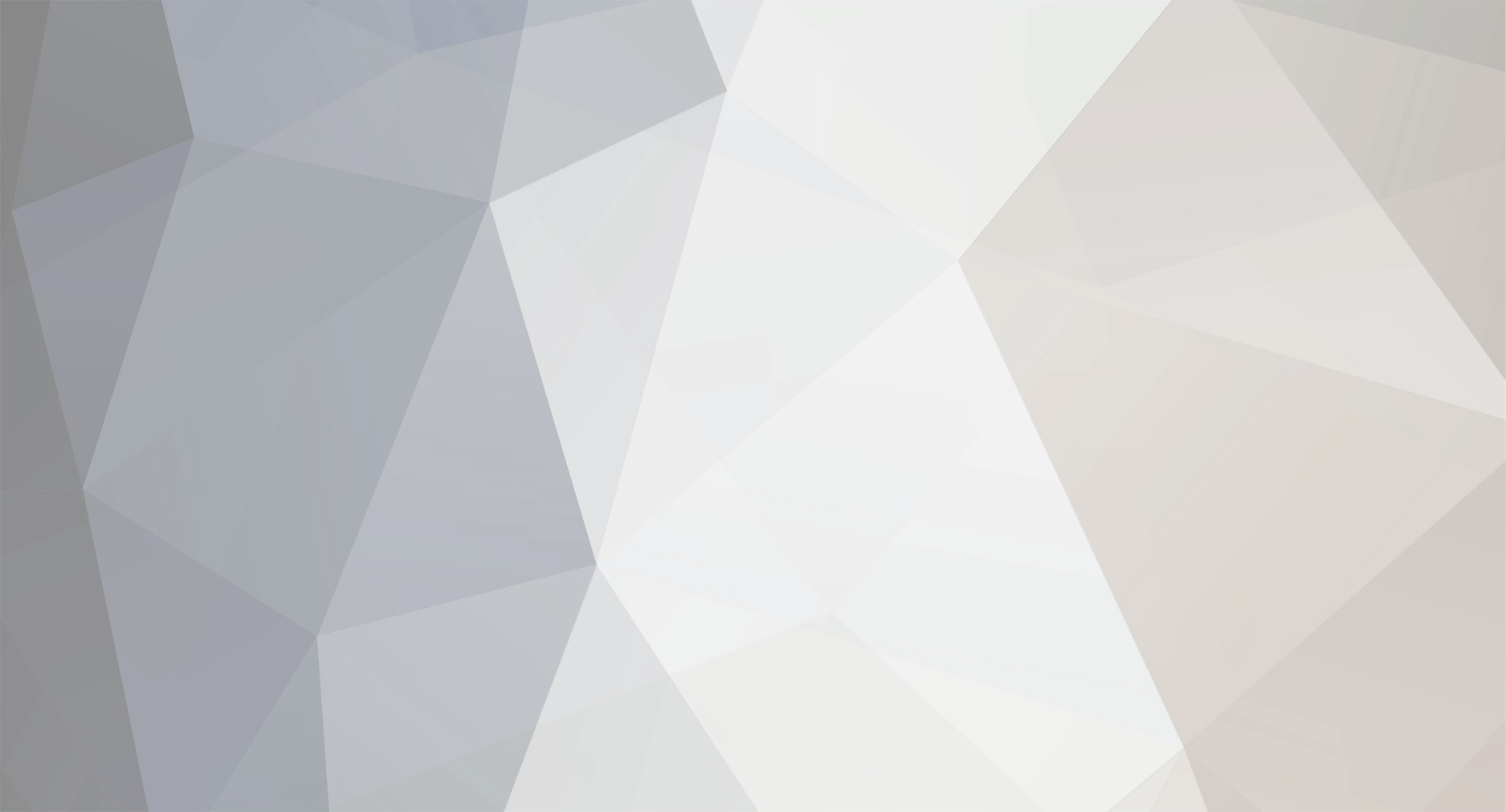
coolAlias
Members-
Posts
2805 -
Joined
-
Last visited
Everything posted by coolAlias
-
Hardly lots - from the tile entity packet, you would only need the x/y/z coordinates; whatever data you want to send wouldn't have been there anyway, and you need nothing at all from the Packet class itself. This is an entire message + handler that could be used to update specific info in a tile entity - all you would have to do is fill in whatever info you want to send, register it to Side.CLIENT, and use the SimpleNetworkWrapper to sendToAllNearby. In the end, I'd wager it will be less code than you are using right now and it won't have the overhead of inheriting from the vanilla TE update packet. If you still consider that superfluous, well that's your call, but it seems pretty straightforward to me.
-
The question is, though, why does it absolutely have to subclass a vanilla packet? Why not write an IMessage on SimpleNetworkWrapper that sends the exact data you want, rather than trying to force a non-vanilla packet through the vanilla system? It seems to me that that would be the simplest and cleanest solution, since your attempts so far have not gone anywhere. The code I gave earlier does work, though, with vanilla packets. Interesting that sub-classed versions don't work.
-
Ah, well without knowing anything about what you're trying to do, that was the best I could come up with. Why don't you create a custom packet then and send it using your mod network rather than trying to use the vanilla network? That way you can send as much or as little information as you want without worrying about illegal stances (probably because the vanilla system does not recognize your subclass).
-
I'm not too sure why you are getting that message, but I must ask, why do you need to send a TileEntity update packet in this way? Whenever a TE block is marked for an update (which you can do manually, if needed), the TileEntity's description packet is sent automatically to all nearby players - you can override getDescriptionPacket to add any extra information you need. // this will force the block to update, and all nearby clients will get the new description packet // any information you send will be available for rendering, GUIs, etc. worldObj.markBlockForUpdate(xCoord, yCoord, zCoord); @Override public Packet getDescriptionPacket() { NBTTagCompound tag = new NBTTagCompound(); this.writeToNBT(tag); return new S35PacketUpdateTileEntity(xCoord, yCoord, zCoord, 1, tag); } @Override public void onDataPacket(NetworkManager net, S35PacketUpdateTileEntity packet) { readFromNBT(packet.func_148857_g()); }
-
I really don't understand what you are trying to do - based on your code, it looks like you are trying to manipulate items in your Gui, in which case you definitely want to use a Container. Otherwise, if you are absolutely certain you do not need a Container, then you should simply be able to return the client Gui element: @Override public Object getClientGuiElement(int id, EntityPlayer player, World world, int x, int y, int z) { TileEntity te = world.getTileEntity(x, y, z); switch(id) { case 0: if (te instanceof YourTileEntity) { return new YourGui((YourTileEntity) te); } } } If your Gui isn't opening, check that your IDs match (likely culprit if you are using '0', '1', etc. instead of defining constants for your IDs).
-
Interesting. Well, I've only used the server->client way of sending vanilla messages with S12PacketEntityVelocity, so perhaps it doesn't like the TE update packet. It would help if you posted your code and crash report.
-
Construction / NBT loading is only done on the server, so anything you want the client to know about, you need to send a packet.
-
public static void onPlayerTick(EntityPlayer player) That's not how events work. Here is a tutorial on how to use events.
-
Posting your code would be the logical first step.
-
// Client->Server ((EntityClientPlayerMP) player).sendQueue.addToSendQueue(new S35PacketUpdateTileEntity(params)); // Server->Client ((EntityPlayerMP) player).playerNetServerHandler.sendPacket(new S35PacketUpdateTileEntity(params)); You could also consider sending the packet returned from the TileEntity's getDescriptionPacket method.
-
Be aware, however, that DamageSource#getEntity will return, for example, the player responsible for shooting the arrow entity that damaged the target - this may be what you want, it may not (usually it is). DamageSource#getSourceOfDamage() returns the actual entity directly responsible for the damage, which in our example above would be the EntityArrow that hit the target. As jabelar mentioned, there is EntityDamageSource, for which both of the above methods return the same thing, and there is EntityDamageSourceIndirect (for things such as projectiles), for which the two methods return different entities as explained above.
-
Or you could use what I mentioned earlier, though I forgot to mention you need to subtract 1 to get the block underneath: int j = MathHelper.floor_double(player.boundingBox.minY); That way you don't have to worry about any offset, as it's already at your feet regardless of the entity's size.
-
So what's the problem? Every entity has a world object, and the player is an entity.
-
You left setOwner and getOwnerName as empty methods.
-
Even if you could instantiate it, it would be wrong to do so - how did you plan on initializing that object to contain all the correct information? Anyway, at least you did some research. Whenever you are in need of something, chances are you can find it in the parameters passed to whatever method you are in (you are writing this code inside of some class method somewhere, right? like onItemUpdate or something, right???).
-
[SOLVED] [1.7.10] PlayerInteractEvent - Strange behaviour
coolAlias replied to Nicnl's topic in Modder Support
Sometimes, that's just too slow, especially when dealing with things like mouse-clicks. Client clicks mouse -> sends packet to server -> fires interact event -> processes -> sends packet back to client... it's too late, as the server already did its work (barring some convoluted coding). I agree - RIGHT_CLICK_BLOCK is fired on both sides, so why not LEFT_CLICK_BLOCK? It may just be a poor choice of event placement, sort of like the one diesieben07 submitted a PR for last time, or it may be a deeper issue... I haven't looked into it other than noting its behavior. EDIT: Check it out, a PR for this issue: https://github.com/MinecraftForge/MinecraftForge/pull/1121 -
Just Google 'cannot make a static reference' and you'll learn all about it. And no, you are NOT going to be re-writing the class methods of World. You need an instance of World - Google 'instance of class' or 'class object' or go through any basic Java tutorial and you'll learn what that is. Please.
-
Sure, but that is exactly the kind of thing people mean when they say 'Learn Basic Java' - that error message is basic Java, as in you cannot do "World.method()" because the method is not a static method, so you need an instance of World to access it. A little time on Google or Oracle's Java tutorials page goes a long way.
-
<sigh> Your last few comments sorely contest the bold statement in your signature... int i = MathHelper.floor_double(player.posX); int j = MathHelper.floor_double(player.boundingBox.minY); int k = MathHelper.floor_double(player.posZ); Material m = world.getBlock(i, j, k).getMaterial(); boolean flag = (m == Material.water); // or m.isLiquid() or whatever you want.
-
Simply don't have a Container and return null for the server element. All you need is a GuiScreen, and if you want / need to interact with the TileEntity somehow, then you can send a packet (to the server, of course) to its coordinates with whatever action was performed.
-
Why not put it in whatever Item class that has the enchantment? Or would the enchantment be on many different kinds of items? In the latter case, I'd put it in the PlayerTickEvent. I've done something similar in the past, and I found that subtracting the previous motionY before equalizing it gave the best results, something like: flag = is block directly under player a water block? if (flag && player.motionY < 0.0D) { player.posY += -player.motionY; // player is falling, so motionY is negative, but we want to reverse that player.motionY = 0.0D; // no longer falling player.fallDistance = 0.0F; // otherwise I believe it adds up, which may surprise you when you come down }
-
Best way would be to not extend EntityVillager, but implement all the trading stuff yourself; otherwise, I suppose you could override updateAITick and getRecipes, both of which end up calling the private method addDefaultEquipmentAndRecipies which is where the villager's trades are determined. Another option would be to utilize VillagerRegistry.manageVillagerTrades to manage trades for a custom villager type (i.e. not any of the vanilla profession IDs) - you could totally clear the passed in List and add whatever you wanted.
-
[Solved]getEntityData() versus IExtendedEntityProperties
coolAlias replied to jabelar's topic in Modder Support
No, I'm not. The data IS written and read, but it doesn't tell you when it does so meaning you cannot have one central method to write everything you want to save, so you have to make sure you write the data to NBT every single time you change it, or it will be lost. You could, of course, hook into EntityJoinWorldEvent to load your data, and I guess you might be able to do something for logging off, but IEEP has all that built in for you. -
EDIT: Yep, turns out I'm a noob Problem was in my client proxy: @Override public EntityPlayer getPlayerEntity(MessageContext ctx) { return Minecraft.getMinecraft().thePlayer; } Looks fine, but the client proxy method apparently ALWAYS gets called, even when the message is sent to the server (I was getting FALSE for ctx.side.isClient() but TRUE for player.worldObj.isRemote) , meaning I was ending up with a CLIENT player even though I was handling the message on the server... wtf. Anyway, a quick modification to my method seems to have done the trick: @Override public EntityPlayer getPlayerEntity(MessageContext ctx) { // super method in CommonProxy simply returns ctx.getServerHandler().playerEntity return (ctx.side.isClient() ? Minecraft.getMinecraft().thePlayer : super.getPlayerEntity(ctx)); } Still, it seems to be a lot of unnecessary fooling around compared to the previous system, but that's just my opinion. // END EDIT Yes, I am still not at all satisfied with SNW. Perhaps I just haven't figured out how to use it properly, but even without all my shenanigans and using it in the most basic way, I end up with packets that just don't work as they should, most notably with packets that need to be sent both directions, but also some that just don't work as they should. For example: @Override public IMessage handleServerMessage(EntityPlayer player, PlaySoundPacket message, MessageContext ctx) { player.worldObj.playSoundEffect(message.x, message.y, message.z, message.sound, message.volume, message.pitch); return null; } That code worked perfectly fine for playing a sound (yes, on the server) with the old PacketPipeline, but now it does absolutely nothing. It is received fine, everything looks like it should work according to my diagnostics, but no sound plays. It's not the Minecraft / Forge version, either, as using the old system the sound plays just as expected. Anyway, perhaps you will have better luck than me and I can follow your (pending) tutorial
-
[SOLVED] [1.7.10] PlayerInteractEvent - Strange behaviour
coolAlias replied to Nicnl's topic in Modder Support
LEFT_CLICK_BLOCK only fires on the server, not the client. You could try using MouseEvent to intercept client side mouse clicks and determine if the player is about to start mining a block.